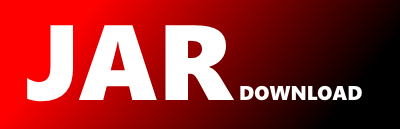
software.amazon.awssdk.services.backup.model.ListCopyJobsRequest Maven / Gradle / Ivy
Show all versions of backup Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.backup.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ListCopyJobsRequest extends BackupRequest implements
ToCopyableBuilder {
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NextToken").getter(getter(ListCopyJobsRequest::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("nextToken").build()).build();
private static final SdkField MAX_RESULTS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxResults").getter(getter(ListCopyJobsRequest::maxResults)).setter(setter(Builder::maxResults))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("maxResults").build()).build();
private static final SdkField BY_RESOURCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ByResourceArn").getter(getter(ListCopyJobsRequest::byResourceArn))
.setter(setter(Builder::byResourceArn))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("resourceArn").build()).build();
private static final SdkField BY_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ByState").getter(getter(ListCopyJobsRequest::byStateAsString)).setter(setter(Builder::byState))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("state").build()).build();
private static final SdkField BY_CREATED_BEFORE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ByCreatedBefore").getter(getter(ListCopyJobsRequest::byCreatedBefore))
.setter(setter(Builder::byCreatedBefore))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("createdBefore").build()).build();
private static final SdkField BY_CREATED_AFTER_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ByCreatedAfter").getter(getter(ListCopyJobsRequest::byCreatedAfter))
.setter(setter(Builder::byCreatedAfter))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("createdAfter").build()).build();
private static final SdkField BY_RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ByResourceType").getter(getter(ListCopyJobsRequest::byResourceType))
.setter(setter(Builder::byResourceType))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("resourceType").build()).build();
private static final SdkField BY_DESTINATION_VAULT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ByDestinationVaultArn").getter(getter(ListCopyJobsRequest::byDestinationVaultArn))
.setter(setter(Builder::byDestinationVaultArn))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("destinationVaultArn").build())
.build();
private static final SdkField BY_ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ByAccountId").getter(getter(ListCopyJobsRequest::byAccountId)).setter(setter(Builder::byAccountId))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("accountId").build()).build();
private static final SdkField BY_COMPLETE_BEFORE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ByCompleteBefore").getter(getter(ListCopyJobsRequest::byCompleteBefore))
.setter(setter(Builder::byCompleteBefore))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("completeBefore").build())
.build();
private static final SdkField BY_COMPLETE_AFTER_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ByCompleteAfter").getter(getter(ListCopyJobsRequest::byCompleteAfter))
.setter(setter(Builder::byCompleteAfter))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("completeAfter").build()).build();
private static final SdkField BY_PARENT_JOB_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ByParentJobId").getter(getter(ListCopyJobsRequest::byParentJobId))
.setter(setter(Builder::byParentJobId))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("parentJobId").build()).build();
private static final SdkField BY_MESSAGE_CATEGORY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ByMessageCategory").getter(getter(ListCopyJobsRequest::byMessageCategory))
.setter(setter(Builder::byMessageCategory))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("messageCategory").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NEXT_TOKEN_FIELD,
MAX_RESULTS_FIELD, BY_RESOURCE_ARN_FIELD, BY_STATE_FIELD, BY_CREATED_BEFORE_FIELD, BY_CREATED_AFTER_FIELD,
BY_RESOURCE_TYPE_FIELD, BY_DESTINATION_VAULT_ARN_FIELD, BY_ACCOUNT_ID_FIELD, BY_COMPLETE_BEFORE_FIELD,
BY_COMPLETE_AFTER_FIELD, BY_PARENT_JOB_ID_FIELD, BY_MESSAGE_CATEGORY_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String nextToken;
private final Integer maxResults;
private final String byResourceArn;
private final String byState;
private final Instant byCreatedBefore;
private final Instant byCreatedAfter;
private final String byResourceType;
private final String byDestinationVaultArn;
private final String byAccountId;
private final Instant byCompleteBefore;
private final Instant byCompleteAfter;
private final String byParentJobId;
private final String byMessageCategory;
private ListCopyJobsRequest(BuilderImpl builder) {
super(builder);
this.nextToken = builder.nextToken;
this.maxResults = builder.maxResults;
this.byResourceArn = builder.byResourceArn;
this.byState = builder.byState;
this.byCreatedBefore = builder.byCreatedBefore;
this.byCreatedAfter = builder.byCreatedAfter;
this.byResourceType = builder.byResourceType;
this.byDestinationVaultArn = builder.byDestinationVaultArn;
this.byAccountId = builder.byAccountId;
this.byCompleteBefore = builder.byCompleteBefore;
this.byCompleteAfter = builder.byCompleteAfter;
this.byParentJobId = builder.byParentJobId;
this.byMessageCategory = builder.byMessageCategory;
}
/**
*
* The next item following a partial list of returned items. For example, if a request is made to return MaxResults
* number of items, NextToken allows you to return more items in your list starting at the location pointed to by
* the next token.
*
*
* @return The next item following a partial list of returned items. For example, if a request is made to return
* MaxResults number of items, NextToken allows you to return more items in your list starting at the
* location pointed to by the next token.
*/
public final String nextToken() {
return nextToken;
}
/**
*
* The maximum number of items to be returned.
*
*
* @return The maximum number of items to be returned.
*/
public final Integer maxResults() {
return maxResults;
}
/**
*
* Returns only copy jobs that match the specified resource Amazon Resource Name (ARN).
*
*
* @return Returns only copy jobs that match the specified resource Amazon Resource Name (ARN).
*/
public final String byResourceArn() {
return byResourceArn;
}
/**
*
* Returns only copy jobs that are in the specified state.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #byState} will
* return {@link CopyJobState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #byStateAsString}.
*
*
* @return Returns only copy jobs that are in the specified state.
* @see CopyJobState
*/
public final CopyJobState byState() {
return CopyJobState.fromValue(byState);
}
/**
*
* Returns only copy jobs that are in the specified state.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #byState} will
* return {@link CopyJobState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #byStateAsString}.
*
*
* @return Returns only copy jobs that are in the specified state.
* @see CopyJobState
*/
public final String byStateAsString() {
return byState;
}
/**
*
* Returns only copy jobs that were created before the specified date.
*
*
* @return Returns only copy jobs that were created before the specified date.
*/
public final Instant byCreatedBefore() {
return byCreatedBefore;
}
/**
*
* Returns only copy jobs that were created after the specified date.
*
*
* @return Returns only copy jobs that were created after the specified date.
*/
public final Instant byCreatedAfter() {
return byCreatedAfter;
}
/**
*
* Returns only backup jobs for the specified resources:
*
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* CloudFormation
for CloudFormation
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* Redshift
for Amazon Redshift
*
*
* -
*
* S3
for Amazon Simple Storage Service (Amazon S3)
*
*
* -
*
* SAP HANA on Amazon EC2
for SAP HANA databases on Amazon Elastic Compute Cloud instances
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* Timestream
for Amazon Timestream
*
*
* -
*
* VirtualMachine
for VMware virtual machines
*
*
*
*
* @return Returns only backup jobs for the specified resources:
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* CloudFormation
for CloudFormation
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* Redshift
for Amazon Redshift
*
*
* -
*
* S3
for Amazon Simple Storage Service (Amazon S3)
*
*
* -
*
* SAP HANA on Amazon EC2
for SAP HANA databases on Amazon Elastic Compute Cloud instances
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* Timestream
for Amazon Timestream
*
*
* -
*
* VirtualMachine
for VMware virtual machines
*
*
*/
public final String byResourceType() {
return byResourceType;
}
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a source backup vault to copy from; for example,
* arn:aws:backup:us-east-1:123456789012:backup-vault:aBackupVault
.
*
*
* @return An Amazon Resource Name (ARN) that uniquely identifies a source backup vault to copy from; for example,
* arn:aws:backup:us-east-1:123456789012:backup-vault:aBackupVault
.
*/
public final String byDestinationVaultArn() {
return byDestinationVaultArn;
}
/**
*
* The account ID to list the jobs from. Returns only copy jobs associated with the specified account ID.
*
*
* @return The account ID to list the jobs from. Returns only copy jobs associated with the specified account ID.
*/
public final String byAccountId() {
return byAccountId;
}
/**
*
* Returns only copy jobs completed before a date expressed in Unix format and Coordinated Universal Time (UTC).
*
*
* @return Returns only copy jobs completed before a date expressed in Unix format and Coordinated Universal Time
* (UTC).
*/
public final Instant byCompleteBefore() {
return byCompleteBefore;
}
/**
*
* Returns only copy jobs completed after a date expressed in Unix format and Coordinated Universal Time (UTC).
*
*
* @return Returns only copy jobs completed after a date expressed in Unix format and Coordinated Universal Time
* (UTC).
*/
public final Instant byCompleteAfter() {
return byCompleteAfter;
}
/**
*
* This is a filter to list child (nested) jobs based on parent job ID.
*
*
* @return This is a filter to list child (nested) jobs based on parent job ID.
*/
public final String byParentJobId() {
return byParentJobId;
}
/**
*
* This is an optional parameter that can be used to filter out jobs with a MessageCategory which matches the value
* you input.
*
*
* Example strings may include AccessDenied
, SUCCESS
, AGGREGATE_ALL
, and
* INVALIDPARAMETERS
.
*
*
* View Monitoring for a list
* of accepted strings.
*
*
* The the value ANY returns count of all message categories.
*
*
* AGGREGATE_ALL
aggregates job counts for all message categories and returns the sum.
*
*
* @return This is an optional parameter that can be used to filter out jobs with a MessageCategory which matches
* the value you input.
*
* Example strings may include AccessDenied
, SUCCESS
, AGGREGATE_ALL
,
* and INVALIDPARAMETERS
.
*
*
* View Monitoring for
* a list of accepted strings.
*
*
* The the value ANY returns count of all message categories.
*
*
* AGGREGATE_ALL
aggregates job counts for all message categories and returns the sum.
*/
public final String byMessageCategory() {
return byMessageCategory;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
hashCode = 31 * hashCode + Objects.hashCode(maxResults());
hashCode = 31 * hashCode + Objects.hashCode(byResourceArn());
hashCode = 31 * hashCode + Objects.hashCode(byStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(byCreatedBefore());
hashCode = 31 * hashCode + Objects.hashCode(byCreatedAfter());
hashCode = 31 * hashCode + Objects.hashCode(byResourceType());
hashCode = 31 * hashCode + Objects.hashCode(byDestinationVaultArn());
hashCode = 31 * hashCode + Objects.hashCode(byAccountId());
hashCode = 31 * hashCode + Objects.hashCode(byCompleteBefore());
hashCode = 31 * hashCode + Objects.hashCode(byCompleteAfter());
hashCode = 31 * hashCode + Objects.hashCode(byParentJobId());
hashCode = 31 * hashCode + Objects.hashCode(byMessageCategory());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ListCopyJobsRequest)) {
return false;
}
ListCopyJobsRequest other = (ListCopyJobsRequest) obj;
return Objects.equals(nextToken(), other.nextToken()) && Objects.equals(maxResults(), other.maxResults())
&& Objects.equals(byResourceArn(), other.byResourceArn())
&& Objects.equals(byStateAsString(), other.byStateAsString())
&& Objects.equals(byCreatedBefore(), other.byCreatedBefore())
&& Objects.equals(byCreatedAfter(), other.byCreatedAfter())
&& Objects.equals(byResourceType(), other.byResourceType())
&& Objects.equals(byDestinationVaultArn(), other.byDestinationVaultArn())
&& Objects.equals(byAccountId(), other.byAccountId())
&& Objects.equals(byCompleteBefore(), other.byCompleteBefore())
&& Objects.equals(byCompleteAfter(), other.byCompleteAfter())
&& Objects.equals(byParentJobId(), other.byParentJobId())
&& Objects.equals(byMessageCategory(), other.byMessageCategory());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ListCopyJobsRequest").add("NextToken", nextToken()).add("MaxResults", maxResults())
.add("ByResourceArn", byResourceArn()).add("ByState", byStateAsString())
.add("ByCreatedBefore", byCreatedBefore()).add("ByCreatedAfter", byCreatedAfter())
.add("ByResourceType", byResourceType()).add("ByDestinationVaultArn", byDestinationVaultArn())
.add("ByAccountId", byAccountId()).add("ByCompleteBefore", byCompleteBefore())
.add("ByCompleteAfter", byCompleteAfter()).add("ByParentJobId", byParentJobId())
.add("ByMessageCategory", byMessageCategory()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "NextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
case "MaxResults":
return Optional.ofNullable(clazz.cast(maxResults()));
case "ByResourceArn":
return Optional.ofNullable(clazz.cast(byResourceArn()));
case "ByState":
return Optional.ofNullable(clazz.cast(byStateAsString()));
case "ByCreatedBefore":
return Optional.ofNullable(clazz.cast(byCreatedBefore()));
case "ByCreatedAfter":
return Optional.ofNullable(clazz.cast(byCreatedAfter()));
case "ByResourceType":
return Optional.ofNullable(clazz.cast(byResourceType()));
case "ByDestinationVaultArn":
return Optional.ofNullable(clazz.cast(byDestinationVaultArn()));
case "ByAccountId":
return Optional.ofNullable(clazz.cast(byAccountId()));
case "ByCompleteBefore":
return Optional.ofNullable(clazz.cast(byCompleteBefore()));
case "ByCompleteAfter":
return Optional.ofNullable(clazz.cast(byCompleteAfter()));
case "ByParentJobId":
return Optional.ofNullable(clazz.cast(byParentJobId()));
case "ByMessageCategory":
return Optional.ofNullable(clazz.cast(byMessageCategory()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("nextToken", NEXT_TOKEN_FIELD);
map.put("maxResults", MAX_RESULTS_FIELD);
map.put("resourceArn", BY_RESOURCE_ARN_FIELD);
map.put("state", BY_STATE_FIELD);
map.put("createdBefore", BY_CREATED_BEFORE_FIELD);
map.put("createdAfter", BY_CREATED_AFTER_FIELD);
map.put("resourceType", BY_RESOURCE_TYPE_FIELD);
map.put("destinationVaultArn", BY_DESTINATION_VAULT_ARN_FIELD);
map.put("accountId", BY_ACCOUNT_ID_FIELD);
map.put("completeBefore", BY_COMPLETE_BEFORE_FIELD);
map.put("completeAfter", BY_COMPLETE_AFTER_FIELD);
map.put("parentJobId", BY_PARENT_JOB_ID_FIELD);
map.put("messageCategory", BY_MESSAGE_CATEGORY_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* CloudFormation
for CloudFormation
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* Redshift
for Amazon Redshift
*
*
* -
*
* S3
for Amazon Simple Storage Service (Amazon S3)
*
*
* -
*
* SAP HANA on Amazon EC2
for SAP HANA databases on Amazon Elastic Compute Cloud instances
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* Timestream
for Amazon Timestream
*
*
* -
*
* VirtualMachine
for VMware virtual machines
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder byResourceType(String byResourceType);
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a source backup vault to copy from; for example,
* arn:aws:backup:us-east-1:123456789012:backup-vault:aBackupVault
.
*
*
* @param byDestinationVaultArn
* An Amazon Resource Name (ARN) that uniquely identifies a source backup vault to copy from; for
* example, arn:aws:backup:us-east-1:123456789012:backup-vault:aBackupVault
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder byDestinationVaultArn(String byDestinationVaultArn);
/**
*
* The account ID to list the jobs from. Returns only copy jobs associated with the specified account ID.
*
*
* @param byAccountId
* The account ID to list the jobs from. Returns only copy jobs associated with the specified account ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder byAccountId(String byAccountId);
/**
*
* Returns only copy jobs completed before a date expressed in Unix format and Coordinated Universal Time (UTC).
*
*
* @param byCompleteBefore
* Returns only copy jobs completed before a date expressed in Unix format and Coordinated Universal Time
* (UTC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder byCompleteBefore(Instant byCompleteBefore);
/**
*
* Returns only copy jobs completed after a date expressed in Unix format and Coordinated Universal Time (UTC).
*
*
* @param byCompleteAfter
* Returns only copy jobs completed after a date expressed in Unix format and Coordinated Universal Time
* (UTC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder byCompleteAfter(Instant byCompleteAfter);
/**
*
* This is a filter to list child (nested) jobs based on parent job ID.
*
*
* @param byParentJobId
* This is a filter to list child (nested) jobs based on parent job ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder byParentJobId(String byParentJobId);
/**
*
* This is an optional parameter that can be used to filter out jobs with a MessageCategory which matches the
* value you input.
*
*
* Example strings may include AccessDenied
, SUCCESS
, AGGREGATE_ALL
, and
* INVALIDPARAMETERS
.
*
*
* View Monitoring for a
* list of accepted strings.
*
*
* The the value ANY returns count of all message categories.
*
*
* AGGREGATE_ALL
aggregates job counts for all message categories and returns the sum.
*
*
* @param byMessageCategory
* This is an optional parameter that can be used to filter out jobs with a MessageCategory which matches
* the value you input.
*
* Example strings may include AccessDenied
, SUCCESS
,
* AGGREGATE_ALL
, and INVALIDPARAMETERS
.
*
*
* View Monitoring
* for a list of accepted strings.
*
*
* The the value ANY returns count of all message categories.
*
*
* AGGREGATE_ALL
aggregates job counts for all message categories and returns the sum.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder byMessageCategory(String byMessageCategory);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends BackupRequest.BuilderImpl implements Builder {
private String nextToken;
private Integer maxResults;
private String byResourceArn;
private String byState;
private Instant byCreatedBefore;
private Instant byCreatedAfter;
private String byResourceType;
private String byDestinationVaultArn;
private String byAccountId;
private Instant byCompleteBefore;
private Instant byCompleteAfter;
private String byParentJobId;
private String byMessageCategory;
private BuilderImpl() {
}
private BuilderImpl(ListCopyJobsRequest model) {
super(model);
nextToken(model.nextToken);
maxResults(model.maxResults);
byResourceArn(model.byResourceArn);
byState(model.byState);
byCreatedBefore(model.byCreatedBefore);
byCreatedAfter(model.byCreatedAfter);
byResourceType(model.byResourceType);
byDestinationVaultArn(model.byDestinationVaultArn);
byAccountId(model.byAccountId);
byCompleteBefore(model.byCompleteBefore);
byCompleteAfter(model.byCompleteAfter);
byParentJobId(model.byParentJobId);
byMessageCategory(model.byMessageCategory);
}
public final String getNextToken() {
return nextToken;
}
public final void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
@Override
public final Builder nextToken(String nextToken) {
this.nextToken = nextToken;
return this;
}
public final Integer getMaxResults() {
return maxResults;
}
public final void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
@Override
public final Builder maxResults(Integer maxResults) {
this.maxResults = maxResults;
return this;
}
public final String getByResourceArn() {
return byResourceArn;
}
public final void setByResourceArn(String byResourceArn) {
this.byResourceArn = byResourceArn;
}
@Override
public final Builder byResourceArn(String byResourceArn) {
this.byResourceArn = byResourceArn;
return this;
}
public final String getByState() {
return byState;
}
public final void setByState(String byState) {
this.byState = byState;
}
@Override
public final Builder byState(String byState) {
this.byState = byState;
return this;
}
@Override
public final Builder byState(CopyJobState byState) {
this.byState(byState == null ? null : byState.toString());
return this;
}
public final Instant getByCreatedBefore() {
return byCreatedBefore;
}
public final void setByCreatedBefore(Instant byCreatedBefore) {
this.byCreatedBefore = byCreatedBefore;
}
@Override
public final Builder byCreatedBefore(Instant byCreatedBefore) {
this.byCreatedBefore = byCreatedBefore;
return this;
}
public final Instant getByCreatedAfter() {
return byCreatedAfter;
}
public final void setByCreatedAfter(Instant byCreatedAfter) {
this.byCreatedAfter = byCreatedAfter;
}
@Override
public final Builder byCreatedAfter(Instant byCreatedAfter) {
this.byCreatedAfter = byCreatedAfter;
return this;
}
public final String getByResourceType() {
return byResourceType;
}
public final void setByResourceType(String byResourceType) {
this.byResourceType = byResourceType;
}
@Override
public final Builder byResourceType(String byResourceType) {
this.byResourceType = byResourceType;
return this;
}
public final String getByDestinationVaultArn() {
return byDestinationVaultArn;
}
public final void setByDestinationVaultArn(String byDestinationVaultArn) {
this.byDestinationVaultArn = byDestinationVaultArn;
}
@Override
public final Builder byDestinationVaultArn(String byDestinationVaultArn) {
this.byDestinationVaultArn = byDestinationVaultArn;
return this;
}
public final String getByAccountId() {
return byAccountId;
}
public final void setByAccountId(String byAccountId) {
this.byAccountId = byAccountId;
}
@Override
public final Builder byAccountId(String byAccountId) {
this.byAccountId = byAccountId;
return this;
}
public final Instant getByCompleteBefore() {
return byCompleteBefore;
}
public final void setByCompleteBefore(Instant byCompleteBefore) {
this.byCompleteBefore = byCompleteBefore;
}
@Override
public final Builder byCompleteBefore(Instant byCompleteBefore) {
this.byCompleteBefore = byCompleteBefore;
return this;
}
public final Instant getByCompleteAfter() {
return byCompleteAfter;
}
public final void setByCompleteAfter(Instant byCompleteAfter) {
this.byCompleteAfter = byCompleteAfter;
}
@Override
public final Builder byCompleteAfter(Instant byCompleteAfter) {
this.byCompleteAfter = byCompleteAfter;
return this;
}
public final String getByParentJobId() {
return byParentJobId;
}
public final void setByParentJobId(String byParentJobId) {
this.byParentJobId = byParentJobId;
}
@Override
public final Builder byParentJobId(String byParentJobId) {
this.byParentJobId = byParentJobId;
return this;
}
public final String getByMessageCategory() {
return byMessageCategory;
}
public final void setByMessageCategory(String byMessageCategory) {
this.byMessageCategory = byMessageCategory;
}
@Override
public final Builder byMessageCategory(String byMessageCategory) {
this.byMessageCategory = byMessageCategory;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public ListCopyJobsRequest build() {
return new ListCopyJobsRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}