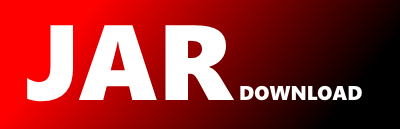
software.amazon.awssdk.services.bedrockagent.model.CreateAgentActionGroupRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.bedrockagent.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateAgentActionGroupRequest extends BedrockAgentRequest implements
ToCopyableBuilder {
private static final SdkField ACTION_GROUP_EXECUTOR_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("actionGroupExecutor")
.getter(getter(CreateAgentActionGroupRequest::actionGroupExecutor)).setter(setter(Builder::actionGroupExecutor))
.constructor(ActionGroupExecutor::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("actionGroupExecutor").build())
.build();
private static final SdkField ACTION_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("actionGroupName").getter(getter(CreateAgentActionGroupRequest::actionGroupName))
.setter(setter(Builder::actionGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("actionGroupName").build()).build();
private static final SdkField ACTION_GROUP_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("actionGroupState").getter(getter(CreateAgentActionGroupRequest::actionGroupStateAsString))
.setter(setter(Builder::actionGroupState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("actionGroupState").build()).build();
private static final SdkField AGENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("agentId").getter(getter(CreateAgentActionGroupRequest::agentId)).setter(setter(Builder::agentId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("agentId").build()).build();
private static final SdkField AGENT_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("agentVersion").getter(getter(CreateAgentActionGroupRequest::agentVersion))
.setter(setter(Builder::agentVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("agentVersion").build()).build();
private static final SdkField API_SCHEMA_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("apiSchema").getter(getter(CreateAgentActionGroupRequest::apiSchema)).setter(setter(Builder::apiSchema))
.constructor(APISchema::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("apiSchema").build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("clientToken")
.getter(getter(CreateAgentActionGroupRequest::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clientToken").build(),
DefaultValueTrait.idempotencyToken()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("description").getter(getter(CreateAgentActionGroupRequest::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField FUNCTION_SCHEMA_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("functionSchema")
.getter(getter(CreateAgentActionGroupRequest::functionSchema)).setter(setter(Builder::functionSchema))
.constructor(FunctionSchema::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("functionSchema").build()).build();
private static final SdkField PARENT_ACTION_GROUP_SIGNATURE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("parentActionGroupSignature")
.getter(getter(CreateAgentActionGroupRequest::parentActionGroupSignatureAsString))
.setter(setter(Builder::parentActionGroupSignature))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("parentActionGroupSignature").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACTION_GROUP_EXECUTOR_FIELD,
ACTION_GROUP_NAME_FIELD, ACTION_GROUP_STATE_FIELD, AGENT_ID_FIELD, AGENT_VERSION_FIELD, API_SCHEMA_FIELD,
CLIENT_TOKEN_FIELD, DESCRIPTION_FIELD, FUNCTION_SCHEMA_FIELD, PARENT_ACTION_GROUP_SIGNATURE_FIELD));
private final ActionGroupExecutor actionGroupExecutor;
private final String actionGroupName;
private final String actionGroupState;
private final String agentId;
private final String agentVersion;
private final APISchema apiSchema;
private final String clientToken;
private final String description;
private final FunctionSchema functionSchema;
private final String parentActionGroupSignature;
private CreateAgentActionGroupRequest(BuilderImpl builder) {
super(builder);
this.actionGroupExecutor = builder.actionGroupExecutor;
this.actionGroupName = builder.actionGroupName;
this.actionGroupState = builder.actionGroupState;
this.agentId = builder.agentId;
this.agentVersion = builder.agentVersion;
this.apiSchema = builder.apiSchema;
this.clientToken = builder.clientToken;
this.description = builder.description;
this.functionSchema = builder.functionSchema;
this.parentActionGroupSignature = builder.parentActionGroupSignature;
}
/**
*
* The Amazon Resource Name (ARN) of the Lambda function containing the business logic that is carried out upon
* invoking the action or the custom control method for handling the information elicited from the user.
*
*
* @return The Amazon Resource Name (ARN) of the Lambda function containing the business logic that is carried out
* upon invoking the action or the custom control method for handling the information elicited from the
* user.
*/
public final ActionGroupExecutor actionGroupExecutor() {
return actionGroupExecutor;
}
/**
*
* The name to give the action group.
*
*
* @return The name to give the action group.
*/
public final String actionGroupName() {
return actionGroupName;
}
/**
*
* Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent
* request.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #actionGroupState}
* will return {@link ActionGroupState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #actionGroupStateAsString}.
*
*
* @return Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent request.
* @see ActionGroupState
*/
public final ActionGroupState actionGroupState() {
return ActionGroupState.fromValue(actionGroupState);
}
/**
*
* Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent
* request.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #actionGroupState}
* will return {@link ActionGroupState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #actionGroupStateAsString}.
*
*
* @return Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent request.
* @see ActionGroupState
*/
public final String actionGroupStateAsString() {
return actionGroupState;
}
/**
*
* The unique identifier of the agent for which to create the action group.
*
*
* @return The unique identifier of the agent for which to create the action group.
*/
public final String agentId() {
return agentId;
}
/**
*
* The version of the agent for which to create the action group.
*
*
* @return The version of the agent for which to create the action group.
*/
public final String agentVersion() {
return agentVersion;
}
/**
*
* Contains either details about the S3 object containing the OpenAPI schema for the action group or the JSON or
* YAML-formatted payload defining the schema. For more information, see Action group OpenAPI
* schemas.
*
*
* @return Contains either details about the S3 object containing the OpenAPI schema for the action group or the
* JSON or YAML-formatted payload defining the schema. For more information, see Action group OpenAPI
* schemas.
*/
public final APISchema apiSchema() {
return apiSchema;
}
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*
* @return A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If
* this token matches a previous request, Amazon Bedrock ignores the request, but does not return an error.
* For more information, see Ensuring
* idempotency.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* A description of the action group.
*
*
* @return A description of the action group.
*/
public final String description() {
return description;
}
/**
*
* Contains details about the function schema for the action group or the JSON or YAML-formatted payload defining
* the schema.
*
*
* @return Contains details about the function schema for the action group or the JSON or YAML-formatted payload
* defining the schema.
*/
public final FunctionSchema functionSchema() {
return functionSchema;
}
/**
*
* To allow your agent to request the user for additional information when trying to complete a task, set this field
* to AMAZON.UserInput
. You must leave the description
, apiSchema
, and
* actionGroupExecutor
fields blank for this action group.
*
*
* To allow your agent to generate, run, and troubleshoot code when trying to complete a task, set this field to
* AMAZON.CodeInterpreter
. You must leave the description
, apiSchema
, and
* actionGroupExecutor
fields blank for this action group.
*
*
* During orchestration, if your agent determines that it needs to invoke an API in an action group, but doesn't
* have enough information to complete the API request, it will invoke this action group instead and return an Observation
* reprompting the user for more information.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #parentActionGroupSignature} will return {@link ActionGroupSignature#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #parentActionGroupSignatureAsString}.
*
*
* @return To allow your agent to request the user for additional information when trying to complete a task, set
* this field to AMAZON.UserInput
. You must leave the description
,
* apiSchema
, and actionGroupExecutor
fields blank for this action group.
*
* To allow your agent to generate, run, and troubleshoot code when trying to complete a task, set this
* field to AMAZON.CodeInterpreter
. You must leave the description
,
* apiSchema
, and actionGroupExecutor
fields blank for this action group.
*
*
* During orchestration, if your agent determines that it needs to invoke an API in an action group, but
* doesn't have enough information to complete the API request, it will invoke this action group instead and
* return an Observation reprompting the user for more information.
* @see ActionGroupSignature
*/
public final ActionGroupSignature parentActionGroupSignature() {
return ActionGroupSignature.fromValue(parentActionGroupSignature);
}
/**
*
* To allow your agent to request the user for additional information when trying to complete a task, set this field
* to AMAZON.UserInput
. You must leave the description
, apiSchema
, and
* actionGroupExecutor
fields blank for this action group.
*
*
* To allow your agent to generate, run, and troubleshoot code when trying to complete a task, set this field to
* AMAZON.CodeInterpreter
. You must leave the description
, apiSchema
, and
* actionGroupExecutor
fields blank for this action group.
*
*
* During orchestration, if your agent determines that it needs to invoke an API in an action group, but doesn't
* have enough information to complete the API request, it will invoke this action group instead and return an Observation
* reprompting the user for more information.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #parentActionGroupSignature} will return {@link ActionGroupSignature#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #parentActionGroupSignatureAsString}.
*
*
* @return To allow your agent to request the user for additional information when trying to complete a task, set
* this field to AMAZON.UserInput
. You must leave the description
,
* apiSchema
, and actionGroupExecutor
fields blank for this action group.
*
* To allow your agent to generate, run, and troubleshoot code when trying to complete a task, set this
* field to AMAZON.CodeInterpreter
. You must leave the description
,
* apiSchema
, and actionGroupExecutor
fields blank for this action group.
*
*
* During orchestration, if your agent determines that it needs to invoke an API in an action group, but
* doesn't have enough information to complete the API request, it will invoke this action group instead and
* return an Observation reprompting the user for more information.
* @see ActionGroupSignature
*/
public final String parentActionGroupSignatureAsString() {
return parentActionGroupSignature;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(actionGroupExecutor());
hashCode = 31 * hashCode + Objects.hashCode(actionGroupName());
hashCode = 31 * hashCode + Objects.hashCode(actionGroupStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(agentId());
hashCode = 31 * hashCode + Objects.hashCode(agentVersion());
hashCode = 31 * hashCode + Objects.hashCode(apiSchema());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(functionSchema());
hashCode = 31 * hashCode + Objects.hashCode(parentActionGroupSignatureAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateAgentActionGroupRequest)) {
return false;
}
CreateAgentActionGroupRequest other = (CreateAgentActionGroupRequest) obj;
return Objects.equals(actionGroupExecutor(), other.actionGroupExecutor())
&& Objects.equals(actionGroupName(), other.actionGroupName())
&& Objects.equals(actionGroupStateAsString(), other.actionGroupStateAsString())
&& Objects.equals(agentId(), other.agentId()) && Objects.equals(agentVersion(), other.agentVersion())
&& Objects.equals(apiSchema(), other.apiSchema()) && Objects.equals(clientToken(), other.clientToken())
&& Objects.equals(description(), other.description()) && Objects.equals(functionSchema(), other.functionSchema())
&& Objects.equals(parentActionGroupSignatureAsString(), other.parentActionGroupSignatureAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateAgentActionGroupRequest").add("ActionGroupExecutor", actionGroupExecutor())
.add("ActionGroupName", actionGroupName()).add("ActionGroupState", actionGroupStateAsString())
.add("AgentId", agentId()).add("AgentVersion", agentVersion()).add("ApiSchema", apiSchema())
.add("ClientToken", clientToken()).add("Description", description()).add("FunctionSchema", functionSchema())
.add("ParentActionGroupSignature", parentActionGroupSignatureAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "actionGroupExecutor":
return Optional.ofNullable(clazz.cast(actionGroupExecutor()));
case "actionGroupName":
return Optional.ofNullable(clazz.cast(actionGroupName()));
case "actionGroupState":
return Optional.ofNullable(clazz.cast(actionGroupStateAsString()));
case "agentId":
return Optional.ofNullable(clazz.cast(agentId()));
case "agentVersion":
return Optional.ofNullable(clazz.cast(agentVersion()));
case "apiSchema":
return Optional.ofNullable(clazz.cast(apiSchema()));
case "clientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "functionSchema":
return Optional.ofNullable(clazz.cast(functionSchema()));
case "parentActionGroupSignature":
return Optional.ofNullable(clazz.cast(parentActionGroupSignatureAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function