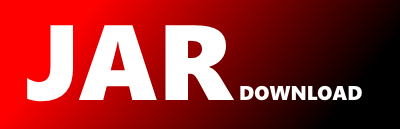
software.amazon.awssdk.services.cloudformation.model.CreateChangeSetRequest Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The input for the CreateChangeSet action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateChangeSetRequest extends CloudFormationRequest implements
ToCopyableBuilder {
private static final SdkField STACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackName").getter(getter(CreateChangeSetRequest::stackName)).setter(setter(Builder::stackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackName").build()).build();
private static final SdkField TEMPLATE_BODY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateBody").getter(getter(CreateChangeSetRequest::templateBody))
.setter(setter(Builder::templateBody))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateBody").build()).build();
private static final SdkField TEMPLATE_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateURL").getter(getter(CreateChangeSetRequest::templateURL)).setter(setter(Builder::templateURL))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateURL").build()).build();
private static final SdkField USE_PREVIOUS_TEMPLATE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("UsePreviousTemplate").getter(getter(CreateChangeSetRequest::usePreviousTemplate))
.setter(setter(Builder::usePreviousTemplate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UsePreviousTemplate").build())
.build();
private static final SdkField> PARAMETERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Parameters")
.getter(getter(CreateChangeSetRequest::parameters))
.setter(setter(Builder::parameters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Parameters").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Parameter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CAPABILITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Capabilities")
.getter(getter(CreateChangeSetRequest::capabilitiesAsStrings))
.setter(setter(Builder::capabilitiesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Capabilities").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> RESOURCE_TYPES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ResourceTypes")
.getter(getter(CreateChangeSetRequest::resourceTypes))
.setter(setter(Builder::resourceTypes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceTypes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleARN").getter(getter(CreateChangeSetRequest::roleARN)).setter(setter(Builder::roleARN))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleARN").build()).build();
private static final SdkField ROLLBACK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("RollbackConfiguration")
.getter(getter(CreateChangeSetRequest::rollbackConfiguration)).setter(setter(Builder::rollbackConfiguration))
.constructor(RollbackConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RollbackConfiguration").build())
.build();
private static final SdkField> NOTIFICATION_AR_NS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NotificationARNs")
.getter(getter(CreateChangeSetRequest::notificationARNs))
.setter(setter(Builder::notificationARNs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationARNs").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateChangeSetRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CHANGE_SET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ChangeSetName").getter(getter(CreateChangeSetRequest::changeSetName))
.setter(setter(Builder::changeSetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChangeSetName").build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClientToken").getter(getter(CreateChangeSetRequest::clientToken)).setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientToken").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CreateChangeSetRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField CHANGE_SET_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ChangeSetType").getter(getter(CreateChangeSetRequest::changeSetTypeAsString))
.setter(setter(Builder::changeSetType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChangeSetType").build()).build();
private static final SdkField> RESOURCES_TO_IMPORT_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ResourcesToImport")
.getter(getter(CreateChangeSetRequest::resourcesToImport))
.setter(setter(Builder::resourcesToImport))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourcesToImport").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ResourceToImport::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INCLUDE_NESTED_STACKS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IncludeNestedStacks").getter(getter(CreateChangeSetRequest::includeNestedStacks))
.setter(setter(Builder::includeNestedStacks))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludeNestedStacks").build())
.build();
private static final SdkField ON_STACK_FAILURE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OnStackFailure").getter(getter(CreateChangeSetRequest::onStackFailureAsString))
.setter(setter(Builder::onStackFailure))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OnStackFailure").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STACK_NAME_FIELD,
TEMPLATE_BODY_FIELD, TEMPLATE_URL_FIELD, USE_PREVIOUS_TEMPLATE_FIELD, PARAMETERS_FIELD, CAPABILITIES_FIELD,
RESOURCE_TYPES_FIELD, ROLE_ARN_FIELD, ROLLBACK_CONFIGURATION_FIELD, NOTIFICATION_AR_NS_FIELD, TAGS_FIELD,
CHANGE_SET_NAME_FIELD, CLIENT_TOKEN_FIELD, DESCRIPTION_FIELD, CHANGE_SET_TYPE_FIELD, RESOURCES_TO_IMPORT_FIELD,
INCLUDE_NESTED_STACKS_FIELD, ON_STACK_FAILURE_FIELD));
private final String stackName;
private final String templateBody;
private final String templateURL;
private final Boolean usePreviousTemplate;
private final List parameters;
private final List capabilities;
private final List resourceTypes;
private final String roleARN;
private final RollbackConfiguration rollbackConfiguration;
private final List notificationARNs;
private final List tags;
private final String changeSetName;
private final String clientToken;
private final String description;
private final String changeSetType;
private final List resourcesToImport;
private final Boolean includeNestedStacks;
private final String onStackFailure;
private CreateChangeSetRequest(BuilderImpl builder) {
super(builder);
this.stackName = builder.stackName;
this.templateBody = builder.templateBody;
this.templateURL = builder.templateURL;
this.usePreviousTemplate = builder.usePreviousTemplate;
this.parameters = builder.parameters;
this.capabilities = builder.capabilities;
this.resourceTypes = builder.resourceTypes;
this.roleARN = builder.roleARN;
this.rollbackConfiguration = builder.rollbackConfiguration;
this.notificationARNs = builder.notificationARNs;
this.tags = builder.tags;
this.changeSetName = builder.changeSetName;
this.clientToken = builder.clientToken;
this.description = builder.description;
this.changeSetType = builder.changeSetType;
this.resourcesToImport = builder.resourcesToImport;
this.includeNestedStacks = builder.includeNestedStacks;
this.onStackFailure = builder.onStackFailure;
}
/**
*
* The name or the unique ID of the stack for which you are creating a change set. CloudFormation generates the
* change set by comparing this stack's information with the information that you submit, such as a modified
* template or different parameter input values.
*
*
* @return The name or the unique ID of the stack for which you are creating a change set. CloudFormation generates
* the change set by comparing this stack's information with the information that you submit, such as a
* modified template or different parameter input values.
*/
public final String stackName() {
return stackName;
}
/**
*
* A structure that contains the body of the revised template, with a minimum length of 1 byte and a maximum length
* of 51,200 bytes. CloudFormation generates the change set by comparing this template with the template of the
* stack that you specified.
*
*
* Conditional: You must specify only TemplateBody
or TemplateURL
.
*
*
* @return A structure that contains the body of the revised template, with a minimum length of 1 byte and a maximum
* length of 51,200 bytes. CloudFormation generates the change set by comparing this template with the
* template of the stack that you specified.
*
* Conditional: You must specify only TemplateBody
or TemplateURL
.
*/
public final String templateBody() {
return templateBody;
}
/**
*
* The location of the file that contains the revised template. The URL must point to a template (max size: 460,800
* bytes) that's located in an Amazon S3 bucket or a Systems Manager document. CloudFormation generates the change
* set by comparing this template with the stack that you specified.
*
*
* Conditional: You must specify only TemplateBody
or TemplateURL
.
*
*
* @return The location of the file that contains the revised template. The URL must point to a template (max size:
* 460,800 bytes) that's located in an Amazon S3 bucket or a Systems Manager document. CloudFormation
* generates the change set by comparing this template with the stack that you specified.
*
* Conditional: You must specify only TemplateBody
or TemplateURL
.
*/
public final String templateURL() {
return templateURL;
}
/**
*
* Whether to reuse the template that's associated with the stack to create the change set.
*
*
* @return Whether to reuse the template that's associated with the stack to create the change set.
*/
public final Boolean usePreviousTemplate() {
return usePreviousTemplate;
}
/**
* For responses, this returns true if the service returned a value for the Parameters property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasParameters() {
return parameters != null && !(parameters instanceof SdkAutoConstructList);
}
/**
*
* A list of Parameter
structures that specify input parameters for the change set. For more
* information, see the Parameter data type.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasParameters} method.
*
*
* @return A list of Parameter
structures that specify input parameters for the change set. For more
* information, see the Parameter data type.
*/
public final List parameters() {
return parameters;
}
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in order
* for CloudFormation to create the stack.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account; for
* example, by creating new Identity and Access Management (IAM) users. For those stacks, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we suggest that you review all permissions associated with them
* and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM resources in CloudFormation templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple actions like
* find-and-replace operations, all the way to extensive transformations of entire templates. Because of this, users
* typically create a change set from the processed template, so that they can review the changes resulting from the
* macros before actually creating the stack. If your stack template contains one or more macros, and you choose to
* create a stack directly from the processed template, without first reviewing the resulting changes in a change
* set, you must acknowledge this capability. This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.
*
*
*
* This capacity doesn't apply to creating change sets, and specifying it when creating change sets has no effect.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you must
* create or update the stack directly from the template using the CreateStack or UpdateStack action,
* and specifying this capability.
*
*
*
* For more information about macros, see Using CloudFormation
* macros to perform custom processing on templates.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapabilities} method.
*
*
* @return In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for CloudFormation to create the stack.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stacks, you
* must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we suggest that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM resources in CloudFormation templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple
* actions like find-and-replace operations, all the way to extensive transformations of entire templates.
* Because of this, users typically create a change set from the processed template, so that they can review
* the changes resulting from the macros before actually creating the stack. If your stack template contains
* one or more macros, and you choose to create a stack directly from the processed template, without first
* reviewing the resulting changes in a change set, you must acknowledge this capability. This includes the
* AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.
*
*
*
* This capacity doesn't apply to creating change sets, and specifying it when creating change sets has no
* effect.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you
* must create or update the stack directly from the template using the CreateStack or
* UpdateStack action, and specifying this capability.
*
*
*
* For more information about macros, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*/
public final List capabilities() {
return CapabilitiesCopier.copyStringToEnum(capabilities);
}
/**
* For responses, this returns true if the service returned a value for the Capabilities property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCapabilities() {
return capabilities != null && !(capabilities instanceof SdkAutoConstructList);
}
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in order
* for CloudFormation to create the stack.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account; for
* example, by creating new Identity and Access Management (IAM) users. For those stacks, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we suggest that you review all permissions associated with them
* and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM resources in CloudFormation templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple actions like
* find-and-replace operations, all the way to extensive transformations of entire templates. Because of this, users
* typically create a change set from the processed template, so that they can review the changes resulting from the
* macros before actually creating the stack. If your stack template contains one or more macros, and you choose to
* create a stack directly from the processed template, without first reviewing the resulting changes in a change
* set, you must acknowledge this capability. This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.
*
*
*
* This capacity doesn't apply to creating change sets, and specifying it when creating change sets has no effect.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you must
* create or update the stack directly from the template using the CreateStack or UpdateStack action,
* and specifying this capability.
*
*
*
* For more information about macros, see Using CloudFormation
* macros to perform custom processing on templates.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapabilities} method.
*
*
* @return In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for CloudFormation to create the stack.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stacks, you
* must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we suggest that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM resources in CloudFormation templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple
* actions like find-and-replace operations, all the way to extensive transformations of entire templates.
* Because of this, users typically create a change set from the processed template, so that they can review
* the changes resulting from the macros before actually creating the stack. If your stack template contains
* one or more macros, and you choose to create a stack directly from the processed template, without first
* reviewing the resulting changes in a change set, you must acknowledge this capability. This includes the
* AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.
*
*
*
* This capacity doesn't apply to creating change sets, and specifying it when creating change sets has no
* effect.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you
* must create or update the stack directly from the template using the CreateStack or
* UpdateStack action, and specifying this capability.
*
*
*
* For more information about macros, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*/
public final List capabilitiesAsStrings() {
return capabilities;
}
/**
* For responses, this returns true if the service returned a value for the ResourceTypes property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasResourceTypes() {
return resourceTypes != null && !(resourceTypes instanceof SdkAutoConstructList);
}
/**
*
* The template resource types that you have permissions to work with if you execute this change set, such as
* AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
.
*
*
* If the list of resource types doesn't include a resource type that you're updating, the stack update fails. By
* default, CloudFormation grants permissions to all resource types. Identity and Access Management (IAM) uses this
* parameter for condition keys in IAM policies for CloudFormation. For more information, see Controlling access
* with Identity and Access Management in the CloudFormation User Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasResourceTypes} method.
*
*
* @return The template resource types that you have permissions to work with if you execute this change set, such
* as AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
* .
*
* If the list of resource types doesn't include a resource type that you're updating, the stack update
* fails. By default, CloudFormation grants permissions to all resource types. Identity and Access
* Management (IAM) uses this parameter for condition keys in IAM policies for CloudFormation. For more
* information, see Controlling
* access with Identity and Access Management in the CloudFormation User Guide.
*/
public final List resourceTypes() {
return resourceTypes;
}
/**
*
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation assumes when
* executing the change set. CloudFormation uses the role's credentials to make calls on your behalf. CloudFormation
* uses this role for all future operations on the stack. Provided that users have permission to operate on the
* stack, CloudFormation uses this role even if the users don't have permission to pass it. Ensure that the role
* grants least permission.
*
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack. If no
* role is available, CloudFormation uses a temporary session that is generated from your user credentials.
*
*
* @return The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation
* assumes when executing the change set. CloudFormation uses the role's credentials to make calls on your
* behalf. CloudFormation uses this role for all future operations on the stack. Provided that users have
* permission to operate on the stack, CloudFormation uses this role even if the users don't have permission
* to pass it. Ensure that the role grants least permission.
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack.
* If no role is available, CloudFormation uses a temporary session that is generated from your user
* credentials.
*/
public final String roleARN() {
return roleARN;
}
/**
*
* The rollback triggers for CloudFormation to monitor during stack creation and updating operations, and for the
* specified monitoring period afterwards.
*
*
* @return The rollback triggers for CloudFormation to monitor during stack creation and updating operations, and
* for the specified monitoring period afterwards.
*/
public final RollbackConfiguration rollbackConfiguration() {
return rollbackConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the NotificationARNs property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNotificationARNs() {
return notificationARNs != null && !(notificationARNs instanceof SdkAutoConstructList);
}
/**
*
* The Amazon Resource Names (ARNs) of Amazon Simple Notification Service (Amazon SNS) topics that CloudFormation
* associates with the stack. To remove all associated notification topics, specify an empty list.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNotificationARNs} method.
*
*
* @return The Amazon Resource Names (ARNs) of Amazon Simple Notification Service (Amazon SNS) topics that
* CloudFormation associates with the stack. To remove all associated notification topics, specify an empty
* list.
*/
public final List notificationARNs() {
return notificationARNs;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* Key-value pairs to associate with this stack. CloudFormation also propagates these tags to resources in the
* stack. You can specify a maximum of 50 tags.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return Key-value pairs to associate with this stack. CloudFormation also propagates these tags to resources in
* the stack. You can specify a maximum of 50 tags.
*/
public final List tags() {
return tags;
}
/**
*
* The name of the change set. The name must be unique among all change sets that are associated with the specified
* stack.
*
*
* A change set name can contain only alphanumeric, case sensitive characters, and hyphens. It must start with an
* alphabetical character and can't exceed 128 characters.
*
*
* @return The name of the change set. The name must be unique among all change sets that are associated with the
* specified stack.
*
* A change set name can contain only alphanumeric, case sensitive characters, and hyphens. It must start
* with an alphabetical character and can't exceed 128 characters.
*/
public final String changeSetName() {
return changeSetName;
}
/**
*
* A unique identifier for this CreateChangeSet
request. Specify this token if you plan to retry
* requests so that CloudFormation knows that you're not attempting to create another change set with the same name.
* You might retry CreateChangeSet
requests to ensure that CloudFormation successfully received them.
*
*
* @return A unique identifier for this CreateChangeSet
request. Specify this token if you plan to
* retry requests so that CloudFormation knows that you're not attempting to create another change set with
* the same name. You might retry CreateChangeSet
requests to ensure that CloudFormation
* successfully received them.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* A description to help you identify this change set.
*
*
* @return A description to help you identify this change set.
*/
public final String description() {
return description;
}
/**
*
* The type of change set operation. To create a change set for a new stack, specify CREATE
. To create
* a change set for an existing stack, specify UPDATE
. To create a change set for an import operation,
* specify IMPORT
.
*
*
* If you create a change set for a new stack, CloudFormation creates a stack with a unique stack ID, but no
* template or resources. The stack will be in the REVIEW_IN_PROGRESS state until you execute the change set.
*
*
* By default, CloudFormation specifies UPDATE
. You can't use the UPDATE
type to create a
* change set for a new stack or the CREATE
type to create a change set for an existing stack.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #changeSetType}
* will return {@link ChangeSetType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #changeSetTypeAsString}.
*
*
* @return The type of change set operation. To create a change set for a new stack, specify CREATE
. To
* create a change set for an existing stack, specify UPDATE
. To create a change set for an
* import operation, specify IMPORT
.
*
* If you create a change set for a new stack, CloudFormation creates a stack with a unique stack ID, but no
* template or resources. The stack will be in the REVIEW_IN_PROGRESS state until you execute the change set.
*
*
* By default, CloudFormation specifies UPDATE
. You can't use the UPDATE
type to
* create a change set for a new stack or the CREATE
type to create a change set for an
* existing stack.
* @see ChangeSetType
*/
public final ChangeSetType changeSetType() {
return ChangeSetType.fromValue(changeSetType);
}
/**
*
* The type of change set operation. To create a change set for a new stack, specify CREATE
. To create
* a change set for an existing stack, specify UPDATE
. To create a change set for an import operation,
* specify IMPORT
.
*
*
* If you create a change set for a new stack, CloudFormation creates a stack with a unique stack ID, but no
* template or resources. The stack will be in the REVIEW_IN_PROGRESS state until you execute the change set.
*
*
* By default, CloudFormation specifies UPDATE
. You can't use the UPDATE
type to create a
* change set for a new stack or the CREATE
type to create a change set for an existing stack.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #changeSetType}
* will return {@link ChangeSetType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #changeSetTypeAsString}.
*
*
* @return The type of change set operation. To create a change set for a new stack, specify CREATE
. To
* create a change set for an existing stack, specify UPDATE
. To create a change set for an
* import operation, specify IMPORT
.
*
* If you create a change set for a new stack, CloudFormation creates a stack with a unique stack ID, but no
* template or resources. The stack will be in the REVIEW_IN_PROGRESS state until you execute the change set.
*
*
* By default, CloudFormation specifies UPDATE
. You can't use the UPDATE
type to
* create a change set for a new stack or the CREATE
type to create a change set for an
* existing stack.
* @see ChangeSetType
*/
public final String changeSetTypeAsString() {
return changeSetType;
}
/**
* For responses, this returns true if the service returned a value for the ResourcesToImport property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasResourcesToImport() {
return resourcesToImport != null && !(resourcesToImport instanceof SdkAutoConstructList);
}
/**
*
* The resources to import into your stack.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasResourcesToImport} method.
*
*
* @return The resources to import into your stack.
*/
public final List resourcesToImport() {
return resourcesToImport;
}
/**
*
* Creates a change set for the all nested stacks specified in the template. The default behavior of this action is
* set to False
. To include nested sets in a change set, specify True
.
*
*
* @return Creates a change set for the all nested stacks specified in the template. The default behavior of this
* action is set to False
. To include nested sets in a change set, specify True
.
*/
public final Boolean includeNestedStacks() {
return includeNestedStacks;
}
/**
*
* Determines what action will be taken if stack creation fails. If this parameter is specified, the
* DisableRollback
parameter to the ExecuteChangeSet API operation must not be specified. This must be one of these values:
*
*
* -
*
* DELETE
- Deletes the change set if the stack creation fails. This is only valid when the
* ChangeSetType
parameter is set to CREATE
. If the deletion of the stack fails, the
* status of the stack is DELETE_FAILED
.
*
*
* -
*
* DO_NOTHING
- if the stack creation fails, do nothing. This is equivalent to specifying
* true
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
* -
*
* ROLLBACK
- if the stack creation fails, roll back the stack. This is equivalent to specifying
* false
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
*
*
* For nested stacks, when the OnStackFailure
parameter is set to DELETE
for the change
* set for the parent stack, any failure in a child stack will cause the parent stack creation to fail and all
* stacks to be deleted.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #onStackFailure}
* will return {@link OnStackFailure#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #onStackFailureAsString}.
*
*
* @return Determines what action will be taken if stack creation fails. If this parameter is specified, the
* DisableRollback
parameter to the ExecuteChangeSet API operation must not be specified. This must be one of these values:
*
* -
*
* DELETE
- Deletes the change set if the stack creation fails. This is only valid when the
* ChangeSetType
parameter is set to CREATE
. If the deletion of the stack fails,
* the status of the stack is DELETE_FAILED
.
*
*
* -
*
* DO_NOTHING
- if the stack creation fails, do nothing. This is equivalent to specifying
* true
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
* -
*
* ROLLBACK
- if the stack creation fails, roll back the stack. This is equivalent to
* specifying false
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
*
*
* For nested stacks, when the OnStackFailure
parameter is set to DELETE
for the
* change set for the parent stack, any failure in a child stack will cause the parent stack creation to
* fail and all stacks to be deleted.
* @see OnStackFailure
*/
public final OnStackFailure onStackFailure() {
return OnStackFailure.fromValue(onStackFailure);
}
/**
*
* Determines what action will be taken if stack creation fails. If this parameter is specified, the
* DisableRollback
parameter to the ExecuteChangeSet API operation must not be specified. This must be one of these values:
*
*
* -
*
* DELETE
- Deletes the change set if the stack creation fails. This is only valid when the
* ChangeSetType
parameter is set to CREATE
. If the deletion of the stack fails, the
* status of the stack is DELETE_FAILED
.
*
*
* -
*
* DO_NOTHING
- if the stack creation fails, do nothing. This is equivalent to specifying
* true
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
* -
*
* ROLLBACK
- if the stack creation fails, roll back the stack. This is equivalent to specifying
* false
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
*
*
* For nested stacks, when the OnStackFailure
parameter is set to DELETE
for the change
* set for the parent stack, any failure in a child stack will cause the parent stack creation to fail and all
* stacks to be deleted.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #onStackFailure}
* will return {@link OnStackFailure#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #onStackFailureAsString}.
*
*
* @return Determines what action will be taken if stack creation fails. If this parameter is specified, the
* DisableRollback
parameter to the ExecuteChangeSet API operation must not be specified. This must be one of these values:
*
* -
*
* DELETE
- Deletes the change set if the stack creation fails. This is only valid when the
* ChangeSetType
parameter is set to CREATE
. If the deletion of the stack fails,
* the status of the stack is DELETE_FAILED
.
*
*
* -
*
* DO_NOTHING
- if the stack creation fails, do nothing. This is equivalent to specifying
* true
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
* -
*
* ROLLBACK
- if the stack creation fails, roll back the stack. This is equivalent to
* specifying false
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
*
*
* For nested stacks, when the OnStackFailure
parameter is set to DELETE
for the
* change set for the parent stack, any failure in a child stack will cause the parent stack creation to
* fail and all stacks to be deleted.
* @see OnStackFailure
*/
public final String onStackFailureAsString() {
return onStackFailure;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(stackName());
hashCode = 31 * hashCode + Objects.hashCode(templateBody());
hashCode = 31 * hashCode + Objects.hashCode(templateURL());
hashCode = 31 * hashCode + Objects.hashCode(usePreviousTemplate());
hashCode = 31 * hashCode + Objects.hashCode(hasParameters() ? parameters() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCapabilities() ? capabilitiesAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasResourceTypes() ? resourceTypes() : null);
hashCode = 31 * hashCode + Objects.hashCode(roleARN());
hashCode = 31 * hashCode + Objects.hashCode(rollbackConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasNotificationARNs() ? notificationARNs() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(changeSetName());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(changeSetTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasResourcesToImport() ? resourcesToImport() : null);
hashCode = 31 * hashCode + Objects.hashCode(includeNestedStacks());
hashCode = 31 * hashCode + Objects.hashCode(onStackFailureAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateChangeSetRequest)) {
return false;
}
CreateChangeSetRequest other = (CreateChangeSetRequest) obj;
return Objects.equals(stackName(), other.stackName()) && Objects.equals(templateBody(), other.templateBody())
&& Objects.equals(templateURL(), other.templateURL())
&& Objects.equals(usePreviousTemplate(), other.usePreviousTemplate()) && hasParameters() == other.hasParameters()
&& Objects.equals(parameters(), other.parameters()) && hasCapabilities() == other.hasCapabilities()
&& Objects.equals(capabilitiesAsStrings(), other.capabilitiesAsStrings())
&& hasResourceTypes() == other.hasResourceTypes() && Objects.equals(resourceTypes(), other.resourceTypes())
&& Objects.equals(roleARN(), other.roleARN())
&& Objects.equals(rollbackConfiguration(), other.rollbackConfiguration())
&& hasNotificationARNs() == other.hasNotificationARNs()
&& Objects.equals(notificationARNs(), other.notificationARNs()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(changeSetName(), other.changeSetName())
&& Objects.equals(clientToken(), other.clientToken()) && Objects.equals(description(), other.description())
&& Objects.equals(changeSetTypeAsString(), other.changeSetTypeAsString())
&& hasResourcesToImport() == other.hasResourcesToImport()
&& Objects.equals(resourcesToImport(), other.resourcesToImport())
&& Objects.equals(includeNestedStacks(), other.includeNestedStacks())
&& Objects.equals(onStackFailureAsString(), other.onStackFailureAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateChangeSetRequest").add("StackName", stackName()).add("TemplateBody", templateBody())
.add("TemplateURL", templateURL()).add("UsePreviousTemplate", usePreviousTemplate())
.add("Parameters", hasParameters() ? parameters() : null)
.add("Capabilities", hasCapabilities() ? capabilitiesAsStrings() : null)
.add("ResourceTypes", hasResourceTypes() ? resourceTypes() : null).add("RoleARN", roleARN())
.add("RollbackConfiguration", rollbackConfiguration())
.add("NotificationARNs", hasNotificationARNs() ? notificationARNs() : null)
.add("Tags", hasTags() ? tags() : null).add("ChangeSetName", changeSetName()).add("ClientToken", clientToken())
.add("Description", description()).add("ChangeSetType", changeSetTypeAsString())
.add("ResourcesToImport", hasResourcesToImport() ? resourcesToImport() : null)
.add("IncludeNestedStacks", includeNestedStacks()).add("OnStackFailure", onStackFailureAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackName":
return Optional.ofNullable(clazz.cast(stackName()));
case "TemplateBody":
return Optional.ofNullable(clazz.cast(templateBody()));
case "TemplateURL":
return Optional.ofNullable(clazz.cast(templateURL()));
case "UsePreviousTemplate":
return Optional.ofNullable(clazz.cast(usePreviousTemplate()));
case "Parameters":
return Optional.ofNullable(clazz.cast(parameters()));
case "Capabilities":
return Optional.ofNullable(clazz.cast(capabilitiesAsStrings()));
case "ResourceTypes":
return Optional.ofNullable(clazz.cast(resourceTypes()));
case "RoleARN":
return Optional.ofNullable(clazz.cast(roleARN()));
case "RollbackConfiguration":
return Optional.ofNullable(clazz.cast(rollbackConfiguration()));
case "NotificationARNs":
return Optional.ofNullable(clazz.cast(notificationARNs()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "ChangeSetName":
return Optional.ofNullable(clazz.cast(changeSetName()));
case "ClientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "ChangeSetType":
return Optional.ofNullable(clazz.cast(changeSetTypeAsString()));
case "ResourcesToImport":
return Optional.ofNullable(clazz.cast(resourcesToImport()));
case "IncludeNestedStacks":
return Optional.ofNullable(clazz.cast(includeNestedStacks()));
case "OnStackFailure":
return Optional.ofNullable(clazz.cast(onStackFailureAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Conditional: You must specify only TemplateBody
or TemplateURL
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder templateBody(String templateBody);
/**
*
* The location of the file that contains the revised template. The URL must point to a template (max size:
* 460,800 bytes) that's located in an Amazon S3 bucket or a Systems Manager document. CloudFormation generates
* the change set by comparing this template with the stack that you specified.
*
*
* Conditional: You must specify only TemplateBody
or TemplateURL
.
*
*
* @param templateURL
* The location of the file that contains the revised template. The URL must point to a template (max
* size: 460,800 bytes) that's located in an Amazon S3 bucket or a Systems Manager document.
* CloudFormation generates the change set by comparing this template with the stack that you
* specified.
*
* Conditional: You must specify only TemplateBody
or TemplateURL
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder templateURL(String templateURL);
/**
*
* Whether to reuse the template that's associated with the stack to create the change set.
*
*
* @param usePreviousTemplate
* Whether to reuse the template that's associated with the stack to create the change set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder usePreviousTemplate(Boolean usePreviousTemplate);
/**
*
* A list of Parameter
structures that specify input parameters for the change set. For more
* information, see the Parameter data type.
*
*
* @param parameters
* A list of Parameter
structures that specify input parameters for the change set. For more
* information, see the Parameter data type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder parameters(Collection parameters);
/**
*
* A list of Parameter
structures that specify input parameters for the change set. For more
* information, see the Parameter data type.
*
*
* @param parameters
* A list of Parameter
structures that specify input parameters for the change set. For more
* information, see the Parameter data type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder parameters(Parameter... parameters);
/**
*
* A list of Parameter
structures that specify input parameters for the change set. For more
* information, see the Parameter data type.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudformation.model.Parameter.Builder} avoiding the need to create
* one manually via {@link software.amazon.awssdk.services.cloudformation.model.Parameter#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudformation.model.Parameter.Builder#build()} is called immediately
* and its result is passed to {@link #parameters(List)}.
*
* @param parameters
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudformation.model.Parameter.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #parameters(java.util.Collection)
*/
Builder parameters(Consumer... parameters);
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for CloudFormation to create the stack.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account;
* for example, by creating new Identity and Access Management (IAM) users. For those stacks, you must
* explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we suggest that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM resources in CloudFormation templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple actions
* like find-and-replace operations, all the way to extensive transformations of entire templates. Because of
* this, users typically create a change set from the processed template, so that they can review the changes
* resulting from the macros before actually creating the stack. If your stack template contains one or more
* macros, and you choose to create a stack directly from the processed template, without first reviewing the
* resulting changes in a change set, you must acknowledge this capability. This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.
*
*
*
* This capacity doesn't apply to creating change sets, and specifying it when creating change sets has no
* effect.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you must
* create or update the stack directly from the template using the CreateStack or UpdateStack
* action, and specifying this capability.
*
*
*
* For more information about macros, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities
* in order for CloudFormation to create the stack.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stacks,
* you must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
* .
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we suggest that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM resources in CloudFormation templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple
* actions like find-and-replace operations, all the way to extensive transformations of entire
* templates. Because of this, users typically create a change set from the processed template, so that
* they can review the changes resulting from the macros before actually creating the stack. If your
* stack template contains one or more macros, and you choose to create a stack directly from the
* processed template, without first reviewing the resulting changes in a change set, you must
* acknowledge this capability. This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.
*
*
*
* This capacity doesn't apply to creating change sets, and specifying it when creating change sets has
* no effect.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you
* must create or update the stack directly from the template using the CreateStack or
* UpdateStack action, and specifying this capability.
*
*
*
* For more information about macros, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilitiesWithStrings(Collection capabilities);
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for CloudFormation to create the stack.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account;
* for example, by creating new Identity and Access Management (IAM) users. For those stacks, you must
* explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we suggest that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM resources in CloudFormation templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple actions
* like find-and-replace operations, all the way to extensive transformations of entire templates. Because of
* this, users typically create a change set from the processed template, so that they can review the changes
* resulting from the macros before actually creating the stack. If your stack template contains one or more
* macros, and you choose to create a stack directly from the processed template, without first reviewing the
* resulting changes in a change set, you must acknowledge this capability. This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.
*
*
*
* This capacity doesn't apply to creating change sets, and specifying it when creating change sets has no
* effect.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you must
* create or update the stack directly from the template using the CreateStack or UpdateStack
* action, and specifying this capability.
*
*
*
* For more information about macros, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities
* in order for CloudFormation to create the stack.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stacks,
* you must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
* .
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we suggest that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM resources in CloudFormation templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple
* actions like find-and-replace operations, all the way to extensive transformations of entire
* templates. Because of this, users typically create a change set from the processed template, so that
* they can review the changes resulting from the macros before actually creating the stack. If your
* stack template contains one or more macros, and you choose to create a stack directly from the
* processed template, without first reviewing the resulting changes in a change set, you must
* acknowledge this capability. This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.
*
*
*
* This capacity doesn't apply to creating change sets, and specifying it when creating change sets has
* no effect.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you
* must create or update the stack directly from the template using the CreateStack or
* UpdateStack action, and specifying this capability.
*
*
*
* For more information about macros, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilitiesWithStrings(String... capabilities);
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for CloudFormation to create the stack.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account;
* for example, by creating new Identity and Access Management (IAM) users. For those stacks, you must
* explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we suggest that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM resources in CloudFormation templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple actions
* like find-and-replace operations, all the way to extensive transformations of entire templates. Because of
* this, users typically create a change set from the processed template, so that they can review the changes
* resulting from the macros before actually creating the stack. If your stack template contains one or more
* macros, and you choose to create a stack directly from the processed template, without first reviewing the
* resulting changes in a change set, you must acknowledge this capability. This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.
*
*
*
* This capacity doesn't apply to creating change sets, and specifying it when creating change sets has no
* effect.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you must
* create or update the stack directly from the template using the CreateStack or UpdateStack
* action, and specifying this capability.
*
*
*
* For more information about macros, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities
* in order for CloudFormation to create the stack.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stacks,
* you must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
* .
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we suggest that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM resources in CloudFormation templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple
* actions like find-and-replace operations, all the way to extensive transformations of entire
* templates. Because of this, users typically create a change set from the processed template, so that
* they can review the changes resulting from the macros before actually creating the stack. If your
* stack template contains one or more macros, and you choose to create a stack directly from the
* processed template, without first reviewing the resulting changes in a change set, you must
* acknowledge this capability. This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.
*
*
*
* This capacity doesn't apply to creating change sets, and specifying it when creating change sets has
* no effect.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you
* must create or update the stack directly from the template using the CreateStack or
* UpdateStack action, and specifying this capability.
*
*
*
* For more information about macros, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilities(Collection capabilities);
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for CloudFormation to create the stack.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account;
* for example, by creating new Identity and Access Management (IAM) users. For those stacks, you must
* explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we suggest that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM resources in CloudFormation templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple actions
* like find-and-replace operations, all the way to extensive transformations of entire templates. Because of
* this, users typically create a change set from the processed template, so that they can review the changes
* resulting from the macros before actually creating the stack. If your stack template contains one or more
* macros, and you choose to create a stack directly from the processed template, without first reviewing the
* resulting changes in a change set, you must acknowledge this capability. This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.
*
*
*
* This capacity doesn't apply to creating change sets, and specifying it when creating change sets has no
* effect.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you must
* create or update the stack directly from the template using the CreateStack or UpdateStack
* action, and specifying this capability.
*
*
*
* For more information about macros, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities
* in order for CloudFormation to create the stack.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stacks,
* you must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
* .
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we suggest that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM resources in CloudFormation templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple
* actions like find-and-replace operations, all the way to extensive transformations of entire
* templates. Because of this, users typically create a change set from the processed template, so that
* they can review the changes resulting from the macros before actually creating the stack. If your
* stack template contains one or more macros, and you choose to create a stack directly from the
* processed template, without first reviewing the resulting changes in a change set, you must
* acknowledge this capability. This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.
*
*
*
* This capacity doesn't apply to creating change sets, and specifying it when creating change sets has
* no effect.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you
* must create or update the stack directly from the template using the CreateStack or
* UpdateStack action, and specifying this capability.
*
*
*
* For more information about macros, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilities(Capability... capabilities);
/**
*
* The template resource types that you have permissions to work with if you execute this change set, such as
* AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
.
*
*
* If the list of resource types doesn't include a resource type that you're updating, the stack update fails.
* By default, CloudFormation grants permissions to all resource types. Identity and Access Management (IAM)
* uses this parameter for condition keys in IAM policies for CloudFormation. For more information, see Controlling
* access with Identity and Access Management in the CloudFormation User Guide.
*
*
* @param resourceTypes
* The template resource types that you have permissions to work with if you execute this change set,
* such as AWS::EC2::Instance
, AWS::EC2::*
, or
* Custom::MyCustomInstance
.
*
* If the list of resource types doesn't include a resource type that you're updating, the stack update
* fails. By default, CloudFormation grants permissions to all resource types. Identity and Access
* Management (IAM) uses this parameter for condition keys in IAM policies for CloudFormation. For more
* information, see Controlling access with Identity and Access Management in the CloudFormation User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder resourceTypes(Collection resourceTypes);
/**
*
* The template resource types that you have permissions to work with if you execute this change set, such as
* AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
.
*
*
* If the list of resource types doesn't include a resource type that you're updating, the stack update fails.
* By default, CloudFormation grants permissions to all resource types. Identity and Access Management (IAM)
* uses this parameter for condition keys in IAM policies for CloudFormation. For more information, see Controlling
* access with Identity and Access Management in the CloudFormation User Guide.
*
*
* @param resourceTypes
* The template resource types that you have permissions to work with if you execute this change set,
* such as AWS::EC2::Instance
, AWS::EC2::*
, or
* Custom::MyCustomInstance
.
*
* If the list of resource types doesn't include a resource type that you're updating, the stack update
* fails. By default, CloudFormation grants permissions to all resource types. Identity and Access
* Management (IAM) uses this parameter for condition keys in IAM policies for CloudFormation. For more
* information, see Controlling access with Identity and Access Management in the CloudFormation User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder resourceTypes(String... resourceTypes);
/**
*
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation assumes
* when executing the change set. CloudFormation uses the role's credentials to make calls on your behalf.
* CloudFormation uses this role for all future operations on the stack. Provided that users have permission to
* operate on the stack, CloudFormation uses this role even if the users don't have permission to pass it.
* Ensure that the role grants least permission.
*
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack. If
* no role is available, CloudFormation uses a temporary session that is generated from your user credentials.
*
*
* @param roleARN
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation
* assumes when executing the change set. CloudFormation uses the role's credentials to make calls on
* your behalf. CloudFormation uses this role for all future operations on the stack. Provided that users
* have permission to operate on the stack, CloudFormation uses this role even if the users don't have
* permission to pass it. Ensure that the role grants least permission.
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the
* stack. If no role is available, CloudFormation uses a temporary session that is generated from your
* user credentials.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder roleARN(String roleARN);
/**
*
* The rollback triggers for CloudFormation to monitor during stack creation and updating operations, and for
* the specified monitoring period afterwards.
*
*
* @param rollbackConfiguration
* The rollback triggers for CloudFormation to monitor during stack creation and updating operations, and
* for the specified monitoring period afterwards.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder rollbackConfiguration(RollbackConfiguration rollbackConfiguration);
/**
*
* The rollback triggers for CloudFormation to monitor during stack creation and updating operations, and for
* the specified monitoring period afterwards.
*
* This is a convenience method that creates an instance of the {@link RollbackConfiguration.Builder} avoiding
* the need to create one manually via {@link RollbackConfiguration#builder()}.
*
*
* When the {@link Consumer} completes, {@link RollbackConfiguration.Builder#build()} is called immediately and
* its result is passed to {@link #rollbackConfiguration(RollbackConfiguration)}.
*
* @param rollbackConfiguration
* a consumer that will call methods on {@link RollbackConfiguration.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #rollbackConfiguration(RollbackConfiguration)
*/
default Builder rollbackConfiguration(Consumer rollbackConfiguration) {
return rollbackConfiguration(RollbackConfiguration.builder().applyMutation(rollbackConfiguration).build());
}
/**
*
* The Amazon Resource Names (ARNs) of Amazon Simple Notification Service (Amazon SNS) topics that
* CloudFormation associates with the stack. To remove all associated notification topics, specify an empty
* list.
*
*
* @param notificationARNs
* The Amazon Resource Names (ARNs) of Amazon Simple Notification Service (Amazon SNS) topics that
* CloudFormation associates with the stack. To remove all associated notification topics, specify an
* empty list.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder notificationARNs(Collection notificationARNs);
/**
*
* The Amazon Resource Names (ARNs) of Amazon Simple Notification Service (Amazon SNS) topics that
* CloudFormation associates with the stack. To remove all associated notification topics, specify an empty
* list.
*
*
* @param notificationARNs
* The Amazon Resource Names (ARNs) of Amazon Simple Notification Service (Amazon SNS) topics that
* CloudFormation associates with the stack. To remove all associated notification topics, specify an
* empty list.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder notificationARNs(String... notificationARNs);
/**
*
* Key-value pairs to associate with this stack. CloudFormation also propagates these tags to resources in the
* stack. You can specify a maximum of 50 tags.
*
*
* @param tags
* Key-value pairs to associate with this stack. CloudFormation also propagates these tags to resources
* in the stack. You can specify a maximum of 50 tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* Key-value pairs to associate with this stack. CloudFormation also propagates these tags to resources in the
* stack. You can specify a maximum of 50 tags.
*
*
* @param tags
* Key-value pairs to associate with this stack. CloudFormation also propagates these tags to resources
* in the stack. You can specify a maximum of 50 tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* Key-value pairs to associate with this stack. CloudFormation also propagates these tags to resources in the
* stack. You can specify a maximum of 50 tags.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudformation.model.Tag.Builder} avoiding the need to create one
* manually via {@link software.amazon.awssdk.services.cloudformation.model.Tag#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudformation.model.Tag.Builder#build()} is called immediately and
* its result is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudformation.model.Tag.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(java.util.Collection)
*/
Builder tags(Consumer... tags);
/**
*
* The name of the change set. The name must be unique among all change sets that are associated with the
* specified stack.
*
*
* A change set name can contain only alphanumeric, case sensitive characters, and hyphens. It must start with
* an alphabetical character and can't exceed 128 characters.
*
*
* @param changeSetName
* The name of the change set. The name must be unique among all change sets that are associated with the
* specified stack.
*
* A change set name can contain only alphanumeric, case sensitive characters, and hyphens. It must start
* with an alphabetical character and can't exceed 128 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder changeSetName(String changeSetName);
/**
*
* A unique identifier for this CreateChangeSet
request. Specify this token if you plan to retry
* requests so that CloudFormation knows that you're not attempting to create another change set with the same
* name. You might retry CreateChangeSet
requests to ensure that CloudFormation successfully
* received them.
*
*
* @param clientToken
* A unique identifier for this CreateChangeSet
request. Specify this token if you plan to
* retry requests so that CloudFormation knows that you're not attempting to create another change set
* with the same name. You might retry CreateChangeSet
requests to ensure that
* CloudFormation successfully received them.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clientToken(String clientToken);
/**
*
* A description to help you identify this change set.
*
*
* @param description
* A description to help you identify this change set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
/**
*
* The type of change set operation. To create a change set for a new stack, specify CREATE
. To
* create a change set for an existing stack, specify UPDATE
. To create a change set for an import
* operation, specify IMPORT
.
*
*
* If you create a change set for a new stack, CloudFormation creates a stack with a unique stack ID, but no
* template or resources. The stack will be in the REVIEW_IN_PROGRESS state until you execute the change set.
*
*
* By default, CloudFormation specifies UPDATE
. You can't use the UPDATE
type to
* create a change set for a new stack or the CREATE
type to create a change set for an existing
* stack.
*
*
* @param changeSetType
* The type of change set operation. To create a change set for a new stack, specify CREATE
.
* To create a change set for an existing stack, specify UPDATE
. To create a change set for
* an import operation, specify IMPORT
.
*
* If you create a change set for a new stack, CloudFormation creates a stack with a unique stack ID, but
* no template or resources. The stack will be in the REVIEW_IN_PROGRESS state until you execute the change set.
*
*
* By default, CloudFormation specifies UPDATE
. You can't use the UPDATE
type
* to create a change set for a new stack or the CREATE
type to create a change set for an
* existing stack.
* @see ChangeSetType
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChangeSetType
*/
Builder changeSetType(String changeSetType);
/**
*
* The type of change set operation. To create a change set for a new stack, specify CREATE
. To
* create a change set for an existing stack, specify UPDATE
. To create a change set for an import
* operation, specify IMPORT
.
*
*
* If you create a change set for a new stack, CloudFormation creates a stack with a unique stack ID, but no
* template or resources. The stack will be in the REVIEW_IN_PROGRESS state until you execute the change set.
*
*
* By default, CloudFormation specifies UPDATE
. You can't use the UPDATE
type to
* create a change set for a new stack or the CREATE
type to create a change set for an existing
* stack.
*
*
* @param changeSetType
* The type of change set operation. To create a change set for a new stack, specify CREATE
.
* To create a change set for an existing stack, specify UPDATE
. To create a change set for
* an import operation, specify IMPORT
.
*
* If you create a change set for a new stack, CloudFormation creates a stack with a unique stack ID, but
* no template or resources. The stack will be in the REVIEW_IN_PROGRESS state until you execute the change set.
*
*
* By default, CloudFormation specifies UPDATE
. You can't use the UPDATE
type
* to create a change set for a new stack or the CREATE
type to create a change set for an
* existing stack.
* @see ChangeSetType
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChangeSetType
*/
Builder changeSetType(ChangeSetType changeSetType);
/**
*
* The resources to import into your stack.
*
*
* @param resourcesToImport
* The resources to import into your stack.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder resourcesToImport(Collection resourcesToImport);
/**
*
* The resources to import into your stack.
*
*
* @param resourcesToImport
* The resources to import into your stack.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder resourcesToImport(ResourceToImport... resourcesToImport);
/**
*
* The resources to import into your stack.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudformation.model.ResourceToImport.Builder} avoiding the need to
* create one manually via
* {@link software.amazon.awssdk.services.cloudformation.model.ResourceToImport#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudformation.model.ResourceToImport.Builder#build()} is called
* immediately and its result is passed to {@link #resourcesToImport(List)}.
*
* @param resourcesToImport
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudformation.model.ResourceToImport.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #resourcesToImport(java.util.Collection)
*/
Builder resourcesToImport(Consumer... resourcesToImport);
/**
*
* Creates a change set for the all nested stacks specified in the template. The default behavior of this action
* is set to False
. To include nested sets in a change set, specify True
.
*
*
* @param includeNestedStacks
* Creates a change set for the all nested stacks specified in the template. The default behavior of this
* action is set to False
. To include nested sets in a change set, specify True
* .
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder includeNestedStacks(Boolean includeNestedStacks);
/**
*
* Determines what action will be taken if stack creation fails. If this parameter is specified, the
* DisableRollback
parameter to the ExecuteChangeSet API operation must not be specified. This must be one of these values:
*
*
* -
*
* DELETE
- Deletes the change set if the stack creation fails. This is only valid when the
* ChangeSetType
parameter is set to CREATE
. If the deletion of the stack fails, the
* status of the stack is DELETE_FAILED
.
*
*
* -
*
* DO_NOTHING
- if the stack creation fails, do nothing. This is equivalent to specifying
* true
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
* -
*
* ROLLBACK
- if the stack creation fails, roll back the stack. This is equivalent to specifying
* false
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
*
*
* For nested stacks, when the OnStackFailure
parameter is set to DELETE
for the
* change set for the parent stack, any failure in a child stack will cause the parent stack creation to fail
* and all stacks to be deleted.
*
*
* @param onStackFailure
* Determines what action will be taken if stack creation fails. If this parameter is specified, the
* DisableRollback
parameter to the ExecuteChangeSet API operation must not be specified. This must be one of these values:
*
* -
*
* DELETE
- Deletes the change set if the stack creation fails. This is only valid when the
* ChangeSetType
parameter is set to CREATE
. If the deletion of the stack
* fails, the status of the stack is DELETE_FAILED
.
*
*
* -
*
* DO_NOTHING
- if the stack creation fails, do nothing. This is equivalent to specifying
* true
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
* -
*
* ROLLBACK
- if the stack creation fails, roll back the stack. This is equivalent to
* specifying false
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
*
*
* For nested stacks, when the OnStackFailure
parameter is set to DELETE
for
* the change set for the parent stack, any failure in a child stack will cause the parent stack creation
* to fail and all stacks to be deleted.
* @see OnStackFailure
* @return Returns a reference to this object so that method calls can be chained together.
* @see OnStackFailure
*/
Builder onStackFailure(String onStackFailure);
/**
*
* Determines what action will be taken if stack creation fails. If this parameter is specified, the
* DisableRollback
parameter to the ExecuteChangeSet API operation must not be specified. This must be one of these values:
*
*
* -
*
* DELETE
- Deletes the change set if the stack creation fails. This is only valid when the
* ChangeSetType
parameter is set to CREATE
. If the deletion of the stack fails, the
* status of the stack is DELETE_FAILED
.
*
*
* -
*
* DO_NOTHING
- if the stack creation fails, do nothing. This is equivalent to specifying
* true
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
* -
*
* ROLLBACK
- if the stack creation fails, roll back the stack. This is equivalent to specifying
* false
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
*
*
* For nested stacks, when the OnStackFailure
parameter is set to DELETE
for the
* change set for the parent stack, any failure in a child stack will cause the parent stack creation to fail
* and all stacks to be deleted.
*
*
* @param onStackFailure
* Determines what action will be taken if stack creation fails. If this parameter is specified, the
* DisableRollback
parameter to the ExecuteChangeSet API operation must not be specified. This must be one of these values:
*
* -
*
* DELETE
- Deletes the change set if the stack creation fails. This is only valid when the
* ChangeSetType
parameter is set to CREATE
. If the deletion of the stack
* fails, the status of the stack is DELETE_FAILED
.
*
*
* -
*
* DO_NOTHING
- if the stack creation fails, do nothing. This is equivalent to specifying
* true
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
* -
*
* ROLLBACK
- if the stack creation fails, roll back the stack. This is equivalent to
* specifying false
for the DisableRollback
parameter to the ExecuteChangeSet API operation.
*
*
*
*
* For nested stacks, when the OnStackFailure
parameter is set to DELETE
for
* the change set for the parent stack, any failure in a child stack will cause the parent stack creation
* to fail and all stacks to be deleted.
* @see OnStackFailure
* @return Returns a reference to this object so that method calls can be chained together.
* @see OnStackFailure
*/
Builder onStackFailure(OnStackFailure onStackFailure);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends CloudFormationRequest.BuilderImpl implements Builder {
private String stackName;
private String templateBody;
private String templateURL;
private Boolean usePreviousTemplate;
private List parameters = DefaultSdkAutoConstructList.getInstance();
private List capabilities = DefaultSdkAutoConstructList.getInstance();
private List resourceTypes = DefaultSdkAutoConstructList.getInstance();
private String roleARN;
private RollbackConfiguration rollbackConfiguration;
private List notificationARNs = DefaultSdkAutoConstructList.getInstance();
private List tags = DefaultSdkAutoConstructList.getInstance();
private String changeSetName;
private String clientToken;
private String description;
private String changeSetType;
private List resourcesToImport = DefaultSdkAutoConstructList.getInstance();
private Boolean includeNestedStacks;
private String onStackFailure;
private BuilderImpl() {
}
private BuilderImpl(CreateChangeSetRequest model) {
super(model);
stackName(model.stackName);
templateBody(model.templateBody);
templateURL(model.templateURL);
usePreviousTemplate(model.usePreviousTemplate);
parameters(model.parameters);
capabilitiesWithStrings(model.capabilities);
resourceTypes(model.resourceTypes);
roleARN(model.roleARN);
rollbackConfiguration(model.rollbackConfiguration);
notificationARNs(model.notificationARNs);
tags(model.tags);
changeSetName(model.changeSetName);
clientToken(model.clientToken);
description(model.description);
changeSetType(model.changeSetType);
resourcesToImport(model.resourcesToImport);
includeNestedStacks(model.includeNestedStacks);
onStackFailure(model.onStackFailure);
}
public final String getStackName() {
return stackName;
}
public final void setStackName(String stackName) {
this.stackName = stackName;
}
@Override
public final Builder stackName(String stackName) {
this.stackName = stackName;
return this;
}
public final String getTemplateBody() {
return templateBody;
}
public final void setTemplateBody(String templateBody) {
this.templateBody = templateBody;
}
@Override
public final Builder templateBody(String templateBody) {
this.templateBody = templateBody;
return this;
}
public final String getTemplateURL() {
return templateURL;
}
public final void setTemplateURL(String templateURL) {
this.templateURL = templateURL;
}
@Override
public final Builder templateURL(String templateURL) {
this.templateURL = templateURL;
return this;
}
public final Boolean getUsePreviousTemplate() {
return usePreviousTemplate;
}
public final void setUsePreviousTemplate(Boolean usePreviousTemplate) {
this.usePreviousTemplate = usePreviousTemplate;
}
@Override
public final Builder usePreviousTemplate(Boolean usePreviousTemplate) {
this.usePreviousTemplate = usePreviousTemplate;
return this;
}
public final List getParameters() {
List result = ParametersCopier.copyToBuilder(this.parameters);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setParameters(Collection parameters) {
this.parameters = ParametersCopier.copyFromBuilder(parameters);
}
@Override
public final Builder parameters(Collection parameters) {
this.parameters = ParametersCopier.copy(parameters);
return this;
}
@Override
@SafeVarargs
public final Builder parameters(Parameter... parameters) {
parameters(Arrays.asList(parameters));
return this;
}
@Override
@SafeVarargs
public final Builder parameters(Consumer... parameters) {
parameters(Stream.of(parameters).map(c -> Parameter.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final Collection getCapabilities() {
if (capabilities instanceof SdkAutoConstructList) {
return null;
}
return capabilities;
}
public final void setCapabilities(Collection capabilities) {
this.capabilities = CapabilitiesCopier.copy(capabilities);
}
@Override
public final Builder capabilitiesWithStrings(Collection capabilities) {
this.capabilities = CapabilitiesCopier.copy(capabilities);
return this;
}
@Override
@SafeVarargs
public final Builder capabilitiesWithStrings(String... capabilities) {
capabilitiesWithStrings(Arrays.asList(capabilities));
return this;
}
@Override
public final Builder capabilities(Collection capabilities) {
this.capabilities = CapabilitiesCopier.copyEnumToString(capabilities);
return this;
}
@Override
@SafeVarargs
public final Builder capabilities(Capability... capabilities) {
capabilities(Arrays.asList(capabilities));
return this;
}
public final Collection getResourceTypes() {
if (resourceTypes instanceof SdkAutoConstructList) {
return null;
}
return resourceTypes;
}
public final void setResourceTypes(Collection resourceTypes) {
this.resourceTypes = ResourceTypesCopier.copy(resourceTypes);
}
@Override
public final Builder resourceTypes(Collection resourceTypes) {
this.resourceTypes = ResourceTypesCopier.copy(resourceTypes);
return this;
}
@Override
@SafeVarargs
public final Builder resourceTypes(String... resourceTypes) {
resourceTypes(Arrays.asList(resourceTypes));
return this;
}
public final String getRoleARN() {
return roleARN;
}
public final void setRoleARN(String roleARN) {
this.roleARN = roleARN;
}
@Override
public final Builder roleARN(String roleARN) {
this.roleARN = roleARN;
return this;
}
public final RollbackConfiguration.Builder getRollbackConfiguration() {
return rollbackConfiguration != null ? rollbackConfiguration.toBuilder() : null;
}
public final void setRollbackConfiguration(RollbackConfiguration.BuilderImpl rollbackConfiguration) {
this.rollbackConfiguration = rollbackConfiguration != null ? rollbackConfiguration.build() : null;
}
@Override
public final Builder rollbackConfiguration(RollbackConfiguration rollbackConfiguration) {
this.rollbackConfiguration = rollbackConfiguration;
return this;
}
public final Collection getNotificationARNs() {
if (notificationARNs instanceof SdkAutoConstructList) {
return null;
}
return notificationARNs;
}
public final void setNotificationARNs(Collection notificationARNs) {
this.notificationARNs = NotificationARNsCopier.copy(notificationARNs);
}
@Override
public final Builder notificationARNs(Collection notificationARNs) {
this.notificationARNs = NotificationARNsCopier.copy(notificationARNs);
return this;
}
@Override
@SafeVarargs
public final Builder notificationARNs(String... notificationARNs) {
notificationARNs(Arrays.asList(notificationARNs));
return this;
}
public final List getTags() {
List result = TagsCopier.copyToBuilder(this.tags);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTags(Collection tags) {
this.tags = TagsCopier.copyFromBuilder(tags);
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagsCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final String getChangeSetName() {
return changeSetName;
}
public final void setChangeSetName(String changeSetName) {
this.changeSetName = changeSetName;
}
@Override
public final Builder changeSetName(String changeSetName) {
this.changeSetName = changeSetName;
return this;
}
public final String getClientToken() {
return clientToken;
}
public final void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
@Override
public final Builder clientToken(String clientToken) {
this.clientToken = clientToken;
return this;
}
public final String getDescription() {
return description;
}
public final void setDescription(String description) {
this.description = description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final String getChangeSetType() {
return changeSetType;
}
public final void setChangeSetType(String changeSetType) {
this.changeSetType = changeSetType;
}
@Override
public final Builder changeSetType(String changeSetType) {
this.changeSetType = changeSetType;
return this;
}
@Override
public final Builder changeSetType(ChangeSetType changeSetType) {
this.changeSetType(changeSetType == null ? null : changeSetType.toString());
return this;
}
public final List getResourcesToImport() {
List result = ResourcesToImportCopier.copyToBuilder(this.resourcesToImport);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setResourcesToImport(Collection resourcesToImport) {
this.resourcesToImport = ResourcesToImportCopier.copyFromBuilder(resourcesToImport);
}
@Override
public final Builder resourcesToImport(Collection resourcesToImport) {
this.resourcesToImport = ResourcesToImportCopier.copy(resourcesToImport);
return this;
}
@Override
@SafeVarargs
public final Builder resourcesToImport(ResourceToImport... resourcesToImport) {
resourcesToImport(Arrays.asList(resourcesToImport));
return this;
}
@Override
@SafeVarargs
public final Builder resourcesToImport(Consumer... resourcesToImport) {
resourcesToImport(Stream.of(resourcesToImport).map(c -> ResourceToImport.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final Boolean getIncludeNestedStacks() {
return includeNestedStacks;
}
public final void setIncludeNestedStacks(Boolean includeNestedStacks) {
this.includeNestedStacks = includeNestedStacks;
}
@Override
public final Builder includeNestedStacks(Boolean includeNestedStacks) {
this.includeNestedStacks = includeNestedStacks;
return this;
}
public final String getOnStackFailure() {
return onStackFailure;
}
public final void setOnStackFailure(String onStackFailure) {
this.onStackFailure = onStackFailure;
}
@Override
public final Builder onStackFailure(String onStackFailure) {
this.onStackFailure = onStackFailure;
return this;
}
@Override
public final Builder onStackFailure(OnStackFailure onStackFailure) {
this.onStackFailure(onStackFailure == null ? null : onStackFailure.toString());
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateChangeSetRequest build() {
return new CreateChangeSetRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}