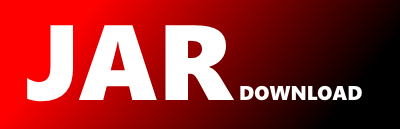
software.amazon.awssdk.services.clouddirectory.CloudDirectoryClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.clouddirectory;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.clouddirectory.model.AccessDeniedException;
import software.amazon.awssdk.services.clouddirectory.model.AddFacetToObjectRequest;
import software.amazon.awssdk.services.clouddirectory.model.AddFacetToObjectResponse;
import software.amazon.awssdk.services.clouddirectory.model.ApplySchemaRequest;
import software.amazon.awssdk.services.clouddirectory.model.ApplySchemaResponse;
import software.amazon.awssdk.services.clouddirectory.model.AttachObjectRequest;
import software.amazon.awssdk.services.clouddirectory.model.AttachObjectResponse;
import software.amazon.awssdk.services.clouddirectory.model.AttachPolicyRequest;
import software.amazon.awssdk.services.clouddirectory.model.AttachPolicyResponse;
import software.amazon.awssdk.services.clouddirectory.model.AttachToIndexRequest;
import software.amazon.awssdk.services.clouddirectory.model.AttachToIndexResponse;
import software.amazon.awssdk.services.clouddirectory.model.AttachTypedLinkRequest;
import software.amazon.awssdk.services.clouddirectory.model.AttachTypedLinkResponse;
import software.amazon.awssdk.services.clouddirectory.model.BatchReadRequest;
import software.amazon.awssdk.services.clouddirectory.model.BatchReadResponse;
import software.amazon.awssdk.services.clouddirectory.model.BatchWriteException;
import software.amazon.awssdk.services.clouddirectory.model.BatchWriteRequest;
import software.amazon.awssdk.services.clouddirectory.model.BatchWriteResponse;
import software.amazon.awssdk.services.clouddirectory.model.CannotListParentOfRootException;
import software.amazon.awssdk.services.clouddirectory.model.CloudDirectoryException;
import software.amazon.awssdk.services.clouddirectory.model.CreateDirectoryRequest;
import software.amazon.awssdk.services.clouddirectory.model.CreateDirectoryResponse;
import software.amazon.awssdk.services.clouddirectory.model.CreateFacetRequest;
import software.amazon.awssdk.services.clouddirectory.model.CreateFacetResponse;
import software.amazon.awssdk.services.clouddirectory.model.CreateIndexRequest;
import software.amazon.awssdk.services.clouddirectory.model.CreateIndexResponse;
import software.amazon.awssdk.services.clouddirectory.model.CreateObjectRequest;
import software.amazon.awssdk.services.clouddirectory.model.CreateObjectResponse;
import software.amazon.awssdk.services.clouddirectory.model.CreateSchemaRequest;
import software.amazon.awssdk.services.clouddirectory.model.CreateSchemaResponse;
import software.amazon.awssdk.services.clouddirectory.model.CreateTypedLinkFacetRequest;
import software.amazon.awssdk.services.clouddirectory.model.CreateTypedLinkFacetResponse;
import software.amazon.awssdk.services.clouddirectory.model.DeleteDirectoryRequest;
import software.amazon.awssdk.services.clouddirectory.model.DeleteDirectoryResponse;
import software.amazon.awssdk.services.clouddirectory.model.DeleteFacetRequest;
import software.amazon.awssdk.services.clouddirectory.model.DeleteFacetResponse;
import software.amazon.awssdk.services.clouddirectory.model.DeleteObjectRequest;
import software.amazon.awssdk.services.clouddirectory.model.DeleteObjectResponse;
import software.amazon.awssdk.services.clouddirectory.model.DeleteSchemaRequest;
import software.amazon.awssdk.services.clouddirectory.model.DeleteSchemaResponse;
import software.amazon.awssdk.services.clouddirectory.model.DeleteTypedLinkFacetRequest;
import software.amazon.awssdk.services.clouddirectory.model.DeleteTypedLinkFacetResponse;
import software.amazon.awssdk.services.clouddirectory.model.DetachFromIndexRequest;
import software.amazon.awssdk.services.clouddirectory.model.DetachFromIndexResponse;
import software.amazon.awssdk.services.clouddirectory.model.DetachObjectRequest;
import software.amazon.awssdk.services.clouddirectory.model.DetachObjectResponse;
import software.amazon.awssdk.services.clouddirectory.model.DetachPolicyRequest;
import software.amazon.awssdk.services.clouddirectory.model.DetachPolicyResponse;
import software.amazon.awssdk.services.clouddirectory.model.DetachTypedLinkRequest;
import software.amazon.awssdk.services.clouddirectory.model.DetachTypedLinkResponse;
import software.amazon.awssdk.services.clouddirectory.model.DirectoryAlreadyExistsException;
import software.amazon.awssdk.services.clouddirectory.model.DirectoryDeletedException;
import software.amazon.awssdk.services.clouddirectory.model.DirectoryNotDisabledException;
import software.amazon.awssdk.services.clouddirectory.model.DirectoryNotEnabledException;
import software.amazon.awssdk.services.clouddirectory.model.DisableDirectoryRequest;
import software.amazon.awssdk.services.clouddirectory.model.DisableDirectoryResponse;
import software.amazon.awssdk.services.clouddirectory.model.EnableDirectoryRequest;
import software.amazon.awssdk.services.clouddirectory.model.EnableDirectoryResponse;
import software.amazon.awssdk.services.clouddirectory.model.FacetAlreadyExistsException;
import software.amazon.awssdk.services.clouddirectory.model.FacetInUseException;
import software.amazon.awssdk.services.clouddirectory.model.FacetNotFoundException;
import software.amazon.awssdk.services.clouddirectory.model.FacetValidationException;
import software.amazon.awssdk.services.clouddirectory.model.GetAppliedSchemaVersionRequest;
import software.amazon.awssdk.services.clouddirectory.model.GetAppliedSchemaVersionResponse;
import software.amazon.awssdk.services.clouddirectory.model.GetDirectoryRequest;
import software.amazon.awssdk.services.clouddirectory.model.GetDirectoryResponse;
import software.amazon.awssdk.services.clouddirectory.model.GetFacetRequest;
import software.amazon.awssdk.services.clouddirectory.model.GetFacetResponse;
import software.amazon.awssdk.services.clouddirectory.model.GetLinkAttributesRequest;
import software.amazon.awssdk.services.clouddirectory.model.GetLinkAttributesResponse;
import software.amazon.awssdk.services.clouddirectory.model.GetObjectAttributesRequest;
import software.amazon.awssdk.services.clouddirectory.model.GetObjectAttributesResponse;
import software.amazon.awssdk.services.clouddirectory.model.GetObjectInformationRequest;
import software.amazon.awssdk.services.clouddirectory.model.GetObjectInformationResponse;
import software.amazon.awssdk.services.clouddirectory.model.GetSchemaAsJsonRequest;
import software.amazon.awssdk.services.clouddirectory.model.GetSchemaAsJsonResponse;
import software.amazon.awssdk.services.clouddirectory.model.GetTypedLinkFacetInformationRequest;
import software.amazon.awssdk.services.clouddirectory.model.GetTypedLinkFacetInformationResponse;
import software.amazon.awssdk.services.clouddirectory.model.IncompatibleSchemaException;
import software.amazon.awssdk.services.clouddirectory.model.IndexedAttributeMissingException;
import software.amazon.awssdk.services.clouddirectory.model.InternalServiceException;
import software.amazon.awssdk.services.clouddirectory.model.InvalidArnException;
import software.amazon.awssdk.services.clouddirectory.model.InvalidAttachmentException;
import software.amazon.awssdk.services.clouddirectory.model.InvalidFacetUpdateException;
import software.amazon.awssdk.services.clouddirectory.model.InvalidNextTokenException;
import software.amazon.awssdk.services.clouddirectory.model.InvalidRuleException;
import software.amazon.awssdk.services.clouddirectory.model.InvalidSchemaDocException;
import software.amazon.awssdk.services.clouddirectory.model.InvalidTaggingRequestException;
import software.amazon.awssdk.services.clouddirectory.model.LimitExceededException;
import software.amazon.awssdk.services.clouddirectory.model.LinkNameAlreadyInUseException;
import software.amazon.awssdk.services.clouddirectory.model.ListAppliedSchemaArnsRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListAppliedSchemaArnsResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListAttachedIndicesRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListAttachedIndicesResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListDevelopmentSchemaArnsRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListDevelopmentSchemaArnsResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListDirectoriesRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListDirectoriesResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListFacetAttributesRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListFacetAttributesResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListFacetNamesRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListFacetNamesResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListIncomingTypedLinksRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListIncomingTypedLinksResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListIndexRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListIndexResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListManagedSchemaArnsRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListManagedSchemaArnsResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListObjectAttributesRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListObjectAttributesResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListObjectChildrenRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListObjectChildrenResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListObjectParentPathsRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListObjectParentPathsResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListObjectParentsRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListObjectParentsResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListObjectPoliciesRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListObjectPoliciesResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListOutgoingTypedLinksRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListOutgoingTypedLinksResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListPolicyAttachmentsRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListPolicyAttachmentsResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListPublishedSchemaArnsRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListPublishedSchemaArnsResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetAttributesRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetAttributesResponse;
import software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetNamesRequest;
import software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetNamesResponse;
import software.amazon.awssdk.services.clouddirectory.model.LookupPolicyRequest;
import software.amazon.awssdk.services.clouddirectory.model.LookupPolicyResponse;
import software.amazon.awssdk.services.clouddirectory.model.NotIndexException;
import software.amazon.awssdk.services.clouddirectory.model.NotNodeException;
import software.amazon.awssdk.services.clouddirectory.model.NotPolicyException;
import software.amazon.awssdk.services.clouddirectory.model.ObjectAlreadyDetachedException;
import software.amazon.awssdk.services.clouddirectory.model.ObjectNotDetachedException;
import software.amazon.awssdk.services.clouddirectory.model.PublishSchemaRequest;
import software.amazon.awssdk.services.clouddirectory.model.PublishSchemaResponse;
import software.amazon.awssdk.services.clouddirectory.model.PutSchemaFromJsonRequest;
import software.amazon.awssdk.services.clouddirectory.model.PutSchemaFromJsonResponse;
import software.amazon.awssdk.services.clouddirectory.model.RemoveFacetFromObjectRequest;
import software.amazon.awssdk.services.clouddirectory.model.RemoveFacetFromObjectResponse;
import software.amazon.awssdk.services.clouddirectory.model.ResourceNotFoundException;
import software.amazon.awssdk.services.clouddirectory.model.RetryableConflictException;
import software.amazon.awssdk.services.clouddirectory.model.SchemaAlreadyExistsException;
import software.amazon.awssdk.services.clouddirectory.model.SchemaAlreadyPublishedException;
import software.amazon.awssdk.services.clouddirectory.model.StillContainsLinksException;
import software.amazon.awssdk.services.clouddirectory.model.TagResourceRequest;
import software.amazon.awssdk.services.clouddirectory.model.TagResourceResponse;
import software.amazon.awssdk.services.clouddirectory.model.UnsupportedIndexTypeException;
import software.amazon.awssdk.services.clouddirectory.model.UntagResourceRequest;
import software.amazon.awssdk.services.clouddirectory.model.UntagResourceResponse;
import software.amazon.awssdk.services.clouddirectory.model.UpdateFacetRequest;
import software.amazon.awssdk.services.clouddirectory.model.UpdateFacetResponse;
import software.amazon.awssdk.services.clouddirectory.model.UpdateLinkAttributesRequest;
import software.amazon.awssdk.services.clouddirectory.model.UpdateLinkAttributesResponse;
import software.amazon.awssdk.services.clouddirectory.model.UpdateObjectAttributesRequest;
import software.amazon.awssdk.services.clouddirectory.model.UpdateObjectAttributesResponse;
import software.amazon.awssdk.services.clouddirectory.model.UpdateSchemaRequest;
import software.amazon.awssdk.services.clouddirectory.model.UpdateSchemaResponse;
import software.amazon.awssdk.services.clouddirectory.model.UpdateTypedLinkFacetRequest;
import software.amazon.awssdk.services.clouddirectory.model.UpdateTypedLinkFacetResponse;
import software.amazon.awssdk.services.clouddirectory.model.UpgradeAppliedSchemaRequest;
import software.amazon.awssdk.services.clouddirectory.model.UpgradeAppliedSchemaResponse;
import software.amazon.awssdk.services.clouddirectory.model.UpgradePublishedSchemaRequest;
import software.amazon.awssdk.services.clouddirectory.model.UpgradePublishedSchemaResponse;
import software.amazon.awssdk.services.clouddirectory.model.ValidationException;
import software.amazon.awssdk.services.clouddirectory.paginators.ListAppliedSchemaArnsIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListAttachedIndicesIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListDevelopmentSchemaArnsIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListDirectoriesIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListFacetAttributesIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListFacetNamesIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListIndexIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListManagedSchemaArnsIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListObjectAttributesIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListObjectChildrenIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentPathsIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentsIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListObjectPoliciesIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListPolicyAttachmentsIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListPublishedSchemaArnsIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListTagsForResourceIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetAttributesIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetNamesIterable;
import software.amazon.awssdk.services.clouddirectory.paginators.LookupPolicyIterable;
/**
* Service client for accessing Amazon CloudDirectory. This can be created using the static {@link #builder()} method.
*
* Amazon Cloud Directory
*
* Amazon Cloud Directory is a component of the AWS Directory Service that simplifies the development and management of
* cloud-scale web, mobile, and IoT applications. This guide describes the Cloud Directory operations that you can call
* programmatically and includes detailed information on data types and errors. For information about Cloud Directory
* features, see AWS Directory Service and the Amazon Cloud
* Directory Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface CloudDirectoryClient extends AwsClient {
String SERVICE_NAME = "clouddirectory";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "clouddirectory";
/**
*
* Adds a new Facet to an object. An object can have more than one facet applied on it.
*
*
* @param addFacetToObjectRequest
* @return Result of the AddFacetToObject operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.AddFacetToObject
* @see AWS API Documentation
*/
default AddFacetToObjectResponse addFacetToObject(AddFacetToObjectRequest addFacetToObjectRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Adds a new Facet to an object. An object can have more than one facet applied on it.
*
*
*
* This is a convenience which creates an instance of the {@link AddFacetToObjectRequest.Builder} avoiding the need
* to create one manually via {@link AddFacetToObjectRequest#builder()}
*
*
* @param addFacetToObjectRequest
* A {@link Consumer} that will call methods on {@link AddFacetToObjectRequest.Builder} to create a request.
* @return Result of the AddFacetToObject operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.AddFacetToObject
* @see AWS API Documentation
*/
default AddFacetToObjectResponse addFacetToObject(Consumer addFacetToObjectRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
return addFacetToObject(AddFacetToObjectRequest.builder().applyMutation(addFacetToObjectRequest).build());
}
/**
*
* Copies the input published schema, at the specified version, into the Directory with the same name and
* version as that of the published schema.
*
*
* @param applySchemaRequest
* @return Result of the ApplySchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws SchemaAlreadyExistsException
* Indicates that a schema could not be created due to a naming conflict. Please select a different name and
* then try again.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidAttachmentException
* Indicates that an attempt to make an attachment was invalid. For example, attaching two nodes with a link
* type that is not applicable to the nodes or attempting to apply a schema to a directory a second time.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ApplySchema
* @see AWS
* API Documentation
*/
default ApplySchemaResponse applySchema(ApplySchemaRequest applySchemaRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
SchemaAlreadyExistsException, ResourceNotFoundException, InvalidAttachmentException, AwsServiceException,
SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Copies the input published schema, at the specified version, into the Directory with the same name and
* version as that of the published schema.
*
*
*
* This is a convenience which creates an instance of the {@link ApplySchemaRequest.Builder} avoiding the need to
* create one manually via {@link ApplySchemaRequest#builder()}
*
*
* @param applySchemaRequest
* A {@link Consumer} that will call methods on {@link ApplySchemaRequest.Builder} to create a request.
* @return Result of the ApplySchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws SchemaAlreadyExistsException
* Indicates that a schema could not be created due to a naming conflict. Please select a different name and
* then try again.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidAttachmentException
* Indicates that an attempt to make an attachment was invalid. For example, attaching two nodes with a link
* type that is not applicable to the nodes or attempting to apply a schema to a directory a second time.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ApplySchema
* @see AWS
* API Documentation
*/
default ApplySchemaResponse applySchema(Consumer applySchemaRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, SchemaAlreadyExistsException, ResourceNotFoundException,
InvalidAttachmentException, AwsServiceException, SdkClientException, CloudDirectoryException {
return applySchema(ApplySchemaRequest.builder().applyMutation(applySchemaRequest).build());
}
/**
*
* Attaches an existing object to another object. An object can be accessed in two ways:
*
*
* -
*
* Using the path
*
*
* -
*
* Using ObjectIdentifier
*
*
*
*
* @param attachObjectRequest
* @return Result of the AttachObject operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws LinkNameAlreadyInUseException
* Indicates that a link could not be created due to a naming conflict. Choose a different name and then try
* again.
* @throws InvalidAttachmentException
* Indicates that an attempt to make an attachment was invalid. For example, attaching two nodes with a link
* type that is not applicable to the nodes or attempting to apply a schema to a directory a second time.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.AttachObject
* @see AWS
* API Documentation
*/
default AttachObjectResponse attachObject(AttachObjectRequest attachObjectRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, LinkNameAlreadyInUseException, InvalidAttachmentException,
FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Attaches an existing object to another object. An object can be accessed in two ways:
*
*
* -
*
* Using the path
*
*
* -
*
* Using ObjectIdentifier
*
*
*
*
*
* This is a convenience which creates an instance of the {@link AttachObjectRequest.Builder} avoiding the need to
* create one manually via {@link AttachObjectRequest#builder()}
*
*
* @param attachObjectRequest
* A {@link Consumer} that will call methods on {@link AttachObjectRequest.Builder} to create a request.
* @return Result of the AttachObject operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws LinkNameAlreadyInUseException
* Indicates that a link could not be created due to a naming conflict. Choose a different name and then try
* again.
* @throws InvalidAttachmentException
* Indicates that an attempt to make an attachment was invalid. For example, attaching two nodes with a link
* type that is not applicable to the nodes or attempting to apply a schema to a directory a second time.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.AttachObject
* @see AWS
* API Documentation
*/
default AttachObjectResponse attachObject(Consumer attachObjectRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
LinkNameAlreadyInUseException, InvalidAttachmentException, FacetValidationException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return attachObject(AttachObjectRequest.builder().applyMutation(attachObjectRequest).build());
}
/**
*
* Attaches a policy object to a regular object. An object can have a limited number of attached policies.
*
*
* @param attachPolicyRequest
* @return Result of the AttachPolicy operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotPolicyException
* Indicates that the requested operation can only operate on policy objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.AttachPolicy
* @see AWS
* API Documentation
*/
default AttachPolicyResponse attachPolicy(AttachPolicyRequest attachPolicyRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, NotPolicyException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Attaches a policy object to a regular object. An object can have a limited number of attached policies.
*
*
*
* This is a convenience which creates an instance of the {@link AttachPolicyRequest.Builder} avoiding the need to
* create one manually via {@link AttachPolicyRequest#builder()}
*
*
* @param attachPolicyRequest
* A {@link Consumer} that will call methods on {@link AttachPolicyRequest.Builder} to create a request.
* @return Result of the AttachPolicy operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotPolicyException
* Indicates that the requested operation can only operate on policy objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.AttachPolicy
* @see AWS
* API Documentation
*/
default AttachPolicyResponse attachPolicy(Consumer attachPolicyRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
NotPolicyException, AwsServiceException, SdkClientException, CloudDirectoryException {
return attachPolicy(AttachPolicyRequest.builder().applyMutation(attachPolicyRequest).build());
}
/**
*
* Attaches the specified object to the specified index.
*
*
* @param attachToIndexRequest
* @return Result of the AttachToIndex operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidAttachmentException
* Indicates that an attempt to make an attachment was invalid. For example, attaching two nodes with a link
* type that is not applicable to the nodes or attempting to apply a schema to a directory a second time.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws LinkNameAlreadyInUseException
* Indicates that a link could not be created due to a naming conflict. Choose a different name and then try
* again.
* @throws IndexedAttributeMissingException
* An object has been attempted to be attached to an object that does not have the appropriate attribute
* value.
* @throws NotIndexException
* Indicates that the requested operation can only operate on index objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.AttachToIndex
* @see AWS
* API Documentation
*/
default AttachToIndexResponse attachToIndex(AttachToIndexRequest attachToIndexRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, InvalidAttachmentException, ResourceNotFoundException, LinkNameAlreadyInUseException,
IndexedAttributeMissingException, NotIndexException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Attaches the specified object to the specified index.
*
*
*
* This is a convenience which creates an instance of the {@link AttachToIndexRequest.Builder} avoiding the need to
* create one manually via {@link AttachToIndexRequest#builder()}
*
*
* @param attachToIndexRequest
* A {@link Consumer} that will call methods on {@link AttachToIndexRequest.Builder} to create a request.
* @return Result of the AttachToIndex operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidAttachmentException
* Indicates that an attempt to make an attachment was invalid. For example, attaching two nodes with a link
* type that is not applicable to the nodes or attempting to apply a schema to a directory a second time.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws LinkNameAlreadyInUseException
* Indicates that a link could not be created due to a naming conflict. Choose a different name and then try
* again.
* @throws IndexedAttributeMissingException
* An object has been attempted to be attached to an object that does not have the appropriate attribute
* value.
* @throws NotIndexException
* Indicates that the requested operation can only operate on index objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.AttachToIndex
* @see AWS
* API Documentation
*/
default AttachToIndexResponse attachToIndex(Consumer attachToIndexRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, InvalidAttachmentException,
ResourceNotFoundException, LinkNameAlreadyInUseException, IndexedAttributeMissingException, NotIndexException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return attachToIndex(AttachToIndexRequest.builder().applyMutation(attachToIndexRequest).build());
}
/**
*
* Attaches a typed link to a specified source and target object. For more information, see Typed Links.
*
*
* @param attachTypedLinkRequest
* @return Result of the AttachTypedLink operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidAttachmentException
* Indicates that an attempt to make an attachment was invalid. For example, attaching two nodes with a link
* type that is not applicable to the nodes or attempting to apply a schema to a directory a second time.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.AttachTypedLink
* @see AWS API Documentation
*/
default AttachTypedLinkResponse attachTypedLink(AttachTypedLinkRequest attachTypedLinkRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidAttachmentException, FacetValidationException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Attaches a typed link to a specified source and target object. For more information, see Typed Links.
*
*
*
* This is a convenience which creates an instance of the {@link AttachTypedLinkRequest.Builder} avoiding the need
* to create one manually via {@link AttachTypedLinkRequest#builder()}
*
*
* @param attachTypedLinkRequest
* A {@link Consumer} that will call methods on {@link AttachTypedLinkRequest.Builder} to create a request.
* @return Result of the AttachTypedLink operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidAttachmentException
* Indicates that an attempt to make an attachment was invalid. For example, attaching two nodes with a link
* type that is not applicable to the nodes or attempting to apply a schema to a directory a second time.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.AttachTypedLink
* @see AWS API Documentation
*/
default AttachTypedLinkResponse attachTypedLink(Consumer attachTypedLinkRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidAttachmentException, FacetValidationException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return attachTypedLink(AttachTypedLinkRequest.builder().applyMutation(attachTypedLinkRequest).build());
}
/**
*
* Performs all the read operations in a batch.
*
*
* @param batchReadRequest
* @return Result of the BatchRead operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.BatchRead
* @see AWS API
* Documentation
*/
default BatchReadResponse batchRead(BatchReadRequest batchReadRequest) throws InternalServiceException, InvalidArnException,
RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Performs all the read operations in a batch.
*
*
*
* This is a convenience which creates an instance of the {@link BatchReadRequest.Builder} avoiding the need to
* create one manually via {@link BatchReadRequest#builder()}
*
*
* @param batchReadRequest
* A {@link Consumer} that will call methods on {@link BatchReadRequest.Builder} to create a request.
* @return Result of the BatchRead operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.BatchRead
* @see AWS API
* Documentation
*/
default BatchReadResponse batchRead(Consumer batchReadRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, AwsServiceException, SdkClientException, CloudDirectoryException {
return batchRead(BatchReadRequest.builder().applyMutation(batchReadRequest).build());
}
/**
*
* Performs all the write operations in a batch. Either all the operations succeed or none.
*
*
* @param batchWriteRequest
* @return Result of the BatchWrite operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws BatchWriteException
* A BatchWrite
exception has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.BatchWrite
* @see AWS API
* Documentation
*/
default BatchWriteResponse batchWrite(BatchWriteRequest batchWriteRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, BatchWriteException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Performs all the write operations in a batch. Either all the operations succeed or none.
*
*
*
* This is a convenience which creates an instance of the {@link BatchWriteRequest.Builder} avoiding the need to
* create one manually via {@link BatchWriteRequest#builder()}
*
*
* @param batchWriteRequest
* A {@link Consumer} that will call methods on {@link BatchWriteRequest.Builder} to create a request.
* @return Result of the BatchWrite operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws BatchWriteException
* A BatchWrite
exception has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.BatchWrite
* @see AWS API
* Documentation
*/
default BatchWriteResponse batchWrite(Consumer batchWriteRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, BatchWriteException, AwsServiceException, SdkClientException, CloudDirectoryException {
return batchWrite(BatchWriteRequest.builder().applyMutation(batchWriteRequest).build());
}
/**
*
* Creates a Directory by copying the published schema into the directory. A directory cannot be created
* without a schema.
*
*
* You can also quickly create a directory using a managed schema, called the QuickStartSchema
. For
* more information, see Managed Schema
* in the Amazon Cloud Directory Developer Guide.
*
*
* @param createDirectoryRequest
* @return Result of the CreateDirectory operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryAlreadyExistsException
* Indicates that a Directory could not be created due to a naming conflict. Choose a different name
* and try again.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.CreateDirectory
* @see AWS API Documentation
*/
default CreateDirectoryResponse createDirectory(CreateDirectoryRequest createDirectoryRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryAlreadyExistsException, ResourceNotFoundException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a Directory by copying the published schema into the directory. A directory cannot be created
* without a schema.
*
*
* You can also quickly create a directory using a managed schema, called the QuickStartSchema
. For
* more information, see Managed Schema
* in the Amazon Cloud Directory Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDirectoryRequest.Builder} avoiding the need
* to create one manually via {@link CreateDirectoryRequest#builder()}
*
*
* @param createDirectoryRequest
* A {@link Consumer} that will call methods on {@link CreateDirectoryRequest.Builder} to create a request.
* @return Result of the CreateDirectory operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryAlreadyExistsException
* Indicates that a Directory could not be created due to a naming conflict. Choose a different name
* and try again.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.CreateDirectory
* @see AWS API Documentation
*/
default CreateDirectoryResponse createDirectory(Consumer createDirectoryRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryAlreadyExistsException, ResourceNotFoundException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return createDirectory(CreateDirectoryRequest.builder().applyMutation(createDirectoryRequest).build());
}
/**
*
* Creates a new Facet in a schema. Facet creation is allowed only in development or applied schemas.
*
*
* @param createFacetRequest
* @return Result of the CreateFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetAlreadyExistsException
* A facet with the same name already exists.
* @throws InvalidRuleException
* Occurs when any of the rule parameter keys or values are invalid.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.CreateFacet
* @see AWS
* API Documentation
*/
default CreateFacetResponse createFacet(CreateFacetRequest createFacetRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, FacetAlreadyExistsException, InvalidRuleException, FacetValidationException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new Facet in a schema. Facet creation is allowed only in development or applied schemas.
*
*
*
* This is a convenience which creates an instance of the {@link CreateFacetRequest.Builder} avoiding the need to
* create one manually via {@link CreateFacetRequest#builder()}
*
*
* @param createFacetRequest
* A {@link Consumer} that will call methods on {@link CreateFacetRequest.Builder} to create a request.
* @return Result of the CreateFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetAlreadyExistsException
* A facet with the same name already exists.
* @throws InvalidRuleException
* Occurs when any of the rule parameter keys or values are invalid.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.CreateFacet
* @see AWS
* API Documentation
*/
default CreateFacetResponse createFacet(Consumer createFacetRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, FacetAlreadyExistsException,
InvalidRuleException, FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
return createFacet(CreateFacetRequest.builder().applyMutation(createFacetRequest).build());
}
/**
*
* Creates an index object. See Indexing and
* search for more information.
*
*
* @param createIndexRequest
* @return Result of the CreateIndex operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws LinkNameAlreadyInUseException
* Indicates that a link could not be created due to a naming conflict. Choose a different name and then try
* again.
* @throws UnsupportedIndexTypeException
* Indicates that the requested index type is not supported.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.CreateIndex
* @see AWS
* API Documentation
*/
default CreateIndexResponse createIndex(CreateIndexRequest createIndexRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, FacetValidationException, LinkNameAlreadyInUseException,
UnsupportedIndexTypeException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an index object. See Indexing and
* search for more information.
*
*
*
* This is a convenience which creates an instance of the {@link CreateIndexRequest.Builder} avoiding the need to
* create one manually via {@link CreateIndexRequest#builder()}
*
*
* @param createIndexRequest
* A {@link Consumer} that will call methods on {@link CreateIndexRequest.Builder} to create a request.
* @return Result of the CreateIndex operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws LinkNameAlreadyInUseException
* Indicates that a link could not be created due to a naming conflict. Choose a different name and then try
* again.
* @throws UnsupportedIndexTypeException
* Indicates that the requested index type is not supported.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.CreateIndex
* @see AWS
* API Documentation
*/
default CreateIndexResponse createIndex(Consumer createIndexRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
FacetValidationException, LinkNameAlreadyInUseException, UnsupportedIndexTypeException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return createIndex(CreateIndexRequest.builder().applyMutation(createIndexRequest).build());
}
/**
*
* Creates an object in a Directory. Additionally attaches the object to a parent, if a parent reference and
* LinkName
is specified. An object is simply a collection of Facet attributes. You can also use
* this API call to create a policy object, if the facet from which you create the object is a policy facet.
*
*
* @param createObjectRequest
* @return Result of the CreateObject operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws LinkNameAlreadyInUseException
* Indicates that a link could not be created due to a naming conflict. Choose a different name and then try
* again.
* @throws UnsupportedIndexTypeException
* Indicates that the requested index type is not supported.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.CreateObject
* @see AWS
* API Documentation
*/
default CreateObjectResponse createObject(CreateObjectRequest createObjectRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, FacetValidationException, LinkNameAlreadyInUseException,
UnsupportedIndexTypeException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an object in a Directory. Additionally attaches the object to a parent, if a parent reference and
* LinkName
is specified. An object is simply a collection of Facet attributes. You can also use
* this API call to create a policy object, if the facet from which you create the object is a policy facet.
*
*
*
* This is a convenience which creates an instance of the {@link CreateObjectRequest.Builder} avoiding the need to
* create one manually via {@link CreateObjectRequest#builder()}
*
*
* @param createObjectRequest
* A {@link Consumer} that will call methods on {@link CreateObjectRequest.Builder} to create a request.
* @return Result of the CreateObject operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws LinkNameAlreadyInUseException
* Indicates that a link could not be created due to a naming conflict. Choose a different name and then try
* again.
* @throws UnsupportedIndexTypeException
* Indicates that the requested index type is not supported.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.CreateObject
* @see AWS
* API Documentation
*/
default CreateObjectResponse createObject(Consumer createObjectRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
FacetValidationException, LinkNameAlreadyInUseException, UnsupportedIndexTypeException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return createObject(CreateObjectRequest.builder().applyMutation(createObjectRequest).build());
}
/**
*
* Creates a new schema in a development state. A schema can exist in three phases:
*
*
* -
*
* Development: This is a mutable phase of the schema. All new schemas are in the development phase. Once the
* schema is finalized, it can be published.
*
*
* -
*
* Published: Published schemas are immutable and have a version associated with them.
*
*
* -
*
* Applied: Applied schemas are mutable in a way that allows you to add new schema facets. You can also add
* new, nonrequired attributes to existing schema facets. You can apply only published schemas to directories.
*
*
*
*
* @param createSchemaRequest
* @return Result of the CreateSchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws SchemaAlreadyExistsException
* Indicates that a schema could not be created due to a naming conflict. Please select a different name and
* then try again.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.CreateSchema
* @see AWS
* API Documentation
*/
default CreateSchemaResponse createSchema(CreateSchemaRequest createSchemaRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
SchemaAlreadyExistsException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new schema in a development state. A schema can exist in three phases:
*
*
* -
*
* Development: This is a mutable phase of the schema. All new schemas are in the development phase. Once the
* schema is finalized, it can be published.
*
*
* -
*
* Published: Published schemas are immutable and have a version associated with them.
*
*
* -
*
* Applied: Applied schemas are mutable in a way that allows you to add new schema facets. You can also add
* new, nonrequired attributes to existing schema facets. You can apply only published schemas to directories.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CreateSchemaRequest.Builder} avoiding the need to
* create one manually via {@link CreateSchemaRequest#builder()}
*
*
* @param createSchemaRequest
* A {@link Consumer} that will call methods on {@link CreateSchemaRequest.Builder} to create a request.
* @return Result of the CreateSchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws SchemaAlreadyExistsException
* Indicates that a schema could not be created due to a naming conflict. Please select a different name and
* then try again.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.CreateSchema
* @see AWS
* API Documentation
*/
default CreateSchemaResponse createSchema(Consumer createSchemaRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, SchemaAlreadyExistsException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return createSchema(CreateSchemaRequest.builder().applyMutation(createSchemaRequest).build());
}
/**
*
* Creates a TypedLinkFacet. For more information, see Typed Links.
*
*
* @param createTypedLinkFacetRequest
* @return Result of the CreateTypedLinkFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetAlreadyExistsException
* A facet with the same name already exists.
* @throws InvalidRuleException
* Occurs when any of the rule parameter keys or values are invalid.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.CreateTypedLinkFacet
* @see AWS API Documentation
*/
default CreateTypedLinkFacetResponse createTypedLinkFacet(CreateTypedLinkFacetRequest createTypedLinkFacetRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, FacetAlreadyExistsException,
InvalidRuleException, FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a TypedLinkFacet. For more information, see Typed Links.
*
*
*
* This is a convenience which creates an instance of the {@link CreateTypedLinkFacetRequest.Builder} avoiding the
* need to create one manually via {@link CreateTypedLinkFacetRequest#builder()}
*
*
* @param createTypedLinkFacetRequest
* A {@link Consumer} that will call methods on {@link CreateTypedLinkFacetRequest.Builder} to create a
* request.
* @return Result of the CreateTypedLinkFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetAlreadyExistsException
* A facet with the same name already exists.
* @throws InvalidRuleException
* Occurs when any of the rule parameter keys or values are invalid.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.CreateTypedLinkFacet
* @see AWS API Documentation
*/
default CreateTypedLinkFacetResponse createTypedLinkFacet(
Consumer createTypedLinkFacetRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, FacetAlreadyExistsException, InvalidRuleException, FacetValidationException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return createTypedLinkFacet(CreateTypedLinkFacetRequest.builder().applyMutation(createTypedLinkFacetRequest).build());
}
/**
*
* Deletes a directory. Only disabled directories can be deleted. A deleted directory cannot be undone. Exercise
* extreme caution when deleting directories.
*
*
* @param deleteDirectoryRequest
* @return Result of the DeleteDirectory operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws DirectoryNotDisabledException
* An operation can only operate on a disabled directory.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryDeletedException
* A directory that has been deleted and to which access has been attempted. Note: The requested resource
* will eventually cease to exist.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DeleteDirectory
* @see AWS API Documentation
*/
default DeleteDirectoryResponse deleteDirectory(DeleteDirectoryRequest deleteDirectoryRequest)
throws ResourceNotFoundException, DirectoryNotDisabledException, InternalServiceException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryDeletedException, RetryableConflictException,
InvalidArnException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a directory. Only disabled directories can be deleted. A deleted directory cannot be undone. Exercise
* extreme caution when deleting directories.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDirectoryRequest.Builder} avoiding the need
* to create one manually via {@link DeleteDirectoryRequest#builder()}
*
*
* @param deleteDirectoryRequest
* A {@link Consumer} that will call methods on {@link DeleteDirectoryRequest.Builder} to create a request.
* @return Result of the DeleteDirectory operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws DirectoryNotDisabledException
* An operation can only operate on a disabled directory.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryDeletedException
* A directory that has been deleted and to which access has been attempted. Note: The requested resource
* will eventually cease to exist.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DeleteDirectory
* @see AWS API Documentation
*/
default DeleteDirectoryResponse deleteDirectory(Consumer deleteDirectoryRequest)
throws ResourceNotFoundException, DirectoryNotDisabledException, InternalServiceException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryDeletedException, RetryableConflictException,
InvalidArnException, AwsServiceException, SdkClientException, CloudDirectoryException {
return deleteDirectory(DeleteDirectoryRequest.builder().applyMutation(deleteDirectoryRequest).build());
}
/**
*
* Deletes a given Facet. All attributes and Rules that are associated with the facet will be deleted.
* Only development schema facets are allowed deletion.
*
*
* @param deleteFacetRequest
* @return Result of the DeleteFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws FacetInUseException
* Occurs when deleting a facet that contains an attribute that is a target to an attribute reference in a
* different facet.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DeleteFacet
* @see AWS
* API Documentation
*/
default DeleteFacetResponse deleteFacet(DeleteFacetRequest deleteFacetRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, FacetNotFoundException, FacetInUseException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a given Facet. All attributes and Rules that are associated with the facet will be deleted.
* Only development schema facets are allowed deletion.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteFacetRequest.Builder} avoiding the need to
* create one manually via {@link DeleteFacetRequest#builder()}
*
*
* @param deleteFacetRequest
* A {@link Consumer} that will call methods on {@link DeleteFacetRequest.Builder} to create a request.
* @return Result of the DeleteFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws FacetInUseException
* Occurs when deleting a facet that contains an attribute that is a target to an attribute reference in a
* different facet.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DeleteFacet
* @see AWS
* API Documentation
*/
default DeleteFacetResponse deleteFacet(Consumer deleteFacetRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, FacetNotFoundException,
FacetInUseException, AwsServiceException, SdkClientException, CloudDirectoryException {
return deleteFacet(DeleteFacetRequest.builder().applyMutation(deleteFacetRequest).build());
}
/**
*
* Deletes an object and its associated attributes. Only objects with no children and no parents can be deleted. The
* maximum number of attributes that can be deleted during an object deletion is 30. For more information, see Amazon Cloud Directory
* Limits.
*
*
* @param deleteObjectRequest
* @return Result of the DeleteObject operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ObjectNotDetachedException
* Indicates that the requested operation cannot be completed because the object has not been detached from
* the tree.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DeleteObject
* @see AWS
* API Documentation
*/
default DeleteObjectResponse deleteObject(DeleteObjectRequest deleteObjectRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, ObjectNotDetachedException, AwsServiceException,
SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an object and its associated attributes. Only objects with no children and no parents can be deleted. The
* maximum number of attributes that can be deleted during an object deletion is 30. For more information, see Amazon Cloud Directory
* Limits.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteObjectRequest.Builder} avoiding the need to
* create one manually via {@link DeleteObjectRequest#builder()}
*
*
* @param deleteObjectRequest
* A {@link Consumer} that will call methods on {@link DeleteObjectRequest.Builder} to create a request.
* @return Result of the DeleteObject operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ObjectNotDetachedException
* Indicates that the requested operation cannot be completed because the object has not been detached from
* the tree.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DeleteObject
* @see AWS
* API Documentation
*/
default DeleteObjectResponse deleteObject(Consumer deleteObjectRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
ObjectNotDetachedException, AwsServiceException, SdkClientException, CloudDirectoryException {
return deleteObject(DeleteObjectRequest.builder().applyMutation(deleteObjectRequest).build());
}
/**
*
* Deletes a given schema. Schemas in a development and published state can only be deleted.
*
*
* @param deleteSchemaRequest
* @return Result of the DeleteSchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws StillContainsLinksException
* The object could not be deleted because links still exist. Remove the links and then try the operation
* again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DeleteSchema
* @see AWS
* API Documentation
*/
default DeleteSchemaResponse deleteSchema(DeleteSchemaRequest deleteSchemaRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, StillContainsLinksException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a given schema. Schemas in a development and published state can only be deleted.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSchemaRequest.Builder} avoiding the need to
* create one manually via {@link DeleteSchemaRequest#builder()}
*
*
* @param deleteSchemaRequest
* A {@link Consumer} that will call methods on {@link DeleteSchemaRequest.Builder} to create a request.
* @return Result of the DeleteSchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws StillContainsLinksException
* The object could not be deleted because links still exist. Remove the links and then try the operation
* again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DeleteSchema
* @see AWS
* API Documentation
*/
default DeleteSchemaResponse deleteSchema(Consumer deleteSchemaRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, StillContainsLinksException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return deleteSchema(DeleteSchemaRequest.builder().applyMutation(deleteSchemaRequest).build());
}
/**
*
* Deletes a TypedLinkFacet. For more information, see Typed Links.
*
*
* @param deleteTypedLinkFacetRequest
* @return Result of the DeleteTypedLinkFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DeleteTypedLinkFacet
* @see AWS API Documentation
*/
default DeleteTypedLinkFacetResponse deleteTypedLinkFacet(DeleteTypedLinkFacetRequest deleteTypedLinkFacetRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, FacetNotFoundException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a TypedLinkFacet. For more information, see Typed Links.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTypedLinkFacetRequest.Builder} avoiding the
* need to create one manually via {@link DeleteTypedLinkFacetRequest#builder()}
*
*
* @param deleteTypedLinkFacetRequest
* A {@link Consumer} that will call methods on {@link DeleteTypedLinkFacetRequest.Builder} to create a
* request.
* @return Result of the DeleteTypedLinkFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DeleteTypedLinkFacet
* @see AWS API Documentation
*/
default DeleteTypedLinkFacetResponse deleteTypedLinkFacet(
Consumer deleteTypedLinkFacetRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, FacetNotFoundException, AwsServiceException, SdkClientException, CloudDirectoryException {
return deleteTypedLinkFacet(DeleteTypedLinkFacetRequest.builder().applyMutation(deleteTypedLinkFacetRequest).build());
}
/**
*
* Detaches the specified object from the specified index.
*
*
* @param detachFromIndexRequest
* @return Result of the DetachFromIndex operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ObjectAlreadyDetachedException
* Indicates that the object is not attached to the index.
* @throws NotIndexException
* Indicates that the requested operation can only operate on index objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DetachFromIndex
* @see AWS API Documentation
*/
default DetachFromIndexResponse detachFromIndex(DetachFromIndexRequest detachFromIndexRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
ObjectAlreadyDetachedException, NotIndexException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Detaches the specified object from the specified index.
*
*
*
* This is a convenience which creates an instance of the {@link DetachFromIndexRequest.Builder} avoiding the need
* to create one manually via {@link DetachFromIndexRequest#builder()}
*
*
* @param detachFromIndexRequest
* A {@link Consumer} that will call methods on {@link DetachFromIndexRequest.Builder} to create a request.
* @return Result of the DetachFromIndex operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ObjectAlreadyDetachedException
* Indicates that the object is not attached to the index.
* @throws NotIndexException
* Indicates that the requested operation can only operate on index objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DetachFromIndex
* @see AWS API Documentation
*/
default DetachFromIndexResponse detachFromIndex(Consumer detachFromIndexRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
ObjectAlreadyDetachedException, NotIndexException, AwsServiceException, SdkClientException, CloudDirectoryException {
return detachFromIndex(DetachFromIndexRequest.builder().applyMutation(detachFromIndexRequest).build());
}
/**
*
* Detaches a given object from the parent object. The object that is to be detached from the parent is specified by
* the link name.
*
*
* @param detachObjectRequest
* @return Result of the DetachObject operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotNodeException
* Occurs when any invalid operations are performed on an object that is not a node, such as calling
* ListObjectChildren
for a leaf node object.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DetachObject
* @see AWS
* API Documentation
*/
default DetachObjectResponse detachObject(DetachObjectRequest detachObjectRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, NotNodeException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Detaches a given object from the parent object. The object that is to be detached from the parent is specified by
* the link name.
*
*
*
* This is a convenience which creates an instance of the {@link DetachObjectRequest.Builder} avoiding the need to
* create one manually via {@link DetachObjectRequest#builder()}
*
*
* @param detachObjectRequest
* A {@link Consumer} that will call methods on {@link DetachObjectRequest.Builder} to create a request.
* @return Result of the DetachObject operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotNodeException
* Occurs when any invalid operations are performed on an object that is not a node, such as calling
* ListObjectChildren
for a leaf node object.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DetachObject
* @see AWS
* API Documentation
*/
default DetachObjectResponse detachObject(Consumer detachObjectRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
NotNodeException, AwsServiceException, SdkClientException, CloudDirectoryException {
return detachObject(DetachObjectRequest.builder().applyMutation(detachObjectRequest).build());
}
/**
*
* Detaches a policy from an object.
*
*
* @param detachPolicyRequest
* @return Result of the DetachPolicy operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotPolicyException
* Indicates that the requested operation can only operate on policy objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DetachPolicy
* @see AWS
* API Documentation
*/
default DetachPolicyResponse detachPolicy(DetachPolicyRequest detachPolicyRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, NotPolicyException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Detaches a policy from an object.
*
*
*
* This is a convenience which creates an instance of the {@link DetachPolicyRequest.Builder} avoiding the need to
* create one manually via {@link DetachPolicyRequest#builder()}
*
*
* @param detachPolicyRequest
* A {@link Consumer} that will call methods on {@link DetachPolicyRequest.Builder} to create a request.
* @return Result of the DetachPolicy operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotPolicyException
* Indicates that the requested operation can only operate on policy objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DetachPolicy
* @see AWS
* API Documentation
*/
default DetachPolicyResponse detachPolicy(Consumer detachPolicyRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
NotPolicyException, AwsServiceException, SdkClientException, CloudDirectoryException {
return detachPolicy(DetachPolicyRequest.builder().applyMutation(detachPolicyRequest).build());
}
/**
*
* Detaches a typed link from a specified source and target object. For more information, see Typed Links.
*
*
* @param detachTypedLinkRequest
* @return Result of the DetachTypedLink operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DetachTypedLink
* @see AWS API Documentation
*/
default DetachTypedLinkResponse detachTypedLink(DetachTypedLinkRequest detachTypedLinkRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Detaches a typed link from a specified source and target object. For more information, see Typed Links.
*
*
*
* This is a convenience which creates an instance of the {@link DetachTypedLinkRequest.Builder} avoiding the need
* to create one manually via {@link DetachTypedLinkRequest#builder()}
*
*
* @param detachTypedLinkRequest
* A {@link Consumer} that will call methods on {@link DetachTypedLinkRequest.Builder} to create a request.
* @return Result of the DetachTypedLink operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DetachTypedLink
* @see AWS API Documentation
*/
default DetachTypedLinkResponse detachTypedLink(Consumer detachTypedLinkRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
return detachTypedLink(DetachTypedLinkRequest.builder().applyMutation(detachTypedLinkRequest).build());
}
/**
*
* Disables the specified directory. Disabled directories cannot be read or written to. Only enabled directories can
* be disabled. Disabled directories may be reenabled.
*
*
* @param disableDirectoryRequest
* @return Result of the DisableDirectory operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws DirectoryDeletedException
* A directory that has been deleted and to which access has been attempted. Note: The requested resource
* will eventually cease to exist.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DisableDirectory
* @see AWS API Documentation
*/
default DisableDirectoryResponse disableDirectory(DisableDirectoryRequest disableDirectoryRequest)
throws ResourceNotFoundException, DirectoryDeletedException, InternalServiceException, ValidationException,
LimitExceededException, AccessDeniedException, RetryableConflictException, InvalidArnException, AwsServiceException,
SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Disables the specified directory. Disabled directories cannot be read or written to. Only enabled directories can
* be disabled. Disabled directories may be reenabled.
*
*
*
* This is a convenience which creates an instance of the {@link DisableDirectoryRequest.Builder} avoiding the need
* to create one manually via {@link DisableDirectoryRequest#builder()}
*
*
* @param disableDirectoryRequest
* A {@link Consumer} that will call methods on {@link DisableDirectoryRequest.Builder} to create a request.
* @return Result of the DisableDirectory operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws DirectoryDeletedException
* A directory that has been deleted and to which access has been attempted. Note: The requested resource
* will eventually cease to exist.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.DisableDirectory
* @see AWS API Documentation
*/
default DisableDirectoryResponse disableDirectory(Consumer disableDirectoryRequest)
throws ResourceNotFoundException, DirectoryDeletedException, InternalServiceException, ValidationException,
LimitExceededException, AccessDeniedException, RetryableConflictException, InvalidArnException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return disableDirectory(DisableDirectoryRequest.builder().applyMutation(disableDirectoryRequest).build());
}
/**
*
* Enables the specified directory. Only disabled directories can be enabled. Once enabled, the directory can then
* be read and written to.
*
*
* @param enableDirectoryRequest
* @return Result of the EnableDirectory operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws DirectoryDeletedException
* A directory that has been deleted and to which access has been attempted. Note: The requested resource
* will eventually cease to exist.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.EnableDirectory
* @see AWS API Documentation
*/
default EnableDirectoryResponse enableDirectory(EnableDirectoryRequest enableDirectoryRequest)
throws ResourceNotFoundException, DirectoryDeletedException, InternalServiceException, ValidationException,
LimitExceededException, AccessDeniedException, RetryableConflictException, InvalidArnException, AwsServiceException,
SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Enables the specified directory. Only disabled directories can be enabled. Once enabled, the directory can then
* be read and written to.
*
*
*
* This is a convenience which creates an instance of the {@link EnableDirectoryRequest.Builder} avoiding the need
* to create one manually via {@link EnableDirectoryRequest#builder()}
*
*
* @param enableDirectoryRequest
* A {@link Consumer} that will call methods on {@link EnableDirectoryRequest.Builder} to create a request.
* @return Result of the EnableDirectory operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws DirectoryDeletedException
* A directory that has been deleted and to which access has been attempted. Note: The requested resource
* will eventually cease to exist.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.EnableDirectory
* @see AWS API Documentation
*/
default EnableDirectoryResponse enableDirectory(Consumer enableDirectoryRequest)
throws ResourceNotFoundException, DirectoryDeletedException, InternalServiceException, ValidationException,
LimitExceededException, AccessDeniedException, RetryableConflictException, InvalidArnException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return enableDirectory(EnableDirectoryRequest.builder().applyMutation(enableDirectoryRequest).build());
}
/**
*
* Returns current applied schema version ARN, including the minor version in use.
*
*
* @param getAppliedSchemaVersionRequest
* @return Result of the GetAppliedSchemaVersion operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetAppliedSchemaVersion
* @see AWS API Documentation
*/
default GetAppliedSchemaVersionResponse getAppliedSchemaVersion(GetAppliedSchemaVersionRequest getAppliedSchemaVersionRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns current applied schema version ARN, including the minor version in use.
*
*
*
* This is a convenience which creates an instance of the {@link GetAppliedSchemaVersionRequest.Builder} avoiding
* the need to create one manually via {@link GetAppliedSchemaVersionRequest#builder()}
*
*
* @param getAppliedSchemaVersionRequest
* A {@link Consumer} that will call methods on {@link GetAppliedSchemaVersionRequest.Builder} to create a
* request.
* @return Result of the GetAppliedSchemaVersion operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetAppliedSchemaVersion
* @see AWS API Documentation
*/
default GetAppliedSchemaVersionResponse getAppliedSchemaVersion(
Consumer getAppliedSchemaVersionRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CloudDirectoryException {
return getAppliedSchemaVersion(GetAppliedSchemaVersionRequest.builder().applyMutation(getAppliedSchemaVersionRequest)
.build());
}
/**
*
* Retrieves metadata about a directory.
*
*
* @param getDirectoryRequest
* @return Result of the GetDirectory operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetDirectory
* @see AWS
* API Documentation
*/
default GetDirectoryResponse getDirectory(GetDirectoryRequest getDirectoryRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves metadata about a directory.
*
*
*
* This is a convenience which creates an instance of the {@link GetDirectoryRequest.Builder} avoiding the need to
* create one manually via {@link GetDirectoryRequest#builder()}
*
*
* @param getDirectoryRequest
* A {@link Consumer} that will call methods on {@link GetDirectoryRequest.Builder} to create a request.
* @return Result of the GetDirectory operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetDirectory
* @see AWS
* API Documentation
*/
default GetDirectoryResponse getDirectory(Consumer getDirectoryRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, AwsServiceException, SdkClientException, CloudDirectoryException {
return getDirectory(GetDirectoryRequest.builder().applyMutation(getDirectoryRequest).build());
}
/**
*
* Gets details of the Facet, such as facet name, attributes, Rules, or ObjectType
. You
* can call this on all kinds of schema facets -- published, development, or applied.
*
*
* @param getFacetRequest
* @return Result of the GetFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetFacet
* @see AWS API
* Documentation
*/
default GetFacetResponse getFacet(GetFacetRequest getFacetRequest) throws InternalServiceException, InvalidArnException,
RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, FacetNotFoundException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Gets details of the Facet, such as facet name, attributes, Rules, or ObjectType
. You
* can call this on all kinds of schema facets -- published, development, or applied.
*
*
*
* This is a convenience which creates an instance of the {@link GetFacetRequest.Builder} avoiding the need to
* create one manually via {@link GetFacetRequest#builder()}
*
*
* @param getFacetRequest
* A {@link Consumer} that will call methods on {@link GetFacetRequest.Builder} to create a request.
* @return Result of the GetFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetFacet
* @see AWS API
* Documentation
*/
default GetFacetResponse getFacet(Consumer getFacetRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, FacetNotFoundException, AwsServiceException, SdkClientException, CloudDirectoryException {
return getFacet(GetFacetRequest.builder().applyMutation(getFacetRequest).build());
}
/**
*
* Retrieves attributes that are associated with a typed link.
*
*
* @param getLinkAttributesRequest
* @return Result of the GetLinkAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetLinkAttributes
* @see AWS API Documentation
*/
default GetLinkAttributesResponse getLinkAttributes(GetLinkAttributesRequest getLinkAttributesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves attributes that are associated with a typed link.
*
*
*
* This is a convenience which creates an instance of the {@link GetLinkAttributesRequest.Builder} avoiding the need
* to create one manually via {@link GetLinkAttributesRequest#builder()}
*
*
* @param getLinkAttributesRequest
* A {@link Consumer} that will call methods on {@link GetLinkAttributesRequest.Builder} to create a request.
* @return Result of the GetLinkAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetLinkAttributes
* @see AWS API Documentation
*/
default GetLinkAttributesResponse getLinkAttributes(Consumer getLinkAttributesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
return getLinkAttributes(GetLinkAttributesRequest.builder().applyMutation(getLinkAttributesRequest).build());
}
/**
*
* Retrieves attributes within a facet that are associated with an object.
*
*
* @param getObjectAttributesRequest
* @return Result of the GetObjectAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetObjectAttributes
* @see AWS API Documentation
*/
default GetObjectAttributesResponse getObjectAttributes(GetObjectAttributesRequest getObjectAttributesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves attributes within a facet that are associated with an object.
*
*
*
* This is a convenience which creates an instance of the {@link GetObjectAttributesRequest.Builder} avoiding the
* need to create one manually via {@link GetObjectAttributesRequest#builder()}
*
*
* @param getObjectAttributesRequest
* A {@link Consumer} that will call methods on {@link GetObjectAttributesRequest.Builder} to create a
* request.
* @return Result of the GetObjectAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetObjectAttributes
* @see AWS API Documentation
*/
default GetObjectAttributesResponse getObjectAttributes(
Consumer getObjectAttributesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, FacetValidationException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return getObjectAttributes(GetObjectAttributesRequest.builder().applyMutation(getObjectAttributesRequest).build());
}
/**
*
* Retrieves metadata about an object.
*
*
* @param getObjectInformationRequest
* @return Result of the GetObjectInformation operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetObjectInformation
* @see AWS API Documentation
*/
default GetObjectInformationResponse getObjectInformation(GetObjectInformationRequest getObjectInformationRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves metadata about an object.
*
*
*
* This is a convenience which creates an instance of the {@link GetObjectInformationRequest.Builder} avoiding the
* need to create one manually via {@link GetObjectInformationRequest#builder()}
*
*
* @param getObjectInformationRequest
* A {@link Consumer} that will call methods on {@link GetObjectInformationRequest.Builder} to create a
* request.
* @return Result of the GetObjectInformation operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetObjectInformation
* @see AWS API Documentation
*/
default GetObjectInformationResponse getObjectInformation(
Consumer getObjectInformationRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return getObjectInformation(GetObjectInformationRequest.builder().applyMutation(getObjectInformationRequest).build());
}
/**
*
* Retrieves a JSON representation of the schema. See JSON
* Schema Format for more information.
*
*
* @param getSchemaAsJsonRequest
* @return Result of the GetSchemaAsJson operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetSchemaAsJson
* @see AWS API Documentation
*/
default GetSchemaAsJsonResponse getSchemaAsJson(GetSchemaAsJsonRequest getSchemaAsJsonRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a JSON representation of the schema. See JSON
* Schema Format for more information.
*
*
*
* This is a convenience which creates an instance of the {@link GetSchemaAsJsonRequest.Builder} avoiding the need
* to create one manually via {@link GetSchemaAsJsonRequest#builder()}
*
*
* @param getSchemaAsJsonRequest
* A {@link Consumer} that will call methods on {@link GetSchemaAsJsonRequest.Builder} to create a request.
* @return Result of the GetSchemaAsJson operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetSchemaAsJson
* @see AWS API Documentation
*/
default GetSchemaAsJsonResponse getSchemaAsJson(Consumer getSchemaAsJsonRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return getSchemaAsJson(GetSchemaAsJsonRequest.builder().applyMutation(getSchemaAsJsonRequest).build());
}
/**
*
* Returns the identity attribute order for a specific TypedLinkFacet. For more information, see Typed Links.
*
*
* @param getTypedLinkFacetInformationRequest
* @return Result of the GetTypedLinkFacetInformation operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetTypedLinkFacetInformation
* @see AWS API Documentation
*/
default GetTypedLinkFacetInformationResponse getTypedLinkFacetInformation(
GetTypedLinkFacetInformationRequest getTypedLinkFacetInformationRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, FacetNotFoundException, AwsServiceException,
SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the identity attribute order for a specific TypedLinkFacet. For more information, see Typed Links.
*
*
*
* This is a convenience which creates an instance of the {@link GetTypedLinkFacetInformationRequest.Builder}
* avoiding the need to create one manually via {@link GetTypedLinkFacetInformationRequest#builder()}
*
*
* @param getTypedLinkFacetInformationRequest
* A {@link Consumer} that will call methods on {@link GetTypedLinkFacetInformationRequest.Builder} to create
* a request.
* @return Result of the GetTypedLinkFacetInformation operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.GetTypedLinkFacetInformation
* @see AWS API Documentation
*/
default GetTypedLinkFacetInformationResponse getTypedLinkFacetInformation(
Consumer getTypedLinkFacetInformationRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, InvalidNextTokenException,
FacetNotFoundException, AwsServiceException, SdkClientException, CloudDirectoryException {
return getTypedLinkFacetInformation(GetTypedLinkFacetInformationRequest.builder()
.applyMutation(getTypedLinkFacetInformationRequest).build());
}
/**
*
* Lists schema major versions applied to a directory. If SchemaArn
is provided, lists the minor
* version.
*
*
* @param listAppliedSchemaArnsRequest
* @return Result of the ListAppliedSchemaArns operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListAppliedSchemaArns
* @see AWS API Documentation
*/
default ListAppliedSchemaArnsResponse listAppliedSchemaArns(ListAppliedSchemaArnsRequest listAppliedSchemaArnsRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, InvalidNextTokenException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists schema major versions applied to a directory. If SchemaArn
is provided, lists the minor
* version.
*
*
*
* This is a convenience which creates an instance of the {@link ListAppliedSchemaArnsRequest.Builder} avoiding the
* need to create one manually via {@link ListAppliedSchemaArnsRequest#builder()}
*
*
* @param listAppliedSchemaArnsRequest
* A {@link Consumer} that will call methods on {@link ListAppliedSchemaArnsRequest.Builder} to create a
* request.
* @return Result of the ListAppliedSchemaArns operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListAppliedSchemaArns
* @see AWS API Documentation
*/
default ListAppliedSchemaArnsResponse listAppliedSchemaArns(
Consumer listAppliedSchemaArnsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listAppliedSchemaArns(ListAppliedSchemaArnsRequest.builder().applyMutation(listAppliedSchemaArnsRequest).build());
}
/**
*
* Lists schema major versions applied to a directory. If SchemaArn
is provided, lists the minor
* version.
*
*
*
* This is a variant of
* {@link #listAppliedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListAppliedSchemaArnsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListAppliedSchemaArnsIterable responses = client.listAppliedSchemaArnsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListAppliedSchemaArnsIterable responses = client
* .listAppliedSchemaArnsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListAppliedSchemaArnsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListAppliedSchemaArnsIterable responses = client.listAppliedSchemaArnsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAppliedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListAppliedSchemaArnsRequest)}
* operation.
*
*
* @param listAppliedSchemaArnsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListAppliedSchemaArns
* @see AWS API Documentation
*/
default ListAppliedSchemaArnsIterable listAppliedSchemaArnsPaginator(ListAppliedSchemaArnsRequest listAppliedSchemaArnsRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, InvalidNextTokenException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists schema major versions applied to a directory. If SchemaArn
is provided, lists the minor
* version.
*
*
*
* This is a variant of
* {@link #listAppliedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListAppliedSchemaArnsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListAppliedSchemaArnsIterable responses = client.listAppliedSchemaArnsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListAppliedSchemaArnsIterable responses = client
* .listAppliedSchemaArnsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListAppliedSchemaArnsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListAppliedSchemaArnsIterable responses = client.listAppliedSchemaArnsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAppliedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListAppliedSchemaArnsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListAppliedSchemaArnsRequest.Builder} avoiding the
* need to create one manually via {@link ListAppliedSchemaArnsRequest#builder()}
*
*
* @param listAppliedSchemaArnsRequest
* A {@link Consumer} that will call methods on {@link ListAppliedSchemaArnsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListAppliedSchemaArns
* @see AWS API Documentation
*/
default ListAppliedSchemaArnsIterable listAppliedSchemaArnsPaginator(
Consumer listAppliedSchemaArnsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listAppliedSchemaArnsPaginator(ListAppliedSchemaArnsRequest.builder().applyMutation(listAppliedSchemaArnsRequest)
.build());
}
/**
*
* Lists indices attached to the specified object.
*
*
* @param listAttachedIndicesRequest
* @return Result of the ListAttachedIndices operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListAttachedIndices
* @see AWS API Documentation
*/
default ListAttachedIndicesResponse listAttachedIndices(ListAttachedIndicesRequest listAttachedIndicesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists indices attached to the specified object.
*
*
*
* This is a convenience which creates an instance of the {@link ListAttachedIndicesRequest.Builder} avoiding the
* need to create one manually via {@link ListAttachedIndicesRequest#builder()}
*
*
* @param listAttachedIndicesRequest
* A {@link Consumer} that will call methods on {@link ListAttachedIndicesRequest.Builder} to create a
* request.
* @return Result of the ListAttachedIndices operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListAttachedIndices
* @see AWS API Documentation
*/
default ListAttachedIndicesResponse listAttachedIndices(
Consumer listAttachedIndicesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listAttachedIndices(ListAttachedIndicesRequest.builder().applyMutation(listAttachedIndicesRequest).build());
}
/**
*
* Lists indices attached to the specified object.
*
*
*
* This is a variant of
* {@link #listAttachedIndices(software.amazon.awssdk.services.clouddirectory.model.ListAttachedIndicesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListAttachedIndicesIterable responses = client.listAttachedIndicesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListAttachedIndicesIterable responses = client
* .listAttachedIndicesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListAttachedIndicesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListAttachedIndicesIterable responses = client.listAttachedIndicesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttachedIndices(software.amazon.awssdk.services.clouddirectory.model.ListAttachedIndicesRequest)}
* operation.
*
*
* @param listAttachedIndicesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListAttachedIndices
* @see AWS API Documentation
*/
default ListAttachedIndicesIterable listAttachedIndicesPaginator(ListAttachedIndicesRequest listAttachedIndicesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists indices attached to the specified object.
*
*
*
* This is a variant of
* {@link #listAttachedIndices(software.amazon.awssdk.services.clouddirectory.model.ListAttachedIndicesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListAttachedIndicesIterable responses = client.listAttachedIndicesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListAttachedIndicesIterable responses = client
* .listAttachedIndicesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListAttachedIndicesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListAttachedIndicesIterable responses = client.listAttachedIndicesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttachedIndices(software.amazon.awssdk.services.clouddirectory.model.ListAttachedIndicesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListAttachedIndicesRequest.Builder} avoiding the
* need to create one manually via {@link ListAttachedIndicesRequest#builder()}
*
*
* @param listAttachedIndicesRequest
* A {@link Consumer} that will call methods on {@link ListAttachedIndicesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListAttachedIndices
* @see AWS API Documentation
*/
default ListAttachedIndicesIterable listAttachedIndicesPaginator(
Consumer listAttachedIndicesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listAttachedIndicesPaginator(ListAttachedIndicesRequest.builder().applyMutation(listAttachedIndicesRequest)
.build());
}
/**
*
* Retrieves each Amazon Resource Name (ARN) of schemas in the development state.
*
*
* @return Result of the ListDevelopmentSchemaArns operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListDevelopmentSchemaArns
* @see #listDevelopmentSchemaArns(ListDevelopmentSchemaArnsRequest)
* @see AWS API Documentation
*/
default ListDevelopmentSchemaArnsResponse listDevelopmentSchemaArns() throws InternalServiceException, InvalidArnException,
RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listDevelopmentSchemaArns(ListDevelopmentSchemaArnsRequest.builder().build());
}
/**
*
* Retrieves each Amazon Resource Name (ARN) of schemas in the development state.
*
*
* @param listDevelopmentSchemaArnsRequest
* @return Result of the ListDevelopmentSchemaArns operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListDevelopmentSchemaArns
* @see AWS API Documentation
*/
default ListDevelopmentSchemaArnsResponse listDevelopmentSchemaArns(
ListDevelopmentSchemaArnsRequest listDevelopmentSchemaArnsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves each Amazon Resource Name (ARN) of schemas in the development state.
*
*
*
* This is a convenience which creates an instance of the {@link ListDevelopmentSchemaArnsRequest.Builder} avoiding
* the need to create one manually via {@link ListDevelopmentSchemaArnsRequest#builder()}
*
*
* @param listDevelopmentSchemaArnsRequest
* A {@link Consumer} that will call methods on {@link ListDevelopmentSchemaArnsRequest.Builder} to create a
* request.
* @return Result of the ListDevelopmentSchemaArns operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListDevelopmentSchemaArns
* @see AWS API Documentation
*/
default ListDevelopmentSchemaArnsResponse listDevelopmentSchemaArns(
Consumer listDevelopmentSchemaArnsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listDevelopmentSchemaArns(ListDevelopmentSchemaArnsRequest.builder()
.applyMutation(listDevelopmentSchemaArnsRequest).build());
}
/**
*
* Retrieves each Amazon Resource Name (ARN) of schemas in the development state.
*
*
*
* This is a variant of
* {@link #listDevelopmentSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListDevelopmentSchemaArnsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDevelopmentSchemaArnsIterable responses = client.listDevelopmentSchemaArnsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDevelopmentSchemaArnsIterable responses = client
* .listDevelopmentSchemaArnsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListDevelopmentSchemaArnsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDevelopmentSchemaArnsIterable responses = client.listDevelopmentSchemaArnsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDevelopmentSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListDevelopmentSchemaArnsRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListDevelopmentSchemaArns
* @see #listDevelopmentSchemaArnsPaginator(ListDevelopmentSchemaArnsRequest)
* @see AWS API Documentation
*/
default ListDevelopmentSchemaArnsIterable listDevelopmentSchemaArnsPaginator() throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listDevelopmentSchemaArnsPaginator(ListDevelopmentSchemaArnsRequest.builder().build());
}
/**
*
* Retrieves each Amazon Resource Name (ARN) of schemas in the development state.
*
*
*
* This is a variant of
* {@link #listDevelopmentSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListDevelopmentSchemaArnsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDevelopmentSchemaArnsIterable responses = client.listDevelopmentSchemaArnsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDevelopmentSchemaArnsIterable responses = client
* .listDevelopmentSchemaArnsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListDevelopmentSchemaArnsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDevelopmentSchemaArnsIterable responses = client.listDevelopmentSchemaArnsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDevelopmentSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListDevelopmentSchemaArnsRequest)}
* operation.
*
*
* @param listDevelopmentSchemaArnsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListDevelopmentSchemaArns
* @see AWS API Documentation
*/
default ListDevelopmentSchemaArnsIterable listDevelopmentSchemaArnsPaginator(
ListDevelopmentSchemaArnsRequest listDevelopmentSchemaArnsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves each Amazon Resource Name (ARN) of schemas in the development state.
*
*
*
* This is a variant of
* {@link #listDevelopmentSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListDevelopmentSchemaArnsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDevelopmentSchemaArnsIterable responses = client.listDevelopmentSchemaArnsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDevelopmentSchemaArnsIterable responses = client
* .listDevelopmentSchemaArnsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListDevelopmentSchemaArnsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDevelopmentSchemaArnsIterable responses = client.listDevelopmentSchemaArnsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDevelopmentSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListDevelopmentSchemaArnsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListDevelopmentSchemaArnsRequest.Builder} avoiding
* the need to create one manually via {@link ListDevelopmentSchemaArnsRequest#builder()}
*
*
* @param listDevelopmentSchemaArnsRequest
* A {@link Consumer} that will call methods on {@link ListDevelopmentSchemaArnsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListDevelopmentSchemaArns
* @see AWS API Documentation
*/
default ListDevelopmentSchemaArnsIterable listDevelopmentSchemaArnsPaginator(
Consumer listDevelopmentSchemaArnsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listDevelopmentSchemaArnsPaginator(ListDevelopmentSchemaArnsRequest.builder()
.applyMutation(listDevelopmentSchemaArnsRequest).build());
}
/**
*
* Lists directories created within an account.
*
*
* @return Result of the ListDirectories operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListDirectories
* @see #listDirectories(ListDirectoriesRequest)
* @see AWS API Documentation
*/
default ListDirectoriesResponse listDirectories() throws InternalServiceException, InvalidArnException,
RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CloudDirectoryException {
return listDirectories(ListDirectoriesRequest.builder().build());
}
/**
*
* Lists directories created within an account.
*
*
* @param listDirectoriesRequest
* @return Result of the ListDirectories operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListDirectories
* @see AWS API Documentation
*/
default ListDirectoriesResponse listDirectories(ListDirectoriesRequest listDirectoriesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists directories created within an account.
*
*
*
* This is a convenience which creates an instance of the {@link ListDirectoriesRequest.Builder} avoiding the need
* to create one manually via {@link ListDirectoriesRequest#builder()}
*
*
* @param listDirectoriesRequest
* A {@link Consumer} that will call methods on {@link ListDirectoriesRequest.Builder} to create a request.
* @return Result of the ListDirectories operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListDirectories
* @see AWS API Documentation
*/
default ListDirectoriesResponse listDirectories(Consumer listDirectoriesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listDirectories(ListDirectoriesRequest.builder().applyMutation(listDirectoriesRequest).build());
}
/**
*
* Lists directories created within an account.
*
*
*
* This is a variant of
* {@link #listDirectories(software.amazon.awssdk.services.clouddirectory.model.ListDirectoriesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDirectoriesIterable responses = client.listDirectoriesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDirectoriesIterable responses = client
* .listDirectoriesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListDirectoriesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDirectoriesIterable responses = client.listDirectoriesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDirectories(software.amazon.awssdk.services.clouddirectory.model.ListDirectoriesRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListDirectories
* @see #listDirectoriesPaginator(ListDirectoriesRequest)
* @see AWS API Documentation
*/
default ListDirectoriesIterable listDirectoriesPaginator() throws InternalServiceException, InvalidArnException,
RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CloudDirectoryException {
return listDirectoriesPaginator(ListDirectoriesRequest.builder().build());
}
/**
*
* Lists directories created within an account.
*
*
*
* This is a variant of
* {@link #listDirectories(software.amazon.awssdk.services.clouddirectory.model.ListDirectoriesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDirectoriesIterable responses = client.listDirectoriesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDirectoriesIterable responses = client
* .listDirectoriesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListDirectoriesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDirectoriesIterable responses = client.listDirectoriesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDirectories(software.amazon.awssdk.services.clouddirectory.model.ListDirectoriesRequest)}
* operation.
*
*
* @param listDirectoriesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListDirectories
* @see AWS API Documentation
*/
default ListDirectoriesIterable listDirectoriesPaginator(ListDirectoriesRequest listDirectoriesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists directories created within an account.
*
*
*
* This is a variant of
* {@link #listDirectories(software.amazon.awssdk.services.clouddirectory.model.ListDirectoriesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDirectoriesIterable responses = client.listDirectoriesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDirectoriesIterable responses = client
* .listDirectoriesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListDirectoriesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListDirectoriesIterable responses = client.listDirectoriesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDirectories(software.amazon.awssdk.services.clouddirectory.model.ListDirectoriesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListDirectoriesRequest.Builder} avoiding the need
* to create one manually via {@link ListDirectoriesRequest#builder()}
*
*
* @param listDirectoriesRequest
* A {@link Consumer} that will call methods on {@link ListDirectoriesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListDirectories
* @see AWS API Documentation
*/
default ListDirectoriesIterable listDirectoriesPaginator(Consumer listDirectoriesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listDirectoriesPaginator(ListDirectoriesRequest.builder().applyMutation(listDirectoriesRequest).build());
}
/**
*
* Retrieves attributes attached to the facet.
*
*
* @param listFacetAttributesRequest
* @return Result of the ListFacetAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListFacetAttributes
* @see AWS API Documentation
*/
default ListFacetAttributesResponse listFacetAttributes(ListFacetAttributesRequest listFacetAttributesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, FacetNotFoundException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves attributes attached to the facet.
*
*
*
* This is a convenience which creates an instance of the {@link ListFacetAttributesRequest.Builder} avoiding the
* need to create one manually via {@link ListFacetAttributesRequest#builder()}
*
*
* @param listFacetAttributesRequest
* A {@link Consumer} that will call methods on {@link ListFacetAttributesRequest.Builder} to create a
* request.
* @return Result of the ListFacetAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListFacetAttributes
* @see AWS API Documentation
*/
default ListFacetAttributesResponse listFacetAttributes(
Consumer listFacetAttributesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, FacetNotFoundException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return listFacetAttributes(ListFacetAttributesRequest.builder().applyMutation(listFacetAttributesRequest).build());
}
/**
*
* Retrieves attributes attached to the facet.
*
*
*
* This is a variant of
* {@link #listFacetAttributes(software.amazon.awssdk.services.clouddirectory.model.ListFacetAttributesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListFacetAttributesIterable responses = client.listFacetAttributesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListFacetAttributesIterable responses = client
* .listFacetAttributesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListFacetAttributesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListFacetAttributesIterable responses = client.listFacetAttributesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listFacetAttributes(software.amazon.awssdk.services.clouddirectory.model.ListFacetAttributesRequest)}
* operation.
*
*
* @param listFacetAttributesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListFacetAttributes
* @see AWS API Documentation
*/
default ListFacetAttributesIterable listFacetAttributesPaginator(ListFacetAttributesRequest listFacetAttributesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, FacetNotFoundException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves attributes attached to the facet.
*
*
*
* This is a variant of
* {@link #listFacetAttributes(software.amazon.awssdk.services.clouddirectory.model.ListFacetAttributesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListFacetAttributesIterable responses = client.listFacetAttributesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListFacetAttributesIterable responses = client
* .listFacetAttributesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListFacetAttributesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListFacetAttributesIterable responses = client.listFacetAttributesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listFacetAttributes(software.amazon.awssdk.services.clouddirectory.model.ListFacetAttributesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListFacetAttributesRequest.Builder} avoiding the
* need to create one manually via {@link ListFacetAttributesRequest#builder()}
*
*
* @param listFacetAttributesRequest
* A {@link Consumer} that will call methods on {@link ListFacetAttributesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListFacetAttributes
* @see AWS API Documentation
*/
default ListFacetAttributesIterable listFacetAttributesPaginator(
Consumer listFacetAttributesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, FacetNotFoundException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return listFacetAttributesPaginator(ListFacetAttributesRequest.builder().applyMutation(listFacetAttributesRequest)
.build());
}
/**
*
* Retrieves the names of facets that exist in a schema.
*
*
* @param listFacetNamesRequest
* @return Result of the ListFacetNames operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListFacetNames
* @see AWS
* API Documentation
*/
default ListFacetNamesResponse listFacetNames(ListFacetNamesRequest listFacetNamesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the names of facets that exist in a schema.
*
*
*
* This is a convenience which creates an instance of the {@link ListFacetNamesRequest.Builder} avoiding the need to
* create one manually via {@link ListFacetNamesRequest#builder()}
*
*
* @param listFacetNamesRequest
* A {@link Consumer} that will call methods on {@link ListFacetNamesRequest.Builder} to create a request.
* @return Result of the ListFacetNames operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListFacetNames
* @see AWS
* API Documentation
*/
default ListFacetNamesResponse listFacetNames(Consumer listFacetNamesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, InvalidNextTokenException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return listFacetNames(ListFacetNamesRequest.builder().applyMutation(listFacetNamesRequest).build());
}
/**
*
* Retrieves the names of facets that exist in a schema.
*
*
*
* This is a variant of
* {@link #listFacetNames(software.amazon.awssdk.services.clouddirectory.model.ListFacetNamesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListFacetNamesIterable responses = client.listFacetNamesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListFacetNamesIterable responses = client
* .listFacetNamesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListFacetNamesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListFacetNamesIterable responses = client.listFacetNamesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listFacetNames(software.amazon.awssdk.services.clouddirectory.model.ListFacetNamesRequest)}
* operation.
*
*
* @param listFacetNamesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListFacetNames
* @see AWS
* API Documentation
*/
default ListFacetNamesIterable listFacetNamesPaginator(ListFacetNamesRequest listFacetNamesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, InvalidNextTokenException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the names of facets that exist in a schema.
*
*
*
* This is a variant of
* {@link #listFacetNames(software.amazon.awssdk.services.clouddirectory.model.ListFacetNamesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListFacetNamesIterable responses = client.listFacetNamesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListFacetNamesIterable responses = client
* .listFacetNamesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListFacetNamesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListFacetNamesIterable responses = client.listFacetNamesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listFacetNames(software.amazon.awssdk.services.clouddirectory.model.ListFacetNamesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListFacetNamesRequest.Builder} avoiding the need to
* create one manually via {@link ListFacetNamesRequest#builder()}
*
*
* @param listFacetNamesRequest
* A {@link Consumer} that will call methods on {@link ListFacetNamesRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListFacetNames
* @see AWS
* API Documentation
*/
default ListFacetNamesIterable listFacetNamesPaginator(Consumer listFacetNamesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, InvalidNextTokenException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return listFacetNamesPaginator(ListFacetNamesRequest.builder().applyMutation(listFacetNamesRequest).build());
}
/**
*
* Returns a paginated list of all the incoming TypedLinkSpecifier information for an object. It also
* supports filtering by typed link facet and identity attributes. For more information, see Typed Links.
*
*
* @param listIncomingTypedLinksRequest
* @return Result of the ListIncomingTypedLinks operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListIncomingTypedLinks
* @see AWS API Documentation
*/
default ListIncomingTypedLinksResponse listIncomingTypedLinks(ListIncomingTypedLinksRequest listIncomingTypedLinksRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a paginated list of all the incoming TypedLinkSpecifier information for an object. It also
* supports filtering by typed link facet and identity attributes. For more information, see Typed Links.
*
*
*
* This is a convenience which creates an instance of the {@link ListIncomingTypedLinksRequest.Builder} avoiding the
* need to create one manually via {@link ListIncomingTypedLinksRequest#builder()}
*
*
* @param listIncomingTypedLinksRequest
* A {@link Consumer} that will call methods on {@link ListIncomingTypedLinksRequest.Builder} to create a
* request.
* @return Result of the ListIncomingTypedLinks operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListIncomingTypedLinks
* @see AWS API Documentation
*/
default ListIncomingTypedLinksResponse listIncomingTypedLinks(
Consumer listIncomingTypedLinksRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, InvalidNextTokenException, FacetValidationException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return listIncomingTypedLinks(ListIncomingTypedLinksRequest.builder().applyMutation(listIncomingTypedLinksRequest)
.build());
}
/**
*
* Lists objects attached to the specified index.
*
*
* @param listIndexRequest
* @return Result of the ListIndex operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotIndexException
* Indicates that the requested operation can only operate on index objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListIndex
* @see AWS API
* Documentation
*/
default ListIndexResponse listIndex(ListIndexRequest listIndexRequest) throws InternalServiceException, InvalidArnException,
RetryableConflictException, FacetValidationException, ValidationException, LimitExceededException,
AccessDeniedException, DirectoryNotEnabledException, InvalidNextTokenException, ResourceNotFoundException,
NotIndexException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists objects attached to the specified index.
*
*
*
* This is a convenience which creates an instance of the {@link ListIndexRequest.Builder} avoiding the need to
* create one manually via {@link ListIndexRequest#builder()}
*
*
* @param listIndexRequest
* A {@link Consumer} that will call methods on {@link ListIndexRequest.Builder} to create a request.
* @return Result of the ListIndex operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotIndexException
* Indicates that the requested operation can only operate on index objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListIndex
* @see AWS API
* Documentation
*/
default ListIndexResponse listIndex(Consumer listIndexRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, FacetValidationException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, InvalidNextTokenException,
ResourceNotFoundException, NotIndexException, AwsServiceException, SdkClientException, CloudDirectoryException {
return listIndex(ListIndexRequest.builder().applyMutation(listIndexRequest).build());
}
/**
*
* Lists objects attached to the specified index.
*
*
*
* This is a variant of {@link #listIndex(software.amazon.awssdk.services.clouddirectory.model.ListIndexRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListIndexIterable responses = client.listIndexPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListIndexIterable responses = client.listIndexPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListIndexResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListIndexIterable responses = client.listIndexPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listIndex(software.amazon.awssdk.services.clouddirectory.model.ListIndexRequest)} operation.
*
*
* @param listIndexRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotIndexException
* Indicates that the requested operation can only operate on index objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListIndex
* @see AWS API
* Documentation
*/
default ListIndexIterable listIndexPaginator(ListIndexRequest listIndexRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, FacetValidationException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, InvalidNextTokenException,
ResourceNotFoundException, NotIndexException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists objects attached to the specified index.
*
*
*
* This is a variant of {@link #listIndex(software.amazon.awssdk.services.clouddirectory.model.ListIndexRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListIndexIterable responses = client.listIndexPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListIndexIterable responses = client.listIndexPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListIndexResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListIndexIterable responses = client.listIndexPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listIndex(software.amazon.awssdk.services.clouddirectory.model.ListIndexRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListIndexRequest.Builder} avoiding the need to
* create one manually via {@link ListIndexRequest#builder()}
*
*
* @param listIndexRequest
* A {@link Consumer} that will call methods on {@link ListIndexRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotIndexException
* Indicates that the requested operation can only operate on index objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListIndex
* @see AWS API
* Documentation
*/
default ListIndexIterable listIndexPaginator(Consumer listIndexRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, FacetValidationException,
ValidationException, LimitExceededException, AccessDeniedException, DirectoryNotEnabledException,
InvalidNextTokenException, ResourceNotFoundException, NotIndexException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listIndexPaginator(ListIndexRequest.builder().applyMutation(listIndexRequest).build());
}
/**
*
* Lists the major version families of each managed schema. If a major version ARN is provided as SchemaArn, the
* minor version revisions in that family are listed instead.
*
*
* @return Result of the ListManagedSchemaArns operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListManagedSchemaArns
* @see #listManagedSchemaArns(ListManagedSchemaArnsRequest)
* @see AWS API Documentation
*/
default ListManagedSchemaArnsResponse listManagedSchemaArns() throws InternalServiceException, InvalidArnException,
ValidationException, AccessDeniedException, ResourceNotFoundException, InvalidNextTokenException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return listManagedSchemaArns(ListManagedSchemaArnsRequest.builder().build());
}
/**
*
* Lists the major version families of each managed schema. If a major version ARN is provided as SchemaArn, the
* minor version revisions in that family are listed instead.
*
*
* @param listManagedSchemaArnsRequest
* @return Result of the ListManagedSchemaArns operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListManagedSchemaArns
* @see AWS API Documentation
*/
default ListManagedSchemaArnsResponse listManagedSchemaArns(ListManagedSchemaArnsRequest listManagedSchemaArnsRequest)
throws InternalServiceException, InvalidArnException, ValidationException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the major version families of each managed schema. If a major version ARN is provided as SchemaArn, the
* minor version revisions in that family are listed instead.
*
*
*
* This is a convenience which creates an instance of the {@link ListManagedSchemaArnsRequest.Builder} avoiding the
* need to create one manually via {@link ListManagedSchemaArnsRequest#builder()}
*
*
* @param listManagedSchemaArnsRequest
* A {@link Consumer} that will call methods on {@link ListManagedSchemaArnsRequest.Builder} to create a
* request.
* @return Result of the ListManagedSchemaArns operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListManagedSchemaArns
* @see AWS API Documentation
*/
default ListManagedSchemaArnsResponse listManagedSchemaArns(
Consumer listManagedSchemaArnsRequest) throws InternalServiceException,
InvalidArnException, ValidationException, AccessDeniedException, ResourceNotFoundException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CloudDirectoryException {
return listManagedSchemaArns(ListManagedSchemaArnsRequest.builder().applyMutation(listManagedSchemaArnsRequest).build());
}
/**
*
* Lists the major version families of each managed schema. If a major version ARN is provided as SchemaArn, the
* minor version revisions in that family are listed instead.
*
*
*
* This is a variant of
* {@link #listManagedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListManagedSchemaArnsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListManagedSchemaArnsIterable responses = client.listManagedSchemaArnsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListManagedSchemaArnsIterable responses = client
* .listManagedSchemaArnsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListManagedSchemaArnsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListManagedSchemaArnsIterable responses = client.listManagedSchemaArnsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listManagedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListManagedSchemaArnsRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListManagedSchemaArns
* @see #listManagedSchemaArnsPaginator(ListManagedSchemaArnsRequest)
* @see AWS API Documentation
*/
default ListManagedSchemaArnsIterable listManagedSchemaArnsPaginator() throws InternalServiceException, InvalidArnException,
ValidationException, AccessDeniedException, ResourceNotFoundException, InvalidNextTokenException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return listManagedSchemaArnsPaginator(ListManagedSchemaArnsRequest.builder().build());
}
/**
*
* Lists the major version families of each managed schema. If a major version ARN is provided as SchemaArn, the
* minor version revisions in that family are listed instead.
*
*
*
* This is a variant of
* {@link #listManagedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListManagedSchemaArnsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListManagedSchemaArnsIterable responses = client.listManagedSchemaArnsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListManagedSchemaArnsIterable responses = client
* .listManagedSchemaArnsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListManagedSchemaArnsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListManagedSchemaArnsIterable responses = client.listManagedSchemaArnsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listManagedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListManagedSchemaArnsRequest)}
* operation.
*
*
* @param listManagedSchemaArnsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListManagedSchemaArns
* @see AWS API Documentation
*/
default ListManagedSchemaArnsIterable listManagedSchemaArnsPaginator(ListManagedSchemaArnsRequest listManagedSchemaArnsRequest)
throws InternalServiceException, InvalidArnException, ValidationException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the major version families of each managed schema. If a major version ARN is provided as SchemaArn, the
* minor version revisions in that family are listed instead.
*
*
*
* This is a variant of
* {@link #listManagedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListManagedSchemaArnsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListManagedSchemaArnsIterable responses = client.listManagedSchemaArnsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListManagedSchemaArnsIterable responses = client
* .listManagedSchemaArnsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListManagedSchemaArnsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListManagedSchemaArnsIterable responses = client.listManagedSchemaArnsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listManagedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListManagedSchemaArnsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListManagedSchemaArnsRequest.Builder} avoiding the
* need to create one manually via {@link ListManagedSchemaArnsRequest#builder()}
*
*
* @param listManagedSchemaArnsRequest
* A {@link Consumer} that will call methods on {@link ListManagedSchemaArnsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListManagedSchemaArns
* @see AWS API Documentation
*/
default ListManagedSchemaArnsIterable listManagedSchemaArnsPaginator(
Consumer listManagedSchemaArnsRequest) throws InternalServiceException,
InvalidArnException, ValidationException, AccessDeniedException, ResourceNotFoundException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CloudDirectoryException {
return listManagedSchemaArnsPaginator(ListManagedSchemaArnsRequest.builder().applyMutation(listManagedSchemaArnsRequest)
.build());
}
/**
*
* Lists all attributes that are associated with an object.
*
*
* @param listObjectAttributesRequest
* @return Result of the ListObjectAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectAttributes
* @see AWS API Documentation
*/
default ListObjectAttributesResponse listObjectAttributes(ListObjectAttributesRequest listObjectAttributesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all attributes that are associated with an object.
*
*
*
* This is a convenience which creates an instance of the {@link ListObjectAttributesRequest.Builder} avoiding the
* need to create one manually via {@link ListObjectAttributesRequest#builder()}
*
*
* @param listObjectAttributesRequest
* A {@link Consumer} that will call methods on {@link ListObjectAttributesRequest.Builder} to create a
* request.
* @return Result of the ListObjectAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectAttributes
* @see AWS API Documentation
*/
default ListObjectAttributesResponse listObjectAttributes(
Consumer listObjectAttributesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, InvalidNextTokenException, FacetValidationException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return listObjectAttributes(ListObjectAttributesRequest.builder().applyMutation(listObjectAttributesRequest).build());
}
/**
*
* Lists all attributes that are associated with an object.
*
*
*
* This is a variant of
* {@link #listObjectAttributes(software.amazon.awssdk.services.clouddirectory.model.ListObjectAttributesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectAttributesIterable responses = client.listObjectAttributesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectAttributesIterable responses = client
* .listObjectAttributesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListObjectAttributesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectAttributesIterable responses = client.listObjectAttributesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listObjectAttributes(software.amazon.awssdk.services.clouddirectory.model.ListObjectAttributesRequest)}
* operation.
*
*
* @param listObjectAttributesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectAttributes
* @see AWS API Documentation
*/
default ListObjectAttributesIterable listObjectAttributesPaginator(ListObjectAttributesRequest listObjectAttributesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all attributes that are associated with an object.
*
*
*
* This is a variant of
* {@link #listObjectAttributes(software.amazon.awssdk.services.clouddirectory.model.ListObjectAttributesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectAttributesIterable responses = client.listObjectAttributesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectAttributesIterable responses = client
* .listObjectAttributesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListObjectAttributesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectAttributesIterable responses = client.listObjectAttributesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listObjectAttributes(software.amazon.awssdk.services.clouddirectory.model.ListObjectAttributesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListObjectAttributesRequest.Builder} avoiding the
* need to create one manually via {@link ListObjectAttributesRequest#builder()}
*
*
* @param listObjectAttributesRequest
* A {@link Consumer} that will call methods on {@link ListObjectAttributesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectAttributes
* @see AWS API Documentation
*/
default ListObjectAttributesIterable listObjectAttributesPaginator(
Consumer listObjectAttributesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, InvalidNextTokenException, FacetValidationException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return listObjectAttributesPaginator(ListObjectAttributesRequest.builder().applyMutation(listObjectAttributesRequest)
.build());
}
/**
*
* Returns a paginated list of child objects that are associated with a given object.
*
*
* @param listObjectChildrenRequest
* @return Result of the ListObjectChildren operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws NotNodeException
* Occurs when any invalid operations are performed on an object that is not a node, such as calling
* ListObjectChildren
for a leaf node object.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectChildren
* @see AWS API Documentation
*/
default ListObjectChildrenResponse listObjectChildren(ListObjectChildrenRequest listObjectChildrenRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, NotNodeException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a paginated list of child objects that are associated with a given object.
*
*
*
* This is a convenience which creates an instance of the {@link ListObjectChildrenRequest.Builder} avoiding the
* need to create one manually via {@link ListObjectChildrenRequest#builder()}
*
*
* @param listObjectChildrenRequest
* A {@link Consumer} that will call methods on {@link ListObjectChildrenRequest.Builder} to create a
* request.
* @return Result of the ListObjectChildren operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws NotNodeException
* Occurs when any invalid operations are performed on an object that is not a node, such as calling
* ListObjectChildren
for a leaf node object.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectChildren
* @see AWS API Documentation
*/
default ListObjectChildrenResponse listObjectChildren(Consumer listObjectChildrenRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, NotNodeException, AwsServiceException, SdkClientException, CloudDirectoryException {
return listObjectChildren(ListObjectChildrenRequest.builder().applyMutation(listObjectChildrenRequest).build());
}
/**
*
* Returns a paginated list of child objects that are associated with a given object.
*
*
*
* This is a variant of
* {@link #listObjectChildren(software.amazon.awssdk.services.clouddirectory.model.ListObjectChildrenRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectChildrenIterable responses = client.listObjectChildrenPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectChildrenIterable responses = client
* .listObjectChildrenPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListObjectChildrenResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectChildrenIterable responses = client.listObjectChildrenPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listObjectChildren(software.amazon.awssdk.services.clouddirectory.model.ListObjectChildrenRequest)}
* operation.
*
*
* @param listObjectChildrenRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws NotNodeException
* Occurs when any invalid operations are performed on an object that is not a node, such as calling
* ListObjectChildren
for a leaf node object.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectChildren
* @see AWS API Documentation
*/
default ListObjectChildrenIterable listObjectChildrenPaginator(ListObjectChildrenRequest listObjectChildrenRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, NotNodeException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a paginated list of child objects that are associated with a given object.
*
*
*
* This is a variant of
* {@link #listObjectChildren(software.amazon.awssdk.services.clouddirectory.model.ListObjectChildrenRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectChildrenIterable responses = client.listObjectChildrenPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectChildrenIterable responses = client
* .listObjectChildrenPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListObjectChildrenResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectChildrenIterable responses = client.listObjectChildrenPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listObjectChildren(software.amazon.awssdk.services.clouddirectory.model.ListObjectChildrenRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListObjectChildrenRequest.Builder} avoiding the
* need to create one manually via {@link ListObjectChildrenRequest#builder()}
*
*
* @param listObjectChildrenRequest
* A {@link Consumer} that will call methods on {@link ListObjectChildrenRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws NotNodeException
* Occurs when any invalid operations are performed on an object that is not a node, such as calling
* ListObjectChildren
for a leaf node object.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectChildren
* @see AWS API Documentation
*/
default ListObjectChildrenIterable listObjectChildrenPaginator(
Consumer listObjectChildrenRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, InvalidNextTokenException, NotNodeException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return listObjectChildrenPaginator(ListObjectChildrenRequest.builder().applyMutation(listObjectChildrenRequest).build());
}
/**
*
* Retrieves all available parent paths for any object type such as node, leaf node, policy node, and index node
* objects. For more information about objects, see Directory Structure.
*
*
* Use this API to evaluate all parents for an object. The call returns all objects from the root of the directory
* up to the requested object. The API returns the number of paths based on user-defined MaxResults
, in
* case there are multiple paths to the parent. The order of the paths and nodes returned is consistent among
* multiple API calls unless the objects are deleted or moved. Paths not leading to the directory root are ignored
* from the target object.
*
*
* @param listObjectParentPathsRequest
* @return Result of the ListObjectParentPaths operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectParentPaths
* @see AWS API Documentation
*/
default ListObjectParentPathsResponse listObjectParentPaths(ListObjectParentPathsRequest listObjectParentPathsRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, InvalidNextTokenException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves all available parent paths for any object type such as node, leaf node, policy node, and index node
* objects. For more information about objects, see Directory Structure.
*
*
* Use this API to evaluate all parents for an object. The call returns all objects from the root of the directory
* up to the requested object. The API returns the number of paths based on user-defined MaxResults
, in
* case there are multiple paths to the parent. The order of the paths and nodes returned is consistent among
* multiple API calls unless the objects are deleted or moved. Paths not leading to the directory root are ignored
* from the target object.
*
*
*
* This is a convenience which creates an instance of the {@link ListObjectParentPathsRequest.Builder} avoiding the
* need to create one manually via {@link ListObjectParentPathsRequest#builder()}
*
*
* @param listObjectParentPathsRequest
* A {@link Consumer} that will call methods on {@link ListObjectParentPathsRequest.Builder} to create a
* request.
* @return Result of the ListObjectParentPaths operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectParentPaths
* @see AWS API Documentation
*/
default ListObjectParentPathsResponse listObjectParentPaths(
Consumer listObjectParentPathsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, InvalidNextTokenException, ResourceNotFoundException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return listObjectParentPaths(ListObjectParentPathsRequest.builder().applyMutation(listObjectParentPathsRequest).build());
}
/**
*
* Retrieves all available parent paths for any object type such as node, leaf node, policy node, and index node
* objects. For more information about objects, see Directory Structure.
*
*
* Use this API to evaluate all parents for an object. The call returns all objects from the root of the directory
* up to the requested object. The API returns the number of paths based on user-defined MaxResults
, in
* case there are multiple paths to the parent. The order of the paths and nodes returned is consistent among
* multiple API calls unless the objects are deleted or moved. Paths not leading to the directory root are ignored
* from the target object.
*
*
*
* This is a variant of
* {@link #listObjectParentPaths(software.amazon.awssdk.services.clouddirectory.model.ListObjectParentPathsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentPathsIterable responses = client.listObjectParentPathsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentPathsIterable responses = client
* .listObjectParentPathsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListObjectParentPathsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentPathsIterable responses = client.listObjectParentPathsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listObjectParentPaths(software.amazon.awssdk.services.clouddirectory.model.ListObjectParentPathsRequest)}
* operation.
*
*
* @param listObjectParentPathsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectParentPaths
* @see AWS API Documentation
*/
default ListObjectParentPathsIterable listObjectParentPathsPaginator(ListObjectParentPathsRequest listObjectParentPathsRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, InvalidNextTokenException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves all available parent paths for any object type such as node, leaf node, policy node, and index node
* objects. For more information about objects, see Directory Structure.
*
*
* Use this API to evaluate all parents for an object. The call returns all objects from the root of the directory
* up to the requested object. The API returns the number of paths based on user-defined MaxResults
, in
* case there are multiple paths to the parent. The order of the paths and nodes returned is consistent among
* multiple API calls unless the objects are deleted or moved. Paths not leading to the directory root are ignored
* from the target object.
*
*
*
* This is a variant of
* {@link #listObjectParentPaths(software.amazon.awssdk.services.clouddirectory.model.ListObjectParentPathsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentPathsIterable responses = client.listObjectParentPathsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentPathsIterable responses = client
* .listObjectParentPathsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListObjectParentPathsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentPathsIterable responses = client.listObjectParentPathsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listObjectParentPaths(software.amazon.awssdk.services.clouddirectory.model.ListObjectParentPathsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListObjectParentPathsRequest.Builder} avoiding the
* need to create one manually via {@link ListObjectParentPathsRequest#builder()}
*
*
* @param listObjectParentPathsRequest
* A {@link Consumer} that will call methods on {@link ListObjectParentPathsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectParentPaths
* @see AWS API Documentation
*/
default ListObjectParentPathsIterable listObjectParentPathsPaginator(
Consumer listObjectParentPathsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, InvalidNextTokenException, ResourceNotFoundException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return listObjectParentPathsPaginator(ListObjectParentPathsRequest.builder().applyMutation(listObjectParentPathsRequest)
.build());
}
/**
*
* Lists parent objects that are associated with a given object in pagination fashion.
*
*
* @param listObjectParentsRequest
* @return Result of the ListObjectParents operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws CannotListParentOfRootException
* Cannot list the parents of a Directory root.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectParents
* @see AWS API Documentation
*/
default ListObjectParentsResponse listObjectParents(ListObjectParentsRequest listObjectParentsRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, CannotListParentOfRootException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists parent objects that are associated with a given object in pagination fashion.
*
*
*
* This is a convenience which creates an instance of the {@link ListObjectParentsRequest.Builder} avoiding the need
* to create one manually via {@link ListObjectParentsRequest#builder()}
*
*
* @param listObjectParentsRequest
* A {@link Consumer} that will call methods on {@link ListObjectParentsRequest.Builder} to create a request.
* @return Result of the ListObjectParents operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws CannotListParentOfRootException
* Cannot list the parents of a Directory root.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectParents
* @see AWS API Documentation
*/
default ListObjectParentsResponse listObjectParents(Consumer listObjectParentsRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, CannotListParentOfRootException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listObjectParents(ListObjectParentsRequest.builder().applyMutation(listObjectParentsRequest).build());
}
/**
*
* Lists parent objects that are associated with a given object in pagination fashion.
*
*
*
* This is a variant of
* {@link #listObjectParents(software.amazon.awssdk.services.clouddirectory.model.ListObjectParentsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentsIterable responses = client.listObjectParentsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentsIterable responses = client
* .listObjectParentsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListObjectParentsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentsIterable responses = client.listObjectParentsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listObjectParents(software.amazon.awssdk.services.clouddirectory.model.ListObjectParentsRequest)}
* operation.
*
*
* @param listObjectParentsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws CannotListParentOfRootException
* Cannot list the parents of a Directory root.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectParents
* @see AWS API Documentation
*/
default ListObjectParentsIterable listObjectParentsPaginator(ListObjectParentsRequest listObjectParentsRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, CannotListParentOfRootException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists parent objects that are associated with a given object in pagination fashion.
*
*
*
* This is a variant of
* {@link #listObjectParents(software.amazon.awssdk.services.clouddirectory.model.ListObjectParentsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentsIterable responses = client.listObjectParentsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentsIterable responses = client
* .listObjectParentsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListObjectParentsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectParentsIterable responses = client.listObjectParentsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listObjectParents(software.amazon.awssdk.services.clouddirectory.model.ListObjectParentsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListObjectParentsRequest.Builder} avoiding the need
* to create one manually via {@link ListObjectParentsRequest#builder()}
*
*
* @param listObjectParentsRequest
* A {@link Consumer} that will call methods on {@link ListObjectParentsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws CannotListParentOfRootException
* Cannot list the parents of a Directory root.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectParents
* @see AWS API Documentation
*/
default ListObjectParentsIterable listObjectParentsPaginator(
Consumer listObjectParentsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, InvalidNextTokenException, CannotListParentOfRootException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return listObjectParentsPaginator(ListObjectParentsRequest.builder().applyMutation(listObjectParentsRequest).build());
}
/**
*
* Returns policies attached to an object in pagination fashion.
*
*
* @param listObjectPoliciesRequest
* @return Result of the ListObjectPolicies operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectPolicies
* @see AWS API Documentation
*/
default ListObjectPoliciesResponse listObjectPolicies(ListObjectPoliciesRequest listObjectPoliciesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns policies attached to an object in pagination fashion.
*
*
*
* This is a convenience which creates an instance of the {@link ListObjectPoliciesRequest.Builder} avoiding the
* need to create one manually via {@link ListObjectPoliciesRequest#builder()}
*
*
* @param listObjectPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListObjectPoliciesRequest.Builder} to create a
* request.
* @return Result of the ListObjectPolicies operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectPolicies
* @see AWS API Documentation
*/
default ListObjectPoliciesResponse listObjectPolicies(Consumer listObjectPoliciesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CloudDirectoryException {
return listObjectPolicies(ListObjectPoliciesRequest.builder().applyMutation(listObjectPoliciesRequest).build());
}
/**
*
* Returns policies attached to an object in pagination fashion.
*
*
*
* This is a variant of
* {@link #listObjectPolicies(software.amazon.awssdk.services.clouddirectory.model.ListObjectPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectPoliciesIterable responses = client.listObjectPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectPoliciesIterable responses = client
* .listObjectPoliciesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListObjectPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectPoliciesIterable responses = client.listObjectPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listObjectPolicies(software.amazon.awssdk.services.clouddirectory.model.ListObjectPoliciesRequest)}
* operation.
*
*
* @param listObjectPoliciesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectPolicies
* @see AWS API Documentation
*/
default ListObjectPoliciesIterable listObjectPoliciesPaginator(ListObjectPoliciesRequest listObjectPoliciesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns policies attached to an object in pagination fashion.
*
*
*
* This is a variant of
* {@link #listObjectPolicies(software.amazon.awssdk.services.clouddirectory.model.ListObjectPoliciesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectPoliciesIterable responses = client.listObjectPoliciesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectPoliciesIterable responses = client
* .listObjectPoliciesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListObjectPoliciesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListObjectPoliciesIterable responses = client.listObjectPoliciesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listObjectPolicies(software.amazon.awssdk.services.clouddirectory.model.ListObjectPoliciesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListObjectPoliciesRequest.Builder} avoiding the
* need to create one manually via {@link ListObjectPoliciesRequest#builder()}
*
*
* @param listObjectPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListObjectPoliciesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListObjectPolicies
* @see AWS API Documentation
*/
default ListObjectPoliciesIterable listObjectPoliciesPaginator(
Consumer listObjectPoliciesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return listObjectPoliciesPaginator(ListObjectPoliciesRequest.builder().applyMutation(listObjectPoliciesRequest).build());
}
/**
*
* Returns a paginated list of all the outgoing TypedLinkSpecifier information for an object. It also
* supports filtering by typed link facet and identity attributes. For more information, see Typed Links.
*
*
* @param listOutgoingTypedLinksRequest
* @return Result of the ListOutgoingTypedLinks operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListOutgoingTypedLinks
* @see AWS API Documentation
*/
default ListOutgoingTypedLinksResponse listOutgoingTypedLinks(ListOutgoingTypedLinksRequest listOutgoingTypedLinksRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
InvalidNextTokenException, FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a paginated list of all the outgoing TypedLinkSpecifier information for an object. It also
* supports filtering by typed link facet and identity attributes. For more information, see Typed Links.
*
*
*
* This is a convenience which creates an instance of the {@link ListOutgoingTypedLinksRequest.Builder} avoiding the
* need to create one manually via {@link ListOutgoingTypedLinksRequest#builder()}
*
*
* @param listOutgoingTypedLinksRequest
* A {@link Consumer} that will call methods on {@link ListOutgoingTypedLinksRequest.Builder} to create a
* request.
* @return Result of the ListOutgoingTypedLinks operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListOutgoingTypedLinks
* @see AWS API Documentation
*/
default ListOutgoingTypedLinksResponse listOutgoingTypedLinks(
Consumer listOutgoingTypedLinksRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, InvalidNextTokenException, FacetValidationException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return listOutgoingTypedLinks(ListOutgoingTypedLinksRequest.builder().applyMutation(listOutgoingTypedLinksRequest)
.build());
}
/**
*
* Returns all of the ObjectIdentifiers
to which a given policy is attached.
*
*
* @param listPolicyAttachmentsRequest
* @return Result of the ListPolicyAttachments operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotPolicyException
* Indicates that the requested operation can only operate on policy objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListPolicyAttachments
* @see AWS API Documentation
*/
default ListPolicyAttachmentsResponse listPolicyAttachments(ListPolicyAttachmentsRequest listPolicyAttachmentsRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, InvalidNextTokenException,
ResourceNotFoundException, NotPolicyException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns all of the ObjectIdentifiers
to which a given policy is attached.
*
*
*
* This is a convenience which creates an instance of the {@link ListPolicyAttachmentsRequest.Builder} avoiding the
* need to create one manually via {@link ListPolicyAttachmentsRequest#builder()}
*
*
* @param listPolicyAttachmentsRequest
* A {@link Consumer} that will call methods on {@link ListPolicyAttachmentsRequest.Builder} to create a
* request.
* @return Result of the ListPolicyAttachments operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotPolicyException
* Indicates that the requested operation can only operate on policy objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListPolicyAttachments
* @see AWS API Documentation
*/
default ListPolicyAttachmentsResponse listPolicyAttachments(
Consumer listPolicyAttachmentsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, InvalidNextTokenException, ResourceNotFoundException, NotPolicyException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return listPolicyAttachments(ListPolicyAttachmentsRequest.builder().applyMutation(listPolicyAttachmentsRequest).build());
}
/**
*
* Returns all of the ObjectIdentifiers
to which a given policy is attached.
*
*
*
* This is a variant of
* {@link #listPolicyAttachments(software.amazon.awssdk.services.clouddirectory.model.ListPolicyAttachmentsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPolicyAttachmentsIterable responses = client.listPolicyAttachmentsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPolicyAttachmentsIterable responses = client
* .listPolicyAttachmentsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListPolicyAttachmentsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPolicyAttachmentsIterable responses = client.listPolicyAttachmentsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicyAttachments(software.amazon.awssdk.services.clouddirectory.model.ListPolicyAttachmentsRequest)}
* operation.
*
*
* @param listPolicyAttachmentsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotPolicyException
* Indicates that the requested operation can only operate on policy objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListPolicyAttachments
* @see AWS API Documentation
*/
default ListPolicyAttachmentsIterable listPolicyAttachmentsPaginator(ListPolicyAttachmentsRequest listPolicyAttachmentsRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, InvalidNextTokenException,
ResourceNotFoundException, NotPolicyException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns all of the ObjectIdentifiers
to which a given policy is attached.
*
*
*
* This is a variant of
* {@link #listPolicyAttachments(software.amazon.awssdk.services.clouddirectory.model.ListPolicyAttachmentsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPolicyAttachmentsIterable responses = client.listPolicyAttachmentsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPolicyAttachmentsIterable responses = client
* .listPolicyAttachmentsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListPolicyAttachmentsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPolicyAttachmentsIterable responses = client.listPolicyAttachmentsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicyAttachments(software.amazon.awssdk.services.clouddirectory.model.ListPolicyAttachmentsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListPolicyAttachmentsRequest.Builder} avoiding the
* need to create one manually via {@link ListPolicyAttachmentsRequest#builder()}
*
*
* @param listPolicyAttachmentsRequest
* A {@link Consumer} that will call methods on {@link ListPolicyAttachmentsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws NotPolicyException
* Indicates that the requested operation can only operate on policy objects.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListPolicyAttachments
* @see AWS API Documentation
*/
default ListPolicyAttachmentsIterable listPolicyAttachmentsPaginator(
Consumer listPolicyAttachmentsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, InvalidNextTokenException, ResourceNotFoundException, NotPolicyException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return listPolicyAttachmentsPaginator(ListPolicyAttachmentsRequest.builder().applyMutation(listPolicyAttachmentsRequest)
.build());
}
/**
*
* Lists the major version families of each published schema. If a major version ARN is provided as
* SchemaArn
, the minor version revisions in that family are listed instead.
*
*
* @return Result of the ListPublishedSchemaArns operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListPublishedSchemaArns
* @see #listPublishedSchemaArns(ListPublishedSchemaArnsRequest)
* @see AWS API Documentation
*/
default ListPublishedSchemaArnsResponse listPublishedSchemaArns() throws InternalServiceException, InvalidArnException,
RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listPublishedSchemaArns(ListPublishedSchemaArnsRequest.builder().build());
}
/**
*
* Lists the major version families of each published schema. If a major version ARN is provided as
* SchemaArn
, the minor version revisions in that family are listed instead.
*
*
* @param listPublishedSchemaArnsRequest
* @return Result of the ListPublishedSchemaArns operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListPublishedSchemaArns
* @see AWS API Documentation
*/
default ListPublishedSchemaArnsResponse listPublishedSchemaArns(ListPublishedSchemaArnsRequest listPublishedSchemaArnsRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, InvalidNextTokenException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the major version families of each published schema. If a major version ARN is provided as
* SchemaArn
, the minor version revisions in that family are listed instead.
*
*
*
* This is a convenience which creates an instance of the {@link ListPublishedSchemaArnsRequest.Builder} avoiding
* the need to create one manually via {@link ListPublishedSchemaArnsRequest#builder()}
*
*
* @param listPublishedSchemaArnsRequest
* A {@link Consumer} that will call methods on {@link ListPublishedSchemaArnsRequest.Builder} to create a
* request.
* @return Result of the ListPublishedSchemaArns operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListPublishedSchemaArns
* @see AWS API Documentation
*/
default ListPublishedSchemaArnsResponse listPublishedSchemaArns(
Consumer listPublishedSchemaArnsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listPublishedSchemaArns(ListPublishedSchemaArnsRequest.builder().applyMutation(listPublishedSchemaArnsRequest)
.build());
}
/**
*
* Lists the major version families of each published schema. If a major version ARN is provided as
* SchemaArn
, the minor version revisions in that family are listed instead.
*
*
*
* This is a variant of
* {@link #listPublishedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListPublishedSchemaArnsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPublishedSchemaArnsIterable responses = client.listPublishedSchemaArnsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPublishedSchemaArnsIterable responses = client
* .listPublishedSchemaArnsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListPublishedSchemaArnsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPublishedSchemaArnsIterable responses = client.listPublishedSchemaArnsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPublishedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListPublishedSchemaArnsRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListPublishedSchemaArns
* @see #listPublishedSchemaArnsPaginator(ListPublishedSchemaArnsRequest)
* @see AWS API Documentation
*/
default ListPublishedSchemaArnsIterable listPublishedSchemaArnsPaginator() throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listPublishedSchemaArnsPaginator(ListPublishedSchemaArnsRequest.builder().build());
}
/**
*
* Lists the major version families of each published schema. If a major version ARN is provided as
* SchemaArn
, the minor version revisions in that family are listed instead.
*
*
*
* This is a variant of
* {@link #listPublishedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListPublishedSchemaArnsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPublishedSchemaArnsIterable responses = client.listPublishedSchemaArnsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPublishedSchemaArnsIterable responses = client
* .listPublishedSchemaArnsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListPublishedSchemaArnsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPublishedSchemaArnsIterable responses = client.listPublishedSchemaArnsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPublishedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListPublishedSchemaArnsRequest)}
* operation.
*
*
* @param listPublishedSchemaArnsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListPublishedSchemaArns
* @see AWS API Documentation
*/
default ListPublishedSchemaArnsIterable listPublishedSchemaArnsPaginator(
ListPublishedSchemaArnsRequest listPublishedSchemaArnsRequest) throws InternalServiceException, InvalidArnException,
RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the major version families of each published schema. If a major version ARN is provided as
* SchemaArn
, the minor version revisions in that family are listed instead.
*
*
*
* This is a variant of
* {@link #listPublishedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListPublishedSchemaArnsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPublishedSchemaArnsIterable responses = client.listPublishedSchemaArnsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPublishedSchemaArnsIterable responses = client
* .listPublishedSchemaArnsPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListPublishedSchemaArnsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListPublishedSchemaArnsIterable responses = client.listPublishedSchemaArnsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPublishedSchemaArns(software.amazon.awssdk.services.clouddirectory.model.ListPublishedSchemaArnsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListPublishedSchemaArnsRequest.Builder} avoiding
* the need to create one manually via {@link ListPublishedSchemaArnsRequest#builder()}
*
*
* @param listPublishedSchemaArnsRequest
* A {@link Consumer} that will call methods on {@link ListPublishedSchemaArnsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListPublishedSchemaArns
* @see AWS API Documentation
*/
default ListPublishedSchemaArnsIterable listPublishedSchemaArnsPaginator(
Consumer listPublishedSchemaArnsRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listPublishedSchemaArnsPaginator(ListPublishedSchemaArnsRequest.builder()
.applyMutation(listPublishedSchemaArnsRequest).build());
}
/**
*
* Returns tags for a resource. Tagging is currently supported only for directories with a limit of 50 tags per
* directory. All 50 tags are returned for a given directory with this API call.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidTaggingRequestException
* Can occur for multiple reasons such as when you tag a resource that doesn’t exist or if you specify a
* higher number of tags for a resource than the allowed limit. Allowed limit is 50 tags per resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, InvalidTaggingRequestException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns tags for a resource. Tagging is currently supported only for directories with a limit of 50 tags per
* directory. All 50 tags are returned for a given directory with this API call.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidTaggingRequestException
* Can occur for multiple reasons such as when you tag a resource that doesn’t exist or if you specify a
* higher number of tags for a resource than the allowed limit. Allowed limit is 50 tags per resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidTaggingRequestException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Returns tags for a resource. Tagging is currently supported only for directories with a limit of 50 tags per
* directory. All 50 tags are returned for a given directory with this API call.
*
*
*
* This is a variant of
* {@link #listTagsForResource(software.amazon.awssdk.services.clouddirectory.model.ListTagsForResourceRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTagsForResourceIterable responses = client.listTagsForResourcePaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTagsForResourceIterable responses = client
* .listTagsForResourcePaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListTagsForResourceResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTagsForResourceIterable responses = client.listTagsForResourcePaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTagsForResource(software.amazon.awssdk.services.clouddirectory.model.ListTagsForResourceRequest)}
* operation.
*
*
* @param listTagsForResourceRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidTaggingRequestException
* Can occur for multiple reasons such as when you tag a resource that doesn’t exist or if you specify a
* higher number of tags for a resource than the allowed limit. Allowed limit is 50 tags per resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceIterable listTagsForResourcePaginator(ListTagsForResourceRequest listTagsForResourceRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, InvalidTaggingRequestException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns tags for a resource. Tagging is currently supported only for directories with a limit of 50 tags per
* directory. All 50 tags are returned for a given directory with this API call.
*
*
*
* This is a variant of
* {@link #listTagsForResource(software.amazon.awssdk.services.clouddirectory.model.ListTagsForResourceRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTagsForResourceIterable responses = client.listTagsForResourcePaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTagsForResourceIterable responses = client
* .listTagsForResourcePaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListTagsForResourceResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTagsForResourceIterable responses = client.listTagsForResourcePaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTagsForResource(software.amazon.awssdk.services.clouddirectory.model.ListTagsForResourceRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidTaggingRequestException
* Can occur for multiple reasons such as when you tag a resource that doesn’t exist or if you specify a
* higher number of tags for a resource than the allowed limit. Allowed limit is 50 tags per resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListTagsForResource
* @see AWS API Documentation
*/
default ListTagsForResourceIterable listTagsForResourcePaginator(
Consumer listTagsForResourceRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidTaggingRequestException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listTagsForResourcePaginator(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest)
.build());
}
/**
*
* Returns a paginated list of all attribute definitions for a particular TypedLinkFacet. For more
* information, see Typed Links.
*
*
* @param listTypedLinkFacetAttributesRequest
* @return Result of the ListTypedLinkFacetAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListTypedLinkFacetAttributes
* @see AWS API Documentation
*/
default ListTypedLinkFacetAttributesResponse listTypedLinkFacetAttributes(
ListTypedLinkFacetAttributesRequest listTypedLinkFacetAttributesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, FacetNotFoundException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a paginated list of all attribute definitions for a particular TypedLinkFacet. For more
* information, see Typed Links.
*
*
*
* This is a convenience which creates an instance of the {@link ListTypedLinkFacetAttributesRequest.Builder}
* avoiding the need to create one manually via {@link ListTypedLinkFacetAttributesRequest#builder()}
*
*
* @param listTypedLinkFacetAttributesRequest
* A {@link Consumer} that will call methods on {@link ListTypedLinkFacetAttributesRequest.Builder} to create
* a request.
* @return Result of the ListTypedLinkFacetAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListTypedLinkFacetAttributes
* @see AWS API Documentation
*/
default ListTypedLinkFacetAttributesResponse listTypedLinkFacetAttributes(
Consumer listTypedLinkFacetAttributesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, FacetNotFoundException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CloudDirectoryException {
return listTypedLinkFacetAttributes(ListTypedLinkFacetAttributesRequest.builder()
.applyMutation(listTypedLinkFacetAttributesRequest).build());
}
/**
*
* Returns a paginated list of all attribute definitions for a particular TypedLinkFacet. For more
* information, see Typed Links.
*
*
*
* This is a variant of
* {@link #listTypedLinkFacetAttributes(software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetAttributesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetAttributesIterable responses = client.listTypedLinkFacetAttributesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetAttributesIterable responses = client
* .listTypedLinkFacetAttributesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetAttributesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetAttributesIterable responses = client.listTypedLinkFacetAttributesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTypedLinkFacetAttributes(software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetAttributesRequest)}
* operation.
*
*
* @param listTypedLinkFacetAttributesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListTypedLinkFacetAttributes
* @see AWS API Documentation
*/
default ListTypedLinkFacetAttributesIterable listTypedLinkFacetAttributesPaginator(
ListTypedLinkFacetAttributesRequest listTypedLinkFacetAttributesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, FacetNotFoundException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a paginated list of all attribute definitions for a particular TypedLinkFacet. For more
* information, see Typed Links.
*
*
*
* This is a variant of
* {@link #listTypedLinkFacetAttributes(software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetAttributesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetAttributesIterable responses = client.listTypedLinkFacetAttributesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetAttributesIterable responses = client
* .listTypedLinkFacetAttributesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetAttributesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetAttributesIterable responses = client.listTypedLinkFacetAttributesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTypedLinkFacetAttributes(software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetAttributesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTypedLinkFacetAttributesRequest.Builder}
* avoiding the need to create one manually via {@link ListTypedLinkFacetAttributesRequest#builder()}
*
*
* @param listTypedLinkFacetAttributesRequest
* A {@link Consumer} that will call methods on {@link ListTypedLinkFacetAttributesRequest.Builder} to create
* a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListTypedLinkFacetAttributes
* @see AWS API Documentation
*/
default ListTypedLinkFacetAttributesIterable listTypedLinkFacetAttributesPaginator(
Consumer listTypedLinkFacetAttributesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, FacetNotFoundException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CloudDirectoryException {
return listTypedLinkFacetAttributesPaginator(ListTypedLinkFacetAttributesRequest.builder()
.applyMutation(listTypedLinkFacetAttributesRequest).build());
}
/**
*
* Returns a paginated list of TypedLink
facet names for a particular schema. For more information, see
* Typed Links.
*
*
* @param listTypedLinkFacetNamesRequest
* @return Result of the ListTypedLinkFacetNames operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListTypedLinkFacetNames
* @see AWS API Documentation
*/
default ListTypedLinkFacetNamesResponse listTypedLinkFacetNames(ListTypedLinkFacetNamesRequest listTypedLinkFacetNamesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, InvalidNextTokenException,
AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a paginated list of TypedLink
facet names for a particular schema. For more information, see
* Typed Links.
*
*
*
* This is a convenience which creates an instance of the {@link ListTypedLinkFacetNamesRequest.Builder} avoiding
* the need to create one manually via {@link ListTypedLinkFacetNamesRequest#builder()}
*
*
* @param listTypedLinkFacetNamesRequest
* A {@link Consumer} that will call methods on {@link ListTypedLinkFacetNamesRequest.Builder} to create a
* request.
* @return Result of the ListTypedLinkFacetNames operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListTypedLinkFacetNames
* @see AWS API Documentation
*/
default ListTypedLinkFacetNamesResponse listTypedLinkFacetNames(
Consumer listTypedLinkFacetNamesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listTypedLinkFacetNames(ListTypedLinkFacetNamesRequest.builder().applyMutation(listTypedLinkFacetNamesRequest)
.build());
}
/**
*
* Returns a paginated list of TypedLink
facet names for a particular schema. For more information, see
* Typed Links.
*
*
*
* This is a variant of
* {@link #listTypedLinkFacetNames(software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetNamesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetNamesIterable responses = client.listTypedLinkFacetNamesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetNamesIterable responses = client
* .listTypedLinkFacetNamesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetNamesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetNamesIterable responses = client.listTypedLinkFacetNamesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTypedLinkFacetNames(software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetNamesRequest)}
* operation.
*
*
* @param listTypedLinkFacetNamesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListTypedLinkFacetNames
* @see AWS API Documentation
*/
default ListTypedLinkFacetNamesIterable listTypedLinkFacetNamesPaginator(
ListTypedLinkFacetNamesRequest listTypedLinkFacetNamesRequest) throws InternalServiceException, InvalidArnException,
RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a paginated list of TypedLink
facet names for a particular schema. For more information, see
* Typed Links.
*
*
*
* This is a variant of
* {@link #listTypedLinkFacetNames(software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetNamesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetNamesIterable responses = client.listTypedLinkFacetNamesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetNamesIterable responses = client
* .listTypedLinkFacetNamesPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetNamesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.ListTypedLinkFacetNamesIterable responses = client.listTypedLinkFacetNamesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTypedLinkFacetNames(software.amazon.awssdk.services.clouddirectory.model.ListTypedLinkFacetNamesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTypedLinkFacetNamesRequest.Builder} avoiding
* the need to create one manually via {@link ListTypedLinkFacetNamesRequest#builder()}
*
*
* @param listTypedLinkFacetNamesRequest
* A {@link Consumer} that will call methods on {@link ListTypedLinkFacetNamesRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.ListTypedLinkFacetNames
* @see AWS API Documentation
*/
default ListTypedLinkFacetNamesIterable listTypedLinkFacetNamesPaginator(
Consumer listTypedLinkFacetNamesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return listTypedLinkFacetNamesPaginator(ListTypedLinkFacetNamesRequest.builder()
.applyMutation(listTypedLinkFacetNamesRequest).build());
}
/**
*
* Lists all policies from the root of the Directory to the object specified. If there are no policies
* present, an empty list is returned. If policies are present, and if some objects don't have the policies
* attached, it returns the ObjectIdentifier
for such objects. If policies are present, it returns
* ObjectIdentifier
, policyId
, and policyType
. Paths that don't lead to the
* root from the target object are ignored. For more information, see Policies.
*
*
* @param lookupPolicyRequest
* @return Result of the LookupPolicy operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.LookupPolicy
* @see AWS
* API Documentation
*/
default LookupPolicyResponse lookupPolicy(LookupPolicyRequest lookupPolicyRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, InvalidNextTokenException, ResourceNotFoundException, AwsServiceException,
SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all policies from the root of the Directory to the object specified. If there are no policies
* present, an empty list is returned. If policies are present, and if some objects don't have the policies
* attached, it returns the ObjectIdentifier
for such objects. If policies are present, it returns
* ObjectIdentifier
, policyId
, and policyType
. Paths that don't lead to the
* root from the target object are ignored. For more information, see Policies.
*
*
*
* This is a convenience which creates an instance of the {@link LookupPolicyRequest.Builder} avoiding the need to
* create one manually via {@link LookupPolicyRequest#builder()}
*
*
* @param lookupPolicyRequest
* A {@link Consumer} that will call methods on {@link LookupPolicyRequest.Builder} to create a request.
* @return Result of the LookupPolicy operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.LookupPolicy
* @see AWS
* API Documentation
*/
default LookupPolicyResponse lookupPolicy(Consumer lookupPolicyRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, InvalidNextTokenException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CloudDirectoryException {
return lookupPolicy(LookupPolicyRequest.builder().applyMutation(lookupPolicyRequest).build());
}
/**
*
* Lists all policies from the root of the Directory to the object specified. If there are no policies
* present, an empty list is returned. If policies are present, and if some objects don't have the policies
* attached, it returns the ObjectIdentifier
for such objects. If policies are present, it returns
* ObjectIdentifier
, policyId
, and policyType
. Paths that don't lead to the
* root from the target object are ignored. For more information, see Policies.
*
*
*
* This is a variant of
* {@link #lookupPolicy(software.amazon.awssdk.services.clouddirectory.model.LookupPolicyRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.LookupPolicyIterable responses = client.lookupPolicyPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.LookupPolicyIterable responses = client
* .lookupPolicyPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.LookupPolicyResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.LookupPolicyIterable responses = client.lookupPolicyPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #lookupPolicy(software.amazon.awssdk.services.clouddirectory.model.LookupPolicyRequest)} operation.
*
*
* @param lookupPolicyRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.LookupPolicy
* @see AWS
* API Documentation
*/
default LookupPolicyIterable lookupPolicyPaginator(LookupPolicyRequest lookupPolicyRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, InvalidNextTokenException, ResourceNotFoundException, AwsServiceException,
SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all policies from the root of the Directory to the object specified. If there are no policies
* present, an empty list is returned. If policies are present, and if some objects don't have the policies
* attached, it returns the ObjectIdentifier
for such objects. If policies are present, it returns
* ObjectIdentifier
, policyId
, and policyType
. Paths that don't lead to the
* root from the target object are ignored. For more information, see Policies.
*
*
*
* This is a variant of
* {@link #lookupPolicy(software.amazon.awssdk.services.clouddirectory.model.LookupPolicyRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.LookupPolicyIterable responses = client.lookupPolicyPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.clouddirectory.paginators.LookupPolicyIterable responses = client
* .lookupPolicyPaginator(request);
* for (software.amazon.awssdk.services.clouddirectory.model.LookupPolicyResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.clouddirectory.paginators.LookupPolicyIterable responses = client.lookupPolicyPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #lookupPolicy(software.amazon.awssdk.services.clouddirectory.model.LookupPolicyRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link LookupPolicyRequest.Builder} avoiding the need to
* create one manually via {@link LookupPolicyRequest#builder()}
*
*
* @param lookupPolicyRequest
* A {@link Consumer} that will call methods on {@link LookupPolicyRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws InvalidNextTokenException
* Indicates that the NextToken
value is not valid.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.LookupPolicy
* @see AWS
* API Documentation
*/
default LookupPolicyIterable lookupPolicyPaginator(Consumer lookupPolicyRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, InvalidNextTokenException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CloudDirectoryException {
return lookupPolicyPaginator(LookupPolicyRequest.builder().applyMutation(lookupPolicyRequest).build());
}
/**
*
* Publishes a development schema with a major version and a recommended minor version.
*
*
* @param publishSchemaRequest
* @return Result of the PublishSchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SchemaAlreadyPublishedException
* Indicates that a schema is already published.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.PublishSchema
* @see AWS
* API Documentation
*/
default PublishSchemaResponse publishSchema(PublishSchemaRequest publishSchemaRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, SchemaAlreadyPublishedException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Publishes a development schema with a major version and a recommended minor version.
*
*
*
* This is a convenience which creates an instance of the {@link PublishSchemaRequest.Builder} avoiding the need to
* create one manually via {@link PublishSchemaRequest#builder()}
*
*
* @param publishSchemaRequest
* A {@link Consumer} that will call methods on {@link PublishSchemaRequest.Builder} to create a request.
* @return Result of the PublishSchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SchemaAlreadyPublishedException
* Indicates that a schema is already published.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.PublishSchema
* @see AWS
* API Documentation
*/
default PublishSchemaResponse publishSchema(Consumer publishSchemaRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, SchemaAlreadyPublishedException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return publishSchema(PublishSchemaRequest.builder().applyMutation(publishSchemaRequest).build());
}
/**
*
* Allows a schema to be updated using JSON upload. Only available for development schemas. See JSON
* Schema Format for more information.
*
*
* @param putSchemaFromJsonRequest
* @return Result of the PutSchemaFromJson operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws InvalidSchemaDocException
* Indicates that the provided SchemaDoc
value is not valid.
* @throws InvalidRuleException
* Occurs when any of the rule parameter keys or values are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.PutSchemaFromJson
* @see AWS API Documentation
*/
default PutSchemaFromJsonResponse putSchemaFromJson(PutSchemaFromJsonRequest putSchemaFromJsonRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, InvalidSchemaDocException, InvalidRuleException, AwsServiceException,
SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Allows a schema to be updated using JSON upload. Only available for development schemas. See JSON
* Schema Format for more information.
*
*
*
* This is a convenience which creates an instance of the {@link PutSchemaFromJsonRequest.Builder} avoiding the need
* to create one manually via {@link PutSchemaFromJsonRequest#builder()}
*
*
* @param putSchemaFromJsonRequest
* A {@link Consumer} that will call methods on {@link PutSchemaFromJsonRequest.Builder} to create a request.
* @return Result of the PutSchemaFromJson operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws InvalidSchemaDocException
* Indicates that the provided SchemaDoc
value is not valid.
* @throws InvalidRuleException
* Occurs when any of the rule parameter keys or values are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.PutSchemaFromJson
* @see AWS API Documentation
*/
default PutSchemaFromJsonResponse putSchemaFromJson(Consumer putSchemaFromJsonRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, InvalidSchemaDocException, InvalidRuleException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return putSchemaFromJson(PutSchemaFromJsonRequest.builder().applyMutation(putSchemaFromJsonRequest).build());
}
/**
*
* Removes the specified facet from the specified object.
*
*
* @param removeFacetFromObjectRequest
* @return Result of the RemoveFacetFromObject operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.RemoveFacetFromObject
* @see AWS API Documentation
*/
default RemoveFacetFromObjectResponse removeFacetFromObject(RemoveFacetFromObjectRequest removeFacetFromObjectRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified facet from the specified object.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveFacetFromObjectRequest.Builder} avoiding the
* need to create one manually via {@link RemoveFacetFromObjectRequest#builder()}
*
*
* @param removeFacetFromObjectRequest
* A {@link Consumer} that will call methods on {@link RemoveFacetFromObjectRequest.Builder} to create a
* request.
* @return Result of the RemoveFacetFromObject operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.RemoveFacetFromObject
* @see AWS API Documentation
*/
default RemoveFacetFromObjectResponse removeFacetFromObject(
Consumer removeFacetFromObjectRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, FacetValidationException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return removeFacetFromObject(RemoveFacetFromObjectRequest.builder().applyMutation(removeFacetFromObjectRequest).build());
}
/**
*
* An API operation for adding tags to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidTaggingRequestException
* Can occur for multiple reasons such as when you tag a resource that doesn’t exist or if you specify a
* higher number of tags for a resource than the allowed limit. Allowed limit is 50 tags per resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.TagResource
* @see AWS
* API Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidTaggingRequestException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* An API operation for adding tags to a resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidTaggingRequestException
* Can occur for multiple reasons such as when you tag a resource that doesn’t exist or if you specify a
* higher number of tags for a resource than the allowed limit. Allowed limit is 50 tags per resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.TagResource
* @see AWS
* API Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, InvalidTaggingRequestException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* An API operation for removing tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidTaggingRequestException
* Can occur for multiple reasons such as when you tag a resource that doesn’t exist or if you specify a
* higher number of tags for a resource than the allowed limit. Allowed limit is 50 tags per resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UntagResource
* @see AWS
* API Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, InvalidTaggingRequestException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* An API operation for removing tags from a resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidTaggingRequestException
* Can occur for multiple reasons such as when you tag a resource that doesn’t exist or if you specify a
* higher number of tags for a resource than the allowed limit. Allowed limit is 50 tags per resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UntagResource
* @see AWS
* API Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, InvalidTaggingRequestException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Does the following:
*
*
* -
*
* Adds new Attributes
, Rules
, or ObjectTypes
.
*
*
* -
*
* Updates existing Attributes
, Rules
, or ObjectTypes
.
*
*
* -
*
* Deletes existing Attributes
, Rules
, or ObjectTypes
.
*
*
*
*
* @param updateFacetRequest
* @return Result of the UpdateFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws InvalidFacetUpdateException
* An attempt to modify a Facet resulted in an invalid schema exception.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws InvalidRuleException
* Occurs when any of the rule parameter keys or values are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpdateFacet
* @see AWS
* API Documentation
*/
default UpdateFacetResponse updateFacet(UpdateFacetRequest updateFacetRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
InvalidFacetUpdateException, FacetValidationException, ResourceNotFoundException, FacetNotFoundException,
InvalidRuleException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Does the following:
*
*
* -
*
* Adds new Attributes
, Rules
, or ObjectTypes
.
*
*
* -
*
* Updates existing Attributes
, Rules
, or ObjectTypes
.
*
*
* -
*
* Deletes existing Attributes
, Rules
, or ObjectTypes
.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link UpdateFacetRequest.Builder} avoiding the need to
* create one manually via {@link UpdateFacetRequest#builder()}
*
*
* @param updateFacetRequest
* A {@link Consumer} that will call methods on {@link UpdateFacetRequest.Builder} to create a request.
* @return Result of the UpdateFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws InvalidFacetUpdateException
* An attempt to modify a Facet resulted in an invalid schema exception.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws InvalidRuleException
* Occurs when any of the rule parameter keys or values are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpdateFacet
* @see AWS
* API Documentation
*/
default UpdateFacetResponse updateFacet(Consumer updateFacetRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, InvalidFacetUpdateException, FacetValidationException,
ResourceNotFoundException, FacetNotFoundException, InvalidRuleException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return updateFacet(UpdateFacetRequest.builder().applyMutation(updateFacetRequest).build());
}
/**
*
* Updates a given typed link’s attributes. Attributes to be updated must not contribute to the typed link’s
* identity, as defined by its IdentityAttributeOrder
.
*
*
* @param updateLinkAttributesRequest
* @return Result of the UpdateLinkAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpdateLinkAttributes
* @see AWS API Documentation
*/
default UpdateLinkAttributesResponse updateLinkAttributes(UpdateLinkAttributesRequest updateLinkAttributesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
FacetValidationException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a given typed link’s attributes. Attributes to be updated must not contribute to the typed link’s
* identity, as defined by its IdentityAttributeOrder
.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateLinkAttributesRequest.Builder} avoiding the
* need to create one manually via {@link UpdateLinkAttributesRequest#builder()}
*
*
* @param updateLinkAttributesRequest
* A {@link Consumer} that will call methods on {@link UpdateLinkAttributesRequest.Builder} to create a
* request.
* @return Result of the UpdateLinkAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpdateLinkAttributes
* @see AWS API Documentation
*/
default UpdateLinkAttributesResponse updateLinkAttributes(
Consumer updateLinkAttributesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, FacetValidationException, AwsServiceException,
SdkClientException, CloudDirectoryException {
return updateLinkAttributes(UpdateLinkAttributesRequest.builder().applyMutation(updateLinkAttributesRequest).build());
}
/**
*
* Updates a given object's attributes.
*
*
* @param updateObjectAttributesRequest
* @return Result of the UpdateObjectAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws LinkNameAlreadyInUseException
* Indicates that a link could not be created due to a naming conflict. Choose a different name and then try
* again.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpdateObjectAttributes
* @see AWS API Documentation
*/
default UpdateObjectAttributesResponse updateObjectAttributes(UpdateObjectAttributesRequest updateObjectAttributesRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, DirectoryNotEnabledException, ResourceNotFoundException,
LinkNameAlreadyInUseException, FacetValidationException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a given object's attributes.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateObjectAttributesRequest.Builder} avoiding the
* need to create one manually via {@link UpdateObjectAttributesRequest#builder()}
*
*
* @param updateObjectAttributesRequest
* A {@link Consumer} that will call methods on {@link UpdateObjectAttributesRequest.Builder} to create a
* request.
* @return Result of the UpdateObjectAttributes operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws DirectoryNotEnabledException
* Operations are only permitted on enabled directories.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws LinkNameAlreadyInUseException
* Indicates that a link could not be created due to a naming conflict. Choose a different name and then try
* again.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpdateObjectAttributes
* @see AWS API Documentation
*/
default UpdateObjectAttributesResponse updateObjectAttributes(
Consumer updateObjectAttributesRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
DirectoryNotEnabledException, ResourceNotFoundException, LinkNameAlreadyInUseException, FacetValidationException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return updateObjectAttributes(UpdateObjectAttributesRequest.builder().applyMutation(updateObjectAttributesRequest)
.build());
}
/**
*
* Updates the schema name with a new name. Only development schema names can be updated.
*
*
* @param updateSchemaRequest
* @return Result of the UpdateSchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpdateSchema
* @see AWS
* API Documentation
*/
default UpdateSchemaResponse updateSchema(UpdateSchemaRequest updateSchemaRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
ResourceNotFoundException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the schema name with a new name. Only development schema names can be updated.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSchemaRequest.Builder} avoiding the need to
* create one manually via {@link UpdateSchemaRequest#builder()}
*
*
* @param updateSchemaRequest
* A {@link Consumer} that will call methods on {@link UpdateSchemaRequest.Builder} to create a request.
* @return Result of the UpdateSchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpdateSchema
* @see AWS
* API Documentation
*/
default UpdateSchemaResponse updateSchema(Consumer updateSchemaRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, ResourceNotFoundException, AwsServiceException, SdkClientException,
CloudDirectoryException {
return updateSchema(UpdateSchemaRequest.builder().applyMutation(updateSchemaRequest).build());
}
/**
*
* Updates a TypedLinkFacet. For more information, see Typed Links.
*
*
* @param updateTypedLinkFacetRequest
* @return Result of the UpdateTypedLinkFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws InvalidFacetUpdateException
* An attempt to modify a Facet resulted in an invalid schema exception.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws InvalidRuleException
* Occurs when any of the rule parameter keys or values are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpdateTypedLinkFacet
* @see AWS API Documentation
*/
default UpdateTypedLinkFacetResponse updateTypedLinkFacet(UpdateTypedLinkFacetRequest updateTypedLinkFacetRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
LimitExceededException, AccessDeniedException, FacetValidationException, InvalidFacetUpdateException,
ResourceNotFoundException, FacetNotFoundException, InvalidRuleException, AwsServiceException, SdkClientException,
CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a TypedLinkFacet. For more information, see Typed Links.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateTypedLinkFacetRequest.Builder} avoiding the
* need to create one manually via {@link UpdateTypedLinkFacetRequest#builder()}
*
*
* @param updateTypedLinkFacetRequest
* A {@link Consumer} that will call methods on {@link UpdateTypedLinkFacetRequest.Builder} to create a
* request.
* @return Result of the UpdateTypedLinkFacet operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws FacetValidationException
* The Facet that you provided was not well formed or could not be validated with the schema.
* @throws InvalidFacetUpdateException
* An attempt to modify a Facet resulted in an invalid schema exception.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws FacetNotFoundException
* The specified Facet could not be found.
* @throws InvalidRuleException
* Occurs when any of the rule parameter keys or values are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpdateTypedLinkFacet
* @see AWS API Documentation
*/
default UpdateTypedLinkFacetResponse updateTypedLinkFacet(
Consumer updateTypedLinkFacetRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, LimitExceededException, AccessDeniedException,
FacetValidationException, InvalidFacetUpdateException, ResourceNotFoundException, FacetNotFoundException,
InvalidRuleException, AwsServiceException, SdkClientException, CloudDirectoryException {
return updateTypedLinkFacet(UpdateTypedLinkFacetRequest.builder().applyMutation(updateTypedLinkFacetRequest).build());
}
/**
*
* Upgrades a single directory in-place using the PublishedSchemaArn
with schema updates found in
* MinorVersion
. Backwards-compatible minor version upgrades are instantaneously available for readers
* on all objects in the directory. Note: This is a synchronous API call and upgrades only one schema on a given
* directory per call. To upgrade multiple directories from one schema, you would need to call this API on each
* directory.
*
*
* @param upgradeAppliedSchemaRequest
* @return Result of the UpgradeAppliedSchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws IncompatibleSchemaException
* Indicates a failure occurred while performing a check for backward compatibility between the specified
* schema and the schema that is currently applied to the directory.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidAttachmentException
* Indicates that an attempt to make an attachment was invalid. For example, attaching two nodes with a link
* type that is not applicable to the nodes or attempting to apply a schema to a directory a second time.
* @throws SchemaAlreadyExistsException
* Indicates that a schema could not be created due to a naming conflict. Please select a different name and
* then try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpgradeAppliedSchema
* @see AWS API Documentation
*/
default UpgradeAppliedSchemaResponse upgradeAppliedSchema(UpgradeAppliedSchemaRequest upgradeAppliedSchemaRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
IncompatibleSchemaException, AccessDeniedException, ResourceNotFoundException, InvalidAttachmentException,
SchemaAlreadyExistsException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Upgrades a single directory in-place using the PublishedSchemaArn
with schema updates found in
* MinorVersion
. Backwards-compatible minor version upgrades are instantaneously available for readers
* on all objects in the directory. Note: This is a synchronous API call and upgrades only one schema on a given
* directory per call. To upgrade multiple directories from one schema, you would need to call this API on each
* directory.
*
*
*
* This is a convenience which creates an instance of the {@link UpgradeAppliedSchemaRequest.Builder} avoiding the
* need to create one manually via {@link UpgradeAppliedSchemaRequest#builder()}
*
*
* @param upgradeAppliedSchemaRequest
* A {@link Consumer} that will call methods on {@link UpgradeAppliedSchemaRequest.Builder} to create a
* request.
* @return Result of the UpgradeAppliedSchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws IncompatibleSchemaException
* Indicates a failure occurred while performing a check for backward compatibility between the specified
* schema and the schema that is currently applied to the directory.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidAttachmentException
* Indicates that an attempt to make an attachment was invalid. For example, attaching two nodes with a link
* type that is not applicable to the nodes or attempting to apply a schema to a directory a second time.
* @throws SchemaAlreadyExistsException
* Indicates that a schema could not be created due to a naming conflict. Please select a different name and
* then try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpgradeAppliedSchema
* @see AWS API Documentation
*/
default UpgradeAppliedSchemaResponse upgradeAppliedSchema(
Consumer upgradeAppliedSchemaRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, IncompatibleSchemaException,
AccessDeniedException, ResourceNotFoundException, InvalidAttachmentException, SchemaAlreadyExistsException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return upgradeAppliedSchema(UpgradeAppliedSchemaRequest.builder().applyMutation(upgradeAppliedSchemaRequest).build());
}
/**
*
* Upgrades a published schema under a new minor version revision using the current contents of
* DevelopmentSchemaArn
.
*
*
* @param upgradePublishedSchemaRequest
* @return Result of the UpgradePublishedSchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws IncompatibleSchemaException
* Indicates a failure occurred while performing a check for backward compatibility between the specified
* schema and the schema that is currently applied to the directory.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidAttachmentException
* Indicates that an attempt to make an attachment was invalid. For example, attaching two nodes with a link
* type that is not applicable to the nodes or attempting to apply a schema to a directory a second time.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpgradePublishedSchema
* @see AWS API Documentation
*/
default UpgradePublishedSchemaResponse upgradePublishedSchema(UpgradePublishedSchemaRequest upgradePublishedSchemaRequest)
throws InternalServiceException, InvalidArnException, RetryableConflictException, ValidationException,
IncompatibleSchemaException, AccessDeniedException, ResourceNotFoundException, InvalidAttachmentException,
LimitExceededException, AwsServiceException, SdkClientException, CloudDirectoryException {
throw new UnsupportedOperationException();
}
/**
*
* Upgrades a published schema under a new minor version revision using the current contents of
* DevelopmentSchemaArn
.
*
*
*
* This is a convenience which creates an instance of the {@link UpgradePublishedSchemaRequest.Builder} avoiding the
* need to create one manually via {@link UpgradePublishedSchemaRequest#builder()}
*
*
* @param upgradePublishedSchemaRequest
* A {@link Consumer} that will call methods on {@link UpgradePublishedSchemaRequest.Builder} to create a
* request.
* @return Result of the UpgradePublishedSchema operation returned by the service.
* @throws InternalServiceException
* Indicates a problem that must be resolved by Amazon Web Services. This might be a transient error in
* which case you can retry your request until it succeeds. Otherwise, go to the AWS Service Health Dashboard site to see if there are any
* operational issues with the service.
* @throws InvalidArnException
* Indicates that the provided ARN value is not valid.
* @throws RetryableConflictException
* Occurs when a conflict with a previous successful write is detected. For example, if a write operation
* occurs on an object and then an attempt is made to read the object using “SERIALIZABLE” consistency, this
* exception may result. This generally occurs when the previous write did not have time to propagate to the
* host serving the current request. A retry (with appropriate backoff logic) is the recommended response to
* this exception.
* @throws ValidationException
* Indicates that your request is malformed in some manner. See the exception message.
* @throws IncompatibleSchemaException
* Indicates a failure occurred while performing a check for backward compatibility between the specified
* schema and the schema that is currently applied to the directory.
* @throws AccessDeniedException
* Access denied or directory not found. Either you don't have permissions for this directory or the
* directory does not exist. Try calling ListDirectories and check your permissions.
* @throws ResourceNotFoundException
* The specified resource could not be found.
* @throws InvalidAttachmentException
* Indicates that an attempt to make an attachment was invalid. For example, attaching two nodes with a link
* type that is not applicable to the nodes or attempting to apply a schema to a directory a second time.
* @throws LimitExceededException
* Indicates that limits are exceeded. See Limits for more
* information.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudDirectoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudDirectoryClient.UpgradePublishedSchema
* @see AWS API Documentation
*/
default UpgradePublishedSchemaResponse upgradePublishedSchema(
Consumer upgradePublishedSchemaRequest) throws InternalServiceException,
InvalidArnException, RetryableConflictException, ValidationException, IncompatibleSchemaException,
AccessDeniedException, ResourceNotFoundException, InvalidAttachmentException, LimitExceededException,
AwsServiceException, SdkClientException, CloudDirectoryException {
return upgradePublishedSchema(UpgradePublishedSchemaRequest.builder().applyMutation(upgradePublishedSchemaRequest)
.build());
}
/**
* Create a {@link CloudDirectoryClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static CloudDirectoryClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link CloudDirectoryClient}.
*/
static CloudDirectoryClientBuilder builder() {
return new DefaultCloudDirectoryClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default CloudDirectoryServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}