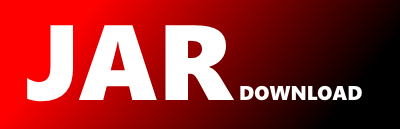
software.amazon.awssdk.services.autoscaling.model.CreateLaunchConfigurationRequest Maven / Gradle / Ivy
Show all versions of autoscaling Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.autoscaling.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateLaunchConfigurationRequest extends AutoScalingRequest implements
ToCopyableBuilder {
private static final SdkField LAUNCH_CONFIGURATION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LaunchConfigurationName").getter(getter(CreateLaunchConfigurationRequest::launchConfigurationName))
.setter(setter(Builder::launchConfigurationName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchConfigurationName").build())
.build();
private static final SdkField IMAGE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ImageId").getter(getter(CreateLaunchConfigurationRequest::imageId)).setter(setter(Builder::imageId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageId").build()).build();
private static final SdkField KEY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KeyName").getter(getter(CreateLaunchConfigurationRequest::keyName)).setter(setter(Builder::keyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeyName").build()).build();
private static final SdkField> SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SecurityGroups")
.getter(getter(CreateLaunchConfigurationRequest::securityGroups))
.setter(setter(Builder::securityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CLASSIC_LINK_VPC_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClassicLinkVPCId").getter(getter(CreateLaunchConfigurationRequest::classicLinkVPCId))
.setter(setter(Builder::classicLinkVPCId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClassicLinkVPCId").build()).build();
private static final SdkField> CLASSIC_LINK_VPC_SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ClassicLinkVPCSecurityGroups")
.getter(getter(CreateLaunchConfigurationRequest::classicLinkVPCSecurityGroups))
.setter(setter(Builder::classicLinkVPCSecurityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClassicLinkVPCSecurityGroups")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField USER_DATA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UserData").getter(getter(CreateLaunchConfigurationRequest::userData)).setter(setter(Builder::userData))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserData").build()).build();
private static final SdkField INSTANCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceId").getter(getter(CreateLaunchConfigurationRequest::instanceId))
.setter(setter(Builder::instanceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceId").build()).build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceType").getter(getter(CreateLaunchConfigurationRequest::instanceType))
.setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType").build()).build();
private static final SdkField KERNEL_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KernelId").getter(getter(CreateLaunchConfigurationRequest::kernelId)).setter(setter(Builder::kernelId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KernelId").build()).build();
private static final SdkField RAMDISK_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RamdiskId").getter(getter(CreateLaunchConfigurationRequest::ramdiskId))
.setter(setter(Builder::ramdiskId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RamdiskId").build()).build();
private static final SdkField> BLOCK_DEVICE_MAPPINGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("BlockDeviceMappings")
.getter(getter(CreateLaunchConfigurationRequest::blockDeviceMappings))
.setter(setter(Builder::blockDeviceMappings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BlockDeviceMappings").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(BlockDeviceMapping::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INSTANCE_MONITORING_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InstanceMonitoring")
.getter(getter(CreateLaunchConfigurationRequest::instanceMonitoring)).setter(setter(Builder::instanceMonitoring))
.constructor(InstanceMonitoring::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceMonitoring").build())
.build();
private static final SdkField SPOT_PRICE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SpotPrice").getter(getter(CreateLaunchConfigurationRequest::spotPrice))
.setter(setter(Builder::spotPrice))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SpotPrice").build()).build();
private static final SdkField IAM_INSTANCE_PROFILE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IamInstanceProfile").getter(getter(CreateLaunchConfigurationRequest::iamInstanceProfile))
.setter(setter(Builder::iamInstanceProfile))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IamInstanceProfile").build())
.build();
private static final SdkField EBS_OPTIMIZED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("EbsOptimized").getter(getter(CreateLaunchConfigurationRequest::ebsOptimized))
.setter(setter(Builder::ebsOptimized))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EbsOptimized").build()).build();
private static final SdkField ASSOCIATE_PUBLIC_IP_ADDRESS_FIELD = SdkField
. builder(MarshallingType.BOOLEAN).memberName("AssociatePublicIpAddress")
.getter(getter(CreateLaunchConfigurationRequest::associatePublicIpAddress))
.setter(setter(Builder::associatePublicIpAddress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssociatePublicIpAddress").build())
.build();
private static final SdkField PLACEMENT_TENANCY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PlacementTenancy").getter(getter(CreateLaunchConfigurationRequest::placementTenancy))
.setter(setter(Builder::placementTenancy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlacementTenancy").build()).build();
private static final SdkField METADATA_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("MetadataOptions")
.getter(getter(CreateLaunchConfigurationRequest::metadataOptions)).setter(setter(Builder::metadataOptions))
.constructor(InstanceMetadataOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetadataOptions").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
LAUNCH_CONFIGURATION_NAME_FIELD, IMAGE_ID_FIELD, KEY_NAME_FIELD, SECURITY_GROUPS_FIELD, CLASSIC_LINK_VPC_ID_FIELD,
CLASSIC_LINK_VPC_SECURITY_GROUPS_FIELD, USER_DATA_FIELD, INSTANCE_ID_FIELD, INSTANCE_TYPE_FIELD, KERNEL_ID_FIELD,
RAMDISK_ID_FIELD, BLOCK_DEVICE_MAPPINGS_FIELD, INSTANCE_MONITORING_FIELD, SPOT_PRICE_FIELD,
IAM_INSTANCE_PROFILE_FIELD, EBS_OPTIMIZED_FIELD, ASSOCIATE_PUBLIC_IP_ADDRESS_FIELD, PLACEMENT_TENANCY_FIELD,
METADATA_OPTIONS_FIELD));
private final String launchConfigurationName;
private final String imageId;
private final String keyName;
private final List securityGroups;
private final String classicLinkVPCId;
private final List classicLinkVPCSecurityGroups;
private final String userData;
private final String instanceId;
private final String instanceType;
private final String kernelId;
private final String ramdiskId;
private final List blockDeviceMappings;
private final InstanceMonitoring instanceMonitoring;
private final String spotPrice;
private final String iamInstanceProfile;
private final Boolean ebsOptimized;
private final Boolean associatePublicIpAddress;
private final String placementTenancy;
private final InstanceMetadataOptions metadataOptions;
private CreateLaunchConfigurationRequest(BuilderImpl builder) {
super(builder);
this.launchConfigurationName = builder.launchConfigurationName;
this.imageId = builder.imageId;
this.keyName = builder.keyName;
this.securityGroups = builder.securityGroups;
this.classicLinkVPCId = builder.classicLinkVPCId;
this.classicLinkVPCSecurityGroups = builder.classicLinkVPCSecurityGroups;
this.userData = builder.userData;
this.instanceId = builder.instanceId;
this.instanceType = builder.instanceType;
this.kernelId = builder.kernelId;
this.ramdiskId = builder.ramdiskId;
this.blockDeviceMappings = builder.blockDeviceMappings;
this.instanceMonitoring = builder.instanceMonitoring;
this.spotPrice = builder.spotPrice;
this.iamInstanceProfile = builder.iamInstanceProfile;
this.ebsOptimized = builder.ebsOptimized;
this.associatePublicIpAddress = builder.associatePublicIpAddress;
this.placementTenancy = builder.placementTenancy;
this.metadataOptions = builder.metadataOptions;
}
/**
*
* The name of the launch configuration. This name must be unique per Region per account.
*
*
* @return The name of the launch configuration. This name must be unique per Region per account.
*/
public final String launchConfigurationName() {
return launchConfigurationName;
}
/**
*
* The ID of the Amazon Machine Image (AMI) that was assigned during registration. For more information, see Find a Linux AMI in the
* Amazon EC2 User Guide for Linux Instances.
*
*
* If you specify InstanceId
, an ImageId
is not required.
*
*
* @return The ID of the Amazon Machine Image (AMI) that was assigned during registration. For more information, see
* Find a Linux AMI in
* the Amazon EC2 User Guide for Linux Instances.
*
* If you specify InstanceId
, an ImageId
is not required.
*/
public final String imageId() {
return imageId;
}
/**
*
* The name of the key pair. For more information, see Amazon EC2 key pairs and Amazon EC2
* instances in the Amazon EC2 User Guide for Linux Instances.
*
*
* @return The name of the key pair. For more information, see Amazon EC2 key pairs and
* Amazon EC2 instances in the Amazon EC2 User Guide for Linux Instances.
*/
public final String keyName() {
return keyName;
}
/**
* For responses, this returns true if the service returned a value for the SecurityGroups property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecurityGroups() {
return securityGroups != null && !(securityGroups instanceof SdkAutoConstructList);
}
/**
*
* A list that contains the security group IDs to assign to the instances in the Auto Scaling group. For more
* information, see Control
* traffic to your Amazon Web Services resources using security groups in the Amazon Virtual Private Cloud
* User Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecurityGroups} method.
*
*
* @return A list that contains the security group IDs to assign to the instances in the Auto Scaling group. For
* more information, see Control traffic to your
* Amazon Web Services resources using security groups in the Amazon Virtual Private Cloud User
* Guide.
*/
public final List securityGroups() {
return securityGroups;
}
/**
*
* Available for backward compatibility.
*
*
* @return Available for backward compatibility.
*/
public final String classicLinkVPCId() {
return classicLinkVPCId;
}
/**
* For responses, this returns true if the service returned a value for the ClassicLinkVPCSecurityGroups property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasClassicLinkVPCSecurityGroups() {
return classicLinkVPCSecurityGroups != null && !(classicLinkVPCSecurityGroups instanceof SdkAutoConstructList);
}
/**
*
* Available for backward compatibility.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasClassicLinkVPCSecurityGroups} method.
*
*
* @return Available for backward compatibility.
*/
public final List classicLinkVPCSecurityGroups() {
return classicLinkVPCSecurityGroups;
}
/**
*
* The user data to make available to the launched EC2 instances. For more information, see Instance metadata and user
* data (Linux) and Instance metadata and
* user data (Windows). If you are using a command line tool, base64-encoding is performed for you, and you can
* load the text from a file. Otherwise, you must provide base64-encoded text. User data is limited to 16 KB.
*
*
* @return The user data to make available to the launched EC2 instances. For more information, see Instance metadata
* and user data (Linux) and Instance
* metadata and user data (Windows). If you are using a command line tool, base64-encoding is performed
* for you, and you can load the text from a file. Otherwise, you must provide base64-encoded text. User
* data is limited to 16 KB.
*/
public final String userData() {
return userData;
}
/**
*
* The ID of the instance to use to create the launch configuration. The new launch configuration derives attributes
* from the instance, except for the block device mapping.
*
*
* To create a launch configuration with a block device mapping or override any other instance attributes, specify
* them as part of the same request.
*
*
* For more information, see Create a launch
* configuration in the Amazon EC2 Auto Scaling User Guide.
*
*
* @return The ID of the instance to use to create the launch configuration. The new launch configuration derives
* attributes from the instance, except for the block device mapping.
*
* To create a launch configuration with a block device mapping or override any other instance attributes,
* specify them as part of the same request.
*
*
* For more information, see Create a launch
* configuration in the Amazon EC2 Auto Scaling User Guide.
*/
public final String instanceId() {
return instanceId;
}
/**
*
* Specifies the instance type of the EC2 instance. For information about available instance types, see Available
* instance types in the Amazon EC2 User Guide for Linux Instances.
*
*
* If you specify InstanceId
, an InstanceType
is not required.
*
*
* @return Specifies the instance type of the EC2 instance. For information about available instance types, see
* Available instance types in the Amazon EC2 User Guide for Linux Instances.
*
* If you specify InstanceId
, an InstanceType
is not required.
*/
public final String instanceType() {
return instanceType;
}
/**
*
* The ID of the kernel associated with the AMI.
*
*
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see User provided kernels in
* the Amazon EC2 User Guide for Linux Instances.
*
*
*
* @return The ID of the kernel associated with the AMI.
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see User provided
* kernels in the Amazon EC2 User Guide for Linux Instances.
*
*/
public final String kernelId() {
return kernelId;
}
/**
*
* The ID of the RAM disk to select.
*
*
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see User provided kernels in
* the Amazon EC2 User Guide for Linux Instances.
*
*
*
* @return The ID of the RAM disk to select.
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see User provided
* kernels in the Amazon EC2 User Guide for Linux Instances.
*
*/
public final String ramdiskId() {
return ramdiskId;
}
/**
* For responses, this returns true if the service returned a value for the BlockDeviceMappings property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasBlockDeviceMappings() {
return blockDeviceMappings != null && !(blockDeviceMappings instanceof SdkAutoConstructList);
}
/**
*
* The block device mapping entries that define the block devices to attach to the instances at launch. By default,
* the block devices specified in the block device mapping for the AMI are used. For more information, see Block device
* mappings in the Amazon EC2 User Guide for Linux Instances.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasBlockDeviceMappings} method.
*
*
* @return The block device mapping entries that define the block devices to attach to the instances at launch. By
* default, the block devices specified in the block device mapping for the AMI are used. For more
* information, see Block
* device mappings in the Amazon EC2 User Guide for Linux Instances.
*/
public final List blockDeviceMappings() {
return blockDeviceMappings;
}
/**
*
* Controls whether instances in this group are launched with detailed (true
) or basic (
* false
) monitoring.
*
*
* The default value is true
(enabled).
*
*
*
* When detailed monitoring is enabled, Amazon CloudWatch generates metrics every minute and your account is charged
* a fee. When you disable detailed monitoring, CloudWatch generates metrics every 5 minutes. For more information,
* see Configure
* monitoring for Auto Scaling instances in the Amazon EC2 Auto Scaling User Guide.
*
*
*
* @return Controls whether instances in this group are launched with detailed (true
) or basic (
* false
) monitoring.
*
* The default value is true
(enabled).
*
*
*
* When detailed monitoring is enabled, Amazon CloudWatch generates metrics every minute and your account is
* charged a fee. When you disable detailed monitoring, CloudWatch generates metrics every 5 minutes. For
* more information, see Configure
* monitoring for Auto Scaling instances in the Amazon EC2 Auto Scaling User Guide.
*
*/
public final InstanceMonitoring instanceMonitoring() {
return instanceMonitoring;
}
/**
*
* The maximum hourly price to be paid for any Spot Instance launched to fulfill the request. Spot Instances are
* launched when the price you specify exceeds the current Spot price. For more information, see Request Spot
* Instances for fault-tolerant and flexible applications in the Amazon EC2 Auto Scaling User Guide.
*
*
* Valid Range: Minimum value of 0.001
*
*
*
* When you change your maximum price by creating a new launch configuration, running instances will continue to run
* as long as the maximum price for those running instances is higher than the current Spot price.
*
*
*
* @return The maximum hourly price to be paid for any Spot Instance launched to fulfill the request. Spot Instances
* are launched when the price you specify exceeds the current Spot price. For more information, see Request
* Spot Instances for fault-tolerant and flexible applications in the Amazon EC2 Auto Scaling User
* Guide.
*
* Valid Range: Minimum value of 0.001
*
*
*
* When you change your maximum price by creating a new launch configuration, running instances will
* continue to run as long as the maximum price for those running instances is higher than the current Spot
* price.
*
*/
public final String spotPrice() {
return spotPrice;
}
/**
*
* The name or the Amazon Resource Name (ARN) of the instance profile associated with the IAM role for the instance.
* The instance profile contains the IAM role. For more information, see IAM role for applications that run
* on Amazon EC2 instances in the Amazon EC2 Auto Scaling User Guide.
*
*
* @return The name or the Amazon Resource Name (ARN) of the instance profile associated with the IAM role for the
* instance. The instance profile contains the IAM role. For more information, see IAM role for applications
* that run on Amazon EC2 instances in the Amazon EC2 Auto Scaling User Guide.
*/
public final String iamInstanceProfile() {
return iamInstanceProfile;
}
/**
*
* Specifies whether the launch configuration is optimized for EBS I/O (true
) or not (
* false
). The optimization provides dedicated throughput to Amazon EBS and an optimized configuration
* stack to provide optimal I/O performance. This optimization is not available with all instance types. Additional
* fees are incurred when you enable EBS optimization for an instance type that is not EBS-optimized by default. For
* more information, see Amazon
* EBS-optimized instances in the Amazon EC2 User Guide for Linux Instances.
*
*
* The default value is false
.
*
*
* @return Specifies whether the launch configuration is optimized for EBS I/O (true
) or not (
* false
). The optimization provides dedicated throughput to Amazon EBS and an optimized
* configuration stack to provide optimal I/O performance. This optimization is not available with all
* instance types. Additional fees are incurred when you enable EBS optimization for an instance type that
* is not EBS-optimized by default. For more information, see Amazon EBS-optimized
* instances in the Amazon EC2 User Guide for Linux Instances.
*
* The default value is false
.
*/
public final Boolean ebsOptimized() {
return ebsOptimized;
}
/**
*
* Specifies whether to assign a public IPv4 address to the group's instances. If the instance is launched into a
* default subnet, the default is to assign a public IPv4 address, unless you disabled the option to assign a public
* IPv4 address on the subnet. If the instance is launched into a nondefault subnet, the default is not to assign a
* public IPv4 address, unless you enabled the option to assign a public IPv4 address on the subnet.
*
*
* If you specify true
, each instance in the Auto Scaling group receives a unique public IPv4 address.
* For more information, see Provide
* network connectivity for your Auto Scaling instances using Amazon VPC in the Amazon EC2 Auto Scaling User
* Guide.
*
*
* If you specify this property, you must specify at least one subnet for VPCZoneIdentifier
when you
* create your group.
*
*
* @return Specifies whether to assign a public IPv4 address to the group's instances. If the instance is launched
* into a default subnet, the default is to assign a public IPv4 address, unless you disabled the option to
* assign a public IPv4 address on the subnet. If the instance is launched into a nondefault subnet, the
* default is not to assign a public IPv4 address, unless you enabled the option to assign a public IPv4
* address on the subnet.
*
* If you specify true
, each instance in the Auto Scaling group receives a unique public IPv4
* address. For more information, see Provide network connectivity
* for your Auto Scaling instances using Amazon VPC in the Amazon EC2 Auto Scaling User Guide.
*
*
* If you specify this property, you must specify at least one subnet for VPCZoneIdentifier
* when you create your group.
*/
public final Boolean associatePublicIpAddress() {
return associatePublicIpAddress;
}
/**
*
* The tenancy of the instance, either default
or dedicated
. An instance with
* dedicated
tenancy runs on isolated, single-tenant hardware and can only be launched into a VPC. To
* launch dedicated instances into a shared tenancy VPC (a VPC with the instance placement tenancy attribute set to
* default
), you must set the value of this property to dedicated
.
*
*
* If you specify PlacementTenancy
, you must specify at least one subnet for
* VPCZoneIdentifier
when you create your group.
*
*
* Valid values: default
| dedicated
*
*
* @return The tenancy of the instance, either default
or dedicated
. An instance with
* dedicated
tenancy runs on isolated, single-tenant hardware and can only be launched into a
* VPC. To launch dedicated instances into a shared tenancy VPC (a VPC with the instance placement tenancy
* attribute set to default
), you must set the value of this property to dedicated
* .
*
* If you specify PlacementTenancy
, you must specify at least one subnet for
* VPCZoneIdentifier
when you create your group.
*
*
* Valid values: default
| dedicated
*/
public final String placementTenancy() {
return placementTenancy;
}
/**
*
* The metadata options for the instances. For more information, see Configure the instance metadata options in the Amazon EC2 Auto Scaling User Guide.
*
*
* @return The metadata options for the instances. For more information, see Configure the instance metadata options in the Amazon EC2 Auto Scaling User Guide.
*/
public final InstanceMetadataOptions metadataOptions() {
return metadataOptions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(launchConfigurationName());
hashCode = 31 * hashCode + Objects.hashCode(imageId());
hashCode = 31 * hashCode + Objects.hashCode(keyName());
hashCode = 31 * hashCode + Objects.hashCode(hasSecurityGroups() ? securityGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(classicLinkVPCId());
hashCode = 31 * hashCode + Objects.hashCode(hasClassicLinkVPCSecurityGroups() ? classicLinkVPCSecurityGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(userData());
hashCode = 31 * hashCode + Objects.hashCode(instanceId());
hashCode = 31 * hashCode + Objects.hashCode(instanceType());
hashCode = 31 * hashCode + Objects.hashCode(kernelId());
hashCode = 31 * hashCode + Objects.hashCode(ramdiskId());
hashCode = 31 * hashCode + Objects.hashCode(hasBlockDeviceMappings() ? blockDeviceMappings() : null);
hashCode = 31 * hashCode + Objects.hashCode(instanceMonitoring());
hashCode = 31 * hashCode + Objects.hashCode(spotPrice());
hashCode = 31 * hashCode + Objects.hashCode(iamInstanceProfile());
hashCode = 31 * hashCode + Objects.hashCode(ebsOptimized());
hashCode = 31 * hashCode + Objects.hashCode(associatePublicIpAddress());
hashCode = 31 * hashCode + Objects.hashCode(placementTenancy());
hashCode = 31 * hashCode + Objects.hashCode(metadataOptions());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateLaunchConfigurationRequest)) {
return false;
}
CreateLaunchConfigurationRequest other = (CreateLaunchConfigurationRequest) obj;
return Objects.equals(launchConfigurationName(), other.launchConfigurationName())
&& Objects.equals(imageId(), other.imageId()) && Objects.equals(keyName(), other.keyName())
&& hasSecurityGroups() == other.hasSecurityGroups() && Objects.equals(securityGroups(), other.securityGroups())
&& Objects.equals(classicLinkVPCId(), other.classicLinkVPCId())
&& hasClassicLinkVPCSecurityGroups() == other.hasClassicLinkVPCSecurityGroups()
&& Objects.equals(classicLinkVPCSecurityGroups(), other.classicLinkVPCSecurityGroups())
&& Objects.equals(userData(), other.userData()) && Objects.equals(instanceId(), other.instanceId())
&& Objects.equals(instanceType(), other.instanceType()) && Objects.equals(kernelId(), other.kernelId())
&& Objects.equals(ramdiskId(), other.ramdiskId()) && hasBlockDeviceMappings() == other.hasBlockDeviceMappings()
&& Objects.equals(blockDeviceMappings(), other.blockDeviceMappings())
&& Objects.equals(instanceMonitoring(), other.instanceMonitoring())
&& Objects.equals(spotPrice(), other.spotPrice())
&& Objects.equals(iamInstanceProfile(), other.iamInstanceProfile())
&& Objects.equals(ebsOptimized(), other.ebsOptimized())
&& Objects.equals(associatePublicIpAddress(), other.associatePublicIpAddress())
&& Objects.equals(placementTenancy(), other.placementTenancy())
&& Objects.equals(metadataOptions(), other.metadataOptions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateLaunchConfigurationRequest").add("LaunchConfigurationName", launchConfigurationName())
.add("ImageId", imageId()).add("KeyName", keyName())
.add("SecurityGroups", hasSecurityGroups() ? securityGroups() : null).add("ClassicLinkVPCId", classicLinkVPCId())
.add("ClassicLinkVPCSecurityGroups", hasClassicLinkVPCSecurityGroups() ? classicLinkVPCSecurityGroups() : null)
.add("UserData", userData()).add("InstanceId", instanceId()).add("InstanceType", instanceType())
.add("KernelId", kernelId()).add("RamdiskId", ramdiskId())
.add("BlockDeviceMappings", hasBlockDeviceMappings() ? blockDeviceMappings() : null)
.add("InstanceMonitoring", instanceMonitoring()).add("SpotPrice", spotPrice())
.add("IamInstanceProfile", iamInstanceProfile()).add("EbsOptimized", ebsOptimized())
.add("AssociatePublicIpAddress", associatePublicIpAddress()).add("PlacementTenancy", placementTenancy())
.add("MetadataOptions", metadataOptions()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "LaunchConfigurationName":
return Optional.ofNullable(clazz.cast(launchConfigurationName()));
case "ImageId":
return Optional.ofNullable(clazz.cast(imageId()));
case "KeyName":
return Optional.ofNullable(clazz.cast(keyName()));
case "SecurityGroups":
return Optional.ofNullable(clazz.cast(securityGroups()));
case "ClassicLinkVPCId":
return Optional.ofNullable(clazz.cast(classicLinkVPCId()));
case "ClassicLinkVPCSecurityGroups":
return Optional.ofNullable(clazz.cast(classicLinkVPCSecurityGroups()));
case "UserData":
return Optional.ofNullable(clazz.cast(userData()));
case "InstanceId":
return Optional.ofNullable(clazz.cast(instanceId()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceType()));
case "KernelId":
return Optional.ofNullable(clazz.cast(kernelId()));
case "RamdiskId":
return Optional.ofNullable(clazz.cast(ramdiskId()));
case "BlockDeviceMappings":
return Optional.ofNullable(clazz.cast(blockDeviceMappings()));
case "InstanceMonitoring":
return Optional.ofNullable(clazz.cast(instanceMonitoring()));
case "SpotPrice":
return Optional.ofNullable(clazz.cast(spotPrice()));
case "IamInstanceProfile":
return Optional.ofNullable(clazz.cast(iamInstanceProfile()));
case "EbsOptimized":
return Optional.ofNullable(clazz.cast(ebsOptimized()));
case "AssociatePublicIpAddress":
return Optional.ofNullable(clazz.cast(associatePublicIpAddress()));
case "PlacementTenancy":
return Optional.ofNullable(clazz.cast(placementTenancy()));
case "MetadataOptions":
return Optional.ofNullable(clazz.cast(metadataOptions()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function