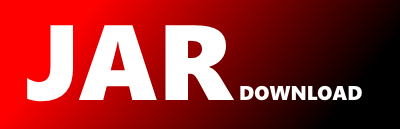
software.amazon.awssdk.services.cloudformation.model.RollbackConfiguration Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Structure containing the rollback triggers for CloudFormation to monitor during stack creation and updating
* operations, and for the specified monitoring period afterwards.
*
*
* Rollback triggers enable you to have CloudFormation monitor the state of your application during stack creation and
* updating, and to roll back that operation if the application breaches the threshold of any of the alarms you've
* specified. For more information, see Monitor and
* Roll Back Stack Operations.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RollbackConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> ROLLBACK_TRIGGERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("RollbackTriggers")
.getter(getter(RollbackConfiguration::rollbackTriggers))
.setter(setter(Builder::rollbackTriggers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RollbackTriggers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(RollbackTrigger::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField MONITORING_TIME_IN_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MonitoringTimeInMinutes").getter(getter(RollbackConfiguration::monitoringTimeInMinutes))
.setter(setter(Builder::monitoringTimeInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MonitoringTimeInMinutes").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ROLLBACK_TRIGGERS_FIELD,
MONITORING_TIME_IN_MINUTES_FIELD));
private static final long serialVersionUID = 1L;
private final List rollbackTriggers;
private final Integer monitoringTimeInMinutes;
private RollbackConfiguration(BuilderImpl builder) {
this.rollbackTriggers = builder.rollbackTriggers;
this.monitoringTimeInMinutes = builder.monitoringTimeInMinutes;
}
/**
* For responses, this returns true if the service returned a value for the RollbackTriggers property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasRollbackTriggers() {
return rollbackTriggers != null && !(rollbackTriggers instanceof SdkAutoConstructList);
}
/**
*
* The triggers to monitor during stack creation or update actions.
*
*
* By default, CloudFormation saves the rollback triggers specified for a stack and applies them to any subsequent
* update operations for the stack, unless you specify otherwise. If you do specify rollback triggers for this
* parameter, those triggers replace any list of triggers previously specified for the stack. This means:
*
*
* -
*
* To use the rollback triggers previously specified for this stack, if any, don't specify this parameter.
*
*
* -
*
* To specify new or updated rollback triggers, you must specify all the triggers that you want used for this
* stack, even triggers you've specified before (for example, when creating the stack or during a previous stack
* update). Any triggers that you don't include in the updated list of triggers are no longer applied to the stack.
*
*
* -
*
* To remove all currently specified triggers, specify an empty list for this parameter.
*
*
*
*
* If a specified trigger is missing, the entire stack operation fails and is rolled back.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRollbackTriggers} method.
*
*
* @return The triggers to monitor during stack creation or update actions.
*
* By default, CloudFormation saves the rollback triggers specified for a stack and applies them to any
* subsequent update operations for the stack, unless you specify otherwise. If you do specify rollback
* triggers for this parameter, those triggers replace any list of triggers previously specified for the
* stack. This means:
*
*
* -
*
* To use the rollback triggers previously specified for this stack, if any, don't specify this parameter.
*
*
* -
*
* To specify new or updated rollback triggers, you must specify all the triggers that you want used
* for this stack, even triggers you've specified before (for example, when creating the stack or during a
* previous stack update). Any triggers that you don't include in the updated list of triggers are no longer
* applied to the stack.
*
*
* -
*
* To remove all currently specified triggers, specify an empty list for this parameter.
*
*
*
*
* If a specified trigger is missing, the entire stack operation fails and is rolled back.
*/
public final List rollbackTriggers() {
return rollbackTriggers;
}
/**
*
* The amount of time, in minutes, during which CloudFormation should monitor all the rollback triggers after the
* stack creation or update operation deploys all necessary resources.
*
*
* The default is 0 minutes.
*
*
* If you specify a monitoring period but don't specify any rollback triggers, CloudFormation still waits the
* specified period of time before cleaning up old resources after update operations. You can use this monitoring
* period to perform any manual stack validation desired, and manually cancel the stack creation or update (using
* CancelUpdateStack, for example) as necessary.
*
*
* If you specify 0 for this parameter, CloudFormation still monitors the specified rollback triggers during stack
* creation and update operations. Then, for update operations, it begins disposing of old resources immediately
* once the operation completes.
*
*
* @return The amount of time, in minutes, during which CloudFormation should monitor all the rollback triggers
* after the stack creation or update operation deploys all necessary resources.
*
* The default is 0 minutes.
*
*
* If you specify a monitoring period but don't specify any rollback triggers, CloudFormation still waits
* the specified period of time before cleaning up old resources after update operations. You can use this
* monitoring period to perform any manual stack validation desired, and manually cancel the stack creation
* or update (using CancelUpdateStack, for example) as necessary.
*
*
* If you specify 0 for this parameter, CloudFormation still monitors the specified rollback triggers during
* stack creation and update operations. Then, for update operations, it begins disposing of old resources
* immediately once the operation completes.
*/
public final Integer monitoringTimeInMinutes() {
return monitoringTimeInMinutes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasRollbackTriggers() ? rollbackTriggers() : null);
hashCode = 31 * hashCode + Objects.hashCode(monitoringTimeInMinutes());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RollbackConfiguration)) {
return false;
}
RollbackConfiguration other = (RollbackConfiguration) obj;
return hasRollbackTriggers() == other.hasRollbackTriggers()
&& Objects.equals(rollbackTriggers(), other.rollbackTriggers())
&& Objects.equals(monitoringTimeInMinutes(), other.monitoringTimeInMinutes());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RollbackConfiguration")
.add("RollbackTriggers", hasRollbackTriggers() ? rollbackTriggers() : null)
.add("MonitoringTimeInMinutes", monitoringTimeInMinutes()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RollbackTriggers":
return Optional.ofNullable(clazz.cast(rollbackTriggers()));
case "MonitoringTimeInMinutes":
return Optional.ofNullable(clazz.cast(monitoringTimeInMinutes()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* By default, CloudFormation saves the rollback triggers specified for a stack and applies them to any
* subsequent update operations for the stack, unless you specify otherwise. If you do specify rollback
* triggers for this parameter, those triggers replace any list of triggers previously specified for the
* stack. This means:
*
*
* -
*
* To use the rollback triggers previously specified for this stack, if any, don't specify this
* parameter.
*
*
* -
*
* To specify new or updated rollback triggers, you must specify all the triggers that you want
* used for this stack, even triggers you've specified before (for example, when creating the stack or
* during a previous stack update). Any triggers that you don't include in the updated list of triggers
* are no longer applied to the stack.
*
*
* -
*
* To remove all currently specified triggers, specify an empty list for this parameter.
*
*
*
*
* If a specified trigger is missing, the entire stack operation fails and is rolled back.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder rollbackTriggers(Collection rollbackTriggers);
/**
*
* The triggers to monitor during stack creation or update actions.
*
*
* By default, CloudFormation saves the rollback triggers specified for a stack and applies them to any
* subsequent update operations for the stack, unless you specify otherwise. If you do specify rollback triggers
* for this parameter, those triggers replace any list of triggers previously specified for the stack. This
* means:
*
*
* -
*
* To use the rollback triggers previously specified for this stack, if any, don't specify this parameter.
*
*
* -
*
* To specify new or updated rollback triggers, you must specify all the triggers that you want used for
* this stack, even triggers you've specified before (for example, when creating the stack or during a previous
* stack update). Any triggers that you don't include in the updated list of triggers are no longer applied to
* the stack.
*
*
* -
*
* To remove all currently specified triggers, specify an empty list for this parameter.
*
*
*
*
* If a specified trigger is missing, the entire stack operation fails and is rolled back.
*
*
* @param rollbackTriggers
* The triggers to monitor during stack creation or update actions.
*
* By default, CloudFormation saves the rollback triggers specified for a stack and applies them to any
* subsequent update operations for the stack, unless you specify otherwise. If you do specify rollback
* triggers for this parameter, those triggers replace any list of triggers previously specified for the
* stack. This means:
*
*
* -
*
* To use the rollback triggers previously specified for this stack, if any, don't specify this
* parameter.
*
*
* -
*
* To specify new or updated rollback triggers, you must specify all the triggers that you want
* used for this stack, even triggers you've specified before (for example, when creating the stack or
* during a previous stack update). Any triggers that you don't include in the updated list of triggers
* are no longer applied to the stack.
*
*
* -
*
* To remove all currently specified triggers, specify an empty list for this parameter.
*
*
*
*
* If a specified trigger is missing, the entire stack operation fails and is rolled back.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder rollbackTriggers(RollbackTrigger... rollbackTriggers);
/**
*
* The triggers to monitor during stack creation or update actions.
*
*
* By default, CloudFormation saves the rollback triggers specified for a stack and applies them to any
* subsequent update operations for the stack, unless you specify otherwise. If you do specify rollback triggers
* for this parameter, those triggers replace any list of triggers previously specified for the stack. This
* means:
*
*
* -
*
* To use the rollback triggers previously specified for this stack, if any, don't specify this parameter.
*
*
* -
*
* To specify new or updated rollback triggers, you must specify all the triggers that you want used for
* this stack, even triggers you've specified before (for example, when creating the stack or during a previous
* stack update). Any triggers that you don't include in the updated list of triggers are no longer applied to
* the stack.
*
*
* -
*
* To remove all currently specified triggers, specify an empty list for this parameter.
*
*
*
*
* If a specified trigger is missing, the entire stack operation fails and is rolled back.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudformation.model.RollbackTrigger.Builder} avoiding the need to
* create one manually via
* {@link software.amazon.awssdk.services.cloudformation.model.RollbackTrigger#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudformation.model.RollbackTrigger.Builder#build()} is called
* immediately and its result is passed to {@link #rollbackTriggers(List)}.
*
* @param rollbackTriggers
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudformation.model.RollbackTrigger.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #rollbackTriggers(java.util.Collection)
*/
Builder rollbackTriggers(Consumer... rollbackTriggers);
/**
*
* The amount of time, in minutes, during which CloudFormation should monitor all the rollback triggers after
* the stack creation or update operation deploys all necessary resources.
*
*
* The default is 0 minutes.
*
*
* If you specify a monitoring period but don't specify any rollback triggers, CloudFormation still waits the
* specified period of time before cleaning up old resources after update operations. You can use this
* monitoring period to perform any manual stack validation desired, and manually cancel the stack creation or
* update (using CancelUpdateStack, for example) as necessary.
*
*
* If you specify 0 for this parameter, CloudFormation still monitors the specified rollback triggers during
* stack creation and update operations. Then, for update operations, it begins disposing of old resources
* immediately once the operation completes.
*
*
* @param monitoringTimeInMinutes
* The amount of time, in minutes, during which CloudFormation should monitor all the rollback triggers
* after the stack creation or update operation deploys all necessary resources.
*
* The default is 0 minutes.
*
*
* If you specify a monitoring period but don't specify any rollback triggers, CloudFormation still waits
* the specified period of time before cleaning up old resources after update operations. You can use
* this monitoring period to perform any manual stack validation desired, and manually cancel the stack
* creation or update (using CancelUpdateStack, for example) as necessary.
*
*
* If you specify 0 for this parameter, CloudFormation still monitors the specified rollback triggers
* during stack creation and update operations. Then, for update operations, it begins disposing of old
* resources immediately once the operation completes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder monitoringTimeInMinutes(Integer monitoringTimeInMinutes);
}
static final class BuilderImpl implements Builder {
private List rollbackTriggers = DefaultSdkAutoConstructList.getInstance();
private Integer monitoringTimeInMinutes;
private BuilderImpl() {
}
private BuilderImpl(RollbackConfiguration model) {
rollbackTriggers(model.rollbackTriggers);
monitoringTimeInMinutes(model.monitoringTimeInMinutes);
}
public final List getRollbackTriggers() {
List result = RollbackTriggersCopier.copyToBuilder(this.rollbackTriggers);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setRollbackTriggers(Collection rollbackTriggers) {
this.rollbackTriggers = RollbackTriggersCopier.copyFromBuilder(rollbackTriggers);
}
@Override
public final Builder rollbackTriggers(Collection rollbackTriggers) {
this.rollbackTriggers = RollbackTriggersCopier.copy(rollbackTriggers);
return this;
}
@Override
@SafeVarargs
public final Builder rollbackTriggers(RollbackTrigger... rollbackTriggers) {
rollbackTriggers(Arrays.asList(rollbackTriggers));
return this;
}
@Override
@SafeVarargs
public final Builder rollbackTriggers(Consumer... rollbackTriggers) {
rollbackTriggers(Stream.of(rollbackTriggers).map(c -> RollbackTrigger.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final Integer getMonitoringTimeInMinutes() {
return monitoringTimeInMinutes;
}
public final void setMonitoringTimeInMinutes(Integer monitoringTimeInMinutes) {
this.monitoringTimeInMinutes = monitoringTimeInMinutes;
}
@Override
public final Builder monitoringTimeInMinutes(Integer monitoringTimeInMinutes) {
this.monitoringTimeInMinutes = monitoringTimeInMinutes;
return this;
}
@Override
public RollbackConfiguration build() {
return new RollbackConfiguration(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}