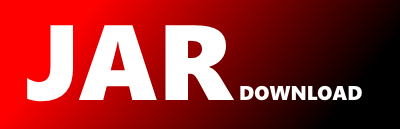
software.amazon.awssdk.services.cloudformation.model.ChangeSetSummary Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.time.Instant;
import java.util.Optional;
import javax.annotation.Generated;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The ChangeSetSummary
structure describes a change set, its status, and the stack with which it's
* associated.
*
*/
@Generated("software.amazon.awssdk:codegen")
public class ChangeSetSummary implements ToCopyableBuilder {
private final String stackId;
private final String stackName;
private final String changeSetId;
private final String changeSetName;
private final String executionStatus;
private final String status;
private final String statusReason;
private final Instant creationTime;
private final String description;
private ChangeSetSummary(BuilderImpl builder) {
this.stackId = builder.stackId;
this.stackName = builder.stackName;
this.changeSetId = builder.changeSetId;
this.changeSetName = builder.changeSetName;
this.executionStatus = builder.executionStatus;
this.status = builder.status;
this.statusReason = builder.statusReason;
this.creationTime = builder.creationTime;
this.description = builder.description;
}
/**
*
* The ID of the stack with which the change set is associated.
*
*
* @return The ID of the stack with which the change set is associated.
*/
public String stackId() {
return stackId;
}
/**
*
* The name of the stack with which the change set is associated.
*
*
* @return The name of the stack with which the change set is associated.
*/
public String stackName() {
return stackName;
}
/**
*
* The ID of the change set.
*
*
* @return The ID of the change set.
*/
public String changeSetId() {
return changeSetId;
}
/**
*
* The name of the change set.
*
*
* @return The name of the change set.
*/
public String changeSetName() {
return changeSetName;
}
/**
*
* If the change set execution status is AVAILABLE
, you can execute the change set. If you can’t
* execute the change set, the status indicates why. For example, a change set might be in an
* UNAVAILABLE
state because AWS CloudFormation is still creating it or in an OBSOLETE
* state because the stack was already updated.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #executionStatus}
* will return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #executionStatusString}.
*
*
* @return If the change set execution status is AVAILABLE
, you can execute the change set. If you
* can’t execute the change set, the status indicates why. For example, a change set might be in an
* UNAVAILABLE
state because AWS CloudFormation is still creating it or in an
* OBSOLETE
state because the stack was already updated.
* @see ExecutionStatus
*/
public ExecutionStatus executionStatus() {
return ExecutionStatus.fromValue(executionStatus);
}
/**
*
* If the change set execution status is AVAILABLE
, you can execute the change set. If you can’t
* execute the change set, the status indicates why. For example, a change set might be in an
* UNAVAILABLE
state because AWS CloudFormation is still creating it or in an OBSOLETE
* state because the stack was already updated.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #executionStatus}
* will return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #executionStatusString}.
*
*
* @return If the change set execution status is AVAILABLE
, you can execute the change set. If you
* can’t execute the change set, the status indicates why. For example, a change set might be in an
* UNAVAILABLE
state because AWS CloudFormation is still creating it or in an
* OBSOLETE
state because the stack was already updated.
* @see ExecutionStatus
*/
public String executionStatusString() {
return executionStatus;
}
/**
*
* The state of the change set, such as CREATE_IN_PROGRESS
, CREATE_COMPLETE
, or
* FAILED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ChangeSetStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusString}.
*
*
* @return The state of the change set, such as CREATE_IN_PROGRESS
, CREATE_COMPLETE
, or
* FAILED
.
* @see ChangeSetStatus
*/
public ChangeSetStatus status() {
return ChangeSetStatus.fromValue(status);
}
/**
*
* The state of the change set, such as CREATE_IN_PROGRESS
, CREATE_COMPLETE
, or
* FAILED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ChangeSetStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusString}.
*
*
* @return The state of the change set, such as CREATE_IN_PROGRESS
, CREATE_COMPLETE
, or
* FAILED
.
* @see ChangeSetStatus
*/
public String statusString() {
return status;
}
/**
*
* A description of the change set's status. For example, if your change set is in the FAILED
state,
* AWS CloudFormation shows the error message.
*
*
* @return A description of the change set's status. For example, if your change set is in the FAILED
* state, AWS CloudFormation shows the error message.
*/
public String statusReason() {
return statusReason;
}
/**
*
* The start time when the change set was created, in UTC.
*
*
* @return The start time when the change set was created, in UTC.
*/
public Instant creationTime() {
return creationTime;
}
/**
*
* Descriptive information about the change set.
*
*
* @return Descriptive information about the change set.
*/
public String description() {
return description;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((stackId() == null) ? 0 : stackId().hashCode());
hashCode = 31 * hashCode + ((stackName() == null) ? 0 : stackName().hashCode());
hashCode = 31 * hashCode + ((changeSetId() == null) ? 0 : changeSetId().hashCode());
hashCode = 31 * hashCode + ((changeSetName() == null) ? 0 : changeSetName().hashCode());
hashCode = 31 * hashCode + ((executionStatusString() == null) ? 0 : executionStatusString().hashCode());
hashCode = 31 * hashCode + ((statusString() == null) ? 0 : statusString().hashCode());
hashCode = 31 * hashCode + ((statusReason() == null) ? 0 : statusReason().hashCode());
hashCode = 31 * hashCode + ((creationTime() == null) ? 0 : creationTime().hashCode());
hashCode = 31 * hashCode + ((description() == null) ? 0 : description().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ChangeSetSummary)) {
return false;
}
ChangeSetSummary other = (ChangeSetSummary) obj;
if (other.stackId() == null ^ this.stackId() == null) {
return false;
}
if (other.stackId() != null && !other.stackId().equals(this.stackId())) {
return false;
}
if (other.stackName() == null ^ this.stackName() == null) {
return false;
}
if (other.stackName() != null && !other.stackName().equals(this.stackName())) {
return false;
}
if (other.changeSetId() == null ^ this.changeSetId() == null) {
return false;
}
if (other.changeSetId() != null && !other.changeSetId().equals(this.changeSetId())) {
return false;
}
if (other.changeSetName() == null ^ this.changeSetName() == null) {
return false;
}
if (other.changeSetName() != null && !other.changeSetName().equals(this.changeSetName())) {
return false;
}
if (other.executionStatusString() == null ^ this.executionStatusString() == null) {
return false;
}
if (other.executionStatusString() != null && !other.executionStatusString().equals(this.executionStatusString())) {
return false;
}
if (other.statusString() == null ^ this.statusString() == null) {
return false;
}
if (other.statusString() != null && !other.statusString().equals(this.statusString())) {
return false;
}
if (other.statusReason() == null ^ this.statusReason() == null) {
return false;
}
if (other.statusReason() != null && !other.statusReason().equals(this.statusReason())) {
return false;
}
if (other.creationTime() == null ^ this.creationTime() == null) {
return false;
}
if (other.creationTime() != null && !other.creationTime().equals(this.creationTime())) {
return false;
}
if (other.description() == null ^ this.description() == null) {
return false;
}
if (other.description() != null && !other.description().equals(this.description())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder("{");
if (stackId() != null) {
sb.append("StackId: ").append(stackId()).append(",");
}
if (stackName() != null) {
sb.append("StackName: ").append(stackName()).append(",");
}
if (changeSetId() != null) {
sb.append("ChangeSetId: ").append(changeSetId()).append(",");
}
if (changeSetName() != null) {
sb.append("ChangeSetName: ").append(changeSetName()).append(",");
}
if (executionStatusString() != null) {
sb.append("ExecutionStatus: ").append(executionStatusString()).append(",");
}
if (statusString() != null) {
sb.append("Status: ").append(statusString()).append(",");
}
if (statusReason() != null) {
sb.append("StatusReason: ").append(statusReason()).append(",");
}
if (creationTime() != null) {
sb.append("CreationTime: ").append(creationTime()).append(",");
}
if (description() != null) {
sb.append("Description: ").append(description()).append(",");
}
if (sb.length() > 1) {
sb.setLength(sb.length() - 1);
}
sb.append("}");
return sb.toString();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackId":
return Optional.of(clazz.cast(stackId()));
case "StackName":
return Optional.of(clazz.cast(stackName()));
case "ChangeSetId":
return Optional.of(clazz.cast(changeSetId()));
case "ChangeSetName":
return Optional.of(clazz.cast(changeSetName()));
case "ExecutionStatus":
return Optional.of(clazz.cast(executionStatusString()));
case "Status":
return Optional.of(clazz.cast(statusString()));
case "StatusReason":
return Optional.of(clazz.cast(statusReason()));
case "CreationTime":
return Optional.of(clazz.cast(creationTime()));
case "Description":
return Optional.of(clazz.cast(description()));
default:
return Optional.empty();
}
}
public interface Builder extends CopyableBuilder {
/**
*
* The ID of the stack with which the change set is associated.
*
*
* @param stackId
* The ID of the stack with which the change set is associated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stackId(String stackId);
/**
*
* The name of the stack with which the change set is associated.
*
*
* @param stackName
* The name of the stack with which the change set is associated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stackName(String stackName);
/**
*
* The ID of the change set.
*
*
* @param changeSetId
* The ID of the change set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder changeSetId(String changeSetId);
/**
*
* The name of the change set.
*
*
* @param changeSetName
* The name of the change set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder changeSetName(String changeSetName);
/**
*
* If the change set execution status is AVAILABLE
, you can execute the change set. If you can’t
* execute the change set, the status indicates why. For example, a change set might be in an
* UNAVAILABLE
state because AWS CloudFormation is still creating it or in an OBSOLETE
* state because the stack was already updated.
*
*
* @param executionStatus
* If the change set execution status is AVAILABLE
, you can execute the change set. If you
* can’t execute the change set, the status indicates why. For example, a change set might be in an
* UNAVAILABLE
state because AWS CloudFormation is still creating it or in an
* OBSOLETE
state because the stack was already updated.
* @see ExecutionStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExecutionStatus
*/
Builder executionStatus(String executionStatus);
/**
*
* If the change set execution status is AVAILABLE
, you can execute the change set. If you can’t
* execute the change set, the status indicates why. For example, a change set might be in an
* UNAVAILABLE
state because AWS CloudFormation is still creating it or in an OBSOLETE
* state because the stack was already updated.
*
*
* @param executionStatus
* If the change set execution status is AVAILABLE
, you can execute the change set. If you
* can’t execute the change set, the status indicates why. For example, a change set might be in an
* UNAVAILABLE
state because AWS CloudFormation is still creating it or in an
* OBSOLETE
state because the stack was already updated.
* @see ExecutionStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExecutionStatus
*/
Builder executionStatus(ExecutionStatus executionStatus);
/**
*
* The state of the change set, such as CREATE_IN_PROGRESS
, CREATE_COMPLETE
, or
* FAILED
.
*
*
* @param status
* The state of the change set, such as CREATE_IN_PROGRESS
, CREATE_COMPLETE
, or
* FAILED
.
* @see ChangeSetStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChangeSetStatus
*/
Builder status(String status);
/**
*
* The state of the change set, such as CREATE_IN_PROGRESS
, CREATE_COMPLETE
, or
* FAILED
.
*
*
* @param status
* The state of the change set, such as CREATE_IN_PROGRESS
, CREATE_COMPLETE
, or
* FAILED
.
* @see ChangeSetStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChangeSetStatus
*/
Builder status(ChangeSetStatus status);
/**
*
* A description of the change set's status. For example, if your change set is in the FAILED
* state, AWS CloudFormation shows the error message.
*
*
* @param statusReason
* A description of the change set's status. For example, if your change set is in the
* FAILED
state, AWS CloudFormation shows the error message.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder statusReason(String statusReason);
/**
*
* The start time when the change set was created, in UTC.
*
*
* @param creationTime
* The start time when the change set was created, in UTC.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder creationTime(Instant creationTime);
/**
*
* Descriptive information about the change set.
*
*
* @param description
* Descriptive information about the change set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
}
static final class BuilderImpl implements Builder {
private String stackId;
private String stackName;
private String changeSetId;
private String changeSetName;
private String executionStatus;
private String status;
private String statusReason;
private Instant creationTime;
private String description;
private BuilderImpl() {
}
private BuilderImpl(ChangeSetSummary model) {
stackId(model.stackId);
stackName(model.stackName);
changeSetId(model.changeSetId);
changeSetName(model.changeSetName);
executionStatus(model.executionStatus);
status(model.status);
statusReason(model.statusReason);
creationTime(model.creationTime);
description(model.description);
}
public final String getStackId() {
return stackId;
}
@Override
public final Builder stackId(String stackId) {
this.stackId = stackId;
return this;
}
public final void setStackId(String stackId) {
this.stackId = stackId;
}
public final String getStackName() {
return stackName;
}
@Override
public final Builder stackName(String stackName) {
this.stackName = stackName;
return this;
}
public final void setStackName(String stackName) {
this.stackName = stackName;
}
public final String getChangeSetId() {
return changeSetId;
}
@Override
public final Builder changeSetId(String changeSetId) {
this.changeSetId = changeSetId;
return this;
}
public final void setChangeSetId(String changeSetId) {
this.changeSetId = changeSetId;
}
public final String getChangeSetName() {
return changeSetName;
}
@Override
public final Builder changeSetName(String changeSetName) {
this.changeSetName = changeSetName;
return this;
}
public final void setChangeSetName(String changeSetName) {
this.changeSetName = changeSetName;
}
public final String getExecutionStatus() {
return executionStatus;
}
@Override
public final Builder executionStatus(String executionStatus) {
this.executionStatus = executionStatus;
return this;
}
@Override
public final Builder executionStatus(ExecutionStatus executionStatus) {
this.executionStatus(executionStatus.toString());
return this;
}
public final void setExecutionStatus(String executionStatus) {
this.executionStatus = executionStatus;
}
public final String getStatus() {
return status;
}
@Override
public final Builder status(String status) {
this.status = status;
return this;
}
@Override
public final Builder status(ChangeSetStatus status) {
this.status(status.toString());
return this;
}
public final void setStatus(String status) {
this.status = status;
}
public final String getStatusReason() {
return statusReason;
}
@Override
public final Builder statusReason(String statusReason) {
this.statusReason = statusReason;
return this;
}
public final void setStatusReason(String statusReason) {
this.statusReason = statusReason;
}
public final Instant getCreationTime() {
return creationTime;
}
@Override
public final Builder creationTime(Instant creationTime) {
this.creationTime = creationTime;
return this;
}
public final void setCreationTime(Instant creationTime) {
this.creationTime = creationTime;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
@Override
public ChangeSetSummary build() {
return new ChangeSetSummary(this);
}
}
}