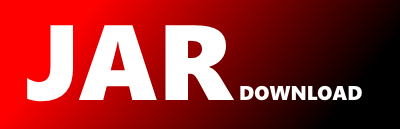
software.amazon.awssdk.services.cloudformation.model.DescribeChangeSetResponse Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The output for the DescribeChangeSet action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeChangeSetResponse extends CloudFormationResponse implements
ToCopyableBuilder {
private static final SdkField CHANGE_SET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeChangeSetResponse::changeSetName)).setter(setter(Builder::changeSetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChangeSetName").build()).build();
private static final SdkField CHANGE_SET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeChangeSetResponse::changeSetId)).setter(setter(Builder::changeSetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChangeSetId").build()).build();
private static final SdkField STACK_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeChangeSetResponse::stackId)).setter(setter(Builder::stackId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackId").build()).build();
private static final SdkField STACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeChangeSetResponse::stackName)).setter(setter(Builder::stackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackName").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeChangeSetResponse::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField> PARAMETERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(DescribeChangeSetResponse::parameters))
.setter(setter(Builder::parameters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Parameters").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Parameter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(DescribeChangeSetResponse::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField EXECUTION_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeChangeSetResponse::executionStatusAsString)).setter(setter(Builder::executionStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExecutionStatus").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeChangeSetResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField STATUS_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeChangeSetResponse::statusReason)).setter(setter(Builder::statusReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusReason").build()).build();
private static final SdkField> NOTIFICATION_AR_NS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(DescribeChangeSetResponse::notificationARNs))
.setter(setter(Builder::notificationARNs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationARNs").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ROLLBACK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(DescribeChangeSetResponse::rollbackConfiguration)).setter(setter(Builder::rollbackConfiguration))
.constructor(RollbackConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RollbackConfiguration").build())
.build();
private static final SdkField> CAPABILITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(DescribeChangeSetResponse::capabilitiesAsStrings))
.setter(setter(Builder::capabilitiesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Capabilities").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(DescribeChangeSetResponse::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CHANGES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(DescribeChangeSetResponse::changes))
.setter(setter(Builder::changes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Changes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Change::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DescribeChangeSetResponse::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextToken").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CHANGE_SET_NAME_FIELD,
CHANGE_SET_ID_FIELD, STACK_ID_FIELD, STACK_NAME_FIELD, DESCRIPTION_FIELD, PARAMETERS_FIELD, CREATION_TIME_FIELD,
EXECUTION_STATUS_FIELD, STATUS_FIELD, STATUS_REASON_FIELD, NOTIFICATION_AR_NS_FIELD, ROLLBACK_CONFIGURATION_FIELD,
CAPABILITIES_FIELD, TAGS_FIELD, CHANGES_FIELD, NEXT_TOKEN_FIELD));
private final String changeSetName;
private final String changeSetId;
private final String stackId;
private final String stackName;
private final String description;
private final List parameters;
private final Instant creationTime;
private final String executionStatus;
private final String status;
private final String statusReason;
private final List notificationARNs;
private final RollbackConfiguration rollbackConfiguration;
private final List capabilities;
private final List tags;
private final List changes;
private final String nextToken;
private DescribeChangeSetResponse(BuilderImpl builder) {
super(builder);
this.changeSetName = builder.changeSetName;
this.changeSetId = builder.changeSetId;
this.stackId = builder.stackId;
this.stackName = builder.stackName;
this.description = builder.description;
this.parameters = builder.parameters;
this.creationTime = builder.creationTime;
this.executionStatus = builder.executionStatus;
this.status = builder.status;
this.statusReason = builder.statusReason;
this.notificationARNs = builder.notificationARNs;
this.rollbackConfiguration = builder.rollbackConfiguration;
this.capabilities = builder.capabilities;
this.tags = builder.tags;
this.changes = builder.changes;
this.nextToken = builder.nextToken;
}
/**
*
* The name of the change set.
*
*
* @return The name of the change set.
*/
public String changeSetName() {
return changeSetName;
}
/**
*
* The ARN of the change set.
*
*
* @return The ARN of the change set.
*/
public String changeSetId() {
return changeSetId;
}
/**
*
* The ARN of the stack that is associated with the change set.
*
*
* @return The ARN of the stack that is associated with the change set.
*/
public String stackId() {
return stackId;
}
/**
*
* The name of the stack that is associated with the change set.
*
*
* @return The name of the stack that is associated with the change set.
*/
public String stackName() {
return stackName;
}
/**
*
* Information about the change set.
*
*
* @return Information about the change set.
*/
public String description() {
return description;
}
/**
* Returns true if the Parameters property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasParameters() {
return parameters != null && !(parameters instanceof SdkAutoConstructList);
}
/**
*
* A list of Parameter
structures that describes the input parameters and their values used to create
* the change set. For more information, see the Parameter data
* type.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasParameters()} to see if a value was sent in this field.
*
*
* @return A list of Parameter
structures that describes the input parameters and their values used to
* create the change set. For more information, see the Parameter
* data type.
*/
public List parameters() {
return parameters;
}
/**
*
* The start time when the change set was created, in UTC.
*
*
* @return The start time when the change set was created, in UTC.
*/
public Instant creationTime() {
return creationTime;
}
/**
*
* If the change set execution status is AVAILABLE
, you can execute the change set. If you can’t
* execute the change set, the status indicates why. For example, a change set might be in an
* UNAVAILABLE
state because AWS CloudFormation is still creating it or in an OBSOLETE
* state because the stack was already updated.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #executionStatus}
* will return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #executionStatusAsString}.
*
*
* @return If the change set execution status is AVAILABLE
, you can execute the change set. If you
* can’t execute the change set, the status indicates why. For example, a change set might be in an
* UNAVAILABLE
state because AWS CloudFormation is still creating it or in an
* OBSOLETE
state because the stack was already updated.
* @see ExecutionStatus
*/
public ExecutionStatus executionStatus() {
return ExecutionStatus.fromValue(executionStatus);
}
/**
*
* If the change set execution status is AVAILABLE
, you can execute the change set. If you can’t
* execute the change set, the status indicates why. For example, a change set might be in an
* UNAVAILABLE
state because AWS CloudFormation is still creating it or in an OBSOLETE
* state because the stack was already updated.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #executionStatus}
* will return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #executionStatusAsString}.
*
*
* @return If the change set execution status is AVAILABLE
, you can execute the change set. If you
* can’t execute the change set, the status indicates why. For example, a change set might be in an
* UNAVAILABLE
state because AWS CloudFormation is still creating it or in an
* OBSOLETE
state because the stack was already updated.
* @see ExecutionStatus
*/
public String executionStatusAsString() {
return executionStatus;
}
/**
*
* The current status of the change set, such as CREATE_IN_PROGRESS
, CREATE_COMPLETE
, or
* FAILED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ChangeSetStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current status of the change set, such as CREATE_IN_PROGRESS
,
* CREATE_COMPLETE
, or FAILED
.
* @see ChangeSetStatus
*/
public ChangeSetStatus status() {
return ChangeSetStatus.fromValue(status);
}
/**
*
* The current status of the change set, such as CREATE_IN_PROGRESS
, CREATE_COMPLETE
, or
* FAILED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ChangeSetStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current status of the change set, such as CREATE_IN_PROGRESS
,
* CREATE_COMPLETE
, or FAILED
.
* @see ChangeSetStatus
*/
public String statusAsString() {
return status;
}
/**
*
* A description of the change set's status. For example, if your attempt to create a change set failed, AWS
* CloudFormation shows the error message.
*
*
* @return A description of the change set's status. For example, if your attempt to create a change set failed, AWS
* CloudFormation shows the error message.
*/
public String statusReason() {
return statusReason;
}
/**
* Returns true if the NotificationARNs property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasNotificationARNs() {
return notificationARNs != null && !(notificationARNs instanceof SdkAutoConstructList);
}
/**
*
* The ARNs of the Amazon Simple Notification Service (Amazon SNS) topics that will be associated with the stack if
* you execute the change set.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasNotificationARNs()} to see if a value was sent in this field.
*
*
* @return The ARNs of the Amazon Simple Notification Service (Amazon SNS) topics that will be associated with the
* stack if you execute the change set.
*/
public List notificationARNs() {
return notificationARNs;
}
/**
*
* The rollback triggers for AWS CloudFormation to monitor during stack creation and updating operations, and for
* the specified monitoring period afterwards.
*
*
* @return The rollback triggers for AWS CloudFormation to monitor during stack creation and updating operations,
* and for the specified monitoring period afterwards.
*/
public RollbackConfiguration rollbackConfiguration() {
return rollbackConfiguration;
}
/**
*
* If you execute the change set, the list of capabilities that were explicitly acknowledged when the change set was
* created.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasCapabilities()} to see if a value was sent in this field.
*
*
* @return If you execute the change set, the list of capabilities that were explicitly acknowledged when the change
* set was created.
*/
public List capabilities() {
return CapabilitiesCopier.copyStringToEnum(capabilities);
}
/**
* Returns true if the Capabilities property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasCapabilities() {
return capabilities != null && !(capabilities instanceof SdkAutoConstructList);
}
/**
*
* If you execute the change set, the list of capabilities that were explicitly acknowledged when the change set was
* created.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasCapabilities()} to see if a value was sent in this field.
*
*
* @return If you execute the change set, the list of capabilities that were explicitly acknowledged when the change
* set was created.
*/
public List capabilitiesAsStrings() {
return capabilities;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* If you execute the change set, the tags that will be associated with the stack.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return If you execute the change set, the tags that will be associated with the stack.
*/
public List tags() {
return tags;
}
/**
* Returns true if the Changes property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasChanges() {
return changes != null && !(changes instanceof SdkAutoConstructList);
}
/**
*
* A list of Change
structures that describes the resources AWS CloudFormation changes if you execute
* the change set.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasChanges()} to see if a value was sent in this field.
*
*
* @return A list of Change
structures that describes the resources AWS CloudFormation changes if you
* execute the change set.
*/
public List changes() {
return changes;
}
/**
*
* If the output exceeds 1 MB, a string that identifies the next page of changes. If there is no additional page,
* this value is null.
*
*
* @return If the output exceeds 1 MB, a string that identifies the next page of changes. If there is no additional
* page, this value is null.
*/
public String nextToken() {
return nextToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(changeSetName());
hashCode = 31 * hashCode + Objects.hashCode(changeSetId());
hashCode = 31 * hashCode + Objects.hashCode(stackId());
hashCode = 31 * hashCode + Objects.hashCode(stackName());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(parameters());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(executionStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusReason());
hashCode = 31 * hashCode + Objects.hashCode(notificationARNs());
hashCode = 31 * hashCode + Objects.hashCode(rollbackConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(capabilitiesAsStrings());
hashCode = 31 * hashCode + Objects.hashCode(tags());
hashCode = 31 * hashCode + Objects.hashCode(changes());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeChangeSetResponse)) {
return false;
}
DescribeChangeSetResponse other = (DescribeChangeSetResponse) obj;
return Objects.equals(changeSetName(), other.changeSetName()) && Objects.equals(changeSetId(), other.changeSetId())
&& Objects.equals(stackId(), other.stackId()) && Objects.equals(stackName(), other.stackName())
&& Objects.equals(description(), other.description()) && Objects.equals(parameters(), other.parameters())
&& Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(executionStatusAsString(), other.executionStatusAsString())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(statusReason(), other.statusReason())
&& Objects.equals(notificationARNs(), other.notificationARNs())
&& Objects.equals(rollbackConfiguration(), other.rollbackConfiguration())
&& Objects.equals(capabilitiesAsStrings(), other.capabilitiesAsStrings()) && Objects.equals(tags(), other.tags())
&& Objects.equals(changes(), other.changes()) && Objects.equals(nextToken(), other.nextToken());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("DescribeChangeSetResponse").add("ChangeSetName", changeSetName())
.add("ChangeSetId", changeSetId()).add("StackId", stackId()).add("StackName", stackName())
.add("Description", description()).add("Parameters", parameters()).add("CreationTime", creationTime())
.add("ExecutionStatus", executionStatusAsString()).add("Status", statusAsString())
.add("StatusReason", statusReason()).add("NotificationARNs", notificationARNs())
.add("RollbackConfiguration", rollbackConfiguration()).add("Capabilities", capabilitiesAsStrings())
.add("Tags", tags()).add("Changes", changes()).add("NextToken", nextToken()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ChangeSetName":
return Optional.ofNullable(clazz.cast(changeSetName()));
case "ChangeSetId":
return Optional.ofNullable(clazz.cast(changeSetId()));
case "StackId":
return Optional.ofNullable(clazz.cast(stackId()));
case "StackName":
return Optional.ofNullable(clazz.cast(stackName()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Parameters":
return Optional.ofNullable(clazz.cast(parameters()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "ExecutionStatus":
return Optional.ofNullable(clazz.cast(executionStatusAsString()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "StatusReason":
return Optional.ofNullable(clazz.cast(statusReason()));
case "NotificationARNs":
return Optional.ofNullable(clazz.cast(notificationARNs()));
case "RollbackConfiguration":
return Optional.ofNullable(clazz.cast(rollbackConfiguration()));
case "Capabilities":
return Optional.ofNullable(clazz.cast(capabilitiesAsStrings()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "Changes":
return Optional.ofNullable(clazz.cast(changes()));
case "NextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function