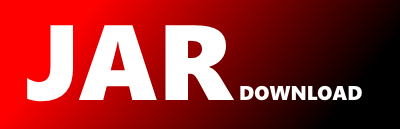
software.amazon.awssdk.services.appstream.model.ImageBuilder Maven / Gradle / Ivy
Show all versions of appstream Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.appstream.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a virtual machine that is used to create an image.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ImageBuilder implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(ImageBuilder::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(ImageBuilder::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField IMAGE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ImageArn").getter(getter(ImageBuilder::imageArn)).setter(setter(Builder::imageArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageArn").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(ImageBuilder::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField DISPLAY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DisplayName").getter(getter(ImageBuilder::displayName)).setter(setter(Builder::displayName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DisplayName").build()).build();
private static final SdkField VPC_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("VpcConfig").getter(getter(ImageBuilder::vpcConfig)).setter(setter(Builder::vpcConfig))
.constructor(VpcConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcConfig").build()).build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceType").getter(getter(ImageBuilder::instanceType)).setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType").build()).build();
private static final SdkField PLATFORM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Platform").getter(getter(ImageBuilder::platformAsString)).setter(setter(Builder::platform))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Platform").build()).build();
private static final SdkField IAM_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IamRoleArn").getter(getter(ImageBuilder::iamRoleArn)).setter(setter(Builder::iamRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IamRoleArn").build()).build();
private static final SdkField STATE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("State")
.getter(getter(ImageBuilder::stateAsString)).setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State").build()).build();
private static final SdkField STATE_CHANGE_REASON_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StateChangeReason")
.getter(getter(ImageBuilder::stateChangeReason)).setter(setter(Builder::stateChangeReason))
.constructor(ImageBuilderStateChangeReason::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateChangeReason").build()).build();
private static final SdkField CREATED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedTime").getter(getter(ImageBuilder::createdTime)).setter(setter(Builder::createdTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedTime").build()).build();
private static final SdkField ENABLE_DEFAULT_INTERNET_ACCESS_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EnableDefaultInternetAccess")
.getter(getter(ImageBuilder::enableDefaultInternetAccess))
.setter(setter(Builder::enableDefaultInternetAccess))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnableDefaultInternetAccess")
.build()).build();
private static final SdkField DOMAIN_JOIN_INFO_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DomainJoinInfo")
.getter(getter(ImageBuilder::domainJoinInfo)).setter(setter(Builder::domainJoinInfo))
.constructor(DomainJoinInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DomainJoinInfo").build()).build();
private static final SdkField NETWORK_ACCESS_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("NetworkAccessConfiguration")
.getter(getter(ImageBuilder::networkAccessConfiguration))
.setter(setter(Builder::networkAccessConfiguration))
.constructor(NetworkAccessConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkAccessConfiguration").build())
.build();
private static final SdkField> IMAGE_BUILDER_ERRORS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ImageBuilderErrors")
.getter(getter(ImageBuilder::imageBuilderErrors))
.setter(setter(Builder::imageBuilderErrors))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageBuilderErrors").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ResourceError::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField APPSTREAM_AGENT_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AppstreamAgentVersion").getter(getter(ImageBuilder::appstreamAgentVersion))
.setter(setter(Builder::appstreamAgentVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AppstreamAgentVersion").build())
.build();
private static final SdkField> ACCESS_ENDPOINTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AccessEndpoints")
.getter(getter(ImageBuilder::accessEndpoints))
.setter(setter(Builder::accessEndpoints))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccessEndpoints").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AccessEndpoint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField LATEST_APPSTREAM_AGENT_VERSION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LatestAppstreamAgentVersion")
.getter(getter(ImageBuilder::latestAppstreamAgentVersionAsString))
.setter(setter(Builder::latestAppstreamAgentVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LatestAppstreamAgentVersion")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, ARN_FIELD,
IMAGE_ARN_FIELD, DESCRIPTION_FIELD, DISPLAY_NAME_FIELD, VPC_CONFIG_FIELD, INSTANCE_TYPE_FIELD, PLATFORM_FIELD,
IAM_ROLE_ARN_FIELD, STATE_FIELD, STATE_CHANGE_REASON_FIELD, CREATED_TIME_FIELD, ENABLE_DEFAULT_INTERNET_ACCESS_FIELD,
DOMAIN_JOIN_INFO_FIELD, NETWORK_ACCESS_CONFIGURATION_FIELD, IMAGE_BUILDER_ERRORS_FIELD,
APPSTREAM_AGENT_VERSION_FIELD, ACCESS_ENDPOINTS_FIELD, LATEST_APPSTREAM_AGENT_VERSION_FIELD));
private static final long serialVersionUID = 1L;
private final String name;
private final String arn;
private final String imageArn;
private final String description;
private final String displayName;
private final VpcConfig vpcConfig;
private final String instanceType;
private final String platform;
private final String iamRoleArn;
private final String state;
private final ImageBuilderStateChangeReason stateChangeReason;
private final Instant createdTime;
private final Boolean enableDefaultInternetAccess;
private final DomainJoinInfo domainJoinInfo;
private final NetworkAccessConfiguration networkAccessConfiguration;
private final List imageBuilderErrors;
private final String appstreamAgentVersion;
private final List accessEndpoints;
private final String latestAppstreamAgentVersion;
private ImageBuilder(BuilderImpl builder) {
this.name = builder.name;
this.arn = builder.arn;
this.imageArn = builder.imageArn;
this.description = builder.description;
this.displayName = builder.displayName;
this.vpcConfig = builder.vpcConfig;
this.instanceType = builder.instanceType;
this.platform = builder.platform;
this.iamRoleArn = builder.iamRoleArn;
this.state = builder.state;
this.stateChangeReason = builder.stateChangeReason;
this.createdTime = builder.createdTime;
this.enableDefaultInternetAccess = builder.enableDefaultInternetAccess;
this.domainJoinInfo = builder.domainJoinInfo;
this.networkAccessConfiguration = builder.networkAccessConfiguration;
this.imageBuilderErrors = builder.imageBuilderErrors;
this.appstreamAgentVersion = builder.appstreamAgentVersion;
this.accessEndpoints = builder.accessEndpoints;
this.latestAppstreamAgentVersion = builder.latestAppstreamAgentVersion;
}
/**
*
* The name of the image builder.
*
*
* @return The name of the image builder.
*/
public final String name() {
return name;
}
/**
*
* The ARN for the image builder.
*
*
* @return The ARN for the image builder.
*/
public final String arn() {
return arn;
}
/**
*
* The ARN of the image from which this builder was created.
*
*
* @return The ARN of the image from which this builder was created.
*/
public final String imageArn() {
return imageArn;
}
/**
*
* The description to display.
*
*
* @return The description to display.
*/
public final String description() {
return description;
}
/**
*
* The image builder name to display.
*
*
* @return The image builder name to display.
*/
public final String displayName() {
return displayName;
}
/**
*
* The VPC configuration of the image builder.
*
*
* @return The VPC configuration of the image builder.
*/
public final VpcConfig vpcConfig() {
return vpcConfig;
}
/**
*
* The instance type for the image builder. The following instance types are available:
*
*
* -
*
* stream.standard.small
*
*
* -
*
* stream.standard.medium
*
*
* -
*
* stream.standard.large
*
*
* -
*
* stream.compute.large
*
*
* -
*
* stream.compute.xlarge
*
*
* -
*
* stream.compute.2xlarge
*
*
* -
*
* stream.compute.4xlarge
*
*
* -
*
* stream.compute.8xlarge
*
*
* -
*
* stream.memory.large
*
*
* -
*
* stream.memory.xlarge
*
*
* -
*
* stream.memory.2xlarge
*
*
* -
*
* stream.memory.4xlarge
*
*
* -
*
* stream.memory.8xlarge
*
*
* -
*
* stream.memory.z1d.large
*
*
* -
*
* stream.memory.z1d.xlarge
*
*
* -
*
* stream.memory.z1d.2xlarge
*
*
* -
*
* stream.memory.z1d.3xlarge
*
*
* -
*
* stream.memory.z1d.6xlarge
*
*
* -
*
* stream.memory.z1d.12xlarge
*
*
* -
*
* stream.graphics-design.large
*
*
* -
*
* stream.graphics-design.xlarge
*
*
* -
*
* stream.graphics-design.2xlarge
*
*
* -
*
* stream.graphics-design.4xlarge
*
*
* -
*
* stream.graphics-desktop.2xlarge
*
*
* -
*
* stream.graphics.g4dn.xlarge
*
*
* -
*
* stream.graphics.g4dn.2xlarge
*
*
* -
*
* stream.graphics.g4dn.4xlarge
*
*
* -
*
* stream.graphics.g4dn.8xlarge
*
*
* -
*
* stream.graphics.g4dn.12xlarge
*
*
* -
*
* stream.graphics.g4dn.16xlarge
*
*
* -
*
* stream.graphics-pro.4xlarge
*
*
* -
*
* stream.graphics-pro.8xlarge
*
*
* -
*
* stream.graphics-pro.16xlarge
*
*
*
*
* @return The instance type for the image builder. The following instance types are available:
*
* -
*
* stream.standard.small
*
*
* -
*
* stream.standard.medium
*
*
* -
*
* stream.standard.large
*
*
* -
*
* stream.compute.large
*
*
* -
*
* stream.compute.xlarge
*
*
* -
*
* stream.compute.2xlarge
*
*
* -
*
* stream.compute.4xlarge
*
*
* -
*
* stream.compute.8xlarge
*
*
* -
*
* stream.memory.large
*
*
* -
*
* stream.memory.xlarge
*
*
* -
*
* stream.memory.2xlarge
*
*
* -
*
* stream.memory.4xlarge
*
*
* -
*
* stream.memory.8xlarge
*
*
* -
*
* stream.memory.z1d.large
*
*
* -
*
* stream.memory.z1d.xlarge
*
*
* -
*
* stream.memory.z1d.2xlarge
*
*
* -
*
* stream.memory.z1d.3xlarge
*
*
* -
*
* stream.memory.z1d.6xlarge
*
*
* -
*
* stream.memory.z1d.12xlarge
*
*
* -
*
* stream.graphics-design.large
*
*
* -
*
* stream.graphics-design.xlarge
*
*
* -
*
* stream.graphics-design.2xlarge
*
*
* -
*
* stream.graphics-design.4xlarge
*
*
* -
*
* stream.graphics-desktop.2xlarge
*
*
* -
*
* stream.graphics.g4dn.xlarge
*
*
* -
*
* stream.graphics.g4dn.2xlarge
*
*
* -
*
* stream.graphics.g4dn.4xlarge
*
*
* -
*
* stream.graphics.g4dn.8xlarge
*
*
* -
*
* stream.graphics.g4dn.12xlarge
*
*
* -
*
* stream.graphics.g4dn.16xlarge
*
*
* -
*
* stream.graphics-pro.4xlarge
*
*
* -
*
* stream.graphics-pro.8xlarge
*
*
* -
*
* stream.graphics-pro.16xlarge
*
*
*/
public final String instanceType() {
return instanceType;
}
/**
*
* The operating system platform of the image builder.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #platform} will
* return {@link PlatformType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #platformAsString}.
*
*
* @return The operating system platform of the image builder.
* @see PlatformType
*/
public final PlatformType platform() {
return PlatformType.fromValue(platform);
}
/**
*
* The operating system platform of the image builder.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #platform} will
* return {@link PlatformType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #platformAsString}.
*
*
* @return The operating system platform of the image builder.
* @see PlatformType
*/
public final String platformAsString() {
return platform;
}
/**
*
* The ARN of the IAM role that is applied to the image builder. To assume a role, the image builder calls the AWS
* Security Token Service (STS) AssumeRole
API operation and passes the ARN of the role to use. The
* operation creates a new session with temporary credentials. AppStream 2.0 retrieves the temporary credentials and
* creates the appstream_machine_role credential profile on the instance.
*
*
* For more information, see Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming
* Instances in the Amazon AppStream 2.0 Administration Guide.
*
*
* @return The ARN of the IAM role that is applied to the image builder. To assume a role, the image builder calls
* the AWS Security Token Service (STS) AssumeRole
API operation and passes the ARN of the role
* to use. The operation creates a new session with temporary credentials. AppStream 2.0 retrieves the
* temporary credentials and creates the appstream_machine_role credential profile on the
* instance.
*
* For more information, see Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming
* Instances in the Amazon AppStream 2.0 Administration Guide.
*/
public final String iamRoleArn() {
return iamRoleArn;
}
/**
*
* The state of the image builder.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link ImageBuilderState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The state of the image builder.
* @see ImageBuilderState
*/
public final ImageBuilderState state() {
return ImageBuilderState.fromValue(state);
}
/**
*
* The state of the image builder.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link ImageBuilderState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The state of the image builder.
* @see ImageBuilderState
*/
public final String stateAsString() {
return state;
}
/**
*
* The reason why the last state change occurred.
*
*
* @return The reason why the last state change occurred.
*/
public final ImageBuilderStateChangeReason stateChangeReason() {
return stateChangeReason;
}
/**
*
* The time stamp when the image builder was created.
*
*
* @return The time stamp when the image builder was created.
*/
public final Instant createdTime() {
return createdTime;
}
/**
*
* Enables or disables default internet access for the image builder.
*
*
* @return Enables or disables default internet access for the image builder.
*/
public final Boolean enableDefaultInternetAccess() {
return enableDefaultInternetAccess;
}
/**
*
* The name of the directory and organizational unit (OU) to use to join the image builder to a Microsoft Active
* Directory domain.
*
*
* @return The name of the directory and organizational unit (OU) to use to join the image builder to a Microsoft
* Active Directory domain.
*/
public final DomainJoinInfo domainJoinInfo() {
return domainJoinInfo;
}
/**
* Returns the value of the NetworkAccessConfiguration property for this object.
*
* @return The value of the NetworkAccessConfiguration property for this object.
*/
public final NetworkAccessConfiguration networkAccessConfiguration() {
return networkAccessConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the ImageBuilderErrors property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasImageBuilderErrors() {
return imageBuilderErrors != null && !(imageBuilderErrors instanceof SdkAutoConstructList);
}
/**
*
* The image builder errors.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasImageBuilderErrors} method.
*
*
* @return The image builder errors.
*/
public final List imageBuilderErrors() {
return imageBuilderErrors;
}
/**
*
* The version of the AppStream 2.0 agent that is currently being used by the image builder.
*
*
* @return The version of the AppStream 2.0 agent that is currently being used by the image builder.
*/
public final String appstreamAgentVersion() {
return appstreamAgentVersion;
}
/**
* For responses, this returns true if the service returned a value for the AccessEndpoints property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAccessEndpoints() {
return accessEndpoints != null && !(accessEndpoints instanceof SdkAutoConstructList);
}
/**
*
* The list of virtual private cloud (VPC) interface endpoint objects. Administrators can connect to the image
* builder only through the specified endpoints.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAccessEndpoints} method.
*
*
* @return The list of virtual private cloud (VPC) interface endpoint objects. Administrators can connect to the
* image builder only through the specified endpoints.
*/
public final List accessEndpoints() {
return accessEndpoints;
}
/**
*
* Indicates whether the image builder is using the latest AppStream 2.0 agent version or not.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #latestAppstreamAgentVersion} will return {@link LatestAppstreamAgentVersion#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #latestAppstreamAgentVersionAsString}.
*
*
* @return Indicates whether the image builder is using the latest AppStream 2.0 agent version or not.
* @see LatestAppstreamAgentVersion
*/
public final LatestAppstreamAgentVersion latestAppstreamAgentVersion() {
return LatestAppstreamAgentVersion.fromValue(latestAppstreamAgentVersion);
}
/**
*
* Indicates whether the image builder is using the latest AppStream 2.0 agent version or not.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #latestAppstreamAgentVersion} will return {@link LatestAppstreamAgentVersion#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #latestAppstreamAgentVersionAsString}.
*
*
* @return Indicates whether the image builder is using the latest AppStream 2.0 agent version or not.
* @see LatestAppstreamAgentVersion
*/
public final String latestAppstreamAgentVersionAsString() {
return latestAppstreamAgentVersion;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(imageArn());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(displayName());
hashCode = 31 * hashCode + Objects.hashCode(vpcConfig());
hashCode = 31 * hashCode + Objects.hashCode(instanceType());
hashCode = 31 * hashCode + Objects.hashCode(platformAsString());
hashCode = 31 * hashCode + Objects.hashCode(iamRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(stateChangeReason());
hashCode = 31 * hashCode + Objects.hashCode(createdTime());
hashCode = 31 * hashCode + Objects.hashCode(enableDefaultInternetAccess());
hashCode = 31 * hashCode + Objects.hashCode(domainJoinInfo());
hashCode = 31 * hashCode + Objects.hashCode(networkAccessConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasImageBuilderErrors() ? imageBuilderErrors() : null);
hashCode = 31 * hashCode + Objects.hashCode(appstreamAgentVersion());
hashCode = 31 * hashCode + Objects.hashCode(hasAccessEndpoints() ? accessEndpoints() : null);
hashCode = 31 * hashCode + Objects.hashCode(latestAppstreamAgentVersionAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ImageBuilder)) {
return false;
}
ImageBuilder other = (ImageBuilder) obj;
return Objects.equals(name(), other.name()) && Objects.equals(arn(), other.arn())
&& Objects.equals(imageArn(), other.imageArn()) && Objects.equals(description(), other.description())
&& Objects.equals(displayName(), other.displayName()) && Objects.equals(vpcConfig(), other.vpcConfig())
&& Objects.equals(instanceType(), other.instanceType())
&& Objects.equals(platformAsString(), other.platformAsString())
&& Objects.equals(iamRoleArn(), other.iamRoleArn()) && Objects.equals(stateAsString(), other.stateAsString())
&& Objects.equals(stateChangeReason(), other.stateChangeReason())
&& Objects.equals(createdTime(), other.createdTime())
&& Objects.equals(enableDefaultInternetAccess(), other.enableDefaultInternetAccess())
&& Objects.equals(domainJoinInfo(), other.domainJoinInfo())
&& Objects.equals(networkAccessConfiguration(), other.networkAccessConfiguration())
&& hasImageBuilderErrors() == other.hasImageBuilderErrors()
&& Objects.equals(imageBuilderErrors(), other.imageBuilderErrors())
&& Objects.equals(appstreamAgentVersion(), other.appstreamAgentVersion())
&& hasAccessEndpoints() == other.hasAccessEndpoints()
&& Objects.equals(accessEndpoints(), other.accessEndpoints())
&& Objects.equals(latestAppstreamAgentVersionAsString(), other.latestAppstreamAgentVersionAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ImageBuilder").add("Name", name()).add("Arn", arn()).add("ImageArn", imageArn())
.add("Description", description()).add("DisplayName", displayName()).add("VpcConfig", vpcConfig())
.add("InstanceType", instanceType()).add("Platform", platformAsString()).add("IamRoleArn", iamRoleArn())
.add("State", stateAsString()).add("StateChangeReason", stateChangeReason()).add("CreatedTime", createdTime())
.add("EnableDefaultInternetAccess", enableDefaultInternetAccess()).add("DomainJoinInfo", domainJoinInfo())
.add("NetworkAccessConfiguration", networkAccessConfiguration())
.add("ImageBuilderErrors", hasImageBuilderErrors() ? imageBuilderErrors() : null)
.add("AppstreamAgentVersion", appstreamAgentVersion())
.add("AccessEndpoints", hasAccessEndpoints() ? accessEndpoints() : null)
.add("LatestAppstreamAgentVersion", latestAppstreamAgentVersionAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "ImageArn":
return Optional.ofNullable(clazz.cast(imageArn()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "DisplayName":
return Optional.ofNullable(clazz.cast(displayName()));
case "VpcConfig":
return Optional.ofNullable(clazz.cast(vpcConfig()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceType()));
case "Platform":
return Optional.ofNullable(clazz.cast(platformAsString()));
case "IamRoleArn":
return Optional.ofNullable(clazz.cast(iamRoleArn()));
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "StateChangeReason":
return Optional.ofNullable(clazz.cast(stateChangeReason()));
case "CreatedTime":
return Optional.ofNullable(clazz.cast(createdTime()));
case "EnableDefaultInternetAccess":
return Optional.ofNullable(clazz.cast(enableDefaultInternetAccess()));
case "DomainJoinInfo":
return Optional.ofNullable(clazz.cast(domainJoinInfo()));
case "NetworkAccessConfiguration":
return Optional.ofNullable(clazz.cast(networkAccessConfiguration()));
case "ImageBuilderErrors":
return Optional.ofNullable(clazz.cast(imageBuilderErrors()));
case "AppstreamAgentVersion":
return Optional.ofNullable(clazz.cast(appstreamAgentVersion()));
case "AccessEndpoints":
return Optional.ofNullable(clazz.cast(accessEndpoints()));
case "LatestAppstreamAgentVersion":
return Optional.ofNullable(clazz.cast(latestAppstreamAgentVersionAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function