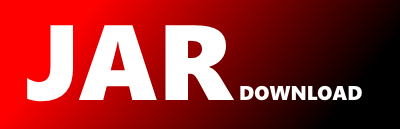
software.amazon.awssdk.services.backup.model.DescribeRestoreJobResponse Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.backup.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeRestoreJobResponse extends BackupResponse implements
ToCopyableBuilder {
private static final SdkField RESTORE_JOB_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeRestoreJobResponse::restoreJobId)).setter(setter(Builder::restoreJobId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RestoreJobId").build()).build();
private static final SdkField RECOVERY_POINT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeRestoreJobResponse::recoveryPointArn)).setter(setter(Builder::recoveryPointArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecoveryPointArn").build()).build();
private static final SdkField CREATION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(DescribeRestoreJobResponse::creationDate)).setter(setter(Builder::creationDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationDate").build()).build();
private static final SdkField COMPLETION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(DescribeRestoreJobResponse::completionDate)).setter(setter(Builder::completionDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompletionDate").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeRestoreJobResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField STATUS_MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeRestoreJobResponse::statusMessage)).setter(setter(Builder::statusMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusMessage").build()).build();
private static final SdkField PERCENT_DONE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeRestoreJobResponse::percentDone)).setter(setter(Builder::percentDone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PercentDone").build()).build();
private static final SdkField BACKUP_SIZE_IN_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.getter(getter(DescribeRestoreJobResponse::backupSizeInBytes)).setter(setter(Builder::backupSizeInBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupSizeInBytes").build()).build();
private static final SdkField IAM_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeRestoreJobResponse::iamRoleArn)).setter(setter(Builder::iamRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IamRoleArn").build()).build();
private static final SdkField EXPECTED_COMPLETION_TIME_MINUTES_FIELD = SdkField
. builder(MarshallingType.LONG)
.getter(getter(DescribeRestoreJobResponse::expectedCompletionTimeMinutes))
.setter(setter(Builder::expectedCompletionTimeMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedCompletionTimeMinutes")
.build()).build();
private static final SdkField CREATED_RESOURCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeRestoreJobResponse::createdResourceArn)).setter(setter(Builder::createdResourceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedResourceArn").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(RESTORE_JOB_ID_FIELD,
RECOVERY_POINT_ARN_FIELD, CREATION_DATE_FIELD, COMPLETION_DATE_FIELD, STATUS_FIELD, STATUS_MESSAGE_FIELD,
PERCENT_DONE_FIELD, BACKUP_SIZE_IN_BYTES_FIELD, IAM_ROLE_ARN_FIELD, EXPECTED_COMPLETION_TIME_MINUTES_FIELD,
CREATED_RESOURCE_ARN_FIELD));
private final String restoreJobId;
private final String recoveryPointArn;
private final Instant creationDate;
private final Instant completionDate;
private final String status;
private final String statusMessage;
private final String percentDone;
private final Long backupSizeInBytes;
private final String iamRoleArn;
private final Long expectedCompletionTimeMinutes;
private final String createdResourceArn;
private DescribeRestoreJobResponse(BuilderImpl builder) {
super(builder);
this.restoreJobId = builder.restoreJobId;
this.recoveryPointArn = builder.recoveryPointArn;
this.creationDate = builder.creationDate;
this.completionDate = builder.completionDate;
this.status = builder.status;
this.statusMessage = builder.statusMessage;
this.percentDone = builder.percentDone;
this.backupSizeInBytes = builder.backupSizeInBytes;
this.iamRoleArn = builder.iamRoleArn;
this.expectedCompletionTimeMinutes = builder.expectedCompletionTimeMinutes;
this.createdResourceArn = builder.createdResourceArn;
}
/**
*
* Uniquely identifies the job that restores a recovery point.
*
*
* @return Uniquely identifies the job that restores a recovery point.
*/
public String restoreJobId() {
return restoreJobId;
}
/**
*
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @return An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*/
public String recoveryPointArn() {
return recoveryPointArn;
}
/**
*
* The date and time that a restore job is created, in Unix format and Coordinated Universal Time (UTC). The value
* of CreationDate
is accurate to milliseconds. For example, the value 1516925490.087 represents
* Friday, January 26, 2018 12:11:30.087 AM.
*
*
* @return The date and time that a restore job is created, in Unix format and Coordinated Universal Time (UTC). The
* value of CreationDate
is accurate to milliseconds. For example, the value 1516925490.087
* represents Friday, January 26, 2018 12:11:30.087 AM.
*/
public Instant creationDate() {
return creationDate;
}
/**
*
* The date and time that a job to restore a recovery point is completed, in Unix format and Coordinated Universal
* Time (UTC). The value of CompletionDate
is accurate to milliseconds. For example, the value
* 1516925490.087 represents Friday, January 26, 2018 12:11:30.087 AM.
*
*
* @return The date and time that a job to restore a recovery point is completed, in Unix format and Coordinated
* Universal Time (UTC). The value of CompletionDate
is accurate to milliseconds. For example,
* the value 1516925490.087 represents Friday, January 26, 2018 12:11:30.087 AM.
*/
public Instant completionDate() {
return completionDate;
}
/**
*
* Status code specifying the state of the job that is initiated by AWS Backup to restore a recovery point.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link RestoreJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return Status code specifying the state of the job that is initiated by AWS Backup to restore a recovery point.
* @see RestoreJobStatus
*/
public RestoreJobStatus status() {
return RestoreJobStatus.fromValue(status);
}
/**
*
* Status code specifying the state of the job that is initiated by AWS Backup to restore a recovery point.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link RestoreJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return Status code specifying the state of the job that is initiated by AWS Backup to restore a recovery point.
* @see RestoreJobStatus
*/
public String statusAsString() {
return status;
}
/**
*
* A detailed message explaining the status of a job to restore a recovery point.
*
*
* @return A detailed message explaining the status of a job to restore a recovery point.
*/
public String statusMessage() {
return statusMessage;
}
/**
*
* Contains an estimated percentage that is complete of a job at the time the job status was queried.
*
*
* @return Contains an estimated percentage that is complete of a job at the time the job status was queried.
*/
public String percentDone() {
return percentDone;
}
/**
*
* The size, in bytes, of the restored resource.
*
*
* @return The size, in bytes, of the restored resource.
*/
public Long backupSizeInBytes() {
return backupSizeInBytes;
}
/**
*
* Specifies the IAM role ARN used to create the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*
*
* @return Specifies the IAM role ARN used to create the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*/
public String iamRoleArn() {
return iamRoleArn;
}
/**
*
* The amount of time in minutes that a job restoring a recovery point is expected to take.
*
*
* @return The amount of time in minutes that a job restoring a recovery point is expected to take.
*/
public Long expectedCompletionTimeMinutes() {
return expectedCompletionTimeMinutes;
}
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a resource whose recovery point is being restored. The
* format of the ARN depends on the resource type of the backed-up resource.
*
*
* @return An Amazon Resource Name (ARN) that uniquely identifies a resource whose recovery point is being restored.
* The format of the ARN depends on the resource type of the backed-up resource.
*/
public String createdResourceArn() {
return createdResourceArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(restoreJobId());
hashCode = 31 * hashCode + Objects.hashCode(recoveryPointArn());
hashCode = 31 * hashCode + Objects.hashCode(creationDate());
hashCode = 31 * hashCode + Objects.hashCode(completionDate());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusMessage());
hashCode = 31 * hashCode + Objects.hashCode(percentDone());
hashCode = 31 * hashCode + Objects.hashCode(backupSizeInBytes());
hashCode = 31 * hashCode + Objects.hashCode(iamRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(expectedCompletionTimeMinutes());
hashCode = 31 * hashCode + Objects.hashCode(createdResourceArn());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeRestoreJobResponse)) {
return false;
}
DescribeRestoreJobResponse other = (DescribeRestoreJobResponse) obj;
return Objects.equals(restoreJobId(), other.restoreJobId())
&& Objects.equals(recoveryPointArn(), other.recoveryPointArn())
&& Objects.equals(creationDate(), other.creationDate())
&& Objects.equals(completionDate(), other.completionDate())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(statusMessage(), other.statusMessage()) && Objects.equals(percentDone(), other.percentDone())
&& Objects.equals(backupSizeInBytes(), other.backupSizeInBytes())
&& Objects.equals(iamRoleArn(), other.iamRoleArn())
&& Objects.equals(expectedCompletionTimeMinutes(), other.expectedCompletionTimeMinutes())
&& Objects.equals(createdResourceArn(), other.createdResourceArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("DescribeRestoreJobResponse").add("RestoreJobId", restoreJobId())
.add("RecoveryPointArn", recoveryPointArn()).add("CreationDate", creationDate())
.add("CompletionDate", completionDate()).add("Status", statusAsString()).add("StatusMessage", statusMessage())
.add("PercentDone", percentDone()).add("BackupSizeInBytes", backupSizeInBytes()).add("IamRoleArn", iamRoleArn())
.add("ExpectedCompletionTimeMinutes", expectedCompletionTimeMinutes())
.add("CreatedResourceArn", createdResourceArn()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RestoreJobId":
return Optional.ofNullable(clazz.cast(restoreJobId()));
case "RecoveryPointArn":
return Optional.ofNullable(clazz.cast(recoveryPointArn()));
case "CreationDate":
return Optional.ofNullable(clazz.cast(creationDate()));
case "CompletionDate":
return Optional.ofNullable(clazz.cast(completionDate()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "StatusMessage":
return Optional.ofNullable(clazz.cast(statusMessage()));
case "PercentDone":
return Optional.ofNullable(clazz.cast(percentDone()));
case "BackupSizeInBytes":
return Optional.ofNullable(clazz.cast(backupSizeInBytes()));
case "IamRoleArn":
return Optional.ofNullable(clazz.cast(iamRoleArn()));
case "ExpectedCompletionTimeMinutes":
return Optional.ofNullable(clazz.cast(expectedCompletionTimeMinutes()));
case "CreatedResourceArn":
return Optional.ofNullable(clazz.cast(createdResourceArn()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function