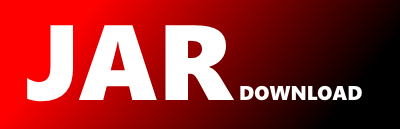
software.amazon.awssdk.services.appsync.model.UpdateFunctionRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.appsync.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateFunctionRequest extends AppSyncRequest implements
ToCopyableBuilder {
private static final SdkField API_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("apiId")
.getter(getter(UpdateFunctionRequest::apiId)).setter(setter(Builder::apiId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("apiId").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(UpdateFunctionRequest::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("description").getter(getter(UpdateFunctionRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField FUNCTION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("functionId").getter(getter(UpdateFunctionRequest::functionId)).setter(setter(Builder::functionId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("functionId").build()).build();
private static final SdkField DATA_SOURCE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("dataSourceName").getter(getter(UpdateFunctionRequest::dataSourceName))
.setter(setter(Builder::dataSourceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dataSourceName").build()).build();
private static final SdkField REQUEST_MAPPING_TEMPLATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("requestMappingTemplate").getter(getter(UpdateFunctionRequest::requestMappingTemplate))
.setter(setter(Builder::requestMappingTemplate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("requestMappingTemplate").build())
.build();
private static final SdkField RESPONSE_MAPPING_TEMPLATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("responseMappingTemplate").getter(getter(UpdateFunctionRequest::responseMappingTemplate))
.setter(setter(Builder::responseMappingTemplate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("responseMappingTemplate").build())
.build();
private static final SdkField FUNCTION_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("functionVersion").getter(getter(UpdateFunctionRequest::functionVersion))
.setter(setter(Builder::functionVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("functionVersion").build()).build();
private static final SdkField SYNC_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("syncConfig").getter(getter(UpdateFunctionRequest::syncConfig)).setter(setter(Builder::syncConfig))
.constructor(SyncConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("syncConfig").build()).build();
private static final SdkField MAX_BATCH_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("maxBatchSize").getter(getter(UpdateFunctionRequest::maxBatchSize)).setter(setter(Builder::maxBatchSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("maxBatchSize").build()).build();
private static final SdkField RUNTIME_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("runtime").getter(getter(UpdateFunctionRequest::runtime)).setter(setter(Builder::runtime))
.constructor(AppSyncRuntime::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("runtime").build()).build();
private static final SdkField CODE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("code")
.getter(getter(UpdateFunctionRequest::code)).setter(setter(Builder::code))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("code").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(API_ID_FIELD, NAME_FIELD,
DESCRIPTION_FIELD, FUNCTION_ID_FIELD, DATA_SOURCE_NAME_FIELD, REQUEST_MAPPING_TEMPLATE_FIELD,
RESPONSE_MAPPING_TEMPLATE_FIELD, FUNCTION_VERSION_FIELD, SYNC_CONFIG_FIELD, MAX_BATCH_SIZE_FIELD, RUNTIME_FIELD,
CODE_FIELD));
private final String apiId;
private final String name;
private final String description;
private final String functionId;
private final String dataSourceName;
private final String requestMappingTemplate;
private final String responseMappingTemplate;
private final String functionVersion;
private final SyncConfig syncConfig;
private final Integer maxBatchSize;
private final AppSyncRuntime runtime;
private final String code;
private UpdateFunctionRequest(BuilderImpl builder) {
super(builder);
this.apiId = builder.apiId;
this.name = builder.name;
this.description = builder.description;
this.functionId = builder.functionId;
this.dataSourceName = builder.dataSourceName;
this.requestMappingTemplate = builder.requestMappingTemplate;
this.responseMappingTemplate = builder.responseMappingTemplate;
this.functionVersion = builder.functionVersion;
this.syncConfig = builder.syncConfig;
this.maxBatchSize = builder.maxBatchSize;
this.runtime = builder.runtime;
this.code = builder.code;
}
/**
*
* The GraphQL API ID.
*
*
* @return The GraphQL API ID.
*/
public final String apiId() {
return apiId;
}
/**
*
* The Function
name.
*
*
* @return The Function
name.
*/
public final String name() {
return name;
}
/**
*
* The Function
description.
*
*
* @return The Function
description.
*/
public final String description() {
return description;
}
/**
*
* The function ID.
*
*
* @return The function ID.
*/
public final String functionId() {
return functionId;
}
/**
*
* The Function
DataSource
name.
*
*
* @return The Function
DataSource
name.
*/
public final String dataSourceName() {
return dataSourceName;
}
/**
*
* The Function
request mapping template. Functions support only the 2018-05-29 version of the request
* mapping template.
*
*
* @return The Function
request mapping template. Functions support only the 2018-05-29 version of the
* request mapping template.
*/
public final String requestMappingTemplate() {
return requestMappingTemplate;
}
/**
*
* The Function
request mapping template.
*
*
* @return The Function
request mapping template.
*/
public final String responseMappingTemplate() {
return responseMappingTemplate;
}
/**
*
* The version
of the request mapping template. Currently, the supported value is 2018-05-29. Note that
* when using VTL and mapping templates, the functionVersion
is required.
*
*
* @return The version
of the request mapping template. Currently, the supported value is 2018-05-29.
* Note that when using VTL and mapping templates, the functionVersion
is required.
*/
public final String functionVersion() {
return functionVersion;
}
/**
* Returns the value of the SyncConfig property for this object.
*
* @return The value of the SyncConfig property for this object.
*/
public final SyncConfig syncConfig() {
return syncConfig;
}
/**
*
* The maximum batching size for a resolver.
*
*
* @return The maximum batching size for a resolver.
*/
public final Integer maxBatchSize() {
return maxBatchSize;
}
/**
* Returns the value of the Runtime property for this object.
*
* @return The value of the Runtime property for this object.
*/
public final AppSyncRuntime runtime() {
return runtime;
}
/**
*
* The function
code that contains the request and response functions. When code is used, the
* runtime
is required. The runtime
value must be APPSYNC_JS
.
*
*
* @return The function
code that contains the request and response functions. When code is used, the
* runtime
is required. The runtime
value must be APPSYNC_JS
.
*/
public final String code() {
return code;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(apiId());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(functionId());
hashCode = 31 * hashCode + Objects.hashCode(dataSourceName());
hashCode = 31 * hashCode + Objects.hashCode(requestMappingTemplate());
hashCode = 31 * hashCode + Objects.hashCode(responseMappingTemplate());
hashCode = 31 * hashCode + Objects.hashCode(functionVersion());
hashCode = 31 * hashCode + Objects.hashCode(syncConfig());
hashCode = 31 * hashCode + Objects.hashCode(maxBatchSize());
hashCode = 31 * hashCode + Objects.hashCode(runtime());
hashCode = 31 * hashCode + Objects.hashCode(code());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateFunctionRequest)) {
return false;
}
UpdateFunctionRequest other = (UpdateFunctionRequest) obj;
return Objects.equals(apiId(), other.apiId()) && Objects.equals(name(), other.name())
&& Objects.equals(description(), other.description()) && Objects.equals(functionId(), other.functionId())
&& Objects.equals(dataSourceName(), other.dataSourceName())
&& Objects.equals(requestMappingTemplate(), other.requestMappingTemplate())
&& Objects.equals(responseMappingTemplate(), other.responseMappingTemplate())
&& Objects.equals(functionVersion(), other.functionVersion()) && Objects.equals(syncConfig(), other.syncConfig())
&& Objects.equals(maxBatchSize(), other.maxBatchSize()) && Objects.equals(runtime(), other.runtime())
&& Objects.equals(code(), other.code());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateFunctionRequest").add("ApiId", apiId()).add("Name", name())
.add("Description", description()).add("FunctionId", functionId()).add("DataSourceName", dataSourceName())
.add("RequestMappingTemplate", requestMappingTemplate())
.add("ResponseMappingTemplate", responseMappingTemplate()).add("FunctionVersion", functionVersion())
.add("SyncConfig", syncConfig()).add("MaxBatchSize", maxBatchSize()).add("Runtime", runtime())
.add("Code", code()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "apiId":
return Optional.ofNullable(clazz.cast(apiId()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "functionId":
return Optional.ofNullable(clazz.cast(functionId()));
case "dataSourceName":
return Optional.ofNullable(clazz.cast(dataSourceName()));
case "requestMappingTemplate":
return Optional.ofNullable(clazz.cast(requestMappingTemplate()));
case "responseMappingTemplate":
return Optional.ofNullable(clazz.cast(responseMappingTemplate()));
case "functionVersion":
return Optional.ofNullable(clazz.cast(functionVersion()));
case "syncConfig":
return Optional.ofNullable(clazz.cast(syncConfig()));
case "maxBatchSize":
return Optional.ofNullable(clazz.cast(maxBatchSize()));
case "runtime":
return Optional.ofNullable(clazz.cast(runtime()));
case "code":
return Optional.ofNullable(clazz.cast(code()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function