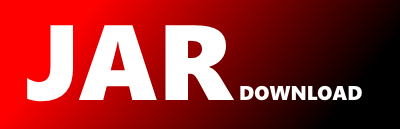
software.amazon.awssdk.services.cloudformation.model.StackSetSummary Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The structures that contain summary information about the specified stack set.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class StackSetSummary implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField STACK_SET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackSetName").getter(getter(StackSetSummary::stackSetName)).setter(setter(Builder::stackSetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackSetName").build()).build();
private static final SdkField STACK_SET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackSetId").getter(getter(StackSetSummary::stackSetId)).setter(setter(Builder::stackSetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackSetId").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(StackSetSummary::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(StackSetSummary::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField AUTO_DEPLOYMENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AutoDeployment")
.getter(getter(StackSetSummary::autoDeployment)).setter(setter(Builder::autoDeployment))
.constructor(AutoDeployment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoDeployment").build()).build();
private static final SdkField PERMISSION_MODEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PermissionModel").getter(getter(StackSetSummary::permissionModelAsString))
.setter(setter(Builder::permissionModel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PermissionModel").build()).build();
private static final SdkField DRIFT_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DriftStatus").getter(getter(StackSetSummary::driftStatusAsString)).setter(setter(Builder::driftStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DriftStatus").build()).build();
private static final SdkField LAST_DRIFT_CHECK_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastDriftCheckTimestamp").getter(getter(StackSetSummary::lastDriftCheckTimestamp))
.setter(setter(Builder::lastDriftCheckTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastDriftCheckTimestamp").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STACK_SET_NAME_FIELD,
STACK_SET_ID_FIELD, DESCRIPTION_FIELD, STATUS_FIELD, AUTO_DEPLOYMENT_FIELD, PERMISSION_MODEL_FIELD,
DRIFT_STATUS_FIELD, LAST_DRIFT_CHECK_TIMESTAMP_FIELD));
private static final long serialVersionUID = 1L;
private final String stackSetName;
private final String stackSetId;
private final String description;
private final String status;
private final AutoDeployment autoDeployment;
private final String permissionModel;
private final String driftStatus;
private final Instant lastDriftCheckTimestamp;
private StackSetSummary(BuilderImpl builder) {
this.stackSetName = builder.stackSetName;
this.stackSetId = builder.stackSetId;
this.description = builder.description;
this.status = builder.status;
this.autoDeployment = builder.autoDeployment;
this.permissionModel = builder.permissionModel;
this.driftStatus = builder.driftStatus;
this.lastDriftCheckTimestamp = builder.lastDriftCheckTimestamp;
}
/**
*
* The name of the stack set.
*
*
* @return The name of the stack set.
*/
public final String stackSetName() {
return stackSetName;
}
/**
*
* The ID of the stack set.
*
*
* @return The ID of the stack set.
*/
public final String stackSetId() {
return stackSetId;
}
/**
*
* A description of the stack set that you specify when the stack set is created or updated.
*
*
* @return A description of the stack set that you specify when the stack set is created or updated.
*/
public final String description() {
return description;
}
/**
*
* The status of the stack set.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link StackSetStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the stack set.
* @see StackSetStatus
*/
public final StackSetStatus status() {
return StackSetStatus.fromValue(status);
}
/**
*
* The status of the stack set.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link StackSetStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the stack set.
* @see StackSetStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* [Service-managed permissions] Describes whether StackSets automatically deploys to AWS Organizations accounts
* that are added to a target organizational unit (OU).
*
*
* @return [Service-managed permissions] Describes whether StackSets automatically deploys to AWS Organizations
* accounts that are added to a target organizational unit (OU).
*/
public final AutoDeployment autoDeployment() {
return autoDeployment;
}
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by AWS Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #permissionModel}
* will return {@link PermissionModels#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #permissionModelAsString}.
*
*
* @return Describes how the IAM roles required for stack set operations are created.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles
* required to deploy to target accounts. For more information, see Grant Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by AWS Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
*/
public final PermissionModels permissionModel() {
return PermissionModels.fromValue(permissionModel);
}
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by AWS Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #permissionModel}
* will return {@link PermissionModels#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #permissionModelAsString}.
*
*
* @return Describes how the IAM roles required for stack set operations are created.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles
* required to deploy to target accounts. For more information, see Grant Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by AWS Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
*/
public final String permissionModelAsString() {
return permissionModel;
}
/**
*
* Status of the stack set's actual configuration compared to its expected template and parameter configuration. A
* stack set is considered to have drifted if one or more of its stack instances have drifted from their expected
* template and parameter configuration.
*
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from the
* expected template and parameter configuration. A stack instance is considered to have drifted if one or more of
* the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation has not checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All of the stack instances belonging to the stack set stack match from the expected
* template and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #driftStatus} will
* return {@link StackDriftStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #driftStatusAsString}.
*
*
* @return Status of the stack set's actual configuration compared to its expected template and parameter
* configuration. A stack set is considered to have drifted if one or more of its stack instances have
* drifted from their expected template and parameter configuration.
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from
* the expected template and parameter configuration. A stack instance is considered to have drifted if one
* or more of the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation has not checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All of the stack instances belonging to the stack set stack match from the expected
* template and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @see StackDriftStatus
*/
public final StackDriftStatus driftStatus() {
return StackDriftStatus.fromValue(driftStatus);
}
/**
*
* Status of the stack set's actual configuration compared to its expected template and parameter configuration. A
* stack set is considered to have drifted if one or more of its stack instances have drifted from their expected
* template and parameter configuration.
*
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from the
* expected template and parameter configuration. A stack instance is considered to have drifted if one or more of
* the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation has not checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All of the stack instances belonging to the stack set stack match from the expected
* template and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #driftStatus} will
* return {@link StackDriftStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #driftStatusAsString}.
*
*
* @return Status of the stack set's actual configuration compared to its expected template and parameter
* configuration. A stack set is considered to have drifted if one or more of its stack instances have
* drifted from their expected template and parameter configuration.
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from
* the expected template and parameter configuration. A stack instance is considered to have drifted if one
* or more of the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation has not checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All of the stack instances belonging to the stack set stack match from the expected
* template and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @see StackDriftStatus
*/
public final String driftStatusAsString() {
return driftStatus;
}
/**
*
* Most recent time when CloudFormation performed a drift detection operation on the stack set. This value will be
* NULL
for any stack set on which drift detection has not yet been performed.
*
*
* @return Most recent time when CloudFormation performed a drift detection operation on the stack set. This value
* will be NULL
for any stack set on which drift detection has not yet been performed.
*/
public final Instant lastDriftCheckTimestamp() {
return lastDriftCheckTimestamp;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(stackSetName());
hashCode = 31 * hashCode + Objects.hashCode(stackSetId());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(autoDeployment());
hashCode = 31 * hashCode + Objects.hashCode(permissionModelAsString());
hashCode = 31 * hashCode + Objects.hashCode(driftStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(lastDriftCheckTimestamp());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StackSetSummary)) {
return false;
}
StackSetSummary other = (StackSetSummary) obj;
return Objects.equals(stackSetName(), other.stackSetName()) && Objects.equals(stackSetId(), other.stackSetId())
&& Objects.equals(description(), other.description()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(autoDeployment(), other.autoDeployment())
&& Objects.equals(permissionModelAsString(), other.permissionModelAsString())
&& Objects.equals(driftStatusAsString(), other.driftStatusAsString())
&& Objects.equals(lastDriftCheckTimestamp(), other.lastDriftCheckTimestamp());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StackSetSummary").add("StackSetName", stackSetName()).add("StackSetId", stackSetId())
.add("Description", description()).add("Status", statusAsString()).add("AutoDeployment", autoDeployment())
.add("PermissionModel", permissionModelAsString()).add("DriftStatus", driftStatusAsString())
.add("LastDriftCheckTimestamp", lastDriftCheckTimestamp()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackSetName":
return Optional.ofNullable(clazz.cast(stackSetName()));
case "StackSetId":
return Optional.ofNullable(clazz.cast(stackSetId()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "AutoDeployment":
return Optional.ofNullable(clazz.cast(autoDeployment()));
case "PermissionModel":
return Optional.ofNullable(clazz.cast(permissionModelAsString()));
case "DriftStatus":
return Optional.ofNullable(clazz.cast(driftStatusAsString()));
case "LastDriftCheckTimestamp":
return Optional.ofNullable(clazz.cast(lastDriftCheckTimestamp()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function