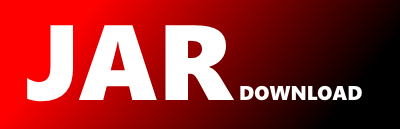
software.amazon.awssdk.services.appstream.model.Session Maven / Gradle / Ivy
Show all versions of appstream Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.appstream.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a streaming session.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Session implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Session::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField USER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Session::userId)).setter(setter(Builder::userId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserId").build()).build();
private static final SdkField STACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Session::stackName)).setter(setter(Builder::stackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackName").build()).build();
private static final SdkField FLEET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Session::fleetName)).setter(setter(Builder::fleetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FleetName").build()).build();
private static final SdkField STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Session::stateAsString)).setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State").build()).build();
private static final SdkField CONNECTION_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Session::connectionStateAsString)).setter(setter(Builder::connectionState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConnectionState").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(Session::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTime").build()).build();
private static final SdkField MAX_EXPIRATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(Session::maxExpirationTime)).setter(setter(Builder::maxExpirationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxExpirationTime").build()).build();
private static final SdkField AUTHENTICATION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Session::authenticationTypeAsString)).setter(setter(Builder::authenticationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthenticationType").build())
.build();
private static final SdkField NETWORK_ACCESS_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(Session::networkAccessConfiguration))
.setter(setter(Builder::networkAccessConfiguration))
.constructor(NetworkAccessConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkAccessConfiguration").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, USER_ID_FIELD,
STACK_NAME_FIELD, FLEET_NAME_FIELD, STATE_FIELD, CONNECTION_STATE_FIELD, START_TIME_FIELD, MAX_EXPIRATION_TIME_FIELD,
AUTHENTICATION_TYPE_FIELD, NETWORK_ACCESS_CONFIGURATION_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String userId;
private final String stackName;
private final String fleetName;
private final String state;
private final String connectionState;
private final Instant startTime;
private final Instant maxExpirationTime;
private final String authenticationType;
private final NetworkAccessConfiguration networkAccessConfiguration;
private Session(BuilderImpl builder) {
this.id = builder.id;
this.userId = builder.userId;
this.stackName = builder.stackName;
this.fleetName = builder.fleetName;
this.state = builder.state;
this.connectionState = builder.connectionState;
this.startTime = builder.startTime;
this.maxExpirationTime = builder.maxExpirationTime;
this.authenticationType = builder.authenticationType;
this.networkAccessConfiguration = builder.networkAccessConfiguration;
}
/**
*
* The identifier of the streaming session.
*
*
* @return The identifier of the streaming session.
*/
public String id() {
return id;
}
/**
*
* The identifier of the user for whom the session was created.
*
*
* @return The identifier of the user for whom the session was created.
*/
public String userId() {
return userId;
}
/**
*
* The name of the stack for the streaming session.
*
*
* @return The name of the stack for the streaming session.
*/
public String stackName() {
return stackName;
}
/**
*
* The name of the fleet for the streaming session.
*
*
* @return The name of the fleet for the streaming session.
*/
public String fleetName() {
return fleetName;
}
/**
*
* The current state of the streaming session.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link SessionState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The current state of the streaming session.
* @see SessionState
*/
public SessionState state() {
return SessionState.fromValue(state);
}
/**
*
* The current state of the streaming session.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link SessionState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The current state of the streaming session.
* @see SessionState
*/
public String stateAsString() {
return state;
}
/**
*
* Specifies whether a user is connected to the streaming session.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #connectionState}
* will return {@link SessionConnectionState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #connectionStateAsString}.
*
*
* @return Specifies whether a user is connected to the streaming session.
* @see SessionConnectionState
*/
public SessionConnectionState connectionState() {
return SessionConnectionState.fromValue(connectionState);
}
/**
*
* Specifies whether a user is connected to the streaming session.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #connectionState}
* will return {@link SessionConnectionState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #connectionStateAsString}.
*
*
* @return Specifies whether a user is connected to the streaming session.
* @see SessionConnectionState
*/
public String connectionStateAsString() {
return connectionState;
}
/**
*
* The time when a streaming instance is dedicated for the user.
*
*
* @return The time when a streaming instance is dedicated for the user.
*/
public Instant startTime() {
return startTime;
}
/**
*
* The time when the streaming session is set to expire. This time is based on the
* MaxUserDurationinSeconds
value, which determines the maximum length of time that a streaming session
* can run. A streaming session might end earlier than the time specified in SessionMaxExpirationTime
,
* when the DisconnectTimeOutInSeconds
elapses or the user chooses to end his or her session. If the
* DisconnectTimeOutInSeconds
elapses, or the user chooses to end his or her session, the streaming
* instance is terminated and the streaming session ends.
*
*
* @return The time when the streaming session is set to expire. This time is based on the
* MaxUserDurationinSeconds
value, which determines the maximum length of time that a streaming
* session can run. A streaming session might end earlier than the time specified in
* SessionMaxExpirationTime
, when the DisconnectTimeOutInSeconds
elapses or the
* user chooses to end his or her session. If the DisconnectTimeOutInSeconds
elapses, or the
* user chooses to end his or her session, the streaming instance is terminated and the streaming session
* ends.
*/
public Instant maxExpirationTime() {
return maxExpirationTime;
}
/**
*
* The authentication method. The user is authenticated using a streaming URL (API
) or SAML 2.0
* federation (SAML
).
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #authenticationType} will return {@link AuthenticationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #authenticationTypeAsString}.
*
*
* @return The authentication method. The user is authenticated using a streaming URL (API
) or SAML 2.0
* federation (SAML
).
* @see AuthenticationType
*/
public AuthenticationType authenticationType() {
return AuthenticationType.fromValue(authenticationType);
}
/**
*
* The authentication method. The user is authenticated using a streaming URL (API
) or SAML 2.0
* federation (SAML
).
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #authenticationType} will return {@link AuthenticationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #authenticationTypeAsString}.
*
*
* @return The authentication method. The user is authenticated using a streaming URL (API
) or SAML 2.0
* federation (SAML
).
* @see AuthenticationType
*/
public String authenticationTypeAsString() {
return authenticationType;
}
/**
*
* The network details for the streaming session.
*
*
* @return The network details for the streaming session.
*/
public NetworkAccessConfiguration networkAccessConfiguration() {
return networkAccessConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(userId());
hashCode = 31 * hashCode + Objects.hashCode(stackName());
hashCode = 31 * hashCode + Objects.hashCode(fleetName());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(connectionStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(maxExpirationTime());
hashCode = 31 * hashCode + Objects.hashCode(authenticationTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(networkAccessConfiguration());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Session)) {
return false;
}
Session other = (Session) obj;
return Objects.equals(id(), other.id()) && Objects.equals(userId(), other.userId())
&& Objects.equals(stackName(), other.stackName()) && Objects.equals(fleetName(), other.fleetName())
&& Objects.equals(stateAsString(), other.stateAsString())
&& Objects.equals(connectionStateAsString(), other.connectionStateAsString())
&& Objects.equals(startTime(), other.startTime())
&& Objects.equals(maxExpirationTime(), other.maxExpirationTime())
&& Objects.equals(authenticationTypeAsString(), other.authenticationTypeAsString())
&& Objects.equals(networkAccessConfiguration(), other.networkAccessConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("Session").add("Id", id()).add("UserId", userId()).add("StackName", stackName())
.add("FleetName", fleetName()).add("State", stateAsString()).add("ConnectionState", connectionStateAsString())
.add("StartTime", startTime()).add("MaxExpirationTime", maxExpirationTime())
.add("AuthenticationType", authenticationTypeAsString())
.add("NetworkAccessConfiguration", networkAccessConfiguration()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "UserId":
return Optional.ofNullable(clazz.cast(userId()));
case "StackName":
return Optional.ofNullable(clazz.cast(stackName()));
case "FleetName":
return Optional.ofNullable(clazz.cast(fleetName()));
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "ConnectionState":
return Optional.ofNullable(clazz.cast(connectionStateAsString()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "MaxExpirationTime":
return Optional.ofNullable(clazz.cast(maxExpirationTime()));
case "AuthenticationType":
return Optional.ofNullable(clazz.cast(authenticationTypeAsString()));
case "NetworkAccessConfiguration":
return Optional.ofNullable(clazz.cast(networkAccessConfiguration()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function