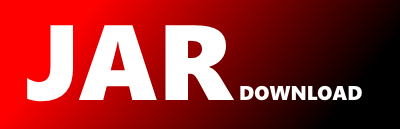
software.amazon.awssdk.services.bedrockruntime.model.InvokeModelWithResponseStreamRequest Maven / Gradle / Ivy
Show all versions of bedrockruntime Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.bedrockruntime.model;
import java.nio.ByteBuffer;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkBytes;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.PayloadTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class InvokeModelWithResponseStreamRequest extends BedrockRuntimeRequest implements
ToCopyableBuilder {
private static final SdkField BODY_FIELD = SdkField
. builder(MarshallingType.SDK_BYTES)
.memberName("body")
.getter(getter(InvokeModelWithResponseStreamRequest::body))
.setter(setter(Builder::body))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("body").build(),
PayloadTrait.create()).build();
private static final SdkField CONTENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("contentType").getter(getter(InvokeModelWithResponseStreamRequest::contentType))
.setter(setter(Builder::contentType))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Content-Type").build()).build();
private static final SdkField ACCEPT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("accept")
.getter(getter(InvokeModelWithResponseStreamRequest::accept)).setter(setter(Builder::accept))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("X-Amzn-Bedrock-Accept").build())
.build();
private static final SdkField MODEL_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("modelId").getter(getter(InvokeModelWithResponseStreamRequest::modelId)).setter(setter(Builder::modelId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("modelId").build()).build();
private static final SdkField TRACE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("trace")
.getter(getter(InvokeModelWithResponseStreamRequest::traceAsString)).setter(setter(Builder::trace))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("X-Amzn-Bedrock-Trace").build())
.build();
private static final SdkField GUARDRAIL_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("guardrailIdentifier")
.getter(getter(InvokeModelWithResponseStreamRequest::guardrailIdentifier))
.setter(setter(Builder::guardrailIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("X-Amzn-Bedrock-GuardrailIdentifier")
.build()).build();
private static final SdkField GUARDRAIL_VERSION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("guardrailVersion")
.getter(getter(InvokeModelWithResponseStreamRequest::guardrailVersion))
.setter(setter(Builder::guardrailVersion))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("X-Amzn-Bedrock-GuardrailVersion")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BODY_FIELD,
CONTENT_TYPE_FIELD, ACCEPT_FIELD, MODEL_ID_FIELD, TRACE_FIELD, GUARDRAIL_IDENTIFIER_FIELD, GUARDRAIL_VERSION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("body", BODY_FIELD);
put("Content-Type", CONTENT_TYPE_FIELD);
put("X-Amzn-Bedrock-Accept", ACCEPT_FIELD);
put("modelId", MODEL_ID_FIELD);
put("X-Amzn-Bedrock-Trace", TRACE_FIELD);
put("X-Amzn-Bedrock-GuardrailIdentifier", GUARDRAIL_IDENTIFIER_FIELD);
put("X-Amzn-Bedrock-GuardrailVersion", GUARDRAIL_VERSION_FIELD);
}
});
private final SdkBytes body;
private final String contentType;
private final String accept;
private final String modelId;
private final String trace;
private final String guardrailIdentifier;
private final String guardrailVersion;
private InvokeModelWithResponseStreamRequest(BuilderImpl builder) {
super(builder);
this.body = builder.body;
this.contentType = builder.contentType;
this.accept = builder.accept;
this.modelId = builder.modelId;
this.trace = builder.trace;
this.guardrailIdentifier = builder.guardrailIdentifier;
this.guardrailVersion = builder.guardrailVersion;
}
/**
*
* The prompt and inference parameters in the format specified in the contentType
in the header. You
* must provide the body in JSON format. To see the format and content of the request and response bodies for
* different models, refer to Inference parameters. For
* more information, see Run
* inference in the Bedrock User Guide.
*
*
* @return The prompt and inference parameters in the format specified in the contentType
in the
* header. You must provide the body in JSON format. To see the format and content of the request and
* response bodies for different models, refer to Inference
* parameters. For more information, see Run inference in the
* Bedrock User Guide.
*/
public final SdkBytes body() {
return body;
}
/**
*
* The MIME type of the input data in the request. You must specify application/json
.
*
*
* @return The MIME type of the input data in the request. You must specify application/json
.
*/
public final String contentType() {
return contentType;
}
/**
*
* The desired MIME type of the inference body in the response. The default value is application/json
.
*
*
* @return The desired MIME type of the inference body in the response. The default value is
* application/json
.
*/
public final String accept() {
return accept;
}
/**
*
* The unique identifier of the model to invoke to run inference.
*
*
* The modelId
to provide depends on the type of model that you use:
*
*
* -
*
* If you use a base model, specify the model ID or its ARN. For a list of model IDs for base models, see Amazon Bedrock base
* model IDs (on-demand throughput) in the Amazon Bedrock User Guide.
*
*
* -
*
* If you use a provisioned model, specify the ARN of the Provisioned Throughput. For more information, see Run inference using a Provisioned
* Throughput in the Amazon Bedrock User Guide.
*
*
* -
*
* If you use a custom model, first purchase Provisioned Throughput for it. Then specify the ARN of the resulting
* provisioned model. For more information, see Use a custom model in
* Amazon Bedrock in the Amazon Bedrock User Guide.
*
*
* -
*
* If you use an imported
* model, specify the ARN of the imported model. You can get the model ARN from a successful call to CreateModelImportJob or from the Imported models page in the Amazon Bedrock console.
*
*
*
*
* @return The unique identifier of the model to invoke to run inference.
*
* The modelId
to provide depends on the type of model that you use:
*
*
* -
*
* If you use a base model, specify the model ID or its ARN. For a list of model IDs for base models, see Amazon Bedrock
* base model IDs (on-demand throughput) in the Amazon Bedrock User Guide.
*
*
* -
*
* If you use a provisioned model, specify the ARN of the Provisioned Throughput. For more information, see
* Run inference using a
* Provisioned Throughput in the Amazon Bedrock User Guide.
*
*
* -
*
* If you use a custom model, first purchase Provisioned Throughput for it. Then specify the ARN of the
* resulting provisioned model. For more information, see Use a custom
* model in Amazon Bedrock in the Amazon Bedrock User Guide.
*
*
* -
*
* If you use an imported model, specify the ARN of the imported model. You can get the model ARN from a successful
* call to
* CreateModelImportJob or from the Imported models page in the Amazon Bedrock console.
*
*
*/
public final String modelId() {
return modelId;
}
/**
*
* Specifies whether to enable or disable the Bedrock trace. If enabled, you can see the full Bedrock trace.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #trace} will return
* {@link Trace#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #traceAsString}.
*
*
* @return Specifies whether to enable or disable the Bedrock trace. If enabled, you can see the full Bedrock trace.
* @see Trace
*/
public final Trace trace() {
return Trace.fromValue(trace);
}
/**
*
* Specifies whether to enable or disable the Bedrock trace. If enabled, you can see the full Bedrock trace.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #trace} will return
* {@link Trace#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #traceAsString}.
*
*
* @return Specifies whether to enable or disable the Bedrock trace. If enabled, you can see the full Bedrock trace.
* @see Trace
*/
public final String traceAsString() {
return trace;
}
/**
*
* The unique identifier of the guardrail that you want to use. If you don't provide a value, no guardrail is
* applied to the invocation.
*
*
* An error is thrown in the following situations.
*
*
* -
*
* You don't provide a guardrail identifier but you specify the amazon-bedrock-guardrailConfig
field in
* the request body.
*
*
* -
*
* You enable the guardrail but the contentType
isn't application/json
.
*
*
* -
*
* You provide a guardrail identifier, but guardrailVersion
isn't specified.
*
*
*
*
* @return The unique identifier of the guardrail that you want to use. If you don't provide a value, no guardrail
* is applied to the invocation.
*
* An error is thrown in the following situations.
*
*
* -
*
* You don't provide a guardrail identifier but you specify the amazon-bedrock-guardrailConfig
* field in the request body.
*
*
* -
*
* You enable the guardrail but the contentType
isn't application/json
.
*
*
* -
*
* You provide a guardrail identifier, but guardrailVersion
isn't specified.
*
*
*/
public final String guardrailIdentifier() {
return guardrailIdentifier;
}
/**
*
* The version number for the guardrail. The value can also be DRAFT
.
*
*
* @return The version number for the guardrail. The value can also be DRAFT
.
*/
public final String guardrailVersion() {
return guardrailVersion;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(body());
hashCode = 31 * hashCode + Objects.hashCode(contentType());
hashCode = 31 * hashCode + Objects.hashCode(accept());
hashCode = 31 * hashCode + Objects.hashCode(modelId());
hashCode = 31 * hashCode + Objects.hashCode(traceAsString());
hashCode = 31 * hashCode + Objects.hashCode(guardrailIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(guardrailVersion());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof InvokeModelWithResponseStreamRequest)) {
return false;
}
InvokeModelWithResponseStreamRequest other = (InvokeModelWithResponseStreamRequest) obj;
return Objects.equals(body(), other.body()) && Objects.equals(contentType(), other.contentType())
&& Objects.equals(accept(), other.accept()) && Objects.equals(modelId(), other.modelId())
&& Objects.equals(traceAsString(), other.traceAsString())
&& Objects.equals(guardrailIdentifier(), other.guardrailIdentifier())
&& Objects.equals(guardrailVersion(), other.guardrailVersion());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("InvokeModelWithResponseStreamRequest")
.add("Body", body() == null ? null : "*** Sensitive Data Redacted ***").add("ContentType", contentType())
.add("Accept", accept()).add("ModelId", modelId()).add("Trace", traceAsString())
.add("GuardrailIdentifier", guardrailIdentifier()).add("GuardrailVersion", guardrailVersion()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "body":
return Optional.ofNullable(clazz.cast(body()));
case "contentType":
return Optional.ofNullable(clazz.cast(contentType()));
case "accept":
return Optional.ofNullable(clazz.cast(accept()));
case "modelId":
return Optional.ofNullable(clazz.cast(modelId()));
case "trace":
return Optional.ofNullable(clazz.cast(traceAsString()));
case "guardrailIdentifier":
return Optional.ofNullable(clazz.cast(guardrailIdentifier()));
case "guardrailVersion":
return Optional.ofNullable(clazz.cast(guardrailVersion()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function