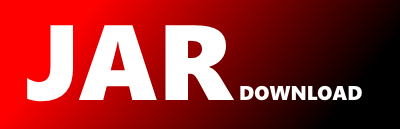
software.amazon.awssdk.services.bedrockruntime.BedrockRuntimeClient Maven / Gradle / Ivy
Show all versions of bedrockruntime Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.bedrockruntime;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.bedrockruntime.model.AccessDeniedException;
import software.amazon.awssdk.services.bedrockruntime.model.ApplyGuardrailRequest;
import software.amazon.awssdk.services.bedrockruntime.model.ApplyGuardrailResponse;
import software.amazon.awssdk.services.bedrockruntime.model.BedrockRuntimeException;
import software.amazon.awssdk.services.bedrockruntime.model.ConverseRequest;
import software.amazon.awssdk.services.bedrockruntime.model.ConverseResponse;
import software.amazon.awssdk.services.bedrockruntime.model.InternalServerException;
import software.amazon.awssdk.services.bedrockruntime.model.InvokeModelRequest;
import software.amazon.awssdk.services.bedrockruntime.model.InvokeModelResponse;
import software.amazon.awssdk.services.bedrockruntime.model.ModelErrorException;
import software.amazon.awssdk.services.bedrockruntime.model.ModelNotReadyException;
import software.amazon.awssdk.services.bedrockruntime.model.ModelTimeoutException;
import software.amazon.awssdk.services.bedrockruntime.model.ResourceNotFoundException;
import software.amazon.awssdk.services.bedrockruntime.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.bedrockruntime.model.ServiceUnavailableException;
import software.amazon.awssdk.services.bedrockruntime.model.ThrottlingException;
import software.amazon.awssdk.services.bedrockruntime.model.ValidationException;
/**
* Service client for accessing Amazon Bedrock Runtime. This can be created using the static {@link #builder()} method.
*
*
* Describes the API operations for running inference using Amazon Bedrock models.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface BedrockRuntimeClient extends AwsClient {
String SERVICE_NAME = "bedrock";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "bedrock-runtime";
/**
*
* The action to apply a guardrail.
*
*
* @param applyGuardrailRequest
* @return Result of the ApplyGuardrail operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ResourceNotFoundException
* The specified resource ARN was not found. Check the ARN and try your request again.
* @throws ThrottlingException
* Your request was throttled because of service-wide limitations. Resubmit your request later or in a
* different region. You can also purchase Provisioned
* Throughput to increase the rate or number of tokens you can process.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ServiceQuotaExceededException
* Your request exceeds the service quota for your account. You can view your quotas at Viewing service
* quotas. You can resubmit your request later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BedrockRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BedrockRuntimeClient.ApplyGuardrail
* @see AWS API Documentation
*/
default ApplyGuardrailResponse applyGuardrail(ApplyGuardrailRequest applyGuardrailRequest) throws AccessDeniedException,
ResourceNotFoundException, ThrottlingException, InternalServerException, ValidationException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, BedrockRuntimeException {
throw new UnsupportedOperationException();
}
/**
*
* The action to apply a guardrail.
*
*
*
* This is a convenience which creates an instance of the {@link ApplyGuardrailRequest.Builder} avoiding the need to
* create one manually via {@link ApplyGuardrailRequest#builder()}
*
*
* @param applyGuardrailRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.bedrockruntime.model.ApplyGuardrailRequest.Builder} to create a
* request.
* @return Result of the ApplyGuardrail operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ResourceNotFoundException
* The specified resource ARN was not found. Check the ARN and try your request again.
* @throws ThrottlingException
* Your request was throttled because of service-wide limitations. Resubmit your request later or in a
* different region. You can also purchase Provisioned
* Throughput to increase the rate or number of tokens you can process.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ServiceQuotaExceededException
* Your request exceeds the service quota for your account. You can view your quotas at Viewing service
* quotas. You can resubmit your request later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BedrockRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BedrockRuntimeClient.ApplyGuardrail
* @see AWS API Documentation
*/
default ApplyGuardrailResponse applyGuardrail(Consumer applyGuardrailRequest)
throws AccessDeniedException, ResourceNotFoundException, ThrottlingException, InternalServerException,
ValidationException, ServiceQuotaExceededException, AwsServiceException, SdkClientException, BedrockRuntimeException {
return applyGuardrail(ApplyGuardrailRequest.builder().applyMutation(applyGuardrailRequest).build());
}
/**
*
* Sends messages to the specified Amazon Bedrock model. Converse
provides a consistent interface that
* works with all models that support messages. This allows you to write code once and use it with different models.
* If a model has unique inference parameters, you can also pass those unique parameters to the model.
*
*
* Amazon Bedrock doesn't store any text, images, or documents that you provide as content. The data is only used to
* generate the response.
*
*
* For information about the Converse API, see Use the Converse API in the Amazon Bedrock User Guide.
* To use a guardrail, see Use a guardrail with the Converse API in the Amazon Bedrock User Guide. To
* use a tool with a model, see Tool use (Function calling) in the Amazon Bedrock User Guide
*
*
* For example code, see Converse API examples in the Amazon Bedrock User Guide.
*
*
* This operation requires permission for the bedrock:InvokeModel
action.
*
*
* @param converseRequest
* @return Result of the Converse operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ResourceNotFoundException
* The specified resource ARN was not found. Check the ARN and try your request again.
* @throws ThrottlingException
* Your request was throttled because of service-wide limitations. Resubmit your request later or in a
* different region. You can also purchase Provisioned
* Throughput to increase the rate or number of tokens you can process.
* @throws ModelTimeoutException
* The request took too long to process. Processing time exceeded the model timeout length.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ServiceUnavailableException
* The service isn't currently available. Try again later.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ModelNotReadyException
* The model specified in the request is not ready to serve inference requests.
* @throws ModelErrorException
* The request failed due to an error while processing the model.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BedrockRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BedrockRuntimeClient.Converse
* @see AWS API
* Documentation
*/
default ConverseResponse converse(ConverseRequest converseRequest) throws AccessDeniedException, ResourceNotFoundException,
ThrottlingException, ModelTimeoutException, InternalServerException, ServiceUnavailableException,
ValidationException, ModelNotReadyException, ModelErrorException, AwsServiceException, SdkClientException,
BedrockRuntimeException {
throw new UnsupportedOperationException();
}
/**
*
* Sends messages to the specified Amazon Bedrock model. Converse
provides a consistent interface that
* works with all models that support messages. This allows you to write code once and use it with different models.
* If a model has unique inference parameters, you can also pass those unique parameters to the model.
*
*
* Amazon Bedrock doesn't store any text, images, or documents that you provide as content. The data is only used to
* generate the response.
*
*
* For information about the Converse API, see Use the Converse API in the Amazon Bedrock User Guide.
* To use a guardrail, see Use a guardrail with the Converse API in the Amazon Bedrock User Guide. To
* use a tool with a model, see Tool use (Function calling) in the Amazon Bedrock User Guide
*
*
* For example code, see Converse API examples in the Amazon Bedrock User Guide.
*
*
* This operation requires permission for the bedrock:InvokeModel
action.
*
*
*
* This is a convenience which creates an instance of the {@link ConverseRequest.Builder} avoiding the need to
* create one manually via {@link ConverseRequest#builder()}
*
*
* @param converseRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.bedrockruntime.model.ConverseRequest.Builder} to create a request.
* @return Result of the Converse operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ResourceNotFoundException
* The specified resource ARN was not found. Check the ARN and try your request again.
* @throws ThrottlingException
* Your request was throttled because of service-wide limitations. Resubmit your request later or in a
* different region. You can also purchase Provisioned
* Throughput to increase the rate or number of tokens you can process.
* @throws ModelTimeoutException
* The request took too long to process. Processing time exceeded the model timeout length.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ServiceUnavailableException
* The service isn't currently available. Try again later.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ModelNotReadyException
* The model specified in the request is not ready to serve inference requests.
* @throws ModelErrorException
* The request failed due to an error while processing the model.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BedrockRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BedrockRuntimeClient.Converse
* @see AWS API
* Documentation
*/
default ConverseResponse converse(Consumer converseRequest) throws AccessDeniedException,
ResourceNotFoundException, ThrottlingException, ModelTimeoutException, InternalServerException,
ServiceUnavailableException, ValidationException, ModelNotReadyException, ModelErrorException, AwsServiceException,
SdkClientException, BedrockRuntimeException {
return converse(ConverseRequest.builder().applyMutation(converseRequest).build());
}
/**
*
* Invokes the specified Amazon Bedrock model to run inference using the prompt and inference parameters provided in
* the request body. You use model inference to generate text, images, and embeddings.
*
*
* For example code, see Invoke model code examples in the Amazon Bedrock User Guide.
*
*
* This operation requires permission for the bedrock:InvokeModel
action.
*
*
* @param invokeModelRequest
* @return Result of the InvokeModel operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ResourceNotFoundException
* The specified resource ARN was not found. Check the ARN and try your request again.
* @throws ThrottlingException
* Your request was throttled because of service-wide limitations. Resubmit your request later or in a
* different region. You can also purchase Provisioned
* Throughput to increase the rate or number of tokens you can process.
* @throws ModelTimeoutException
* The request took too long to process. Processing time exceeded the model timeout length.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ServiceUnavailableException
* The service isn't currently available. Try again later.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ModelNotReadyException
* The model specified in the request is not ready to serve inference requests.
* @throws ServiceQuotaExceededException
* Your request exceeds the service quota for your account. You can view your quotas at Viewing service
* quotas. You can resubmit your request later.
* @throws ModelErrorException
* The request failed due to an error while processing the model.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BedrockRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BedrockRuntimeClient.InvokeModel
* @see AWS
* API Documentation
*/
default InvokeModelResponse invokeModel(InvokeModelRequest invokeModelRequest) throws AccessDeniedException,
ResourceNotFoundException, ThrottlingException, ModelTimeoutException, InternalServerException,
ServiceUnavailableException, ValidationException, ModelNotReadyException, ServiceQuotaExceededException,
ModelErrorException, AwsServiceException, SdkClientException, BedrockRuntimeException {
throw new UnsupportedOperationException();
}
/**
*
* Invokes the specified Amazon Bedrock model to run inference using the prompt and inference parameters provided in
* the request body. You use model inference to generate text, images, and embeddings.
*
*
* For example code, see Invoke model code examples in the Amazon Bedrock User Guide.
*
*
* This operation requires permission for the bedrock:InvokeModel
action.
*
*
*
* This is a convenience which creates an instance of the {@link InvokeModelRequest.Builder} avoiding the need to
* create one manually via {@link InvokeModelRequest#builder()}
*
*
* @param invokeModelRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.bedrockruntime.model.InvokeModelRequest.Builder} to create a
* request.
* @return Result of the InvokeModel operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ResourceNotFoundException
* The specified resource ARN was not found. Check the ARN and try your request again.
* @throws ThrottlingException
* Your request was throttled because of service-wide limitations. Resubmit your request later or in a
* different region. You can also purchase Provisioned
* Throughput to increase the rate or number of tokens you can process.
* @throws ModelTimeoutException
* The request took too long to process. Processing time exceeded the model timeout length.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ServiceUnavailableException
* The service isn't currently available. Try again later.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ModelNotReadyException
* The model specified in the request is not ready to serve inference requests.
* @throws ServiceQuotaExceededException
* Your request exceeds the service quota for your account. You can view your quotas at Viewing service
* quotas. You can resubmit your request later.
* @throws ModelErrorException
* The request failed due to an error while processing the model.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BedrockRuntimeException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BedrockRuntimeClient.InvokeModel
* @see AWS
* API Documentation
*/
default InvokeModelResponse invokeModel(Consumer invokeModelRequest)
throws AccessDeniedException, ResourceNotFoundException, ThrottlingException, ModelTimeoutException,
InternalServerException, ServiceUnavailableException, ValidationException, ModelNotReadyException,
ServiceQuotaExceededException, ModelErrorException, AwsServiceException, SdkClientException, BedrockRuntimeException {
return invokeModel(InvokeModelRequest.builder().applyMutation(invokeModelRequest).build());
}
/**
* Create a {@link BedrockRuntimeClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static BedrockRuntimeClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link BedrockRuntimeClient}.
*/
static BedrockRuntimeClientBuilder builder() {
return new DefaultBedrockRuntimeClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default BedrockRuntimeServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}