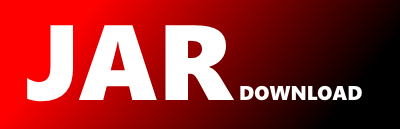
software.amazon.awssdk.services.appstream.DefaultAppStreamClient Maven / Gradle / Ivy
Show all versions of appstream Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.appstream;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.appstream.internal.AppStreamServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.appstream.model.AppStreamException;
import software.amazon.awssdk.services.appstream.model.AssociateAppBlockBuilderAppBlockRequest;
import software.amazon.awssdk.services.appstream.model.AssociateAppBlockBuilderAppBlockResponse;
import software.amazon.awssdk.services.appstream.model.AssociateApplicationFleetRequest;
import software.amazon.awssdk.services.appstream.model.AssociateApplicationFleetResponse;
import software.amazon.awssdk.services.appstream.model.AssociateApplicationToEntitlementRequest;
import software.amazon.awssdk.services.appstream.model.AssociateApplicationToEntitlementResponse;
import software.amazon.awssdk.services.appstream.model.AssociateFleetRequest;
import software.amazon.awssdk.services.appstream.model.AssociateFleetResponse;
import software.amazon.awssdk.services.appstream.model.BatchAssociateUserStackRequest;
import software.amazon.awssdk.services.appstream.model.BatchAssociateUserStackResponse;
import software.amazon.awssdk.services.appstream.model.BatchDisassociateUserStackRequest;
import software.amazon.awssdk.services.appstream.model.BatchDisassociateUserStackResponse;
import software.amazon.awssdk.services.appstream.model.ConcurrentModificationException;
import software.amazon.awssdk.services.appstream.model.CopyImageRequest;
import software.amazon.awssdk.services.appstream.model.CopyImageResponse;
import software.amazon.awssdk.services.appstream.model.CreateAppBlockBuilderRequest;
import software.amazon.awssdk.services.appstream.model.CreateAppBlockBuilderResponse;
import software.amazon.awssdk.services.appstream.model.CreateAppBlockBuilderStreamingUrlRequest;
import software.amazon.awssdk.services.appstream.model.CreateAppBlockBuilderStreamingUrlResponse;
import software.amazon.awssdk.services.appstream.model.CreateAppBlockRequest;
import software.amazon.awssdk.services.appstream.model.CreateAppBlockResponse;
import software.amazon.awssdk.services.appstream.model.CreateApplicationRequest;
import software.amazon.awssdk.services.appstream.model.CreateApplicationResponse;
import software.amazon.awssdk.services.appstream.model.CreateDirectoryConfigRequest;
import software.amazon.awssdk.services.appstream.model.CreateDirectoryConfigResponse;
import software.amazon.awssdk.services.appstream.model.CreateEntitlementRequest;
import software.amazon.awssdk.services.appstream.model.CreateEntitlementResponse;
import software.amazon.awssdk.services.appstream.model.CreateFleetRequest;
import software.amazon.awssdk.services.appstream.model.CreateFleetResponse;
import software.amazon.awssdk.services.appstream.model.CreateImageBuilderRequest;
import software.amazon.awssdk.services.appstream.model.CreateImageBuilderResponse;
import software.amazon.awssdk.services.appstream.model.CreateImageBuilderStreamingUrlRequest;
import software.amazon.awssdk.services.appstream.model.CreateImageBuilderStreamingUrlResponse;
import software.amazon.awssdk.services.appstream.model.CreateStackRequest;
import software.amazon.awssdk.services.appstream.model.CreateStackResponse;
import software.amazon.awssdk.services.appstream.model.CreateStreamingUrlRequest;
import software.amazon.awssdk.services.appstream.model.CreateStreamingUrlResponse;
import software.amazon.awssdk.services.appstream.model.CreateThemeForStackRequest;
import software.amazon.awssdk.services.appstream.model.CreateThemeForStackResponse;
import software.amazon.awssdk.services.appstream.model.CreateUpdatedImageRequest;
import software.amazon.awssdk.services.appstream.model.CreateUpdatedImageResponse;
import software.amazon.awssdk.services.appstream.model.CreateUsageReportSubscriptionRequest;
import software.amazon.awssdk.services.appstream.model.CreateUsageReportSubscriptionResponse;
import software.amazon.awssdk.services.appstream.model.CreateUserRequest;
import software.amazon.awssdk.services.appstream.model.CreateUserResponse;
import software.amazon.awssdk.services.appstream.model.DeleteAppBlockBuilderRequest;
import software.amazon.awssdk.services.appstream.model.DeleteAppBlockBuilderResponse;
import software.amazon.awssdk.services.appstream.model.DeleteAppBlockRequest;
import software.amazon.awssdk.services.appstream.model.DeleteAppBlockResponse;
import software.amazon.awssdk.services.appstream.model.DeleteApplicationRequest;
import software.amazon.awssdk.services.appstream.model.DeleteApplicationResponse;
import software.amazon.awssdk.services.appstream.model.DeleteDirectoryConfigRequest;
import software.amazon.awssdk.services.appstream.model.DeleteDirectoryConfigResponse;
import software.amazon.awssdk.services.appstream.model.DeleteEntitlementRequest;
import software.amazon.awssdk.services.appstream.model.DeleteEntitlementResponse;
import software.amazon.awssdk.services.appstream.model.DeleteFleetRequest;
import software.amazon.awssdk.services.appstream.model.DeleteFleetResponse;
import software.amazon.awssdk.services.appstream.model.DeleteImageBuilderRequest;
import software.amazon.awssdk.services.appstream.model.DeleteImageBuilderResponse;
import software.amazon.awssdk.services.appstream.model.DeleteImagePermissionsRequest;
import software.amazon.awssdk.services.appstream.model.DeleteImagePermissionsResponse;
import software.amazon.awssdk.services.appstream.model.DeleteImageRequest;
import software.amazon.awssdk.services.appstream.model.DeleteImageResponse;
import software.amazon.awssdk.services.appstream.model.DeleteStackRequest;
import software.amazon.awssdk.services.appstream.model.DeleteStackResponse;
import software.amazon.awssdk.services.appstream.model.DeleteThemeForStackRequest;
import software.amazon.awssdk.services.appstream.model.DeleteThemeForStackResponse;
import software.amazon.awssdk.services.appstream.model.DeleteUsageReportSubscriptionRequest;
import software.amazon.awssdk.services.appstream.model.DeleteUsageReportSubscriptionResponse;
import software.amazon.awssdk.services.appstream.model.DeleteUserRequest;
import software.amazon.awssdk.services.appstream.model.DeleteUserResponse;
import software.amazon.awssdk.services.appstream.model.DescribeAppBlockBuilderAppBlockAssociationsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeAppBlockBuilderAppBlockAssociationsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeAppBlockBuildersRequest;
import software.amazon.awssdk.services.appstream.model.DescribeAppBlockBuildersResponse;
import software.amazon.awssdk.services.appstream.model.DescribeAppBlocksRequest;
import software.amazon.awssdk.services.appstream.model.DescribeAppBlocksResponse;
import software.amazon.awssdk.services.appstream.model.DescribeApplicationFleetAssociationsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeApplicationFleetAssociationsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeApplicationsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeApplicationsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeDirectoryConfigsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeDirectoryConfigsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeEntitlementsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeEntitlementsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeFleetsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeFleetsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeImageBuildersRequest;
import software.amazon.awssdk.services.appstream.model.DescribeImageBuildersResponse;
import software.amazon.awssdk.services.appstream.model.DescribeImagePermissionsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeImagePermissionsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeImagesRequest;
import software.amazon.awssdk.services.appstream.model.DescribeImagesResponse;
import software.amazon.awssdk.services.appstream.model.DescribeSessionsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeSessionsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeStacksRequest;
import software.amazon.awssdk.services.appstream.model.DescribeStacksResponse;
import software.amazon.awssdk.services.appstream.model.DescribeThemeForStackRequest;
import software.amazon.awssdk.services.appstream.model.DescribeThemeForStackResponse;
import software.amazon.awssdk.services.appstream.model.DescribeUsageReportSubscriptionsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeUsageReportSubscriptionsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeUserStackAssociationsRequest;
import software.amazon.awssdk.services.appstream.model.DescribeUserStackAssociationsResponse;
import software.amazon.awssdk.services.appstream.model.DescribeUsersRequest;
import software.amazon.awssdk.services.appstream.model.DescribeUsersResponse;
import software.amazon.awssdk.services.appstream.model.DisableUserRequest;
import software.amazon.awssdk.services.appstream.model.DisableUserResponse;
import software.amazon.awssdk.services.appstream.model.DisassociateAppBlockBuilderAppBlockRequest;
import software.amazon.awssdk.services.appstream.model.DisassociateAppBlockBuilderAppBlockResponse;
import software.amazon.awssdk.services.appstream.model.DisassociateApplicationFleetRequest;
import software.amazon.awssdk.services.appstream.model.DisassociateApplicationFleetResponse;
import software.amazon.awssdk.services.appstream.model.DisassociateApplicationFromEntitlementRequest;
import software.amazon.awssdk.services.appstream.model.DisassociateApplicationFromEntitlementResponse;
import software.amazon.awssdk.services.appstream.model.DisassociateFleetRequest;
import software.amazon.awssdk.services.appstream.model.DisassociateFleetResponse;
import software.amazon.awssdk.services.appstream.model.EnableUserRequest;
import software.amazon.awssdk.services.appstream.model.EnableUserResponse;
import software.amazon.awssdk.services.appstream.model.EntitlementAlreadyExistsException;
import software.amazon.awssdk.services.appstream.model.EntitlementNotFoundException;
import software.amazon.awssdk.services.appstream.model.ExpireSessionRequest;
import software.amazon.awssdk.services.appstream.model.ExpireSessionResponse;
import software.amazon.awssdk.services.appstream.model.IncompatibleImageException;
import software.amazon.awssdk.services.appstream.model.InvalidAccountStatusException;
import software.amazon.awssdk.services.appstream.model.InvalidParameterCombinationException;
import software.amazon.awssdk.services.appstream.model.InvalidRoleException;
import software.amazon.awssdk.services.appstream.model.LimitExceededException;
import software.amazon.awssdk.services.appstream.model.ListAssociatedFleetsRequest;
import software.amazon.awssdk.services.appstream.model.ListAssociatedFleetsResponse;
import software.amazon.awssdk.services.appstream.model.ListAssociatedStacksRequest;
import software.amazon.awssdk.services.appstream.model.ListAssociatedStacksResponse;
import software.amazon.awssdk.services.appstream.model.ListEntitledApplicationsRequest;
import software.amazon.awssdk.services.appstream.model.ListEntitledApplicationsResponse;
import software.amazon.awssdk.services.appstream.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.appstream.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.appstream.model.OperationNotPermittedException;
import software.amazon.awssdk.services.appstream.model.RequestLimitExceededException;
import software.amazon.awssdk.services.appstream.model.ResourceAlreadyExistsException;
import software.amazon.awssdk.services.appstream.model.ResourceInUseException;
import software.amazon.awssdk.services.appstream.model.ResourceNotAvailableException;
import software.amazon.awssdk.services.appstream.model.ResourceNotFoundException;
import software.amazon.awssdk.services.appstream.model.StartAppBlockBuilderRequest;
import software.amazon.awssdk.services.appstream.model.StartAppBlockBuilderResponse;
import software.amazon.awssdk.services.appstream.model.StartFleetRequest;
import software.amazon.awssdk.services.appstream.model.StartFleetResponse;
import software.amazon.awssdk.services.appstream.model.StartImageBuilderRequest;
import software.amazon.awssdk.services.appstream.model.StartImageBuilderResponse;
import software.amazon.awssdk.services.appstream.model.StopAppBlockBuilderRequest;
import software.amazon.awssdk.services.appstream.model.StopAppBlockBuilderResponse;
import software.amazon.awssdk.services.appstream.model.StopFleetRequest;
import software.amazon.awssdk.services.appstream.model.StopFleetResponse;
import software.amazon.awssdk.services.appstream.model.StopImageBuilderRequest;
import software.amazon.awssdk.services.appstream.model.StopImageBuilderResponse;
import software.amazon.awssdk.services.appstream.model.TagResourceRequest;
import software.amazon.awssdk.services.appstream.model.TagResourceResponse;
import software.amazon.awssdk.services.appstream.model.UntagResourceRequest;
import software.amazon.awssdk.services.appstream.model.UntagResourceResponse;
import software.amazon.awssdk.services.appstream.model.UpdateAppBlockBuilderRequest;
import software.amazon.awssdk.services.appstream.model.UpdateAppBlockBuilderResponse;
import software.amazon.awssdk.services.appstream.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.appstream.model.UpdateApplicationResponse;
import software.amazon.awssdk.services.appstream.model.UpdateDirectoryConfigRequest;
import software.amazon.awssdk.services.appstream.model.UpdateDirectoryConfigResponse;
import software.amazon.awssdk.services.appstream.model.UpdateEntitlementRequest;
import software.amazon.awssdk.services.appstream.model.UpdateEntitlementResponse;
import software.amazon.awssdk.services.appstream.model.UpdateFleetRequest;
import software.amazon.awssdk.services.appstream.model.UpdateFleetResponse;
import software.amazon.awssdk.services.appstream.model.UpdateImagePermissionsRequest;
import software.amazon.awssdk.services.appstream.model.UpdateImagePermissionsResponse;
import software.amazon.awssdk.services.appstream.model.UpdateStackRequest;
import software.amazon.awssdk.services.appstream.model.UpdateStackResponse;
import software.amazon.awssdk.services.appstream.model.UpdateThemeForStackRequest;
import software.amazon.awssdk.services.appstream.model.UpdateThemeForStackResponse;
import software.amazon.awssdk.services.appstream.transform.AssociateAppBlockBuilderAppBlockRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.AssociateApplicationFleetRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.AssociateApplicationToEntitlementRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.AssociateFleetRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.BatchAssociateUserStackRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.BatchDisassociateUserStackRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CopyImageRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateAppBlockBuilderRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateAppBlockBuilderStreamingUrlRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateAppBlockRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateApplicationRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateDirectoryConfigRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateEntitlementRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateFleetRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateImageBuilderRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateImageBuilderStreamingUrlRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateStackRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateStreamingUrlRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateThemeForStackRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateUpdatedImageRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateUsageReportSubscriptionRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.CreateUserRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteAppBlockBuilderRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteAppBlockRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteApplicationRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteDirectoryConfigRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteEntitlementRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteFleetRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteImageBuilderRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteImagePermissionsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteImageRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteStackRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteThemeForStackRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteUsageReportSubscriptionRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DeleteUserRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeAppBlockBuilderAppBlockAssociationsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeAppBlockBuildersRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeAppBlocksRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeApplicationFleetAssociationsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeApplicationsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeDirectoryConfigsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeEntitlementsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeFleetsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeImageBuildersRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeImagePermissionsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeImagesRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeSessionsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeStacksRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeThemeForStackRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeUsageReportSubscriptionsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeUserStackAssociationsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DescribeUsersRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DisableUserRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DisassociateAppBlockBuilderAppBlockRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DisassociateApplicationFleetRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DisassociateApplicationFromEntitlementRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.DisassociateFleetRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.EnableUserRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.ExpireSessionRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.ListAssociatedFleetsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.ListAssociatedStacksRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.ListEntitledApplicationsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.StartAppBlockBuilderRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.StartFleetRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.StartImageBuilderRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.StopAppBlockBuilderRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.StopFleetRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.StopImageBuilderRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.UpdateAppBlockBuilderRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.UpdateApplicationRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.UpdateDirectoryConfigRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.UpdateEntitlementRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.UpdateFleetRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.UpdateImagePermissionsRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.UpdateStackRequestMarshaller;
import software.amazon.awssdk.services.appstream.transform.UpdateThemeForStackRequestMarshaller;
import software.amazon.awssdk.services.appstream.waiters.AppStreamWaiter;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link AppStreamClient}.
*
* @see AppStreamClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultAppStreamClient implements AppStreamClient {
private static final Logger log = Logger.loggerFor(DefaultAppStreamClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultAppStreamClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Associates the specified app block builder with the specified app block.
*
*
* @param associateAppBlockBuilderAppBlockRequest
* @return Result of the AssociateAppBlockBuilderAppBlock operation returned by the service.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.AssociateAppBlockBuilderAppBlock
* @see AWS API Documentation
*/
@Override
public AssociateAppBlockBuilderAppBlockResponse associateAppBlockBuilderAppBlock(
AssociateAppBlockBuilderAppBlockRequest associateAppBlockBuilderAppBlockRequest)
throws ConcurrentModificationException, LimitExceededException, ResourceNotFoundException,
InvalidParameterCombinationException, OperationNotPermittedException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateAppBlockBuilderAppBlockResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateAppBlockBuilderAppBlockRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
associateAppBlockBuilderAppBlockRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateAppBlockBuilderAppBlock");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateAppBlockBuilderAppBlock").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(associateAppBlockBuilderAppBlockRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateAppBlockBuilderAppBlockRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates the specified application with the specified fleet. This is only supported for Elastic fleets.
*
*
* @param associateApplicationFleetRequest
* @return Result of the AssociateApplicationFleet operation returned by the service.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.AssociateApplicationFleet
* @see AWS API Documentation
*/
@Override
public AssociateApplicationFleetResponse associateApplicationFleet(
AssociateApplicationFleetRequest associateApplicationFleetRequest) throws ConcurrentModificationException,
LimitExceededException, ResourceNotFoundException, InvalidParameterCombinationException,
OperationNotPermittedException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateApplicationFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateApplicationFleetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateApplicationFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateApplicationFleet");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateApplicationFleet").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(associateApplicationFleetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateApplicationFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates an application to entitle.
*
*
* @param associateApplicationToEntitlementRequest
* @return Result of the AssociateApplicationToEntitlement operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws EntitlementNotFoundException
* The entitlement can't be found.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.AssociateApplicationToEntitlement
* @see AWS API Documentation
*/
@Override
public AssociateApplicationToEntitlementResponse associateApplicationToEntitlement(
AssociateApplicationToEntitlementRequest associateApplicationToEntitlementRequest)
throws OperationNotPermittedException, ResourceNotFoundException, EntitlementNotFoundException,
LimitExceededException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateApplicationToEntitlementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateApplicationToEntitlementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
associateApplicationToEntitlementRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateApplicationToEntitlement");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateApplicationToEntitlement").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(associateApplicationToEntitlementRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateApplicationToEntitlementRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates the specified fleet with the specified stack.
*
*
* @param associateFleetRequest
* @return Result of the AssociateFleet operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.AssociateFleet
* @see AWS API
* Documentation
*/
@Override
public AssociateFleetResponse associateFleet(AssociateFleetRequest associateFleetRequest) throws LimitExceededException,
InvalidAccountStatusException, ResourceNotFoundException, ConcurrentModificationException,
IncompatibleImageException, OperationNotPermittedException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AssociateFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateFleetRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateFleet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AssociateFleet").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(associateFleetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates the specified users with the specified stacks. Users in a user pool cannot be assigned to stacks with
* fleets that are joined to an Active Directory domain.
*
*
* @param batchAssociateUserStackRequest
* @return Result of the BatchAssociateUserStack operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.BatchAssociateUserStack
* @see AWS API Documentation
*/
@Override
public BatchAssociateUserStackResponse batchAssociateUserStack(BatchAssociateUserStackRequest batchAssociateUserStackRequest)
throws OperationNotPermittedException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchAssociateUserStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchAssociateUserStackRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchAssociateUserStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchAssociateUserStack");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchAssociateUserStack").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(batchAssociateUserStackRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchAssociateUserStackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disassociates the specified users from the specified stacks.
*
*
* @param batchDisassociateUserStackRequest
* @return Result of the BatchDisassociateUserStack operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.BatchDisassociateUserStack
* @see AWS API Documentation
*/
@Override
public BatchDisassociateUserStackResponse batchDisassociateUserStack(
BatchDisassociateUserStackRequest batchDisassociateUserStackRequest) throws OperationNotPermittedException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchDisassociateUserStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchDisassociateUserStackRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDisassociateUserStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDisassociateUserStack");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchDisassociateUserStack").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(batchDisassociateUserStackRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchDisassociateUserStackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Copies the image within the same region or to a new region within the same AWS account. Note that any tags you
* added to the image will not be copied.
*
*
* @param copyImageRequest
* @return Result of the CopyImage operation returned by the service.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CopyImage
* @see AWS API
* Documentation
*/
@Override
public CopyImageResponse copyImage(CopyImageRequest copyImageRequest) throws ResourceAlreadyExistsException,
ResourceNotFoundException, ResourceNotAvailableException, LimitExceededException, InvalidAccountStatusException,
IncompatibleImageException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CopyImageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(copyImageRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, copyImageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CopyImage");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CopyImage").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(copyImageRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CopyImageRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates an app block.
*
*
* App blocks are an Amazon AppStream 2.0 resource that stores the details about the virtual hard disk in an S3
* bucket. It also stores the setup script with details about how to mount the virtual hard disk. The virtual hard
* disk includes the application binaries and other files necessary to launch your applications. Multiple
* applications can be assigned to a single app block.
*
*
* This is only supported for Elastic fleets.
*
*
* @param createAppBlockRequest
* @return Result of the CreateAppBlock operation returned by the service.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateAppBlock
* @see AWS API
* Documentation
*/
@Override
public CreateAppBlockResponse createAppBlock(CreateAppBlockRequest createAppBlockRequest)
throws ConcurrentModificationException, LimitExceededException, OperationNotPermittedException,
ResourceAlreadyExistsException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateAppBlockResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAppBlockRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAppBlockRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAppBlock");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateAppBlock").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createAppBlockRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateAppBlockRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates an app block builder.
*
*
* @param createAppBlockBuilderRequest
* @return Result of the CreateAppBlockBuilder operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws RequestLimitExceededException
* AppStream 2.0 can’t process the request right now because the Describe calls from your AWS account are
* being throttled by Amazon EC2. Try again later.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateAppBlockBuilder
* @see AWS API Documentation
*/
@Override
public CreateAppBlockBuilderResponse createAppBlockBuilder(CreateAppBlockBuilderRequest createAppBlockBuilderRequest)
throws LimitExceededException, RequestLimitExceededException, InvalidAccountStatusException, InvalidRoleException,
ConcurrentModificationException, OperationNotPermittedException, ResourceAlreadyExistsException,
ResourceNotAvailableException, ResourceNotFoundException, InvalidParameterCombinationException, AwsServiceException,
SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateAppBlockBuilderResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAppBlockBuilderRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAppBlockBuilderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAppBlockBuilder");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateAppBlockBuilder").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createAppBlockBuilderRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateAppBlockBuilderRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a URL to start a create app block builder streaming session.
*
*
* @param createAppBlockBuilderStreamingUrlRequest
* @return Result of the CreateAppBlockBuilderStreamingURL operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateAppBlockBuilderStreamingURL
* @see AWS API Documentation
*/
@Override
public CreateAppBlockBuilderStreamingUrlResponse createAppBlockBuilderStreamingURL(
CreateAppBlockBuilderStreamingUrlRequest createAppBlockBuilderStreamingUrlRequest) throws ResourceNotFoundException,
OperationNotPermittedException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateAppBlockBuilderStreamingUrlResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAppBlockBuilderStreamingUrlRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createAppBlockBuilderStreamingUrlRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAppBlockBuilderStreamingURL");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAppBlockBuilderStreamingURL").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createAppBlockBuilderStreamingUrlRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateAppBlockBuilderStreamingUrlRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates an application.
*
*
* Applications are an Amazon AppStream 2.0 resource that stores the details about how to launch applications on
* Elastic fleet streaming instances. An application consists of the launch details, icon, and display name.
* Applications are associated with an app block that contains the application binaries and other files. The
* applications assigned to an Elastic fleet are the applications users can launch.
*
*
* This is only supported for Elastic fleets.
*
*
* @param createApplicationRequest
* @return Result of the CreateApplication operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateApplication
* @see AWS
* API Documentation
*/
@Override
public CreateApplicationResponse createApplication(CreateApplicationRequest createApplicationRequest)
throws OperationNotPermittedException, ResourceAlreadyExistsException, LimitExceededException,
ConcurrentModificationException, ResourceNotFoundException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateApplication");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateApplication").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createApplicationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateApplicationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a Directory Config object in AppStream 2.0. This object includes the configuration information required
* to join fleets and image builders to Microsoft Active Directory domains.
*
*
* @param createDirectoryConfigRequest
* @return Result of the CreateDirectoryConfig operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateDirectoryConfig
* @see AWS API Documentation
*/
@Override
public CreateDirectoryConfigResponse createDirectoryConfig(CreateDirectoryConfigRequest createDirectoryConfigRequest)
throws ResourceNotFoundException, ResourceAlreadyExistsException, LimitExceededException,
InvalidAccountStatusException, OperationNotPermittedException, InvalidRoleException, AwsServiceException,
SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDirectoryConfigResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDirectoryConfigRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDirectoryConfigRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDirectoryConfig");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateDirectoryConfig").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createDirectoryConfigRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateDirectoryConfigRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new entitlement. Entitlements control access to specific applications within a stack, based on user
* attributes. Entitlements apply to SAML 2.0 federated user identities. Amazon AppStream 2.0 user pool and
* streaming URL users are entitled to all applications in a stack. Entitlements don't apply to the desktop stream
* view application, or to applications managed by a dynamic app provider using the Dynamic Application Framework.
*
*
* @param createEntitlementRequest
* @return Result of the CreateEntitlement operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws EntitlementAlreadyExistsException
* The entitlement already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateEntitlement
* @see AWS
* API Documentation
*/
@Override
public CreateEntitlementResponse createEntitlement(CreateEntitlementRequest createEntitlementRequest)
throws OperationNotPermittedException, ResourceNotFoundException, LimitExceededException,
EntitlementAlreadyExistsException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateEntitlementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createEntitlementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createEntitlementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateEntitlement");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateEntitlement").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createEntitlementRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateEntitlementRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a fleet. A fleet consists of streaming instances that your users access for their applications and
* desktops.
*
*
* @param createFleetRequest
* @return Result of the CreateFleet operation returned by the service.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws RequestLimitExceededException
* AppStream 2.0 can’t process the request right now because the Describe calls from your AWS account are
* being throttled by Amazon EC2. Try again later.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateFleet
* @see AWS API
* Documentation
*/
@Override
public CreateFleetResponse createFleet(CreateFleetRequest createFleetRequest) throws ResourceAlreadyExistsException,
ResourceNotAvailableException, ResourceNotFoundException, LimitExceededException, RequestLimitExceededException,
InvalidAccountStatusException, InvalidRoleException, ConcurrentModificationException,
InvalidParameterCombinationException, IncompatibleImageException, OperationNotPermittedException,
AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createFleetRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateFleet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateFleet").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(createFleetRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates an image builder. An image builder is a virtual machine that is used to create an image.
*
*
* The initial state of the builder is PENDING
. When it is ready, the state is RUNNING
.
*
*
* @param createImageBuilderRequest
* @return Result of the CreateImageBuilder operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws RequestLimitExceededException
* AppStream 2.0 can’t process the request right now because the Describe calls from your AWS account are
* being throttled by Amazon EC2. Try again later.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateImageBuilder
* @see AWS
* API Documentation
*/
@Override
public CreateImageBuilderResponse createImageBuilder(CreateImageBuilderRequest createImageBuilderRequest)
throws LimitExceededException, RequestLimitExceededException, InvalidAccountStatusException,
ResourceAlreadyExistsException, ResourceNotAvailableException, ResourceNotFoundException, InvalidRoleException,
ConcurrentModificationException, InvalidParameterCombinationException, IncompatibleImageException,
OperationNotPermittedException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateImageBuilderResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createImageBuilderRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createImageBuilderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateImageBuilder");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateImageBuilder").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createImageBuilderRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateImageBuilderRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a URL to start an image builder streaming session.
*
*
* @param createImageBuilderStreamingUrlRequest
* @return Result of the CreateImageBuilderStreamingURL operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateImageBuilderStreamingURL
* @see AWS API Documentation
*/
@Override
public CreateImageBuilderStreamingUrlResponse createImageBuilderStreamingURL(
CreateImageBuilderStreamingUrlRequest createImageBuilderStreamingUrlRequest) throws OperationNotPermittedException,
ResourceNotFoundException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateImageBuilderStreamingUrlResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createImageBuilderStreamingUrlRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createImageBuilderStreamingUrlRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateImageBuilderStreamingURL");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateImageBuilderStreamingURL").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createImageBuilderStreamingUrlRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateImageBuilderStreamingUrlRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a stack to start streaming applications to users. A stack consists of an associated fleet, user access
* policies, and storage configurations.
*
*
* @param createStackRequest
* @return Result of the CreateStack operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateStack
* @see AWS API
* Documentation
*/
@Override
public CreateStackResponse createStack(CreateStackRequest createStackRequest) throws LimitExceededException,
InvalidAccountStatusException, ResourceAlreadyExistsException, ConcurrentModificationException, InvalidRoleException,
ResourceNotFoundException, InvalidParameterCombinationException, OperationNotPermittedException, AwsServiceException,
SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createStackRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateStack");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateStack").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(createStackRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateStackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a temporary URL to start an AppStream 2.0 streaming session for the specified user. A streaming URL
* enables application streaming to be tested without user setup.
*
*
* @param createStreamingUrlRequest
* @return Result of the CreateStreamingURL operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateStreamingURL
* @see AWS
* API Documentation
*/
@Override
public CreateStreamingUrlResponse createStreamingURL(CreateStreamingUrlRequest createStreamingUrlRequest)
throws ResourceNotFoundException, ResourceNotAvailableException, OperationNotPermittedException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateStreamingUrlResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createStreamingUrlRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createStreamingUrlRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateStreamingURL");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateStreamingURL").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createStreamingUrlRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateStreamingUrlRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates custom branding that customizes the appearance of the streaming application catalog page.
*
*
* @param createThemeForStackRequest
* @return Result of the CreateThemeForStack operation returned by the service.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateThemeForStack
* @see AWS
* API Documentation
*/
@Override
public CreateThemeForStackResponse createThemeForStack(CreateThemeForStackRequest createThemeForStackRequest)
throws ConcurrentModificationException, ResourceNotFoundException, ResourceAlreadyExistsException,
LimitExceededException, InvalidAccountStatusException, OperationNotPermittedException, AwsServiceException,
SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateThemeForStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createThemeForStackRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createThemeForStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateThemeForStack");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateThemeForStack").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createThemeForStackRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateThemeForStackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new image with the latest Windows operating system updates, driver updates, and AppStream 2.0 agent
* software.
*
*
* For more information, see the "Update an Image by Using Managed AppStream 2.0 Image Updates" section in Administer Your
* AppStream 2.0 Images, in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param createUpdatedImageRequest
* @return Result of the CreateUpdatedImage operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateUpdatedImage
* @see AWS
* API Documentation
*/
@Override
public CreateUpdatedImageResponse createUpdatedImage(CreateUpdatedImageRequest createUpdatedImageRequest)
throws LimitExceededException, InvalidAccountStatusException, OperationNotPermittedException,
ResourceAlreadyExistsException, ResourceNotFoundException, ConcurrentModificationException,
IncompatibleImageException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateUpdatedImageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createUpdatedImageRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUpdatedImageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUpdatedImage");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateUpdatedImage").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createUpdatedImageRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateUpdatedImageRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a usage report subscription. Usage reports are generated daily.
*
*
* @param createUsageReportSubscriptionRequest
* @return Result of the CreateUsageReportSubscription operation returned by the service.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateUsageReportSubscription
* @see AWS API Documentation
*/
@Override
public CreateUsageReportSubscriptionResponse createUsageReportSubscription(
CreateUsageReportSubscriptionRequest createUsageReportSubscriptionRequest) throws InvalidRoleException,
InvalidAccountStatusException, LimitExceededException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateUsageReportSubscriptionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createUsageReportSubscriptionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createUsageReportSubscriptionRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUsageReportSubscription");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateUsageReportSubscription").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createUsageReportSubscriptionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateUsageReportSubscriptionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new user in the user pool.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.CreateUser
* @see AWS API
* Documentation
*/
@Override
public CreateUserResponse createUser(CreateUserRequest createUserRequest) throws ResourceAlreadyExistsException,
InvalidAccountStatusException, InvalidParameterCombinationException, LimitExceededException,
OperationNotPermittedException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createUserRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUser");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateUser").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(createUserRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateUserRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes an app block.
*
*
* @param deleteAppBlockRequest
* @return Result of the DeleteAppBlock operation returned by the service.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteAppBlock
* @see AWS API
* Documentation
*/
@Override
public DeleteAppBlockResponse deleteAppBlock(DeleteAppBlockRequest deleteAppBlockRequest)
throws ConcurrentModificationException, ResourceInUseException, ResourceNotFoundException, AwsServiceException,
SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteAppBlockResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAppBlockRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAppBlockRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAppBlock");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteAppBlock").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteAppBlockRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteAppBlockRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes an app block builder.
*
*
* An app block builder can only be deleted when it has no association with an app block.
*
*
* @param deleteAppBlockBuilderRequest
* @return Result of the DeleteAppBlockBuilder operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteAppBlockBuilder
* @see AWS API Documentation
*/
@Override
public DeleteAppBlockBuilderResponse deleteAppBlockBuilder(DeleteAppBlockBuilderRequest deleteAppBlockBuilderRequest)
throws OperationNotPermittedException, ConcurrentModificationException, ResourceInUseException,
ResourceNotFoundException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAppBlockBuilderResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAppBlockBuilderRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAppBlockBuilderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAppBlockBuilder");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteAppBlockBuilder").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteAppBlockBuilderRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteAppBlockBuilderRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes an application.
*
*
* @param deleteApplicationRequest
* @return Result of the DeleteApplication operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteApplication
* @see AWS
* API Documentation
*/
@Override
public DeleteApplicationResponse deleteApplication(DeleteApplicationRequest deleteApplicationRequest)
throws OperationNotPermittedException, ResourceInUseException, ResourceNotFoundException,
ConcurrentModificationException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApplication");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteApplication").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteApplicationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteApplicationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified Directory Config object from AppStream 2.0. This object includes the information required
* to join streaming instances to an Active Directory domain.
*
*
* @param deleteDirectoryConfigRequest
* @return Result of the DeleteDirectoryConfig operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteDirectoryConfig
* @see AWS API Documentation
*/
@Override
public DeleteDirectoryConfigResponse deleteDirectoryConfig(DeleteDirectoryConfigRequest deleteDirectoryConfigRequest)
throws ResourceInUseException, ResourceNotFoundException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteDirectoryConfigResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteDirectoryConfigRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteDirectoryConfigRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDirectoryConfig");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteDirectoryConfig").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteDirectoryConfigRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteDirectoryConfigRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified entitlement.
*
*
* @param deleteEntitlementRequest
* @return Result of the DeleteEntitlement operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws EntitlementNotFoundException
* The entitlement can't be found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteEntitlement
* @see AWS
* API Documentation
*/
@Override
public DeleteEntitlementResponse deleteEntitlement(DeleteEntitlementRequest deleteEntitlementRequest)
throws OperationNotPermittedException, ResourceNotFoundException, EntitlementNotFoundException,
ConcurrentModificationException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteEntitlementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteEntitlementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteEntitlementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteEntitlement");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteEntitlement").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteEntitlementRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteEntitlementRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified fleet.
*
*
* @param deleteFleetRequest
* @return Result of the DeleteFleet operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteFleet
* @see AWS API
* Documentation
*/
@Override
public DeleteFleetResponse deleteFleet(DeleteFleetRequest deleteFleetRequest) throws ResourceInUseException,
ResourceNotFoundException, ConcurrentModificationException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteFleetRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteFleet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteFleet").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(deleteFleetRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified image. You cannot delete an image when it is in use. After you delete an image, you cannot
* provision new capacity using the image.
*
*
* @param deleteImageRequest
* @return Result of the DeleteImage operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteImage
* @see AWS API
* Documentation
*/
@Override
public DeleteImageResponse deleteImage(DeleteImageRequest deleteImageRequest) throws ResourceInUseException,
ResourceNotFoundException, OperationNotPermittedException, ConcurrentModificationException, AwsServiceException,
SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteImageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteImageRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteImageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteImage");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteImage").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(deleteImageRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteImageRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified image builder and releases the capacity.
*
*
* @param deleteImageBuilderRequest
* @return Result of the DeleteImageBuilder operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteImageBuilder
* @see AWS
* API Documentation
*/
@Override
public DeleteImageBuilderResponse deleteImageBuilder(DeleteImageBuilderRequest deleteImageBuilderRequest)
throws ResourceNotFoundException, OperationNotPermittedException, ConcurrentModificationException,
AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteImageBuilderResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteImageBuilderRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteImageBuilderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteImageBuilder");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteImageBuilder").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteImageBuilderRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteImageBuilderRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes permissions for the specified private image. After you delete permissions for an image, AWS accounts to
* which you previously granted these permissions can no longer use the image.
*
*
* @param deleteImagePermissionsRequest
* @return Result of the DeleteImagePermissions operation returned by the service.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteImagePermissions
* @see AWS API Documentation
*/
@Override
public DeleteImagePermissionsResponse deleteImagePermissions(DeleteImagePermissionsRequest deleteImagePermissionsRequest)
throws ResourceNotAvailableException, ResourceNotFoundException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteImagePermissionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteImagePermissionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteImagePermissionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteImagePermissions");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteImagePermissions").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteImagePermissionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteImagePermissionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified stack. After the stack is deleted, the application streaming environment provided by the
* stack is no longer available to users. Also, any reservations made for application streaming sessions for the
* stack are released.
*
*
* @param deleteStackRequest
* @return Result of the DeleteStack operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteStack
* @see AWS API
* Documentation
*/
@Override
public DeleteStackResponse deleteStack(DeleteStackRequest deleteStackRequest) throws ResourceInUseException,
ResourceNotFoundException, OperationNotPermittedException, ConcurrentModificationException, AwsServiceException,
SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteStackRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteStack");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteStack").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(deleteStackRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteStackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes custom branding that customizes the appearance of the streaming application catalog page.
*
*
* @param deleteThemeForStackRequest
* @return Result of the DeleteThemeForStack operation returned by the service.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteThemeForStack
* @see AWS
* API Documentation
*/
@Override
public DeleteThemeForStackResponse deleteThemeForStack(DeleteThemeForStackRequest deleteThemeForStackRequest)
throws ConcurrentModificationException, ResourceNotFoundException, OperationNotPermittedException,
AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteThemeForStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteThemeForStackRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteThemeForStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteThemeForStack");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteThemeForStack").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteThemeForStackRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteThemeForStackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disables usage report generation.
*
*
* @param deleteUsageReportSubscriptionRequest
* @return Result of the DeleteUsageReportSubscription operation returned by the service.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteUsageReportSubscription
* @see AWS API Documentation
*/
@Override
public DeleteUsageReportSubscriptionResponse deleteUsageReportSubscription(
DeleteUsageReportSubscriptionRequest deleteUsageReportSubscriptionRequest) throws InvalidAccountStatusException,
ResourceNotFoundException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteUsageReportSubscriptionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteUsageReportSubscriptionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteUsageReportSubscriptionRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUsageReportSubscription");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteUsageReportSubscription").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteUsageReportSubscriptionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteUsageReportSubscriptionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a user from the user pool.
*
*
* @param deleteUserRequest
* @return Result of the DeleteUser operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DeleteUser
* @see AWS API
* Documentation
*/
@Override
public DeleteUserResponse deleteUser(DeleteUserRequest deleteUserRequest) throws ResourceNotFoundException,
AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteUserRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUser");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteUser").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(deleteUserRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteUserRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one or more app block builder associations.
*
*
* @param describeAppBlockBuilderAppBlockAssociationsRequest
* @return Result of the DescribeAppBlockBuilderAppBlockAssociations operation returned by the service.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeAppBlockBuilderAppBlockAssociations
* @see AWS API Documentation
*/
@Override
public DescribeAppBlockBuilderAppBlockAssociationsResponse describeAppBlockBuilderAppBlockAssociations(
DescribeAppBlockBuilderAppBlockAssociationsRequest describeAppBlockBuilderAppBlockAssociationsRequest)
throws InvalidParameterCombinationException, OperationNotPermittedException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DescribeAppBlockBuilderAppBlockAssociationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(
describeAppBlockBuilderAppBlockAssociationsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeAppBlockBuilderAppBlockAssociationsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAppBlockBuilderAppBlockAssociations");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeAppBlockBuilderAppBlockAssociations")
.withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(describeAppBlockBuilderAppBlockAssociationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeAppBlockBuilderAppBlockAssociationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one or more app block builders.
*
*
* @param describeAppBlockBuildersRequest
* @return Result of the DescribeAppBlockBuilders operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeAppBlockBuilders
* @see AWS API Documentation
*/
@Override
public DescribeAppBlockBuildersResponse describeAppBlockBuilders(
DescribeAppBlockBuildersRequest describeAppBlockBuildersRequest) throws OperationNotPermittedException,
ResourceNotFoundException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeAppBlockBuildersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeAppBlockBuildersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAppBlockBuildersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAppBlockBuilders");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeAppBlockBuilders").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeAppBlockBuildersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeAppBlockBuildersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one or more app blocks.
*
*
* @param describeAppBlocksRequest
* @return Result of the DescribeAppBlocks operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeAppBlocks
* @see AWS
* API Documentation
*/
@Override
public DescribeAppBlocksResponse describeAppBlocks(DescribeAppBlocksRequest describeAppBlocksRequest)
throws OperationNotPermittedException, ResourceNotFoundException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeAppBlocksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeAppBlocksRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAppBlocksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAppBlocks");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeAppBlocks").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeAppBlocksRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeAppBlocksRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one or more application fleet associations. Either ApplicationArn or FleetName
* must be specified.
*
*
* @param describeApplicationFleetAssociationsRequest
* @return Result of the DescribeApplicationFleetAssociations operation returned by the service.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeApplicationFleetAssociations
* @see AWS API Documentation
*/
@Override
public DescribeApplicationFleetAssociationsResponse describeApplicationFleetAssociations(
DescribeApplicationFleetAssociationsRequest describeApplicationFleetAssociationsRequest)
throws InvalidParameterCombinationException, OperationNotPermittedException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DescribeApplicationFleetAssociationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeApplicationFleetAssociationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeApplicationFleetAssociationsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeApplicationFleetAssociations");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeApplicationFleetAssociations").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeApplicationFleetAssociationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeApplicationFleetAssociationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one or more applications.
*
*
* @param describeApplicationsRequest
* @return Result of the DescribeApplications operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeApplications
* @see AWS API Documentation
*/
@Override
public DescribeApplicationsResponse describeApplications(DescribeApplicationsRequest describeApplicationsRequest)
throws OperationNotPermittedException, ResourceNotFoundException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeApplicationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeApplicationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeApplicationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeApplications");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeApplications").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeApplicationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeApplicationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one or more specified Directory Config objects for AppStream 2.0, if the names
* for these objects are provided. Otherwise, all Directory Config objects in the account are described. These
* objects include the configuration information required to join fleets and image builders to Microsoft Active
* Directory domains.
*
*
* Although the response syntax in this topic includes the account password, this password is not returned in the
* actual response.
*
*
* @param describeDirectoryConfigsRequest
* @return Result of the DescribeDirectoryConfigs operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeDirectoryConfigs
* @see AWS API Documentation
*/
@Override
public DescribeDirectoryConfigsResponse describeDirectoryConfigs(
DescribeDirectoryConfigsRequest describeDirectoryConfigsRequest) throws ResourceNotFoundException,
AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDirectoryConfigsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeDirectoryConfigsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDirectoryConfigsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDirectoryConfigs");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDirectoryConfigs").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeDirectoryConfigsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeDirectoryConfigsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one of more entitlements.
*
*
* @param describeEntitlementsRequest
* @return Result of the DescribeEntitlements operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws EntitlementNotFoundException
* The entitlement can't be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeEntitlements
* @see AWS API Documentation
*/
@Override
public DescribeEntitlementsResponse describeEntitlements(DescribeEntitlementsRequest describeEntitlementsRequest)
throws OperationNotPermittedException, ResourceNotFoundException, EntitlementNotFoundException, AwsServiceException,
SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeEntitlementsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeEntitlementsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeEntitlementsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeEntitlements");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeEntitlements").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeEntitlementsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeEntitlementsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one or more specified fleets, if the fleet names are provided. Otherwise, all
* fleets in the account are described.
*
*
* @param describeFleetsRequest
* @return Result of the DescribeFleets operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeFleets
* @see AWS API
* Documentation
*/
@Override
public DescribeFleetsResponse describeFleets(DescribeFleetsRequest describeFleetsRequest) throws ResourceNotFoundException,
AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeFleetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeFleetsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeFleetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeFleets");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeFleets").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeFleetsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeFleetsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one or more specified image builders, if the image builder names are provided.
* Otherwise, all image builders in the account are described.
*
*
* @param describeImageBuildersRequest
* @return Result of the DescribeImageBuilders operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeImageBuilders
* @see AWS API Documentation
*/
@Override
public DescribeImageBuildersResponse describeImageBuilders(DescribeImageBuildersRequest describeImageBuildersRequest)
throws ResourceNotFoundException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeImageBuildersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeImageBuildersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeImageBuildersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeImageBuilders");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeImageBuilders").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeImageBuildersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeImageBuildersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes the permissions for shared AWS account IDs on a private image that you own.
*
*
* @param describeImagePermissionsRequest
* @return Result of the DescribeImagePermissions operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeImagePermissions
* @see AWS API Documentation
*/
@Override
public DescribeImagePermissionsResponse describeImagePermissions(
DescribeImagePermissionsRequest describeImagePermissionsRequest) throws ResourceNotFoundException,
AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeImagePermissionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeImagePermissionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeImagePermissionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeImagePermissions");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeImagePermissions").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeImagePermissionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeImagePermissionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided.
* Otherwise, all images in the account are described.
*
*
* @param describeImagesRequest
* @return Result of the DescribeImages operation returned by the service.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeImages
* @see AWS API
* Documentation
*/
@Override
public DescribeImagesResponse describeImages(DescribeImagesRequest describeImagesRequest)
throws InvalidParameterCombinationException, ResourceNotFoundException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeImagesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeImagesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeImagesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeImages");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeImages").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeImagesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeImagesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes the streaming sessions for a specified stack and fleet. If a UserId is provided
* for the stack and fleet, only streaming sessions for that user are described. If an authentication type is not
* provided, the default is to authenticate users using a streaming URL.
*
*
* @param describeSessionsRequest
* @return Result of the DescribeSessions operation returned by the service.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeSessions
* @see AWS
* API Documentation
*/
@Override
public DescribeSessionsResponse describeSessions(DescribeSessionsRequest describeSessionsRequest)
throws InvalidParameterCombinationException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeSessionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeSessionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeSessionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeSessions");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeSessions").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeSessionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeSessionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one or more specified stacks, if the stack names are provided. Otherwise, all
* stacks in the account are described.
*
*
* @param describeStacksRequest
* @return Result of the DescribeStacks operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeStacks
* @see AWS API
* Documentation
*/
@Override
public DescribeStacksResponse describeStacks(DescribeStacksRequest describeStacksRequest) throws ResourceNotFoundException,
AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeStacksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStacksRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStacksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStacks");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeStacks").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeStacksRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeStacksRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes the theme for a specified stack. A theme is custom branding that customizes the
* appearance of the streaming application catalog page.
*
*
* @param describeThemeForStackRequest
* @return Result of the DescribeThemeForStack operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeThemeForStack
* @see AWS API Documentation
*/
@Override
public DescribeThemeForStackResponse describeThemeForStack(DescribeThemeForStackRequest describeThemeForStackRequest)
throws ResourceNotFoundException, OperationNotPermittedException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeThemeForStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeThemeForStackRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeThemeForStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeThemeForStack");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeThemeForStack").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeThemeForStackRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeThemeForStackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one or more usage report subscriptions.
*
*
* @param describeUsageReportSubscriptionsRequest
* @return Result of the DescribeUsageReportSubscriptions operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeUsageReportSubscriptions
* @see AWS API Documentation
*/
@Override
public DescribeUsageReportSubscriptionsResponse describeUsageReportSubscriptions(
DescribeUsageReportSubscriptionsRequest describeUsageReportSubscriptionsRequest) throws ResourceNotFoundException,
InvalidAccountStatusException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeUsageReportSubscriptionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeUsageReportSubscriptionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeUsageReportSubscriptionsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUsageReportSubscriptions");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeUsageReportSubscriptions").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeUsageReportSubscriptionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeUsageReportSubscriptionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes the UserStackAssociation objects. You must specify either or both of the
* following:
*
*
* -
*
* The stack name
*
*
* -
*
* The user name (email address of the user associated with the stack) and the authentication type for the user
*
*
*
*
* @param describeUserStackAssociationsRequest
* @return Result of the DescribeUserStackAssociations operation returned by the service.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeUserStackAssociations
* @see AWS API Documentation
*/
@Override
public DescribeUserStackAssociationsResponse describeUserStackAssociations(
DescribeUserStackAssociationsRequest describeUserStackAssociationsRequest)
throws InvalidParameterCombinationException, OperationNotPermittedException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeUserStackAssociationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeUserStackAssociationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeUserStackAssociationsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUserStackAssociations");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeUserStackAssociations").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeUserStackAssociationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeUserStackAssociationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list that describes one or more specified users in the user pool.
*
*
* @param describeUsersRequest
* @return Result of the DescribeUsers operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DescribeUsers
* @see AWS API
* Documentation
*/
@Override
public DescribeUsersResponse describeUsers(DescribeUsersRequest describeUsersRequest) throws ResourceNotFoundException,
InvalidParameterCombinationException, OperationNotPermittedException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeUsersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeUsersRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeUsersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUsers");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeUsers").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeUsersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeUsersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disables the specified user in the user pool. Users can't sign in to AppStream 2.0 until they are re-enabled.
* This action does not delete the user.
*
*
* @param disableUserRequest
* @return Result of the DisableUser operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DisableUser
* @see AWS API
* Documentation
*/
@Override
public DisableUserResponse disableUser(DisableUserRequest disableUserRequest) throws ResourceNotFoundException,
AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DisableUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disableUserRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disableUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisableUser");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DisableUser").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(disableUserRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisableUserRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disassociates a specified app block builder from a specified app block.
*
*
* @param disassociateAppBlockBuilderAppBlockRequest
* @return Result of the DisassociateAppBlockBuilderAppBlock operation returned by the service.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DisassociateAppBlockBuilderAppBlock
* @see AWS API Documentation
*/
@Override
public DisassociateAppBlockBuilderAppBlockResponse disassociateAppBlockBuilderAppBlock(
DisassociateAppBlockBuilderAppBlockRequest disassociateAppBlockBuilderAppBlockRequest)
throws ConcurrentModificationException, InvalidParameterCombinationException, OperationNotPermittedException,
ResourceNotFoundException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateAppBlockBuilderAppBlockResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateAppBlockBuilderAppBlockRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateAppBlockBuilderAppBlockRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateAppBlockBuilderAppBlock");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateAppBlockBuilderAppBlock").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(disassociateAppBlockBuilderAppBlockRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateAppBlockBuilderAppBlockRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disassociates the specified application from the fleet.
*
*
* @param disassociateApplicationFleetRequest
* @return Result of the DisassociateApplicationFleet operation returned by the service.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DisassociateApplicationFleet
* @see AWS API Documentation
*/
@Override
public DisassociateApplicationFleetResponse disassociateApplicationFleet(
DisassociateApplicationFleetRequest disassociateApplicationFleetRequest) throws ConcurrentModificationException,
InvalidParameterCombinationException, OperationNotPermittedException, AwsServiceException, SdkClientException,
AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateApplicationFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateApplicationFleetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateApplicationFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateApplicationFleet");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateApplicationFleet").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(disassociateApplicationFleetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateApplicationFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified application from the specified entitlement.
*
*
* @param disassociateApplicationFromEntitlementRequest
* @return Result of the DisassociateApplicationFromEntitlement operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws EntitlementNotFoundException
* The entitlement can't be found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DisassociateApplicationFromEntitlement
* @see AWS API Documentation
*/
@Override
public DisassociateApplicationFromEntitlementResponse disassociateApplicationFromEntitlement(
DisassociateApplicationFromEntitlementRequest disassociateApplicationFromEntitlementRequest)
throws OperationNotPermittedException, ResourceNotFoundException, EntitlementNotFoundException, AwsServiceException,
SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DisassociateApplicationFromEntitlementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateApplicationFromEntitlementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateApplicationFromEntitlementRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateApplicationFromEntitlement");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateApplicationFromEntitlement").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration)
.withInput(disassociateApplicationFromEntitlementRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateApplicationFromEntitlementRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disassociates the specified fleet from the specified stack.
*
*
* @param disassociateFleetRequest
* @return Result of the DisassociateFleet operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.DisassociateFleet
* @see AWS
* API Documentation
*/
@Override
public DisassociateFleetResponse disassociateFleet(DisassociateFleetRequest disassociateFleetRequest)
throws ResourceInUseException, ResourceNotFoundException, ConcurrentModificationException,
OperationNotPermittedException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DisassociateFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateFleetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateFleet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DisassociateFleet").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(disassociateFleetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Enables a user in the user pool. After being enabled, users can sign in to AppStream 2.0 and open applications
* from the stacks to which they are assigned.
*
*
* @param enableUserRequest
* @return Result of the EnableUser operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.EnableUser
* @see AWS API
* Documentation
*/
@Override
public EnableUserResponse enableUser(EnableUserRequest enableUserRequest) throws ResourceNotFoundException,
InvalidAccountStatusException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
EnableUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(enableUserRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, enableUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "EnableUser");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("EnableUser").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(enableUserRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new EnableUserRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Immediately stops the specified streaming session.
*
*
* @param expireSessionRequest
* @return Result of the ExpireSession operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.ExpireSession
* @see AWS API
* Documentation
*/
@Override
public ExpireSessionResponse expireSession(ExpireSessionRequest expireSessionRequest) throws AwsServiceException,
SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ExpireSessionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(expireSessionRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, expireSessionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ExpireSession");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ExpireSession").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(expireSessionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ExpireSessionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the name of the fleet that is associated with the specified stack.
*
*
* @param listAssociatedFleetsRequest
* @return Result of the ListAssociatedFleets operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.ListAssociatedFleets
* @see AWS API Documentation
*/
@Override
public ListAssociatedFleetsResponse listAssociatedFleets(ListAssociatedFleetsRequest listAssociatedFleetsRequest)
throws AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListAssociatedFleetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAssociatedFleetsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAssociatedFleetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAssociatedFleets");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListAssociatedFleets").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listAssociatedFleetsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListAssociatedFleetsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the name of the stack with which the specified fleet is associated.
*
*
* @param listAssociatedStacksRequest
* @return Result of the ListAssociatedStacks operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.ListAssociatedStacks
* @see AWS API Documentation
*/
@Override
public ListAssociatedStacksResponse listAssociatedStacks(ListAssociatedStacksRequest listAssociatedStacksRequest)
throws AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListAssociatedStacksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAssociatedStacksRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAssociatedStacksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAssociatedStacks");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListAssociatedStacks").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listAssociatedStacksRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListAssociatedStacksRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list of entitled applications.
*
*
* @param listEntitledApplicationsRequest
* @return Result of the ListEntitledApplications operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws EntitlementNotFoundException
* The entitlement can't be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.ListEntitledApplications
* @see AWS API Documentation
*/
@Override
public ListEntitledApplicationsResponse listEntitledApplications(
ListEntitledApplicationsRequest listEntitledApplicationsRequest) throws OperationNotPermittedException,
ResourceNotFoundException, EntitlementNotFoundException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListEntitledApplicationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listEntitledApplicationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listEntitledApplicationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "AppStream");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListEntitledApplications");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListEntitledApplications").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listEntitledApplicationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListEntitledApplicationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list of all tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image builders,
* images, fleets, and stacks.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws AppStreamException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample AppStreamClient.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws ResourceNotFoundException, AwsServiceException, SdkClientException, AppStreamException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List