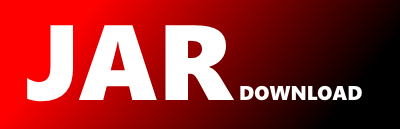
software.amazon.awssdk.services.bedrockagent.model.ParsingConfiguration Maven / Gradle / Ivy
Show all versions of bedrockagent Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.bedrockagent.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Settings for parsing document contents. By default, the service converts the contents of each document into text
* before splitting it into chunks. To improve processing of PDF files with tables and images, you can configure the
* data source to convert the pages of text into images and use a model to describe the contents of each page.
*
*
* To use a model to parse PDF documents, set the parsing strategy to BEDROCK_FOUNDATION_MODEL
and specify
* the model or inference
* profile to use by ARN. You can also override the default parsing prompt with instructions for how to interpret
* images and tables in your documents. The following models are supported.
*
*
* -
*
* Anthropic Claude 3 Sonnet - anthropic.claude-3-sonnet-20240229-v1:0
*
*
* -
*
* Anthropic Claude 3 Haiku - anthropic.claude-3-haiku-20240307-v1:0
*
*
*
*
* You can get the ARN of a model with the ListFoundationModels
* action. Standard model usage charges apply for the foundation model parsing strategy.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ParsingConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField BEDROCK_FOUNDATION_MODEL_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("bedrockFoundationModelConfiguration")
.getter(getter(ParsingConfiguration::bedrockFoundationModelConfiguration))
.setter(setter(Builder::bedrockFoundationModelConfiguration))
.constructor(BedrockFoundationModelConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("bedrockFoundationModelConfiguration").build()).build();
private static final SdkField PARSING_STRATEGY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("parsingStrategy").getter(getter(ParsingConfiguration::parsingStrategyAsString))
.setter(setter(Builder::parsingStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("parsingStrategy").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
BEDROCK_FOUNDATION_MODEL_CONFIGURATION_FIELD, PARSING_STRATEGY_FIELD));
private static final long serialVersionUID = 1L;
private final BedrockFoundationModelConfiguration bedrockFoundationModelConfiguration;
private final String parsingStrategy;
private ParsingConfiguration(BuilderImpl builder) {
this.bedrockFoundationModelConfiguration = builder.bedrockFoundationModelConfiguration;
this.parsingStrategy = builder.parsingStrategy;
}
/**
*
* Settings for a foundation model used to parse documents for a data source.
*
*
* @return Settings for a foundation model used to parse documents for a data source.
*/
public final BedrockFoundationModelConfiguration bedrockFoundationModelConfiguration() {
return bedrockFoundationModelConfiguration;
}
/**
*
* The parsing strategy for the data source.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #parsingStrategy}
* will return {@link ParsingStrategy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #parsingStrategyAsString}.
*
*
* @return The parsing strategy for the data source.
* @see ParsingStrategy
*/
public final ParsingStrategy parsingStrategy() {
return ParsingStrategy.fromValue(parsingStrategy);
}
/**
*
* The parsing strategy for the data source.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #parsingStrategy}
* will return {@link ParsingStrategy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #parsingStrategyAsString}.
*
*
* @return The parsing strategy for the data source.
* @see ParsingStrategy
*/
public final String parsingStrategyAsString() {
return parsingStrategy;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(bedrockFoundationModelConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(parsingStrategyAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ParsingConfiguration)) {
return false;
}
ParsingConfiguration other = (ParsingConfiguration) obj;
return Objects.equals(bedrockFoundationModelConfiguration(), other.bedrockFoundationModelConfiguration())
&& Objects.equals(parsingStrategyAsString(), other.parsingStrategyAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ParsingConfiguration")
.add("BedrockFoundationModelConfiguration", bedrockFoundationModelConfiguration())
.add("ParsingStrategy", parsingStrategyAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "bedrockFoundationModelConfiguration":
return Optional.ofNullable(clazz.cast(bedrockFoundationModelConfiguration()));
case "parsingStrategy":
return Optional.ofNullable(clazz.cast(parsingStrategyAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function