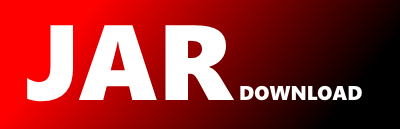
software.amazon.awssdk.services.cleanrooms.model.MemberSummary Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cleanrooms.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The member object listed by the request.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class MemberSummary implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("accountId").getter(getter(MemberSummary::accountId)).setter(setter(Builder::accountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("accountId").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(MemberSummary::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField DISPLAY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("displayName").getter(getter(MemberSummary::displayName)).setter(setter(Builder::displayName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("displayName").build()).build();
private static final SdkField> ABILITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("abilities")
.getter(getter(MemberSummary::abilitiesAsStrings))
.setter(setter(Builder::abilitiesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("abilities").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("createTime").getter(getter(MemberSummary::createTime)).setter(setter(Builder::createTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createTime").build()).build();
private static final SdkField UPDATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("updateTime").getter(getter(MemberSummary::updateTime)).setter(setter(Builder::updateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updateTime").build()).build();
private static final SdkField MEMBERSHIP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("membershipId").getter(getter(MemberSummary::membershipId)).setter(setter(Builder::membershipId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("membershipId").build()).build();
private static final SdkField MEMBERSHIP_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("membershipArn").getter(getter(MemberSummary::membershipArn)).setter(setter(Builder::membershipArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("membershipArn").build()).build();
private static final SdkField PAYMENT_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("paymentConfiguration")
.getter(getter(MemberSummary::paymentConfiguration)).setter(setter(Builder::paymentConfiguration))
.constructor(PaymentConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("paymentConfiguration").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACCOUNT_ID_FIELD,
STATUS_FIELD, DISPLAY_NAME_FIELD, ABILITIES_FIELD, CREATE_TIME_FIELD, UPDATE_TIME_FIELD, MEMBERSHIP_ID_FIELD,
MEMBERSHIP_ARN_FIELD, PAYMENT_CONFIGURATION_FIELD));
private static final long serialVersionUID = 1L;
private final String accountId;
private final String status;
private final String displayName;
private final List abilities;
private final Instant createTime;
private final Instant updateTime;
private final String membershipId;
private final String membershipArn;
private final PaymentConfiguration paymentConfiguration;
private MemberSummary(BuilderImpl builder) {
this.accountId = builder.accountId;
this.status = builder.status;
this.displayName = builder.displayName;
this.abilities = builder.abilities;
this.createTime = builder.createTime;
this.updateTime = builder.updateTime;
this.membershipId = builder.membershipId;
this.membershipArn = builder.membershipArn;
this.paymentConfiguration = builder.paymentConfiguration;
}
/**
*
* The identifier used to reference members of the collaboration. Currently only supports Amazon Web Services
* account ID.
*
*
* @return The identifier used to reference members of the collaboration. Currently only supports Amazon Web
* Services account ID.
*/
public final String accountId() {
return accountId;
}
/**
*
* The status of the member.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link MemberStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the member.
* @see MemberStatus
*/
public final MemberStatus status() {
return MemberStatus.fromValue(status);
}
/**
*
* The status of the member.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link MemberStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the member.
* @see MemberStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The member's display name.
*
*
* @return The member's display name.
*/
public final String displayName() {
return displayName;
}
/**
*
* The abilities granted to the collaboration member.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAbilities} method.
*
*
* @return The abilities granted to the collaboration member.
*/
public final List abilities() {
return MemberAbilitiesCopier.copyStringToEnum(abilities);
}
/**
* For responses, this returns true if the service returned a value for the Abilities property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasAbilities() {
return abilities != null && !(abilities instanceof SdkAutoConstructList);
}
/**
*
* The abilities granted to the collaboration member.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAbilities} method.
*
*
* @return The abilities granted to the collaboration member.
*/
public final List abilitiesAsStrings() {
return abilities;
}
/**
*
* The time when the member was created.
*
*
* @return The time when the member was created.
*/
public final Instant createTime() {
return createTime;
}
/**
*
* The time the member metadata was last updated.
*
*
* @return The time the member metadata was last updated.
*/
public final Instant updateTime() {
return updateTime;
}
/**
*
* The unique ID for the member's associated membership, if present.
*
*
* @return The unique ID for the member's associated membership, if present.
*/
public final String membershipId() {
return membershipId;
}
/**
*
* The unique ARN for the member's associated membership, if present.
*
*
* @return The unique ARN for the member's associated membership, if present.
*/
public final String membershipArn() {
return membershipArn;
}
/**
*
* The collaboration member's payment responsibilities set by the collaboration creator.
*
*
* @return The collaboration member's payment responsibilities set by the collaboration creator.
*/
public final PaymentConfiguration paymentConfiguration() {
return paymentConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(accountId());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(displayName());
hashCode = 31 * hashCode + Objects.hashCode(hasAbilities() ? abilitiesAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(createTime());
hashCode = 31 * hashCode + Objects.hashCode(updateTime());
hashCode = 31 * hashCode + Objects.hashCode(membershipId());
hashCode = 31 * hashCode + Objects.hashCode(membershipArn());
hashCode = 31 * hashCode + Objects.hashCode(paymentConfiguration());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MemberSummary)) {
return false;
}
MemberSummary other = (MemberSummary) obj;
return Objects.equals(accountId(), other.accountId()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(displayName(), other.displayName()) && hasAbilities() == other.hasAbilities()
&& Objects.equals(abilitiesAsStrings(), other.abilitiesAsStrings())
&& Objects.equals(createTime(), other.createTime()) && Objects.equals(updateTime(), other.updateTime())
&& Objects.equals(membershipId(), other.membershipId()) && Objects.equals(membershipArn(), other.membershipArn())
&& Objects.equals(paymentConfiguration(), other.paymentConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("MemberSummary").add("AccountId", accountId()).add("Status", statusAsString())
.add("DisplayName", displayName()).add("Abilities", hasAbilities() ? abilitiesAsStrings() : null)
.add("CreateTime", createTime()).add("UpdateTime", updateTime()).add("MembershipId", membershipId())
.add("MembershipArn", membershipArn()).add("PaymentConfiguration", paymentConfiguration()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "accountId":
return Optional.ofNullable(clazz.cast(accountId()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "displayName":
return Optional.ofNullable(clazz.cast(displayName()));
case "abilities":
return Optional.ofNullable(clazz.cast(abilitiesAsStrings()));
case "createTime":
return Optional.ofNullable(clazz.cast(createTime()));
case "updateTime":
return Optional.ofNullable(clazz.cast(updateTime()));
case "membershipId":
return Optional.ofNullable(clazz.cast(membershipId()));
case "membershipArn":
return Optional.ofNullable(clazz.cast(membershipArn()));
case "paymentConfiguration":
return Optional.ofNullable(clazz.cast(paymentConfiguration()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function