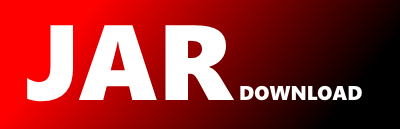
software.amazon.awssdk.services.budgets.BudgetsAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.budgets;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.budgets.model.CreateBudgetActionRequest;
import software.amazon.awssdk.services.budgets.model.CreateBudgetActionResponse;
import software.amazon.awssdk.services.budgets.model.CreateBudgetRequest;
import software.amazon.awssdk.services.budgets.model.CreateBudgetResponse;
import software.amazon.awssdk.services.budgets.model.CreateNotificationRequest;
import software.amazon.awssdk.services.budgets.model.CreateNotificationResponse;
import software.amazon.awssdk.services.budgets.model.CreateSubscriberRequest;
import software.amazon.awssdk.services.budgets.model.CreateSubscriberResponse;
import software.amazon.awssdk.services.budgets.model.DeleteBudgetActionRequest;
import software.amazon.awssdk.services.budgets.model.DeleteBudgetActionResponse;
import software.amazon.awssdk.services.budgets.model.DeleteBudgetRequest;
import software.amazon.awssdk.services.budgets.model.DeleteBudgetResponse;
import software.amazon.awssdk.services.budgets.model.DeleteNotificationRequest;
import software.amazon.awssdk.services.budgets.model.DeleteNotificationResponse;
import software.amazon.awssdk.services.budgets.model.DeleteSubscriberRequest;
import software.amazon.awssdk.services.budgets.model.DeleteSubscriberResponse;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetActionHistoriesRequest;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetActionHistoriesResponse;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetActionRequest;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetActionResponse;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForAccountRequest;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForAccountResponse;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForBudgetRequest;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForBudgetResponse;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetPerformanceHistoryRequest;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetPerformanceHistoryResponse;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetRequest;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetResponse;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetsRequest;
import software.amazon.awssdk.services.budgets.model.DescribeBudgetsResponse;
import software.amazon.awssdk.services.budgets.model.DescribeNotificationsForBudgetRequest;
import software.amazon.awssdk.services.budgets.model.DescribeNotificationsForBudgetResponse;
import software.amazon.awssdk.services.budgets.model.DescribeSubscribersForNotificationRequest;
import software.amazon.awssdk.services.budgets.model.DescribeSubscribersForNotificationResponse;
import software.amazon.awssdk.services.budgets.model.ExecuteBudgetActionRequest;
import software.amazon.awssdk.services.budgets.model.ExecuteBudgetActionResponse;
import software.amazon.awssdk.services.budgets.model.UpdateBudgetActionRequest;
import software.amazon.awssdk.services.budgets.model.UpdateBudgetActionResponse;
import software.amazon.awssdk.services.budgets.model.UpdateBudgetRequest;
import software.amazon.awssdk.services.budgets.model.UpdateBudgetResponse;
import software.amazon.awssdk.services.budgets.model.UpdateNotificationRequest;
import software.amazon.awssdk.services.budgets.model.UpdateNotificationResponse;
import software.amazon.awssdk.services.budgets.model.UpdateSubscriberRequest;
import software.amazon.awssdk.services.budgets.model.UpdateSubscriberResponse;
import software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionHistoriesPublisher;
import software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionsForAccountPublisher;
import software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionsForBudgetPublisher;
import software.amazon.awssdk.services.budgets.paginators.DescribeBudgetPerformanceHistoryPublisher;
import software.amazon.awssdk.services.budgets.paginators.DescribeBudgetsPublisher;
import software.amazon.awssdk.services.budgets.paginators.DescribeNotificationsForBudgetPublisher;
import software.amazon.awssdk.services.budgets.paginators.DescribeSubscribersForNotificationPublisher;
/**
* Service client for accessing AWSBudgets asynchronously. This can be created using the static {@link #builder()}
* method.
*
*
* The AWS Budgets API enables you to use AWS Budgets to plan your service usage, service costs, and instance
* reservations. The API reference provides descriptions, syntax, and usage examples for each of the actions and data
* types for AWS Budgets.
*
*
* Budgets provide you with a way to see the following information:
*
*
* -
*
* How close your plan is to your budgeted amount or to the free tier limits
*
*
* -
*
* Your usage-to-date, including how much you've used of your Reserved Instances (RIs)
*
*
* -
*
* Your current estimated charges from AWS, and how much your predicted usage will accrue in charges by the end of the
* month
*
*
* -
*
* How much of your budget has been used
*
*
*
*
* AWS updates your budget status several times a day. Budgets track your unblended costs, subscriptions, refunds, and
* RIs. You can create the following types of budgets:
*
*
* -
*
* Cost budgets - Plan how much you want to spend on a service.
*
*
* -
*
* Usage budgets - Plan how much you want to use one or more services.
*
*
* -
*
* RI utilization budgets - Define a utilization threshold, and receive alerts when your RI usage falls below
* that threshold. This lets you see if your RIs are unused or under-utilized.
*
*
* -
*
* RI coverage budgets - Define a coverage threshold, and receive alerts when the number of your instance hours
* that are covered by RIs fall below that threshold. This lets you see how much of your instance usage is covered by a
* reservation.
*
*
*
*
* Service Endpoint
*
*
* The AWS Budgets API provides the following endpoint:
*
*
* -
*
* https://budgets.amazonaws.com
*
*
*
*
* For information about costs that are associated with the AWS Budgets API, see AWS Cost Management Pricing.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface BudgetsAsyncClient extends SdkClient {
String SERVICE_NAME = "budgets";
/**
* Create a {@link BudgetsAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static BudgetsAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link BudgetsAsyncClient}.
*/
static BudgetsAsyncClientBuilder builder() {
return new DefaultBudgetsAsyncClientBuilder();
}
/**
*
* Creates a budget and, if included, notifications and subscribers.
*
*
*
* Only one of BudgetLimit
or PlannedBudgetLimits
can be present in the syntax at one
* time. Use the syntax that matches your case. The Request Syntax section shows the BudgetLimit
* syntax. For PlannedBudgetLimits
, see the Examples section.
*
*
*
* @param createBudgetRequest
* Request of CreateBudget
* @return A Java Future containing the result of the CreateBudget operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - CreationLimitExceededException You've exceeded the notification or subscriber limit.
* - DuplicateRecordException The budget name already exists. Budget names must be unique within an
* account.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.CreateBudget
*/
default CompletableFuture createBudget(CreateBudgetRequest createBudgetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a budget and, if included, notifications and subscribers.
*
*
*
* Only one of BudgetLimit
or PlannedBudgetLimits
can be present in the syntax at one
* time. Use the syntax that matches your case. The Request Syntax section shows the BudgetLimit
* syntax. For PlannedBudgetLimits
, see the Examples section.
*
*
*
* This is a convenience which creates an instance of the {@link CreateBudgetRequest.Builder} avoiding the need to
* create one manually via {@link CreateBudgetRequest#builder()}
*
*
* @param createBudgetRequest
* A {@link Consumer} that will call methods on {@link CreateBudgetRequest.Builder} to create a request.
* Request of CreateBudget
* @return A Java Future containing the result of the CreateBudget operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - CreationLimitExceededException You've exceeded the notification or subscriber limit.
* - DuplicateRecordException The budget name already exists. Budget names must be unique within an
* account.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.CreateBudget
*/
default CompletableFuture createBudget(Consumer createBudgetRequest) {
return createBudget(CreateBudgetRequest.builder().applyMutation(createBudgetRequest).build());
}
/**
*
* Creates a budget action.
*
*
* @param createBudgetActionRequest
* @return A Java Future containing the result of the CreateBudgetAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - CreationLimitExceededException You've exceeded the notification or subscriber limit.
* - DuplicateRecordException The budget name already exists. Budget names must be unique within an
* account.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.CreateBudgetAction
*/
default CompletableFuture createBudgetAction(CreateBudgetActionRequest createBudgetActionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a budget action.
*
*
*
* This is a convenience which creates an instance of the {@link CreateBudgetActionRequest.Builder} avoiding the
* need to create one manually via {@link CreateBudgetActionRequest#builder()}
*
*
* @param createBudgetActionRequest
* A {@link Consumer} that will call methods on {@link CreateBudgetActionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateBudgetAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - CreationLimitExceededException You've exceeded the notification or subscriber limit.
* - DuplicateRecordException The budget name already exists. Budget names must be unique within an
* account.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.CreateBudgetAction
*/
default CompletableFuture createBudgetAction(
Consumer createBudgetActionRequest) {
return createBudgetAction(CreateBudgetActionRequest.builder().applyMutation(createBudgetActionRequest).build());
}
/**
*
* Creates a notification. You must create the budget before you create the associated notification.
*
*
* @param createNotificationRequest
* Request of CreateNotification
* @return A Java Future containing the result of the CreateNotification operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - CreationLimitExceededException You've exceeded the notification or subscriber limit.
* - DuplicateRecordException The budget name already exists. Budget names must be unique within an
* account.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.CreateNotification
*/
default CompletableFuture createNotification(CreateNotificationRequest createNotificationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a notification. You must create the budget before you create the associated notification.
*
*
*
* This is a convenience which creates an instance of the {@link CreateNotificationRequest.Builder} avoiding the
* need to create one manually via {@link CreateNotificationRequest#builder()}
*
*
* @param createNotificationRequest
* A {@link Consumer} that will call methods on {@link CreateNotificationRequest.Builder} to create a
* request. Request of CreateNotification
* @return A Java Future containing the result of the CreateNotification operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - CreationLimitExceededException You've exceeded the notification or subscriber limit.
* - DuplicateRecordException The budget name already exists. Budget names must be unique within an
* account.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.CreateNotification
*/
default CompletableFuture createNotification(
Consumer createNotificationRequest) {
return createNotification(CreateNotificationRequest.builder().applyMutation(createNotificationRequest).build());
}
/**
*
* Creates a subscriber. You must create the associated budget and notification before you create the subscriber.
*
*
* @param createSubscriberRequest
* Request of CreateSubscriber
* @return A Java Future containing the result of the CreateSubscriber operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - CreationLimitExceededException You've exceeded the notification or subscriber limit.
* - DuplicateRecordException The budget name already exists. Budget names must be unique within an
* account.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.CreateSubscriber
*/
default CompletableFuture createSubscriber(CreateSubscriberRequest createSubscriberRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a subscriber. You must create the associated budget and notification before you create the subscriber.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSubscriberRequest.Builder} avoiding the need
* to create one manually via {@link CreateSubscriberRequest#builder()}
*
*
* @param createSubscriberRequest
* A {@link Consumer} that will call methods on {@link CreateSubscriberRequest.Builder} to create a request.
* Request of CreateSubscriber
* @return A Java Future containing the result of the CreateSubscriber operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - CreationLimitExceededException You've exceeded the notification or subscriber limit.
* - DuplicateRecordException The budget name already exists. Budget names must be unique within an
* account.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.CreateSubscriber
*/
default CompletableFuture createSubscriber(
Consumer createSubscriberRequest) {
return createSubscriber(CreateSubscriberRequest.builder().applyMutation(createSubscriberRequest).build());
}
/**
*
* Deletes a budget. You can delete your budget at any time.
*
*
*
* Deleting a budget also deletes the notifications and subscribers that are associated with that budget.
*
*
*
* @param deleteBudgetRequest
* Request of DeleteBudget
* @return A Java Future containing the result of the DeleteBudget operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DeleteBudget
*/
default CompletableFuture deleteBudget(DeleteBudgetRequest deleteBudgetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a budget. You can delete your budget at any time.
*
*
*
* Deleting a budget also deletes the notifications and subscribers that are associated with that budget.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteBudgetRequest.Builder} avoiding the need to
* create one manually via {@link DeleteBudgetRequest#builder()}
*
*
* @param deleteBudgetRequest
* A {@link Consumer} that will call methods on {@link DeleteBudgetRequest.Builder} to create a request.
* Request of DeleteBudget
* @return A Java Future containing the result of the DeleteBudget operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DeleteBudget
*/
default CompletableFuture deleteBudget(Consumer deleteBudgetRequest) {
return deleteBudget(DeleteBudgetRequest.builder().applyMutation(deleteBudgetRequest).build());
}
/**
*
* Deletes a budget action.
*
*
* @param deleteBudgetActionRequest
* @return A Java Future containing the result of the DeleteBudgetAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - ResourceLockedException The request was received and recognized by the server, but the server
* rejected that particular method for the requested resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DeleteBudgetAction
*/
default CompletableFuture deleteBudgetAction(DeleteBudgetActionRequest deleteBudgetActionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a budget action.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteBudgetActionRequest.Builder} avoiding the
* need to create one manually via {@link DeleteBudgetActionRequest#builder()}
*
*
* @param deleteBudgetActionRequest
* A {@link Consumer} that will call methods on {@link DeleteBudgetActionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteBudgetAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - ResourceLockedException The request was received and recognized by the server, but the server
* rejected that particular method for the requested resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DeleteBudgetAction
*/
default CompletableFuture deleteBudgetAction(
Consumer deleteBudgetActionRequest) {
return deleteBudgetAction(DeleteBudgetActionRequest.builder().applyMutation(deleteBudgetActionRequest).build());
}
/**
*
* Deletes a notification.
*
*
*
* Deleting a notification also deletes the subscribers that are associated with the notification.
*
*
*
* @param deleteNotificationRequest
* Request of DeleteNotification
* @return A Java Future containing the result of the DeleteNotification operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DeleteNotification
*/
default CompletableFuture deleteNotification(DeleteNotificationRequest deleteNotificationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a notification.
*
*
*
* Deleting a notification also deletes the subscribers that are associated with the notification.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteNotificationRequest.Builder} avoiding the
* need to create one manually via {@link DeleteNotificationRequest#builder()}
*
*
* @param deleteNotificationRequest
* A {@link Consumer} that will call methods on {@link DeleteNotificationRequest.Builder} to create a
* request. Request of DeleteNotification
* @return A Java Future containing the result of the DeleteNotification operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DeleteNotification
*/
default CompletableFuture deleteNotification(
Consumer deleteNotificationRequest) {
return deleteNotification(DeleteNotificationRequest.builder().applyMutation(deleteNotificationRequest).build());
}
/**
*
* Deletes a subscriber.
*
*
*
* Deleting the last subscriber to a notification also deletes the notification.
*
*
*
* @param deleteSubscriberRequest
* Request of DeleteSubscriber
* @return A Java Future containing the result of the DeleteSubscriber operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DeleteSubscriber
*/
default CompletableFuture deleteSubscriber(DeleteSubscriberRequest deleteSubscriberRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a subscriber.
*
*
*
* Deleting the last subscriber to a notification also deletes the notification.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSubscriberRequest.Builder} avoiding the need
* to create one manually via {@link DeleteSubscriberRequest#builder()}
*
*
* @param deleteSubscriberRequest
* A {@link Consumer} that will call methods on {@link DeleteSubscriberRequest.Builder} to create a request.
* Request of DeleteSubscriber
* @return A Java Future containing the result of the DeleteSubscriber operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DeleteSubscriber
*/
default CompletableFuture deleteSubscriber(
Consumer deleteSubscriberRequest) {
return deleteSubscriber(DeleteSubscriberRequest.builder().applyMutation(deleteSubscriberRequest).build());
}
/**
*
* Describes a budget.
*
*
*
* The Request Syntax section shows the BudgetLimit
syntax. For PlannedBudgetLimits
, see
* the Examples section.
*
*
*
* @param describeBudgetRequest
* Request of DescribeBudget
* @return A Java Future containing the result of the DescribeBudget operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudget
*/
default CompletableFuture describeBudget(DescribeBudgetRequest describeBudgetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes a budget.
*
*
*
* The Request Syntax section shows the BudgetLimit
syntax. For PlannedBudgetLimits
, see
* the Examples section.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeBudgetRequest.Builder} avoiding the need to
* create one manually via {@link DescribeBudgetRequest#builder()}
*
*
* @param describeBudgetRequest
* A {@link Consumer} that will call methods on {@link DescribeBudgetRequest.Builder} to create a request.
* Request of DescribeBudget
* @return A Java Future containing the result of the DescribeBudget operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudget
*/
default CompletableFuture describeBudget(Consumer describeBudgetRequest) {
return describeBudget(DescribeBudgetRequest.builder().applyMutation(describeBudgetRequest).build());
}
/**
*
* Describes a budget action detail.
*
*
* @param describeBudgetActionRequest
* @return A Java Future containing the result of the DescribeBudgetAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetAction
*/
default CompletableFuture describeBudgetAction(
DescribeBudgetActionRequest describeBudgetActionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes a budget action detail.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeBudgetActionRequest.Builder} avoiding the
* need to create one manually via {@link DescribeBudgetActionRequest#builder()}
*
*
* @param describeBudgetActionRequest
* A {@link Consumer} that will call methods on {@link DescribeBudgetActionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeBudgetAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetAction
*/
default CompletableFuture describeBudgetAction(
Consumer describeBudgetActionRequest) {
return describeBudgetAction(DescribeBudgetActionRequest.builder().applyMutation(describeBudgetActionRequest).build());
}
/**
*
* Describes a budget action history detail.
*
*
* @param describeBudgetActionHistoriesRequest
* @return A Java Future containing the result of the DescribeBudgetActionHistories operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - InvalidNextTokenException The pagination token is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetActionHistories
*/
default CompletableFuture describeBudgetActionHistories(
DescribeBudgetActionHistoriesRequest describeBudgetActionHistoriesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes a budget action history detail.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeBudgetActionHistoriesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeBudgetActionHistoriesRequest#builder()}
*
*
* @param describeBudgetActionHistoriesRequest
* A {@link Consumer} that will call methods on {@link DescribeBudgetActionHistoriesRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeBudgetActionHistories operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - InvalidNextTokenException The pagination token is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetActionHistories
*/
default CompletableFuture describeBudgetActionHistories(
Consumer describeBudgetActionHistoriesRequest) {
return describeBudgetActionHistories(DescribeBudgetActionHistoriesRequest.builder()
.applyMutation(describeBudgetActionHistoriesRequest).build());
}
/**
*
* Describes a budget action history detail.
*
*
*
* This is a variant of
* {@link #describeBudgetActionHistories(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionHistoriesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionHistoriesPublisher publisher = client.describeBudgetActionHistoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionHistoriesPublisher publisher = client.describeBudgetActionHistoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionHistoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBudgetActionHistories(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionHistoriesRequest)}
* operation.
*
*
* @param describeBudgetActionHistoriesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - InvalidNextTokenException The pagination token is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetActionHistories
*/
default DescribeBudgetActionHistoriesPublisher describeBudgetActionHistoriesPaginator(
DescribeBudgetActionHistoriesRequest describeBudgetActionHistoriesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes a budget action history detail.
*
*
*
* This is a variant of
* {@link #describeBudgetActionHistories(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionHistoriesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionHistoriesPublisher publisher = client.describeBudgetActionHistoriesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionHistoriesPublisher publisher = client.describeBudgetActionHistoriesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionHistoriesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBudgetActionHistories(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionHistoriesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeBudgetActionHistoriesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeBudgetActionHistoriesRequest#builder()}
*
*
* @param describeBudgetActionHistoriesRequest
* A {@link Consumer} that will call methods on {@link DescribeBudgetActionHistoriesRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - InvalidNextTokenException The pagination token is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetActionHistories
*/
default DescribeBudgetActionHistoriesPublisher describeBudgetActionHistoriesPaginator(
Consumer describeBudgetActionHistoriesRequest) {
return describeBudgetActionHistoriesPaginator(DescribeBudgetActionHistoriesRequest.builder()
.applyMutation(describeBudgetActionHistoriesRequest).build());
}
/**
*
* Describes all of the budget actions for an account.
*
*
* @param describeBudgetActionsForAccountRequest
* @return A Java Future containing the result of the DescribeBudgetActionsForAccount operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - InvalidNextTokenException The pagination token is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetActionsForAccount
*/
default CompletableFuture describeBudgetActionsForAccount(
DescribeBudgetActionsForAccountRequest describeBudgetActionsForAccountRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes all of the budget actions for an account.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeBudgetActionsForAccountRequest.Builder}
* avoiding the need to create one manually via {@link DescribeBudgetActionsForAccountRequest#builder()}
*
*
* @param describeBudgetActionsForAccountRequest
* A {@link Consumer} that will call methods on {@link DescribeBudgetActionsForAccountRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeBudgetActionsForAccount operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - InvalidNextTokenException The pagination token is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetActionsForAccount
*/
default CompletableFuture describeBudgetActionsForAccount(
Consumer describeBudgetActionsForAccountRequest) {
return describeBudgetActionsForAccount(DescribeBudgetActionsForAccountRequest.builder()
.applyMutation(describeBudgetActionsForAccountRequest).build());
}
/**
*
* Describes all of the budget actions for an account.
*
*
*
* This is a variant of
* {@link #describeBudgetActionsForAccount(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForAccountRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionsForAccountPublisher publisher = client.describeBudgetActionsForAccountPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionsForAccountPublisher publisher = client.describeBudgetActionsForAccountPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForAccountResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBudgetActionsForAccount(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForAccountRequest)}
* operation.
*
*
* @param describeBudgetActionsForAccountRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - InvalidNextTokenException The pagination token is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetActionsForAccount
*/
default DescribeBudgetActionsForAccountPublisher describeBudgetActionsForAccountPaginator(
DescribeBudgetActionsForAccountRequest describeBudgetActionsForAccountRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes all of the budget actions for an account.
*
*
*
* This is a variant of
* {@link #describeBudgetActionsForAccount(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForAccountRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionsForAccountPublisher publisher = client.describeBudgetActionsForAccountPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionsForAccountPublisher publisher = client.describeBudgetActionsForAccountPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForAccountResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBudgetActionsForAccount(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForAccountRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeBudgetActionsForAccountRequest.Builder}
* avoiding the need to create one manually via {@link DescribeBudgetActionsForAccountRequest#builder()}
*
*
* @param describeBudgetActionsForAccountRequest
* A {@link Consumer} that will call methods on {@link DescribeBudgetActionsForAccountRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - InvalidNextTokenException The pagination token is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetActionsForAccount
*/
default DescribeBudgetActionsForAccountPublisher describeBudgetActionsForAccountPaginator(
Consumer describeBudgetActionsForAccountRequest) {
return describeBudgetActionsForAccountPaginator(DescribeBudgetActionsForAccountRequest.builder()
.applyMutation(describeBudgetActionsForAccountRequest).build());
}
/**
*
* Describes all of the budget actions for a budget.
*
*
* @param describeBudgetActionsForBudgetRequest
* @return A Java Future containing the result of the DescribeBudgetActionsForBudget operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - InvalidNextTokenException The pagination token is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetActionsForBudget
*/
default CompletableFuture describeBudgetActionsForBudget(
DescribeBudgetActionsForBudgetRequest describeBudgetActionsForBudgetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes all of the budget actions for a budget.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeBudgetActionsForBudgetRequest.Builder}
* avoiding the need to create one manually via {@link DescribeBudgetActionsForBudgetRequest#builder()}
*
*
* @param describeBudgetActionsForBudgetRequest
* A {@link Consumer} that will call methods on {@link DescribeBudgetActionsForBudgetRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeBudgetActionsForBudget operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - InvalidNextTokenException The pagination token is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetActionsForBudget
*/
default CompletableFuture describeBudgetActionsForBudget(
Consumer describeBudgetActionsForBudgetRequest) {
return describeBudgetActionsForBudget(DescribeBudgetActionsForBudgetRequest.builder()
.applyMutation(describeBudgetActionsForBudgetRequest).build());
}
/**
*
* Describes all of the budget actions for a budget.
*
*
*
* This is a variant of
* {@link #describeBudgetActionsForBudget(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForBudgetRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionsForBudgetPublisher publisher = client.describeBudgetActionsForBudgetPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionsForBudgetPublisher publisher = client.describeBudgetActionsForBudgetPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForBudgetResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBudgetActionsForBudget(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForBudgetRequest)}
* operation.
*
*
* @param describeBudgetActionsForBudgetRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - InvalidNextTokenException The pagination token is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetActionsForBudget
*/
default DescribeBudgetActionsForBudgetPublisher describeBudgetActionsForBudgetPaginator(
DescribeBudgetActionsForBudgetRequest describeBudgetActionsForBudgetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes all of the budget actions for a budget.
*
*
*
* This is a variant of
* {@link #describeBudgetActionsForBudget(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForBudgetRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionsForBudgetPublisher publisher = client.describeBudgetActionsForBudgetPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetActionsForBudgetPublisher publisher = client.describeBudgetActionsForBudgetPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForBudgetResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBudgetActionsForBudget(software.amazon.awssdk.services.budgets.model.DescribeBudgetActionsForBudgetRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeBudgetActionsForBudgetRequest.Builder}
* avoiding the need to create one manually via {@link DescribeBudgetActionsForBudgetRequest#builder()}
*
*
* @param describeBudgetActionsForBudgetRequest
* A {@link Consumer} that will call methods on {@link DescribeBudgetActionsForBudgetRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - InvalidNextTokenException The pagination token is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetActionsForBudget
*/
default DescribeBudgetActionsForBudgetPublisher describeBudgetActionsForBudgetPaginator(
Consumer describeBudgetActionsForBudgetRequest) {
return describeBudgetActionsForBudgetPaginator(DescribeBudgetActionsForBudgetRequest.builder()
.applyMutation(describeBudgetActionsForBudgetRequest).build());
}
/**
*
* Describes the history for DAILY
, MONTHLY
, and QUARTERLY
budgets. Budget
* history isn't available for ANNUAL
budgets.
*
*
* @param describeBudgetPerformanceHistoryRequest
* @return A Java Future containing the result of the DescribeBudgetPerformanceHistory operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetPerformanceHistory
*/
default CompletableFuture describeBudgetPerformanceHistory(
DescribeBudgetPerformanceHistoryRequest describeBudgetPerformanceHistoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the history for DAILY
, MONTHLY
, and QUARTERLY
budgets. Budget
* history isn't available for ANNUAL
budgets.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeBudgetPerformanceHistoryRequest.Builder}
* avoiding the need to create one manually via {@link DescribeBudgetPerformanceHistoryRequest#builder()}
*
*
* @param describeBudgetPerformanceHistoryRequest
* A {@link Consumer} that will call methods on {@link DescribeBudgetPerformanceHistoryRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeBudgetPerformanceHistory operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetPerformanceHistory
*/
default CompletableFuture describeBudgetPerformanceHistory(
Consumer describeBudgetPerformanceHistoryRequest) {
return describeBudgetPerformanceHistory(DescribeBudgetPerformanceHistoryRequest.builder()
.applyMutation(describeBudgetPerformanceHistoryRequest).build());
}
/**
*
* Describes the history for DAILY
, MONTHLY
, and QUARTERLY
budgets. Budget
* history isn't available for ANNUAL
budgets.
*
*
*
* This is a variant of
* {@link #describeBudgetPerformanceHistory(software.amazon.awssdk.services.budgets.model.DescribeBudgetPerformanceHistoryRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetPerformanceHistoryPublisher publisher = client.describeBudgetPerformanceHistoryPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetPerformanceHistoryPublisher publisher = client.describeBudgetPerformanceHistoryPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeBudgetPerformanceHistoryResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBudgetPerformanceHistory(software.amazon.awssdk.services.budgets.model.DescribeBudgetPerformanceHistoryRequest)}
* operation.
*
*
* @param describeBudgetPerformanceHistoryRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetPerformanceHistory
*/
default DescribeBudgetPerformanceHistoryPublisher describeBudgetPerformanceHistoryPaginator(
DescribeBudgetPerformanceHistoryRequest describeBudgetPerformanceHistoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the history for DAILY
, MONTHLY
, and QUARTERLY
budgets. Budget
* history isn't available for ANNUAL
budgets.
*
*
*
* This is a variant of
* {@link #describeBudgetPerformanceHistory(software.amazon.awssdk.services.budgets.model.DescribeBudgetPerformanceHistoryRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetPerformanceHistoryPublisher publisher = client.describeBudgetPerformanceHistoryPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetPerformanceHistoryPublisher publisher = client.describeBudgetPerformanceHistoryPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeBudgetPerformanceHistoryResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBudgetPerformanceHistory(software.amazon.awssdk.services.budgets.model.DescribeBudgetPerformanceHistoryRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeBudgetPerformanceHistoryRequest.Builder}
* avoiding the need to create one manually via {@link DescribeBudgetPerformanceHistoryRequest#builder()}
*
*
* @param describeBudgetPerformanceHistoryRequest
* A {@link Consumer} that will call methods on {@link DescribeBudgetPerformanceHistoryRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgetPerformanceHistory
*/
default DescribeBudgetPerformanceHistoryPublisher describeBudgetPerformanceHistoryPaginator(
Consumer describeBudgetPerformanceHistoryRequest) {
return describeBudgetPerformanceHistoryPaginator(DescribeBudgetPerformanceHistoryRequest.builder()
.applyMutation(describeBudgetPerformanceHistoryRequest).build());
}
/**
*
* Lists the budgets that are associated with an account.
*
*
*
* The Request Syntax section shows the BudgetLimit
syntax. For PlannedBudgetLimits
, see
* the Examples section.
*
*
*
* @param describeBudgetsRequest
* Request of DescribeBudgets
* @return A Java Future containing the result of the DescribeBudgets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgets
*/
default CompletableFuture describeBudgets(DescribeBudgetsRequest describeBudgetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the budgets that are associated with an account.
*
*
*
* The Request Syntax section shows the BudgetLimit
syntax. For PlannedBudgetLimits
, see
* the Examples section.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeBudgetsRequest.Builder} avoiding the need
* to create one manually via {@link DescribeBudgetsRequest#builder()}
*
*
* @param describeBudgetsRequest
* A {@link Consumer} that will call methods on {@link DescribeBudgetsRequest.Builder} to create a request.
* Request of DescribeBudgets
* @return A Java Future containing the result of the DescribeBudgets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgets
*/
default CompletableFuture describeBudgets(
Consumer describeBudgetsRequest) {
return describeBudgets(DescribeBudgetsRequest.builder().applyMutation(describeBudgetsRequest).build());
}
/**
*
* Lists the budgets that are associated with an account.
*
*
*
* The Request Syntax section shows the BudgetLimit
syntax. For PlannedBudgetLimits
, see
* the Examples section.
*
*
*
* This is a variant of
* {@link #describeBudgets(software.amazon.awssdk.services.budgets.model.DescribeBudgetsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetsPublisher publisher = client.describeBudgetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetsPublisher publisher = client.describeBudgetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeBudgetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBudgets(software.amazon.awssdk.services.budgets.model.DescribeBudgetsRequest)} operation.
*
*
* @param describeBudgetsRequest
* Request of DescribeBudgets
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgets
*/
default DescribeBudgetsPublisher describeBudgetsPaginator(DescribeBudgetsRequest describeBudgetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the budgets that are associated with an account.
*
*
*
* The Request Syntax section shows the BudgetLimit
syntax. For PlannedBudgetLimits
, see
* the Examples section.
*
*
*
* This is a variant of
* {@link #describeBudgets(software.amazon.awssdk.services.budgets.model.DescribeBudgetsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetsPublisher publisher = client.describeBudgetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeBudgetsPublisher publisher = client.describeBudgetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeBudgetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBudgets(software.amazon.awssdk.services.budgets.model.DescribeBudgetsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeBudgetsRequest.Builder} avoiding the need
* to create one manually via {@link DescribeBudgetsRequest#builder()}
*
*
* @param describeBudgetsRequest
* A {@link Consumer} that will call methods on {@link DescribeBudgetsRequest.Builder} to create a request.
* Request of DescribeBudgets
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeBudgets
*/
default DescribeBudgetsPublisher describeBudgetsPaginator(Consumer describeBudgetsRequest) {
return describeBudgetsPaginator(DescribeBudgetsRequest.builder().applyMutation(describeBudgetsRequest).build());
}
/**
*
* Lists the notifications that are associated with a budget.
*
*
* @param describeNotificationsForBudgetRequest
* Request of DescribeNotificationsForBudget
* @return A Java Future containing the result of the DescribeNotificationsForBudget operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeNotificationsForBudget
*/
default CompletableFuture describeNotificationsForBudget(
DescribeNotificationsForBudgetRequest describeNotificationsForBudgetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the notifications that are associated with a budget.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeNotificationsForBudgetRequest.Builder}
* avoiding the need to create one manually via {@link DescribeNotificationsForBudgetRequest#builder()}
*
*
* @param describeNotificationsForBudgetRequest
* A {@link Consumer} that will call methods on {@link DescribeNotificationsForBudgetRequest.Builder} to
* create a request. Request of DescribeNotificationsForBudget
* @return A Java Future containing the result of the DescribeNotificationsForBudget operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeNotificationsForBudget
*/
default CompletableFuture describeNotificationsForBudget(
Consumer describeNotificationsForBudgetRequest) {
return describeNotificationsForBudget(DescribeNotificationsForBudgetRequest.builder()
.applyMutation(describeNotificationsForBudgetRequest).build());
}
/**
*
* Lists the notifications that are associated with a budget.
*
*
*
* This is a variant of
* {@link #describeNotificationsForBudget(software.amazon.awssdk.services.budgets.model.DescribeNotificationsForBudgetRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeNotificationsForBudgetPublisher publisher = client.describeNotificationsForBudgetPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeNotificationsForBudgetPublisher publisher = client.describeNotificationsForBudgetPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeNotificationsForBudgetResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeNotificationsForBudget(software.amazon.awssdk.services.budgets.model.DescribeNotificationsForBudgetRequest)}
* operation.
*
*
* @param describeNotificationsForBudgetRequest
* Request of DescribeNotificationsForBudget
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeNotificationsForBudget
*/
default DescribeNotificationsForBudgetPublisher describeNotificationsForBudgetPaginator(
DescribeNotificationsForBudgetRequest describeNotificationsForBudgetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the notifications that are associated with a budget.
*
*
*
* This is a variant of
* {@link #describeNotificationsForBudget(software.amazon.awssdk.services.budgets.model.DescribeNotificationsForBudgetRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeNotificationsForBudgetPublisher publisher = client.describeNotificationsForBudgetPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeNotificationsForBudgetPublisher publisher = client.describeNotificationsForBudgetPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeNotificationsForBudgetResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeNotificationsForBudget(software.amazon.awssdk.services.budgets.model.DescribeNotificationsForBudgetRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeNotificationsForBudgetRequest.Builder}
* avoiding the need to create one manually via {@link DescribeNotificationsForBudgetRequest#builder()}
*
*
* @param describeNotificationsForBudgetRequest
* A {@link Consumer} that will call methods on {@link DescribeNotificationsForBudgetRequest.Builder} to
* create a request. Request of DescribeNotificationsForBudget
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeNotificationsForBudget
*/
default DescribeNotificationsForBudgetPublisher describeNotificationsForBudgetPaginator(
Consumer describeNotificationsForBudgetRequest) {
return describeNotificationsForBudgetPaginator(DescribeNotificationsForBudgetRequest.builder()
.applyMutation(describeNotificationsForBudgetRequest).build());
}
/**
*
* Lists the subscribers that are associated with a notification.
*
*
* @param describeSubscribersForNotificationRequest
* Request of DescribeSubscribersForNotification
* @return A Java Future containing the result of the DescribeSubscribersForNotification operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeSubscribersForNotification
*/
default CompletableFuture describeSubscribersForNotification(
DescribeSubscribersForNotificationRequest describeSubscribersForNotificationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the subscribers that are associated with a notification.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeSubscribersForNotificationRequest.Builder}
* avoiding the need to create one manually via {@link DescribeSubscribersForNotificationRequest#builder()}
*
*
* @param describeSubscribersForNotificationRequest
* A {@link Consumer} that will call methods on {@link DescribeSubscribersForNotificationRequest.Builder} to
* create a request. Request of DescribeSubscribersForNotification
* @return A Java Future containing the result of the DescribeSubscribersForNotification operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeSubscribersForNotification
*/
default CompletableFuture describeSubscribersForNotification(
Consumer describeSubscribersForNotificationRequest) {
return describeSubscribersForNotification(DescribeSubscribersForNotificationRequest.builder()
.applyMutation(describeSubscribersForNotificationRequest).build());
}
/**
*
* Lists the subscribers that are associated with a notification.
*
*
*
* This is a variant of
* {@link #describeSubscribersForNotification(software.amazon.awssdk.services.budgets.model.DescribeSubscribersForNotificationRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeSubscribersForNotificationPublisher publisher = client.describeSubscribersForNotificationPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeSubscribersForNotificationPublisher publisher = client.describeSubscribersForNotificationPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeSubscribersForNotificationResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeSubscribersForNotification(software.amazon.awssdk.services.budgets.model.DescribeSubscribersForNotificationRequest)}
* operation.
*
*
* @param describeSubscribersForNotificationRequest
* Request of DescribeSubscribersForNotification
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeSubscribersForNotification
*/
default DescribeSubscribersForNotificationPublisher describeSubscribersForNotificationPaginator(
DescribeSubscribersForNotificationRequest describeSubscribersForNotificationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the subscribers that are associated with a notification.
*
*
*
* This is a variant of
* {@link #describeSubscribersForNotification(software.amazon.awssdk.services.budgets.model.DescribeSubscribersForNotificationRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeSubscribersForNotificationPublisher publisher = client.describeSubscribersForNotificationPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.budgets.paginators.DescribeSubscribersForNotificationPublisher publisher = client.describeSubscribersForNotificationPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.budgets.model.DescribeSubscribersForNotificationResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeSubscribersForNotification(software.amazon.awssdk.services.budgets.model.DescribeSubscribersForNotificationRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeSubscribersForNotificationRequest.Builder}
* avoiding the need to create one manually via {@link DescribeSubscribersForNotificationRequest#builder()}
*
*
* @param describeSubscribersForNotificationRequest
* A {@link Consumer} that will call methods on {@link DescribeSubscribersForNotificationRequest.Builder} to
* create a request. Request of DescribeSubscribersForNotification
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - NotFoundException We can’t locate the resource that you specified.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - InvalidNextTokenException The pagination token is invalid.
* - ExpiredNextTokenException The pagination token expired.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.DescribeSubscribersForNotification
*/
default DescribeSubscribersForNotificationPublisher describeSubscribersForNotificationPaginator(
Consumer describeSubscribersForNotificationRequest) {
return describeSubscribersForNotificationPaginator(DescribeSubscribersForNotificationRequest.builder()
.applyMutation(describeSubscribersForNotificationRequest).build());
}
/**
*
* Executes a budget action.
*
*
* @param executeBudgetActionRequest
* @return A Java Future containing the result of the ExecuteBudgetAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - ResourceLockedException The request was received and recognized by the server, but the server
* rejected that particular method for the requested resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.ExecuteBudgetAction
*/
default CompletableFuture executeBudgetAction(
ExecuteBudgetActionRequest executeBudgetActionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Executes a budget action.
*
*
*
* This is a convenience which creates an instance of the {@link ExecuteBudgetActionRequest.Builder} avoiding the
* need to create one manually via {@link ExecuteBudgetActionRequest#builder()}
*
*
* @param executeBudgetActionRequest
* A {@link Consumer} that will call methods on {@link ExecuteBudgetActionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ExecuteBudgetAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - ResourceLockedException The request was received and recognized by the server, but the server
* rejected that particular method for the requested resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.ExecuteBudgetAction
*/
default CompletableFuture executeBudgetAction(
Consumer executeBudgetActionRequest) {
return executeBudgetAction(ExecuteBudgetActionRequest.builder().applyMutation(executeBudgetActionRequest).build());
}
/**
*
* Updates a budget. You can change every part of a budget except for the budgetName
and the
* calculatedSpend
. When you modify a budget, the calculatedSpend
drops to zero until AWS
* has new usage data to use for forecasting.
*
*
*
* Only one of BudgetLimit
or PlannedBudgetLimits
can be present in the syntax at one
* time. Use the syntax that matches your case. The Request Syntax section shows the BudgetLimit
* syntax. For PlannedBudgetLimits
, see the Examples section.
*
*
*
* @param updateBudgetRequest
* Request of UpdateBudget
* @return A Java Future containing the result of the UpdateBudget operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.UpdateBudget
*/
default CompletableFuture updateBudget(UpdateBudgetRequest updateBudgetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a budget. You can change every part of a budget except for the budgetName
and the
* calculatedSpend
. When you modify a budget, the calculatedSpend
drops to zero until AWS
* has new usage data to use for forecasting.
*
*
*
* Only one of BudgetLimit
or PlannedBudgetLimits
can be present in the syntax at one
* time. Use the syntax that matches your case. The Request Syntax section shows the BudgetLimit
* syntax. For PlannedBudgetLimits
, see the Examples section.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateBudgetRequest.Builder} avoiding the need to
* create one manually via {@link UpdateBudgetRequest#builder()}
*
*
* @param updateBudgetRequest
* A {@link Consumer} that will call methods on {@link UpdateBudgetRequest.Builder} to create a request.
* Request of UpdateBudget
* @return A Java Future containing the result of the UpdateBudget operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.UpdateBudget
*/
default CompletableFuture updateBudget(Consumer updateBudgetRequest) {
return updateBudget(UpdateBudgetRequest.builder().applyMutation(updateBudgetRequest).build());
}
/**
*
* Updates a budget action.
*
*
* @param updateBudgetActionRequest
* @return A Java Future containing the result of the UpdateBudgetAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - ResourceLockedException The request was received and recognized by the server, but the server
* rejected that particular method for the requested resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.UpdateBudgetAction
*/
default CompletableFuture updateBudgetAction(UpdateBudgetActionRequest updateBudgetActionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a budget action.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateBudgetActionRequest.Builder} avoiding the
* need to create one manually via {@link UpdateBudgetActionRequest#builder()}
*
*
* @param updateBudgetActionRequest
* A {@link Consumer} that will call methods on {@link UpdateBudgetActionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateBudgetAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - ResourceLockedException The request was received and recognized by the server, but the server
* rejected that particular method for the requested resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.UpdateBudgetAction
*/
default CompletableFuture updateBudgetAction(
Consumer updateBudgetActionRequest) {
return updateBudgetAction(UpdateBudgetActionRequest.builder().applyMutation(updateBudgetActionRequest).build());
}
/**
*
* Updates a notification.
*
*
* @param updateNotificationRequest
* Request of UpdateNotification
* @return A Java Future containing the result of the UpdateNotification operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - DuplicateRecordException The budget name already exists. Budget names must be unique within an
* account.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.UpdateNotification
*/
default CompletableFuture updateNotification(UpdateNotificationRequest updateNotificationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a notification.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateNotificationRequest.Builder} avoiding the
* need to create one manually via {@link UpdateNotificationRequest#builder()}
*
*
* @param updateNotificationRequest
* A {@link Consumer} that will call methods on {@link UpdateNotificationRequest.Builder} to create a
* request. Request of UpdateNotification
* @return A Java Future containing the result of the UpdateNotification operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - DuplicateRecordException The budget name already exists. Budget names must be unique within an
* account.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.UpdateNotification
*/
default CompletableFuture updateNotification(
Consumer updateNotificationRequest) {
return updateNotification(UpdateNotificationRequest.builder().applyMutation(updateNotificationRequest).build());
}
/**
*
* Updates a subscriber.
*
*
* @param updateSubscriberRequest
* Request of UpdateSubscriber
* @return A Java Future containing the result of the UpdateSubscriber operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - DuplicateRecordException The budget name already exists. Budget names must be unique within an
* account.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.UpdateSubscriber
*/
default CompletableFuture updateSubscriber(UpdateSubscriberRequest updateSubscriberRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a subscriber.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSubscriberRequest.Builder} avoiding the need
* to create one manually via {@link UpdateSubscriberRequest#builder()}
*
*
* @param updateSubscriberRequest
* A {@link Consumer} that will call methods on {@link UpdateSubscriberRequest.Builder} to create a request.
* Request of UpdateSubscriber
* @return A Java Future containing the result of the UpdateSubscriber operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException An error on the server occurred during the processing of your request. Try
* again later.
* - InvalidParameterException An error on the client occurred. Typically, the cause is an invalid input
* value.
* - NotFoundException We can’t locate the resource that you specified.
* - DuplicateRecordException The budget name already exists. Budget names must be unique within an
* account.
* - AccessDeniedException You are not authorized to use this operation with the given parameters.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - BudgetsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample BudgetsAsyncClient.UpdateSubscriber
*/
default CompletableFuture updateSubscriber(
Consumer updateSubscriberRequest) {
return updateSubscriber(UpdateSubscriberRequest.builder().applyMutation(updateSubscriberRequest).build());
}
}