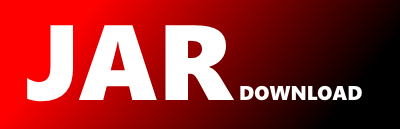
software.amazon.awssdk.services.clouddirectory.model.BatchCreateObject Maven / Gradle / Ivy
Show all versions of clouddirectory Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.clouddirectory.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the output of a CreateObject operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class BatchCreateObject implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> SCHEMA_FACET_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SchemaFacet")
.getter(getter(BatchCreateObject::schemaFacet))
.setter(setter(Builder::schemaFacet))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SchemaFacet").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SchemaFacet::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> OBJECT_ATTRIBUTE_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ObjectAttributeList")
.getter(getter(BatchCreateObject::objectAttributeList))
.setter(setter(Builder::objectAttributeList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ObjectAttributeList").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AttributeKeyAndValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PARENT_REFERENCE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ParentReference")
.getter(getter(BatchCreateObject::parentReference)).setter(setter(Builder::parentReference))
.constructor(ObjectReference::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ParentReference").build()).build();
private static final SdkField LINK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LinkName").getter(getter(BatchCreateObject::linkName)).setter(setter(Builder::linkName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LinkName").build()).build();
private static final SdkField BATCH_REFERENCE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BatchReferenceName").getter(getter(BatchCreateObject::batchReferenceName))
.setter(setter(Builder::batchReferenceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BatchReferenceName").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SCHEMA_FACET_FIELD,
OBJECT_ATTRIBUTE_LIST_FIELD, PARENT_REFERENCE_FIELD, LINK_NAME_FIELD, BATCH_REFERENCE_NAME_FIELD));
private static final long serialVersionUID = 1L;
private final List schemaFacet;
private final List objectAttributeList;
private final ObjectReference parentReference;
private final String linkName;
private final String batchReferenceName;
private BatchCreateObject(BuilderImpl builder) {
this.schemaFacet = builder.schemaFacet;
this.objectAttributeList = builder.objectAttributeList;
this.parentReference = builder.parentReference;
this.linkName = builder.linkName;
this.batchReferenceName = builder.batchReferenceName;
}
/**
* Returns true if the SchemaFacet property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasSchemaFacet() {
return schemaFacet != null && !(schemaFacet instanceof SdkAutoConstructList);
}
/**
*
* A list of FacetArns
that will be associated with the object. For more information, see arns.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasSchemaFacet()} to see if a value was sent in this field.
*
*
* @return A list of FacetArns
that will be associated with the object. For more information, see
* arns.
*/
public final List schemaFacet() {
return schemaFacet;
}
/**
* Returns true if the ObjectAttributeList property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasObjectAttributeList() {
return objectAttributeList != null && !(objectAttributeList instanceof SdkAutoConstructList);
}
/**
*
* An attribute map, which contains an attribute ARN as the key and attribute value as the map value.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasObjectAttributeList()} to see if a value was sent in this field.
*
*
* @return An attribute map, which contains an attribute ARN as the key and attribute value as the map value.
*/
public final List objectAttributeList() {
return objectAttributeList;
}
/**
*
* If specified, the parent reference to which this object will be attached.
*
*
* @return If specified, the parent reference to which this object will be attached.
*/
public final ObjectReference parentReference() {
return parentReference;
}
/**
*
* The name of the link.
*
*
* @return The name of the link.
*/
public final String linkName() {
return linkName;
}
/**
*
* The batch reference name. See Transaction
* Support for more information.
*
*
* @return The batch reference name. See Transaction Support for more information.
*/
public final String batchReferenceName() {
return batchReferenceName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasSchemaFacet() ? schemaFacet() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasObjectAttributeList() ? objectAttributeList() : null);
hashCode = 31 * hashCode + Objects.hashCode(parentReference());
hashCode = 31 * hashCode + Objects.hashCode(linkName());
hashCode = 31 * hashCode + Objects.hashCode(batchReferenceName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BatchCreateObject)) {
return false;
}
BatchCreateObject other = (BatchCreateObject) obj;
return hasSchemaFacet() == other.hasSchemaFacet() && Objects.equals(schemaFacet(), other.schemaFacet())
&& hasObjectAttributeList() == other.hasObjectAttributeList()
&& Objects.equals(objectAttributeList(), other.objectAttributeList())
&& Objects.equals(parentReference(), other.parentReference()) && Objects.equals(linkName(), other.linkName())
&& Objects.equals(batchReferenceName(), other.batchReferenceName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("BatchCreateObject").add("SchemaFacet", hasSchemaFacet() ? schemaFacet() : null)
.add("ObjectAttributeList", hasObjectAttributeList() ? objectAttributeList() : null)
.add("ParentReference", parentReference()).add("LinkName", linkName())
.add("BatchReferenceName", batchReferenceName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "SchemaFacet":
return Optional.ofNullable(clazz.cast(schemaFacet()));
case "ObjectAttributeList":
return Optional.ofNullable(clazz.cast(objectAttributeList()));
case "ParentReference":
return Optional.ofNullable(clazz.cast(parentReference()));
case "LinkName":
return Optional.ofNullable(clazz.cast(linkName()));
case "BatchReferenceName":
return Optional.ofNullable(clazz.cast(batchReferenceName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function