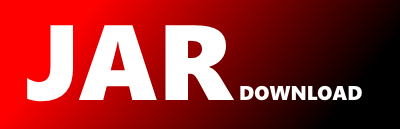
software.amazon.awssdk.services.cloudformation.model.ContinueUpdateRollbackRequest Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The input for the ContinueUpdateRollback action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ContinueUpdateRollbackRequest extends CloudFormationRequest implements
ToCopyableBuilder {
private static final SdkField STACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackName").getter(getter(ContinueUpdateRollbackRequest::stackName)).setter(setter(Builder::stackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackName").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleARN").getter(getter(ContinueUpdateRollbackRequest::roleARN)).setter(setter(Builder::roleARN))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleARN").build()).build();
private static final SdkField> RESOURCES_TO_SKIP_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ResourcesToSkip")
.getter(getter(ContinueUpdateRollbackRequest::resourcesToSkip))
.setter(setter(Builder::resourcesToSkip))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourcesToSkip").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CLIENT_REQUEST_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClientRequestToken").getter(getter(ContinueUpdateRollbackRequest::clientRequestToken))
.setter(setter(Builder::clientRequestToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientRequestToken").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STACK_NAME_FIELD,
ROLE_ARN_FIELD, RESOURCES_TO_SKIP_FIELD, CLIENT_REQUEST_TOKEN_FIELD));
private final String stackName;
private final String roleARN;
private final List resourcesToSkip;
private final String clientRequestToken;
private ContinueUpdateRollbackRequest(BuilderImpl builder) {
super(builder);
this.stackName = builder.stackName;
this.roleARN = builder.roleARN;
this.resourcesToSkip = builder.resourcesToSkip;
this.clientRequestToken = builder.clientRequestToken;
}
/**
*
* The name or the unique ID of the stack that you want to continue rolling back.
*
*
*
* Don't specify the name of a nested stack (a stack that was created by using the
* AWS::CloudFormation::Stack
resource). Instead, use this operation on the parent stack (the stack
* that contains the AWS::CloudFormation::Stack
resource).
*
*
*
* @return The name or the unique ID of the stack that you want to continue rolling back.
*
* Don't specify the name of a nested stack (a stack that was created by using the
* AWS::CloudFormation::Stack
resource). Instead, use this operation on the parent stack (the
* stack that contains the AWS::CloudFormation::Stack
resource).
*
*/
public final String stackName() {
return stackName;
}
/**
*
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation assumes to
* roll back the stack. CloudFormation uses the role's credentials to make calls on your behalf. CloudFormation
* always uses this role for all future operations on the stack. Provided that users have permission to operate on
* the stack, CloudFormation uses this role even if the users don't have permission to pass it. Ensure that the role
* grants least permission.
*
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack. If no
* role is available, CloudFormation uses a temporary session that's generated from your user credentials.
*
*
* @return The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation
* assumes to roll back the stack. CloudFormation uses the role's credentials to make calls on your behalf.
* CloudFormation always uses this role for all future operations on the stack. Provided that users have
* permission to operate on the stack, CloudFormation uses this role even if the users don't have permission
* to pass it. Ensure that the role grants least permission.
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack.
* If no role is available, CloudFormation uses a temporary session that's generated from your user
* credentials.
*/
public final String roleARN() {
return roleARN;
}
/**
* For responses, this returns true if the service returned a value for the ResourcesToSkip property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasResourcesToSkip() {
return resourcesToSkip != null && !(resourcesToSkip instanceof SdkAutoConstructList);
}
/**
*
* A list of the logical IDs of the resources that CloudFormation skips during the continue update rollback
* operation. You can specify only resources that are in the UPDATE_FAILED
state because a rollback
* failed. You can't specify resources that are in the UPDATE_FAILED
state for other reasons, for
* example, because an update was canceled. To check why a resource update failed, use the
* DescribeStackResources action, and view the resource status reason.
*
*
*
* Specify this property to skip rolling back resources that CloudFormation can't successfully roll back. We
* recommend that you troubleshoot resources before skipping them. CloudFormation sets the status of the specified resources to
* UPDATE_COMPLETE
and continues to roll back the stack. After the rollback is complete, the state of
* the skipped resources will be inconsistent with the state of the resources in the stack template. Before
* performing another stack update, you must update the stack or resources to be consistent with each other. If you
* don't, subsequent stack updates might fail, and the stack will become unrecoverable.
*
*
*
* Specify the minimum number of resources required to successfully roll back your stack. For example, a failed
* resource update might cause dependent resources to fail. In this case, it might not be necessary to skip the
* dependent resources.
*
*
* To skip resources that are part of nested stacks, use the following format:
* NestedStackName.ResourceLogicalID
. If you want to specify the logical ID of a stack resource (
* Type: AWS::CloudFormation::Stack
) in the ResourcesToSkip
list, then its corresponding
* embedded stack must be in one of the following states: DELETE_IN_PROGRESS
,
* DELETE_COMPLETE
, or DELETE_FAILED
.
*
*
*
* Don't confuse a child stack's name with its corresponding logical ID defined in the parent stack. For an example
* of a continue update rollback operation with nested stacks, see Using ResourcesToSkip to recover a nested stacks hierarchy.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasResourcesToSkip} method.
*
*
* @return A list of the logical IDs of the resources that CloudFormation skips during the continue update rollback
* operation. You can specify only resources that are in the UPDATE_FAILED
state because a
* rollback failed. You can't specify resources that are in the UPDATE_FAILED
state for other
* reasons, for example, because an update was canceled. To check why a resource update failed, use the
* DescribeStackResources action, and view the resource status reason.
*
* Specify this property to skip rolling back resources that CloudFormation can't successfully roll back. We
* recommend that you troubleshoot resources before skipping them. CloudFormation sets the status of the specified
* resources to UPDATE_COMPLETE
and continues to roll back the stack. After the rollback is
* complete, the state of the skipped resources will be inconsistent with the state of the resources in the
* stack template. Before performing another stack update, you must update the stack or resources to be
* consistent with each other. If you don't, subsequent stack updates might fail, and the stack will become
* unrecoverable.
*
*
*
* Specify the minimum number of resources required to successfully roll back your stack. For example, a
* failed resource update might cause dependent resources to fail. In this case, it might not be necessary
* to skip the dependent resources.
*
*
* To skip resources that are part of nested stacks, use the following format:
* NestedStackName.ResourceLogicalID
. If you want to specify the logical ID of a stack resource
* (Type: AWS::CloudFormation::Stack
) in the ResourcesToSkip
list, then its
* corresponding embedded stack must be in one of the following states: DELETE_IN_PROGRESS
,
* DELETE_COMPLETE
, or DELETE_FAILED
.
*
*
*
* Don't confuse a child stack's name with its corresponding logical ID defined in the parent stack. For an
* example of a continue update rollback operation with nested stacks, see Using ResourcesToSkip to recover a nested stacks hierarchy.
*
*/
public final List resourcesToSkip() {
return resourcesToSkip;
}
/**
*
* A unique identifier for this ContinueUpdateRollback
request. Specify this token if you plan to retry
* requests so that CloudFormationknows that you're not attempting to continue the rollback to a stack with the same
* name. You might retry ContinueUpdateRollback
requests to ensure that CloudFormation successfully
* received them.
*
*
* @return A unique identifier for this ContinueUpdateRollback
request. Specify this token if you plan
* to retry requests so that CloudFormationknows that you're not attempting to continue the rollback to a
* stack with the same name. You might retry ContinueUpdateRollback
requests to ensure that
* CloudFormation successfully received them.
*/
public final String clientRequestToken() {
return clientRequestToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(stackName());
hashCode = 31 * hashCode + Objects.hashCode(roleARN());
hashCode = 31 * hashCode + Objects.hashCode(hasResourcesToSkip() ? resourcesToSkip() : null);
hashCode = 31 * hashCode + Objects.hashCode(clientRequestToken());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ContinueUpdateRollbackRequest)) {
return false;
}
ContinueUpdateRollbackRequest other = (ContinueUpdateRollbackRequest) obj;
return Objects.equals(stackName(), other.stackName()) && Objects.equals(roleARN(), other.roleARN())
&& hasResourcesToSkip() == other.hasResourcesToSkip()
&& Objects.equals(resourcesToSkip(), other.resourcesToSkip())
&& Objects.equals(clientRequestToken(), other.clientRequestToken());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ContinueUpdateRollbackRequest").add("StackName", stackName()).add("RoleARN", roleARN())
.add("ResourcesToSkip", hasResourcesToSkip() ? resourcesToSkip() : null)
.add("ClientRequestToken", clientRequestToken()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackName":
return Optional.ofNullable(clazz.cast(stackName()));
case "RoleARN":
return Optional.ofNullable(clazz.cast(roleARN()));
case "ResourcesToSkip":
return Optional.ofNullable(clazz.cast(resourcesToSkip()));
case "ClientRequestToken":
return Optional.ofNullable(clazz.cast(clientRequestToken()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function