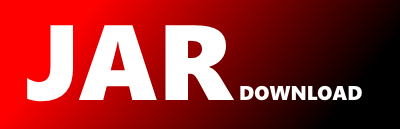
software.amazon.awssdk.services.budgets.DefaultBudgetsClient Maven / Gradle / Ivy
Show all versions of budgets Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.budgets;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.budgets.model.AccessDeniedException;
import software.amazon.awssdk.services.budgets.model.BudgetsException;
import software.amazon.awssdk.services.budgets.model.CreateBudgetRequest;
import software.amazon.awssdk.services.budgets.model.CreateBudgetResponse;
import software.amazon.awssdk.services.budgets.model.CreateNotificationRequest;
import software.amazon.awssdk.services.budgets.model.CreateNotificationResponse;
import software.amazon.awssdk.services.budgets.model.CreateSubscriberRequest;
import software.amazon.awssdk.services.budgets.model.CreateSubscriberResponse;
import software.amazon.awssdk.services.budgets.model.CreationLimitExceededException;
import software.amazon.awssdk.services.budgets.model.DeleteBudgetRequest;
import software.amazon.awssdk.services.budgets.model.DeleteBudgetResponse;
import software.amazon.awssdk.services.budgets.model.DeleteNotificationRequest;
import software.amazon.awssdk.services.budgets.model.DeleteNotificationResponse;
import software.amazon.awssdk.services.budgets.model.DeleteSubscriberRequest;
import software.amazon.awssdk.services.budgets.model.DeleteSubscriberResponse;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetPerformanceHistoryRequest;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetPerformanceHistoryResponse;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetRequest;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetResponse;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetsRequest;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetsResponse;
import software.amazon.awssdk.services.budgets.model.DescribeNotificationsForBudgetRequest;
import software.amazon.awssdk.services.budgets.model.DescribeNotificationsForBudgetResponse;
import software.amazon.awssdk.services.budgets.model.DescribeSubscribersForNotificationRequest;
import software.amazon.awssdk.services.budgets.model.DescribeSubscribersForNotificationResponse;
import software.amazon.awssdk.services.budgets.model.DuplicateRecordException;
import software.amazon.awssdk.services.budgets.model.ExpiredNextTokenException;
import software.amazon.awssdk.services.budgets.model.InternalErrorException;
import software.amazon.awssdk.services.budgets.model.InvalidNextTokenException;
import software.amazon.awssdk.services.budgets.model.InvalidParameterException;
import software.amazon.awssdk.services.budgets.model.NotFoundException;
import software.amazon.awssdk.services.budgets.model.UpdateBudgetRequest;
import software.amazon.awssdk.services.budgets.model.UpdateBudgetResponse;
import software.amazon.awssdk.services.budgets.model.UpdateNotificationRequest;
import software.amazon.awssdk.services.budgets.model.UpdateNotificationResponse;
import software.amazon.awssdk.services.budgets.model.UpdateSubscriberRequest;
import software.amazon.awssdk.services.budgets.model.UpdateSubscriberResponse;
import software.amazon.awssdk.services.budgets.transform.CreateBudgetRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.CreateNotificationRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.CreateSubscriberRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.DeleteBudgetRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.DeleteNotificationRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.DeleteSubscriberRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.DescribeBudgetPerformanceHistoryRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.DescribeBudgetRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.DescribeBudgetsRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.DescribeNotificationsForBudgetRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.DescribeSubscribersForNotificationRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.UpdateBudgetRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.UpdateNotificationRequestMarshaller;
import software.amazon.awssdk.services.budgets.transform.UpdateSubscriberRequestMarshaller;
/**
* Internal implementation of {@link BudgetsClient}.
*
* @see BudgetsClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultBudgetsClient implements BudgetsClient {
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultBudgetsClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Creates a budget and, if included, notifications and subscribers.
*
*
*
* Only one of BudgetLimit
or PlannedBudgetLimits
can be present in the syntax at one
* time. Use the syntax that matches your case. The Request Syntax section shows the BudgetLimit
* syntax. For PlannedBudgetLimits
, see the Examples section.
*
*
*
* @param createBudgetRequest
* Request of CreateBudget
* @return Result of the CreateBudget operation returned by the service.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws CreationLimitExceededException
* You've exceeded the notification or subscriber limit.
* @throws DuplicateRecordException
* The budget name already exists. Budget names must be unique within an account.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.CreateBudget
*/
@Override
public CreateBudgetResponse createBudget(CreateBudgetRequest createBudgetRequest) throws InvalidParameterException,
InternalErrorException, CreationLimitExceededException, DuplicateRecordException, AccessDeniedException,
AwsServiceException, SdkClientException, BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateBudgetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateBudget").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createBudgetRequest)
.withMarshaller(new CreateBudgetRequestMarshaller(protocolFactory)));
}
/**
*
* Creates a notification. You must create the budget before you create the associated notification.
*
*
* @param createNotificationRequest
* Request of CreateNotification
* @return Result of the CreateNotification operation returned by the service.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws CreationLimitExceededException
* You've exceeded the notification or subscriber limit.
* @throws DuplicateRecordException
* The budget name already exists. Budget names must be unique within an account.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.CreateNotification
*/
@Override
public CreateNotificationResponse createNotification(CreateNotificationRequest createNotificationRequest)
throws InternalErrorException, InvalidParameterException, NotFoundException, CreationLimitExceededException,
DuplicateRecordException, AccessDeniedException, AwsServiceException, SdkClientException, BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateNotificationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateNotification").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createNotificationRequest)
.withMarshaller(new CreateNotificationRequestMarshaller(protocolFactory)));
}
/**
*
* Creates a subscriber. You must create the associated budget and notification before you create the subscriber.
*
*
* @param createSubscriberRequest
* Request of CreateSubscriber
* @return Result of the CreateSubscriber operation returned by the service.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws CreationLimitExceededException
* You've exceeded the notification or subscriber limit.
* @throws DuplicateRecordException
* The budget name already exists. Budget names must be unique within an account.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.CreateSubscriber
*/
@Override
public CreateSubscriberResponse createSubscriber(CreateSubscriberRequest createSubscriberRequest)
throws InternalErrorException, InvalidParameterException, CreationLimitExceededException, DuplicateRecordException,
NotFoundException, AccessDeniedException, AwsServiceException, SdkClientException, BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateSubscriberResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateSubscriber").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createSubscriberRequest)
.withMarshaller(new CreateSubscriberRequestMarshaller(protocolFactory)));
}
/**
*
* Deletes a budget. You can delete your budget at any time.
*
*
*
* Deleting a budget also deletes the notifications and subscribers that are associated with that budget.
*
*
*
* @param deleteBudgetRequest
* Request of DeleteBudget
* @return Result of the DeleteBudget operation returned by the service.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.DeleteBudget
*/
@Override
public DeleteBudgetResponse deleteBudget(DeleteBudgetRequest deleteBudgetRequest) throws InternalErrorException,
InvalidParameterException, NotFoundException, AccessDeniedException, AwsServiceException, SdkClientException,
BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteBudgetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteBudget").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteBudgetRequest)
.withMarshaller(new DeleteBudgetRequestMarshaller(protocolFactory)));
}
/**
*
* Deletes a notification.
*
*
*
* Deleting a notification also deletes the subscribers that are associated with the notification.
*
*
*
* @param deleteNotificationRequest
* Request of DeleteNotification
* @return Result of the DeleteNotification operation returned by the service.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.DeleteNotification
*/
@Override
public DeleteNotificationResponse deleteNotification(DeleteNotificationRequest deleteNotificationRequest)
throws InvalidParameterException, InternalErrorException, NotFoundException, AccessDeniedException,
AwsServiceException, SdkClientException, BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteNotificationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteNotification").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteNotificationRequest)
.withMarshaller(new DeleteNotificationRequestMarshaller(protocolFactory)));
}
/**
*
* Deletes a subscriber.
*
*
*
* Deleting the last subscriber to a notification also deletes the notification.
*
*
*
* @param deleteSubscriberRequest
* Request of DeleteSubscriber
* @return Result of the DeleteSubscriber operation returned by the service.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.DeleteSubscriber
*/
@Override
public DeleteSubscriberResponse deleteSubscriber(DeleteSubscriberRequest deleteSubscriberRequest)
throws InternalErrorException, InvalidParameterException, NotFoundException, AccessDeniedException,
AwsServiceException, SdkClientException, BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteSubscriberResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteSubscriber").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteSubscriberRequest)
.withMarshaller(new DeleteSubscriberRequestMarshaller(protocolFactory)));
}
/**
*
* Describes a budget.
*
*
*
* The Request Syntax section shows the BudgetLimit
syntax. For PlannedBudgetLimits
, see
* the Examples section.
*
*
*
* @param describeBudgetRequest
* Request of DescribeBudget
* @return Result of the DescribeBudget operation returned by the service.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.DescribeBudget
*/
@Override
public DescribeBudgetResponse describeBudget(DescribeBudgetRequest describeBudgetRequest) throws InternalErrorException,
InvalidParameterException, NotFoundException, AccessDeniedException, AwsServiceException, SdkClientException,
BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeBudgetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeBudget").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeBudgetRequest)
.withMarshaller(new DescribeBudgetRequestMarshaller(protocolFactory)));
}
/**
*
* Describes the history for DAILY
, MONTHLY
, and QUARTERLY
budgets. Budget
* history isn't available for ANNUAL
budgets.
*
*
* @param describeBudgetPerformanceHistoryRequest
* @return Result of the DescribeBudgetPerformanceHistory operation returned by the service.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws InvalidNextTokenException
* The pagination token is invalid.
* @throws ExpiredNextTokenException
* The pagination token expired.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.DescribeBudgetPerformanceHistory
*/
@Override
public DescribeBudgetPerformanceHistoryResponse describeBudgetPerformanceHistory(
DescribeBudgetPerformanceHistoryRequest describeBudgetPerformanceHistoryRequest) throws InternalErrorException,
InvalidParameterException, NotFoundException, InvalidNextTokenException, ExpiredNextTokenException,
AccessDeniedException, AwsServiceException, SdkClientException, BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeBudgetPerformanceHistoryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeBudgetPerformanceHistory").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeBudgetPerformanceHistoryRequest)
.withMarshaller(new DescribeBudgetPerformanceHistoryRequestMarshaller(protocolFactory)));
}
/**
*
* Lists the budgets that are associated with an account.
*
*
*
* The Request Syntax section shows the BudgetLimit
syntax. For PlannedBudgetLimits
, see
* the Examples section.
*
*
*
* @param describeBudgetsRequest
* Request of DescribeBudgets
* @return Result of the DescribeBudgets operation returned by the service.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws InvalidNextTokenException
* The pagination token is invalid.
* @throws ExpiredNextTokenException
* The pagination token expired.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.DescribeBudgets
*/
@Override
public DescribeBudgetsResponse describeBudgets(DescribeBudgetsRequest describeBudgetsRequest) throws InternalErrorException,
InvalidParameterException, NotFoundException, InvalidNextTokenException, ExpiredNextTokenException,
AccessDeniedException, AwsServiceException, SdkClientException, BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeBudgetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeBudgets").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeBudgetsRequest)
.withMarshaller(new DescribeBudgetsRequestMarshaller(protocolFactory)));
}
/**
*
* Lists the notifications that are associated with a budget.
*
*
* @param describeNotificationsForBudgetRequest
* Request of DescribeNotificationsForBudget
* @return Result of the DescribeNotificationsForBudget operation returned by the service.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws InvalidNextTokenException
* The pagination token is invalid.
* @throws ExpiredNextTokenException
* The pagination token expired.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.DescribeNotificationsForBudget
*/
@Override
public DescribeNotificationsForBudgetResponse describeNotificationsForBudget(
DescribeNotificationsForBudgetRequest describeNotificationsForBudgetRequest) throws InternalErrorException,
InvalidParameterException, NotFoundException, InvalidNextTokenException, ExpiredNextTokenException,
AccessDeniedException, AwsServiceException, SdkClientException, BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeNotificationsForBudgetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeNotificationsForBudget").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeNotificationsForBudgetRequest)
.withMarshaller(new DescribeNotificationsForBudgetRequestMarshaller(protocolFactory)));
}
/**
*
* Lists the subscribers that are associated with a notification.
*
*
* @param describeSubscribersForNotificationRequest
* Request of DescribeSubscribersForNotification
* @return Result of the DescribeSubscribersForNotification operation returned by the service.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws InvalidNextTokenException
* The pagination token is invalid.
* @throws ExpiredNextTokenException
* The pagination token expired.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.DescribeSubscribersForNotification
*/
@Override
public DescribeSubscribersForNotificationResponse describeSubscribersForNotification(
DescribeSubscribersForNotificationRequest describeSubscribersForNotificationRequest) throws InternalErrorException,
NotFoundException, InvalidParameterException, InvalidNextTokenException, ExpiredNextTokenException,
AccessDeniedException, AwsServiceException, SdkClientException, BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeSubscribersForNotificationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeSubscribersForNotification").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeSubscribersForNotificationRequest)
.withMarshaller(new DescribeSubscribersForNotificationRequestMarshaller(protocolFactory)));
}
/**
*
* Updates a budget. You can change every part of a budget except for the budgetName
and the
* calculatedSpend
. When you modify a budget, the calculatedSpend
drops to zero until AWS
* has new usage data to use for forecasting.
*
*
*
* Only one of BudgetLimit
or PlannedBudgetLimits
can be present in the syntax at one
* time. Use the syntax that matches your case. The Request Syntax section shows the BudgetLimit
* syntax. For PlannedBudgetLimits
, see the Examples section.
*
*
*
* @param updateBudgetRequest
* Request of UpdateBudget
* @return Result of the UpdateBudget operation returned by the service.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.UpdateBudget
*/
@Override
public UpdateBudgetResponse updateBudget(UpdateBudgetRequest updateBudgetRequest) throws InternalErrorException,
InvalidParameterException, NotFoundException, AccessDeniedException, AwsServiceException, SdkClientException,
BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateBudgetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateBudget").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateBudgetRequest)
.withMarshaller(new UpdateBudgetRequestMarshaller(protocolFactory)));
}
/**
*
* Updates a notification.
*
*
* @param updateNotificationRequest
* Request of UpdateNotification
* @return Result of the UpdateNotification operation returned by the service.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws DuplicateRecordException
* The budget name already exists. Budget names must be unique within an account.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.UpdateNotification
*/
@Override
public UpdateNotificationResponse updateNotification(UpdateNotificationRequest updateNotificationRequest)
throws InternalErrorException, InvalidParameterException, NotFoundException, DuplicateRecordException,
AccessDeniedException, AwsServiceException, SdkClientException, BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateNotificationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateNotification").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateNotificationRequest)
.withMarshaller(new UpdateNotificationRequestMarshaller(protocolFactory)));
}
/**
*
* Updates a subscriber.
*
*
* @param updateSubscriberRequest
* Request of UpdateSubscriber
* @return Result of the UpdateSubscriber operation returned by the service.
* @throws InternalErrorException
* An error on the server occurred during the processing of your request. Try again later.
* @throws InvalidParameterException
* An error on the client occurred. Typically, the cause is an invalid input value.
* @throws NotFoundException
* We can’t locate the resource that you specified.
* @throws DuplicateRecordException
* The budget name already exists. Budget names must be unique within an account.
* @throws AccessDeniedException
* You are not authorized to use this operation with the given parameters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws BudgetsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample BudgetsClient.UpdateSubscriber
*/
@Override
public UpdateSubscriberResponse updateSubscriber(UpdateSubscriberRequest updateSubscriberRequest)
throws InternalErrorException, InvalidParameterException, NotFoundException, DuplicateRecordException,
AccessDeniedException, AwsServiceException, SdkClientException, BudgetsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateSubscriberResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateSubscriber").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateSubscriberRequest)
.withMarshaller(new UpdateSubscriberRequestMarshaller(protocolFactory)));
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(BudgetsException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidParameterException")
.exceptionBuilderSupplier(InvalidParameterException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NotFoundException")
.exceptionBuilderSupplier(NotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DuplicateRecordException")
.exceptionBuilderSupplier(DuplicateRecordException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalErrorException")
.exceptionBuilderSupplier(InternalErrorException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("CreationLimitExceededException")
.exceptionBuilderSupplier(CreationLimitExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidNextTokenException")
.exceptionBuilderSupplier(InvalidNextTokenException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ExpiredNextTokenException")
.exceptionBuilderSupplier(ExpiredNextTokenException::builder).build());
}
@Override
public void close() {
clientHandler.close();
}
}