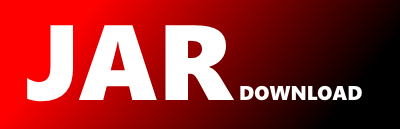
software.amazon.awssdk.services.cloudformation.model.UpdateStackSetRequest Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateStackSetRequest extends CloudFormationRequest implements
ToCopyableBuilder {
private static final SdkField STACK_SET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateStackSetRequest::stackSetName)).setter(setter(Builder::stackSetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackSetName").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateStackSetRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField TEMPLATE_BODY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateStackSetRequest::templateBody)).setter(setter(Builder::templateBody))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateBody").build()).build();
private static final SdkField TEMPLATE_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateStackSetRequest::templateURL)).setter(setter(Builder::templateURL))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateURL").build()).build();
private static final SdkField USE_PREVIOUS_TEMPLATE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(UpdateStackSetRequest::usePreviousTemplate)).setter(setter(Builder::usePreviousTemplate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UsePreviousTemplate").build())
.build();
private static final SdkField> PARAMETERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(UpdateStackSetRequest::parameters))
.setter(setter(Builder::parameters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Parameters").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Parameter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CAPABILITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(UpdateStackSetRequest::capabilitiesAsStrings))
.setter(setter(Builder::capabilitiesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Capabilities").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(UpdateStackSetRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField OPERATION_PREFERENCES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(UpdateStackSetRequest::operationPreferences)).setter(setter(Builder::operationPreferences))
.constructor(StackSetOperationPreferences::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OperationPreferences").build())
.build();
private static final SdkField ADMINISTRATION_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateStackSetRequest::administrationRoleARN)).setter(setter(Builder::administrationRoleARN))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdministrationRoleARN").build())
.build();
private static final SdkField EXECUTION_ROLE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateStackSetRequest::executionRoleName)).setter(setter(Builder::executionRoleName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExecutionRoleName").build()).build();
private static final SdkField DEPLOYMENT_TARGETS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(UpdateStackSetRequest::deploymentTargets))
.setter(setter(Builder::deploymentTargets)).constructor(DeploymentTargets::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeploymentTargets").build()).build();
private static final SdkField PERMISSION_MODEL_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(UpdateStackSetRequest::permissionModelAsString)).setter(setter(Builder::permissionModel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PermissionModel").build()).build();
private static final SdkField AUTO_DEPLOYMENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(UpdateStackSetRequest::autoDeployment))
.setter(setter(Builder::autoDeployment)).constructor(AutoDeployment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoDeployment").build()).build();
private static final SdkField OPERATION_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(UpdateStackSetRequest::operationId))
.setter(setter(Builder::operationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OperationId").build(),
DefaultValueTrait.idempotencyToken()).build();
private static final SdkField> ACCOUNTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(UpdateStackSetRequest::accounts))
.setter(setter(Builder::accounts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Accounts").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> REGIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(UpdateStackSetRequest::regions))
.setter(setter(Builder::regions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Regions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STACK_SET_NAME_FIELD,
DESCRIPTION_FIELD, TEMPLATE_BODY_FIELD, TEMPLATE_URL_FIELD, USE_PREVIOUS_TEMPLATE_FIELD, PARAMETERS_FIELD,
CAPABILITIES_FIELD, TAGS_FIELD, OPERATION_PREFERENCES_FIELD, ADMINISTRATION_ROLE_ARN_FIELD,
EXECUTION_ROLE_NAME_FIELD, DEPLOYMENT_TARGETS_FIELD, PERMISSION_MODEL_FIELD, AUTO_DEPLOYMENT_FIELD,
OPERATION_ID_FIELD, ACCOUNTS_FIELD, REGIONS_FIELD));
private final String stackSetName;
private final String description;
private final String templateBody;
private final String templateURL;
private final Boolean usePreviousTemplate;
private final List parameters;
private final List capabilities;
private final List tags;
private final StackSetOperationPreferences operationPreferences;
private final String administrationRoleARN;
private final String executionRoleName;
private final DeploymentTargets deploymentTargets;
private final String permissionModel;
private final AutoDeployment autoDeployment;
private final String operationId;
private final List accounts;
private final List regions;
private UpdateStackSetRequest(BuilderImpl builder) {
super(builder);
this.stackSetName = builder.stackSetName;
this.description = builder.description;
this.templateBody = builder.templateBody;
this.templateURL = builder.templateURL;
this.usePreviousTemplate = builder.usePreviousTemplate;
this.parameters = builder.parameters;
this.capabilities = builder.capabilities;
this.tags = builder.tags;
this.operationPreferences = builder.operationPreferences;
this.administrationRoleARN = builder.administrationRoleARN;
this.executionRoleName = builder.executionRoleName;
this.deploymentTargets = builder.deploymentTargets;
this.permissionModel = builder.permissionModel;
this.autoDeployment = builder.autoDeployment;
this.operationId = builder.operationId;
this.accounts = builder.accounts;
this.regions = builder.regions;
}
/**
*
* The name or unique ID of the stack set that you want to update.
*
*
* @return The name or unique ID of the stack set that you want to update.
*/
public String stackSetName() {
return stackSetName;
}
/**
*
* A brief description of updates that you are making.
*
*
* @return A brief description of updates that you are making.
*/
public String description() {
return description;
}
/**
*
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of 51,200
* bytes. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @return The structure that contains the template body, with a minimum length of 1 byte and a maximum length of
* 51,200 bytes. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*/
public String templateBody() {
return templateBody;
}
/**
*
* The location of the file that contains the template body. The URL must point to a template (maximum size: 460,800
* bytes) that is located in an Amazon S3 bucket. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @return The location of the file that contains the template body. The URL must point to a template (maximum size:
* 460,800 bytes) that is located in an Amazon S3 bucket. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*/
public String templateURL() {
return templateURL;
}
/**
*
* Use the existing template that's associated with the stack set that you're updating.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @return Use the existing template that's associated with the stack set that you're updating.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*/
public Boolean usePreviousTemplate() {
return usePreviousTemplate;
}
/**
* Returns true if the Parameters property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasParameters() {
return parameters != null && !(parameters instanceof SdkAutoConstructList);
}
/**
*
* A list of input parameters for the stack set template.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasParameters()} to see if a value was sent in this field.
*
*
* @return A list of input parameters for the stack set template.
*/
public List parameters() {
return parameters;
}
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in order
* for AWS CloudFormation to update the stack set and its associated stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stacks sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to update a
* stack directly from the processed template, without first reviewing the resulting changes in a change set, you
* must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasCapabilities()} to see if a value was sent in this field.
*
*
* @return In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for AWS CloudFormation to update the stack set and its associated stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for
* example, by creating new AWS Identity and Access Management (IAM) users. For those stacks sets, you must
* explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to
* update a stack directly from the processed template, without first reviewing the resulting changes in a
* change set, you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*/
public List capabilities() {
return CapabilitiesCopier.copyStringToEnum(capabilities);
}
/**
* Returns true if the Capabilities property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasCapabilities() {
return capabilities != null && !(capabilities instanceof SdkAutoConstructList);
}
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in order
* for AWS CloudFormation to update the stack set and its associated stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stacks sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to update a
* stack directly from the processed template, without first reviewing the resulting changes in a change set, you
* must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasCapabilities()} to see if a value was sent in this field.
*
*
* @return In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for AWS CloudFormation to update the stack set and its associated stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for
* example, by creating new AWS Identity and Access Management (IAM) users. For those stacks sets, you must
* explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to
* update a stack directly from the processed template, without first reviewing the resulting changes in a
* change set, you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*/
public List capabilitiesAsStrings() {
return capabilities;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. You can specify a maximum number of
* 50 tags.
*
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated with
* this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack set or
* during a previous update of the stack set.). Any tags that you don't include in the updated list of tags are
* removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to see if you
* have the required IAM permission to tag resources. If you omit tags that are currently associated with the stack
* set from the list of tags you specify, AWS CloudFormation assumes that you want to remove those tags from the
* stack set, and checks to see if you have permission to untag resources. If you don't have the necessary
* permission(s), the entire UpdateStackSet
action fails with an access denied
error, and
* the stack set is not updated.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation
* also propagates these tags to supported resources that are created in the stacks. You can specify a
* maximum number of 50 tags.
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated
* with this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack
* set or during a previous update of the stack set.). Any tags that you don't include in the updated list
* of tags are removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to
* see if you have the required IAM permission to tag resources. If you omit tags that are currently
* associated with the stack set from the list of tags you specify, AWS CloudFormation assumes that you want
* to remove those tags from the stack set, and checks to see if you have permission to untag resources. If
* you don't have the necessary permission(s), the entire UpdateStackSet
action fails with an
* access denied
error, and the stack set is not updated.
*/
public List tags() {
return tags;
}
/**
*
* Preferences for how AWS CloudFormation performs this stack set operation.
*
*
* @return Preferences for how AWS CloudFormation performs this stack set operation.
*/
public StackSetOperationPreferences operationPreferences() {
return operationPreferences;
}
/**
*
* The Amazon Resource Number (ARN) of the IAM role to use to update this stack set.
*
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups can
* manage specific stack sets within the same administrator account. For more information, see Granting Permissions
* for Stack Set Operations in the AWS CloudFormation User Guide.
*
*
* If you specified a customized administrator role when you created the stack set, you must specify a customized
* administrator role, even if it is the same customized administrator role used with this stack set previously.
*
*
* @return The Amazon Resource Number (ARN) of the IAM role to use to update this stack set.
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups
* can manage specific stack sets within the same administrator account. For more information, see Granting
* Permissions for Stack Set Operations in the AWS CloudFormation User Guide.
*
*
* If you specified a customized administrator role when you created the stack set, you must specify a
* customized administrator role, even if it is the same customized administrator role used with this stack
* set previously.
*/
public String administrationRoleARN() {
return administrationRoleARN;
}
/**
*
* The name of the IAM execution role to use to update the stack set. If you do not specify an execution role, AWS
* CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack set operation.
*
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources users and
* groups can include in their stack sets.
*
*
* If you specify a customized execution role, AWS CloudFormation uses that role to update the stack. If you do not
* specify a customized execution role, AWS CloudFormation performs the update using the role previously associated
* with the stack set, so long as you have permissions to perform operations on the stack set.
*
*
* @return The name of the IAM execution role to use to update the stack set. If you do not specify an execution
* role, AWS CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack
* set operation.
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources
* users and groups can include in their stack sets.
*
*
* If you specify a customized execution role, AWS CloudFormation uses that role to update the stack. If you
* do not specify a customized execution role, AWS CloudFormation performs the update using the role
* previously associated with the stack set, so long as you have permissions to perform operations on the
* stack set.
*/
public String executionRoleName() {
return executionRoleName;
}
/**
*
* [Service-managed
permissions] The AWS Organizations accounts in which to update associated stack
* instances.
*
*
* To update all the stack instances associated with this stack set, do not specify DeploymentTargets
* or Regions
.
*
*
* If the stack set update includes changes to the template (that is, if TemplateBody
or
* TemplateURL
is specified), or the Parameters
, AWS CloudFormation marks all stack
* instances with a status of OUTDATED
prior to updating the stack instances in the specified accounts
* and Regions. If the stack set update does not include changes to the template or parameters, AWS CloudFormation
* updates the stack instances in the specified accounts and Regions, while leaving all other stack instances with
* their existing stack instance status.
*
*
* @return [Service-managed
permissions] The AWS Organizations accounts in which to update associated
* stack instances.
*
* To update all the stack instances associated with this stack set, do not specify
* DeploymentTargets
or Regions
.
*
*
* If the stack set update includes changes to the template (that is, if TemplateBody
or
* TemplateURL
is specified), or the Parameters
, AWS CloudFormation marks all
* stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and Regions. If the stack set update does not include changes to the template or
* parameters, AWS CloudFormation updates the stack instances in the specified accounts and Regions, while
* leaving all other stack instances with their existing stack instance status.
*/
public DeploymentTargets deploymentTargets() {
return deploymentTargets;
}
/**
*
* Describes how the IAM roles required for stack set operations are created. You cannot modify
* PermissionModel
if there are stack instances associated with your stack set.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by AWS Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #permissionModel}
* will return {@link PermissionModels#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #permissionModelAsString}.
*
*
* @return Describes how the IAM roles required for stack set operations are created. You cannot modify
* PermissionModel
if there are stack instances associated with your stack set.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles
* required to deploy to target accounts. For more information, see Grant Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by AWS Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
*/
public PermissionModels permissionModel() {
return PermissionModels.fromValue(permissionModel);
}
/**
*
* Describes how the IAM roles required for stack set operations are created. You cannot modify
* PermissionModel
if there are stack instances associated with your stack set.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by AWS Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #permissionModel}
* will return {@link PermissionModels#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #permissionModelAsString}.
*
*
* @return Describes how the IAM roles required for stack set operations are created. You cannot modify
* PermissionModel
if there are stack instances associated with your stack set.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles
* required to deploy to target accounts. For more information, see Grant Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by AWS Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
*/
public String permissionModelAsString() {
return permissionModel;
}
/**
*
* [Service-managed
permissions] Describes whether StackSets automatically deploys to AWS Organizations
* accounts that are added to a target organization or organizational unit (OU).
*
*
* If you specify AutoDeployment
, do not specify DeploymentTargets
or Regions
* .
*
*
* @return [Service-managed
permissions] Describes whether StackSets automatically deploys to AWS
* Organizations accounts that are added to a target organization or organizational unit (OU).
*
* If you specify AutoDeployment
, do not specify DeploymentTargets
or
* Regions
.
*/
public AutoDeployment autoDeployment() {
return autoDeployment;
}
/**
*
* The unique ID for this stack set operation.
*
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the stack set
* operation only once, even if you retry the request multiple times. You might retry stack set operation requests
* to ensure that AWS CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, AWS CloudFormation generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*
*
* @return The unique ID for this stack set operation.
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the
* stack set operation only once, even if you retry the request multiple times. You might retry stack set
* operation requests to ensure that AWS CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, AWS CloudFormation generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*/
public String operationId() {
return operationId;
}
/**
* Returns true if the Accounts property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasAccounts() {
return accounts != null && !(accounts instanceof SdkAutoConstructList);
}
/**
*
* [Self-managed
permissions] The accounts in which to update associated stack instances. If you
* specify accounts, you must also specify the Regions in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the Accounts
* or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS CloudFormation
* marks all stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and Regions. If the stack set update does not include changes to the template or parameters,
* AWS CloudFormation updates the stack instances in the specified accounts and Regions, while leaving all other
* stack instances with their existing stack instance status.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasAccounts()} to see if a value was sent in this field.
*
*
* @return [Self-managed
permissions] The accounts in which to update associated stack instances. If
* you specify accounts, you must also specify the Regions in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and Regions. If the stack set update does not include changes
* to the template or parameters, AWS CloudFormation updates the stack instances in the specified accounts
* and Regions, while leaving all other stack instances with their existing stack instance status.
*/
public List accounts() {
return accounts;
}
/**
* Returns true if the Regions property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasRegions() {
return regions != null && !(regions instanceof SdkAutoConstructList);
}
/**
*
* The Regions in which to update associated stack instances. If you specify Regions, you must also specify accounts
* in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the Accounts
* or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS CloudFormation
* marks all stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and Regions. If the stack set update does not include changes to the template or parameters,
* AWS CloudFormation updates the stack instances in the specified accounts and Regions, while leaving all other
* stack instances with their existing stack instance status.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasRegions()} to see if a value was sent in this field.
*
*
* @return The Regions in which to update associated stack instances. If you specify Regions, you must also specify
* accounts in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and Regions. If the stack set update does not include changes
* to the template or parameters, AWS CloudFormation updates the stack instances in the specified accounts
* and Regions, while leaving all other stack instances with their existing stack instance status.
*/
public List regions() {
return regions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(stackSetName());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(templateBody());
hashCode = 31 * hashCode + Objects.hashCode(templateURL());
hashCode = 31 * hashCode + Objects.hashCode(usePreviousTemplate());
hashCode = 31 * hashCode + Objects.hashCode(parameters());
hashCode = 31 * hashCode + Objects.hashCode(capabilitiesAsStrings());
hashCode = 31 * hashCode + Objects.hashCode(tags());
hashCode = 31 * hashCode + Objects.hashCode(operationPreferences());
hashCode = 31 * hashCode + Objects.hashCode(administrationRoleARN());
hashCode = 31 * hashCode + Objects.hashCode(executionRoleName());
hashCode = 31 * hashCode + Objects.hashCode(deploymentTargets());
hashCode = 31 * hashCode + Objects.hashCode(permissionModelAsString());
hashCode = 31 * hashCode + Objects.hashCode(autoDeployment());
hashCode = 31 * hashCode + Objects.hashCode(operationId());
hashCode = 31 * hashCode + Objects.hashCode(accounts());
hashCode = 31 * hashCode + Objects.hashCode(regions());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateStackSetRequest)) {
return false;
}
UpdateStackSetRequest other = (UpdateStackSetRequest) obj;
return Objects.equals(stackSetName(), other.stackSetName()) && Objects.equals(description(), other.description())
&& Objects.equals(templateBody(), other.templateBody()) && Objects.equals(templateURL(), other.templateURL())
&& Objects.equals(usePreviousTemplate(), other.usePreviousTemplate())
&& Objects.equals(parameters(), other.parameters())
&& Objects.equals(capabilitiesAsStrings(), other.capabilitiesAsStrings()) && Objects.equals(tags(), other.tags())
&& Objects.equals(operationPreferences(), other.operationPreferences())
&& Objects.equals(administrationRoleARN(), other.administrationRoleARN())
&& Objects.equals(executionRoleName(), other.executionRoleName())
&& Objects.equals(deploymentTargets(), other.deploymentTargets())
&& Objects.equals(permissionModelAsString(), other.permissionModelAsString())
&& Objects.equals(autoDeployment(), other.autoDeployment()) && Objects.equals(operationId(), other.operationId())
&& Objects.equals(accounts(), other.accounts()) && Objects.equals(regions(), other.regions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("UpdateStackSetRequest").add("StackSetName", stackSetName()).add("Description", description())
.add("TemplateBody", templateBody()).add("TemplateURL", templateURL())
.add("UsePreviousTemplate", usePreviousTemplate()).add("Parameters", parameters())
.add("Capabilities", capabilitiesAsStrings()).add("Tags", tags())
.add("OperationPreferences", operationPreferences()).add("AdministrationRoleARN", administrationRoleARN())
.add("ExecutionRoleName", executionRoleName()).add("DeploymentTargets", deploymentTargets())
.add("PermissionModel", permissionModelAsString()).add("AutoDeployment", autoDeployment())
.add("OperationId", operationId()).add("Accounts", accounts()).add("Regions", regions()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackSetName":
return Optional.ofNullable(clazz.cast(stackSetName()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "TemplateBody":
return Optional.ofNullable(clazz.cast(templateBody()));
case "TemplateURL":
return Optional.ofNullable(clazz.cast(templateURL()));
case "UsePreviousTemplate":
return Optional.ofNullable(clazz.cast(usePreviousTemplate()));
case "Parameters":
return Optional.ofNullable(clazz.cast(parameters()));
case "Capabilities":
return Optional.ofNullable(clazz.cast(capabilitiesAsStrings()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "OperationPreferences":
return Optional.ofNullable(clazz.cast(operationPreferences()));
case "AdministrationRoleARN":
return Optional.ofNullable(clazz.cast(administrationRoleARN()));
case "ExecutionRoleName":
return Optional.ofNullable(clazz.cast(executionRoleName()));
case "DeploymentTargets":
return Optional.ofNullable(clazz.cast(deploymentTargets()));
case "PermissionModel":
return Optional.ofNullable(clazz.cast(permissionModelAsString()));
case "AutoDeployment":
return Optional.ofNullable(clazz.cast(autoDeployment()));
case "OperationId":
return Optional.ofNullable(clazz.cast(operationId()));
case "Accounts":
return Optional.ofNullable(clazz.cast(accounts()));
case "Regions":
return Optional.ofNullable(clazz.cast(regions()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder templateBody(String templateBody);
/**
*
* The location of the file that contains the template body. The URL must point to a template (maximum size:
* 460,800 bytes) that is located in an Amazon S3 bucket. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @param templateURL
* The location of the file that contains the template body. The URL must point to a template (maximum
* size: 460,800 bytes) that is located in an Amazon S3 bucket. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder templateURL(String templateURL);
/**
*
* Use the existing template that's associated with the stack set that you're updating.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @param usePreviousTemplate
* Use the existing template that's associated with the stack set that you're updating.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder usePreviousTemplate(Boolean usePreviousTemplate);
/**
*
* A list of input parameters for the stack set template.
*
*
* @param parameters
* A list of input parameters for the stack set template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder parameters(Collection parameters);
/**
*
* A list of input parameters for the stack set template.
*
*
* @param parameters
* A list of input parameters for the stack set template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder parameters(Parameter... parameters);
/**
*
* A list of input parameters for the stack set template.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need to
* create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its
* result is passed to {@link #parameters(List)}.
*
* @param parameters
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #parameters(List)
*/
Builder parameters(Consumer... parameters);
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for AWS CloudFormation to update the stack set and its associated stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stacks sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to update a
* stack directly from the processed template, without first reviewing the resulting changes in a change set,
* you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities
* in order for AWS CloudFormation to update the stack set and its associated stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for
* example, by creating new AWS Identity and Access Management (IAM) users. For those stacks sets, you
* must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
* .
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions
* associated with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to
* update a stack directly from the processed template, without first reviewing the resulting changes in
* a change set, you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify
* this capability, if you include a macro in your template the stack set operation will fail.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilitiesWithStrings(Collection capabilities);
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for AWS CloudFormation to update the stack set and its associated stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stacks sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to update a
* stack directly from the processed template, without first reviewing the resulting changes in a change set,
* you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities
* in order for AWS CloudFormation to update the stack set and its associated stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for
* example, by creating new AWS Identity and Access Management (IAM) users. For those stacks sets, you
* must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
* .
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions
* associated with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to
* update a stack directly from the processed template, without first reviewing the resulting changes in
* a change set, you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify
* this capability, if you include a macro in your template the stack set operation will fail.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilitiesWithStrings(String... capabilities);
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for AWS CloudFormation to update the stack set and its associated stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stacks sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to update a
* stack directly from the processed template, without first reviewing the resulting changes in a change set,
* you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities
* in order for AWS CloudFormation to update the stack set and its associated stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for
* example, by creating new AWS Identity and Access Management (IAM) users. For those stacks sets, you
* must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
* .
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions
* associated with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to
* update a stack directly from the processed template, without first reviewing the resulting changes in
* a change set, you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify
* this capability, if you include a macro in your template the stack set operation will fail.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilities(Collection capabilities);
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for AWS CloudFormation to update the stack set and its associated stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stacks sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to update a
* stack directly from the processed template, without first reviewing the resulting changes in a change set,
* you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities
* in order for AWS CloudFormation to update the stack set and its associated stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for
* example, by creating new AWS Identity and Access Management (IAM) users. For those stacks sets, you
* must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
* .
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions
* associated with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to
* update a stack directly from the processed template, without first reviewing the resulting changes in
* a change set, you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify
* this capability, if you include a macro in your template the stack set operation will fail.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilities(Capability... capabilities);
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. You can specify a maximum number
* of 50 tags.
*
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated
* with this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack set or
* during a previous update of the stack set.). Any tags that you don't include in the updated list of tags are
* removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to see if
* you have the required IAM permission to tag resources. If you omit tags that are currently associated with
* the stack set from the list of tags you specify, AWS CloudFormation assumes that you want to remove those
* tags from the stack set, and checks to see if you have permission to untag resources. If you don't have the
* necessary permission(s), the entire UpdateStackSet
action fails with an
* access denied
error, and the stack set is not updated.
*
*
* @param tags
* The key-value pairs to associate with this stack set and the stacks created from it. AWS
* CloudFormation also propagates these tags to supported resources that are created in the stacks. You
* can specify a maximum number of 50 tags.
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently
* associated with this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you
* want associated with this stack set, even tags you've specifed before (for example, when creating the
* stack set or during a previous update of the stack set.). Any tags that you don't include in the
* updated list of tags are removed from the stack set, and therefore from the stacks and resources as
* well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to
* see if you have the required IAM permission to tag resources. If you omit tags that are currently
* associated with the stack set from the list of tags you specify, AWS CloudFormation assumes that you
* want to remove those tags from the stack set, and checks to see if you have permission to untag
* resources. If you don't have the necessary permission(s), the entire UpdateStackSet
* action fails with an access denied
error, and the stack set is not updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. You can specify a maximum number
* of 50 tags.
*
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated
* with this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack set or
* during a previous update of the stack set.). Any tags that you don't include in the updated list of tags are
* removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to see if
* you have the required IAM permission to tag resources. If you omit tags that are currently associated with
* the stack set from the list of tags you specify, AWS CloudFormation assumes that you want to remove those
* tags from the stack set, and checks to see if you have permission to untag resources. If you don't have the
* necessary permission(s), the entire UpdateStackSet
action fails with an
* access denied
error, and the stack set is not updated.
*
*
* @param tags
* The key-value pairs to associate with this stack set and the stacks created from it. AWS
* CloudFormation also propagates these tags to supported resources that are created in the stacks. You
* can specify a maximum number of 50 tags.
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently
* associated with this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you
* want associated with this stack set, even tags you've specifed before (for example, when creating the
* stack set or during a previous update of the stack set.). Any tags that you don't include in the
* updated list of tags are removed from the stack set, and therefore from the stacks and resources as
* well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to
* see if you have the required IAM permission to tag resources. If you omit tags that are currently
* associated with the stack set from the list of tags you specify, AWS CloudFormation assumes that you
* want to remove those tags from the stack set, and checks to see if you have permission to untag
* resources. If you don't have the necessary permission(s), the entire UpdateStackSet
* action fails with an access denied
error, and the stack set is not updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. You can specify a maximum number
* of 50 tags.
*
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated
* with this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack set or
* during a previous update of the stack set.). Any tags that you don't include in the updated list of tags are
* removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to see if
* you have the required IAM permission to tag resources. If you omit tags that are currently associated with
* the stack set from the list of tags you specify, AWS CloudFormation assumes that you want to remove those
* tags from the stack set, and checks to see if you have permission to untag resources. If you don't have the
* necessary permission(s), the entire UpdateStackSet
action fails with an
* access denied
error, and the stack set is not updated.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need to create
* one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its result
* is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(List)
*/
Builder tags(Consumer... tags);
/**
*
* Preferences for how AWS CloudFormation performs this stack set operation.
*
*
* @param operationPreferences
* Preferences for how AWS CloudFormation performs this stack set operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder operationPreferences(StackSetOperationPreferences operationPreferences);
/**
*
* Preferences for how AWS CloudFormation performs this stack set operation.
*
* This is a convenience that creates an instance of the {@link StackSetOperationPreferences.Builder} avoiding
* the need to create one manually via {@link StackSetOperationPreferences#builder()}.
*
* When the {@link Consumer} completes, {@link StackSetOperationPreferences.Builder#build()} is called
* immediately and its result is passed to {@link #operationPreferences(StackSetOperationPreferences)}.
*
* @param operationPreferences
* a consumer that will call methods on {@link StackSetOperationPreferences.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #operationPreferences(StackSetOperationPreferences)
*/
default Builder operationPreferences(Consumer operationPreferences) {
return operationPreferences(StackSetOperationPreferences.builder().applyMutation(operationPreferences).build());
}
/**
*
* The Amazon Resource Number (ARN) of the IAM role to use to update this stack set.
*
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups can
* manage specific stack sets within the same administrator account. For more information, see Granting
* Permissions for Stack Set Operations in the AWS CloudFormation User Guide.
*
*
* If you specified a customized administrator role when you created the stack set, you must specify a
* customized administrator role, even if it is the same customized administrator role used with this stack set
* previously.
*
*
* @param administrationRoleARN
* The Amazon Resource Number (ARN) of the IAM role to use to update this stack set.
*
* Specify an IAM role only if you are using customized administrator roles to control which users or
* groups can manage specific stack sets within the same administrator account. For more information, see
* Granting
* Permissions for Stack Set Operations in the AWS CloudFormation User Guide.
*
*
* If you specified a customized administrator role when you created the stack set, you must specify a
* customized administrator role, even if it is the same customized administrator role used with this
* stack set previously.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder administrationRoleARN(String administrationRoleARN);
/**
*
* The name of the IAM execution role to use to update the stack set. If you do not specify an execution role,
* AWS CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack set
* operation.
*
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources users
* and groups can include in their stack sets.
*
*
* If you specify a customized execution role, AWS CloudFormation uses that role to update the stack. If you do
* not specify a customized execution role, AWS CloudFormation performs the update using the role previously
* associated with the stack set, so long as you have permissions to perform operations on the stack set.
*
*
* @param executionRoleName
* The name of the IAM execution role to use to update the stack set. If you do not specify an execution
* role, AWS CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the
* stack set operation.
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources
* users and groups can include in their stack sets.
*
*
* If you specify a customized execution role, AWS CloudFormation uses that role to update the stack. If
* you do not specify a customized execution role, AWS CloudFormation performs the update using the role
* previously associated with the stack set, so long as you have permissions to perform operations on the
* stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder executionRoleName(String executionRoleName);
/**
*
* [Service-managed
permissions] The AWS Organizations accounts in which to update associated stack
* instances.
*
*
* To update all the stack instances associated with this stack set, do not specify
* DeploymentTargets
or Regions
.
*
*
* If the stack set update includes changes to the template (that is, if TemplateBody
or
* TemplateURL
is specified), or the Parameters
, AWS CloudFormation marks all stack
* instances with a status of OUTDATED
prior to updating the stack instances in the specified
* accounts and Regions. If the stack set update does not include changes to the template or parameters, AWS
* CloudFormation updates the stack instances in the specified accounts and Regions, while leaving all other
* stack instances with their existing stack instance status.
*
*
* @param deploymentTargets
* [Service-managed
permissions] The AWS Organizations accounts in which to update
* associated stack instances.
*
* To update all the stack instances associated with this stack set, do not specify
* DeploymentTargets
or Regions
.
*
*
* If the stack set update includes changes to the template (that is, if TemplateBody
or
* TemplateURL
is specified), or the Parameters
, AWS CloudFormation marks all
* stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and Regions. If the stack set update does not include changes to the template or
* parameters, AWS CloudFormation updates the stack instances in the specified accounts and Regions,
* while leaving all other stack instances with their existing stack instance status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder deploymentTargets(DeploymentTargets deploymentTargets);
/**
*
* [Service-managed
permissions] The AWS Organizations accounts in which to update associated stack
* instances.
*
*
* To update all the stack instances associated with this stack set, do not specify
* DeploymentTargets
or Regions
.
*
*
* If the stack set update includes changes to the template (that is, if TemplateBody
or
* TemplateURL
is specified), or the Parameters
, AWS CloudFormation marks all stack
* instances with a status of OUTDATED
prior to updating the stack instances in the specified
* accounts and Regions. If the stack set update does not include changes to the template or parameters, AWS
* CloudFormation updates the stack instances in the specified accounts and Regions, while leaving all other
* stack instances with their existing stack instance status.
*
* This is a convenience that creates an instance of the {@link DeploymentTargets.Builder} avoiding the need to
* create one manually via {@link DeploymentTargets#builder()}.
*
* When the {@link Consumer} completes, {@link DeploymentTargets.Builder#build()} is called immediately and its
* result is passed to {@link #deploymentTargets(DeploymentTargets)}.
*
* @param deploymentTargets
* a consumer that will call methods on {@link DeploymentTargets.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #deploymentTargets(DeploymentTargets)
*/
default Builder deploymentTargets(Consumer deploymentTargets) {
return deploymentTargets(DeploymentTargets.builder().applyMutation(deploymentTargets).build());
}
/**
*
* Describes how the IAM roles required for stack set operations are created. You cannot modify
* PermissionModel
if there are stack instances associated with your stack set.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by AWS Organizations. For more information, see Grant
* Service-Managed Stack Set Permissions.
*
*
*
*
* @param permissionModel
* Describes how the IAM roles required for stack set operations are created. You cannot modify
* PermissionModel
if there are stack instances associated with your stack set.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles
* required to deploy to target accounts. For more information, see Grant Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required
* to deploy to accounts managed by AWS Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
* @return Returns a reference to this object so that method calls can be chained together.
* @see PermissionModels
*/
Builder permissionModel(String permissionModel);
/**
*
* Describes how the IAM roles required for stack set operations are created. You cannot modify
* PermissionModel
if there are stack instances associated with your stack set.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by AWS Organizations. For more information, see Grant
* Service-Managed Stack Set Permissions.
*
*
*
*
* @param permissionModel
* Describes how the IAM roles required for stack set operations are created. You cannot modify
* PermissionModel
if there are stack instances associated with your stack set.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles
* required to deploy to target accounts. For more information, see Grant Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required
* to deploy to accounts managed by AWS Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
* @return Returns a reference to this object so that method calls can be chained together.
* @see PermissionModels
*/
Builder permissionModel(PermissionModels permissionModel);
/**
*
* [Service-managed
permissions] Describes whether StackSets automatically deploys to AWS
* Organizations accounts that are added to a target organization or organizational unit (OU).
*
*
* If you specify AutoDeployment
, do not specify DeploymentTargets
or
* Regions
.
*
*
* @param autoDeployment
* [Service-managed
permissions] Describes whether StackSets automatically deploys to AWS
* Organizations accounts that are added to a target organization or organizational unit (OU).
*
* If you specify AutoDeployment
, do not specify DeploymentTargets
or
* Regions
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder autoDeployment(AutoDeployment autoDeployment);
/**
*
* [Service-managed
permissions] Describes whether StackSets automatically deploys to AWS
* Organizations accounts that are added to a target organization or organizational unit (OU).
*
*
* If you specify AutoDeployment
, do not specify DeploymentTargets
or
* Regions
.
*
* This is a convenience that creates an instance of the {@link AutoDeployment.Builder} avoiding the need to
* create one manually via {@link AutoDeployment#builder()}.
*
* When the {@link Consumer} completes, {@link AutoDeployment.Builder#build()} is called immediately and its
* result is passed to {@link #autoDeployment(AutoDeployment)}.
*
* @param autoDeployment
* a consumer that will call methods on {@link AutoDeployment.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #autoDeployment(AutoDeployment)
*/
default Builder autoDeployment(Consumer autoDeployment) {
return autoDeployment(AutoDeployment.builder().applyMutation(autoDeployment).build());
}
/**
*
* The unique ID for this stack set operation.
*
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the stack
* set operation only once, even if you retry the request multiple times. You might retry stack set operation
* requests to ensure that AWS CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, AWS CloudFormation generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*
*
* @param operationId
* The unique ID for this stack set operation.
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs
* the stack set operation only once, even if you retry the request multiple times. You might retry stack
* set operation requests to ensure that AWS CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, AWS CloudFormation generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder operationId(String operationId);
/**
*
* [Self-managed
permissions] The accounts in which to update associated stack instances. If you
* specify accounts, you must also specify the Regions in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the stack
* instances in the specified accounts and Regions. If the stack set update does not include changes to the
* template or parameters, AWS CloudFormation updates the stack instances in the specified accounts and Regions,
* while leaving all other stack instances with their existing stack instance status.
*
*
* @param accounts
* [Self-managed
permissions] The accounts in which to update associated stack instances. If
* you specify accounts, you must also specify the Regions in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and Regions. If the stack set update does not include
* changes to the template or parameters, AWS CloudFormation updates the stack instances in the specified
* accounts and Regions, while leaving all other stack instances with their existing stack instance
* status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder accounts(Collection accounts);
/**
*
* [Self-managed
permissions] The accounts in which to update associated stack instances. If you
* specify accounts, you must also specify the Regions in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the stack
* instances in the specified accounts and Regions. If the stack set update does not include changes to the
* template or parameters, AWS CloudFormation updates the stack instances in the specified accounts and Regions,
* while leaving all other stack instances with their existing stack instance status.
*
*
* @param accounts
* [Self-managed
permissions] The accounts in which to update associated stack instances. If
* you specify accounts, you must also specify the Regions in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and Regions. If the stack set update does not include
* changes to the template or parameters, AWS CloudFormation updates the stack instances in the specified
* accounts and Regions, while leaving all other stack instances with their existing stack instance
* status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder accounts(String... accounts);
/**
*
* The Regions in which to update associated stack instances. If you specify Regions, you must also specify
* accounts in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the stack
* instances in the specified accounts and Regions. If the stack set update does not include changes to the
* template or parameters, AWS CloudFormation updates the stack instances in the specified accounts and Regions,
* while leaving all other stack instances with their existing stack instance status.
*
*
* @param regions
* The Regions in which to update associated stack instances. If you specify Regions, you must also
* specify accounts in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and Regions. If the stack set update does not include
* changes to the template or parameters, AWS CloudFormation updates the stack instances in the specified
* accounts and Regions, while leaving all other stack instances with their existing stack instance
* status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder regions(Collection regions);
/**
*
* The Regions in which to update associated stack instances. If you specify Regions, you must also specify
* accounts in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the stack
* instances in the specified accounts and Regions. If the stack set update does not include changes to the
* template or parameters, AWS CloudFormation updates the stack instances in the specified accounts and Regions,
* while leaving all other stack instances with their existing stack instance status.
*
*
* @param regions
* The Regions in which to update associated stack instances. If you specify Regions, you must also
* specify accounts in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and Regions. If the stack set update does not include
* changes to the template or parameters, AWS CloudFormation updates the stack instances in the specified
* accounts and Regions, while leaving all other stack instances with their existing stack instance
* status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder regions(String... regions);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends CloudFormationRequest.BuilderImpl implements Builder {
private String stackSetName;
private String description;
private String templateBody;
private String templateURL;
private Boolean usePreviousTemplate;
private List parameters = DefaultSdkAutoConstructList.getInstance();
private List capabilities = DefaultSdkAutoConstructList.getInstance();
private List tags = DefaultSdkAutoConstructList.getInstance();
private StackSetOperationPreferences operationPreferences;
private String administrationRoleARN;
private String executionRoleName;
private DeploymentTargets deploymentTargets;
private String permissionModel;
private AutoDeployment autoDeployment;
private String operationId;
private List accounts = DefaultSdkAutoConstructList.getInstance();
private List regions = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(UpdateStackSetRequest model) {
super(model);
stackSetName(model.stackSetName);
description(model.description);
templateBody(model.templateBody);
templateURL(model.templateURL);
usePreviousTemplate(model.usePreviousTemplate);
parameters(model.parameters);
capabilitiesWithStrings(model.capabilities);
tags(model.tags);
operationPreferences(model.operationPreferences);
administrationRoleARN(model.administrationRoleARN);
executionRoleName(model.executionRoleName);
deploymentTargets(model.deploymentTargets);
permissionModel(model.permissionModel);
autoDeployment(model.autoDeployment);
operationId(model.operationId);
accounts(model.accounts);
regions(model.regions);
}
public final String getStackSetName() {
return stackSetName;
}
@Override
public final Builder stackSetName(String stackSetName) {
this.stackSetName = stackSetName;
return this;
}
public final void setStackSetName(String stackSetName) {
this.stackSetName = stackSetName;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
public final String getTemplateBody() {
return templateBody;
}
@Override
public final Builder templateBody(String templateBody) {
this.templateBody = templateBody;
return this;
}
public final void setTemplateBody(String templateBody) {
this.templateBody = templateBody;
}
public final String getTemplateURL() {
return templateURL;
}
@Override
public final Builder templateURL(String templateURL) {
this.templateURL = templateURL;
return this;
}
public final void setTemplateURL(String templateURL) {
this.templateURL = templateURL;
}
public final Boolean getUsePreviousTemplate() {
return usePreviousTemplate;
}
@Override
public final Builder usePreviousTemplate(Boolean usePreviousTemplate) {
this.usePreviousTemplate = usePreviousTemplate;
return this;
}
public final void setUsePreviousTemplate(Boolean usePreviousTemplate) {
this.usePreviousTemplate = usePreviousTemplate;
}
public final Collection getParameters() {
return parameters != null ? parameters.stream().map(Parameter::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder parameters(Collection parameters) {
this.parameters = ParametersCopier.copy(parameters);
return this;
}
@Override
@SafeVarargs
public final Builder parameters(Parameter... parameters) {
parameters(Arrays.asList(parameters));
return this;
}
@Override
@SafeVarargs
public final Builder parameters(Consumer... parameters) {
parameters(Stream.of(parameters).map(c -> Parameter.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setParameters(Collection parameters) {
this.parameters = ParametersCopier.copyFromBuilder(parameters);
}
public final Collection getCapabilities() {
return capabilities;
}
@Override
public final Builder capabilitiesWithStrings(Collection capabilities) {
this.capabilities = CapabilitiesCopier.copy(capabilities);
return this;
}
@Override
@SafeVarargs
public final Builder capabilitiesWithStrings(String... capabilities) {
capabilitiesWithStrings(Arrays.asList(capabilities));
return this;
}
@Override
public final Builder capabilities(Collection capabilities) {
this.capabilities = CapabilitiesCopier.copyEnumToString(capabilities);
return this;
}
@Override
@SafeVarargs
public final Builder capabilities(Capability... capabilities) {
capabilities(Arrays.asList(capabilities));
return this;
}
public final void setCapabilities(Collection capabilities) {
this.capabilities = CapabilitiesCopier.copy(capabilities);
}
public final Collection getTags() {
return tags != null ? tags.stream().map(Tag::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagsCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setTags(Collection tags) {
this.tags = TagsCopier.copyFromBuilder(tags);
}
public final StackSetOperationPreferences.Builder getOperationPreferences() {
return operationPreferences != null ? operationPreferences.toBuilder() : null;
}
@Override
public final Builder operationPreferences(StackSetOperationPreferences operationPreferences) {
this.operationPreferences = operationPreferences;
return this;
}
public final void setOperationPreferences(StackSetOperationPreferences.BuilderImpl operationPreferences) {
this.operationPreferences = operationPreferences != null ? operationPreferences.build() : null;
}
public final String getAdministrationRoleARN() {
return administrationRoleARN;
}
@Override
public final Builder administrationRoleARN(String administrationRoleARN) {
this.administrationRoleARN = administrationRoleARN;
return this;
}
public final void setAdministrationRoleARN(String administrationRoleARN) {
this.administrationRoleARN = administrationRoleARN;
}
public final String getExecutionRoleName() {
return executionRoleName;
}
@Override
public final Builder executionRoleName(String executionRoleName) {
this.executionRoleName = executionRoleName;
return this;
}
public final void setExecutionRoleName(String executionRoleName) {
this.executionRoleName = executionRoleName;
}
public final DeploymentTargets.Builder getDeploymentTargets() {
return deploymentTargets != null ? deploymentTargets.toBuilder() : null;
}
@Override
public final Builder deploymentTargets(DeploymentTargets deploymentTargets) {
this.deploymentTargets = deploymentTargets;
return this;
}
public final void setDeploymentTargets(DeploymentTargets.BuilderImpl deploymentTargets) {
this.deploymentTargets = deploymentTargets != null ? deploymentTargets.build() : null;
}
public final String getPermissionModel() {
return permissionModel;
}
@Override
public final Builder permissionModel(String permissionModel) {
this.permissionModel = permissionModel;
return this;
}
@Override
public final Builder permissionModel(PermissionModels permissionModel) {
this.permissionModel(permissionModel == null ? null : permissionModel.toString());
return this;
}
public final void setPermissionModel(String permissionModel) {
this.permissionModel = permissionModel;
}
public final AutoDeployment.Builder getAutoDeployment() {
return autoDeployment != null ? autoDeployment.toBuilder() : null;
}
@Override
public final Builder autoDeployment(AutoDeployment autoDeployment) {
this.autoDeployment = autoDeployment;
return this;
}
public final void setAutoDeployment(AutoDeployment.BuilderImpl autoDeployment) {
this.autoDeployment = autoDeployment != null ? autoDeployment.build() : null;
}
public final String getOperationId() {
return operationId;
}
@Override
public final Builder operationId(String operationId) {
this.operationId = operationId;
return this;
}
public final void setOperationId(String operationId) {
this.operationId = operationId;
}
public final Collection getAccounts() {
return accounts;
}
@Override
public final Builder accounts(Collection accounts) {
this.accounts = AccountListCopier.copy(accounts);
return this;
}
@Override
@SafeVarargs
public final Builder accounts(String... accounts) {
accounts(Arrays.asList(accounts));
return this;
}
public final void setAccounts(Collection accounts) {
this.accounts = AccountListCopier.copy(accounts);
}
public final Collection getRegions() {
return regions;
}
@Override
public final Builder regions(Collection regions) {
this.regions = RegionListCopier.copy(regions);
return this;
}
@Override
@SafeVarargs
public final Builder regions(String... regions) {
regions(Arrays.asList(regions));
return this;
}
public final void setRegions(Collection regions) {
this.regions = RegionListCopier.copy(regions);
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public UpdateStackSetRequest build() {
return new UpdateStackSetRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}