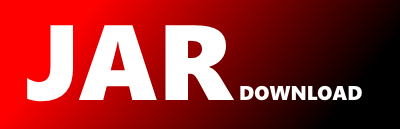
software.amazon.awssdk.services.cloudformation.model.DeploymentTargets Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* [Service-managed permissions] The Organizations accounts to which StackSets deploys. StackSets doesn't deploy stack
* instances to the organization management account, even if the organization management account is in your organization
* or in an OU in your organization.
*
*
* For update operations, you can specify either Accounts
or OrganizationalUnitIds
. For create
* and delete operations, specify OrganizationalUnitIds
.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeploymentTargets implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> ACCOUNTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Accounts")
.getter(getter(DeploymentTargets::accounts))
.setter(setter(Builder::accounts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Accounts").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ACCOUNTS_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AccountsUrl").getter(getter(DeploymentTargets::accountsUrl)).setter(setter(Builder::accountsUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountsUrl").build()).build();
private static final SdkField> ORGANIZATIONAL_UNIT_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OrganizationalUnitIds")
.getter(getter(DeploymentTargets::organizationalUnitIds))
.setter(setter(Builder::organizationalUnitIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OrganizationalUnitIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ACCOUNT_FILTER_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AccountFilterType").getter(getter(DeploymentTargets::accountFilterTypeAsString))
.setter(setter(Builder::accountFilterType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountFilterType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACCOUNTS_FIELD,
ACCOUNTS_URL_FIELD, ORGANIZATIONAL_UNIT_IDS_FIELD, ACCOUNT_FILTER_TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final List accounts;
private final String accountsUrl;
private final List organizationalUnitIds;
private final String accountFilterType;
private DeploymentTargets(BuilderImpl builder) {
this.accounts = builder.accounts;
this.accountsUrl = builder.accountsUrl;
this.organizationalUnitIds = builder.organizationalUnitIds;
this.accountFilterType = builder.accountFilterType;
}
/**
* For responses, this returns true if the service returned a value for the Accounts property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasAccounts() {
return accounts != null && !(accounts instanceof SdkAutoConstructList);
}
/**
*
* The names of one or more Amazon Web Services accounts for which you want to deploy stack set updates.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAccounts} method.
*
*
* @return The names of one or more Amazon Web Services accounts for which you want to deploy stack set updates.
*/
public final List accounts() {
return accounts;
}
/**
*
* Returns the value of the AccountsUrl
property.
*
*
* @return Returns the value of the AccountsUrl
property.
*/
public final String accountsUrl() {
return accountsUrl;
}
/**
* For responses, this returns true if the service returned a value for the OrganizationalUnitIds property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasOrganizationalUnitIds() {
return organizationalUnitIds != null && !(organizationalUnitIds instanceof SdkAutoConstructList);
}
/**
*
* The organization root ID or organizational unit (OU) IDs to which StackSets deploys.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOrganizationalUnitIds} method.
*
*
* @return The organization root ID or organizational unit (OU) IDs to which StackSets deploys.
*/
public final List organizationalUnitIds() {
return organizationalUnitIds;
}
/**
*
* Limit deployment targets to individual accounts or include additional accounts with provided OUs.
*
*
* The following is a list of possible values for the AccountFilterType
operation.
*
*
* -
*
* INTERSECTION
: StackSets deploys to the accounts specified in Accounts
parameter.
*
*
* -
*
* DIFFERENCE
: StackSets excludes the accounts specified in Accounts
parameter. This
* enables user to avoid certain accounts within an OU such as suspended accounts.
*
*
* -
*
* UNION
: StackSets includes additional accounts deployment targets.
*
*
* This is the default value if AccountFilterType
is not provided. This enables user to update an
* entire OU and individual accounts from a different OU in one request, which used to be two separate requests.
*
*
* -
*
* NONE
: Deploys to all the accounts in specified organizational units (OU).
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #accountFilterType}
* will return {@link AccountFilterType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #accountFilterTypeAsString}.
*
*
* @return Limit deployment targets to individual accounts or include additional accounts with provided OUs.
*
* The following is a list of possible values for the AccountFilterType
operation.
*
*
* -
*
* INTERSECTION
: StackSets deploys to the accounts specified in Accounts
* parameter.
*
*
* -
*
* DIFFERENCE
: StackSets excludes the accounts specified in Accounts
parameter.
* This enables user to avoid certain accounts within an OU such as suspended accounts.
*
*
* -
*
* UNION
: StackSets includes additional accounts deployment targets.
*
*
* This is the default value if AccountFilterType
is not provided. This enables user to update
* an entire OU and individual accounts from a different OU in one request, which used to be two separate
* requests.
*
*
* -
*
* NONE
: Deploys to all the accounts in specified organizational units (OU).
*
*
* @see AccountFilterType
*/
public final AccountFilterType accountFilterType() {
return AccountFilterType.fromValue(accountFilterType);
}
/**
*
* Limit deployment targets to individual accounts or include additional accounts with provided OUs.
*
*
* The following is a list of possible values for the AccountFilterType
operation.
*
*
* -
*
* INTERSECTION
: StackSets deploys to the accounts specified in Accounts
parameter.
*
*
* -
*
* DIFFERENCE
: StackSets excludes the accounts specified in Accounts
parameter. This
* enables user to avoid certain accounts within an OU such as suspended accounts.
*
*
* -
*
* UNION
: StackSets includes additional accounts deployment targets.
*
*
* This is the default value if AccountFilterType
is not provided. This enables user to update an
* entire OU and individual accounts from a different OU in one request, which used to be two separate requests.
*
*
* -
*
* NONE
: Deploys to all the accounts in specified organizational units (OU).
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #accountFilterType}
* will return {@link AccountFilterType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #accountFilterTypeAsString}.
*
*
* @return Limit deployment targets to individual accounts or include additional accounts with provided OUs.
*
* The following is a list of possible values for the AccountFilterType
operation.
*
*
* -
*
* INTERSECTION
: StackSets deploys to the accounts specified in Accounts
* parameter.
*
*
* -
*
* DIFFERENCE
: StackSets excludes the accounts specified in Accounts
parameter.
* This enables user to avoid certain accounts within an OU such as suspended accounts.
*
*
* -
*
* UNION
: StackSets includes additional accounts deployment targets.
*
*
* This is the default value if AccountFilterType
is not provided. This enables user to update
* an entire OU and individual accounts from a different OU in one request, which used to be two separate
* requests.
*
*
* -
*
* NONE
: Deploys to all the accounts in specified organizational units (OU).
*
*
* @see AccountFilterType
*/
public final String accountFilterTypeAsString() {
return accountFilterType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasAccounts() ? accounts() : null);
hashCode = 31 * hashCode + Objects.hashCode(accountsUrl());
hashCode = 31 * hashCode + Objects.hashCode(hasOrganizationalUnitIds() ? organizationalUnitIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(accountFilterTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeploymentTargets)) {
return false;
}
DeploymentTargets other = (DeploymentTargets) obj;
return hasAccounts() == other.hasAccounts() && Objects.equals(accounts(), other.accounts())
&& Objects.equals(accountsUrl(), other.accountsUrl())
&& hasOrganizationalUnitIds() == other.hasOrganizationalUnitIds()
&& Objects.equals(organizationalUnitIds(), other.organizationalUnitIds())
&& Objects.equals(accountFilterTypeAsString(), other.accountFilterTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DeploymentTargets").add("Accounts", hasAccounts() ? accounts() : null)
.add("AccountsUrl", accountsUrl())
.add("OrganizationalUnitIds", hasOrganizationalUnitIds() ? organizationalUnitIds() : null)
.add("AccountFilterType", accountFilterTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Accounts":
return Optional.ofNullable(clazz.cast(accounts()));
case "AccountsUrl":
return Optional.ofNullable(clazz.cast(accountsUrl()));
case "OrganizationalUnitIds":
return Optional.ofNullable(clazz.cast(organizationalUnitIds()));
case "AccountFilterType":
return Optional.ofNullable(clazz.cast(accountFilterTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function