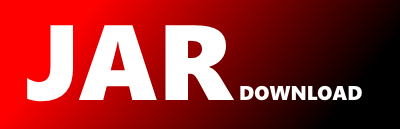
software.amazon.awssdk.services.backupgateway.model.BandwidthRateLimitInterval Maven / Gradle / Ivy
Show all versions of backupgateway Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.backupgateway.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a bandwidth rate limit interval for a gateway. A bandwidth rate limit schedule consists of one or more
* bandwidth rate limit intervals. A bandwidth rate limit interval defines a period of time on one or more days of the
* week, during which bandwidth rate limits are specified for uploading, downloading, or both.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class BandwidthRateLimitInterval implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField AVERAGE_UPLOAD_RATE_LIMIT_IN_BITS_PER_SEC_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("AverageUploadRateLimitInBitsPerSec")
.getter(getter(BandwidthRateLimitInterval::averageUploadRateLimitInBitsPerSec))
.setter(setter(Builder::averageUploadRateLimitInBitsPerSec))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AverageUploadRateLimitInBitsPerSec")
.build()).build();
private static final SdkField> DAYS_OF_WEEK_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DaysOfWeek")
.getter(getter(BandwidthRateLimitInterval::daysOfWeek))
.setter(setter(Builder::daysOfWeek))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DaysOfWeek").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.INTEGER)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField END_HOUR_OF_DAY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("EndHourOfDay").getter(getter(BandwidthRateLimitInterval::endHourOfDay))
.setter(setter(Builder::endHourOfDay))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndHourOfDay").build()).build();
private static final SdkField END_MINUTE_OF_HOUR_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("EndMinuteOfHour").getter(getter(BandwidthRateLimitInterval::endMinuteOfHour))
.setter(setter(Builder::endMinuteOfHour))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndMinuteOfHour").build()).build();
private static final SdkField START_HOUR_OF_DAY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("StartHourOfDay").getter(getter(BandwidthRateLimitInterval::startHourOfDay))
.setter(setter(Builder::startHourOfDay))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartHourOfDay").build()).build();
private static final SdkField START_MINUTE_OF_HOUR_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("StartMinuteOfHour").getter(getter(BandwidthRateLimitInterval::startMinuteOfHour))
.setter(setter(Builder::startMinuteOfHour))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartMinuteOfHour").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
AVERAGE_UPLOAD_RATE_LIMIT_IN_BITS_PER_SEC_FIELD, DAYS_OF_WEEK_FIELD, END_HOUR_OF_DAY_FIELD, END_MINUTE_OF_HOUR_FIELD,
START_HOUR_OF_DAY_FIELD, START_MINUTE_OF_HOUR_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final Long averageUploadRateLimitInBitsPerSec;
private final List daysOfWeek;
private final Integer endHourOfDay;
private final Integer endMinuteOfHour;
private final Integer startHourOfDay;
private final Integer startMinuteOfHour;
private BandwidthRateLimitInterval(BuilderImpl builder) {
this.averageUploadRateLimitInBitsPerSec = builder.averageUploadRateLimitInBitsPerSec;
this.daysOfWeek = builder.daysOfWeek;
this.endHourOfDay = builder.endHourOfDay;
this.endMinuteOfHour = builder.endMinuteOfHour;
this.startHourOfDay = builder.startHourOfDay;
this.startMinuteOfHour = builder.startMinuteOfHour;
}
/**
*
* The average upload rate limit component of the bandwidth rate limit interval, in bits per second. This field does
* not appear in the response if the upload rate limit is not set.
*
*
*
* For Backup Gateway, the minimum value is (Value)
.
*
*
*
* @return The average upload rate limit component of the bandwidth rate limit interval, in bits per second. This
* field does not appear in the response if the upload rate limit is not set.
*
* For Backup Gateway, the minimum value is (Value)
.
*
*/
public final Long averageUploadRateLimitInBitsPerSec() {
return averageUploadRateLimitInBitsPerSec;
}
/**
* For responses, this returns true if the service returned a value for the DaysOfWeek property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasDaysOfWeek() {
return daysOfWeek != null && !(daysOfWeek instanceof SdkAutoConstructList);
}
/**
*
* The days of the week component of the bandwidth rate limit interval, represented as ordinal numbers from 0 to 6,
* where 0 represents Sunday and 6 represents Saturday.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDaysOfWeek} method.
*
*
* @return The days of the week component of the bandwidth rate limit interval, represented as ordinal numbers from
* 0 to 6, where 0 represents Sunday and 6 represents Saturday.
*/
public final List daysOfWeek() {
return daysOfWeek;
}
/**
*
* The hour of the day to end the bandwidth rate limit interval.
*
*
* @return The hour of the day to end the bandwidth rate limit interval.
*/
public final Integer endHourOfDay() {
return endHourOfDay;
}
/**
*
* The minute of the hour to end the bandwidth rate limit interval.
*
*
*
* The bandwidth rate limit interval ends at the end of the minute. To end an interval at the end of an hour, use
* the value 59
.
*
*
*
* @return The minute of the hour to end the bandwidth rate limit interval.
*
* The bandwidth rate limit interval ends at the end of the minute. To end an interval at the end of an
* hour, use the value 59
.
*
*/
public final Integer endMinuteOfHour() {
return endMinuteOfHour;
}
/**
*
* The hour of the day to start the bandwidth rate limit interval.
*
*
* @return The hour of the day to start the bandwidth rate limit interval.
*/
public final Integer startHourOfDay() {
return startHourOfDay;
}
/**
*
* The minute of the hour to start the bandwidth rate limit interval. The interval begins at the start of that
* minute. To begin an interval exactly at the start of the hour, use the value 0
.
*
*
* @return The minute of the hour to start the bandwidth rate limit interval. The interval begins at the start of
* that minute. To begin an interval exactly at the start of the hour, use the value 0
.
*/
public final Integer startMinuteOfHour() {
return startMinuteOfHour;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(averageUploadRateLimitInBitsPerSec());
hashCode = 31 * hashCode + Objects.hashCode(hasDaysOfWeek() ? daysOfWeek() : null);
hashCode = 31 * hashCode + Objects.hashCode(endHourOfDay());
hashCode = 31 * hashCode + Objects.hashCode(endMinuteOfHour());
hashCode = 31 * hashCode + Objects.hashCode(startHourOfDay());
hashCode = 31 * hashCode + Objects.hashCode(startMinuteOfHour());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BandwidthRateLimitInterval)) {
return false;
}
BandwidthRateLimitInterval other = (BandwidthRateLimitInterval) obj;
return Objects.equals(averageUploadRateLimitInBitsPerSec(), other.averageUploadRateLimitInBitsPerSec())
&& hasDaysOfWeek() == other.hasDaysOfWeek() && Objects.equals(daysOfWeek(), other.daysOfWeek())
&& Objects.equals(endHourOfDay(), other.endHourOfDay())
&& Objects.equals(endMinuteOfHour(), other.endMinuteOfHour())
&& Objects.equals(startHourOfDay(), other.startHourOfDay())
&& Objects.equals(startMinuteOfHour(), other.startMinuteOfHour());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("BandwidthRateLimitInterval")
.add("AverageUploadRateLimitInBitsPerSec", averageUploadRateLimitInBitsPerSec())
.add("DaysOfWeek", hasDaysOfWeek() ? daysOfWeek() : null).add("EndHourOfDay", endHourOfDay())
.add("EndMinuteOfHour", endMinuteOfHour()).add("StartHourOfDay", startHourOfDay())
.add("StartMinuteOfHour", startMinuteOfHour()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AverageUploadRateLimitInBitsPerSec":
return Optional.ofNullable(clazz.cast(averageUploadRateLimitInBitsPerSec()));
case "DaysOfWeek":
return Optional.ofNullable(clazz.cast(daysOfWeek()));
case "EndHourOfDay":
return Optional.ofNullable(clazz.cast(endHourOfDay()));
case "EndMinuteOfHour":
return Optional.ofNullable(clazz.cast(endMinuteOfHour()));
case "StartHourOfDay":
return Optional.ofNullable(clazz.cast(startHourOfDay()));
case "StartMinuteOfHour":
return Optional.ofNullable(clazz.cast(startMinuteOfHour()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("AverageUploadRateLimitInBitsPerSec", AVERAGE_UPLOAD_RATE_LIMIT_IN_BITS_PER_SEC_FIELD);
map.put("DaysOfWeek", DAYS_OF_WEEK_FIELD);
map.put("EndHourOfDay", END_HOUR_OF_DAY_FIELD);
map.put("EndMinuteOfHour", END_MINUTE_OF_HOUR_FIELD);
map.put("StartHourOfDay", START_HOUR_OF_DAY_FIELD);
map.put("StartMinuteOfHour", START_MINUTE_OF_HOUR_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function