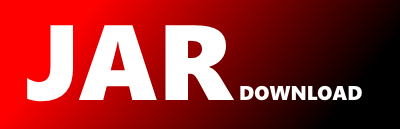
software.amazon.awssdk.services.cloudformation.CloudFormationClient Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.cloudformation.model.AlreadyExistsException;
import software.amazon.awssdk.services.cloudformation.model.CancelUpdateStackRequest;
import software.amazon.awssdk.services.cloudformation.model.CancelUpdateStackResponse;
import software.amazon.awssdk.services.cloudformation.model.CfnRegistryException;
import software.amazon.awssdk.services.cloudformation.model.ChangeSetNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.CloudFormationException;
import software.amazon.awssdk.services.cloudformation.model.ContinueUpdateRollbackRequest;
import software.amazon.awssdk.services.cloudformation.model.ContinueUpdateRollbackResponse;
import software.amazon.awssdk.services.cloudformation.model.CreateChangeSetRequest;
import software.amazon.awssdk.services.cloudformation.model.CreateChangeSetResponse;
import software.amazon.awssdk.services.cloudformation.model.CreateStackInstancesRequest;
import software.amazon.awssdk.services.cloudformation.model.CreateStackInstancesResponse;
import software.amazon.awssdk.services.cloudformation.model.CreateStackRequest;
import software.amazon.awssdk.services.cloudformation.model.CreateStackResponse;
import software.amazon.awssdk.services.cloudformation.model.CreateStackSetRequest;
import software.amazon.awssdk.services.cloudformation.model.CreateStackSetResponse;
import software.amazon.awssdk.services.cloudformation.model.CreatedButModifiedException;
import software.amazon.awssdk.services.cloudformation.model.DeleteChangeSetRequest;
import software.amazon.awssdk.services.cloudformation.model.DeleteChangeSetResponse;
import software.amazon.awssdk.services.cloudformation.model.DeleteStackInstancesRequest;
import software.amazon.awssdk.services.cloudformation.model.DeleteStackInstancesResponse;
import software.amazon.awssdk.services.cloudformation.model.DeleteStackRequest;
import software.amazon.awssdk.services.cloudformation.model.DeleteStackResponse;
import software.amazon.awssdk.services.cloudformation.model.DeleteStackSetRequest;
import software.amazon.awssdk.services.cloudformation.model.DeleteStackSetResponse;
import software.amazon.awssdk.services.cloudformation.model.DeregisterTypeRequest;
import software.amazon.awssdk.services.cloudformation.model.DeregisterTypeResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeChangeSetRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeChangeSetResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackDriftDetectionStatusRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackDriftDetectionStatusResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackEventsRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackEventsResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackInstanceRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackInstanceResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceDriftsRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceDriftsResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackResourcesRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackResourcesResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackSetOperationRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackSetOperationResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackSetRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackSetResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStacksRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStacksResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeTypeRegistrationRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeTypeRegistrationResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeTypeRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeTypeResponse;
import software.amazon.awssdk.services.cloudformation.model.DetectStackDriftRequest;
import software.amazon.awssdk.services.cloudformation.model.DetectStackDriftResponse;
import software.amazon.awssdk.services.cloudformation.model.DetectStackResourceDriftRequest;
import software.amazon.awssdk.services.cloudformation.model.DetectStackResourceDriftResponse;
import software.amazon.awssdk.services.cloudformation.model.DetectStackSetDriftRequest;
import software.amazon.awssdk.services.cloudformation.model.DetectStackSetDriftResponse;
import software.amazon.awssdk.services.cloudformation.model.EstimateTemplateCostRequest;
import software.amazon.awssdk.services.cloudformation.model.EstimateTemplateCostResponse;
import software.amazon.awssdk.services.cloudformation.model.ExecuteChangeSetRequest;
import software.amazon.awssdk.services.cloudformation.model.ExecuteChangeSetResponse;
import software.amazon.awssdk.services.cloudformation.model.GetStackPolicyRequest;
import software.amazon.awssdk.services.cloudformation.model.GetStackPolicyResponse;
import software.amazon.awssdk.services.cloudformation.model.GetTemplateRequest;
import software.amazon.awssdk.services.cloudformation.model.GetTemplateResponse;
import software.amazon.awssdk.services.cloudformation.model.GetTemplateSummaryRequest;
import software.amazon.awssdk.services.cloudformation.model.GetTemplateSummaryResponse;
import software.amazon.awssdk.services.cloudformation.model.InsufficientCapabilitiesException;
import software.amazon.awssdk.services.cloudformation.model.InvalidChangeSetStatusException;
import software.amazon.awssdk.services.cloudformation.model.InvalidOperationException;
import software.amazon.awssdk.services.cloudformation.model.InvalidStateTransitionException;
import software.amazon.awssdk.services.cloudformation.model.LimitExceededException;
import software.amazon.awssdk.services.cloudformation.model.ListChangeSetsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListChangeSetsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListExportsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListExportsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListImportsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListImportsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStackInstancesRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStackInstancesResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStackResourcesRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStackResourcesResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationResultsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationResultsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStacksRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStacksResponse;
import software.amazon.awssdk.services.cloudformation.model.ListTypeRegistrationsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListTypeRegistrationsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListTypeVersionsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListTypeVersionsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListTypesRequest;
import software.amazon.awssdk.services.cloudformation.model.ListTypesResponse;
import software.amazon.awssdk.services.cloudformation.model.NameAlreadyExistsException;
import software.amazon.awssdk.services.cloudformation.model.OperationIdAlreadyExistsException;
import software.amazon.awssdk.services.cloudformation.model.OperationInProgressException;
import software.amazon.awssdk.services.cloudformation.model.OperationNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.OperationStatusCheckFailedException;
import software.amazon.awssdk.services.cloudformation.model.RecordHandlerProgressRequest;
import software.amazon.awssdk.services.cloudformation.model.RecordHandlerProgressResponse;
import software.amazon.awssdk.services.cloudformation.model.RegisterTypeRequest;
import software.amazon.awssdk.services.cloudformation.model.RegisterTypeResponse;
import software.amazon.awssdk.services.cloudformation.model.SetStackPolicyRequest;
import software.amazon.awssdk.services.cloudformation.model.SetStackPolicyResponse;
import software.amazon.awssdk.services.cloudformation.model.SetTypeDefaultVersionRequest;
import software.amazon.awssdk.services.cloudformation.model.SetTypeDefaultVersionResponse;
import software.amazon.awssdk.services.cloudformation.model.SignalResourceRequest;
import software.amazon.awssdk.services.cloudformation.model.SignalResourceResponse;
import software.amazon.awssdk.services.cloudformation.model.StackInstanceNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.StackSetNotEmptyException;
import software.amazon.awssdk.services.cloudformation.model.StackSetNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.StaleRequestException;
import software.amazon.awssdk.services.cloudformation.model.StopStackSetOperationRequest;
import software.amazon.awssdk.services.cloudformation.model.StopStackSetOperationResponse;
import software.amazon.awssdk.services.cloudformation.model.TokenAlreadyExistsException;
import software.amazon.awssdk.services.cloudformation.model.TypeNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.UpdateStackInstancesRequest;
import software.amazon.awssdk.services.cloudformation.model.UpdateStackInstancesResponse;
import software.amazon.awssdk.services.cloudformation.model.UpdateStackRequest;
import software.amazon.awssdk.services.cloudformation.model.UpdateStackResponse;
import software.amazon.awssdk.services.cloudformation.model.UpdateStackSetRequest;
import software.amazon.awssdk.services.cloudformation.model.UpdateStackSetResponse;
import software.amazon.awssdk.services.cloudformation.model.UpdateTerminationProtectionRequest;
import software.amazon.awssdk.services.cloudformation.model.UpdateTerminationProtectionResponse;
import software.amazon.awssdk.services.cloudformation.model.ValidateTemplateRequest;
import software.amazon.awssdk.services.cloudformation.model.ValidateTemplateResponse;
import software.amazon.awssdk.services.cloudformation.paginators.DescribeAccountLimitsIterable;
import software.amazon.awssdk.services.cloudformation.paginators.DescribeStackEventsIterable;
import software.amazon.awssdk.services.cloudformation.paginators.DescribeStackResourceDriftsIterable;
import software.amazon.awssdk.services.cloudformation.paginators.DescribeStacksIterable;
import software.amazon.awssdk.services.cloudformation.paginators.ListChangeSetsIterable;
import software.amazon.awssdk.services.cloudformation.paginators.ListExportsIterable;
import software.amazon.awssdk.services.cloudformation.paginators.ListImportsIterable;
import software.amazon.awssdk.services.cloudformation.paginators.ListStackInstancesIterable;
import software.amazon.awssdk.services.cloudformation.paginators.ListStackResourcesIterable;
import software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationResultsIterable;
import software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationsIterable;
import software.amazon.awssdk.services.cloudformation.paginators.ListStackSetsIterable;
import software.amazon.awssdk.services.cloudformation.paginators.ListStacksIterable;
import software.amazon.awssdk.services.cloudformation.paginators.ListTypeRegistrationsIterable;
import software.amazon.awssdk.services.cloudformation.paginators.ListTypeVersionsIterable;
import software.amazon.awssdk.services.cloudformation.paginators.ListTypesIterable;
/**
* Service client for accessing AWS CloudFormation. This can be created using the static {@link #builder()} method.
*
* AWS CloudFormation
*
* AWS CloudFormation allows you to create and manage AWS infrastructure deployments predictably and repeatedly. You can
* use AWS CloudFormation to leverage AWS products, such as Amazon Elastic Compute Cloud, Amazon Elastic Block Store,
* Amazon Simple Notification Service, Elastic Load Balancing, and Auto Scaling to build highly-reliable, highly
* scalable, cost-effective applications without creating or configuring the underlying AWS infrastructure.
*
*
* With AWS CloudFormation, you declare all of your resources and dependencies in a template file. The template defines
* a collection of resources as a single unit called a stack. AWS CloudFormation creates and deletes all member
* resources of the stack together and manages all dependencies between the resources for you.
*
*
* For more information about AWS CloudFormation, see the AWS
* CloudFormation Product Page.
*
*
* Amazon CloudFormation makes use of other AWS products. If you need additional technical information about a specific
* AWS product, you can find the product's technical documentation at docs.aws.amazon.com.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface CloudFormationClient extends SdkClient {
String SERVICE_NAME = "cloudformation";
/**
* Create a {@link CloudFormationClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static CloudFormationClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link CloudFormationClient}.
*/
static CloudFormationClientBuilder builder() {
return new DefaultCloudFormationClientBuilder();
}
/**
*
* Cancels an update on the specified stack. If the call completes successfully, the stack rolls back the update and
* reverts to the previous stack configuration.
*
*
*
* You can cancel only stacks that are in the UPDATE_IN_PROGRESS state.
*
*
*
* @param cancelUpdateStackRequest
* The input for the CancelUpdateStack action.
* @return Result of the CancelUpdateStack operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.CancelUpdateStack
* @see AWS API Documentation
*/
default CancelUpdateStackResponse cancelUpdateStack(CancelUpdateStackRequest cancelUpdateStackRequest)
throws TokenAlreadyExistsException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Cancels an update on the specified stack. If the call completes successfully, the stack rolls back the update and
* reverts to the previous stack configuration.
*
*
*
* You can cancel only stacks that are in the UPDATE_IN_PROGRESS state.
*
*
*
* This is a convenience which creates an instance of the {@link CancelUpdateStackRequest.Builder} avoiding the need
* to create one manually via {@link CancelUpdateStackRequest#builder()}
*
*
* @param cancelUpdateStackRequest
* A {@link Consumer} that will call methods on {@link CancelUpdateStackInput.Builder} to create a request.
* The input for the CancelUpdateStack action.
* @return Result of the CancelUpdateStack operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.CancelUpdateStack
* @see AWS API Documentation
*/
default CancelUpdateStackResponse cancelUpdateStack(Consumer cancelUpdateStackRequest)
throws TokenAlreadyExistsException, AwsServiceException, SdkClientException, CloudFormationException {
return cancelUpdateStack(CancelUpdateStackRequest.builder().applyMutation(cancelUpdateStackRequest).build());
}
/**
*
* For a specified stack that is in the UPDATE_ROLLBACK_FAILED
state, continues rolling it back to the
* UPDATE_ROLLBACK_COMPLETE
state. Depending on the cause of the failure, you can manually fix the error and continue the rollback. By continuing the rollback, you can return your stack to a working
* state (the UPDATE_ROLLBACK_COMPLETE
state), and then try to update the stack again.
*
*
* A stack goes into the UPDATE_ROLLBACK_FAILED
state when AWS CloudFormation cannot roll back all
* changes after a failed stack update. For example, you might have a stack that is rolling back to an old database
* instance that was deleted outside of AWS CloudFormation. Because AWS CloudFormation doesn't know the database was
* deleted, it assumes that the database instance still exists and attempts to roll back to it, causing the update
* rollback to fail.
*
*
* @param continueUpdateRollbackRequest
* The input for the ContinueUpdateRollback action.
* @return Result of the ContinueUpdateRollback operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ContinueUpdateRollback
* @see AWS API Documentation
*/
default ContinueUpdateRollbackResponse continueUpdateRollback(ContinueUpdateRollbackRequest continueUpdateRollbackRequest)
throws TokenAlreadyExistsException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* For a specified stack that is in the UPDATE_ROLLBACK_FAILED
state, continues rolling it back to the
* UPDATE_ROLLBACK_COMPLETE
state. Depending on the cause of the failure, you can manually fix the error and continue the rollback. By continuing the rollback, you can return your stack to a working
* state (the UPDATE_ROLLBACK_COMPLETE
state), and then try to update the stack again.
*
*
* A stack goes into the UPDATE_ROLLBACK_FAILED
state when AWS CloudFormation cannot roll back all
* changes after a failed stack update. For example, you might have a stack that is rolling back to an old database
* instance that was deleted outside of AWS CloudFormation. Because AWS CloudFormation doesn't know the database was
* deleted, it assumes that the database instance still exists and attempts to roll back to it, causing the update
* rollback to fail.
*
*
*
* This is a convenience which creates an instance of the {@link ContinueUpdateRollbackRequest.Builder} avoiding the
* need to create one manually via {@link ContinueUpdateRollbackRequest#builder()}
*
*
* @param continueUpdateRollbackRequest
* A {@link Consumer} that will call methods on {@link ContinueUpdateRollbackInput.Builder} to create a
* request. The input for the ContinueUpdateRollback action.
* @return Result of the ContinueUpdateRollback operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ContinueUpdateRollback
* @see AWS API Documentation
*/
default ContinueUpdateRollbackResponse continueUpdateRollback(
Consumer continueUpdateRollbackRequest) throws TokenAlreadyExistsException,
AwsServiceException, SdkClientException, CloudFormationException {
return continueUpdateRollback(ContinueUpdateRollbackRequest.builder().applyMutation(continueUpdateRollbackRequest)
.build());
}
/**
*
* Creates a list of changes that will be applied to a stack so that you can review the changes before executing
* them. You can create a change set for a stack that doesn't exist or an existing stack. If you create a change set
* for a stack that doesn't exist, the change set shows all of the resources that AWS CloudFormation will create. If
* you create a change set for an existing stack, AWS CloudFormation compares the stack's information with the
* information that you submit in the change set and lists the differences. Use change sets to understand which
* resources AWS CloudFormation will create or change, and how it will change resources in an existing stack, before
* you create or update a stack.
*
*
* To create a change set for a stack that doesn't exist, for the ChangeSetType
parameter, specify
* CREATE
. To create a change set for an existing stack, specify UPDATE
for the
* ChangeSetType
parameter. To create a change set for an import operation, specify IMPORT
* for the ChangeSetType
parameter. After the CreateChangeSet
call successfully completes,
* AWS CloudFormation starts creating the change set. To check the status of the change set or to review it, use the
* DescribeChangeSet action.
*
*
* When you are satisfied with the changes the change set will make, execute the change set by using the
* ExecuteChangeSet action. AWS CloudFormation doesn't make changes until you execute the change set.
*
*
* @param createChangeSetRequest
* The input for the CreateChangeSet action.
* @return Result of the CreateChangeSet operation returned by the service.
* @throws AlreadyExistsException
* The resource with the name requested already exists.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information on resource and stack limitations, see Limits in the AWS CloudFormation User Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.CreateChangeSet
* @see AWS
* API Documentation
*/
default CreateChangeSetResponse createChangeSet(CreateChangeSetRequest createChangeSetRequest) throws AlreadyExistsException,
InsufficientCapabilitiesException, LimitExceededException, AwsServiceException, SdkClientException,
CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a list of changes that will be applied to a stack so that you can review the changes before executing
* them. You can create a change set for a stack that doesn't exist or an existing stack. If you create a change set
* for a stack that doesn't exist, the change set shows all of the resources that AWS CloudFormation will create. If
* you create a change set for an existing stack, AWS CloudFormation compares the stack's information with the
* information that you submit in the change set and lists the differences. Use change sets to understand which
* resources AWS CloudFormation will create or change, and how it will change resources in an existing stack, before
* you create or update a stack.
*
*
* To create a change set for a stack that doesn't exist, for the ChangeSetType
parameter, specify
* CREATE
. To create a change set for an existing stack, specify UPDATE
for the
* ChangeSetType
parameter. To create a change set for an import operation, specify IMPORT
* for the ChangeSetType
parameter. After the CreateChangeSet
call successfully completes,
* AWS CloudFormation starts creating the change set. To check the status of the change set or to review it, use the
* DescribeChangeSet action.
*
*
* When you are satisfied with the changes the change set will make, execute the change set by using the
* ExecuteChangeSet action. AWS CloudFormation doesn't make changes until you execute the change set.
*
*
*
* This is a convenience which creates an instance of the {@link CreateChangeSetRequest.Builder} avoiding the need
* to create one manually via {@link CreateChangeSetRequest#builder()}
*
*
* @param createChangeSetRequest
* A {@link Consumer} that will call methods on {@link CreateChangeSetInput.Builder} to create a request. The
* input for the CreateChangeSet action.
* @return Result of the CreateChangeSet operation returned by the service.
* @throws AlreadyExistsException
* The resource with the name requested already exists.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information on resource and stack limitations, see Limits in the AWS CloudFormation User Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.CreateChangeSet
* @see AWS
* API Documentation
*/
default CreateChangeSetResponse createChangeSet(Consumer createChangeSetRequest)
throws AlreadyExistsException, InsufficientCapabilitiesException, LimitExceededException, AwsServiceException,
SdkClientException, CloudFormationException {
return createChangeSet(CreateChangeSetRequest.builder().applyMutation(createChangeSetRequest).build());
}
/**
*
* Creates a stack as specified in the template. After the call completes successfully, the stack creation starts.
* You can check the status of the stack via the DescribeStacks API.
*
*
* @param createStackRequest
* The input for CreateStack action.
* @return Result of the CreateStack operation returned by the service.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information on resource and stack limitations, see Limits in the AWS CloudFormation User Guide.
* @throws AlreadyExistsException
* The resource with the name requested already exists.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.CreateStack
* @see AWS API
* Documentation
*/
default CreateStackResponse createStack(CreateStackRequest createStackRequest) throws LimitExceededException,
AlreadyExistsException, TokenAlreadyExistsException, InsufficientCapabilitiesException, AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a stack as specified in the template. After the call completes successfully, the stack creation starts.
* You can check the status of the stack via the DescribeStacks API.
*
*
*
* This is a convenience which creates an instance of the {@link CreateStackRequest.Builder} avoiding the need to
* create one manually via {@link CreateStackRequest#builder()}
*
*
* @param createStackRequest
* A {@link Consumer} that will call methods on {@link CreateStackInput.Builder} to create a request. The
* input for CreateStack action.
* @return Result of the CreateStack operation returned by the service.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information on resource and stack limitations, see Limits in the AWS CloudFormation User Guide.
* @throws AlreadyExistsException
* The resource with the name requested already exists.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.CreateStack
* @see AWS API
* Documentation
*/
default CreateStackResponse createStack(Consumer createStackRequest)
throws LimitExceededException, AlreadyExistsException, TokenAlreadyExistsException,
InsufficientCapabilitiesException, AwsServiceException, SdkClientException, CloudFormationException {
return createStack(CreateStackRequest.builder().applyMutation(createStackRequest).build());
}
/**
*
* Creates stack instances for the specified accounts, within the specified Regions. A stack instance refers to a
* stack in a specific account and Region. You must specify at least one value for either Accounts
or
* DeploymentTargets
, and you must specify at least one value for Regions
.
*
*
* @param createStackInstancesRequest
* @return Result of the CreateStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information on resource and stack limitations, see Limits in the AWS CloudFormation User Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.CreateStackInstances
* @see AWS API Documentation
*/
default CreateStackInstancesResponse createStackInstances(CreateStackInstancesRequest createStackInstancesRequest)
throws StackSetNotFoundException, OperationInProgressException, OperationIdAlreadyExistsException,
StaleRequestException, InvalidOperationException, LimitExceededException, AwsServiceException, SdkClientException,
CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Creates stack instances for the specified accounts, within the specified Regions. A stack instance refers to a
* stack in a specific account and Region. You must specify at least one value for either Accounts
or
* DeploymentTargets
, and you must specify at least one value for Regions
.
*
*
*
* This is a convenience which creates an instance of the {@link CreateStackInstancesRequest.Builder} avoiding the
* need to create one manually via {@link CreateStackInstancesRequest#builder()}
*
*
* @param createStackInstancesRequest
* A {@link Consumer} that will call methods on {@link CreateStackInstancesInput.Builder} to create a
* request.
* @return Result of the CreateStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information on resource and stack limitations, see Limits in the AWS CloudFormation User Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.CreateStackInstances
* @see AWS API Documentation
*/
default CreateStackInstancesResponse createStackInstances(
Consumer createStackInstancesRequest) throws StackSetNotFoundException,
OperationInProgressException, OperationIdAlreadyExistsException, StaleRequestException, InvalidOperationException,
LimitExceededException, AwsServiceException, SdkClientException, CloudFormationException {
return createStackInstances(CreateStackInstancesRequest.builder().applyMutation(createStackInstancesRequest).build());
}
/**
*
* Creates a stack set.
*
*
* @param createStackSetRequest
* @return Result of the CreateStackSet operation returned by the service.
* @throws NameAlreadyExistsException
* The specified name is already in use.
* @throws CreatedButModifiedException
* The specified resource exists, but has been changed.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information on resource and stack limitations, see Limits in the AWS CloudFormation User Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.CreateStackSet
* @see AWS
* API Documentation
*/
default CreateStackSetResponse createStackSet(CreateStackSetRequest createStackSetRequest) throws NameAlreadyExistsException,
CreatedButModifiedException, LimitExceededException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a stack set.
*
*
*
* This is a convenience which creates an instance of the {@link CreateStackSetRequest.Builder} avoiding the need to
* create one manually via {@link CreateStackSetRequest#builder()}
*
*
* @param createStackSetRequest
* A {@link Consumer} that will call methods on {@link CreateStackSetInput.Builder} to create a request.
* @return Result of the CreateStackSet operation returned by the service.
* @throws NameAlreadyExistsException
* The specified name is already in use.
* @throws CreatedButModifiedException
* The specified resource exists, but has been changed.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information on resource and stack limitations, see Limits in the AWS CloudFormation User Guide.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.CreateStackSet
* @see AWS
* API Documentation
*/
default CreateStackSetResponse createStackSet(Consumer createStackSetRequest)
throws NameAlreadyExistsException, CreatedButModifiedException, LimitExceededException, AwsServiceException,
SdkClientException, CloudFormationException {
return createStackSet(CreateStackSetRequest.builder().applyMutation(createStackSetRequest).build());
}
/**
*
* Deletes the specified change set. Deleting change sets ensures that no one executes the wrong change set.
*
*
* If the call successfully completes, AWS CloudFormation successfully deleted the change set.
*
*
* @param deleteChangeSetRequest
* The input for the DeleteChangeSet action.
* @return Result of the DeleteChangeSet operation returned by the service.
* @throws InvalidChangeSetStatusException
* The specified change set can't be used to update the stack. For example, the change set status might be
* CREATE_IN_PROGRESS
, or the stack status might be UPDATE_IN_PROGRESS
.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DeleteChangeSet
* @see AWS
* API Documentation
*/
default DeleteChangeSetResponse deleteChangeSet(DeleteChangeSetRequest deleteChangeSetRequest)
throws InvalidChangeSetStatusException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified change set. Deleting change sets ensures that no one executes the wrong change set.
*
*
* If the call successfully completes, AWS CloudFormation successfully deleted the change set.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteChangeSetRequest.Builder} avoiding the need
* to create one manually via {@link DeleteChangeSetRequest#builder()}
*
*
* @param deleteChangeSetRequest
* A {@link Consumer} that will call methods on {@link DeleteChangeSetInput.Builder} to create a request. The
* input for the DeleteChangeSet action.
* @return Result of the DeleteChangeSet operation returned by the service.
* @throws InvalidChangeSetStatusException
* The specified change set can't be used to update the stack. For example, the change set status might be
* CREATE_IN_PROGRESS
, or the stack status might be UPDATE_IN_PROGRESS
.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DeleteChangeSet
* @see AWS
* API Documentation
*/
default DeleteChangeSetResponse deleteChangeSet(Consumer deleteChangeSetRequest)
throws InvalidChangeSetStatusException, AwsServiceException, SdkClientException, CloudFormationException {
return deleteChangeSet(DeleteChangeSetRequest.builder().applyMutation(deleteChangeSetRequest).build());
}
/**
*
* Deletes a specified stack. Once the call completes successfully, stack deletion starts. Deleted stacks do not
* show up in the DescribeStacks API if the deletion has been completed successfully.
*
*
* @param deleteStackRequest
* The input for DeleteStack action.
* @return Result of the DeleteStack operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DeleteStack
* @see AWS API
* Documentation
*/
default DeleteStackResponse deleteStack(DeleteStackRequest deleteStackRequest) throws TokenAlreadyExistsException,
AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified stack. Once the call completes successfully, stack deletion starts. Deleted stacks do not
* show up in the DescribeStacks API if the deletion has been completed successfully.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteStackRequest.Builder} avoiding the need to
* create one manually via {@link DeleteStackRequest#builder()}
*
*
* @param deleteStackRequest
* A {@link Consumer} that will call methods on {@link DeleteStackInput.Builder} to create a request. The
* input for DeleteStack action.
* @return Result of the DeleteStack operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DeleteStack
* @see AWS API
* Documentation
*/
default DeleteStackResponse deleteStack(Consumer deleteStackRequest)
throws TokenAlreadyExistsException, AwsServiceException, SdkClientException, CloudFormationException {
return deleteStack(DeleteStackRequest.builder().applyMutation(deleteStackRequest).build());
}
/**
*
* Deletes stack instances for the specified accounts, in the specified Regions.
*
*
* @param deleteStackInstancesRequest
* @return Result of the DeleteStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DeleteStackInstances
* @see AWS API Documentation
*/
default DeleteStackInstancesResponse deleteStackInstances(DeleteStackInstancesRequest deleteStackInstancesRequest)
throws StackSetNotFoundException, OperationInProgressException, OperationIdAlreadyExistsException,
StaleRequestException, InvalidOperationException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes stack instances for the specified accounts, in the specified Regions.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteStackInstancesRequest.Builder} avoiding the
* need to create one manually via {@link DeleteStackInstancesRequest#builder()}
*
*
* @param deleteStackInstancesRequest
* A {@link Consumer} that will call methods on {@link DeleteStackInstancesInput.Builder} to create a
* request.
* @return Result of the DeleteStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DeleteStackInstances
* @see AWS API Documentation
*/
default DeleteStackInstancesResponse deleteStackInstances(
Consumer deleteStackInstancesRequest) throws StackSetNotFoundException,
OperationInProgressException, OperationIdAlreadyExistsException, StaleRequestException, InvalidOperationException,
AwsServiceException, SdkClientException, CloudFormationException {
return deleteStackInstances(DeleteStackInstancesRequest.builder().applyMutation(deleteStackInstancesRequest).build());
}
/**
*
* Deletes a stack set. Before you can delete a stack set, all of its member stack instances must be deleted. For
* more information about how to do this, see DeleteStackInstances.
*
*
* @param deleteStackSetRequest
* @return Result of the DeleteStackSet operation returned by the service.
* @throws StackSetNotEmptyException
* You can't yet delete this stack set, because it still contains one or more stack instances. Delete all
* stack instances from the stack set before deleting the stack set.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DeleteStackSet
* @see AWS
* API Documentation
*/
default DeleteStackSetResponse deleteStackSet(DeleteStackSetRequest deleteStackSetRequest) throws StackSetNotEmptyException,
OperationInProgressException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a stack set. Before you can delete a stack set, all of its member stack instances must be deleted. For
* more information about how to do this, see DeleteStackInstances.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteStackSetRequest.Builder} avoiding the need to
* create one manually via {@link DeleteStackSetRequest#builder()}
*
*
* @param deleteStackSetRequest
* A {@link Consumer} that will call methods on {@link DeleteStackSetInput.Builder} to create a request.
* @return Result of the DeleteStackSet operation returned by the service.
* @throws StackSetNotEmptyException
* You can't yet delete this stack set, because it still contains one or more stack instances. Delete all
* stack instances from the stack set before deleting the stack set.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DeleteStackSet
* @see AWS
* API Documentation
*/
default DeleteStackSetResponse deleteStackSet(Consumer deleteStackSetRequest)
throws StackSetNotEmptyException, OperationInProgressException, AwsServiceException, SdkClientException,
CloudFormationException {
return deleteStackSet(DeleteStackSetRequest.builder().applyMutation(deleteStackSetRequest).build());
}
/**
*
* Removes a type or type version from active use in the CloudFormation registry. If a type or type version is
* deregistered, it cannot be used in CloudFormation operations.
*
*
* To deregister a type, you must individually deregister all registered versions of that type. If a type has only a
* single registered version, deregistering that version results in the type itself being deregistered.
*
*
* You cannot deregister the default version of a type, unless it is the only registered version of that type, in
* which case the type itself is deregistered as well.
*
*
* @param deregisterTypeRequest
* @return Result of the DeregisterType operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified type does not exist in the CloudFormation registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DeregisterType
* @see AWS
* API Documentation
*/
default DeregisterTypeResponse deregisterType(DeregisterTypeRequest deregisterTypeRequest) throws CfnRegistryException,
TypeNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Removes a type or type version from active use in the CloudFormation registry. If a type or type version is
* deregistered, it cannot be used in CloudFormation operations.
*
*
* To deregister a type, you must individually deregister all registered versions of that type. If a type has only a
* single registered version, deregistering that version results in the type itself being deregistered.
*
*
* You cannot deregister the default version of a type, unless it is the only registered version of that type, in
* which case the type itself is deregistered as well.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterTypeRequest.Builder} avoiding the need to
* create one manually via {@link DeregisterTypeRequest#builder()}
*
*
* @param deregisterTypeRequest
* A {@link Consumer} that will call methods on {@link DeregisterTypeInput.Builder} to create a request.
* @return Result of the DeregisterType operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified type does not exist in the CloudFormation registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DeregisterType
* @see AWS
* API Documentation
*/
default DeregisterTypeResponse deregisterType(Consumer deregisterTypeRequest)
throws CfnRegistryException, TypeNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
return deregisterType(DeregisterTypeRequest.builder().applyMutation(deregisterTypeRequest).build());
}
/**
*
* Retrieves your account's AWS CloudFormation limits, such as the maximum number of stacks that you can create in
* your account. For more information about account limits, see AWS
* CloudFormation Limits in the AWS CloudFormation User Guide.
*
*
* @return Result of the DescribeAccountLimits operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeAccountLimits
* @see #describeAccountLimits(DescribeAccountLimitsRequest)
* @see AWS API Documentation
*/
default DescribeAccountLimitsResponse describeAccountLimits() throws AwsServiceException, SdkClientException,
CloudFormationException {
return describeAccountLimits(DescribeAccountLimitsRequest.builder().build());
}
/**
*
* Retrieves your account's AWS CloudFormation limits, such as the maximum number of stacks that you can create in
* your account. For more information about account limits, see AWS
* CloudFormation Limits in the AWS CloudFormation User Guide.
*
*
* @param describeAccountLimitsRequest
* The input for the DescribeAccountLimits action.
* @return Result of the DescribeAccountLimits operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeAccountLimits
* @see AWS API Documentation
*/
default DescribeAccountLimitsResponse describeAccountLimits(DescribeAccountLimitsRequest describeAccountLimitsRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves your account's AWS CloudFormation limits, such as the maximum number of stacks that you can create in
* your account. For more information about account limits, see AWS
* CloudFormation Limits in the AWS CloudFormation User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAccountLimitsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeAccountLimitsRequest#builder()}
*
*
* @param describeAccountLimitsRequest
* A {@link Consumer} that will call methods on {@link DescribeAccountLimitsInput.Builder} to create a
* request. The input for the DescribeAccountLimits action.
* @return Result of the DescribeAccountLimits operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeAccountLimits
* @see AWS API Documentation
*/
default DescribeAccountLimitsResponse describeAccountLimits(
Consumer describeAccountLimitsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return describeAccountLimits(DescribeAccountLimitsRequest.builder().applyMutation(describeAccountLimitsRequest).build());
}
/**
*
* Retrieves your account's AWS CloudFormation limits, such as the maximum number of stacks that you can create in
* your account. For more information about account limits, see AWS
* CloudFormation Limits in the AWS CloudFormation User Guide.
*
*
*
* This is a variant of
* {@link #describeAccountLimits(software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeAccountLimitsIterable responses = client.describeAccountLimitsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeAccountLimitsIterable responses = client
* .describeAccountLimitsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeAccountLimitsIterable responses = client.describeAccountLimitsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeAccountLimits(software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeAccountLimits
* @see #describeAccountLimitsPaginator(DescribeAccountLimitsRequest)
* @see AWS API Documentation
*/
default DescribeAccountLimitsIterable describeAccountLimitsPaginator() throws AwsServiceException, SdkClientException,
CloudFormationException {
return describeAccountLimitsPaginator(DescribeAccountLimitsRequest.builder().build());
}
/**
*
* Retrieves your account's AWS CloudFormation limits, such as the maximum number of stacks that you can create in
* your account. For more information about account limits, see AWS
* CloudFormation Limits in the AWS CloudFormation User Guide.
*
*
*
* This is a variant of
* {@link #describeAccountLimits(software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeAccountLimitsIterable responses = client.describeAccountLimitsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeAccountLimitsIterable responses = client
* .describeAccountLimitsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeAccountLimitsIterable responses = client.describeAccountLimitsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeAccountLimits(software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsRequest)}
* operation.
*
*
* @param describeAccountLimitsRequest
* The input for the DescribeAccountLimits action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeAccountLimits
* @see AWS API Documentation
*/
default DescribeAccountLimitsIterable describeAccountLimitsPaginator(DescribeAccountLimitsRequest describeAccountLimitsRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves your account's AWS CloudFormation limits, such as the maximum number of stacks that you can create in
* your account. For more information about account limits, see AWS
* CloudFormation Limits in the AWS CloudFormation User Guide.
*
*
*
* This is a variant of
* {@link #describeAccountLimits(software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeAccountLimitsIterable responses = client.describeAccountLimitsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeAccountLimitsIterable responses = client
* .describeAccountLimitsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeAccountLimitsIterable responses = client.describeAccountLimitsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeAccountLimits(software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeAccountLimitsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeAccountLimitsRequest#builder()}
*
*
* @param describeAccountLimitsRequest
* A {@link Consumer} that will call methods on {@link DescribeAccountLimitsInput.Builder} to create a
* request. The input for the DescribeAccountLimits action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeAccountLimits
* @see AWS API Documentation
*/
default DescribeAccountLimitsIterable describeAccountLimitsPaginator(
Consumer describeAccountLimitsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return describeAccountLimitsPaginator(DescribeAccountLimitsRequest.builder().applyMutation(describeAccountLimitsRequest)
.build());
}
/**
*
* Returns the inputs for the change set and a list of changes that AWS CloudFormation will make if you execute the
* change set. For more information, see Updating Stacks Using Change Sets in the AWS CloudFormation User Guide.
*
*
* @param describeChangeSetRequest
* The input for the DescribeChangeSet action.
* @return Result of the DescribeChangeSet operation returned by the service.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeChangeSet
* @see AWS API Documentation
*/
default DescribeChangeSetResponse describeChangeSet(DescribeChangeSetRequest describeChangeSetRequest)
throws ChangeSetNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the inputs for the change set and a list of changes that AWS CloudFormation will make if you execute the
* change set. For more information, see Updating Stacks Using Change Sets in the AWS CloudFormation User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeChangeSetRequest.Builder} avoiding the need
* to create one manually via {@link DescribeChangeSetRequest#builder()}
*
*
* @param describeChangeSetRequest
* A {@link Consumer} that will call methods on {@link DescribeChangeSetInput.Builder} to create a request.
* The input for the DescribeChangeSet action.
* @return Result of the DescribeChangeSet operation returned by the service.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeChangeSet
* @see AWS API Documentation
*/
default DescribeChangeSetResponse describeChangeSet(Consumer describeChangeSetRequest)
throws ChangeSetNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
return describeChangeSet(DescribeChangeSetRequest.builder().applyMutation(describeChangeSetRequest).build());
}
/**
*
* Returns information about a stack drift detection operation. A stack drift detection operation detects whether a
* stack's actual configuration differs, or has drifted, from it's expected configuration, as defined in the
* stack template and any values specified as template parameters. A stack is considered to have drifted if one or
* more of its resources have drifted. For more information on stack and resource drift, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackDrift to initiate a stack drift detection operation. DetectStackDrift
returns
* a StackDriftDetectionId
you can use to monitor the progress of the operation using
* DescribeStackDriftDetectionStatus
. Once the drift detection operation has completed, use
* DescribeStackResourceDrifts to return drift information about the stack and its resources.
*
*
* @param describeStackDriftDetectionStatusRequest
* @return Result of the DescribeStackDriftDetectionStatus operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackDriftDetectionStatus
* @see AWS API Documentation
*/
default DescribeStackDriftDetectionStatusResponse describeStackDriftDetectionStatus(
DescribeStackDriftDetectionStatusRequest describeStackDriftDetectionStatusRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a stack drift detection operation. A stack drift detection operation detects whether a
* stack's actual configuration differs, or has drifted, from it's expected configuration, as defined in the
* stack template and any values specified as template parameters. A stack is considered to have drifted if one or
* more of its resources have drifted. For more information on stack and resource drift, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackDrift to initiate a stack drift detection operation. DetectStackDrift
returns
* a StackDriftDetectionId
you can use to monitor the progress of the operation using
* DescribeStackDriftDetectionStatus
. Once the drift detection operation has completed, use
* DescribeStackResourceDrifts to return drift information about the stack and its resources.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStackDriftDetectionStatusRequest.Builder}
* avoiding the need to create one manually via {@link DescribeStackDriftDetectionStatusRequest#builder()}
*
*
* @param describeStackDriftDetectionStatusRequest
* A {@link Consumer} that will call methods on {@link DescribeStackDriftDetectionStatusInput.Builder} to
* create a request.
* @return Result of the DescribeStackDriftDetectionStatus operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackDriftDetectionStatus
* @see AWS API Documentation
*/
default DescribeStackDriftDetectionStatusResponse describeStackDriftDetectionStatus(
Consumer describeStackDriftDetectionStatusRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return describeStackDriftDetectionStatus(DescribeStackDriftDetectionStatusRequest.builder()
.applyMutation(describeStackDriftDetectionStatusRequest).build());
}
/**
*
* Returns all stack related events for a specified stack in reverse chronological order. For more information about
* a stack's event history, go to Stacks in the AWS
* CloudFormation User Guide.
*
*
*
* You can list events for stacks that have failed to create or have been deleted by specifying the unique stack
* identifier (stack ID).
*
*
*
* @param describeStackEventsRequest
* The input for DescribeStackEvents action.
* @return Result of the DescribeStackEvents operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackEvents
* @see AWS API Documentation
*/
default DescribeStackEventsResponse describeStackEvents(DescribeStackEventsRequest describeStackEventsRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns all stack related events for a specified stack in reverse chronological order. For more information about
* a stack's event history, go to Stacks in the AWS
* CloudFormation User Guide.
*
*
*
* You can list events for stacks that have failed to create or have been deleted by specifying the unique stack
* identifier (stack ID).
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStackEventsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeStackEventsRequest#builder()}
*
*
* @param describeStackEventsRequest
* A {@link Consumer} that will call methods on {@link DescribeStackEventsInput.Builder} to create a request.
* The input for DescribeStackEvents action.
* @return Result of the DescribeStackEvents operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackEvents
* @see AWS API Documentation
*/
default DescribeStackEventsResponse describeStackEvents(
Consumer describeStackEventsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return describeStackEvents(DescribeStackEventsRequest.builder().applyMutation(describeStackEventsRequest).build());
}
/**
*
* Returns all stack related events for a specified stack in reverse chronological order. For more information about
* a stack's event history, go to Stacks in the AWS
* CloudFormation User Guide.
*
*
*
* You can list events for stacks that have failed to create or have been deleted by specifying the unique stack
* identifier (stack ID).
*
*
*
* This is a variant of
* {@link #describeStackEvents(software.amazon.awssdk.services.cloudformation.model.DescribeStackEventsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStackEventsIterable responses = client.describeStackEventsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStackEventsIterable responses = client
* .describeStackEventsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.DescribeStackEventsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStackEventsIterable responses = client.describeStackEventsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeStackEvents(software.amazon.awssdk.services.cloudformation.model.DescribeStackEventsRequest)}
* operation.
*
*
* @param describeStackEventsRequest
* The input for DescribeStackEvents action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackEvents
* @see AWS API Documentation
*/
default DescribeStackEventsIterable describeStackEventsPaginator(DescribeStackEventsRequest describeStackEventsRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns all stack related events for a specified stack in reverse chronological order. For more information about
* a stack's event history, go to Stacks in the AWS
* CloudFormation User Guide.
*
*
*
* You can list events for stacks that have failed to create or have been deleted by specifying the unique stack
* identifier (stack ID).
*
*
*
* This is a variant of
* {@link #describeStackEvents(software.amazon.awssdk.services.cloudformation.model.DescribeStackEventsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStackEventsIterable responses = client.describeStackEventsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStackEventsIterable responses = client
* .describeStackEventsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.DescribeStackEventsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStackEventsIterable responses = client.describeStackEventsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeStackEvents(software.amazon.awssdk.services.cloudformation.model.DescribeStackEventsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeStackEventsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeStackEventsRequest#builder()}
*
*
* @param describeStackEventsRequest
* A {@link Consumer} that will call methods on {@link DescribeStackEventsInput.Builder} to create a request.
* The input for DescribeStackEvents action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackEvents
* @see AWS API Documentation
*/
default DescribeStackEventsIterable describeStackEventsPaginator(
Consumer describeStackEventsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return describeStackEventsPaginator(DescribeStackEventsRequest.builder().applyMutation(describeStackEventsRequest)
.build());
}
/**
*
* Returns the stack instance that's associated with the specified stack set, AWS account, and Region.
*
*
* For a list of stack instances that are associated with a specific stack set, use ListStackInstances.
*
*
* @param describeStackInstanceRequest
* @return Result of the DescribeStackInstance operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws StackInstanceNotFoundException
* The specified stack instance doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackInstance
* @see AWS API Documentation
*/
default DescribeStackInstanceResponse describeStackInstance(DescribeStackInstanceRequest describeStackInstanceRequest)
throws StackSetNotFoundException, StackInstanceNotFoundException, AwsServiceException, SdkClientException,
CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the stack instance that's associated with the specified stack set, AWS account, and Region.
*
*
* For a list of stack instances that are associated with a specific stack set, use ListStackInstances.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStackInstanceRequest.Builder} avoiding the
* need to create one manually via {@link DescribeStackInstanceRequest#builder()}
*
*
* @param describeStackInstanceRequest
* A {@link Consumer} that will call methods on {@link DescribeStackInstanceInput.Builder} to create a
* request.
* @return Result of the DescribeStackInstance operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws StackInstanceNotFoundException
* The specified stack instance doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackInstance
* @see AWS API Documentation
*/
default DescribeStackInstanceResponse describeStackInstance(
Consumer describeStackInstanceRequest) throws StackSetNotFoundException,
StackInstanceNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
return describeStackInstance(DescribeStackInstanceRequest.builder().applyMutation(describeStackInstanceRequest).build());
}
/**
*
* Returns a description of the specified resource in the specified stack.
*
*
* For deleted stacks, DescribeStackResource returns resource information for up to 90 days after the stack has been
* deleted.
*
*
* @param describeStackResourceRequest
* The input for DescribeStackResource action.
* @return Result of the DescribeStackResource operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackResource
* @see AWS API Documentation
*/
default DescribeStackResourceResponse describeStackResource(DescribeStackResourceRequest describeStackResourceRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a description of the specified resource in the specified stack.
*
*
* For deleted stacks, DescribeStackResource returns resource information for up to 90 days after the stack has been
* deleted.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStackResourceRequest.Builder} avoiding the
* need to create one manually via {@link DescribeStackResourceRequest#builder()}
*
*
* @param describeStackResourceRequest
* A {@link Consumer} that will call methods on {@link DescribeStackResourceInput.Builder} to create a
* request. The input for DescribeStackResource action.
* @return Result of the DescribeStackResource operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackResource
* @see AWS API Documentation
*/
default DescribeStackResourceResponse describeStackResource(
Consumer describeStackResourceRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return describeStackResource(DescribeStackResourceRequest.builder().applyMutation(describeStackResourceRequest).build());
}
/**
*
* Returns drift information for the resources that have been checked for drift in the specified stack. This
* includes actual and expected configuration values for resources where AWS CloudFormation detects configuration
* drift.
*
*
* For a given stack, there will be one StackResourceDrift
for each stack resource that has been
* checked for drift. Resources that have not yet been checked for drift are not included. Resources that do not
* currently support drift detection are not checked, and so not included. For a list of resources that support
* drift detection, see Resources that Support Drift Detection.
*
*
* Use DetectStackResourceDrift to detect drift on individual resources, or DetectStackDrift to detect
* drift on all supported resources for a given stack.
*
*
* @param describeStackResourceDriftsRequest
* @return Result of the DescribeStackResourceDrifts operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackResourceDrifts
* @see AWS API Documentation
*/
default DescribeStackResourceDriftsResponse describeStackResourceDrifts(
DescribeStackResourceDriftsRequest describeStackResourceDriftsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns drift information for the resources that have been checked for drift in the specified stack. This
* includes actual and expected configuration values for resources where AWS CloudFormation detects configuration
* drift.
*
*
* For a given stack, there will be one StackResourceDrift
for each stack resource that has been
* checked for drift. Resources that have not yet been checked for drift are not included. Resources that do not
* currently support drift detection are not checked, and so not included. For a list of resources that support
* drift detection, see Resources that Support Drift Detection.
*
*
* Use DetectStackResourceDrift to detect drift on individual resources, or DetectStackDrift to detect
* drift on all supported resources for a given stack.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStackResourceDriftsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeStackResourceDriftsRequest#builder()}
*
*
* @param describeStackResourceDriftsRequest
* A {@link Consumer} that will call methods on {@link DescribeStackResourceDriftsInput.Builder} to create a
* request.
* @return Result of the DescribeStackResourceDrifts operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackResourceDrifts
* @see AWS API Documentation
*/
default DescribeStackResourceDriftsResponse describeStackResourceDrifts(
Consumer describeStackResourceDriftsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return describeStackResourceDrifts(DescribeStackResourceDriftsRequest.builder()
.applyMutation(describeStackResourceDriftsRequest).build());
}
/**
*
* Returns drift information for the resources that have been checked for drift in the specified stack. This
* includes actual and expected configuration values for resources where AWS CloudFormation detects configuration
* drift.
*
*
* For a given stack, there will be one StackResourceDrift
for each stack resource that has been
* checked for drift. Resources that have not yet been checked for drift are not included. Resources that do not
* currently support drift detection are not checked, and so not included. For a list of resources that support
* drift detection, see Resources that Support Drift Detection.
*
*
* Use DetectStackResourceDrift to detect drift on individual resources, or DetectStackDrift to detect
* drift on all supported resources for a given stack.
*
*
*
* This is a variant of
* {@link #describeStackResourceDrifts(software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceDriftsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStackResourceDriftsIterable responses = client.describeStackResourceDriftsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStackResourceDriftsIterable responses = client
* .describeStackResourceDriftsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceDriftsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStackResourceDriftsIterable responses = client.describeStackResourceDriftsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeStackResourceDrifts(software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceDriftsRequest)}
* operation.
*
*
* @param describeStackResourceDriftsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackResourceDrifts
* @see AWS API Documentation
*/
default DescribeStackResourceDriftsIterable describeStackResourceDriftsPaginator(
DescribeStackResourceDriftsRequest describeStackResourceDriftsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns drift information for the resources that have been checked for drift in the specified stack. This
* includes actual and expected configuration values for resources where AWS CloudFormation detects configuration
* drift.
*
*
* For a given stack, there will be one StackResourceDrift
for each stack resource that has been
* checked for drift. Resources that have not yet been checked for drift are not included. Resources that do not
* currently support drift detection are not checked, and so not included. For a list of resources that support
* drift detection, see Resources that Support Drift Detection.
*
*
* Use DetectStackResourceDrift to detect drift on individual resources, or DetectStackDrift to detect
* drift on all supported resources for a given stack.
*
*
*
* This is a variant of
* {@link #describeStackResourceDrifts(software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceDriftsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStackResourceDriftsIterable responses = client.describeStackResourceDriftsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStackResourceDriftsIterable responses = client
* .describeStackResourceDriftsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceDriftsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStackResourceDriftsIterable responses = client.describeStackResourceDriftsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeStackResourceDrifts(software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceDriftsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeStackResourceDriftsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeStackResourceDriftsRequest#builder()}
*
*
* @param describeStackResourceDriftsRequest
* A {@link Consumer} that will call methods on {@link DescribeStackResourceDriftsInput.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackResourceDrifts
* @see AWS API Documentation
*/
default DescribeStackResourceDriftsIterable describeStackResourceDriftsPaginator(
Consumer describeStackResourceDriftsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return describeStackResourceDriftsPaginator(DescribeStackResourceDriftsRequest.builder()
.applyMutation(describeStackResourceDriftsRequest).build());
}
/**
*
* Returns AWS resource descriptions for running and deleted stacks. If StackName
is specified, all the
* associated resources that are part of the stack are returned. If PhysicalResourceId
is specified,
* the associated resources of the stack that the resource belongs to are returned.
*
*
*
* Only the first 100 resources will be returned. If your stack has more resources than this, you should use
* ListStackResources
instead.
*
*
*
* For deleted stacks, DescribeStackResources
returns resource information for up to 90 days after the
* stack has been deleted.
*
*
* You must specify either StackName
or PhysicalResourceId
, but not both. In addition, you
* can specify LogicalResourceId
to filter the returned result. For more information about resources,
* the LogicalResourceId
and PhysicalResourceId
, go to the AWS CloudFormation User Guide.
*
*
*
* A ValidationError
is returned if you specify both StackName
and
* PhysicalResourceId
in the same request.
*
*
*
* @param describeStackResourcesRequest
* The input for DescribeStackResources action.
* @return Result of the DescribeStackResources operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackResources
* @see AWS API Documentation
*/
default DescribeStackResourcesResponse describeStackResources(DescribeStackResourcesRequest describeStackResourcesRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns AWS resource descriptions for running and deleted stacks. If StackName
is specified, all the
* associated resources that are part of the stack are returned. If PhysicalResourceId
is specified,
* the associated resources of the stack that the resource belongs to are returned.
*
*
*
* Only the first 100 resources will be returned. If your stack has more resources than this, you should use
* ListStackResources
instead.
*
*
*
* For deleted stacks, DescribeStackResources
returns resource information for up to 90 days after the
* stack has been deleted.
*
*
* You must specify either StackName
or PhysicalResourceId
, but not both. In addition, you
* can specify LogicalResourceId
to filter the returned result. For more information about resources,
* the LogicalResourceId
and PhysicalResourceId
, go to the AWS CloudFormation User Guide.
*
*
*
* A ValidationError
is returned if you specify both StackName
and
* PhysicalResourceId
in the same request.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStackResourcesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeStackResourcesRequest#builder()}
*
*
* @param describeStackResourcesRequest
* A {@link Consumer} that will call methods on {@link DescribeStackResourcesInput.Builder} to create a
* request. The input for DescribeStackResources action.
* @return Result of the DescribeStackResources operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackResources
* @see AWS API Documentation
*/
default DescribeStackResourcesResponse describeStackResources(
Consumer describeStackResourcesRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return describeStackResources(DescribeStackResourcesRequest.builder().applyMutation(describeStackResourcesRequest)
.build());
}
/**
*
* Returns the description of the specified stack set.
*
*
* @param describeStackSetRequest
* @return Result of the DescribeStackSet operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackSet
* @see AWS API Documentation
*/
default DescribeStackSetResponse describeStackSet(DescribeStackSetRequest describeStackSetRequest)
throws StackSetNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the description of the specified stack set.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStackSetRequest.Builder} avoiding the need
* to create one manually via {@link DescribeStackSetRequest#builder()}
*
*
* @param describeStackSetRequest
* A {@link Consumer} that will call methods on {@link DescribeStackSetInput.Builder} to create a request.
* @return Result of the DescribeStackSet operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackSet
* @see AWS API Documentation
*/
default DescribeStackSetResponse describeStackSet(Consumer describeStackSetRequest)
throws StackSetNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
return describeStackSet(DescribeStackSetRequest.builder().applyMutation(describeStackSetRequest).build());
}
/**
*
* Returns the description of the specified stack set operation.
*
*
* @param describeStackSetOperationRequest
* @return Result of the DescribeStackSetOperation operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackSetOperation
* @see AWS API Documentation
*/
default DescribeStackSetOperationResponse describeStackSetOperation(
DescribeStackSetOperationRequest describeStackSetOperationRequest) throws StackSetNotFoundException,
OperationNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the description of the specified stack set operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStackSetOperationRequest.Builder} avoiding
* the need to create one manually via {@link DescribeStackSetOperationRequest#builder()}
*
*
* @param describeStackSetOperationRequest
* A {@link Consumer} that will call methods on {@link DescribeStackSetOperationInput.Builder} to create a
* request.
* @return Result of the DescribeStackSetOperation operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStackSetOperation
* @see AWS API Documentation
*/
default DescribeStackSetOperationResponse describeStackSetOperation(
Consumer describeStackSetOperationRequest)
throws StackSetNotFoundException, OperationNotFoundException, AwsServiceException, SdkClientException,
CloudFormationException {
return describeStackSetOperation(DescribeStackSetOperationRequest.builder()
.applyMutation(describeStackSetOperationRequest).build());
}
/**
*
* Returns the description for the specified stack; if no stack name was specified, then it returns the description
* for all the stacks created.
*
*
*
* If the stack does not exist, an AmazonCloudFormationException
is returned.
*
*
*
* @return Result of the DescribeStacks operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStacks
* @see #describeStacks(DescribeStacksRequest)
* @see AWS
* API Documentation
*/
default DescribeStacksResponse describeStacks() throws AwsServiceException, SdkClientException, CloudFormationException {
return describeStacks(DescribeStacksRequest.builder().build());
}
/**
*
* Returns the description for the specified stack; if no stack name was specified, then it returns the description
* for all the stacks created.
*
*
*
* If the stack does not exist, an AmazonCloudFormationException
is returned.
*
*
*
* @param describeStacksRequest
* The input for DescribeStacks action.
* @return Result of the DescribeStacks operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStacks
* @see AWS
* API Documentation
*/
default DescribeStacksResponse describeStacks(DescribeStacksRequest describeStacksRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the description for the specified stack; if no stack name was specified, then it returns the description
* for all the stacks created.
*
*
*
* If the stack does not exist, an AmazonCloudFormationException
is returned.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStacksRequest.Builder} avoiding the need to
* create one manually via {@link DescribeStacksRequest#builder()}
*
*
* @param describeStacksRequest
* A {@link Consumer} that will call methods on {@link DescribeStacksInput.Builder} to create a request. The
* input for DescribeStacks action.
* @return Result of the DescribeStacks operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStacks
* @see AWS
* API Documentation
*/
default DescribeStacksResponse describeStacks(Consumer describeStacksRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return describeStacks(DescribeStacksRequest.builder().applyMutation(describeStacksRequest).build());
}
/**
*
* Returns the description for the specified stack; if no stack name was specified, then it returns the description
* for all the stacks created.
*
*
*
* If the stack does not exist, an AmazonCloudFormationException
is returned.
*
*
*
* This is a variant of
* {@link #describeStacks(software.amazon.awssdk.services.cloudformation.model.DescribeStacksRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStacksIterable responses = client.describeStacksPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStacksIterable responses = client
* .describeStacksPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.DescribeStacksResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStacksIterable responses = client.describeStacksPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeStacks(software.amazon.awssdk.services.cloudformation.model.DescribeStacksRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStacks
* @see #describeStacksPaginator(DescribeStacksRequest)
* @see AWS
* API Documentation
*/
default DescribeStacksIterable describeStacksPaginator() throws AwsServiceException, SdkClientException,
CloudFormationException {
return describeStacksPaginator(DescribeStacksRequest.builder().build());
}
/**
*
* Returns the description for the specified stack; if no stack name was specified, then it returns the description
* for all the stacks created.
*
*
*
* If the stack does not exist, an AmazonCloudFormationException
is returned.
*
*
*
* This is a variant of
* {@link #describeStacks(software.amazon.awssdk.services.cloudformation.model.DescribeStacksRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStacksIterable responses = client.describeStacksPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStacksIterable responses = client
* .describeStacksPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.DescribeStacksResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStacksIterable responses = client.describeStacksPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeStacks(software.amazon.awssdk.services.cloudformation.model.DescribeStacksRequest)}
* operation.
*
*
* @param describeStacksRequest
* The input for DescribeStacks action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStacks
* @see AWS
* API Documentation
*/
default DescribeStacksIterable describeStacksPaginator(DescribeStacksRequest describeStacksRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the description for the specified stack; if no stack name was specified, then it returns the description
* for all the stacks created.
*
*
*
* If the stack does not exist, an AmazonCloudFormationException
is returned.
*
*
*
* This is a variant of
* {@link #describeStacks(software.amazon.awssdk.services.cloudformation.model.DescribeStacksRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStacksIterable responses = client.describeStacksPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStacksIterable responses = client
* .describeStacksPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.DescribeStacksResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.DescribeStacksIterable responses = client.describeStacksPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeStacks(software.amazon.awssdk.services.cloudformation.model.DescribeStacksRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeStacksRequest.Builder} avoiding the need to
* create one manually via {@link DescribeStacksRequest#builder()}
*
*
* @param describeStacksRequest
* A {@link Consumer} that will call methods on {@link DescribeStacksInput.Builder} to create a request. The
* input for DescribeStacks action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeStacks
* @see AWS
* API Documentation
*/
default DescribeStacksIterable describeStacksPaginator(Consumer describeStacksRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return describeStacksPaginator(DescribeStacksRequest.builder().applyMutation(describeStacksRequest).build());
}
/**
*
* Returns detailed information about a type that has been registered.
*
*
* If you specify a VersionId
, DescribeType
returns information about that specific type
* version. Otherwise, it returns information about the default type version.
*
*
* @param describeTypeRequest
* @return Result of the DescribeType operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified type does not exist in the CloudFormation registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeType
* @see AWS
* API Documentation
*/
default DescribeTypeResponse describeType(DescribeTypeRequest describeTypeRequest) throws CfnRegistryException,
TypeNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns detailed information about a type that has been registered.
*
*
* If you specify a VersionId
, DescribeType
returns information about that specific type
* version. Otherwise, it returns information about the default type version.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTypeRequest.Builder} avoiding the need to
* create one manually via {@link DescribeTypeRequest#builder()}
*
*
* @param describeTypeRequest
* A {@link Consumer} that will call methods on {@link DescribeTypeInput.Builder} to create a request.
* @return Result of the DescribeType operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified type does not exist in the CloudFormation registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeType
* @see AWS
* API Documentation
*/
default DescribeTypeResponse describeType(Consumer describeTypeRequest)
throws CfnRegistryException, TypeNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
return describeType(DescribeTypeRequest.builder().applyMutation(describeTypeRequest).build());
}
/**
*
* Returns information about a type's registration, including its current status and type and version identifiers.
*
*
* When you initiate a registration request using RegisterType
, you can then use
* DescribeTypeRegistration
to monitor the progress of that registration request.
*
*
* Once the registration request has completed, use DescribeType
to return detailed
* informaiton about a type.
*
*
* @param describeTypeRegistrationRequest
* @return Result of the DescribeTypeRegistration operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeTypeRegistration
* @see AWS API Documentation
*/
default DescribeTypeRegistrationResponse describeTypeRegistration(
DescribeTypeRegistrationRequest describeTypeRegistrationRequest) throws CfnRegistryException, AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a type's registration, including its current status and type and version identifiers.
*
*
* When you initiate a registration request using RegisterType
, you can then use
* DescribeTypeRegistration
to monitor the progress of that registration request.
*
*
* Once the registration request has completed, use DescribeType
to return detailed
* informaiton about a type.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTypeRegistrationRequest.Builder} avoiding
* the need to create one manually via {@link DescribeTypeRegistrationRequest#builder()}
*
*
* @param describeTypeRegistrationRequest
* A {@link Consumer} that will call methods on {@link DescribeTypeRegistrationInput.Builder} to create a
* request.
* @return Result of the DescribeTypeRegistration operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DescribeTypeRegistration
* @see AWS API Documentation
*/
default DescribeTypeRegistrationResponse describeTypeRegistration(
Consumer describeTypeRegistrationRequest) throws CfnRegistryException,
AwsServiceException, SdkClientException, CloudFormationException {
return describeTypeRegistration(DescribeTypeRegistrationRequest.builder().applyMutation(describeTypeRegistrationRequest)
.build());
}
/**
*
* Detects whether a stack's actual configuration differs, or has drifted, from it's expected configuration,
* as defined in the stack template and any values specified as template parameters. For each resource in the stack
* that supports drift detection, AWS CloudFormation compares the actual configuration of the resource with its
* expected template configuration. Only resource properties explicitly defined in the stack template are checked
* for drift. A stack is considered to have drifted if one or more of its resources differ from their expected
* template configurations. For more information, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackDrift
to detect drift on all supported resources for a given stack, or
* DetectStackResourceDrift to detect drift on individual resources.
*
*
* For a list of stack resources that currently support drift detection, see Resources that Support Drift Detection.
*
*
* DetectStackDrift
can take up to several minutes, depending on the number of resources contained
* within the stack. Use DescribeStackDriftDetectionStatus to monitor the progress of a detect stack drift
* operation. Once the drift detection operation has completed, use DescribeStackResourceDrifts to return
* drift information about the stack and its resources.
*
*
* When detecting drift on a stack, AWS CloudFormation does not detect drift on any nested stacks belonging to that
* stack. Perform DetectStackDrift
directly on the nested stack itself.
*
*
* @param detectStackDriftRequest
* @return Result of the DetectStackDrift operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DetectStackDrift
* @see AWS API Documentation
*/
default DetectStackDriftResponse detectStackDrift(DetectStackDriftRequest detectStackDriftRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Detects whether a stack's actual configuration differs, or has drifted, from it's expected configuration,
* as defined in the stack template and any values specified as template parameters. For each resource in the stack
* that supports drift detection, AWS CloudFormation compares the actual configuration of the resource with its
* expected template configuration. Only resource properties explicitly defined in the stack template are checked
* for drift. A stack is considered to have drifted if one or more of its resources differ from their expected
* template configurations. For more information, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackDrift
to detect drift on all supported resources for a given stack, or
* DetectStackResourceDrift to detect drift on individual resources.
*
*
* For a list of stack resources that currently support drift detection, see Resources that Support Drift Detection.
*
*
* DetectStackDrift
can take up to several minutes, depending on the number of resources contained
* within the stack. Use DescribeStackDriftDetectionStatus to monitor the progress of a detect stack drift
* operation. Once the drift detection operation has completed, use DescribeStackResourceDrifts to return
* drift information about the stack and its resources.
*
*
* When detecting drift on a stack, AWS CloudFormation does not detect drift on any nested stacks belonging to that
* stack. Perform DetectStackDrift
directly on the nested stack itself.
*
*
*
* This is a convenience which creates an instance of the {@link DetectStackDriftRequest.Builder} avoiding the need
* to create one manually via {@link DetectStackDriftRequest#builder()}
*
*
* @param detectStackDriftRequest
* A {@link Consumer} that will call methods on {@link DetectStackDriftInput.Builder} to create a request.
* @return Result of the DetectStackDrift operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DetectStackDrift
* @see AWS API Documentation
*/
default DetectStackDriftResponse detectStackDrift(Consumer detectStackDriftRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return detectStackDrift(DetectStackDriftRequest.builder().applyMutation(detectStackDriftRequest).build());
}
/**
*
* Returns information about whether a resource's actual configuration differs, or has drifted, from it's
* expected configuration, as defined in the stack template and any values specified as template parameters. This
* information includes actual and expected property values for resources in which AWS CloudFormation detects drift.
* Only resource properties explicitly defined in the stack template are checked for drift. For more information
* about stack and resource drift, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackResourceDrift
to detect drift on individual resources, or DetectStackDrift to
* detect drift on all resources in a given stack that support drift detection.
*
*
* Resources that do not currently support drift detection cannot be checked. For a list of resources that support
* drift detection, see Resources that Support Drift Detection.
*
*
* @param detectStackResourceDriftRequest
* @return Result of the DetectStackResourceDrift operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DetectStackResourceDrift
* @see AWS API Documentation
*/
default DetectStackResourceDriftResponse detectStackResourceDrift(
DetectStackResourceDriftRequest detectStackResourceDriftRequest) throws AwsServiceException, SdkClientException,
CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about whether a resource's actual configuration differs, or has drifted, from it's
* expected configuration, as defined in the stack template and any values specified as template parameters. This
* information includes actual and expected property values for resources in which AWS CloudFormation detects drift.
* Only resource properties explicitly defined in the stack template are checked for drift. For more information
* about stack and resource drift, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackResourceDrift
to detect drift on individual resources, or DetectStackDrift to
* detect drift on all resources in a given stack that support drift detection.
*
*
* Resources that do not currently support drift detection cannot be checked. For a list of resources that support
* drift detection, see Resources that Support Drift Detection.
*
*
*
* This is a convenience which creates an instance of the {@link DetectStackResourceDriftRequest.Builder} avoiding
* the need to create one manually via {@link DetectStackResourceDriftRequest#builder()}
*
*
* @param detectStackResourceDriftRequest
* A {@link Consumer} that will call methods on {@link DetectStackResourceDriftInput.Builder} to create a
* request.
* @return Result of the DetectStackResourceDrift operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DetectStackResourceDrift
* @see AWS API Documentation
*/
default DetectStackResourceDriftResponse detectStackResourceDrift(
Consumer detectStackResourceDriftRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return detectStackResourceDrift(DetectStackResourceDriftRequest.builder().applyMutation(detectStackResourceDriftRequest)
.build());
}
/**
*
* Detect drift on a stack set. When CloudFormation performs drift detection on a stack set, it performs drift
* detection on the stack associated with each stack instance in the stack set. For more information, see How CloudFormation
* Performs Drift Detection on a Stack Set.
*
*
* DetectStackSetDrift
returns the OperationId
of the stack set drift detection operation.
* Use this operation id with DescribeStackSetOperation
to monitor the progress of the drift
* detection operation. The drift detection operation may take some time, depending on the number of stack instances
* included in the stack set, as well as the number of resources included in each stack.
*
*
* Once the operation has completed, use the following actions to return drift information:
*
*
* -
*
* Use DescribeStackSet
to return detailed informaiton about the stack set, including detailed
* information about the last completed drift operation performed on the stack set. (Information about drift
* operations that are in progress is not included.)
*
*
* -
*
* Use ListStackInstances
to return a list of stack instances belonging to the stack set,
* including the drift status and last drift time checked of each instance.
*
*
* -
*
* Use DescribeStackInstance
to return detailed information about a specific stack instance,
* including its drift status and last drift time checked.
*
*
*
*
* For more information on performing a drift detection operation on a stack set, see Detecting Unmanaged
* Changes in Stack Sets.
*
*
* You can only run a single drift detection operation on a given stack set at one time.
*
*
* To stop a drift detection stack set operation, use StopStackSetOperation
.
*
*
* @param detectStackSetDriftRequest
* @return Result of the DetectStackSetDrift operation returned by the service.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DetectStackSetDrift
* @see AWS API Documentation
*/
default DetectStackSetDriftResponse detectStackSetDrift(DetectStackSetDriftRequest detectStackSetDriftRequest)
throws InvalidOperationException, OperationInProgressException, StackSetNotFoundException, AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Detect drift on a stack set. When CloudFormation performs drift detection on a stack set, it performs drift
* detection on the stack associated with each stack instance in the stack set. For more information, see How CloudFormation
* Performs Drift Detection on a Stack Set.
*
*
* DetectStackSetDrift
returns the OperationId
of the stack set drift detection operation.
* Use this operation id with DescribeStackSetOperation
to monitor the progress of the drift
* detection operation. The drift detection operation may take some time, depending on the number of stack instances
* included in the stack set, as well as the number of resources included in each stack.
*
*
* Once the operation has completed, use the following actions to return drift information:
*
*
* -
*
* Use DescribeStackSet
to return detailed informaiton about the stack set, including detailed
* information about the last completed drift operation performed on the stack set. (Information about drift
* operations that are in progress is not included.)
*
*
* -
*
* Use ListStackInstances
to return a list of stack instances belonging to the stack set,
* including the drift status and last drift time checked of each instance.
*
*
* -
*
* Use DescribeStackInstance
to return detailed information about a specific stack instance,
* including its drift status and last drift time checked.
*
*
*
*
* For more information on performing a drift detection operation on a stack set, see Detecting Unmanaged
* Changes in Stack Sets.
*
*
* You can only run a single drift detection operation on a given stack set at one time.
*
*
* To stop a drift detection stack set operation, use StopStackSetOperation
.
*
*
*
* This is a convenience which creates an instance of the {@link DetectStackSetDriftRequest.Builder} avoiding the
* need to create one manually via {@link DetectStackSetDriftRequest#builder()}
*
*
* @param detectStackSetDriftRequest
* A {@link Consumer} that will call methods on {@link DetectStackSetDriftInput.Builder} to create a request.
* @return Result of the DetectStackSetDrift operation returned by the service.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.DetectStackSetDrift
* @see AWS API Documentation
*/
default DetectStackSetDriftResponse detectStackSetDrift(
Consumer detectStackSetDriftRequest) throws InvalidOperationException,
OperationInProgressException, StackSetNotFoundException, AwsServiceException, SdkClientException,
CloudFormationException {
return detectStackSetDrift(DetectStackSetDriftRequest.builder().applyMutation(detectStackSetDriftRequest).build());
}
/**
*
* Returns the estimated monthly cost of a template. The return value is an AWS Simple Monthly Calculator URL with a
* query string that describes the resources required to run the template.
*
*
* @param estimateTemplateCostRequest
* The input for an EstimateTemplateCost action.
* @return Result of the EstimateTemplateCost operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.EstimateTemplateCost
* @see AWS API Documentation
*/
default EstimateTemplateCostResponse estimateTemplateCost(EstimateTemplateCostRequest estimateTemplateCostRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the estimated monthly cost of a template. The return value is an AWS Simple Monthly Calculator URL with a
* query string that describes the resources required to run the template.
*
*
*
* This is a convenience which creates an instance of the {@link EstimateTemplateCostRequest.Builder} avoiding the
* need to create one manually via {@link EstimateTemplateCostRequest#builder()}
*
*
* @param estimateTemplateCostRequest
* A {@link Consumer} that will call methods on {@link EstimateTemplateCostInput.Builder} to create a
* request. The input for an EstimateTemplateCost action.
* @return Result of the EstimateTemplateCost operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.EstimateTemplateCost
* @see AWS API Documentation
*/
default EstimateTemplateCostResponse estimateTemplateCost(
Consumer estimateTemplateCostRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return estimateTemplateCost(EstimateTemplateCostRequest.builder().applyMutation(estimateTemplateCostRequest).build());
}
/**
*
* Updates a stack using the input information that was provided when the specified change set was created. After
* the call successfully completes, AWS CloudFormation starts updating the stack. Use the DescribeStacks
* action to view the status of the update.
*
*
* When you execute a change set, AWS CloudFormation deletes all other change sets associated with the stack because
* they aren't valid for the updated stack.
*
*
* If a stack policy is associated with the stack, AWS CloudFormation enforces the policy during the update. You
* can't specify a temporary stack policy that overrides the current policy.
*
*
* @param executeChangeSetRequest
* The input for the ExecuteChangeSet action.
* @return Result of the ExecuteChangeSet operation returned by the service.
* @throws InvalidChangeSetStatusException
* The specified change set can't be used to update the stack. For example, the change set status might be
* CREATE_IN_PROGRESS
, or the stack status might be UPDATE_IN_PROGRESS
.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
action.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ExecuteChangeSet
* @see AWS API Documentation
*/
default ExecuteChangeSetResponse executeChangeSet(ExecuteChangeSetRequest executeChangeSetRequest)
throws InvalidChangeSetStatusException, ChangeSetNotFoundException, InsufficientCapabilitiesException,
TokenAlreadyExistsException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a stack using the input information that was provided when the specified change set was created. After
* the call successfully completes, AWS CloudFormation starts updating the stack. Use the DescribeStacks
* action to view the status of the update.
*
*
* When you execute a change set, AWS CloudFormation deletes all other change sets associated with the stack because
* they aren't valid for the updated stack.
*
*
* If a stack policy is associated with the stack, AWS CloudFormation enforces the policy during the update. You
* can't specify a temporary stack policy that overrides the current policy.
*
*
*
* This is a convenience which creates an instance of the {@link ExecuteChangeSetRequest.Builder} avoiding the need
* to create one manually via {@link ExecuteChangeSetRequest#builder()}
*
*
* @param executeChangeSetRequest
* A {@link Consumer} that will call methods on {@link ExecuteChangeSetInput.Builder} to create a request.
* The input for the ExecuteChangeSet action.
* @return Result of the ExecuteChangeSet operation returned by the service.
* @throws InvalidChangeSetStatusException
* The specified change set can't be used to update the stack. For example, the change set status might be
* CREATE_IN_PROGRESS
, or the stack status might be UPDATE_IN_PROGRESS
.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
action.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ExecuteChangeSet
* @see AWS API Documentation
*/
default ExecuteChangeSetResponse executeChangeSet(Consumer executeChangeSetRequest)
throws InvalidChangeSetStatusException, ChangeSetNotFoundException, InsufficientCapabilitiesException,
TokenAlreadyExistsException, AwsServiceException, SdkClientException, CloudFormationException {
return executeChangeSet(ExecuteChangeSetRequest.builder().applyMutation(executeChangeSetRequest).build());
}
/**
*
* Returns the stack policy for a specified stack. If a stack doesn't have a policy, a null value is returned.
*
*
* @param getStackPolicyRequest
* The input for the GetStackPolicy action.
* @return Result of the GetStackPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.GetStackPolicy
* @see AWS
* API Documentation
*/
default GetStackPolicyResponse getStackPolicy(GetStackPolicyRequest getStackPolicyRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the stack policy for a specified stack. If a stack doesn't have a policy, a null value is returned.
*
*
*
* This is a convenience which creates an instance of the {@link GetStackPolicyRequest.Builder} avoiding the need to
* create one manually via {@link GetStackPolicyRequest#builder()}
*
*
* @param getStackPolicyRequest
* A {@link Consumer} that will call methods on {@link GetStackPolicyInput.Builder} to create a request. The
* input for the GetStackPolicy action.
* @return Result of the GetStackPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.GetStackPolicy
* @see AWS
* API Documentation
*/
default GetStackPolicyResponse getStackPolicy(Consumer getStackPolicyRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return getStackPolicy(GetStackPolicyRequest.builder().applyMutation(getStackPolicyRequest).build());
}
/**
*
* Returns the template body for a specified stack. You can get the template for running or deleted stacks.
*
*
* For deleted stacks, GetTemplate returns the template for up to 90 days after the stack has been deleted.
*
*
*
* If the template does not exist, a ValidationError
is returned.
*
*
*
* @param getTemplateRequest
* The input for a GetTemplate action.
* @return Result of the GetTemplate operation returned by the service.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.GetTemplate
* @see AWS API
* Documentation
*/
default GetTemplateResponse getTemplate(GetTemplateRequest getTemplateRequest) throws ChangeSetNotFoundException,
AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the template body for a specified stack. You can get the template for running or deleted stacks.
*
*
* For deleted stacks, GetTemplate returns the template for up to 90 days after the stack has been deleted.
*
*
*
* If the template does not exist, a ValidationError
is returned.
*
*
*
* This is a convenience which creates an instance of the {@link GetTemplateRequest.Builder} avoiding the need to
* create one manually via {@link GetTemplateRequest#builder()}
*
*
* @param getTemplateRequest
* A {@link Consumer} that will call methods on {@link GetTemplateInput.Builder} to create a request. The
* input for a GetTemplate action.
* @return Result of the GetTemplate operation returned by the service.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.GetTemplate
* @see AWS API
* Documentation
*/
default GetTemplateResponse getTemplate(Consumer getTemplateRequest)
throws ChangeSetNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
return getTemplate(GetTemplateRequest.builder().applyMutation(getTemplateRequest).build());
}
/**
*
* Returns information about a new or existing template. The GetTemplateSummary
action is useful for
* viewing parameter information, such as default parameter values and parameter types, before you create or update
* a stack or stack set.
*
*
* You can use the GetTemplateSummary
action when you submit a template, or you can get template
* information for a stack set, or a running or deleted stack.
*
*
* For deleted stacks, GetTemplateSummary
returns the template information for up to 90 days after the
* stack has been deleted. If the template does not exist, a ValidationError
is returned.
*
*
* @param getTemplateSummaryRequest
* The input for the GetTemplateSummary action.
* @return Result of the GetTemplateSummary operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.GetTemplateSummary
* @see AWS API Documentation
*/
default GetTemplateSummaryResponse getTemplateSummary(GetTemplateSummaryRequest getTemplateSummaryRequest)
throws StackSetNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a new or existing template. The GetTemplateSummary
action is useful for
* viewing parameter information, such as default parameter values and parameter types, before you create or update
* a stack or stack set.
*
*
* You can use the GetTemplateSummary
action when you submit a template, or you can get template
* information for a stack set, or a running or deleted stack.
*
*
* For deleted stacks, GetTemplateSummary
returns the template information for up to 90 days after the
* stack has been deleted. If the template does not exist, a ValidationError
is returned.
*
*
*
* This is a convenience which creates an instance of the {@link GetTemplateSummaryRequest.Builder} avoiding the
* need to create one manually via {@link GetTemplateSummaryRequest#builder()}
*
*
* @param getTemplateSummaryRequest
* A {@link Consumer} that will call methods on {@link GetTemplateSummaryInput.Builder} to create a request.
* The input for the GetTemplateSummary action.
* @return Result of the GetTemplateSummary operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.GetTemplateSummary
* @see AWS API Documentation
*/
default GetTemplateSummaryResponse getTemplateSummary(Consumer getTemplateSummaryRequest)
throws StackSetNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
return getTemplateSummary(GetTemplateSummaryRequest.builder().applyMutation(getTemplateSummaryRequest).build());
}
/**
*
* Returns the ID and status of each active change set for a stack. For example, AWS CloudFormation lists change
* sets that are in the CREATE_IN_PROGRESS
or CREATE_PENDING
state.
*
*
* @param listChangeSetsRequest
* The input for the ListChangeSets action.
* @return Result of the ListChangeSets operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListChangeSets
* @see AWS
* API Documentation
*/
default ListChangeSetsResponse listChangeSets(ListChangeSetsRequest listChangeSetsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the ID and status of each active change set for a stack. For example, AWS CloudFormation lists change
* sets that are in the CREATE_IN_PROGRESS
or CREATE_PENDING
state.
*
*
*
* This is a convenience which creates an instance of the {@link ListChangeSetsRequest.Builder} avoiding the need to
* create one manually via {@link ListChangeSetsRequest#builder()}
*
*
* @param listChangeSetsRequest
* A {@link Consumer} that will call methods on {@link ListChangeSetsInput.Builder} to create a request. The
* input for the ListChangeSets action.
* @return Result of the ListChangeSets operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListChangeSets
* @see AWS
* API Documentation
*/
default ListChangeSetsResponse listChangeSets(Consumer listChangeSetsRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return listChangeSets(ListChangeSetsRequest.builder().applyMutation(listChangeSetsRequest).build());
}
/**
*
* Returns the ID and status of each active change set for a stack. For example, AWS CloudFormation lists change
* sets that are in the CREATE_IN_PROGRESS
or CREATE_PENDING
state.
*
*
*
* This is a variant of
* {@link #listChangeSets(software.amazon.awssdk.services.cloudformation.model.ListChangeSetsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListChangeSetsIterable responses = client.listChangeSetsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListChangeSetsIterable responses = client
* .listChangeSetsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListChangeSetsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListChangeSetsIterable responses = client.listChangeSetsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChangeSets(software.amazon.awssdk.services.cloudformation.model.ListChangeSetsRequest)}
* operation.
*
*
* @param listChangeSetsRequest
* The input for the ListChangeSets action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListChangeSets
* @see AWS
* API Documentation
*/
default ListChangeSetsIterable listChangeSetsPaginator(ListChangeSetsRequest listChangeSetsRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the ID and status of each active change set for a stack. For example, AWS CloudFormation lists change
* sets that are in the CREATE_IN_PROGRESS
or CREATE_PENDING
state.
*
*
*
* This is a variant of
* {@link #listChangeSets(software.amazon.awssdk.services.cloudformation.model.ListChangeSetsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListChangeSetsIterable responses = client.listChangeSetsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListChangeSetsIterable responses = client
* .listChangeSetsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListChangeSetsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListChangeSetsIterable responses = client.listChangeSetsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChangeSets(software.amazon.awssdk.services.cloudformation.model.ListChangeSetsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListChangeSetsRequest.Builder} avoiding the need to
* create one manually via {@link ListChangeSetsRequest#builder()}
*
*
* @param listChangeSetsRequest
* A {@link Consumer} that will call methods on {@link ListChangeSetsInput.Builder} to create a request. The
* input for the ListChangeSets action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListChangeSets
* @see AWS
* API Documentation
*/
default ListChangeSetsIterable listChangeSetsPaginator(Consumer listChangeSetsRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return listChangeSetsPaginator(ListChangeSetsRequest.builder().applyMutation(listChangeSetsRequest).build());
}
/**
*
* Lists all exported output values in the account and Region in which you call this action. Use this action to see
* the exported output values that you can import into other stacks. To import values, use the
* Fn::ImportValue
function.
*
*
* For more information, see AWS
* CloudFormation Export Stack Output Values.
*
*
* @return Result of the ListExports operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListExports
* @see #listExports(ListExportsRequest)
* @see AWS API
* Documentation
*/
default ListExportsResponse listExports() throws AwsServiceException, SdkClientException, CloudFormationException {
return listExports(ListExportsRequest.builder().build());
}
/**
*
* Lists all exported output values in the account and Region in which you call this action. Use this action to see
* the exported output values that you can import into other stacks. To import values, use the
* Fn::ImportValue
function.
*
*
* For more information, see AWS
* CloudFormation Export Stack Output Values.
*
*
* @param listExportsRequest
* @return Result of the ListExports operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListExports
* @see AWS API
* Documentation
*/
default ListExportsResponse listExports(ListExportsRequest listExportsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all exported output values in the account and Region in which you call this action. Use this action to see
* the exported output values that you can import into other stacks. To import values, use the
* Fn::ImportValue
function.
*
*
* For more information, see AWS
* CloudFormation Export Stack Output Values.
*
*
*
* This is a convenience which creates an instance of the {@link ListExportsRequest.Builder} avoiding the need to
* create one manually via {@link ListExportsRequest#builder()}
*
*
* @param listExportsRequest
* A {@link Consumer} that will call methods on {@link ListExportsInput.Builder} to create a request.
* @return Result of the ListExports operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListExports
* @see AWS API
* Documentation
*/
default ListExportsResponse listExports(Consumer listExportsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return listExports(ListExportsRequest.builder().applyMutation(listExportsRequest).build());
}
/**
*
* Lists all exported output values in the account and Region in which you call this action. Use this action to see
* the exported output values that you can import into other stacks. To import values, use the
* Fn::ImportValue
function.
*
*
* For more information, see AWS
* CloudFormation Export Stack Output Values.
*
*
*
* This is a variant of
* {@link #listExports(software.amazon.awssdk.services.cloudformation.model.ListExportsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListExportsIterable responses = client.listExportsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListExportsIterable responses = client
* .listExportsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListExportsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListExportsIterable responses = client.listExportsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listExports(software.amazon.awssdk.services.cloudformation.model.ListExportsRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListExports
* @see #listExportsPaginator(ListExportsRequest)
* @see AWS API
* Documentation
*/
default ListExportsIterable listExportsPaginator() throws AwsServiceException, SdkClientException, CloudFormationException {
return listExportsPaginator(ListExportsRequest.builder().build());
}
/**
*
* Lists all exported output values in the account and Region in which you call this action. Use this action to see
* the exported output values that you can import into other stacks. To import values, use the
* Fn::ImportValue
function.
*
*
* For more information, see AWS
* CloudFormation Export Stack Output Values.
*
*
*
* This is a variant of
* {@link #listExports(software.amazon.awssdk.services.cloudformation.model.ListExportsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListExportsIterable responses = client.listExportsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListExportsIterable responses = client
* .listExportsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListExportsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListExportsIterable responses = client.listExportsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listExports(software.amazon.awssdk.services.cloudformation.model.ListExportsRequest)} operation.
*
*
* @param listExportsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListExports
* @see AWS API
* Documentation
*/
default ListExportsIterable listExportsPaginator(ListExportsRequest listExportsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all exported output values in the account and Region in which you call this action. Use this action to see
* the exported output values that you can import into other stacks. To import values, use the
* Fn::ImportValue
function.
*
*
* For more information, see AWS
* CloudFormation Export Stack Output Values.
*
*
*
* This is a variant of
* {@link #listExports(software.amazon.awssdk.services.cloudformation.model.ListExportsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListExportsIterable responses = client.listExportsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListExportsIterable responses = client
* .listExportsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListExportsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListExportsIterable responses = client.listExportsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listExports(software.amazon.awssdk.services.cloudformation.model.ListExportsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListExportsRequest.Builder} avoiding the need to
* create one manually via {@link ListExportsRequest#builder()}
*
*
* @param listExportsRequest
* A {@link Consumer} that will call methods on {@link ListExportsInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListExports
* @see AWS API
* Documentation
*/
default ListExportsIterable listExportsPaginator(Consumer listExportsRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return listExportsPaginator(ListExportsRequest.builder().applyMutation(listExportsRequest).build());
}
/**
*
* Lists all stacks that are importing an exported output value. To modify or remove an exported output value, first
* use this action to see which stacks are using it. To see the exported output values in your account, see
* ListExports.
*
*
* For more information about importing an exported output value, see the
* Fn::ImportValue
function.
*
*
* @param listImportsRequest
* @return Result of the ListImports operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListImports
* @see AWS API
* Documentation
*/
default ListImportsResponse listImports(ListImportsRequest listImportsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all stacks that are importing an exported output value. To modify or remove an exported output value, first
* use this action to see which stacks are using it. To see the exported output values in your account, see
* ListExports.
*
*
* For more information about importing an exported output value, see the
* Fn::ImportValue
function.
*
*
*
* This is a convenience which creates an instance of the {@link ListImportsRequest.Builder} avoiding the need to
* create one manually via {@link ListImportsRequest#builder()}
*
*
* @param listImportsRequest
* A {@link Consumer} that will call methods on {@link ListImportsInput.Builder} to create a request.
* @return Result of the ListImports operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListImports
* @see AWS API
* Documentation
*/
default ListImportsResponse listImports(Consumer listImportsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return listImports(ListImportsRequest.builder().applyMutation(listImportsRequest).build());
}
/**
*
* Lists all stacks that are importing an exported output value. To modify or remove an exported output value, first
* use this action to see which stacks are using it. To see the exported output values in your account, see
* ListExports.
*
*
* For more information about importing an exported output value, see the
* Fn::ImportValue
function.
*
*
*
* This is a variant of
* {@link #listImports(software.amazon.awssdk.services.cloudformation.model.ListImportsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListImportsIterable responses = client.listImportsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListImportsIterable responses = client
* .listImportsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListImportsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListImportsIterable responses = client.listImportsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImports(software.amazon.awssdk.services.cloudformation.model.ListImportsRequest)} operation.
*
*
* @param listImportsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListImports
* @see AWS API
* Documentation
*/
default ListImportsIterable listImportsPaginator(ListImportsRequest listImportsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all stacks that are importing an exported output value. To modify or remove an exported output value, first
* use this action to see which stacks are using it. To see the exported output values in your account, see
* ListExports.
*
*
* For more information about importing an exported output value, see the
* Fn::ImportValue
function.
*
*
*
* This is a variant of
* {@link #listImports(software.amazon.awssdk.services.cloudformation.model.ListImportsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListImportsIterable responses = client.listImportsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListImportsIterable responses = client
* .listImportsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListImportsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListImportsIterable responses = client.listImportsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listImports(software.amazon.awssdk.services.cloudformation.model.ListImportsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListImportsRequest.Builder} avoiding the need to
* create one manually via {@link ListImportsRequest#builder()}
*
*
* @param listImportsRequest
* A {@link Consumer} that will call methods on {@link ListImportsInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListImports
* @see AWS API
* Documentation
*/
default ListImportsIterable listImportsPaginator(Consumer listImportsRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return listImportsPaginator(ListImportsRequest.builder().applyMutation(listImportsRequest).build());
}
/**
*
* Returns summary information about stack instances that are associated with the specified stack set. You can
* filter for stack instances that are associated with a specific AWS account name or Region, or that have a
* specific status.
*
*
* @param listStackInstancesRequest
* @return Result of the ListStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackInstances
* @see AWS API Documentation
*/
default ListStackInstancesResponse listStackInstances(ListStackInstancesRequest listStackInstancesRequest)
throws StackSetNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns summary information about stack instances that are associated with the specified stack set. You can
* filter for stack instances that are associated with a specific AWS account name or Region, or that have a
* specific status.
*
*
*
* This is a convenience which creates an instance of the {@link ListStackInstancesRequest.Builder} avoiding the
* need to create one manually via {@link ListStackInstancesRequest#builder()}
*
*
* @param listStackInstancesRequest
* A {@link Consumer} that will call methods on {@link ListStackInstancesInput.Builder} to create a request.
* @return Result of the ListStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackInstances
* @see AWS API Documentation
*/
default ListStackInstancesResponse listStackInstances(Consumer listStackInstancesRequest)
throws StackSetNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
return listStackInstances(ListStackInstancesRequest.builder().applyMutation(listStackInstancesRequest).build());
}
/**
*
* Returns summary information about stack instances that are associated with the specified stack set. You can
* filter for stack instances that are associated with a specific AWS account name or Region, or that have a
* specific status.
*
*
*
* This is a variant of
* {@link #listStackInstances(software.amazon.awssdk.services.cloudformation.model.ListStackInstancesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackInstancesIterable responses = client.listStackInstancesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackInstancesIterable responses = client
* .listStackInstancesPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStackInstancesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackInstancesIterable responses = client.listStackInstancesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStackInstances(software.amazon.awssdk.services.cloudformation.model.ListStackInstancesRequest)}
* operation.
*
*
* @param listStackInstancesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackInstances
* @see AWS API Documentation
*/
default ListStackInstancesIterable listStackInstancesPaginator(ListStackInstancesRequest listStackInstancesRequest)
throws StackSetNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns summary information about stack instances that are associated with the specified stack set. You can
* filter for stack instances that are associated with a specific AWS account name or Region, or that have a
* specific status.
*
*
*
* This is a variant of
* {@link #listStackInstances(software.amazon.awssdk.services.cloudformation.model.ListStackInstancesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackInstancesIterable responses = client.listStackInstancesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackInstancesIterable responses = client
* .listStackInstancesPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStackInstancesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackInstancesIterable responses = client.listStackInstancesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStackInstances(software.amazon.awssdk.services.cloudformation.model.ListStackInstancesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListStackInstancesRequest.Builder} avoiding the
* need to create one manually via {@link ListStackInstancesRequest#builder()}
*
*
* @param listStackInstancesRequest
* A {@link Consumer} that will call methods on {@link ListStackInstancesInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackInstances
* @see AWS API Documentation
*/
default ListStackInstancesIterable listStackInstancesPaginator(
Consumer listStackInstancesRequest) throws StackSetNotFoundException,
AwsServiceException, SdkClientException, CloudFormationException {
return listStackInstancesPaginator(ListStackInstancesRequest.builder().applyMutation(listStackInstancesRequest).build());
}
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information for up to 90 days after the stack has been
* deleted.
*
*
* @param listStackResourcesRequest
* The input for the ListStackResource action.
* @return Result of the ListStackResources operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackResources
* @see AWS API Documentation
*/
default ListStackResourcesResponse listStackResources(ListStackResourcesRequest listStackResourcesRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information for up to 90 days after the stack has been
* deleted.
*
*
*
* This is a convenience which creates an instance of the {@link ListStackResourcesRequest.Builder} avoiding the
* need to create one manually via {@link ListStackResourcesRequest#builder()}
*
*
* @param listStackResourcesRequest
* A {@link Consumer} that will call methods on {@link ListStackResourcesInput.Builder} to create a request.
* The input for the ListStackResource action.
* @return Result of the ListStackResources operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackResources
* @see AWS API Documentation
*/
default ListStackResourcesResponse listStackResources(Consumer listStackResourcesRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return listStackResources(ListStackResourcesRequest.builder().applyMutation(listStackResourcesRequest).build());
}
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information for up to 90 days after the stack has been
* deleted.
*
*
*
* This is a variant of
* {@link #listStackResources(software.amazon.awssdk.services.cloudformation.model.ListStackResourcesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackResourcesIterable responses = client.listStackResourcesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackResourcesIterable responses = client
* .listStackResourcesPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStackResourcesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackResourcesIterable responses = client.listStackResourcesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStackResources(software.amazon.awssdk.services.cloudformation.model.ListStackResourcesRequest)}
* operation.
*
*
* @param listStackResourcesRequest
* The input for the ListStackResource action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackResources
* @see AWS API Documentation
*/
default ListStackResourcesIterable listStackResourcesPaginator(ListStackResourcesRequest listStackResourcesRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information for up to 90 days after the stack has been
* deleted.
*
*
*
* This is a variant of
* {@link #listStackResources(software.amazon.awssdk.services.cloudformation.model.ListStackResourcesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackResourcesIterable responses = client.listStackResourcesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackResourcesIterable responses = client
* .listStackResourcesPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStackResourcesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackResourcesIterable responses = client.listStackResourcesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStackResources(software.amazon.awssdk.services.cloudformation.model.ListStackResourcesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListStackResourcesRequest.Builder} avoiding the
* need to create one manually via {@link ListStackResourcesRequest#builder()}
*
*
* @param listStackResourcesRequest
* A {@link Consumer} that will call methods on {@link ListStackResourcesInput.Builder} to create a request.
* The input for the ListStackResource action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackResources
* @see AWS API Documentation
*/
default ListStackResourcesIterable listStackResourcesPaginator(
Consumer listStackResourcesRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return listStackResourcesPaginator(ListStackResourcesRequest.builder().applyMutation(listStackResourcesRequest).build());
}
/**
*
* Returns summary information about the results of a stack set operation.
*
*
* @param listStackSetOperationResultsRequest
* @return Result of the ListStackSetOperationResults operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSetOperationResults
* @see AWS API Documentation
*/
default ListStackSetOperationResultsResponse listStackSetOperationResults(
ListStackSetOperationResultsRequest listStackSetOperationResultsRequest) throws StackSetNotFoundException,
OperationNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns summary information about the results of a stack set operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListStackSetOperationResultsRequest.Builder}
* avoiding the need to create one manually via {@link ListStackSetOperationResultsRequest#builder()}
*
*
* @param listStackSetOperationResultsRequest
* A {@link Consumer} that will call methods on {@link ListStackSetOperationResultsInput.Builder} to create a
* request.
* @return Result of the ListStackSetOperationResults operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSetOperationResults
* @see AWS API Documentation
*/
default ListStackSetOperationResultsResponse listStackSetOperationResults(
Consumer listStackSetOperationResultsRequest)
throws StackSetNotFoundException, OperationNotFoundException, AwsServiceException, SdkClientException,
CloudFormationException {
return listStackSetOperationResults(ListStackSetOperationResultsRequest.builder()
.applyMutation(listStackSetOperationResultsRequest).build());
}
/**
*
* Returns summary information about the results of a stack set operation.
*
*
*
* This is a variant of
* {@link #listStackSetOperationResults(software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationResultsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationResultsIterable responses = client.listStackSetOperationResultsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationResultsIterable responses = client
* .listStackSetOperationResultsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationResultsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationResultsIterable responses = client.listStackSetOperationResultsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStackSetOperationResults(software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationResultsRequest)}
* operation.
*
*
* @param listStackSetOperationResultsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSetOperationResults
* @see AWS API Documentation
*/
default ListStackSetOperationResultsIterable listStackSetOperationResultsPaginator(
ListStackSetOperationResultsRequest listStackSetOperationResultsRequest) throws StackSetNotFoundException,
OperationNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns summary information about the results of a stack set operation.
*
*
*
* This is a variant of
* {@link #listStackSetOperationResults(software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationResultsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationResultsIterable responses = client.listStackSetOperationResultsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationResultsIterable responses = client
* .listStackSetOperationResultsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationResultsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationResultsIterable responses = client.listStackSetOperationResultsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStackSetOperationResults(software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationResultsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListStackSetOperationResultsRequest.Builder}
* avoiding the need to create one manually via {@link ListStackSetOperationResultsRequest#builder()}
*
*
* @param listStackSetOperationResultsRequest
* A {@link Consumer} that will call methods on {@link ListStackSetOperationResultsInput.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSetOperationResults
* @see AWS API Documentation
*/
default ListStackSetOperationResultsIterable listStackSetOperationResultsPaginator(
Consumer listStackSetOperationResultsRequest)
throws StackSetNotFoundException, OperationNotFoundException, AwsServiceException, SdkClientException,
CloudFormationException {
return listStackSetOperationResultsPaginator(ListStackSetOperationResultsRequest.builder()
.applyMutation(listStackSetOperationResultsRequest).build());
}
/**
*
* Returns summary information about operations performed on a stack set.
*
*
* @param listStackSetOperationsRequest
* @return Result of the ListStackSetOperations operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSetOperations
* @see AWS API Documentation
*/
default ListStackSetOperationsResponse listStackSetOperations(ListStackSetOperationsRequest listStackSetOperationsRequest)
throws StackSetNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns summary information about operations performed on a stack set.
*
*
*
* This is a convenience which creates an instance of the {@link ListStackSetOperationsRequest.Builder} avoiding the
* need to create one manually via {@link ListStackSetOperationsRequest#builder()}
*
*
* @param listStackSetOperationsRequest
* A {@link Consumer} that will call methods on {@link ListStackSetOperationsInput.Builder} to create a
* request.
* @return Result of the ListStackSetOperations operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSetOperations
* @see AWS API Documentation
*/
default ListStackSetOperationsResponse listStackSetOperations(
Consumer listStackSetOperationsRequest) throws StackSetNotFoundException,
AwsServiceException, SdkClientException, CloudFormationException {
return listStackSetOperations(ListStackSetOperationsRequest.builder().applyMutation(listStackSetOperationsRequest)
.build());
}
/**
*
* Returns summary information about operations performed on a stack set.
*
*
*
* This is a variant of
* {@link #listStackSetOperations(software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationsIterable responses = client.listStackSetOperationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationsIterable responses = client
* .listStackSetOperationsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationsIterable responses = client.listStackSetOperationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStackSetOperations(software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationsRequest)}
* operation.
*
*
* @param listStackSetOperationsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSetOperations
* @see AWS API Documentation
*/
default ListStackSetOperationsIterable listStackSetOperationsPaginator(
ListStackSetOperationsRequest listStackSetOperationsRequest) throws StackSetNotFoundException, AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns summary information about operations performed on a stack set.
*
*
*
* This is a variant of
* {@link #listStackSetOperations(software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationsIterable responses = client.listStackSetOperationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationsIterable responses = client
* .listStackSetOperationsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetOperationsIterable responses = client.listStackSetOperationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStackSetOperations(software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListStackSetOperationsRequest.Builder} avoiding the
* need to create one manually via {@link ListStackSetOperationsRequest#builder()}
*
*
* @param listStackSetOperationsRequest
* A {@link Consumer} that will call methods on {@link ListStackSetOperationsInput.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSetOperations
* @see AWS API Documentation
*/
default ListStackSetOperationsIterable listStackSetOperationsPaginator(
Consumer listStackSetOperationsRequest) throws StackSetNotFoundException,
AwsServiceException, SdkClientException, CloudFormationException {
return listStackSetOperationsPaginator(ListStackSetOperationsRequest.builder()
.applyMutation(listStackSetOperationsRequest).build());
}
/**
*
* Returns summary information about stack sets that are associated with the user.
*
*
* @return Result of the ListStackSets operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSets
* @see #listStackSets(ListStackSetsRequest)
* @see AWS
* API Documentation
*/
default ListStackSetsResponse listStackSets() throws AwsServiceException, SdkClientException, CloudFormationException {
return listStackSets(ListStackSetsRequest.builder().build());
}
/**
*
* Returns summary information about stack sets that are associated with the user.
*
*
* @param listStackSetsRequest
* @return Result of the ListStackSets operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSets
* @see AWS
* API Documentation
*/
default ListStackSetsResponse listStackSets(ListStackSetsRequest listStackSetsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns summary information about stack sets that are associated with the user.
*
*
*
* This is a convenience which creates an instance of the {@link ListStackSetsRequest.Builder} avoiding the need to
* create one manually via {@link ListStackSetsRequest#builder()}
*
*
* @param listStackSetsRequest
* A {@link Consumer} that will call methods on {@link ListStackSetsInput.Builder} to create a request.
* @return Result of the ListStackSets operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSets
* @see AWS
* API Documentation
*/
default ListStackSetsResponse listStackSets(Consumer listStackSetsRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return listStackSets(ListStackSetsRequest.builder().applyMutation(listStackSetsRequest).build());
}
/**
*
* Returns summary information about stack sets that are associated with the user.
*
*
*
* This is a variant of
* {@link #listStackSets(software.amazon.awssdk.services.cloudformation.model.ListStackSetsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetsIterable responses = client.listStackSetsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetsIterable responses = client
* .listStackSetsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStackSetsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetsIterable responses = client.listStackSetsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStackSets(software.amazon.awssdk.services.cloudformation.model.ListStackSetsRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSets
* @see #listStackSetsPaginator(ListStackSetsRequest)
* @see AWS
* API Documentation
*/
default ListStackSetsIterable listStackSetsPaginator() throws AwsServiceException, SdkClientException,
CloudFormationException {
return listStackSetsPaginator(ListStackSetsRequest.builder().build());
}
/**
*
* Returns summary information about stack sets that are associated with the user.
*
*
*
* This is a variant of
* {@link #listStackSets(software.amazon.awssdk.services.cloudformation.model.ListStackSetsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetsIterable responses = client.listStackSetsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetsIterable responses = client
* .listStackSetsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStackSetsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetsIterable responses = client.listStackSetsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStackSets(software.amazon.awssdk.services.cloudformation.model.ListStackSetsRequest)} operation.
*
*
* @param listStackSetsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSets
* @see AWS
* API Documentation
*/
default ListStackSetsIterable listStackSetsPaginator(ListStackSetsRequest listStackSetsRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns summary information about stack sets that are associated with the user.
*
*
*
* This is a variant of
* {@link #listStackSets(software.amazon.awssdk.services.cloudformation.model.ListStackSetsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetsIterable responses = client.listStackSetsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetsIterable responses = client
* .listStackSetsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStackSetsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStackSetsIterable responses = client.listStackSetsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStackSets(software.amazon.awssdk.services.cloudformation.model.ListStackSetsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListStackSetsRequest.Builder} avoiding the need to
* create one manually via {@link ListStackSetsRequest#builder()}
*
*
* @param listStackSetsRequest
* A {@link Consumer} that will call methods on {@link ListStackSetsInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStackSets
* @see AWS
* API Documentation
*/
default ListStackSetsIterable listStackSetsPaginator(Consumer listStackSetsRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return listStackSetsPaginator(ListStackSetsRequest.builder().applyMutation(listStackSetsRequest).build());
}
/**
*
* Returns the summary information for stacks whose status matches the specified StackStatusFilter. Summary
* information for stacks that have been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is returned (including existing stacks and
* stacks that have been deleted).
*
*
* @return Result of the ListStacks operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStacks
* @see #listStacks(ListStacksRequest)
* @see AWS API
* Documentation
*/
default ListStacksResponse listStacks() throws AwsServiceException, SdkClientException, CloudFormationException {
return listStacks(ListStacksRequest.builder().build());
}
/**
*
* Returns the summary information for stacks whose status matches the specified StackStatusFilter. Summary
* information for stacks that have been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is returned (including existing stacks and
* stacks that have been deleted).
*
*
* @param listStacksRequest
* The input for ListStacks action.
* @return Result of the ListStacks operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStacks
* @see AWS API
* Documentation
*/
default ListStacksResponse listStacks(ListStacksRequest listStacksRequest) throws AwsServiceException, SdkClientException,
CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the summary information for stacks whose status matches the specified StackStatusFilter. Summary
* information for stacks that have been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is returned (including existing stacks and
* stacks that have been deleted).
*
*
*
* This is a convenience which creates an instance of the {@link ListStacksRequest.Builder} avoiding the need to
* create one manually via {@link ListStacksRequest#builder()}
*
*
* @param listStacksRequest
* A {@link Consumer} that will call methods on {@link ListStacksInput.Builder} to create a request. The
* input for ListStacks action.
* @return Result of the ListStacks operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStacks
* @see AWS API
* Documentation
*/
default ListStacksResponse listStacks(Consumer listStacksRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return listStacks(ListStacksRequest.builder().applyMutation(listStacksRequest).build());
}
/**
*
* Returns the summary information for stacks whose status matches the specified StackStatusFilter. Summary
* information for stacks that have been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is returned (including existing stacks and
* stacks that have been deleted).
*
*
*
* This is a variant of {@link #listStacks(software.amazon.awssdk.services.cloudformation.model.ListStacksRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStacksIterable responses = client.listStacksPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStacksIterable responses = client.listStacksPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStacksResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStacksIterable responses = client.listStacksPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStacks(software.amazon.awssdk.services.cloudformation.model.ListStacksRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStacks
* @see #listStacksPaginator(ListStacksRequest)
* @see AWS API
* Documentation
*/
default ListStacksIterable listStacksPaginator() throws AwsServiceException, SdkClientException, CloudFormationException {
return listStacksPaginator(ListStacksRequest.builder().build());
}
/**
*
* Returns the summary information for stacks whose status matches the specified StackStatusFilter. Summary
* information for stacks that have been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is returned (including existing stacks and
* stacks that have been deleted).
*
*
*
* This is a variant of {@link #listStacks(software.amazon.awssdk.services.cloudformation.model.ListStacksRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStacksIterable responses = client.listStacksPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStacksIterable responses = client.listStacksPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStacksResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStacksIterable responses = client.listStacksPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStacks(software.amazon.awssdk.services.cloudformation.model.ListStacksRequest)} operation.
*
*
* @param listStacksRequest
* The input for ListStacks action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStacks
* @see AWS API
* Documentation
*/
default ListStacksIterable listStacksPaginator(ListStacksRequest listStacksRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the summary information for stacks whose status matches the specified StackStatusFilter. Summary
* information for stacks that have been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is returned (including existing stacks and
* stacks that have been deleted).
*
*
*
* This is a variant of {@link #listStacks(software.amazon.awssdk.services.cloudformation.model.ListStacksRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStacksIterable responses = client.listStacksPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListStacksIterable responses = client.listStacksPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListStacksResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListStacksIterable responses = client.listStacksPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStacks(software.amazon.awssdk.services.cloudformation.model.ListStacksRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListStacksRequest.Builder} avoiding the need to
* create one manually via {@link ListStacksRequest#builder()}
*
*
* @param listStacksRequest
* A {@link Consumer} that will call methods on {@link ListStacksInput.Builder} to create a request. The
* input for ListStacks action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListStacks
* @see AWS API
* Documentation
*/
default ListStacksIterable listStacksPaginator(Consumer listStacksRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return listStacksPaginator(ListStacksRequest.builder().applyMutation(listStacksRequest).build());
}
/**
*
* Returns a list of registration tokens for the specified type(s).
*
*
* @param listTypeRegistrationsRequest
* @return Result of the ListTypeRegistrations operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListTypeRegistrations
* @see AWS API Documentation
*/
default ListTypeRegistrationsResponse listTypeRegistrations(ListTypeRegistrationsRequest listTypeRegistrationsRequest)
throws CfnRegistryException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of registration tokens for the specified type(s).
*
*
*
* This is a convenience which creates an instance of the {@link ListTypeRegistrationsRequest.Builder} avoiding the
* need to create one manually via {@link ListTypeRegistrationsRequest#builder()}
*
*
* @param listTypeRegistrationsRequest
* A {@link Consumer} that will call methods on {@link ListTypeRegistrationsInput.Builder} to create a
* request.
* @return Result of the ListTypeRegistrations operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListTypeRegistrations
* @see AWS API Documentation
*/
default ListTypeRegistrationsResponse listTypeRegistrations(
Consumer listTypeRegistrationsRequest) throws CfnRegistryException,
AwsServiceException, SdkClientException, CloudFormationException {
return listTypeRegistrations(ListTypeRegistrationsRequest.builder().applyMutation(listTypeRegistrationsRequest).build());
}
/**
*
* Returns a list of registration tokens for the specified type(s).
*
*
*
* This is a variant of
* {@link #listTypeRegistrations(software.amazon.awssdk.services.cloudformation.model.ListTypeRegistrationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypeRegistrationsIterable responses = client.listTypeRegistrationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypeRegistrationsIterable responses = client
* .listTypeRegistrationsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListTypeRegistrationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypeRegistrationsIterable responses = client.listTypeRegistrationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTypeRegistrations(software.amazon.awssdk.services.cloudformation.model.ListTypeRegistrationsRequest)}
* operation.
*
*
* @param listTypeRegistrationsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListTypeRegistrations
* @see AWS API Documentation
*/
default ListTypeRegistrationsIterable listTypeRegistrationsPaginator(ListTypeRegistrationsRequest listTypeRegistrationsRequest)
throws CfnRegistryException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of registration tokens for the specified type(s).
*
*
*
* This is a variant of
* {@link #listTypeRegistrations(software.amazon.awssdk.services.cloudformation.model.ListTypeRegistrationsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypeRegistrationsIterable responses = client.listTypeRegistrationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypeRegistrationsIterable responses = client
* .listTypeRegistrationsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListTypeRegistrationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypeRegistrationsIterable responses = client.listTypeRegistrationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTypeRegistrations(software.amazon.awssdk.services.cloudformation.model.ListTypeRegistrationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTypeRegistrationsRequest.Builder} avoiding the
* need to create one manually via {@link ListTypeRegistrationsRequest#builder()}
*
*
* @param listTypeRegistrationsRequest
* A {@link Consumer} that will call methods on {@link ListTypeRegistrationsInput.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListTypeRegistrations
* @see AWS API Documentation
*/
default ListTypeRegistrationsIterable listTypeRegistrationsPaginator(
Consumer listTypeRegistrationsRequest) throws CfnRegistryException,
AwsServiceException, SdkClientException, CloudFormationException {
return listTypeRegistrationsPaginator(ListTypeRegistrationsRequest.builder().applyMutation(listTypeRegistrationsRequest)
.build());
}
/**
*
* Returns summary information about the versions of a type.
*
*
* @param listTypeVersionsRequest
* @return Result of the ListTypeVersions operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListTypeVersions
* @see AWS API Documentation
*/
default ListTypeVersionsResponse listTypeVersions(ListTypeVersionsRequest listTypeVersionsRequest)
throws CfnRegistryException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns summary information about the versions of a type.
*
*
*
* This is a convenience which creates an instance of the {@link ListTypeVersionsRequest.Builder} avoiding the need
* to create one manually via {@link ListTypeVersionsRequest#builder()}
*
*
* @param listTypeVersionsRequest
* A {@link Consumer} that will call methods on {@link ListTypeVersionsInput.Builder} to create a request.
* @return Result of the ListTypeVersions operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListTypeVersions
* @see AWS API Documentation
*/
default ListTypeVersionsResponse listTypeVersions(Consumer listTypeVersionsRequest)
throws CfnRegistryException, AwsServiceException, SdkClientException, CloudFormationException {
return listTypeVersions(ListTypeVersionsRequest.builder().applyMutation(listTypeVersionsRequest).build());
}
/**
*
* Returns summary information about the versions of a type.
*
*
*
* This is a variant of
* {@link #listTypeVersions(software.amazon.awssdk.services.cloudformation.model.ListTypeVersionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypeVersionsIterable responses = client.listTypeVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypeVersionsIterable responses = client
* .listTypeVersionsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListTypeVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypeVersionsIterable responses = client.listTypeVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTypeVersions(software.amazon.awssdk.services.cloudformation.model.ListTypeVersionsRequest)}
* operation.
*
*
* @param listTypeVersionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListTypeVersions
* @see AWS API Documentation
*/
default ListTypeVersionsIterable listTypeVersionsPaginator(ListTypeVersionsRequest listTypeVersionsRequest)
throws CfnRegistryException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns summary information about the versions of a type.
*
*
*
* This is a variant of
* {@link #listTypeVersions(software.amazon.awssdk.services.cloudformation.model.ListTypeVersionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypeVersionsIterable responses = client.listTypeVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypeVersionsIterable responses = client
* .listTypeVersionsPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListTypeVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypeVersionsIterable responses = client.listTypeVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTypeVersions(software.amazon.awssdk.services.cloudformation.model.ListTypeVersionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTypeVersionsRequest.Builder} avoiding the need
* to create one manually via {@link ListTypeVersionsRequest#builder()}
*
*
* @param listTypeVersionsRequest
* A {@link Consumer} that will call methods on {@link ListTypeVersionsInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListTypeVersions
* @see AWS API Documentation
*/
default ListTypeVersionsIterable listTypeVersionsPaginator(Consumer listTypeVersionsRequest)
throws CfnRegistryException, AwsServiceException, SdkClientException, CloudFormationException {
return listTypeVersionsPaginator(ListTypeVersionsRequest.builder().applyMutation(listTypeVersionsRequest).build());
}
/**
*
* Returns summary information about types that have been registered with CloudFormation.
*
*
* @param listTypesRequest
* @return Result of the ListTypes operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListTypes
* @see AWS API
* Documentation
*/
default ListTypesResponse listTypes(ListTypesRequest listTypesRequest) throws CfnRegistryException, AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns summary information about types that have been registered with CloudFormation.
*
*
*
* This is a convenience which creates an instance of the {@link ListTypesRequest.Builder} avoiding the need to
* create one manually via {@link ListTypesRequest#builder()}
*
*
* @param listTypesRequest
* A {@link Consumer} that will call methods on {@link ListTypesInput.Builder} to create a request.
* @return Result of the ListTypes operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListTypes
* @see AWS API
* Documentation
*/
default ListTypesResponse listTypes(Consumer listTypesRequest) throws CfnRegistryException,
AwsServiceException, SdkClientException, CloudFormationException {
return listTypes(ListTypesRequest.builder().applyMutation(listTypesRequest).build());
}
/**
*
* Returns summary information about types that have been registered with CloudFormation.
*
*
*
* This is a variant of {@link #listTypes(software.amazon.awssdk.services.cloudformation.model.ListTypesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypesIterable responses = client.listTypesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypesIterable responses = client.listTypesPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListTypesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypesIterable responses = client.listTypesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTypes(software.amazon.awssdk.services.cloudformation.model.ListTypesRequest)} operation.
*
*
* @param listTypesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListTypes
* @see AWS API
* Documentation
*/
default ListTypesIterable listTypesPaginator(ListTypesRequest listTypesRequest) throws CfnRegistryException,
AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Returns summary information about types that have been registered with CloudFormation.
*
*
*
* This is a variant of {@link #listTypes(software.amazon.awssdk.services.cloudformation.model.ListTypesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypesIterable responses = client.listTypesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypesIterable responses = client.listTypesPaginator(request);
* for (software.amazon.awssdk.services.cloudformation.model.ListTypesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cloudformation.paginators.ListTypesIterable responses = client.listTypesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTypes(software.amazon.awssdk.services.cloudformation.model.ListTypesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListTypesRequest.Builder} avoiding the need to
* create one manually via {@link ListTypesRequest#builder()}
*
*
* @param listTypesRequest
* A {@link Consumer} that will call methods on {@link ListTypesInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ListTypes
* @see AWS API
* Documentation
*/
default ListTypesIterable listTypesPaginator(Consumer listTypesRequest)
throws CfnRegistryException, AwsServiceException, SdkClientException, CloudFormationException {
return listTypesPaginator(ListTypesRequest.builder().applyMutation(listTypesRequest).build());
}
/**
*
* Reports progress of a resource handler to CloudFormation.
*
*
* Reserved for use by the CloudFormation CLI. Do not use this API in your code.
*
*
* @param recordHandlerProgressRequest
* @return Result of the RecordHandlerProgress operation returned by the service.
* @throws InvalidStateTransitionException
* Error reserved for use by the CloudFormation CLI. CloudFormation does not return this error to users.
* @throws OperationStatusCheckFailedException
* Error reserved for use by the CloudFormation CLI. CloudFormation does not return this error to users.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.RecordHandlerProgress
* @see AWS API Documentation
*/
default RecordHandlerProgressResponse recordHandlerProgress(RecordHandlerProgressRequest recordHandlerProgressRequest)
throws InvalidStateTransitionException, OperationStatusCheckFailedException, AwsServiceException, SdkClientException,
CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Reports progress of a resource handler to CloudFormation.
*
*
* Reserved for use by the CloudFormation CLI. Do not use this API in your code.
*
*
*
* This is a convenience which creates an instance of the {@link RecordHandlerProgressRequest.Builder} avoiding the
* need to create one manually via {@link RecordHandlerProgressRequest#builder()}
*
*
* @param recordHandlerProgressRequest
* A {@link Consumer} that will call methods on {@link RecordHandlerProgressInput.Builder} to create a
* request.
* @return Result of the RecordHandlerProgress operation returned by the service.
* @throws InvalidStateTransitionException
* Error reserved for use by the CloudFormation CLI. CloudFormation does not return this error to users.
* @throws OperationStatusCheckFailedException
* Error reserved for use by the CloudFormation CLI. CloudFormation does not return this error to users.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.RecordHandlerProgress
* @see AWS API Documentation
*/
default RecordHandlerProgressResponse recordHandlerProgress(
Consumer recordHandlerProgressRequest) throws InvalidStateTransitionException,
OperationStatusCheckFailedException, AwsServiceException, SdkClientException, CloudFormationException {
return recordHandlerProgress(RecordHandlerProgressRequest.builder().applyMutation(recordHandlerProgressRequest).build());
}
/**
*
* Registers a type with the CloudFormation service. Registering a type makes it available for use in CloudFormation
* templates in your AWS account, and includes:
*
*
* -
*
* Validating the resource schema
*
*
* -
*
* Determining which handlers have been specified for the resource
*
*
* -
*
* Making the resource type available for use in your account
*
*
*
*
* For more information on how to develop types and ready them for registeration, see Creating Resource
* Providers in the CloudFormation CLI User Guide.
*
*
* You can have a maximum of 50 resource type versions registered at a time. This maximum is per account and per
* region. Use DeregisterType to
* deregister specific resource type versions if necessary.
*
*
* Once you have initiated a registration request using RegisterType
, you can use
* DescribeTypeRegistration
to monitor the progress of the registration request.
*
*
* @param registerTypeRequest
* @return Result of the RegisterType operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.RegisterType
* @see AWS
* API Documentation
*/
default RegisterTypeResponse registerType(RegisterTypeRequest registerTypeRequest) throws CfnRegistryException,
AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Registers a type with the CloudFormation service. Registering a type makes it available for use in CloudFormation
* templates in your AWS account, and includes:
*
*
* -
*
* Validating the resource schema
*
*
* -
*
* Determining which handlers have been specified for the resource
*
*
* -
*
* Making the resource type available for use in your account
*
*
*
*
* For more information on how to develop types and ready them for registeration, see Creating Resource
* Providers in the CloudFormation CLI User Guide.
*
*
* You can have a maximum of 50 resource type versions registered at a time. This maximum is per account and per
* region. Use DeregisterType to
* deregister specific resource type versions if necessary.
*
*
* Once you have initiated a registration request using RegisterType
, you can use
* DescribeTypeRegistration
to monitor the progress of the registration request.
*
*
*
* This is a convenience which creates an instance of the {@link RegisterTypeRequest.Builder} avoiding the need to
* create one manually via {@link RegisterTypeRequest#builder()}
*
*
* @param registerTypeRequest
* A {@link Consumer} that will call methods on {@link RegisterTypeInput.Builder} to create a request.
* @return Result of the RegisterType operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.RegisterType
* @see AWS
* API Documentation
*/
default RegisterTypeResponse registerType(Consumer registerTypeRequest)
throws CfnRegistryException, AwsServiceException, SdkClientException, CloudFormationException {
return registerType(RegisterTypeRequest.builder().applyMutation(registerTypeRequest).build());
}
/**
*
* Sets a stack policy for a specified stack.
*
*
* @param setStackPolicyRequest
* The input for the SetStackPolicy action.
* @return Result of the SetStackPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.SetStackPolicy
* @see AWS
* API Documentation
*/
default SetStackPolicyResponse setStackPolicy(SetStackPolicyRequest setStackPolicyRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Sets a stack policy for a specified stack.
*
*
*
* This is a convenience which creates an instance of the {@link SetStackPolicyRequest.Builder} avoiding the need to
* create one manually via {@link SetStackPolicyRequest#builder()}
*
*
* @param setStackPolicyRequest
* A {@link Consumer} that will call methods on {@link SetStackPolicyInput.Builder} to create a request. The
* input for the SetStackPolicy action.
* @return Result of the SetStackPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.SetStackPolicy
* @see AWS
* API Documentation
*/
default SetStackPolicyResponse setStackPolicy(Consumer setStackPolicyRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return setStackPolicy(SetStackPolicyRequest.builder().applyMutation(setStackPolicyRequest).build());
}
/**
*
* Specify the default version of a type. The default version of a type will be used in CloudFormation operations.
*
*
* @param setTypeDefaultVersionRequest
* @return Result of the SetTypeDefaultVersion operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified type does not exist in the CloudFormation registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.SetTypeDefaultVersion
* @see AWS API Documentation
*/
default SetTypeDefaultVersionResponse setTypeDefaultVersion(SetTypeDefaultVersionRequest setTypeDefaultVersionRequest)
throws CfnRegistryException, TypeNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Specify the default version of a type. The default version of a type will be used in CloudFormation operations.
*
*
*
* This is a convenience which creates an instance of the {@link SetTypeDefaultVersionRequest.Builder} avoiding the
* need to create one manually via {@link SetTypeDefaultVersionRequest#builder()}
*
*
* @param setTypeDefaultVersionRequest
* A {@link Consumer} that will call methods on {@link SetTypeDefaultVersionInput.Builder} to create a
* request.
* @return Result of the SetTypeDefaultVersion operation returned by the service.
* @throws CfnRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified type does not exist in the CloudFormation registry.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.SetTypeDefaultVersion
* @see AWS API Documentation
*/
default SetTypeDefaultVersionResponse setTypeDefaultVersion(
Consumer setTypeDefaultVersionRequest) throws CfnRegistryException,
TypeNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
return setTypeDefaultVersion(SetTypeDefaultVersionRequest.builder().applyMutation(setTypeDefaultVersionRequest).build());
}
/**
*
* Sends a signal to the specified resource with a success or failure status. You can use the SignalResource API in
* conjunction with a creation policy or update policy. AWS CloudFormation doesn't proceed with a stack creation or
* update until resources receive the required number of signals or the timeout period is exceeded. The
* SignalResource API is useful in cases where you want to send signals from anywhere other than an Amazon EC2
* instance.
*
*
* @param signalResourceRequest
* The input for the SignalResource action.
* @return Result of the SignalResource operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.SignalResource
* @see AWS
* API Documentation
*/
default SignalResourceResponse signalResource(SignalResourceRequest signalResourceRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Sends a signal to the specified resource with a success or failure status. You can use the SignalResource API in
* conjunction with a creation policy or update policy. AWS CloudFormation doesn't proceed with a stack creation or
* update until resources receive the required number of signals or the timeout period is exceeded. The
* SignalResource API is useful in cases where you want to send signals from anywhere other than an Amazon EC2
* instance.
*
*
*
* This is a convenience which creates an instance of the {@link SignalResourceRequest.Builder} avoiding the need to
* create one manually via {@link SignalResourceRequest#builder()}
*
*
* @param signalResourceRequest
* A {@link Consumer} that will call methods on {@link SignalResourceInput.Builder} to create a request. The
* input for the SignalResource action.
* @return Result of the SignalResource operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.SignalResource
* @see AWS
* API Documentation
*/
default SignalResourceResponse signalResource(Consumer signalResourceRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return signalResource(SignalResourceRequest.builder().applyMutation(signalResourceRequest).build());
}
/**
*
* Stops an in-progress operation on a stack set and its associated stack instances.
*
*
* @param stopStackSetOperationRequest
* @return Result of the StopStackSetOperation operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.StopStackSetOperation
* @see AWS API Documentation
*/
default StopStackSetOperationResponse stopStackSetOperation(StopStackSetOperationRequest stopStackSetOperationRequest)
throws StackSetNotFoundException, OperationNotFoundException, InvalidOperationException, AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Stops an in-progress operation on a stack set and its associated stack instances.
*
*
*
* This is a convenience which creates an instance of the {@link StopStackSetOperationRequest.Builder} avoiding the
* need to create one manually via {@link StopStackSetOperationRequest#builder()}
*
*
* @param stopStackSetOperationRequest
* A {@link Consumer} that will call methods on {@link StopStackSetOperationInput.Builder} to create a
* request.
* @return Result of the StopStackSetOperation operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.StopStackSetOperation
* @see AWS API Documentation
*/
default StopStackSetOperationResponse stopStackSetOperation(
Consumer stopStackSetOperationRequest) throws StackSetNotFoundException,
OperationNotFoundException, InvalidOperationException, AwsServiceException, SdkClientException,
CloudFormationException {
return stopStackSetOperation(StopStackSetOperationRequest.builder().applyMutation(stopStackSetOperationRequest).build());
}
/**
*
* Updates a stack as specified in the template. After the call completes successfully, the stack update starts. You
* can check the status of the stack via the DescribeStacks action.
*
*
* To get a copy of the template for an existing stack, you can use the GetTemplate action.
*
*
* For more information about creating an update template, updating a stack, and monitoring the progress of the
* update, see Updating a
* Stack.
*
*
* @param updateStackRequest
* The input for an UpdateStack action.
* @return Result of the UpdateStack operation returned by the service.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.UpdateStack
* @see AWS API
* Documentation
*/
default UpdateStackResponse updateStack(UpdateStackRequest updateStackRequest) throws InsufficientCapabilitiesException,
TokenAlreadyExistsException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a stack as specified in the template. After the call completes successfully, the stack update starts. You
* can check the status of the stack via the DescribeStacks action.
*
*
* To get a copy of the template for an existing stack, you can use the GetTemplate action.
*
*
* For more information about creating an update template, updating a stack, and monitoring the progress of the
* update, see Updating a
* Stack.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateStackRequest.Builder} avoiding the need to
* create one manually via {@link UpdateStackRequest#builder()}
*
*
* @param updateStackRequest
* A {@link Consumer} that will call methods on {@link UpdateStackInput.Builder} to create a request. The
* input for an UpdateStack action.
* @return Result of the UpdateStack operation returned by the service.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.UpdateStack
* @see AWS API
* Documentation
*/
default UpdateStackResponse updateStack(Consumer updateStackRequest)
throws InsufficientCapabilitiesException, TokenAlreadyExistsException, AwsServiceException, SdkClientException,
CloudFormationException {
return updateStack(UpdateStackRequest.builder().applyMutation(updateStackRequest).build());
}
/**
*
* Updates the parameter values for stack instances for the specified accounts, within the specified Regions. A
* stack instance refers to a stack in a specific account and Region.
*
*
* You can only update stack instances in Regions and accounts where they already exist; to create additional stack
* instances, use CreateStackInstances.
*
*
* During stack set updates, any parameters overridden for a stack instance are not updated, but retain their
* overridden value.
*
*
* You can only update the parameter values that are specified in the stack set; to add or delete a parameter
* itself, use UpdateStackSet
* to update the stack set template. If you add a parameter to a template, before you can override the
* parameter value specified in the stack set you must first use UpdateStackSet to update all stack instances with the updated template and parameter value specified in the
* stack set. Once a stack instance has been updated with the new parameter, you can then override the parameter
* value using UpdateStackInstances
.
*
*
* @param updateStackInstancesRequest
* @return Result of the UpdateStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws StackInstanceNotFoundException
* The specified stack instance doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.UpdateStackInstances
* @see AWS API Documentation
*/
default UpdateStackInstancesResponse updateStackInstances(UpdateStackInstancesRequest updateStackInstancesRequest)
throws StackSetNotFoundException, StackInstanceNotFoundException, OperationInProgressException,
OperationIdAlreadyExistsException, StaleRequestException, InvalidOperationException, AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the parameter values for stack instances for the specified accounts, within the specified Regions. A
* stack instance refers to a stack in a specific account and Region.
*
*
* You can only update stack instances in Regions and accounts where they already exist; to create additional stack
* instances, use CreateStackInstances.
*
*
* During stack set updates, any parameters overridden for a stack instance are not updated, but retain their
* overridden value.
*
*
* You can only update the parameter values that are specified in the stack set; to add or delete a parameter
* itself, use UpdateStackSet
* to update the stack set template. If you add a parameter to a template, before you can override the
* parameter value specified in the stack set you must first use UpdateStackSet to update all stack instances with the updated template and parameter value specified in the
* stack set. Once a stack instance has been updated with the new parameter, you can then override the parameter
* value using UpdateStackInstances
.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateStackInstancesRequest.Builder} avoiding the
* need to create one manually via {@link UpdateStackInstancesRequest#builder()}
*
*
* @param updateStackInstancesRequest
* A {@link Consumer} that will call methods on {@link UpdateStackInstancesInput.Builder} to create a
* request.
* @return Result of the UpdateStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws StackInstanceNotFoundException
* The specified stack instance doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.UpdateStackInstances
* @see AWS API Documentation
*/
default UpdateStackInstancesResponse updateStackInstances(
Consumer updateStackInstancesRequest) throws StackSetNotFoundException,
StackInstanceNotFoundException, OperationInProgressException, OperationIdAlreadyExistsException,
StaleRequestException, InvalidOperationException, AwsServiceException, SdkClientException, CloudFormationException {
return updateStackInstances(UpdateStackInstancesRequest.builder().applyMutation(updateStackInstancesRequest).build());
}
/**
*
* Updates the stack set, and associated stack instances in the specified accounts and Regions.
*
*
* Even if the stack set operation created by updating the stack set fails (completely or partially, below or above
* a specified failure tolerance), the stack set is updated with your changes. Subsequent
* CreateStackInstances calls on the specified stack set use the updated stack set.
*
*
* @param updateStackSetRequest
* @return Result of the UpdateStackSet operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws StackInstanceNotFoundException
* The specified stack instance doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.UpdateStackSet
* @see AWS
* API Documentation
*/
default UpdateStackSetResponse updateStackSet(UpdateStackSetRequest updateStackSetRequest) throws StackSetNotFoundException,
OperationInProgressException, OperationIdAlreadyExistsException, StaleRequestException, InvalidOperationException,
StackInstanceNotFoundException, AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the stack set, and associated stack instances in the specified accounts and Regions.
*
*
* Even if the stack set operation created by updating the stack set fails (completely or partially, below or above
* a specified failure tolerance), the stack set is updated with your changes. Subsequent
* CreateStackInstances calls on the specified stack set use the updated stack set.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateStackSetRequest.Builder} avoiding the need to
* create one manually via {@link UpdateStackSetRequest#builder()}
*
*
* @param updateStackSetRequest
* A {@link Consumer} that will call methods on {@link UpdateStackSetInput.Builder} to create a request.
* @return Result of the UpdateStackSet operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws StackInstanceNotFoundException
* The specified stack instance doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.UpdateStackSet
* @see AWS
* API Documentation
*/
default UpdateStackSetResponse updateStackSet(Consumer updateStackSetRequest)
throws StackSetNotFoundException, OperationInProgressException, OperationIdAlreadyExistsException,
StaleRequestException, InvalidOperationException, StackInstanceNotFoundException, AwsServiceException,
SdkClientException, CloudFormationException {
return updateStackSet(UpdateStackSetRequest.builder().applyMutation(updateStackSetRequest).build());
}
/**
*
* Updates termination protection for the specified stack. If a user attempts to delete a stack with termination
* protection enabled, the operation fails and the stack remains unchanged. For more information, see Protecting a
* Stack From Being Deleted in the AWS CloudFormation User Guide.
*
*
* For nested
* stacks, termination protection is set on the root stack and cannot be changed directly on the nested stack.
*
*
* @param updateTerminationProtectionRequest
* @return Result of the UpdateTerminationProtection operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.UpdateTerminationProtection
* @see AWS API Documentation
*/
default UpdateTerminationProtectionResponse updateTerminationProtection(
UpdateTerminationProtectionRequest updateTerminationProtectionRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Updates termination protection for the specified stack. If a user attempts to delete a stack with termination
* protection enabled, the operation fails and the stack remains unchanged. For more information, see Protecting a
* Stack From Being Deleted in the AWS CloudFormation User Guide.
*
*
* For nested
* stacks, termination protection is set on the root stack and cannot be changed directly on the nested stack.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateTerminationProtectionRequest.Builder}
* avoiding the need to create one manually via {@link UpdateTerminationProtectionRequest#builder()}
*
*
* @param updateTerminationProtectionRequest
* A {@link Consumer} that will call methods on {@link UpdateTerminationProtectionInput.Builder} to create a
* request.
* @return Result of the UpdateTerminationProtection operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.UpdateTerminationProtection
* @see AWS API Documentation
*/
default UpdateTerminationProtectionResponse updateTerminationProtection(
Consumer updateTerminationProtectionRequest) throws AwsServiceException,
SdkClientException, CloudFormationException {
return updateTerminationProtection(UpdateTerminationProtectionRequest.builder()
.applyMutation(updateTerminationProtectionRequest).build());
}
/**
*
* Validates a specified template. AWS CloudFormation first checks if the template is valid JSON. If it isn't, AWS
* CloudFormation checks if the template is valid YAML. If both these checks fail, AWS CloudFormation returns a
* template validation error.
*
*
* @param validateTemplateRequest
* The input for ValidateTemplate action.
* @return Result of the ValidateTemplate operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ValidateTemplate
* @see AWS API Documentation
*/
default ValidateTemplateResponse validateTemplate(ValidateTemplateRequest validateTemplateRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
throw new UnsupportedOperationException();
}
/**
*
* Validates a specified template. AWS CloudFormation first checks if the template is valid JSON. If it isn't, AWS
* CloudFormation checks if the template is valid YAML. If both these checks fail, AWS CloudFormation returns a
* template validation error.
*
*
*
* This is a convenience which creates an instance of the {@link ValidateTemplateRequest.Builder} avoiding the need
* to create one manually via {@link ValidateTemplateRequest#builder()}
*
*
* @param validateTemplateRequest
* A {@link Consumer} that will call methods on {@link ValidateTemplateInput.Builder} to create a request.
* The input for ValidateTemplate action.
* @return Result of the ValidateTemplate operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFormationException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFormationClient.ValidateTemplate
* @see AWS API Documentation
*/
default ValidateTemplateResponse validateTemplate(Consumer validateTemplateRequest)
throws AwsServiceException, SdkClientException, CloudFormationException {
return validateTemplate(ValidateTemplateRequest.builder().applyMutation(validateTemplateRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("cloudformation");
}
}