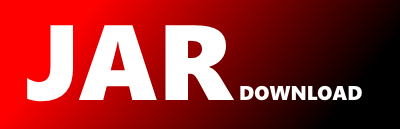
software.amazon.awssdk.services.cloudformation.model.DeleteStackInstancesRequest Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeleteStackInstancesRequest extends CloudFormationRequest implements
ToCopyableBuilder {
private static final SdkField STACK_SET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DeleteStackInstancesRequest::stackSetName)).setter(setter(Builder::stackSetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackSetName").build()).build();
private static final SdkField> ACCOUNTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(DeleteStackInstancesRequest::accounts))
.setter(setter(Builder::accounts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Accounts").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> REGIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(DeleteStackInstancesRequest::regions))
.setter(setter(Builder::regions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Regions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField OPERATION_PREFERENCES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(DeleteStackInstancesRequest::operationPreferences)).setter(setter(Builder::operationPreferences))
.constructor(StackSetOperationPreferences::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OperationPreferences").build())
.build();
private static final SdkField RETAIN_STACKS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(DeleteStackInstancesRequest::retainStacks)).setter(setter(Builder::retainStacks))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RetainStacks").build()).build();
private static final SdkField OPERATION_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(DeleteStackInstancesRequest::operationId))
.setter(setter(Builder::operationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OperationId").build(),
DefaultValueTrait.idempotencyToken()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STACK_SET_NAME_FIELD,
ACCOUNTS_FIELD, REGIONS_FIELD, OPERATION_PREFERENCES_FIELD, RETAIN_STACKS_FIELD, OPERATION_ID_FIELD));
private final String stackSetName;
private final List accounts;
private final List regions;
private final StackSetOperationPreferences operationPreferences;
private final Boolean retainStacks;
private final String operationId;
private DeleteStackInstancesRequest(BuilderImpl builder) {
super(builder);
this.stackSetName = builder.stackSetName;
this.accounts = builder.accounts;
this.regions = builder.regions;
this.operationPreferences = builder.operationPreferences;
this.retainStacks = builder.retainStacks;
this.operationId = builder.operationId;
}
/**
*
* The name or unique ID of the stack set that you want to delete stack instances for.
*
*
* @return The name or unique ID of the stack set that you want to delete stack instances for.
*/
public String stackSetName() {
return stackSetName;
}
/**
* Returns true if the Accounts property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasAccounts() {
return accounts != null && !(accounts instanceof SdkAutoConstructList);
}
/**
*
* The names of the AWS accounts that you want to delete stack instances for.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasAccounts()} to see if a value was sent in this field.
*
*
* @return The names of the AWS accounts that you want to delete stack instances for.
*/
public List accounts() {
return accounts;
}
/**
* Returns true if the Regions property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasRegions() {
return regions != null && !(regions instanceof SdkAutoConstructList);
}
/**
*
* The regions where you want to delete stack set instances.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasRegions()} to see if a value was sent in this field.
*
*
* @return The regions where you want to delete stack set instances.
*/
public List regions() {
return regions;
}
/**
*
* Preferences for how AWS CloudFormation performs this stack set operation.
*
*
* @return Preferences for how AWS CloudFormation performs this stack set operation.
*/
public StackSetOperationPreferences operationPreferences() {
return operationPreferences;
}
/**
*
* Removes the stack instances from the specified stack set, but doesn't delete the stacks. You can't reassociate a
* retained stack or add an existing, saved stack to a new stack set.
*
*
* For more information, see Stack set operation options.
*
*
* @return Removes the stack instances from the specified stack set, but doesn't delete the stacks. You can't
* reassociate a retained stack or add an existing, saved stack to a new stack set.
*
* For more information, see Stack set operation options.
*/
public Boolean retainStacks() {
return retainStacks;
}
/**
*
* The unique identifier for this stack set operation.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the stack set
* operation only once, even if you retry the request multiple times. You can retry stack set operation requests to
* ensure that AWS CloudFormation successfully received them.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*
*
* @return The unique identifier for this stack set operation.
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the
* stack set operation only once, even if you retry the request multiple times. You can retry stack set
* operation requests to ensure that AWS CloudFormation successfully received them.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*/
public String operationId() {
return operationId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(stackSetName());
hashCode = 31 * hashCode + Objects.hashCode(accounts());
hashCode = 31 * hashCode + Objects.hashCode(regions());
hashCode = 31 * hashCode + Objects.hashCode(operationPreferences());
hashCode = 31 * hashCode + Objects.hashCode(retainStacks());
hashCode = 31 * hashCode + Objects.hashCode(operationId());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeleteStackInstancesRequest)) {
return false;
}
DeleteStackInstancesRequest other = (DeleteStackInstancesRequest) obj;
return Objects.equals(stackSetName(), other.stackSetName()) && Objects.equals(accounts(), other.accounts())
&& Objects.equals(regions(), other.regions())
&& Objects.equals(operationPreferences(), other.operationPreferences())
&& Objects.equals(retainStacks(), other.retainStacks()) && Objects.equals(operationId(), other.operationId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("DeleteStackInstancesRequest").add("StackSetName", stackSetName()).add("Accounts", accounts())
.add("Regions", regions()).add("OperationPreferences", operationPreferences())
.add("RetainStacks", retainStacks()).add("OperationId", operationId()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackSetName":
return Optional.ofNullable(clazz.cast(stackSetName()));
case "Accounts":
return Optional.ofNullable(clazz.cast(accounts()));
case "Regions":
return Optional.ofNullable(clazz.cast(regions()));
case "OperationPreferences":
return Optional.ofNullable(clazz.cast(operationPreferences()));
case "RetainStacks":
return Optional.ofNullable(clazz.cast(retainStacks()));
case "OperationId":
return Optional.ofNullable(clazz.cast(operationId()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* For more information, see Stack set operation options.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder retainStacks(Boolean retainStacks);
/**
*
* The unique identifier for this stack set operation.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the stack
* set operation only once, even if you retry the request multiple times. You can retry stack set operation
* requests to ensure that AWS CloudFormation successfully received them.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*
*
* @param operationId
* The unique identifier for this stack set operation.
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs
* the stack set operation only once, even if you retry the request multiple times. You can retry stack
* set operation requests to ensure that AWS CloudFormation successfully received them.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder operationId(String operationId);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends CloudFormationRequest.BuilderImpl implements Builder {
private String stackSetName;
private List accounts = DefaultSdkAutoConstructList.getInstance();
private List regions = DefaultSdkAutoConstructList.getInstance();
private StackSetOperationPreferences operationPreferences;
private Boolean retainStacks;
private String operationId;
private BuilderImpl() {
}
private BuilderImpl(DeleteStackInstancesRequest model) {
super(model);
stackSetName(model.stackSetName);
accounts(model.accounts);
regions(model.regions);
operationPreferences(model.operationPreferences);
retainStacks(model.retainStacks);
operationId(model.operationId);
}
public final String getStackSetName() {
return stackSetName;
}
@Override
public final Builder stackSetName(String stackSetName) {
this.stackSetName = stackSetName;
return this;
}
public final void setStackSetName(String stackSetName) {
this.stackSetName = stackSetName;
}
public final Collection getAccounts() {
return accounts;
}
@Override
public final Builder accounts(Collection accounts) {
this.accounts = AccountListCopier.copy(accounts);
return this;
}
@Override
@SafeVarargs
public final Builder accounts(String... accounts) {
accounts(Arrays.asList(accounts));
return this;
}
public final void setAccounts(Collection accounts) {
this.accounts = AccountListCopier.copy(accounts);
}
public final Collection getRegions() {
return regions;
}
@Override
public final Builder regions(Collection regions) {
this.regions = RegionListCopier.copy(regions);
return this;
}
@Override
@SafeVarargs
public final Builder regions(String... regions) {
regions(Arrays.asList(regions));
return this;
}
public final void setRegions(Collection regions) {
this.regions = RegionListCopier.copy(regions);
}
public final StackSetOperationPreferences.Builder getOperationPreferences() {
return operationPreferences != null ? operationPreferences.toBuilder() : null;
}
@Override
public final Builder operationPreferences(StackSetOperationPreferences operationPreferences) {
this.operationPreferences = operationPreferences;
return this;
}
public final void setOperationPreferences(StackSetOperationPreferences.BuilderImpl operationPreferences) {
this.operationPreferences = operationPreferences != null ? operationPreferences.build() : null;
}
public final Boolean getRetainStacks() {
return retainStacks;
}
@Override
public final Builder retainStacks(Boolean retainStacks) {
this.retainStacks = retainStacks;
return this;
}
public final void setRetainStacks(Boolean retainStacks) {
this.retainStacks = retainStacks;
}
public final String getOperationId() {
return operationId;
}
@Override
public final Builder operationId(String operationId) {
this.operationId = operationId;
return this;
}
public final void setOperationId(String operationId) {
this.operationId = operationId;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public DeleteStackInstancesRequest build() {
return new DeleteStackInstancesRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}