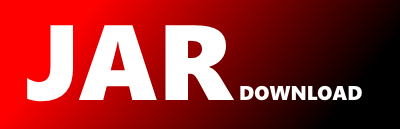
software.amazon.awssdk.services.appstream.model.Image Maven / Gradle / Ivy
Show all versions of appstream Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.appstream.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes an image.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Image implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(Image::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(Image::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField BASE_IMAGE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BaseImageArn").getter(getter(Image::baseImageArn)).setter(setter(Builder::baseImageArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BaseImageArn").build()).build();
private static final SdkField DISPLAY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DisplayName").getter(getter(Image::displayName)).setter(setter(Builder::displayName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DisplayName").build()).build();
private static final SdkField STATE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("State")
.getter(getter(Image::stateAsString)).setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State").build()).build();
private static final SdkField VISIBILITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Visibility").getter(getter(Image::visibilityAsString)).setter(setter(Builder::visibility))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Visibility").build()).build();
private static final SdkField IMAGE_BUILDER_SUPPORTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ImageBuilderSupported").getter(getter(Image::imageBuilderSupported))
.setter(setter(Builder::imageBuilderSupported))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageBuilderSupported").build())
.build();
private static final SdkField IMAGE_BUILDER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ImageBuilderName").getter(getter(Image::imageBuilderName)).setter(setter(Builder::imageBuilderName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageBuilderName").build()).build();
private static final SdkField PLATFORM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Platform").getter(getter(Image::platformAsString)).setter(setter(Builder::platform))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Platform").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(Image::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField STATE_CHANGE_REASON_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StateChangeReason")
.getter(getter(Image::stateChangeReason)).setter(setter(Builder::stateChangeReason))
.constructor(ImageStateChangeReason::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateChangeReason").build()).build();
private static final SdkField> APPLICATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Applications")
.getter(getter(Image::applications))
.setter(setter(Builder::applications))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Applications").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Application::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CREATED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedTime").getter(getter(Image::createdTime)).setter(setter(Builder::createdTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedTime").build()).build();
private static final SdkField PUBLIC_BASE_IMAGE_RELEASED_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("PublicBaseImageReleasedDate")
.getter(getter(Image::publicBaseImageReleasedDate))
.setter(setter(Builder::publicBaseImageReleasedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PublicBaseImageReleasedDate")
.build()).build();
private static final SdkField APPSTREAM_AGENT_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AppstreamAgentVersion").getter(getter(Image::appstreamAgentVersion))
.setter(setter(Builder::appstreamAgentVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AppstreamAgentVersion").build())
.build();
private static final SdkField IMAGE_PERMISSIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ImagePermissions")
.getter(getter(Image::imagePermissions)).setter(setter(Builder::imagePermissions))
.constructor(ImagePermissions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImagePermissions").build()).build();
private static final SdkField> IMAGE_ERRORS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ImageErrors")
.getter(getter(Image::imageErrors))
.setter(setter(Builder::imageErrors))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageErrors").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ResourceError::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, ARN_FIELD,
BASE_IMAGE_ARN_FIELD, DISPLAY_NAME_FIELD, STATE_FIELD, VISIBILITY_FIELD, IMAGE_BUILDER_SUPPORTED_FIELD,
IMAGE_BUILDER_NAME_FIELD, PLATFORM_FIELD, DESCRIPTION_FIELD, STATE_CHANGE_REASON_FIELD, APPLICATIONS_FIELD,
CREATED_TIME_FIELD, PUBLIC_BASE_IMAGE_RELEASED_DATE_FIELD, APPSTREAM_AGENT_VERSION_FIELD, IMAGE_PERMISSIONS_FIELD,
IMAGE_ERRORS_FIELD));
private static final long serialVersionUID = 1L;
private final String name;
private final String arn;
private final String baseImageArn;
private final String displayName;
private final String state;
private final String visibility;
private final Boolean imageBuilderSupported;
private final String imageBuilderName;
private final String platform;
private final String description;
private final ImageStateChangeReason stateChangeReason;
private final List applications;
private final Instant createdTime;
private final Instant publicBaseImageReleasedDate;
private final String appstreamAgentVersion;
private final ImagePermissions imagePermissions;
private final List imageErrors;
private Image(BuilderImpl builder) {
this.name = builder.name;
this.arn = builder.arn;
this.baseImageArn = builder.baseImageArn;
this.displayName = builder.displayName;
this.state = builder.state;
this.visibility = builder.visibility;
this.imageBuilderSupported = builder.imageBuilderSupported;
this.imageBuilderName = builder.imageBuilderName;
this.platform = builder.platform;
this.description = builder.description;
this.stateChangeReason = builder.stateChangeReason;
this.applications = builder.applications;
this.createdTime = builder.createdTime;
this.publicBaseImageReleasedDate = builder.publicBaseImageReleasedDate;
this.appstreamAgentVersion = builder.appstreamAgentVersion;
this.imagePermissions = builder.imagePermissions;
this.imageErrors = builder.imageErrors;
}
/**
*
* The name of the image.
*
*
* @return The name of the image.
*/
public final String name() {
return name;
}
/**
*
* The ARN of the image.
*
*
* @return The ARN of the image.
*/
public final String arn() {
return arn;
}
/**
*
* The ARN of the image from which this image was created.
*
*
* @return The ARN of the image from which this image was created.
*/
public final String baseImageArn() {
return baseImageArn;
}
/**
*
* The image name to display.
*
*
* @return The image name to display.
*/
public final String displayName() {
return displayName;
}
/**
*
* The image starts in the PENDING
state. If image creation succeeds, the state is
* AVAILABLE
. If image creation fails, the state is FAILED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link ImageState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The image starts in the PENDING
state. If image creation succeeds, the state is
* AVAILABLE
. If image creation fails, the state is FAILED
.
* @see ImageState
*/
public final ImageState state() {
return ImageState.fromValue(state);
}
/**
*
* The image starts in the PENDING
state. If image creation succeeds, the state is
* AVAILABLE
. If image creation fails, the state is FAILED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link ImageState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The image starts in the PENDING
state. If image creation succeeds, the state is
* AVAILABLE
. If image creation fails, the state is FAILED
.
* @see ImageState
*/
public final String stateAsString() {
return state;
}
/**
*
* Indicates whether the image is public or private.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #visibility} will
* return {@link VisibilityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #visibilityAsString}.
*
*
* @return Indicates whether the image is public or private.
* @see VisibilityType
*/
public final VisibilityType visibility() {
return VisibilityType.fromValue(visibility);
}
/**
*
* Indicates whether the image is public or private.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #visibility} will
* return {@link VisibilityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #visibilityAsString}.
*
*
* @return Indicates whether the image is public or private.
* @see VisibilityType
*/
public final String visibilityAsString() {
return visibility;
}
/**
*
* Indicates whether an image builder can be launched from this image.
*
*
* @return Indicates whether an image builder can be launched from this image.
*/
public final Boolean imageBuilderSupported() {
return imageBuilderSupported;
}
/**
*
* The name of the image builder that was used to create the private image. If the image is shared, this value is
* null.
*
*
* @return The name of the image builder that was used to create the private image. If the image is shared, this
* value is null.
*/
public final String imageBuilderName() {
return imageBuilderName;
}
/**
*
* The operating system platform of the image.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #platform} will
* return {@link PlatformType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #platformAsString}.
*
*
* @return The operating system platform of the image.
* @see PlatformType
*/
public final PlatformType platform() {
return PlatformType.fromValue(platform);
}
/**
*
* The operating system platform of the image.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #platform} will
* return {@link PlatformType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #platformAsString}.
*
*
* @return The operating system platform of the image.
* @see PlatformType
*/
public final String platformAsString() {
return platform;
}
/**
*
* The description to display.
*
*
* @return The description to display.
*/
public final String description() {
return description;
}
/**
*
* The reason why the last state change occurred.
*
*
* @return The reason why the last state change occurred.
*/
public final ImageStateChangeReason stateChangeReason() {
return stateChangeReason;
}
/**
* Returns true if the Applications property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasApplications() {
return applications != null && !(applications instanceof SdkAutoConstructList);
}
/**
*
* The applications associated with the image.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasApplications()} to see if a value was sent in this field.
*
*
* @return The applications associated with the image.
*/
public final List applications() {
return applications;
}
/**
*
* The time the image was created.
*
*
* @return The time the image was created.
*/
public final Instant createdTime() {
return createdTime;
}
/**
*
* The release date of the public base image. For private images, this date is the release date of the base image
* from which the image was created.
*
*
* @return The release date of the public base image. For private images, this date is the release date of the base
* image from which the image was created.
*/
public final Instant publicBaseImageReleasedDate() {
return publicBaseImageReleasedDate;
}
/**
*
* The version of the AppStream 2.0 agent to use for instances that are launched from this image.
*
*
* @return The version of the AppStream 2.0 agent to use for instances that are launched from this image.
*/
public final String appstreamAgentVersion() {
return appstreamAgentVersion;
}
/**
*
* The permissions to provide to the destination AWS account for the specified image.
*
*
* @return The permissions to provide to the destination AWS account for the specified image.
*/
public final ImagePermissions imagePermissions() {
return imagePermissions;
}
/**
* Returns true if the ImageErrors property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasImageErrors() {
return imageErrors != null && !(imageErrors instanceof SdkAutoConstructList);
}
/**
*
* Describes the errors that are returned when a new image can't be created.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasImageErrors()} to see if a value was sent in this field.
*
*
* @return Describes the errors that are returned when a new image can't be created.
*/
public final List imageErrors() {
return imageErrors;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(baseImageArn());
hashCode = 31 * hashCode + Objects.hashCode(displayName());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(visibilityAsString());
hashCode = 31 * hashCode + Objects.hashCode(imageBuilderSupported());
hashCode = 31 * hashCode + Objects.hashCode(imageBuilderName());
hashCode = 31 * hashCode + Objects.hashCode(platformAsString());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(stateChangeReason());
hashCode = 31 * hashCode + Objects.hashCode(hasApplications() ? applications() : null);
hashCode = 31 * hashCode + Objects.hashCode(createdTime());
hashCode = 31 * hashCode + Objects.hashCode(publicBaseImageReleasedDate());
hashCode = 31 * hashCode + Objects.hashCode(appstreamAgentVersion());
hashCode = 31 * hashCode + Objects.hashCode(imagePermissions());
hashCode = 31 * hashCode + Objects.hashCode(hasImageErrors() ? imageErrors() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Image)) {
return false;
}
Image other = (Image) obj;
return Objects.equals(name(), other.name()) && Objects.equals(arn(), other.arn())
&& Objects.equals(baseImageArn(), other.baseImageArn()) && Objects.equals(displayName(), other.displayName())
&& Objects.equals(stateAsString(), other.stateAsString())
&& Objects.equals(visibilityAsString(), other.visibilityAsString())
&& Objects.equals(imageBuilderSupported(), other.imageBuilderSupported())
&& Objects.equals(imageBuilderName(), other.imageBuilderName())
&& Objects.equals(platformAsString(), other.platformAsString())
&& Objects.equals(description(), other.description())
&& Objects.equals(stateChangeReason(), other.stateChangeReason()) && hasApplications() == other.hasApplications()
&& Objects.equals(applications(), other.applications()) && Objects.equals(createdTime(), other.createdTime())
&& Objects.equals(publicBaseImageReleasedDate(), other.publicBaseImageReleasedDate())
&& Objects.equals(appstreamAgentVersion(), other.appstreamAgentVersion())
&& Objects.equals(imagePermissions(), other.imagePermissions()) && hasImageErrors() == other.hasImageErrors()
&& Objects.equals(imageErrors(), other.imageErrors());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Image").add("Name", name()).add("Arn", arn()).add("BaseImageArn", baseImageArn())
.add("DisplayName", displayName()).add("State", stateAsString()).add("Visibility", visibilityAsString())
.add("ImageBuilderSupported", imageBuilderSupported()).add("ImageBuilderName", imageBuilderName())
.add("Platform", platformAsString()).add("Description", description())
.add("StateChangeReason", stateChangeReason()).add("Applications", hasApplications() ? applications() : null)
.add("CreatedTime", createdTime()).add("PublicBaseImageReleasedDate", publicBaseImageReleasedDate())
.add("AppstreamAgentVersion", appstreamAgentVersion()).add("ImagePermissions", imagePermissions())
.add("ImageErrors", hasImageErrors() ? imageErrors() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "BaseImageArn":
return Optional.ofNullable(clazz.cast(baseImageArn()));
case "DisplayName":
return Optional.ofNullable(clazz.cast(displayName()));
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "Visibility":
return Optional.ofNullable(clazz.cast(visibilityAsString()));
case "ImageBuilderSupported":
return Optional.ofNullable(clazz.cast(imageBuilderSupported()));
case "ImageBuilderName":
return Optional.ofNullable(clazz.cast(imageBuilderName()));
case "Platform":
return Optional.ofNullable(clazz.cast(platformAsString()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "StateChangeReason":
return Optional.ofNullable(clazz.cast(stateChangeReason()));
case "Applications":
return Optional.ofNullable(clazz.cast(applications()));
case "CreatedTime":
return Optional.ofNullable(clazz.cast(createdTime()));
case "PublicBaseImageReleasedDate":
return Optional.ofNullable(clazz.cast(publicBaseImageReleasedDate()));
case "AppstreamAgentVersion":
return Optional.ofNullable(clazz.cast(appstreamAgentVersion()));
case "ImagePermissions":
return Optional.ofNullable(clazz.cast(imagePermissions()));
case "ImageErrors":
return Optional.ofNullable(clazz.cast(imageErrors()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function