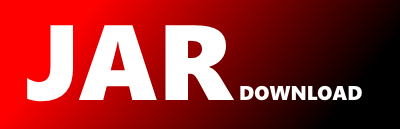
software.amazon.awssdk.services.cloudformation.model.TemplateParameter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudformation Show documentation
Show all versions of cloudformation Show documentation
The AWS Java SDK for AWS CloudFormation module holds the client classes that are used for communicating
with AWS CloudFormation Service
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.util.Optional;
import javax.annotation.Generated;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The TemplateParameter data type.
*
*/
@Generated("software.amazon.awssdk:codegen")
public class TemplateParameter implements ToCopyableBuilder {
private final String parameterKey;
private final String defaultValue;
private final Boolean noEcho;
private final String description;
private TemplateParameter(BuilderImpl builder) {
this.parameterKey = builder.parameterKey;
this.defaultValue = builder.defaultValue;
this.noEcho = builder.noEcho;
this.description = builder.description;
}
/**
*
* The name associated with the parameter.
*
*
* @return The name associated with the parameter.
*/
public String parameterKey() {
return parameterKey;
}
/**
*
* The default value associated with the parameter.
*
*
* @return The default value associated with the parameter.
*/
public String defaultValue() {
return defaultValue;
}
/**
*
* Flag indicating whether the parameter should be displayed as plain text in logs and UIs.
*
*
* @return Flag indicating whether the parameter should be displayed as plain text in logs and UIs.
*/
public Boolean noEcho() {
return noEcho;
}
/**
*
* User defined description associated with the parameter.
*
*
* @return User defined description associated with the parameter.
*/
public String description() {
return description;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((parameterKey() == null) ? 0 : parameterKey().hashCode());
hashCode = 31 * hashCode + ((defaultValue() == null) ? 0 : defaultValue().hashCode());
hashCode = 31 * hashCode + ((noEcho() == null) ? 0 : noEcho().hashCode());
hashCode = 31 * hashCode + ((description() == null) ? 0 : description().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TemplateParameter)) {
return false;
}
TemplateParameter other = (TemplateParameter) obj;
if (other.parameterKey() == null ^ this.parameterKey() == null) {
return false;
}
if (other.parameterKey() != null && !other.parameterKey().equals(this.parameterKey())) {
return false;
}
if (other.defaultValue() == null ^ this.defaultValue() == null) {
return false;
}
if (other.defaultValue() != null && !other.defaultValue().equals(this.defaultValue())) {
return false;
}
if (other.noEcho() == null ^ this.noEcho() == null) {
return false;
}
if (other.noEcho() != null && !other.noEcho().equals(this.noEcho())) {
return false;
}
if (other.description() == null ^ this.description() == null) {
return false;
}
if (other.description() != null && !other.description().equals(this.description())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder("{");
if (parameterKey() != null) {
sb.append("ParameterKey: ").append(parameterKey()).append(",");
}
if (defaultValue() != null) {
sb.append("DefaultValue: ").append(defaultValue()).append(",");
}
if (noEcho() != null) {
sb.append("NoEcho: ").append(noEcho()).append(",");
}
if (description() != null) {
sb.append("Description: ").append(description()).append(",");
}
if (sb.length() > 1) {
sb.setLength(sb.length() - 1);
}
sb.append("}");
return sb.toString();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ParameterKey":
return Optional.of(clazz.cast(parameterKey()));
case "DefaultValue":
return Optional.of(clazz.cast(defaultValue()));
case "NoEcho":
return Optional.of(clazz.cast(noEcho()));
case "Description":
return Optional.of(clazz.cast(description()));
default:
return Optional.empty();
}
}
public interface Builder extends CopyableBuilder {
/**
*
* The name associated with the parameter.
*
*
* @param parameterKey
* The name associated with the parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder parameterKey(String parameterKey);
/**
*
* The default value associated with the parameter.
*
*
* @param defaultValue
* The default value associated with the parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder defaultValue(String defaultValue);
/**
*
* Flag indicating whether the parameter should be displayed as plain text in logs and UIs.
*
*
* @param noEcho
* Flag indicating whether the parameter should be displayed as plain text in logs and UIs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder noEcho(Boolean noEcho);
/**
*
* User defined description associated with the parameter.
*
*
* @param description
* User defined description associated with the parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
}
static final class BuilderImpl implements Builder {
private String parameterKey;
private String defaultValue;
private Boolean noEcho;
private String description;
private BuilderImpl() {
}
private BuilderImpl(TemplateParameter model) {
parameterKey(model.parameterKey);
defaultValue(model.defaultValue);
noEcho(model.noEcho);
description(model.description);
}
public final String getParameterKey() {
return parameterKey;
}
@Override
public final Builder parameterKey(String parameterKey) {
this.parameterKey = parameterKey;
return this;
}
public final void setParameterKey(String parameterKey) {
this.parameterKey = parameterKey;
}
public final String getDefaultValue() {
return defaultValue;
}
@Override
public final Builder defaultValue(String defaultValue) {
this.defaultValue = defaultValue;
return this;
}
public final void setDefaultValue(String defaultValue) {
this.defaultValue = defaultValue;
}
public final Boolean getNoEcho() {
return noEcho;
}
@Override
public final Builder noEcho(Boolean noEcho) {
this.noEcho = noEcho;
return this;
}
public final void setNoEcho(Boolean noEcho) {
this.noEcho = noEcho;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
@Override
public TemplateParameter build() {
return new TemplateParameter(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy