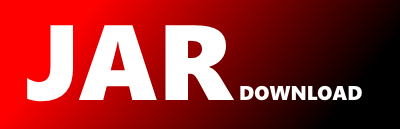
software.amazon.awssdk.services.backup.model.BackupJob Maven / Gradle / Ivy
Show all versions of backup Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.backup.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains detailed information about a backup job.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class BackupJob implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField BACKUP_JOB_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(BackupJob::backupJobId)).setter(setter(Builder::backupJobId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupJobId").build()).build();
private static final SdkField BACKUP_VAULT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(BackupJob::backupVaultName)).setter(setter(Builder::backupVaultName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupVaultName").build()).build();
private static final SdkField BACKUP_VAULT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(BackupJob::backupVaultArn)).setter(setter(Builder::backupVaultArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupVaultArn").build()).build();
private static final SdkField RECOVERY_POINT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(BackupJob::recoveryPointArn)).setter(setter(Builder::recoveryPointArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecoveryPointArn").build()).build();
private static final SdkField RESOURCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(BackupJob::resourceArn)).setter(setter(Builder::resourceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceArn").build()).build();
private static final SdkField CREATION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(BackupJob::creationDate)).setter(setter(Builder::creationDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationDate").build()).build();
private static final SdkField COMPLETION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(BackupJob::completionDate)).setter(setter(Builder::completionDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompletionDate").build()).build();
private static final SdkField STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(BackupJob::stateAsString)).setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State").build()).build();
private static final SdkField STATUS_MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(BackupJob::statusMessage)).setter(setter(Builder::statusMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusMessage").build()).build();
private static final SdkField PERCENT_DONE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(BackupJob::percentDone)).setter(setter(Builder::percentDone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PercentDone").build()).build();
private static final SdkField BACKUP_SIZE_IN_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.getter(getter(BackupJob::backupSizeInBytes)).setter(setter(Builder::backupSizeInBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupSizeInBytes").build()).build();
private static final SdkField IAM_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(BackupJob::iamRoleArn)).setter(setter(Builder::iamRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IamRoleArn").build()).build();
private static final SdkField CREATED_BY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(BackupJob::createdBy))
.setter(setter(Builder::createdBy)).constructor(RecoveryPointCreator::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedBy").build()).build();
private static final SdkField EXPECTED_COMPLETION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(BackupJob::expectedCompletionDate)).setter(setter(Builder::expectedCompletionDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedCompletionDate").build())
.build();
private static final SdkField START_BY_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(BackupJob::startBy)).setter(setter(Builder::startBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartBy").build()).build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(BackupJob::resourceType)).setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceType").build()).build();
private static final SdkField BYTES_TRANSFERRED_FIELD = SdkField. builder(MarshallingType.LONG)
.getter(getter(BackupJob::bytesTransferred)).setter(setter(Builder::bytesTransferred))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BytesTransferred").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BACKUP_JOB_ID_FIELD,
BACKUP_VAULT_NAME_FIELD, BACKUP_VAULT_ARN_FIELD, RECOVERY_POINT_ARN_FIELD, RESOURCE_ARN_FIELD, CREATION_DATE_FIELD,
COMPLETION_DATE_FIELD, STATE_FIELD, STATUS_MESSAGE_FIELD, PERCENT_DONE_FIELD, BACKUP_SIZE_IN_BYTES_FIELD,
IAM_ROLE_ARN_FIELD, CREATED_BY_FIELD, EXPECTED_COMPLETION_DATE_FIELD, START_BY_FIELD, RESOURCE_TYPE_FIELD,
BYTES_TRANSFERRED_FIELD));
private static final long serialVersionUID = 1L;
private final String backupJobId;
private final String backupVaultName;
private final String backupVaultArn;
private final String recoveryPointArn;
private final String resourceArn;
private final Instant creationDate;
private final Instant completionDate;
private final String state;
private final String statusMessage;
private final String percentDone;
private final Long backupSizeInBytes;
private final String iamRoleArn;
private final RecoveryPointCreator createdBy;
private final Instant expectedCompletionDate;
private final Instant startBy;
private final String resourceType;
private final Long bytesTransferred;
private BackupJob(BuilderImpl builder) {
this.backupJobId = builder.backupJobId;
this.backupVaultName = builder.backupVaultName;
this.backupVaultArn = builder.backupVaultArn;
this.recoveryPointArn = builder.recoveryPointArn;
this.resourceArn = builder.resourceArn;
this.creationDate = builder.creationDate;
this.completionDate = builder.completionDate;
this.state = builder.state;
this.statusMessage = builder.statusMessage;
this.percentDone = builder.percentDone;
this.backupSizeInBytes = builder.backupSizeInBytes;
this.iamRoleArn = builder.iamRoleArn;
this.createdBy = builder.createdBy;
this.expectedCompletionDate = builder.expectedCompletionDate;
this.startBy = builder.startBy;
this.resourceType = builder.resourceType;
this.bytesTransferred = builder.bytesTransferred;
}
/**
*
* Uniquely identifies a request to AWS Backup to back up a resource.
*
*
* @return Uniquely identifies a request to AWS Backup to back up a resource.
*/
public String backupJobId() {
return backupJobId;
}
/**
*
* The name of a logical container where backups are stored. Backup vaults are identified by names that are unique
* to the account used to create them and the AWS Region where they are created. They consist of lowercase letters,
* numbers, and hyphens.
*
*
* @return The name of a logical container where backups are stored. Backup vaults are identified by names that are
* unique to the account used to create them and the AWS Region where they are created. They consist of
* lowercase letters, numbers, and hyphens.
*/
public String backupVaultName() {
return backupVaultName;
}
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a backup vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @return An Amazon Resource Name (ARN) that uniquely identifies a backup vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*/
public String backupVaultArn() {
return backupVaultArn;
}
/**
*
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @return An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*/
public String recoveryPointArn() {
return recoveryPointArn;
}
/**
*
* An ARN that uniquely identifies a resource. The format of the ARN depends on the resource type.
*
*
* @return An ARN that uniquely identifies a resource. The format of the ARN depends on the resource type.
*/
public String resourceArn() {
return resourceArn;
}
/**
*
* The date and time a backup job is created, in Unix format and Coordinated Universal Time (UTC). The value of
* CreationDate
is accurate to milliseconds. For example, the value 1516925490.087 represents Friday,
* January 26, 2018 12:11:30.087 AM.
*
*
* @return The date and time a backup job is created, in Unix format and Coordinated Universal Time (UTC). The value
* of CreationDate
is accurate to milliseconds. For example, the value 1516925490.087
* represents Friday, January 26, 2018 12:11:30.087 AM.
*/
public Instant creationDate() {
return creationDate;
}
/**
*
* The date and time a job to create a backup job is completed, in Unix format and Coordinated Universal Time (UTC).
* The value of CompletionDate
is accurate to milliseconds. For example, the value 1516925490.087
* represents Friday, January 26, 2018 12:11:30.087 AM.
*
*
* @return The date and time a job to create a backup job is completed, in Unix format and Coordinated Universal
* Time (UTC). The value of CompletionDate
is accurate to milliseconds. For example, the value
* 1516925490.087 represents Friday, January 26, 2018 12:11:30.087 AM.
*/
public Instant completionDate() {
return completionDate;
}
/**
*
* The current state of a resource recovery point.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link BackupJobState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The current state of a resource recovery point.
* @see BackupJobState
*/
public BackupJobState state() {
return BackupJobState.fromValue(state);
}
/**
*
* The current state of a resource recovery point.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link BackupJobState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The current state of a resource recovery point.
* @see BackupJobState
*/
public String stateAsString() {
return state;
}
/**
*
* A detailed message explaining the status of the job to back up a resource.
*
*
* @return A detailed message explaining the status of the job to back up a resource.
*/
public String statusMessage() {
return statusMessage;
}
/**
*
* Contains an estimated percentage complete of a job at the time the job status was queried.
*
*
* @return Contains an estimated percentage complete of a job at the time the job status was queried.
*/
public String percentDone() {
return percentDone;
}
/**
*
* The size, in bytes, of a backup.
*
*
* @return The size, in bytes, of a backup.
*/
public Long backupSizeInBytes() {
return backupSizeInBytes;
}
/**
*
* Specifies the IAM role ARN used to create the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*
*
* @return Specifies the IAM role ARN used to create the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*/
public String iamRoleArn() {
return iamRoleArn;
}
/**
*
* Contains identifying information about the creation of a backup job, including the BackupPlanArn
,
* BackupPlanId
, BackupPlanVersion
, and BackupRuleId
of the backup plan used
* to create it.
*
*
* @return Contains identifying information about the creation of a backup job, including the
* BackupPlanArn
, BackupPlanId
, BackupPlanVersion
, and
* BackupRuleId
of the backup plan used to create it.
*/
public RecoveryPointCreator createdBy() {
return createdBy;
}
/**
*
* The date and time a job to back up resources is expected to be completed, in Unix format and Coordinated
* Universal Time (UTC). The value of ExpectedCompletionDate
is accurate to milliseconds. For example,
* the value 1516925490.087 represents Friday, January 26, 2018 12:11:30.087 AM.
*
*
* @return The date and time a job to back up resources is expected to be completed, in Unix format and Coordinated
* Universal Time (UTC). The value of ExpectedCompletionDate
is accurate to milliseconds. For
* example, the value 1516925490.087 represents Friday, January 26, 2018 12:11:30.087 AM.
*/
public Instant expectedCompletionDate() {
return expectedCompletionDate;
}
/**
*
* Specifies the time in Unix format and Coordinated Universal Time (UTC) when a backup job must be started before
* it is canceled. The value is calculated by adding the start window to the scheduled time. So if the scheduled
* time were 6:00 PM and the start window is 2 hours, the StartBy
time would be 8:00 PM on the date
* specified. The value of StartBy
is accurate to milliseconds. For example, the value 1516925490.087
* represents Friday, January 26, 2018 12:11:30.087 AM.
*
*
* @return Specifies the time in Unix format and Coordinated Universal Time (UTC) when a backup job must be started
* before it is canceled. The value is calculated by adding the start window to the scheduled time. So if
* the scheduled time were 6:00 PM and the start window is 2 hours, the StartBy
time would be
* 8:00 PM on the date specified. The value of StartBy
is accurate to milliseconds. For
* example, the value 1516925490.087 represents Friday, January 26, 2018 12:11:30.087 AM.
*/
public Instant startBy() {
return startBy;
}
/**
*
* The type of AWS resource to be backed-up; for example, an Amazon Elastic Block Store (Amazon EBS) volume or an
* Amazon Relational Database Service (Amazon RDS) database.
*
*
* @return The type of AWS resource to be backed-up; for example, an Amazon Elastic Block Store (Amazon EBS) volume
* or an Amazon Relational Database Service (Amazon RDS) database.
*/
public String resourceType() {
return resourceType;
}
/**
*
* The size in bytes transferred to a backup vault at the time that the job status was queried.
*
*
* @return The size in bytes transferred to a backup vault at the time that the job status was queried.
*/
public Long bytesTransferred() {
return bytesTransferred;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(backupJobId());
hashCode = 31 * hashCode + Objects.hashCode(backupVaultName());
hashCode = 31 * hashCode + Objects.hashCode(backupVaultArn());
hashCode = 31 * hashCode + Objects.hashCode(recoveryPointArn());
hashCode = 31 * hashCode + Objects.hashCode(resourceArn());
hashCode = 31 * hashCode + Objects.hashCode(creationDate());
hashCode = 31 * hashCode + Objects.hashCode(completionDate());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusMessage());
hashCode = 31 * hashCode + Objects.hashCode(percentDone());
hashCode = 31 * hashCode + Objects.hashCode(backupSizeInBytes());
hashCode = 31 * hashCode + Objects.hashCode(iamRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(createdBy());
hashCode = 31 * hashCode + Objects.hashCode(expectedCompletionDate());
hashCode = 31 * hashCode + Objects.hashCode(startBy());
hashCode = 31 * hashCode + Objects.hashCode(resourceType());
hashCode = 31 * hashCode + Objects.hashCode(bytesTransferred());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BackupJob)) {
return false;
}
BackupJob other = (BackupJob) obj;
return Objects.equals(backupJobId(), other.backupJobId()) && Objects.equals(backupVaultName(), other.backupVaultName())
&& Objects.equals(backupVaultArn(), other.backupVaultArn())
&& Objects.equals(recoveryPointArn(), other.recoveryPointArn())
&& Objects.equals(resourceArn(), other.resourceArn()) && Objects.equals(creationDate(), other.creationDate())
&& Objects.equals(completionDate(), other.completionDate())
&& Objects.equals(stateAsString(), other.stateAsString())
&& Objects.equals(statusMessage(), other.statusMessage()) && Objects.equals(percentDone(), other.percentDone())
&& Objects.equals(backupSizeInBytes(), other.backupSizeInBytes())
&& Objects.equals(iamRoleArn(), other.iamRoleArn()) && Objects.equals(createdBy(), other.createdBy())
&& Objects.equals(expectedCompletionDate(), other.expectedCompletionDate())
&& Objects.equals(startBy(), other.startBy()) && Objects.equals(resourceType(), other.resourceType())
&& Objects.equals(bytesTransferred(), other.bytesTransferred());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("BackupJob").add("BackupJobId", backupJobId()).add("BackupVaultName", backupVaultName())
.add("BackupVaultArn", backupVaultArn()).add("RecoveryPointArn", recoveryPointArn())
.add("ResourceArn", resourceArn()).add("CreationDate", creationDate()).add("CompletionDate", completionDate())
.add("State", stateAsString()).add("StatusMessage", statusMessage()).add("PercentDone", percentDone())
.add("BackupSizeInBytes", backupSizeInBytes()).add("IamRoleArn", iamRoleArn()).add("CreatedBy", createdBy())
.add("ExpectedCompletionDate", expectedCompletionDate()).add("StartBy", startBy())
.add("ResourceType", resourceType()).add("BytesTransferred", bytesTransferred()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "BackupJobId":
return Optional.ofNullable(clazz.cast(backupJobId()));
case "BackupVaultName":
return Optional.ofNullable(clazz.cast(backupVaultName()));
case "BackupVaultArn":
return Optional.ofNullable(clazz.cast(backupVaultArn()));
case "RecoveryPointArn":
return Optional.ofNullable(clazz.cast(recoveryPointArn()));
case "ResourceArn":
return Optional.ofNullable(clazz.cast(resourceArn()));
case "CreationDate":
return Optional.ofNullable(clazz.cast(creationDate()));
case "CompletionDate":
return Optional.ofNullable(clazz.cast(completionDate()));
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "StatusMessage":
return Optional.ofNullable(clazz.cast(statusMessage()));
case "PercentDone":
return Optional.ofNullable(clazz.cast(percentDone()));
case "BackupSizeInBytes":
return Optional.ofNullable(clazz.cast(backupSizeInBytes()));
case "IamRoleArn":
return Optional.ofNullable(clazz.cast(iamRoleArn()));
case "CreatedBy":
return Optional.ofNullable(clazz.cast(createdBy()));
case "ExpectedCompletionDate":
return Optional.ofNullable(clazz.cast(expectedCompletionDate()));
case "StartBy":
return Optional.ofNullable(clazz.cast(startBy()));
case "ResourceType":
return Optional.ofNullable(clazz.cast(resourceType()));
case "BytesTransferred":
return Optional.ofNullable(clazz.cast(bytesTransferred()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function