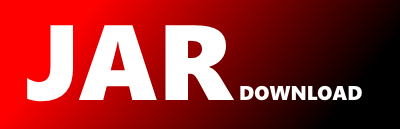
software.amazon.awssdk.services.appstream.AppStreamAsyncClient Maven / Gradle / Ivy
Show all versions of appstream Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.appstream;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.appstream.model.AssociateFleetRequest;
import software.amazon.awssdk.services.appstream.model.AssociateFleetResponse;
import software.amazon.awssdk.services.appstream.model.BatchAssociateUserStackRequest;
import software.amazon.awssdk.services.appstream.model.BatchAssociateUserStackResponse;
import software.amazon.awssdk.services.appstream.model.BatchDisassociateUserStackRequest;
import software.amazon.awssdk.services.appstream.model.BatchDisassociateUserStackResponse;
import software.amazon.awssdk.services.appstream.model.CopyImageRequest;
import software.amazon.awssdk.services.appstream.model.CopyImageResponse;
import software.amazon.awssdk.services.appstream.model.CreateDirectoryConfigRequest;
import software.amazon.awssdk.services.appstream.model.CreateDirectoryConfigResponse;
import software.amazon.awssdk.services.appstream.model.CreateFleetRequest;
import software.amazon.awssdk.services.appstream.model.CreateFleetResponse;
import software.amazon.awssdk.services.appstream.model.CreateImageBuilderRequest;
import software.amazon.awssdk.services.appstream.model.CreateImageBuilderResponse;
import software.amazon.awssdk.services.appstream.model.CreateImageBuilderStreamingUrlRequest;
import software.amazon.awssdk.services.appstream.model.CreateImageBuilderStreamingUrlResponse;
import software.amazon.awssdk.services.appstream.model.CreateStackRequest;
import software.amazon.awssdk.services.appstream.model.CreateStackResponse;
import software.amazon.awssdk.services.appstream.model.CreateStreamingUrlRequest;
import software.amazon.awssdk.services.appstream.model.CreateStreamingUrlResponse;
import software.amazon.awssdk.services.appstream.model.CreateUsageReportSubscriptionRequest;
import software.amazon.awssdk.services.appstream.model.CreateUsageReportSubscriptionResponse;
import software.amazon.awssdk.services.appstream.model.CreateUserRequest;
import software.amazon.awssdk.services.appstream.model.CreateUserResponse;
import software.amazon.awssdk.services.appstream.model.DeleteDirectoryConfigRequest;
import software.amazon.awssdk.services.appstream.model.DeleteDirectoryConfigResponse;
import software.amazon.awssdk.services.appstream.model.DeleteFleetRequest;
import software.amazon.awssdk.services.appstream.model.DeleteFleetResponse;
import software.amazon.awssdk.services.appstream.model.DeleteImageBuilderRequest;
import software.amazon.awssdk.services.appstream.model.DeleteImageBuilderResponse;
import software.amazon.awssdk.services.appstream.model.DeleteImagePermissionsRequest;
import software.amazon.awssdk.services.appstream.model.DeleteImagePermissionsResponse;
import software.amazon.awssdk.services.appstream.model.DeleteImageRequest;
import software.amazon.awssdk.services.appstream.model.DeleteImageResponse;
import software.amazon.awssdk.services.appstream.model.DeleteStackRequest;
import software.amazon.awssdk.services.appstream.model.DeleteStackResponse;
import software.amazon.awssdk.services.appstream.model.DeleteUsageReportSubscriptionRequest;
import software.amazon.awssdk.services.appstream.model.DeleteUsageReportSubscriptionResponse;
import software.amazon.awssdk.services.appstream.model.DeleteUserRequest;
import software.amazon.awssdk.services.appstream.model.DeleteUserResponse;
import software.amazon.awssdk.services.appstream.model.DescribeDirectoryConfigsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeDirectoryConfigsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeFleetsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeFleetsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeImageBuildersRequest;
import software.amazon.awssdk.services.appstream.model.DescribeImageBuildersResponse;
import software.amazon.awssdk.services.appstream.model.DescribeImagePermissionsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeImagePermissionsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeImagesRequest;
import software.amazon.awssdk.services.appstream.model.DescribeImagesResponse;
import software.amazon.awssdk.services.appstream.model.DescribeSessionsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeSessionsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeStacksRequest;
import software.amazon.awssdk.services.appstream.model.DescribeStacksResponse;
import software.amazon.awssdk.services.appstream.model.DescribeUsageReportSubscriptionsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeUsageReportSubscriptionsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeUserStackAssociationsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeUserStackAssociationsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeUsersRequest;
import software.amazon.awssdk.services.appstream.model.DescribeUsersResponse;
import software.amazon.awssdk.services.appstream.model.DisableUserRequest;
import software.amazon.awssdk.services.appstream.model.DisableUserResponse;
import software.amazon.awssdk.services.appstream.model.DisassociateFleetRequest;
import software.amazon.awssdk.services.appstream.model.DisassociateFleetResponse;
import software.amazon.awssdk.services.appstream.model.EnableUserRequest;
import software.amazon.awssdk.services.appstream.model.EnableUserResponse;
import software.amazon.awssdk.services.appstream.model.ExpireSessionRequest;
import software.amazon.awssdk.services.appstream.model.ExpireSessionResponse;
import software.amazon.awssdk.services.appstream.model.ListAssociatedFleetsRequest;
import software.amazon.awssdk.services.appstream.model.ListAssociatedFleetsResponse;
import software.amazon.awssdk.services.appstream.model.ListAssociatedStacksRequest;
import software.amazon.awssdk.services.appstream.model.ListAssociatedStacksResponse;
import software.amazon.awssdk.services.appstream.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.appstream.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.appstream.model.StartFleetRequest;
import software.amazon.awssdk.services.appstream.model.StartFleetResponse;
import software.amazon.awssdk.services.appstream.model.StartImageBuilderRequest;
import software.amazon.awssdk.services.appstream.model.StartImageBuilderResponse;
import software.amazon.awssdk.services.appstream.model.StopFleetRequest;
import software.amazon.awssdk.services.appstream.model.StopFleetResponse;
import software.amazon.awssdk.services.appstream.model.StopImageBuilderRequest;
import software.amazon.awssdk.services.appstream.model.StopImageBuilderResponse;
import software.amazon.awssdk.services.appstream.model.TagResourceRequest;
import software.amazon.awssdk.services.appstream.model.TagResourceResponse;
import software.amazon.awssdk.services.appstream.model.UntagResourceRequest;
import software.amazon.awssdk.services.appstream.model.UntagResourceResponse;
import software.amazon.awssdk.services.appstream.model.UpdateDirectoryConfigRequest;
import software.amazon.awssdk.services.appstream.model.UpdateDirectoryConfigResponse;
import software.amazon.awssdk.services.appstream.model.UpdateFleetRequest;
import software.amazon.awssdk.services.appstream.model.UpdateFleetResponse;
import software.amazon.awssdk.services.appstream.model.UpdateImagePermissionsRequest;
import software.amazon.awssdk.services.appstream.model.UpdateImagePermissionsResponse;
import software.amazon.awssdk.services.appstream.model.UpdateStackRequest;
import software.amazon.awssdk.services.appstream.model.UpdateStackResponse;
import software.amazon.awssdk.services.appstream.paginators.DescribeImagePermissionsPublisher;
import software.amazon.awssdk.services.appstream.paginators.DescribeImagesPublisher;
import software.amazon.awssdk.services.appstream.waiters.AppStreamAsyncWaiter;
/**
* Service client for accessing Amazon AppStream asynchronously. This can be created using the static {@link #builder()}
* method.
*
* Amazon AppStream 2.0
*
* This is the Amazon AppStream 2.0 API Reference. This documentation provides descriptions and syntax for each
* of the actions and data types in AppStream 2.0. AppStream 2.0 is a fully managed, secure application streaming
* service that lets you stream desktop applications to users without rewriting applications. AppStream 2.0 manages the
* AWS resources that are required to host and run your applications, scales automatically, and provides access to your
* users on demand.
*
*
*
* You can call the AppStream 2.0 API operations by using an interface VPC endpoint (interface endpoint). For more
* information, see Access AppStream 2.0 API Operations and CLI Commands Through an Interface VPC Endpoint in the Amazon
* AppStream 2.0 Administration Guide.
*
*
*
* To learn more about AppStream 2.0, see the following resources:
*
*
* -
*
*
* -
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface AppStreamAsyncClient extends SdkClient {
String SERVICE_NAME = "appstream";
/**
* Create a {@link AppStreamAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static AppStreamAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link AppStreamAsyncClient}.
*/
static AppStreamAsyncClientBuilder builder() {
return new DefaultAppStreamAsyncClientBuilder();
}
/**
*
* Associates the specified fleet with the specified stack.
*
*
* @param associateFleetRequest
* @return A Java Future containing the result of the AssociateFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - IncompatibleImageException The image does not support storage connectors.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.AssociateFleet
* @see AWS API
* Documentation
*/
default CompletableFuture associateFleet(AssociateFleetRequest associateFleetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates the specified fleet with the specified stack.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateFleetRequest.Builder} avoiding the need to
* create one manually via {@link AssociateFleetRequest#builder()}
*
*
* @param associateFleetRequest
* A {@link Consumer} that will call methods on {@link AssociateFleetRequest.Builder} to create a request.
* @return A Java Future containing the result of the AssociateFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - IncompatibleImageException The image does not support storage connectors.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.AssociateFleet
* @see AWS API
* Documentation
*/
default CompletableFuture associateFleet(Consumer associateFleetRequest) {
return associateFleet(AssociateFleetRequest.builder().applyMutation(associateFleetRequest).build());
}
/**
*
* Associates the specified users with the specified stacks. Users in a user pool cannot be assigned to stacks with
* fleets that are joined to an Active Directory domain.
*
*
* @param batchAssociateUserStackRequest
* @return A Java Future containing the result of the BatchAssociateUserStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationNotPermittedException The attempted operation is not permitted.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.BatchAssociateUserStack
* @see AWS API Documentation
*/
default CompletableFuture batchAssociateUserStack(
BatchAssociateUserStackRequest batchAssociateUserStackRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates the specified users with the specified stacks. Users in a user pool cannot be assigned to stacks with
* fleets that are joined to an Active Directory domain.
*
*
*
* This is a convenience which creates an instance of the {@link BatchAssociateUserStackRequest.Builder} avoiding
* the need to create one manually via {@link BatchAssociateUserStackRequest#builder()}
*
*
* @param batchAssociateUserStackRequest
* A {@link Consumer} that will call methods on {@link BatchAssociateUserStackRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the BatchAssociateUserStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationNotPermittedException The attempted operation is not permitted.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.BatchAssociateUserStack
* @see AWS API Documentation
*/
default CompletableFuture batchAssociateUserStack(
Consumer batchAssociateUserStackRequest) {
return batchAssociateUserStack(BatchAssociateUserStackRequest.builder().applyMutation(batchAssociateUserStackRequest)
.build());
}
/**
*
* Disassociates the specified users from the specified stacks.
*
*
* @param batchDisassociateUserStackRequest
* @return A Java Future containing the result of the BatchDisassociateUserStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationNotPermittedException The attempted operation is not permitted.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.BatchDisassociateUserStack
* @see AWS API Documentation
*/
default CompletableFuture batchDisassociateUserStack(
BatchDisassociateUserStackRequest batchDisassociateUserStackRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates the specified users from the specified stacks.
*
*
*
* This is a convenience which creates an instance of the {@link BatchDisassociateUserStackRequest.Builder} avoiding
* the need to create one manually via {@link BatchDisassociateUserStackRequest#builder()}
*
*
* @param batchDisassociateUserStackRequest
* A {@link Consumer} that will call methods on {@link BatchDisassociateUserStackRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the BatchDisassociateUserStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationNotPermittedException The attempted operation is not permitted.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.BatchDisassociateUserStack
* @see AWS API Documentation
*/
default CompletableFuture batchDisassociateUserStack(
Consumer batchDisassociateUserStackRequest) {
return batchDisassociateUserStack(BatchDisassociateUserStackRequest.builder()
.applyMutation(batchDisassociateUserStackRequest).build());
}
/**
*
* Copies the image within the same region or to a new region within the same AWS account. Note that any tags you
* added to the image will not be copied.
*
*
* @param copyImageRequest
* @return A Java Future containing the result of the CopyImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The specified resource already exists.
* - ResourceNotFoundException The specified resource was not found.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - IncompatibleImageException The image does not support storage connectors.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CopyImage
* @see AWS API
* Documentation
*/
default CompletableFuture copyImage(CopyImageRequest copyImageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Copies the image within the same region or to a new region within the same AWS account. Note that any tags you
* added to the image will not be copied.
*
*
*
* This is a convenience which creates an instance of the {@link CopyImageRequest.Builder} avoiding the need to
* create one manually via {@link CopyImageRequest#builder()}
*
*
* @param copyImageRequest
* A {@link Consumer} that will call methods on {@link CopyImageRequest.Builder} to create a request.
* @return A Java Future containing the result of the CopyImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The specified resource already exists.
* - ResourceNotFoundException The specified resource was not found.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - IncompatibleImageException The image does not support storage connectors.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CopyImage
* @see AWS API
* Documentation
*/
default CompletableFuture copyImage(Consumer copyImageRequest) {
return copyImage(CopyImageRequest.builder().applyMutation(copyImageRequest).build());
}
/**
*
* Creates a Directory Config object in AppStream 2.0. This object includes the configuration information required
* to join fleets and image builders to Microsoft Active Directory domains.
*
*
* @param createDirectoryConfigRequest
* @return A Java Future containing the result of the CreateDirectoryConfig operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceAlreadyExistsException The specified resource already exists.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - OperationNotPermittedException The attempted operation is not permitted.
* - InvalidRoleException The specified role is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateDirectoryConfig
* @see AWS API Documentation
*/
default CompletableFuture createDirectoryConfig(
CreateDirectoryConfigRequest createDirectoryConfigRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a Directory Config object in AppStream 2.0. This object includes the configuration information required
* to join fleets and image builders to Microsoft Active Directory domains.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDirectoryConfigRequest.Builder} avoiding the
* need to create one manually via {@link CreateDirectoryConfigRequest#builder()}
*
*
* @param createDirectoryConfigRequest
* A {@link Consumer} that will call methods on {@link CreateDirectoryConfigRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateDirectoryConfig operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceAlreadyExistsException The specified resource already exists.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - OperationNotPermittedException The attempted operation is not permitted.
* - InvalidRoleException The specified role is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateDirectoryConfig
* @see AWS API Documentation
*/
default CompletableFuture createDirectoryConfig(
Consumer createDirectoryConfigRequest) {
return createDirectoryConfig(CreateDirectoryConfigRequest.builder().applyMutation(createDirectoryConfigRequest).build());
}
/**
*
* Creates a fleet. A fleet consists of streaming instances that run a specified image.
*
*
* @param createFleetRequest
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The specified resource already exists.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - RequestLimitExceededException AppStream 2.0 can’t process the request right now because the Describe
* calls from your AWS account are being throttled by Amazon EC2. Try again later.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - InvalidRoleException The specified role is invalid.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - IncompatibleImageException The image does not support storage connectors.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateFleet
* @see AWS API
* Documentation
*/
default CompletableFuture createFleet(CreateFleetRequest createFleetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a fleet. A fleet consists of streaming instances that run a specified image.
*
*
*
* This is a convenience which creates an instance of the {@link CreateFleetRequest.Builder} avoiding the need to
* create one manually via {@link CreateFleetRequest#builder()}
*
*
* @param createFleetRequest
* A {@link Consumer} that will call methods on {@link CreateFleetRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The specified resource already exists.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - RequestLimitExceededException AppStream 2.0 can’t process the request right now because the Describe
* calls from your AWS account are being throttled by Amazon EC2. Try again later.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - InvalidRoleException The specified role is invalid.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - IncompatibleImageException The image does not support storage connectors.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateFleet
* @see AWS API
* Documentation
*/
default CompletableFuture createFleet(Consumer createFleetRequest) {
return createFleet(CreateFleetRequest.builder().applyMutation(createFleetRequest).build());
}
/**
*
* Creates an image builder. An image builder is a virtual machine that is used to create an image.
*
*
* The initial state of the builder is PENDING
. When it is ready, the state is RUNNING
.
*
*
* @param createImageBuilderRequest
* @return A Java Future containing the result of the CreateImageBuilder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - RequestLimitExceededException AppStream 2.0 can’t process the request right now because the Describe
* calls from your AWS account are being throttled by Amazon EC2. Try again later.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - ResourceAlreadyExistsException The specified resource already exists.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - ResourceNotFoundException The specified resource was not found.
* - InvalidRoleException The specified role is invalid.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - IncompatibleImageException The image does not support storage connectors.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateImageBuilder
* @see AWS
* API Documentation
*/
default CompletableFuture createImageBuilder(CreateImageBuilderRequest createImageBuilderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an image builder. An image builder is a virtual machine that is used to create an image.
*
*
* The initial state of the builder is PENDING
. When it is ready, the state is RUNNING
.
*
*
*
* This is a convenience which creates an instance of the {@link CreateImageBuilderRequest.Builder} avoiding the
* need to create one manually via {@link CreateImageBuilderRequest#builder()}
*
*
* @param createImageBuilderRequest
* A {@link Consumer} that will call methods on {@link CreateImageBuilderRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateImageBuilder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - RequestLimitExceededException AppStream 2.0 can’t process the request right now because the Describe
* calls from your AWS account are being throttled by Amazon EC2. Try again later.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - ResourceAlreadyExistsException The specified resource already exists.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - ResourceNotFoundException The specified resource was not found.
* - InvalidRoleException The specified role is invalid.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - IncompatibleImageException The image does not support storage connectors.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateImageBuilder
* @see AWS
* API Documentation
*/
default CompletableFuture createImageBuilder(
Consumer createImageBuilderRequest) {
return createImageBuilder(CreateImageBuilderRequest.builder().applyMutation(createImageBuilderRequest).build());
}
/**
*
* Creates a URL to start an image builder streaming session.
*
*
* @param createImageBuilderStreamingUrlRequest
* @return A Java Future containing the result of the CreateImageBuilderStreamingURL operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationNotPermittedException The attempted operation is not permitted.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateImageBuilderStreamingURL
* @see AWS API Documentation
*/
default CompletableFuture createImageBuilderStreamingURL(
CreateImageBuilderStreamingUrlRequest createImageBuilderStreamingUrlRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a URL to start an image builder streaming session.
*
*
*
* This is a convenience which creates an instance of the {@link CreateImageBuilderStreamingUrlRequest.Builder}
* avoiding the need to create one manually via {@link CreateImageBuilderStreamingUrlRequest#builder()}
*
*
* @param createImageBuilderStreamingUrlRequest
* A {@link Consumer} that will call methods on {@link CreateImageBuilderStreamingURLRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateImageBuilderStreamingURL operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationNotPermittedException The attempted operation is not permitted.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateImageBuilderStreamingURL
* @see AWS API Documentation
*/
default CompletableFuture createImageBuilderStreamingURL(
Consumer createImageBuilderStreamingUrlRequest) {
return createImageBuilderStreamingURL(CreateImageBuilderStreamingUrlRequest.builder()
.applyMutation(createImageBuilderStreamingUrlRequest).build());
}
/**
*
* Creates a stack to start streaming applications to users. A stack consists of an associated fleet, user access
* policies, and storage configurations.
*
*
* @param createStackRequest
* @return A Java Future containing the result of the CreateStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - ResourceAlreadyExistsException The specified resource already exists.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - InvalidRoleException The specified role is invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateStack
* @see AWS API
* Documentation
*/
default CompletableFuture createStack(CreateStackRequest createStackRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a stack to start streaming applications to users. A stack consists of an associated fleet, user access
* policies, and storage configurations.
*
*
*
* This is a convenience which creates an instance of the {@link CreateStackRequest.Builder} avoiding the need to
* create one manually via {@link CreateStackRequest#builder()}
*
*
* @param createStackRequest
* A {@link Consumer} that will call methods on {@link CreateStackRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - ResourceAlreadyExistsException The specified resource already exists.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - InvalidRoleException The specified role is invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateStack
* @see AWS API
* Documentation
*/
default CompletableFuture createStack(Consumer createStackRequest) {
return createStack(CreateStackRequest.builder().applyMutation(createStackRequest).build());
}
/**
*
* Creates a temporary URL to start an AppStream 2.0 streaming session for the specified user. A streaming URL
* enables application streaming to be tested without user setup.
*
*
* @param createStreamingUrlRequest
* @return A Java Future containing the result of the CreateStreamingURL operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - OperationNotPermittedException The attempted operation is not permitted.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateStreamingURL
* @see AWS
* API Documentation
*/
default CompletableFuture createStreamingURL(CreateStreamingUrlRequest createStreamingUrlRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a temporary URL to start an AppStream 2.0 streaming session for the specified user. A streaming URL
* enables application streaming to be tested without user setup.
*
*
*
* This is a convenience which creates an instance of the {@link CreateStreamingUrlRequest.Builder} avoiding the
* need to create one manually via {@link CreateStreamingUrlRequest#builder()}
*
*
* @param createStreamingUrlRequest
* A {@link Consumer} that will call methods on {@link CreateStreamingURLRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateStreamingURL operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - OperationNotPermittedException The attempted operation is not permitted.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateStreamingURL
* @see AWS
* API Documentation
*/
default CompletableFuture createStreamingURL(
Consumer createStreamingUrlRequest) {
return createStreamingURL(CreateStreamingUrlRequest.builder().applyMutation(createStreamingUrlRequest).build());
}
/**
*
* Creates a usage report subscription. Usage reports are generated daily.
*
*
* @param createUsageReportSubscriptionRequest
* @return A Java Future containing the result of the CreateUsageReportSubscription operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRoleException The specified role is invalid.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateUsageReportSubscription
* @see AWS API Documentation
*/
default CompletableFuture createUsageReportSubscription(
CreateUsageReportSubscriptionRequest createUsageReportSubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a usage report subscription. Usage reports are generated daily.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUsageReportSubscriptionRequest.Builder}
* avoiding the need to create one manually via {@link CreateUsageReportSubscriptionRequest#builder()}
*
*
* @param createUsageReportSubscriptionRequest
* A {@link Consumer} that will call methods on {@link CreateUsageReportSubscriptionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateUsageReportSubscription operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRoleException The specified role is invalid.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateUsageReportSubscription
* @see AWS API Documentation
*/
default CompletableFuture createUsageReportSubscription(
Consumer createUsageReportSubscriptionRequest) {
return createUsageReportSubscription(CreateUsageReportSubscriptionRequest.builder()
.applyMutation(createUsageReportSubscriptionRequest).build());
}
/**
*
* Creates a new user in the user pool.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The specified resource already exists.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(CreateUserRequest createUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new user in the user pool.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserRequest.Builder} avoiding the need to
* create one manually via {@link CreateUserRequest#builder()}
*
*
* @param createUserRequest
* A {@link Consumer} that will call methods on {@link CreateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The specified resource already exists.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(Consumer createUserRequest) {
return createUser(CreateUserRequest.builder().applyMutation(createUserRequest).build());
}
/**
*
* Deletes the specified Directory Config object from AppStream 2.0. This object includes the information required
* to join streaming instances to an Active Directory domain.
*
*
* @param deleteDirectoryConfigRequest
* @return A Java Future containing the result of the DeleteDirectoryConfig operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteDirectoryConfig
* @see AWS API Documentation
*/
default CompletableFuture deleteDirectoryConfig(
DeleteDirectoryConfigRequest deleteDirectoryConfigRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified Directory Config object from AppStream 2.0. This object includes the information required
* to join streaming instances to an Active Directory domain.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDirectoryConfigRequest.Builder} avoiding the
* need to create one manually via {@link DeleteDirectoryConfigRequest#builder()}
*
*
* @param deleteDirectoryConfigRequest
* A {@link Consumer} that will call methods on {@link DeleteDirectoryConfigRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteDirectoryConfig operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteDirectoryConfig
* @see AWS API Documentation
*/
default CompletableFuture deleteDirectoryConfig(
Consumer deleteDirectoryConfigRequest) {
return deleteDirectoryConfig(DeleteDirectoryConfigRequest.builder().applyMutation(deleteDirectoryConfigRequest).build());
}
/**
*
* Deletes the specified fleet.
*
*
* @param deleteFleetRequest
* @return A Java Future containing the result of the DeleteFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteFleet
* @see AWS API
* Documentation
*/
default CompletableFuture deleteFleet(DeleteFleetRequest deleteFleetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified fleet.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteFleetRequest.Builder} avoiding the need to
* create one manually via {@link DeleteFleetRequest#builder()}
*
*
* @param deleteFleetRequest
* A {@link Consumer} that will call methods on {@link DeleteFleetRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteFleet
* @see AWS API
* Documentation
*/
default CompletableFuture deleteFleet(Consumer deleteFleetRequest) {
return deleteFleet(DeleteFleetRequest.builder().applyMutation(deleteFleetRequest).build());
}
/**
*
* Deletes the specified image. You cannot delete an image when it is in use. After you delete an image, you cannot
* provision new capacity using the image.
*
*
* @param deleteImageRequest
* @return A Java Future containing the result of the DeleteImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - ResourceNotFoundException The specified resource was not found.
* - OperationNotPermittedException The attempted operation is not permitted.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteImage
* @see AWS API
* Documentation
*/
default CompletableFuture deleteImage(DeleteImageRequest deleteImageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified image. You cannot delete an image when it is in use. After you delete an image, you cannot
* provision new capacity using the image.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteImageRequest.Builder} avoiding the need to
* create one manually via {@link DeleteImageRequest#builder()}
*
*
* @param deleteImageRequest
* A {@link Consumer} that will call methods on {@link DeleteImageRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteImage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - ResourceNotFoundException The specified resource was not found.
* - OperationNotPermittedException The attempted operation is not permitted.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteImage
* @see AWS API
* Documentation
*/
default CompletableFuture deleteImage(Consumer deleteImageRequest) {
return deleteImage(DeleteImageRequest.builder().applyMutation(deleteImageRequest).build());
}
/**
*
* Deletes the specified image builder and releases the capacity.
*
*
* @param deleteImageBuilderRequest
* @return A Java Future containing the result of the DeleteImageBuilder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - OperationNotPermittedException The attempted operation is not permitted.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteImageBuilder
* @see AWS
* API Documentation
*/
default CompletableFuture deleteImageBuilder(DeleteImageBuilderRequest deleteImageBuilderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified image builder and releases the capacity.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteImageBuilderRequest.Builder} avoiding the
* need to create one manually via {@link DeleteImageBuilderRequest#builder()}
*
*
* @param deleteImageBuilderRequest
* A {@link Consumer} that will call methods on {@link DeleteImageBuilderRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteImageBuilder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - OperationNotPermittedException The attempted operation is not permitted.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteImageBuilder
* @see AWS
* API Documentation
*/
default CompletableFuture deleteImageBuilder(
Consumer deleteImageBuilderRequest) {
return deleteImageBuilder(DeleteImageBuilderRequest.builder().applyMutation(deleteImageBuilderRequest).build());
}
/**
*
* Deletes permissions for the specified private image. After you delete permissions for an image, AWS accounts to
* which you previously granted these permissions can no longer use the image.
*
*
* @param deleteImagePermissionsRequest
* @return A Java Future containing the result of the DeleteImagePermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteImagePermissions
* @see AWS API Documentation
*/
default CompletableFuture deleteImagePermissions(
DeleteImagePermissionsRequest deleteImagePermissionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes permissions for the specified private image. After you delete permissions for an image, AWS accounts to
* which you previously granted these permissions can no longer use the image.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteImagePermissionsRequest.Builder} avoiding the
* need to create one manually via {@link DeleteImagePermissionsRequest#builder()}
*
*
* @param deleteImagePermissionsRequest
* A {@link Consumer} that will call methods on {@link DeleteImagePermissionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteImagePermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteImagePermissions
* @see AWS API Documentation
*/
default CompletableFuture deleteImagePermissions(
Consumer deleteImagePermissionsRequest) {
return deleteImagePermissions(DeleteImagePermissionsRequest.builder().applyMutation(deleteImagePermissionsRequest)
.build());
}
/**
*
* Deletes the specified stack. After the stack is deleted, the application streaming environment provided by the
* stack is no longer available to users. Also, any reservations made for application streaming sessions for the
* stack are released.
*
*
* @param deleteStackRequest
* @return A Java Future containing the result of the DeleteStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteStack
* @see AWS API
* Documentation
*/
default CompletableFuture deleteStack(DeleteStackRequest deleteStackRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified stack. After the stack is deleted, the application streaming environment provided by the
* stack is no longer available to users. Also, any reservations made for application streaming sessions for the
* stack are released.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteStackRequest.Builder} avoiding the need to
* create one manually via {@link DeleteStackRequest#builder()}
*
*
* @param deleteStackRequest
* A {@link Consumer} that will call methods on {@link DeleteStackRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteStack
* @see AWS API
* Documentation
*/
default CompletableFuture deleteStack(Consumer deleteStackRequest) {
return deleteStack(DeleteStackRequest.builder().applyMutation(deleteStackRequest).build());
}
/**
*
* Disables usage report generation.
*
*
* @param deleteUsageReportSubscriptionRequest
* @return A Java Future containing the result of the DeleteUsageReportSubscription operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteUsageReportSubscription
* @see AWS API Documentation
*/
default CompletableFuture deleteUsageReportSubscription(
DeleteUsageReportSubscriptionRequest deleteUsageReportSubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disables usage report generation.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUsageReportSubscriptionRequest.Builder}
* avoiding the need to create one manually via {@link DeleteUsageReportSubscriptionRequest#builder()}
*
*
* @param deleteUsageReportSubscriptionRequest
* A {@link Consumer} that will call methods on {@link DeleteUsageReportSubscriptionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteUsageReportSubscription operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteUsageReportSubscription
* @see AWS API Documentation
*/
default CompletableFuture deleteUsageReportSubscription(
Consumer deleteUsageReportSubscriptionRequest) {
return deleteUsageReportSubscription(DeleteUsageReportSubscriptionRequest.builder()
.applyMutation(deleteUsageReportSubscriptionRequest).build());
}
/**
*
* Deletes a user from the user pool.
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUser(DeleteUserRequest deleteUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a user from the user pool.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserRequest.Builder} avoiding the need to
* create one manually via {@link DeleteUserRequest#builder()}
*
*
* @param deleteUserRequest
* A {@link Consumer} that will call methods on {@link DeleteUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUser(Consumer deleteUserRequest) {
return deleteUser(DeleteUserRequest.builder().applyMutation(deleteUserRequest).build());
}
/**
*
* Retrieves a list that describes one or more specified Directory Config objects for AppStream 2.0, if the names
* for these objects are provided. Otherwise, all Directory Config objects in the account are described. These
* objects include the configuration information required to join fleets and image builders to Microsoft Active
* Directory domains.
*
*
* Although the response syntax in this topic includes the account password, this password is not returned in the
* actual response.
*
*
* @param describeDirectoryConfigsRequest
* @return A Java Future containing the result of the DescribeDirectoryConfigs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeDirectoryConfigs
* @see AWS API Documentation
*/
default CompletableFuture describeDirectoryConfigs(
DescribeDirectoryConfigsRequest describeDirectoryConfigsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list that describes one or more specified Directory Config objects for AppStream 2.0, if the names
* for these objects are provided. Otherwise, all Directory Config objects in the account are described. These
* objects include the configuration information required to join fleets and image builders to Microsoft Active
* Directory domains.
*
*
* Although the response syntax in this topic includes the account password, this password is not returned in the
* actual response.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDirectoryConfigsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDirectoryConfigsRequest#builder()}
*
*
* @param describeDirectoryConfigsRequest
* A {@link Consumer} that will call methods on {@link DescribeDirectoryConfigsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDirectoryConfigs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeDirectoryConfigs
* @see AWS API Documentation
*/
default CompletableFuture describeDirectoryConfigs(
Consumer describeDirectoryConfigsRequest) {
return describeDirectoryConfigs(DescribeDirectoryConfigsRequest.builder().applyMutation(describeDirectoryConfigsRequest)
.build());
}
/**
*
* Retrieves a list that describes one or more specified Directory Config objects for AppStream 2.0, if the names
* for these objects are provided. Otherwise, all Directory Config objects in the account are described. These
* objects include the configuration information required to join fleets and image builders to Microsoft Active
* Directory domains.
*
*
* Although the response syntax in this topic includes the account password, this password is not returned in the
* actual response.
*
*
* @return A Java Future containing the result of the DescribeDirectoryConfigs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeDirectoryConfigs
* @see AWS API Documentation
*/
default CompletableFuture describeDirectoryConfigs() {
return describeDirectoryConfigs(DescribeDirectoryConfigsRequest.builder().build());
}
/**
*
* Retrieves a list that describes one or more specified fleets, if the fleet names are provided. Otherwise, all
* fleets in the account are described.
*
*
* @param describeFleetsRequest
* @return A Java Future containing the result of the DescribeFleets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeFleets
* @see AWS API
* Documentation
*/
default CompletableFuture describeFleets(DescribeFleetsRequest describeFleetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list that describes one or more specified fleets, if the fleet names are provided. Otherwise, all
* fleets in the account are described.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeFleetsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeFleetsRequest#builder()}
*
*
* @param describeFleetsRequest
* A {@link Consumer} that will call methods on {@link DescribeFleetsRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeFleets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeFleets
* @see AWS API
* Documentation
*/
default CompletableFuture describeFleets(Consumer describeFleetsRequest) {
return describeFleets(DescribeFleetsRequest.builder().applyMutation(describeFleetsRequest).build());
}
/**
*
* Retrieves a list that describes one or more specified fleets, if the fleet names are provided. Otherwise, all
* fleets in the account are described.
*
*
* @return A Java Future containing the result of the DescribeFleets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeFleets
* @see AWS API
* Documentation
*/
default CompletableFuture describeFleets() {
return describeFleets(DescribeFleetsRequest.builder().build());
}
/**
*
* Retrieves a list that describes one or more specified image builders, if the image builder names are provided.
* Otherwise, all image builders in the account are described.
*
*
* @param describeImageBuildersRequest
* @return A Java Future containing the result of the DescribeImageBuilders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImageBuilders
* @see AWS API Documentation
*/
default CompletableFuture describeImageBuilders(
DescribeImageBuildersRequest describeImageBuildersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list that describes one or more specified image builders, if the image builder names are provided.
* Otherwise, all image builders in the account are described.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImageBuildersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeImageBuildersRequest#builder()}
*
*
* @param describeImageBuildersRequest
* A {@link Consumer} that will call methods on {@link DescribeImageBuildersRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeImageBuilders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImageBuilders
* @see AWS API Documentation
*/
default CompletableFuture describeImageBuilders(
Consumer describeImageBuildersRequest) {
return describeImageBuilders(DescribeImageBuildersRequest.builder().applyMutation(describeImageBuildersRequest).build());
}
/**
*
* Retrieves a list that describes one or more specified image builders, if the image builder names are provided.
* Otherwise, all image builders in the account are described.
*
*
* @return A Java Future containing the result of the DescribeImageBuilders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImageBuilders
* @see AWS API Documentation
*/
default CompletableFuture describeImageBuilders() {
return describeImageBuilders(DescribeImageBuildersRequest.builder().build());
}
/**
*
* Retrieves a list that describes the permissions for shared AWS account IDs on a private image that you own.
*
*
* @param describeImagePermissionsRequest
* @return A Java Future containing the result of the DescribeImagePermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImagePermissions
* @see AWS API Documentation
*/
default CompletableFuture describeImagePermissions(
DescribeImagePermissionsRequest describeImagePermissionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list that describes the permissions for shared AWS account IDs on a private image that you own.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImagePermissionsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeImagePermissionsRequest#builder()}
*
*
* @param describeImagePermissionsRequest
* A {@link Consumer} that will call methods on {@link DescribeImagePermissionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeImagePermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImagePermissions
* @see AWS API Documentation
*/
default CompletableFuture describeImagePermissions(
Consumer describeImagePermissionsRequest) {
return describeImagePermissions(DescribeImagePermissionsRequest.builder().applyMutation(describeImagePermissionsRequest)
.build());
}
/**
*
* Retrieves a list that describes the permissions for shared AWS account IDs on a private image that you own.
*
*
*
* This is a variant of
* {@link #describeImagePermissions(software.amazon.awssdk.services.appstream.model.DescribeImagePermissionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.appstream.paginators.DescribeImagePermissionsPublisher publisher = client.describeImagePermissionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.appstream.paginators.DescribeImagePermissionsPublisher publisher = client.describeImagePermissionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.appstream.model.DescribeImagePermissionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImagePermissions(software.amazon.awssdk.services.appstream.model.DescribeImagePermissionsRequest)}
* operation.
*
*
* @param describeImagePermissionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImagePermissions
* @see AWS API Documentation
*/
default DescribeImagePermissionsPublisher describeImagePermissionsPaginator(
DescribeImagePermissionsRequest describeImagePermissionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list that describes the permissions for shared AWS account IDs on a private image that you own.
*
*
*
* This is a variant of
* {@link #describeImagePermissions(software.amazon.awssdk.services.appstream.model.DescribeImagePermissionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.appstream.paginators.DescribeImagePermissionsPublisher publisher = client.describeImagePermissionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.appstream.paginators.DescribeImagePermissionsPublisher publisher = client.describeImagePermissionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.appstream.model.DescribeImagePermissionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImagePermissions(software.amazon.awssdk.services.appstream.model.DescribeImagePermissionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeImagePermissionsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeImagePermissionsRequest#builder()}
*
*
* @param describeImagePermissionsRequest
* A {@link Consumer} that will call methods on {@link DescribeImagePermissionsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImagePermissions
* @see AWS API Documentation
*/
default DescribeImagePermissionsPublisher describeImagePermissionsPaginator(
Consumer describeImagePermissionsRequest) {
return describeImagePermissionsPaginator(DescribeImagePermissionsRequest.builder()
.applyMutation(describeImagePermissionsRequest).build());
}
/**
*
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided.
* Otherwise, all images in the account are described.
*
*
* @param describeImagesRequest
* @return A Java Future containing the result of the DescribeImages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default CompletableFuture describeImages(DescribeImagesRequest describeImagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided.
* Otherwise, all images in the account are described.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeImagesRequest.Builder} avoiding the need to
* create one manually via {@link DescribeImagesRequest#builder()}
*
*
* @param describeImagesRequest
* A {@link Consumer} that will call methods on {@link DescribeImagesRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeImages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default CompletableFuture describeImages(Consumer describeImagesRequest) {
return describeImages(DescribeImagesRequest.builder().applyMutation(describeImagesRequest).build());
}
/**
*
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided.
* Otherwise, all images in the account are described.
*
*
* @return A Java Future containing the result of the DescribeImages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default CompletableFuture describeImages() {
return describeImages(DescribeImagesRequest.builder().build());
}
/**
*
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided.
* Otherwise, all images in the account are described.
*
*
*
* This is a variant of
* {@link #describeImages(software.amazon.awssdk.services.appstream.model.DescribeImagesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.appstream.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.appstream.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.appstream.model.DescribeImagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImages(software.amazon.awssdk.services.appstream.model.DescribeImagesRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default DescribeImagesPublisher describeImagesPaginator() {
return describeImagesPaginator(DescribeImagesRequest.builder().build());
}
/**
*
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided.
* Otherwise, all images in the account are described.
*
*
*
* This is a variant of
* {@link #describeImages(software.amazon.awssdk.services.appstream.model.DescribeImagesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.appstream.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.appstream.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.appstream.model.DescribeImagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImages(software.amazon.awssdk.services.appstream.model.DescribeImagesRequest)} operation.
*
*
* @param describeImagesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default DescribeImagesPublisher describeImagesPaginator(DescribeImagesRequest describeImagesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided.
* Otherwise, all images in the account are described.
*
*
*
* This is a variant of
* {@link #describeImages(software.amazon.awssdk.services.appstream.model.DescribeImagesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.appstream.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.appstream.paginators.DescribeImagesPublisher publisher = client.describeImagesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.appstream.model.DescribeImagesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeImages(software.amazon.awssdk.services.appstream.model.DescribeImagesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeImagesRequest.Builder} avoiding the need to
* create one manually via {@link DescribeImagesRequest#builder()}
*
*
* @param describeImagesRequest
* A {@link Consumer} that will call methods on {@link DescribeImagesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeImages
* @see AWS API
* Documentation
*/
default DescribeImagesPublisher describeImagesPaginator(Consumer describeImagesRequest) {
return describeImagesPaginator(DescribeImagesRequest.builder().applyMutation(describeImagesRequest).build());
}
/**
*
* Retrieves a list that describes the streaming sessions for a specified stack and fleet. If a UserId is provided
* for the stack and fleet, only streaming sessions for that user are described. If an authentication type is not
* provided, the default is to authenticate users using a streaming URL.
*
*
* @param describeSessionsRequest
* @return A Java Future containing the result of the DescribeSessions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeSessions
* @see AWS API
* Documentation
*/
default CompletableFuture describeSessions(DescribeSessionsRequest describeSessionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list that describes the streaming sessions for a specified stack and fleet. If a UserId is provided
* for the stack and fleet, only streaming sessions for that user are described. If an authentication type is not
* provided, the default is to authenticate users using a streaming URL.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeSessionsRequest.Builder} avoiding the need
* to create one manually via {@link DescribeSessionsRequest#builder()}
*
*
* @param describeSessionsRequest
* A {@link Consumer} that will call methods on {@link DescribeSessionsRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeSessions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeSessions
* @see AWS API
* Documentation
*/
default CompletableFuture describeSessions(
Consumer describeSessionsRequest) {
return describeSessions(DescribeSessionsRequest.builder().applyMutation(describeSessionsRequest).build());
}
/**
*
* Retrieves a list that describes one or more specified stacks, if the stack names are provided. Otherwise, all
* stacks in the account are described.
*
*
* @param describeStacksRequest
* @return A Java Future containing the result of the DescribeStacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeStacks
* @see AWS API
* Documentation
*/
default CompletableFuture describeStacks(DescribeStacksRequest describeStacksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list that describes one or more specified stacks, if the stack names are provided. Otherwise, all
* stacks in the account are described.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStacksRequest.Builder} avoiding the need to
* create one manually via {@link DescribeStacksRequest#builder()}
*
*
* @param describeStacksRequest
* A {@link Consumer} that will call methods on {@link DescribeStacksRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeStacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeStacks
* @see AWS API
* Documentation
*/
default CompletableFuture describeStacks(Consumer describeStacksRequest) {
return describeStacks(DescribeStacksRequest.builder().applyMutation(describeStacksRequest).build());
}
/**
*
* Retrieves a list that describes one or more specified stacks, if the stack names are provided. Otherwise, all
* stacks in the account are described.
*
*
* @return A Java Future containing the result of the DescribeStacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeStacks
* @see AWS API
* Documentation
*/
default CompletableFuture describeStacks() {
return describeStacks(DescribeStacksRequest.builder().build());
}
/**
*
* Retrieves a list that describes one or more usage report subscriptions.
*
*
* @param describeUsageReportSubscriptionsRequest
* @return A Java Future containing the result of the DescribeUsageReportSubscriptions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeUsageReportSubscriptions
* @see AWS API Documentation
*/
default CompletableFuture describeUsageReportSubscriptions(
DescribeUsageReportSubscriptionsRequest describeUsageReportSubscriptionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list that describes one or more usage report subscriptions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUsageReportSubscriptionsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeUsageReportSubscriptionsRequest#builder()}
*
*
* @param describeUsageReportSubscriptionsRequest
* A {@link Consumer} that will call methods on {@link DescribeUsageReportSubscriptionsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeUsageReportSubscriptions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeUsageReportSubscriptions
* @see AWS API Documentation
*/
default CompletableFuture describeUsageReportSubscriptions(
Consumer describeUsageReportSubscriptionsRequest) {
return describeUsageReportSubscriptions(DescribeUsageReportSubscriptionsRequest.builder()
.applyMutation(describeUsageReportSubscriptionsRequest).build());
}
/**
*
* Retrieves a list that describes the UserStackAssociation objects. You must specify either or both of the
* following:
*
*
* -
*
* The stack name
*
*
* -
*
* The user name (email address of the user associated with the stack) and the authentication type for the user
*
*
*
*
* @param describeUserStackAssociationsRequest
* @return A Java Future containing the result of the DescribeUserStackAssociations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeUserStackAssociations
* @see AWS API Documentation
*/
default CompletableFuture describeUserStackAssociations(
DescribeUserStackAssociationsRequest describeUserStackAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list that describes the UserStackAssociation objects. You must specify either or both of the
* following:
*
*
* -
*
* The stack name
*
*
* -
*
* The user name (email address of the user associated with the stack) and the authentication type for the user
*
*
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUserStackAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeUserStackAssociationsRequest#builder()}
*
*
* @param describeUserStackAssociationsRequest
* A {@link Consumer} that will call methods on {@link DescribeUserStackAssociationsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeUserStackAssociations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeUserStackAssociations
* @see AWS API Documentation
*/
default CompletableFuture describeUserStackAssociations(
Consumer describeUserStackAssociationsRequest) {
return describeUserStackAssociations(DescribeUserStackAssociationsRequest.builder()
.applyMutation(describeUserStackAssociationsRequest).build());
}
/**
*
* Retrieves a list that describes one or more specified users in the user pool.
*
*
* @param describeUsersRequest
* @return A Java Future containing the result of the DescribeUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeUsers
* @see AWS API
* Documentation
*/
default CompletableFuture describeUsers(DescribeUsersRequest describeUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list that describes one or more specified users in the user pool.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUsersRequest.Builder} avoiding the need to
* create one manually via {@link DescribeUsersRequest#builder()}
*
*
* @param describeUsersRequest
* A {@link Consumer} that will call methods on {@link DescribeUsersRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DescribeUsers
* @see AWS API
* Documentation
*/
default CompletableFuture describeUsers(Consumer describeUsersRequest) {
return describeUsers(DescribeUsersRequest.builder().applyMutation(describeUsersRequest).build());
}
/**
*
* Disables the specified user in the user pool. Users can't sign in to AppStream 2.0 until they are re-enabled.
* This action does not delete the user.
*
*
* @param disableUserRequest
* @return A Java Future containing the result of the DisableUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DisableUser
* @see AWS API
* Documentation
*/
default CompletableFuture disableUser(DisableUserRequest disableUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disables the specified user in the user pool. Users can't sign in to AppStream 2.0 until they are re-enabled.
* This action does not delete the user.
*
*
*
* This is a convenience which creates an instance of the {@link DisableUserRequest.Builder} avoiding the need to
* create one manually via {@link DisableUserRequest#builder()}
*
*
* @param disableUserRequest
* A {@link Consumer} that will call methods on {@link DisableUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DisableUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DisableUser
* @see AWS API
* Documentation
*/
default CompletableFuture disableUser(Consumer disableUserRequest) {
return disableUser(DisableUserRequest.builder().applyMutation(disableUserRequest).build());
}
/**
*
* Disassociates the specified fleet from the specified stack.
*
*
* @param disassociateFleetRequest
* @return A Java Future containing the result of the DisassociateFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DisassociateFleet
* @see AWS
* API Documentation
*/
default CompletableFuture disassociateFleet(DisassociateFleetRequest disassociateFleetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates the specified fleet from the specified stack.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateFleetRequest.Builder} avoiding the need
* to create one manually via {@link DisassociateFleetRequest#builder()}
*
*
* @param disassociateFleetRequest
* A {@link Consumer} that will call methods on {@link DisassociateFleetRequest.Builder} to create a request.
* @return A Java Future containing the result of the DisassociateFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.DisassociateFleet
* @see AWS
* API Documentation
*/
default CompletableFuture disassociateFleet(
Consumer disassociateFleetRequest) {
return disassociateFleet(DisassociateFleetRequest.builder().applyMutation(disassociateFleetRequest).build());
}
/**
*
* Enables a user in the user pool. After being enabled, users can sign in to AppStream 2.0 and open applications
* from the stacks to which they are assigned.
*
*
* @param enableUserRequest
* @return A Java Future containing the result of the EnableUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.EnableUser
* @see AWS API
* Documentation
*/
default CompletableFuture enableUser(EnableUserRequest enableUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Enables a user in the user pool. After being enabled, users can sign in to AppStream 2.0 and open applications
* from the stacks to which they are assigned.
*
*
*
* This is a convenience which creates an instance of the {@link EnableUserRequest.Builder} avoiding the need to
* create one manually via {@link EnableUserRequest#builder()}
*
*
* @param enableUserRequest
* A {@link Consumer} that will call methods on {@link EnableUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the EnableUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.EnableUser
* @see AWS API
* Documentation
*/
default CompletableFuture enableUser(Consumer enableUserRequest) {
return enableUser(EnableUserRequest.builder().applyMutation(enableUserRequest).build());
}
/**
*
* Immediately stops the specified streaming session.
*
*
* @param expireSessionRequest
* @return A Java Future containing the result of the ExpireSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.ExpireSession
* @see AWS API
* Documentation
*/
default CompletableFuture expireSession(ExpireSessionRequest expireSessionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Immediately stops the specified streaming session.
*
*
*
* This is a convenience which creates an instance of the {@link ExpireSessionRequest.Builder} avoiding the need to
* create one manually via {@link ExpireSessionRequest#builder()}
*
*
* @param expireSessionRequest
* A {@link Consumer} that will call methods on {@link ExpireSessionRequest.Builder} to create a request.
* @return A Java Future containing the result of the ExpireSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.ExpireSession
* @see AWS API
* Documentation
*/
default CompletableFuture expireSession(Consumer expireSessionRequest) {
return expireSession(ExpireSessionRequest.builder().applyMutation(expireSessionRequest).build());
}
/**
*
* Retrieves the name of the fleet that is associated with the specified stack.
*
*
* @param listAssociatedFleetsRequest
* @return A Java Future containing the result of the ListAssociatedFleets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.ListAssociatedFleets
* @see AWS
* API Documentation
*/
default CompletableFuture listAssociatedFleets(
ListAssociatedFleetsRequest listAssociatedFleetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the name of the fleet that is associated with the specified stack.
*
*
*
* This is a convenience which creates an instance of the {@link ListAssociatedFleetsRequest.Builder} avoiding the
* need to create one manually via {@link ListAssociatedFleetsRequest#builder()}
*
*
* @param listAssociatedFleetsRequest
* A {@link Consumer} that will call methods on {@link ListAssociatedFleetsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListAssociatedFleets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.ListAssociatedFleets
* @see AWS
* API Documentation
*/
default CompletableFuture listAssociatedFleets(
Consumer listAssociatedFleetsRequest) {
return listAssociatedFleets(ListAssociatedFleetsRequest.builder().applyMutation(listAssociatedFleetsRequest).build());
}
/**
*
* Retrieves the name of the stack with which the specified fleet is associated.
*
*
* @param listAssociatedStacksRequest
* @return A Java Future containing the result of the ListAssociatedStacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.ListAssociatedStacks
* @see AWS
* API Documentation
*/
default CompletableFuture listAssociatedStacks(
ListAssociatedStacksRequest listAssociatedStacksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the name of the stack with which the specified fleet is associated.
*
*
*
* This is a convenience which creates an instance of the {@link ListAssociatedStacksRequest.Builder} avoiding the
* need to create one manually via {@link ListAssociatedStacksRequest#builder()}
*
*
* @param listAssociatedStacksRequest
* A {@link Consumer} that will call methods on {@link ListAssociatedStacksRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListAssociatedStacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.ListAssociatedStacks
* @see AWS
* API Documentation
*/
default CompletableFuture listAssociatedStacks(
Consumer listAssociatedStacksRequest) {
return listAssociatedStacks(ListAssociatedStacksRequest.builder().applyMutation(listAssociatedStacksRequest).build());
}
/**
*
* Retrieves a list of all tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image builders,
* images, fleets, and stacks.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of all tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image builders,
* images, fleets, and stacks.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Starts the specified fleet.
*
*
* @param startFleetRequest
* @return A Java Future containing the result of the StartFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - OperationNotPermittedException The attempted operation is not permitted.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - RequestLimitExceededException AppStream 2.0 can’t process the request right now because the Describe
* calls from your AWS account are being throttled by Amazon EC2. Try again later.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - InvalidRoleException The specified role is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.StartFleet
* @see AWS API
* Documentation
*/
default CompletableFuture startFleet(StartFleetRequest startFleetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts the specified fleet.
*
*
*
* This is a convenience which creates an instance of the {@link StartFleetRequest.Builder} avoiding the need to
* create one manually via {@link StartFleetRequest#builder()}
*
*
* @param startFleetRequest
* A {@link Consumer} that will call methods on {@link StartFleetRequest.Builder} to create a request.
* @return A Java Future containing the result of the StartFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - OperationNotPermittedException The attempted operation is not permitted.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - RequestLimitExceededException AppStream 2.0 can’t process the request right now because the Describe
* calls from your AWS account are being throttled by Amazon EC2. Try again later.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - InvalidRoleException The specified role is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.StartFleet
* @see AWS API
* Documentation
*/
default CompletableFuture startFleet(Consumer startFleetRequest) {
return startFleet(StartFleetRequest.builder().applyMutation(startFleetRequest).build());
}
/**
*
* Starts the specified image builder.
*
*
* @param startImageBuilderRequest
* @return A Java Future containing the result of the StartImageBuilder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - IncompatibleImageException The image does not support storage connectors.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.StartImageBuilder
* @see AWS
* API Documentation
*/
default CompletableFuture startImageBuilder(StartImageBuilderRequest startImageBuilderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts the specified image builder.
*
*
*
* This is a convenience which creates an instance of the {@link StartImageBuilderRequest.Builder} avoiding the need
* to create one manually via {@link StartImageBuilderRequest#builder()}
*
*
* @param startImageBuilderRequest
* A {@link Consumer} that will call methods on {@link StartImageBuilderRequest.Builder} to create a request.
* @return A Java Future containing the result of the StartImageBuilder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - IncompatibleImageException The image does not support storage connectors.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.StartImageBuilder
* @see AWS
* API Documentation
*/
default CompletableFuture startImageBuilder(
Consumer startImageBuilderRequest) {
return startImageBuilder(StartImageBuilderRequest.builder().applyMutation(startImageBuilderRequest).build());
}
/**
*
* Stops the specified fleet.
*
*
* @param stopFleetRequest
* @return A Java Future containing the result of the StopFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.StopFleet
* @see AWS API
* Documentation
*/
default CompletableFuture stopFleet(StopFleetRequest stopFleetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Stops the specified fleet.
*
*
*
* This is a convenience which creates an instance of the {@link StopFleetRequest.Builder} avoiding the need to
* create one manually via {@link StopFleetRequest#builder()}
*
*
* @param stopFleetRequest
* A {@link Consumer} that will call methods on {@link StopFleetRequest.Builder} to create a request.
* @return A Java Future containing the result of the StopFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.StopFleet
* @see AWS API
* Documentation
*/
default CompletableFuture stopFleet(Consumer stopFleetRequest) {
return stopFleet(StopFleetRequest.builder().applyMutation(stopFleetRequest).build());
}
/**
*
* Stops the specified image builder.
*
*
* @param stopImageBuilderRequest
* @return A Java Future containing the result of the StopImageBuilder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - OperationNotPermittedException The attempted operation is not permitted.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.StopImageBuilder
* @see AWS API
* Documentation
*/
default CompletableFuture stopImageBuilder(StopImageBuilderRequest stopImageBuilderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Stops the specified image builder.
*
*
*
* This is a convenience which creates an instance of the {@link StopImageBuilderRequest.Builder} avoiding the need
* to create one manually via {@link StopImageBuilderRequest#builder()}
*
*
* @param stopImageBuilderRequest
* A {@link Consumer} that will call methods on {@link StopImageBuilderRequest.Builder} to create a request.
* @return A Java Future containing the result of the StopImageBuilder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - OperationNotPermittedException The attempted operation is not permitted.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.StopImageBuilder
* @see AWS API
* Documentation
*/
default CompletableFuture stopImageBuilder(
Consumer stopImageBuilderRequest) {
return stopImageBuilder(StopImageBuilderRequest.builder().applyMutation(stopImageBuilderRequest).build());
}
/**
*
* Adds or overwrites one or more tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image
* builders, images, fleets, and stacks.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key, this
* operation updates its value.
*
*
* To list the current tags for your resources, use ListTagsForResource. To disassociate tags from your
* resources, use UntagResource.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds or overwrites one or more tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image
* builders, images, fleets, and stacks.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key, this
* operation updates its value.
*
*
* To list the current tags for your resources, use ListTagsForResource. To disassociate tags from your
* resources, use UntagResource.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Disassociates one or more specified tags from the specified AppStream 2.0 resource.
*
*
* To list the current tags for your resources, use ListTagsForResource.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates one or more specified tags from the specified AppStream 2.0 resource.
*
*
* To list the current tags for your resources, use ListTagsForResource.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates the specified Directory Config object in AppStream 2.0. This object includes the configuration
* information required to join fleets and image builders to Microsoft Active Directory domains.
*
*
* @param updateDirectoryConfigRequest
* @return A Java Future containing the result of the UpdateDirectoryConfig operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - OperationNotPermittedException The attempted operation is not permitted.
* - InvalidRoleException The specified role is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.UpdateDirectoryConfig
* @see AWS API Documentation
*/
default CompletableFuture updateDirectoryConfig(
UpdateDirectoryConfigRequest updateDirectoryConfigRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified Directory Config object in AppStream 2.0. This object includes the configuration
* information required to join fleets and image builders to Microsoft Active Directory domains.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDirectoryConfigRequest.Builder} avoiding the
* need to create one manually via {@link UpdateDirectoryConfigRequest#builder()}
*
*
* @param updateDirectoryConfigRequest
* A {@link Consumer} that will call methods on {@link UpdateDirectoryConfigRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateDirectoryConfig operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - ResourceNotFoundException The specified resource was not found.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - OperationNotPermittedException The attempted operation is not permitted.
* - InvalidRoleException The specified role is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.UpdateDirectoryConfig
* @see AWS API Documentation
*/
default CompletableFuture updateDirectoryConfig(
Consumer updateDirectoryConfigRequest) {
return updateDirectoryConfig(UpdateDirectoryConfigRequest.builder().applyMutation(updateDirectoryConfigRequest).build());
}
/**
*
* Updates the specified fleet.
*
*
* If the fleet is in the STOPPED
state, you can update any attribute except the fleet name. If the
* fleet is in the RUNNING
state, you can update the DisplayName
,
* ComputeCapacity
, ImageARN
, ImageName
,
* IdleDisconnectTimeoutInSeconds
, and DisconnectTimeoutInSeconds
attributes. If the fleet
* is in the STARTING
or STOPPING
state, you can't update it.
*
*
* @param updateFleetRequest
* @return A Java Future containing the result of the UpdateFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - RequestLimitExceededException AppStream 2.0 can’t process the request right now because the Describe
* calls from your AWS account are being throttled by Amazon EC2. Try again later.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - InvalidRoleException The specified role is invalid.
* - ResourceNotFoundException The specified resource was not found.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - IncompatibleImageException The image does not support storage connectors.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.UpdateFleet
* @see AWS API
* Documentation
*/
default CompletableFuture updateFleet(UpdateFleetRequest updateFleetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified fleet.
*
*
* If the fleet is in the STOPPED
state, you can update any attribute except the fleet name. If the
* fleet is in the RUNNING
state, you can update the DisplayName
,
* ComputeCapacity
, ImageARN
, ImageName
,
* IdleDisconnectTimeoutInSeconds
, and DisconnectTimeoutInSeconds
attributes. If the fleet
* is in the STARTING
or STOPPING
state, you can't update it.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateFleetRequest.Builder} avoiding the need to
* create one manually via {@link UpdateFleetRequest#builder()}
*
*
* @param updateFleetRequest
* A {@link Consumer} that will call methods on {@link UpdateFleetRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceInUseException The specified resource is in use.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - RequestLimitExceededException AppStream 2.0 can’t process the request right now because the Describe
* calls from your AWS account are being throttled by Amazon EC2. Try again later.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - InvalidRoleException The specified role is invalid.
* - ResourceNotFoundException The specified resource was not found.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - IncompatibleImageException The image does not support storage connectors.
* - OperationNotPermittedException The attempted operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.UpdateFleet
* @see AWS API
* Documentation
*/
default CompletableFuture updateFleet(Consumer updateFleetRequest) {
return updateFleet(UpdateFleetRequest.builder().applyMutation(updateFleetRequest).build());
}
/**
*
* Adds or updates permissions for the specified private image.
*
*
* @param updateImagePermissionsRequest
* @return A Java Future containing the result of the UpdateImagePermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.UpdateImagePermissions
* @see AWS API Documentation
*/
default CompletableFuture updateImagePermissions(
UpdateImagePermissionsRequest updateImagePermissionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds or updates permissions for the specified private image.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateImagePermissionsRequest.Builder} avoiding the
* need to create one manually via {@link UpdateImagePermissionsRequest#builder()}
*
*
* @param updateImagePermissionsRequest
* A {@link Consumer} that will call methods on {@link UpdateImagePermissionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateImagePermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceNotAvailableException The specified resource exists and is not in use, but isn't available.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.UpdateImagePermissions
* @see AWS API Documentation
*/
default CompletableFuture updateImagePermissions(
Consumer updateImagePermissionsRequest) {
return updateImagePermissions(UpdateImagePermissionsRequest.builder().applyMutation(updateImagePermissionsRequest)
.build());
}
/**
*
* Updates the specified fields for the specified stack.
*
*
* @param updateStackRequest
* @return A Java Future containing the result of the UpdateStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceInUseException The specified resource is in use.
* - InvalidRoleException The specified role is invalid.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - IncompatibleImageException The image does not support storage connectors.
* - OperationNotPermittedException The attempted operation is not permitted.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.UpdateStack
* @see AWS API
* Documentation
*/
default CompletableFuture updateStack(UpdateStackRequest updateStackRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified fields for the specified stack.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateStackRequest.Builder} avoiding the need to
* create one manually via {@link UpdateStackRequest#builder()}
*
*
* @param updateStackRequest
* A {@link Consumer} that will call methods on {@link UpdateStackRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceInUseException The specified resource is in use.
* - InvalidRoleException The specified role is invalid.
* - InvalidParameterCombinationException Indicates an incorrect combination of parameters, or a missing
* parameter.
* - LimitExceededException The requested limit exceeds the permitted limit for an account.
* - InvalidAccountStatusException The resource cannot be created because your AWS account is suspended.
* For assistance, contact AWS Support.
* - IncompatibleImageException The image does not support storage connectors.
* - OperationNotPermittedException The attempted operation is not permitted.
* - ConcurrentModificationException An API error occurred. Wait a few minutes and try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - AppStreamException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample AppStreamAsyncClient.UpdateStack
* @see AWS API
* Documentation
*/
default CompletableFuture updateStack(Consumer updateStackRequest) {
return updateStack(UpdateStackRequest.builder().applyMutation(updateStackRequest).build());
}
/**
* Create an instance of {@link AppStreamAsyncWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link AppStreamAsyncWaiter}
*/
default AppStreamAsyncWaiter waiter() {
throw new UnsupportedOperationException();
}
}