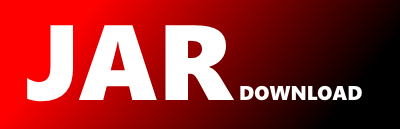
software.amazon.awssdk.services.cloudformation.model.UpdateStackRequest Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import javax.annotation.Generated;
import software.amazon.awssdk.core.AmazonWebServiceRequest;
import software.amazon.awssdk.core.runtime.TypeConverter;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The input for an UpdateStack action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public class UpdateStackRequest extends AmazonWebServiceRequest implements
ToCopyableBuilder {
private final String stackName;
private final String templateBody;
private final String templateURL;
private final Boolean usePreviousTemplate;
private final String stackPolicyDuringUpdateBody;
private final String stackPolicyDuringUpdateURL;
private final List parameters;
private final List capabilities;
private final List resourceTypes;
private final String roleARN;
private final String stackPolicyBody;
private final String stackPolicyURL;
private final List notificationARNs;
private final List tags;
private final String clientRequestToken;
private UpdateStackRequest(BuilderImpl builder) {
this.stackName = builder.stackName;
this.templateBody = builder.templateBody;
this.templateURL = builder.templateURL;
this.usePreviousTemplate = builder.usePreviousTemplate;
this.stackPolicyDuringUpdateBody = builder.stackPolicyDuringUpdateBody;
this.stackPolicyDuringUpdateURL = builder.stackPolicyDuringUpdateURL;
this.parameters = builder.parameters;
this.capabilities = builder.capabilities;
this.resourceTypes = builder.resourceTypes;
this.roleARN = builder.roleARN;
this.stackPolicyBody = builder.stackPolicyBody;
this.stackPolicyURL = builder.stackPolicyURL;
this.notificationARNs = builder.notificationARNs;
this.tags = builder.tags;
this.clientRequestToken = builder.clientRequestToken;
}
/**
*
* The name or unique stack ID of the stack to update.
*
*
* @return The name or unique stack ID of the stack to update.
*/
public String stackName() {
return stackName;
}
/**
*
* Structure containing the template body with a minimum length of 1 byte and a maximum length of 51,200 bytes. (For
* more information, go to Template Anatomy
* in the AWS CloudFormation User Guide.)
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
,
* TemplateURL
, or set the UsePreviousTemplate
to true
.
*
*
* @return Structure containing the template body with a minimum length of 1 byte and a maximum length of 51,200
* bytes. (For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.)
*
* Conditional: You must specify only one of the following parameters: TemplateBody
,
* TemplateURL
, or set the UsePreviousTemplate
to true
.
*/
public String templateBody() {
return templateBody;
}
/**
*
* Location of file containing the template body. The URL must point to a template that is located in an Amazon S3
* bucket. For more information, go to Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
,
* TemplateURL
, or set the UsePreviousTemplate
to true
.
*
*
* @return Location of file containing the template body. The URL must point to a template that is located in an
* Amazon S3 bucket. For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
,
* TemplateURL
, or set the UsePreviousTemplate
to true
.
*/
public String templateURL() {
return templateURL;
}
/**
*
* Reuse the existing template that is associated with the stack that you are updating.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
,
* TemplateURL
, or set the UsePreviousTemplate
to true
.
*
*
* @return Reuse the existing template that is associated with the stack that you are updating.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
,
* TemplateURL
, or set the UsePreviousTemplate
to true
.
*/
public Boolean usePreviousTemplate() {
return usePreviousTemplate;
}
/**
*
* Structure containing the temporary overriding stack policy body. You can specify either the
* StackPolicyDuringUpdateBody
or the StackPolicyDuringUpdateURL
parameter, but not both.
*
*
* If you want to update protected resources, specify a temporary overriding stack policy during this update. If you
* do not specify a stack policy, the current policy that is associated with the stack will be used.
*
*
* @return Structure containing the temporary overriding stack policy body. You can specify either the
* StackPolicyDuringUpdateBody
or the StackPolicyDuringUpdateURL
parameter, but
* not both.
*
* If you want to update protected resources, specify a temporary overriding stack policy during this
* update. If you do not specify a stack policy, the current policy that is associated with the stack will
* be used.
*/
public String stackPolicyDuringUpdateBody() {
return stackPolicyDuringUpdateBody;
}
/**
*
* Location of a file containing the temporary overriding stack policy. The URL must point to a policy (max size:
* 16KB) located in an S3 bucket in the same region as the stack. You can specify either the
* StackPolicyDuringUpdateBody
or the StackPolicyDuringUpdateURL
parameter, but not both.
*
*
* If you want to update protected resources, specify a temporary overriding stack policy during this update. If you
* do not specify a stack policy, the current policy that is associated with the stack will be used.
*
*
* @return Location of a file containing the temporary overriding stack policy. The URL must point to a policy (max
* size: 16KB) located in an S3 bucket in the same region as the stack. You can specify either the
* StackPolicyDuringUpdateBody
or the StackPolicyDuringUpdateURL
parameter, but
* not both.
*
* If you want to update protected resources, specify a temporary overriding stack policy during this
* update. If you do not specify a stack policy, the current policy that is associated with the stack will
* be used.
*/
public String stackPolicyDuringUpdateURL() {
return stackPolicyDuringUpdateURL;
}
/**
*
* A list of Parameter
structures that specify input parameters for the stack. For more information,
* see the Parameter data
* type.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of Parameter
structures that specify input parameters for the stack. For more
* information, see the Parameter
* data type.
*/
public List parameters() {
return parameters;
}
/**
*
* A list of values that you must specify before AWS CloudFormation can update certain stacks. Some stack templates
* might include resources that can affect permissions in your AWS account, for example, by creating new AWS
* Identity and Access Management (IAM) users. For those stacks, you must explicitly acknowledge their capabilities
* by specifying this parameter.
*
*
* The only valid values are CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
. The following
* resources require you to specify this parameter:
* AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::InstanceProfile,
* AWS::IAM::Policy,
* AWS::IAM::Role,
* AWS::IAM::User, and
* AWS::IAM::UserToGroupAddition. If your stack template contains these resources, we recommend that you review
* all permissions associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names, you
* must specify CAPABILITY_NAMED_IAM
. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of values that you must specify before AWS CloudFormation can update certain stacks. Some stack
* templates might include resources that can affect permissions in your AWS account, for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stacks, you must explicitly
* acknowledge their capabilities by specifying this parameter.
*
* The only valid values are CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
. The
* following resources require you to specify this parameter:
* AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::InstanceProfile,
* AWS::IAM::Policy,
* AWS::IAM::Role,
* AWS::IAM::User, and
* AWS::IAM::UserToGroupAddition. If your stack template contains these resources, we recommend that you
* review all permissions associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom
* names, you must specify CAPABILITY_NAMED_IAM
. If you don't specify this parameter, this
* action returns an InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*/
public List capabilities() {
return TypeConverter.convert(capabilities, Capability::fromValue);
}
/**
*
* A list of values that you must specify before AWS CloudFormation can update certain stacks. Some stack templates
* might include resources that can affect permissions in your AWS account, for example, by creating new AWS
* Identity and Access Management (IAM) users. For those stacks, you must explicitly acknowledge their capabilities
* by specifying this parameter.
*
*
* The only valid values are CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
. The following
* resources require you to specify this parameter:
* AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::InstanceProfile,
* AWS::IAM::Policy,
* AWS::IAM::Role,
* AWS::IAM::User, and
* AWS::IAM::UserToGroupAddition. If your stack template contains these resources, we recommend that you review
* all permissions associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names, you
* must specify CAPABILITY_NAMED_IAM
. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of values that you must specify before AWS CloudFormation can update certain stacks. Some stack
* templates might include resources that can affect permissions in your AWS account, for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stacks, you must explicitly
* acknowledge their capabilities by specifying this parameter.
*
* The only valid values are CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
. The
* following resources require you to specify this parameter:
* AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::InstanceProfile,
* AWS::IAM::Policy,
* AWS::IAM::Role,
* AWS::IAM::User, and
* AWS::IAM::UserToGroupAddition. If your stack template contains these resources, we recommend that you
* review all permissions associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom
* names, you must specify CAPABILITY_NAMED_IAM
. If you don't specify this parameter, this
* action returns an InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*/
public List capabilitiesStrings() {
return capabilities;
}
/**
*
* The template resource types that you have permissions to work with for this update stack action, such as
* AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
.
*
*
* If the list of resource types doesn't include a resource that you're updating, the stack update fails. By
* default, AWS CloudFormation grants permissions to all resource types. AWS Identity and Access Management (IAM)
* uses this parameter for AWS CloudFormation-specific condition keys in IAM policies. For more information, see Controlling Access
* with AWS Identity and Access Management.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The template resource types that you have permissions to work with for this update stack action, such as
* AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
.
*
* If the list of resource types doesn't include a resource that you're updating, the stack update fails. By
* default, AWS CloudFormation grants permissions to all resource types. AWS Identity and Access Management
* (IAM) uses this parameter for AWS CloudFormation-specific condition keys in IAM policies. For more
* information, see Controlling
* Access with AWS Identity and Access Management.
*/
public List resourceTypes() {
return resourceTypes;
}
/**
*
* The Amazon Resource Name (ARN) of an AWS Identity and Access Management (IAM) role that AWS CloudFormation
* assumes to update the stack. AWS CloudFormation uses the role's credentials to make calls on your behalf. AWS
* CloudFormation always uses this role for all future operations on the stack. As long as users have permission to
* operate on the stack, AWS CloudFormation uses this role even if the users don't have permission to pass it.
* Ensure that the role grants least privilege.
*
*
* If you don't specify a value, AWS CloudFormation uses the role that was previously associated with the stack. If
* no role is available, AWS CloudFormation uses a temporary session that is generated from your user credentials.
*
*
* @return The Amazon Resource Name (ARN) of an AWS Identity and Access Management (IAM) role that AWS
* CloudFormation assumes to update the stack. AWS CloudFormation uses the role's credentials to make calls
* on your behalf. AWS CloudFormation always uses this role for all future operations on the stack. As long
* as users have permission to operate on the stack, AWS CloudFormation uses this role even if the users
* don't have permission to pass it. Ensure that the role grants least privilege.
*
* If you don't specify a value, AWS CloudFormation uses the role that was previously associated with the
* stack. If no role is available, AWS CloudFormation uses a temporary session that is generated from your
* user credentials.
*/
public String roleARN() {
return roleARN;
}
/**
*
* Structure containing a new stack policy body. You can specify either the StackPolicyBody
or the
* StackPolicyURL
parameter, but not both.
*
*
* You might update the stack policy, for example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy that is associated with the stack is
* unchanged.
*
*
* @return Structure containing a new stack policy body. You can specify either the StackPolicyBody
or
* the StackPolicyURL
parameter, but not both.
*
* You might update the stack policy, for example, in order to protect a new resource that you created
* during a stack update. If you do not specify a stack policy, the current policy that is associated with
* the stack is unchanged.
*/
public String stackPolicyBody() {
return stackPolicyBody;
}
/**
*
* Location of a file containing the updated stack policy. The URL must point to a policy (max size: 16KB) located
* in an S3 bucket in the same region as the stack. You can specify either the StackPolicyBody
or the
* StackPolicyURL
parameter, but not both.
*
*
* You might update the stack policy, for example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy that is associated with the stack is
* unchanged.
*
*
* @return Location of a file containing the updated stack policy. The URL must point to a policy (max size: 16KB)
* located in an S3 bucket in the same region as the stack. You can specify either the
* StackPolicyBody
or the StackPolicyURL
parameter, but not both.
*
* You might update the stack policy, for example, in order to protect a new resource that you created
* during a stack update. If you do not specify a stack policy, the current policy that is associated with
* the stack is unchanged.
*/
public String stackPolicyURL() {
return stackPolicyURL;
}
/**
*
* Amazon Simple Notification Service topic Amazon Resource Names (ARNs) that AWS CloudFormation associates with the
* stack. Specify an empty list to remove all notification topics.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Amazon Simple Notification Service topic Amazon Resource Names (ARNs) that AWS CloudFormation associates
* with the stack. Specify an empty list to remove all notification topics.
*/
public List notificationARNs() {
return notificationARNs;
}
/**
*
* Key-value pairs to associate with this stack. AWS CloudFormation also propagates these tags to supported
* resources in the stack. You can specify a maximum number of 10 tags.
*
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags. If you specify an empty
* value, AWS CloudFormation removes all associated tags.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Key-value pairs to associate with this stack. AWS CloudFormation also propagates these tags to supported
* resources in the stack. You can specify a maximum number of 10 tags.
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags. If you specify
* an empty value, AWS CloudFormation removes all associated tags.
*/
public List tags() {
return tags;
}
/**
*
* A unique identifier for this UpdateStack
request. Specify this token if you plan to retry requests
* so that AWS CloudFormation knows that you're not attempting to update a stack with the same name. You might retry
* UpdateStack
requests to ensure that AWS CloudFormation successfully received them.
*
*
* @return A unique identifier for this UpdateStack
request. Specify this token if you plan to retry
* requests so that AWS CloudFormation knows that you're not attempting to update a stack with the same
* name. You might retry UpdateStack
requests to ensure that AWS CloudFormation successfully
* received them.
*/
public String clientRequestToken() {
return clientRequestToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((stackName() == null) ? 0 : stackName().hashCode());
hashCode = 31 * hashCode + ((templateBody() == null) ? 0 : templateBody().hashCode());
hashCode = 31 * hashCode + ((templateURL() == null) ? 0 : templateURL().hashCode());
hashCode = 31 * hashCode + ((usePreviousTemplate() == null) ? 0 : usePreviousTemplate().hashCode());
hashCode = 31 * hashCode + ((stackPolicyDuringUpdateBody() == null) ? 0 : stackPolicyDuringUpdateBody().hashCode());
hashCode = 31 * hashCode + ((stackPolicyDuringUpdateURL() == null) ? 0 : stackPolicyDuringUpdateURL().hashCode());
hashCode = 31 * hashCode + ((parameters() == null) ? 0 : parameters().hashCode());
hashCode = 31 * hashCode + ((capabilitiesStrings() == null) ? 0 : capabilitiesStrings().hashCode());
hashCode = 31 * hashCode + ((resourceTypes() == null) ? 0 : resourceTypes().hashCode());
hashCode = 31 * hashCode + ((roleARN() == null) ? 0 : roleARN().hashCode());
hashCode = 31 * hashCode + ((stackPolicyBody() == null) ? 0 : stackPolicyBody().hashCode());
hashCode = 31 * hashCode + ((stackPolicyURL() == null) ? 0 : stackPolicyURL().hashCode());
hashCode = 31 * hashCode + ((notificationARNs() == null) ? 0 : notificationARNs().hashCode());
hashCode = 31 * hashCode + ((tags() == null) ? 0 : tags().hashCode());
hashCode = 31 * hashCode + ((clientRequestToken() == null) ? 0 : clientRequestToken().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateStackRequest)) {
return false;
}
UpdateStackRequest other = (UpdateStackRequest) obj;
if (other.stackName() == null ^ this.stackName() == null) {
return false;
}
if (other.stackName() != null && !other.stackName().equals(this.stackName())) {
return false;
}
if (other.templateBody() == null ^ this.templateBody() == null) {
return false;
}
if (other.templateBody() != null && !other.templateBody().equals(this.templateBody())) {
return false;
}
if (other.templateURL() == null ^ this.templateURL() == null) {
return false;
}
if (other.templateURL() != null && !other.templateURL().equals(this.templateURL())) {
return false;
}
if (other.usePreviousTemplate() == null ^ this.usePreviousTemplate() == null) {
return false;
}
if (other.usePreviousTemplate() != null && !other.usePreviousTemplate().equals(this.usePreviousTemplate())) {
return false;
}
if (other.stackPolicyDuringUpdateBody() == null ^ this.stackPolicyDuringUpdateBody() == null) {
return false;
}
if (other.stackPolicyDuringUpdateBody() != null
&& !other.stackPolicyDuringUpdateBody().equals(this.stackPolicyDuringUpdateBody())) {
return false;
}
if (other.stackPolicyDuringUpdateURL() == null ^ this.stackPolicyDuringUpdateURL() == null) {
return false;
}
if (other.stackPolicyDuringUpdateURL() != null
&& !other.stackPolicyDuringUpdateURL().equals(this.stackPolicyDuringUpdateURL())) {
return false;
}
if (other.parameters() == null ^ this.parameters() == null) {
return false;
}
if (other.parameters() != null && !other.parameters().equals(this.parameters())) {
return false;
}
if (other.capabilitiesStrings() == null ^ this.capabilitiesStrings() == null) {
return false;
}
if (other.capabilitiesStrings() != null && !other.capabilitiesStrings().equals(this.capabilitiesStrings())) {
return false;
}
if (other.resourceTypes() == null ^ this.resourceTypes() == null) {
return false;
}
if (other.resourceTypes() != null && !other.resourceTypes().equals(this.resourceTypes())) {
return false;
}
if (other.roleARN() == null ^ this.roleARN() == null) {
return false;
}
if (other.roleARN() != null && !other.roleARN().equals(this.roleARN())) {
return false;
}
if (other.stackPolicyBody() == null ^ this.stackPolicyBody() == null) {
return false;
}
if (other.stackPolicyBody() != null && !other.stackPolicyBody().equals(this.stackPolicyBody())) {
return false;
}
if (other.stackPolicyURL() == null ^ this.stackPolicyURL() == null) {
return false;
}
if (other.stackPolicyURL() != null && !other.stackPolicyURL().equals(this.stackPolicyURL())) {
return false;
}
if (other.notificationARNs() == null ^ this.notificationARNs() == null) {
return false;
}
if (other.notificationARNs() != null && !other.notificationARNs().equals(this.notificationARNs())) {
return false;
}
if (other.tags() == null ^ this.tags() == null) {
return false;
}
if (other.tags() != null && !other.tags().equals(this.tags())) {
return false;
}
if (other.clientRequestToken() == null ^ this.clientRequestToken() == null) {
return false;
}
if (other.clientRequestToken() != null && !other.clientRequestToken().equals(this.clientRequestToken())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder("{");
if (stackName() != null) {
sb.append("StackName: ").append(stackName()).append(",");
}
if (templateBody() != null) {
sb.append("TemplateBody: ").append(templateBody()).append(",");
}
if (templateURL() != null) {
sb.append("TemplateURL: ").append(templateURL()).append(",");
}
if (usePreviousTemplate() != null) {
sb.append("UsePreviousTemplate: ").append(usePreviousTemplate()).append(",");
}
if (stackPolicyDuringUpdateBody() != null) {
sb.append("StackPolicyDuringUpdateBody: ").append(stackPolicyDuringUpdateBody()).append(",");
}
if (stackPolicyDuringUpdateURL() != null) {
sb.append("StackPolicyDuringUpdateURL: ").append(stackPolicyDuringUpdateURL()).append(",");
}
if (parameters() != null) {
sb.append("Parameters: ").append(parameters()).append(",");
}
if (capabilitiesStrings() != null) {
sb.append("Capabilities: ").append(capabilitiesStrings()).append(",");
}
if (resourceTypes() != null) {
sb.append("ResourceTypes: ").append(resourceTypes()).append(",");
}
if (roleARN() != null) {
sb.append("RoleARN: ").append(roleARN()).append(",");
}
if (stackPolicyBody() != null) {
sb.append("StackPolicyBody: ").append(stackPolicyBody()).append(",");
}
if (stackPolicyURL() != null) {
sb.append("StackPolicyURL: ").append(stackPolicyURL()).append(",");
}
if (notificationARNs() != null) {
sb.append("NotificationARNs: ").append(notificationARNs()).append(",");
}
if (tags() != null) {
sb.append("Tags: ").append(tags()).append(",");
}
if (clientRequestToken() != null) {
sb.append("ClientRequestToken: ").append(clientRequestToken()).append(",");
}
if (sb.length() > 1) {
sb.setLength(sb.length() - 1);
}
sb.append("}");
return sb.toString();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackName":
return Optional.of(clazz.cast(stackName()));
case "TemplateBody":
return Optional.of(clazz.cast(templateBody()));
case "TemplateURL":
return Optional.of(clazz.cast(templateURL()));
case "UsePreviousTemplate":
return Optional.of(clazz.cast(usePreviousTemplate()));
case "StackPolicyDuringUpdateBody":
return Optional.of(clazz.cast(stackPolicyDuringUpdateBody()));
case "StackPolicyDuringUpdateURL":
return Optional.of(clazz.cast(stackPolicyDuringUpdateURL()));
case "Parameters":
return Optional.of(clazz.cast(parameters()));
case "Capabilities":
return Optional.of(clazz.cast(capabilitiesStrings()));
case "ResourceTypes":
return Optional.of(clazz.cast(resourceTypes()));
case "RoleARN":
return Optional.of(clazz.cast(roleARN()));
case "StackPolicyBody":
return Optional.of(clazz.cast(stackPolicyBody()));
case "StackPolicyURL":
return Optional.of(clazz.cast(stackPolicyURL()));
case "NotificationARNs":
return Optional.of(clazz.cast(notificationARNs()));
case "Tags":
return Optional.of(clazz.cast(tags()));
case "ClientRequestToken":
return Optional.of(clazz.cast(clientRequestToken()));
default:
return Optional.empty();
}
}
public interface Builder extends CopyableBuilder {
/**
*
* The name or unique stack ID of the stack to update.
*
*
* @param stackName
* The name or unique stack ID of the stack to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stackName(String stackName);
/**
*
* Structure containing the template body with a minimum length of 1 byte and a maximum length of 51,200 bytes.
* (For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.)
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
,
* TemplateURL
, or set the UsePreviousTemplate
to true
.
*
*
* @param templateBody
* Structure containing the template body with a minimum length of 1 byte and a maximum length of 51,200
* bytes. (For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.)
*
* Conditional: You must specify only one of the following parameters: TemplateBody
,
* TemplateURL
, or set the UsePreviousTemplate
to true
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder templateBody(String templateBody);
/**
*
* Location of file containing the template body. The URL must point to a template that is located in an Amazon
* S3 bucket. For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
,
* TemplateURL
, or set the UsePreviousTemplate
to true
.
*
*
* @param templateURL
* Location of file containing the template body. The URL must point to a template that is located in an
* Amazon S3 bucket. For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
,
* TemplateURL
, or set the UsePreviousTemplate
to true
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder templateURL(String templateURL);
/**
*
* Reuse the existing template that is associated with the stack that you are updating.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
,
* TemplateURL
, or set the UsePreviousTemplate
to true
.
*
*
* @param usePreviousTemplate
* Reuse the existing template that is associated with the stack that you are updating.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
,
* TemplateURL
, or set the UsePreviousTemplate
to true
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder usePreviousTemplate(Boolean usePreviousTemplate);
/**
*
* Structure containing the temporary overriding stack policy body. You can specify either the
* StackPolicyDuringUpdateBody
or the StackPolicyDuringUpdateURL
parameter, but not
* both.
*
*
* If you want to update protected resources, specify a temporary overriding stack policy during this update. If
* you do not specify a stack policy, the current policy that is associated with the stack will be used.
*
*
* @param stackPolicyDuringUpdateBody
* Structure containing the temporary overriding stack policy body. You can specify either the
* StackPolicyDuringUpdateBody
or the StackPolicyDuringUpdateURL
parameter, but
* not both.
*
* If you want to update protected resources, specify a temporary overriding stack policy during this
* update. If you do not specify a stack policy, the current policy that is associated with the stack
* will be used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stackPolicyDuringUpdateBody(String stackPolicyDuringUpdateBody);
/**
*
* Location of a file containing the temporary overriding stack policy. The URL must point to a policy (max
* size: 16KB) located in an S3 bucket in the same region as the stack. You can specify either the
* StackPolicyDuringUpdateBody
or the StackPolicyDuringUpdateURL
parameter, but not
* both.
*
*
* If you want to update protected resources, specify a temporary overriding stack policy during this update. If
* you do not specify a stack policy, the current policy that is associated with the stack will be used.
*
*
* @param stackPolicyDuringUpdateURL
* Location of a file containing the temporary overriding stack policy. The URL must point to a policy
* (max size: 16KB) located in an S3 bucket in the same region as the stack. You can specify either the
* StackPolicyDuringUpdateBody
or the StackPolicyDuringUpdateURL
parameter, but
* not both.
*
* If you want to update protected resources, specify a temporary overriding stack policy during this
* update. If you do not specify a stack policy, the current policy that is associated with the stack
* will be used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stackPolicyDuringUpdateURL(String stackPolicyDuringUpdateURL);
/**
*
* A list of Parameter
structures that specify input parameters for the stack. For more
* information, see the Parameter data
* type.
*
*
* @param parameters
* A list of Parameter
structures that specify input parameters for the stack. For more
* information, see the Parameter data type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder parameters(Collection parameters);
/**
*
* A list of Parameter
structures that specify input parameters for the stack. For more
* information, see the Parameter data
* type.
*
*
* @param parameters
* A list of Parameter
structures that specify input parameters for the stack. For more
* information, see the Parameter data type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder parameters(Parameter... parameters);
/**
*
* A list of values that you must specify before AWS CloudFormation can update certain stacks. Some stack
* templates might include resources that can affect permissions in your AWS account, for example, by creating
* new AWS Identity and Access Management (IAM) users. For those stacks, you must explicitly acknowledge their
* capabilities by specifying this parameter.
*
*
* The only valid values are CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
. The following
* resources require you to specify this parameter:
* AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::InstanceProfile,
* AWS::IAM::Policy,
* AWS::IAM::Role,
* AWS::IAM::User, and
* AWS::IAM::UserToGroupAddition. If your stack template contains these resources, we recommend that you
* review all permissions associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names,
* you must specify CAPABILITY_NAMED_IAM
. If you don't specify this parameter, this action returns
* an InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* @param capabilities
* A list of values that you must specify before AWS CloudFormation can update certain stacks. Some stack
* templates might include resources that can affect permissions in your AWS account, for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stacks, you must explicitly
* acknowledge their capabilities by specifying this parameter.
*
* The only valid values are CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
. The
* following resources require you to specify this parameter:
* AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::InstanceProfile,
* AWS::IAM::Policy,
* AWS::IAM::Role,
* AWS::IAM::User, and AWS::IAM::UserToGroupAddition. If your stack template contains these resources, we recommend
* that you review all permissions associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom
* names, you must specify CAPABILITY_NAMED_IAM
. If you don't specify this parameter, this
* action returns an InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilities(Collection capabilities);
/**
*
* A list of values that you must specify before AWS CloudFormation can update certain stacks. Some stack
* templates might include resources that can affect permissions in your AWS account, for example, by creating
* new AWS Identity and Access Management (IAM) users. For those stacks, you must explicitly acknowledge their
* capabilities by specifying this parameter.
*
*
* The only valid values are CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
. The following
* resources require you to specify this parameter:
* AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::InstanceProfile,
* AWS::IAM::Policy,
* AWS::IAM::Role,
* AWS::IAM::User, and
* AWS::IAM::UserToGroupAddition. If your stack template contains these resources, we recommend that you
* review all permissions associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names,
* you must specify CAPABILITY_NAMED_IAM
. If you don't specify this parameter, this action returns
* an InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* @param capabilities
* A list of values that you must specify before AWS CloudFormation can update certain stacks. Some stack
* templates might include resources that can affect permissions in your AWS account, for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stacks, you must explicitly
* acknowledge their capabilities by specifying this parameter.
*
* The only valid values are CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
. The
* following resources require you to specify this parameter:
* AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::InstanceProfile,
* AWS::IAM::Policy,
* AWS::IAM::Role,
* AWS::IAM::User, and AWS::IAM::UserToGroupAddition. If your stack template contains these resources, we recommend
* that you review all permissions associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom
* names, you must specify CAPABILITY_NAMED_IAM
. If you don't specify this parameter, this
* action returns an InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilities(String... capabilities);
/**
*
* The template resource types that you have permissions to work with for this update stack action, such as
* AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
.
*
*
* If the list of resource types doesn't include a resource that you're updating, the stack update fails. By
* default, AWS CloudFormation grants permissions to all resource types. AWS Identity and Access Management
* (IAM) uses this parameter for AWS CloudFormation-specific condition keys in IAM policies. For more
* information, see Controlling
* Access with AWS Identity and Access Management.
*
*
* @param resourceTypes
* The template resource types that you have permissions to work with for this update stack action, such
* as AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
* .
*
* If the list of resource types doesn't include a resource that you're updating, the stack update fails.
* By default, AWS CloudFormation grants permissions to all resource types. AWS Identity and Access
* Management (IAM) uses this parameter for AWS CloudFormation-specific condition keys in IAM policies.
* For more information, see Controlling Access with AWS Identity and Access Management.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder resourceTypes(Collection resourceTypes);
/**
*
* The template resource types that you have permissions to work with for this update stack action, such as
* AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
.
*
*
* If the list of resource types doesn't include a resource that you're updating, the stack update fails. By
* default, AWS CloudFormation grants permissions to all resource types. AWS Identity and Access Management
* (IAM) uses this parameter for AWS CloudFormation-specific condition keys in IAM policies. For more
* information, see Controlling
* Access with AWS Identity and Access Management.
*
*
* @param resourceTypes
* The template resource types that you have permissions to work with for this update stack action, such
* as AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
* .
*
* If the list of resource types doesn't include a resource that you're updating, the stack update fails.
* By default, AWS CloudFormation grants permissions to all resource types. AWS Identity and Access
* Management (IAM) uses this parameter for AWS CloudFormation-specific condition keys in IAM policies.
* For more information, see Controlling Access with AWS Identity and Access Management.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder resourceTypes(String... resourceTypes);
/**
*
* The Amazon Resource Name (ARN) of an AWS Identity and Access Management (IAM) role that AWS CloudFormation
* assumes to update the stack. AWS CloudFormation uses the role's credentials to make calls on your behalf. AWS
* CloudFormation always uses this role for all future operations on the stack. As long as users have permission
* to operate on the stack, AWS CloudFormation uses this role even if the users don't have permission to pass
* it. Ensure that the role grants least privilege.
*
*
* If you don't specify a value, AWS CloudFormation uses the role that was previously associated with the stack.
* If no role is available, AWS CloudFormation uses a temporary session that is generated from your user
* credentials.
*
*
* @param roleARN
* The Amazon Resource Name (ARN) of an AWS Identity and Access Management (IAM) role that AWS
* CloudFormation assumes to update the stack. AWS CloudFormation uses the role's credentials to make
* calls on your behalf. AWS CloudFormation always uses this role for all future operations on the stack.
* As long as users have permission to operate on the stack, AWS CloudFormation uses this role even if
* the users don't have permission to pass it. Ensure that the role grants least privilege.
*
* If you don't specify a value, AWS CloudFormation uses the role that was previously associated with the
* stack. If no role is available, AWS CloudFormation uses a temporary session that is generated from
* your user credentials.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder roleARN(String roleARN);
/**
*
* Structure containing a new stack policy body. You can specify either the StackPolicyBody
or the
* StackPolicyURL
parameter, but not both.
*
*
* You might update the stack policy, for example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy that is associated with the stack is
* unchanged.
*
*
* @param stackPolicyBody
* Structure containing a new stack policy body. You can specify either the StackPolicyBody
* or the StackPolicyURL
parameter, but not both.
*
* You might update the stack policy, for example, in order to protect a new resource that you created
* during a stack update. If you do not specify a stack policy, the current policy that is associated
* with the stack is unchanged.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stackPolicyBody(String stackPolicyBody);
/**
*
* Location of a file containing the updated stack policy. The URL must point to a policy (max size: 16KB)
* located in an S3 bucket in the same region as the stack. You can specify either the
* StackPolicyBody
or the StackPolicyURL
parameter, but not both.
*
*
* You might update the stack policy, for example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy that is associated with the stack is
* unchanged.
*
*
* @param stackPolicyURL
* Location of a file containing the updated stack policy. The URL must point to a policy (max size:
* 16KB) located in an S3 bucket in the same region as the stack. You can specify either the
* StackPolicyBody
or the StackPolicyURL
parameter, but not both.
*
* You might update the stack policy, for example, in order to protect a new resource that you created
* during a stack update. If you do not specify a stack policy, the current policy that is associated
* with the stack is unchanged.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stackPolicyURL(String stackPolicyURL);
/**
*
* Amazon Simple Notification Service topic Amazon Resource Names (ARNs) that AWS CloudFormation associates with
* the stack. Specify an empty list to remove all notification topics.
*
*
* @param notificationARNs
* Amazon Simple Notification Service topic Amazon Resource Names (ARNs) that AWS CloudFormation
* associates with the stack. Specify an empty list to remove all notification topics.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder notificationARNs(Collection notificationARNs);
/**
*
* Amazon Simple Notification Service topic Amazon Resource Names (ARNs) that AWS CloudFormation associates with
* the stack. Specify an empty list to remove all notification topics.
*
*
* @param notificationARNs
* Amazon Simple Notification Service topic Amazon Resource Names (ARNs) that AWS CloudFormation
* associates with the stack. Specify an empty list to remove all notification topics.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder notificationARNs(String... notificationARNs);
/**
*
* Key-value pairs to associate with this stack. AWS CloudFormation also propagates these tags to supported
* resources in the stack. You can specify a maximum number of 10 tags.
*
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags. If you specify an
* empty value, AWS CloudFormation removes all associated tags.
*
*
* @param tags
* Key-value pairs to associate with this stack. AWS CloudFormation also propagates these tags to
* supported resources in the stack. You can specify a maximum number of 10 tags.
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags. If you
* specify an empty value, AWS CloudFormation removes all associated tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* Key-value pairs to associate with this stack. AWS CloudFormation also propagates these tags to supported
* resources in the stack. You can specify a maximum number of 10 tags.
*
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags. If you specify an
* empty value, AWS CloudFormation removes all associated tags.
*
*
* @param tags
* Key-value pairs to associate with this stack. AWS CloudFormation also propagates these tags to
* supported resources in the stack. You can specify a maximum number of 10 tags.
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags. If you
* specify an empty value, AWS CloudFormation removes all associated tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* A unique identifier for this UpdateStack
request. Specify this token if you plan to retry
* requests so that AWS CloudFormation knows that you're not attempting to update a stack with the same name.
* You might retry UpdateStack
requests to ensure that AWS CloudFormation successfully received
* them.
*
*
* @param clientRequestToken
* A unique identifier for this UpdateStack
request. Specify this token if you plan to retry
* requests so that AWS CloudFormation knows that you're not attempting to update a stack with the same
* name. You might retry UpdateStack
requests to ensure that AWS CloudFormation successfully
* received them.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clientRequestToken(String clientRequestToken);
}
static final class BuilderImpl implements Builder {
private String stackName;
private String templateBody;
private String templateURL;
private Boolean usePreviousTemplate;
private String stackPolicyDuringUpdateBody;
private String stackPolicyDuringUpdateURL;
private List parameters;
private List capabilities;
private List resourceTypes;
private String roleARN;
private String stackPolicyBody;
private String stackPolicyURL;
private List notificationARNs;
private List tags;
private String clientRequestToken;
private BuilderImpl() {
}
private BuilderImpl(UpdateStackRequest model) {
stackName(model.stackName);
templateBody(model.templateBody);
templateURL(model.templateURL);
usePreviousTemplate(model.usePreviousTemplate);
stackPolicyDuringUpdateBody(model.stackPolicyDuringUpdateBody);
stackPolicyDuringUpdateURL(model.stackPolicyDuringUpdateURL);
parameters(model.parameters);
capabilities(model.capabilities);
resourceTypes(model.resourceTypes);
roleARN(model.roleARN);
stackPolicyBody(model.stackPolicyBody);
stackPolicyURL(model.stackPolicyURL);
notificationARNs(model.notificationARNs);
tags(model.tags);
clientRequestToken(model.clientRequestToken);
}
public final String getStackName() {
return stackName;
}
@Override
public final Builder stackName(String stackName) {
this.stackName = stackName;
return this;
}
public final void setStackName(String stackName) {
this.stackName = stackName;
}
public final String getTemplateBody() {
return templateBody;
}
@Override
public final Builder templateBody(String templateBody) {
this.templateBody = templateBody;
return this;
}
public final void setTemplateBody(String templateBody) {
this.templateBody = templateBody;
}
public final String getTemplateURL() {
return templateURL;
}
@Override
public final Builder templateURL(String templateURL) {
this.templateURL = templateURL;
return this;
}
public final void setTemplateURL(String templateURL) {
this.templateURL = templateURL;
}
public final Boolean getUsePreviousTemplate() {
return usePreviousTemplate;
}
@Override
public final Builder usePreviousTemplate(Boolean usePreviousTemplate) {
this.usePreviousTemplate = usePreviousTemplate;
return this;
}
public final void setUsePreviousTemplate(Boolean usePreviousTemplate) {
this.usePreviousTemplate = usePreviousTemplate;
}
public final String getStackPolicyDuringUpdateBody() {
return stackPolicyDuringUpdateBody;
}
@Override
public final Builder stackPolicyDuringUpdateBody(String stackPolicyDuringUpdateBody) {
this.stackPolicyDuringUpdateBody = stackPolicyDuringUpdateBody;
return this;
}
public final void setStackPolicyDuringUpdateBody(String stackPolicyDuringUpdateBody) {
this.stackPolicyDuringUpdateBody = stackPolicyDuringUpdateBody;
}
public final String getStackPolicyDuringUpdateURL() {
return stackPolicyDuringUpdateURL;
}
@Override
public final Builder stackPolicyDuringUpdateURL(String stackPolicyDuringUpdateURL) {
this.stackPolicyDuringUpdateURL = stackPolicyDuringUpdateURL;
return this;
}
public final void setStackPolicyDuringUpdateURL(String stackPolicyDuringUpdateURL) {
this.stackPolicyDuringUpdateURL = stackPolicyDuringUpdateURL;
}
public final Collection getParameters() {
return parameters != null ? parameters.stream().map(Parameter::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder parameters(Collection parameters) {
this.parameters = ParametersCopier.copy(parameters);
return this;
}
@Override
@SafeVarargs
public final Builder parameters(Parameter... parameters) {
parameters(Arrays.asList(parameters));
return this;
}
public final void setParameters(Collection parameters) {
this.parameters = ParametersCopier.copyFromBuilder(parameters);
}
public final Collection getCapabilities() {
return capabilities;
}
@Override
public final Builder capabilities(Collection capabilities) {
this.capabilities = CapabilitiesCopier.copy(capabilities);
return this;
}
@Override
@SafeVarargs
public final Builder capabilities(String... capabilities) {
capabilities(Arrays.asList(capabilities));
return this;
}
public final void setCapabilities(Collection capabilities) {
this.capabilities = CapabilitiesCopier.copy(capabilities);
}
public final Collection getResourceTypes() {
return resourceTypes;
}
@Override
public final Builder resourceTypes(Collection resourceTypes) {
this.resourceTypes = ResourceTypesCopier.copy(resourceTypes);
return this;
}
@Override
@SafeVarargs
public final Builder resourceTypes(String... resourceTypes) {
resourceTypes(Arrays.asList(resourceTypes));
return this;
}
public final void setResourceTypes(Collection resourceTypes) {
this.resourceTypes = ResourceTypesCopier.copy(resourceTypes);
}
public final String getRoleARN() {
return roleARN;
}
@Override
public final Builder roleARN(String roleARN) {
this.roleARN = roleARN;
return this;
}
public final void setRoleARN(String roleARN) {
this.roleARN = roleARN;
}
public final String getStackPolicyBody() {
return stackPolicyBody;
}
@Override
public final Builder stackPolicyBody(String stackPolicyBody) {
this.stackPolicyBody = stackPolicyBody;
return this;
}
public final void setStackPolicyBody(String stackPolicyBody) {
this.stackPolicyBody = stackPolicyBody;
}
public final String getStackPolicyURL() {
return stackPolicyURL;
}
@Override
public final Builder stackPolicyURL(String stackPolicyURL) {
this.stackPolicyURL = stackPolicyURL;
return this;
}
public final void setStackPolicyURL(String stackPolicyURL) {
this.stackPolicyURL = stackPolicyURL;
}
public final Collection getNotificationARNs() {
return notificationARNs;
}
@Override
public final Builder notificationARNs(Collection notificationARNs) {
this.notificationARNs = NotificationARNsCopier.copy(notificationARNs);
return this;
}
@Override
@SafeVarargs
public final Builder notificationARNs(String... notificationARNs) {
notificationARNs(Arrays.asList(notificationARNs));
return this;
}
public final void setNotificationARNs(Collection notificationARNs) {
this.notificationARNs = NotificationARNsCopier.copy(notificationARNs);
}
public final Collection getTags() {
return tags != null ? tags.stream().map(Tag::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagsCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
public final void setTags(Collection tags) {
this.tags = TagsCopier.copyFromBuilder(tags);
}
public final String getClientRequestToken() {
return clientRequestToken;
}
@Override
public final Builder clientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
return this;
}
public final void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
@Override
public UpdateStackRequest build() {
return new UpdateStackRequest(this);
}
}
}