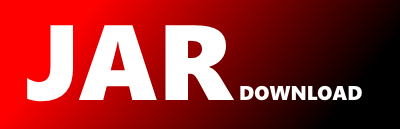
software.amazon.awssdk.services.cloudformation.model.StackSetOperation Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The structure that contains information about a stack set operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class StackSetOperation implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField OPERATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OperationId").getter(getter(StackSetOperation::operationId)).setter(setter(Builder::operationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OperationId").build()).build();
private static final SdkField STACK_SET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackSetId").getter(getter(StackSetOperation::stackSetId)).setter(setter(Builder::stackSetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackSetId").build()).build();
private static final SdkField ACTION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Action")
.getter(getter(StackSetOperation::actionAsString)).setter(setter(Builder::action))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Action").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(StackSetOperation::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField OPERATION_PREFERENCES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OperationPreferences")
.getter(getter(StackSetOperation::operationPreferences)).setter(setter(Builder::operationPreferences))
.constructor(StackSetOperationPreferences::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OperationPreferences").build())
.build();
private static final SdkField RETAIN_STACKS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("RetainStacks").getter(getter(StackSetOperation::retainStacks)).setter(setter(Builder::retainStacks))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RetainStacks").build()).build();
private static final SdkField ADMINISTRATION_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AdministrationRoleARN").getter(getter(StackSetOperation::administrationRoleARN))
.setter(setter(Builder::administrationRoleARN))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdministrationRoleARN").build())
.build();
private static final SdkField EXECUTION_ROLE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExecutionRoleName").getter(getter(StackSetOperation::executionRoleName))
.setter(setter(Builder::executionRoleName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExecutionRoleName").build()).build();
private static final SdkField CREATION_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTimestamp").getter(getter(StackSetOperation::creationTimestamp))
.setter(setter(Builder::creationTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTimestamp").build()).build();
private static final SdkField END_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EndTimestamp").getter(getter(StackSetOperation::endTimestamp)).setter(setter(Builder::endTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndTimestamp").build()).build();
private static final SdkField DEPLOYMENT_TARGETS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DeploymentTargets")
.getter(getter(StackSetOperation::deploymentTargets)).setter(setter(Builder::deploymentTargets))
.constructor(DeploymentTargets::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeploymentTargets").build()).build();
private static final SdkField STACK_SET_DRIFT_DETECTION_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("StackSetDriftDetectionDetails")
.getter(getter(StackSetOperation::stackSetDriftDetectionDetails))
.setter(setter(Builder::stackSetDriftDetectionDetails))
.constructor(StackSetDriftDetectionDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackSetDriftDetectionDetails")
.build()).build();
private static final SdkField STATUS_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StatusReason").getter(getter(StackSetOperation::statusReason)).setter(setter(Builder::statusReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusReason").build()).build();
private static final SdkField STATUS_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StatusDetails")
.getter(getter(StackSetOperation::statusDetails)).setter(setter(Builder::statusDetails))
.constructor(StackSetOperationStatusDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusDetails").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(OPERATION_ID_FIELD,
STACK_SET_ID_FIELD, ACTION_FIELD, STATUS_FIELD, OPERATION_PREFERENCES_FIELD, RETAIN_STACKS_FIELD,
ADMINISTRATION_ROLE_ARN_FIELD, EXECUTION_ROLE_NAME_FIELD, CREATION_TIMESTAMP_FIELD, END_TIMESTAMP_FIELD,
DEPLOYMENT_TARGETS_FIELD, STACK_SET_DRIFT_DETECTION_DETAILS_FIELD, STATUS_REASON_FIELD, STATUS_DETAILS_FIELD));
private static final long serialVersionUID = 1L;
private final String operationId;
private final String stackSetId;
private final String action;
private final String status;
private final StackSetOperationPreferences operationPreferences;
private final Boolean retainStacks;
private final String administrationRoleARN;
private final String executionRoleName;
private final Instant creationTimestamp;
private final Instant endTimestamp;
private final DeploymentTargets deploymentTargets;
private final StackSetDriftDetectionDetails stackSetDriftDetectionDetails;
private final String statusReason;
private final StackSetOperationStatusDetails statusDetails;
private StackSetOperation(BuilderImpl builder) {
this.operationId = builder.operationId;
this.stackSetId = builder.stackSetId;
this.action = builder.action;
this.status = builder.status;
this.operationPreferences = builder.operationPreferences;
this.retainStacks = builder.retainStacks;
this.administrationRoleARN = builder.administrationRoleARN;
this.executionRoleName = builder.executionRoleName;
this.creationTimestamp = builder.creationTimestamp;
this.endTimestamp = builder.endTimestamp;
this.deploymentTargets = builder.deploymentTargets;
this.stackSetDriftDetectionDetails = builder.stackSetDriftDetectionDetails;
this.statusReason = builder.statusReason;
this.statusDetails = builder.statusDetails;
}
/**
*
* The unique ID of a stack set operation.
*
*
* @return The unique ID of a stack set operation.
*/
public final String operationId() {
return operationId;
}
/**
*
* The ID of the stack set.
*
*
* @return The ID of the stack set.
*/
public final String stackSetId() {
return stackSetId;
}
/**
*
* The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create and
* delete operations affect only the specified stack set instances that are associated with the specified stack set.
* Update operations affect both the stack set itself, in addition to all associated stack set instances.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #action} will
* return {@link StackSetOperationAction#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #actionAsString}.
*
*
* @return The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create
* and delete operations affect only the specified stack set instances that are associated with the
* specified stack set. Update operations affect both the stack set itself, in addition to all
* associated stack set instances.
* @see StackSetOperationAction
*/
public final StackSetOperationAction action() {
return StackSetOperationAction.fromValue(action);
}
/**
*
* The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create and
* delete operations affect only the specified stack set instances that are associated with the specified stack set.
* Update operations affect both the stack set itself, in addition to all associated stack set instances.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #action} will
* return {@link StackSetOperationAction#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #actionAsString}.
*
*
* @return The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create
* and delete operations affect only the specified stack set instances that are associated with the
* specified stack set. Update operations affect both the stack set itself, in addition to all
* associated stack set instances.
* @see StackSetOperationAction
*/
public final String actionAsString() {
return action;
}
/**
*
* The status of the operation.
*
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value that
* you've set for an operation is applied for each Region during stack create and update operations. If the number
* of failed stacks within a Region exceeds the failure tolerance, the status of the operation in the Region is set
* to FAILED
. This in turn sets the status of the operation as a whole to FAILED
, and
* CloudFormation cancels the operation in any remaining Regions.
*
*
* -
*
* QUEUED
: [Service-managed permissions] For automatic deployments that require a sequence of
* operations, the operation is queued to be performed. For more information, see the stack set operation status codes in the CloudFormation User Guide.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has canceled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without exceeding
* the failure tolerance for the operation.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link StackSetOperationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the operation.
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value
* that you've set for an operation is applied for each Region during stack create and update operations. If
* the number of failed stacks within a Region exceeds the failure tolerance, the status of the operation in
* the Region is set to FAILED
. This in turn sets the status of the operation as a whole to
* FAILED
, and CloudFormation cancels the operation in any remaining Regions.
*
*
* -
*
* QUEUED
: [Service-managed permissions] For automatic deployments that require a sequence of
* operations, the operation is queued to be performed. For more information, see the stack set operation status codes in the CloudFormation User Guide.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has canceled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without
* exceeding the failure tolerance for the operation.
*
*
* @see StackSetOperationStatus
*/
public final StackSetOperationStatus status() {
return StackSetOperationStatus.fromValue(status);
}
/**
*
* The status of the operation.
*
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value that
* you've set for an operation is applied for each Region during stack create and update operations. If the number
* of failed stacks within a Region exceeds the failure tolerance, the status of the operation in the Region is set
* to FAILED
. This in turn sets the status of the operation as a whole to FAILED
, and
* CloudFormation cancels the operation in any remaining Regions.
*
*
* -
*
* QUEUED
: [Service-managed permissions] For automatic deployments that require a sequence of
* operations, the operation is queued to be performed. For more information, see the stack set operation status codes in the CloudFormation User Guide.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has canceled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without exceeding
* the failure tolerance for the operation.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link StackSetOperationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the operation.
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value
* that you've set for an operation is applied for each Region during stack create and update operations. If
* the number of failed stacks within a Region exceeds the failure tolerance, the status of the operation in
* the Region is set to FAILED
. This in turn sets the status of the operation as a whole to
* FAILED
, and CloudFormation cancels the operation in any remaining Regions.
*
*
* -
*
* QUEUED
: [Service-managed permissions] For automatic deployments that require a sequence of
* operations, the operation is queued to be performed. For more information, see the stack set operation status codes in the CloudFormation User Guide.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has canceled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without
* exceeding the failure tolerance for the operation.
*
*
* @see StackSetOperationStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The preferences for how CloudFormation performs this stack set operation.
*
*
* @return The preferences for how CloudFormation performs this stack set operation.
*/
public final StackSetOperationPreferences operationPreferences() {
return operationPreferences;
}
/**
*
* For stack set operations of action type DELETE
, specifies whether to remove the stack instances from
* the specified stack set, but doesn't delete the stacks. You can't re-associate a retained stack, or add an
* existing, saved stack to a new stack set.
*
*
* @return For stack set operations of action type DELETE
, specifies whether to remove the stack
* instances from the specified stack set, but doesn't delete the stacks. You can't re-associate a retained
* stack, or add an existing, saved stack to a new stack set.
*/
public final Boolean retainStacks() {
return retainStacks;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to perform this stack set operation.
*
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within the
* same administrator account. For more information, see Define Permissions
* for Multiple Administrators in the CloudFormation User Guide.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role used to perform this stack set operation.
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within
* the same administrator account. For more information, see Define
* Permissions for Multiple Administrators in the CloudFormation User Guide.
*/
public final String administrationRoleARN() {
return administrationRoleARN;
}
/**
*
* The name of the IAM execution role used to create or update the stack set.
*
*
* Use customized execution roles to control which stack resources users and groups can include in their stack sets.
*
*
* @return The name of the IAM execution role used to create or update the stack set.
*
* Use customized execution roles to control which stack resources users and groups can include in their
* stack sets.
*/
public final String executionRoleName() {
return executionRoleName;
}
/**
*
* The time at which the operation was initiated. Note that the creation times for the stack set operation might
* differ from the creation time of the individual stacks themselves. This is because CloudFormation needs to
* perform preparatory work for the operation, such as dispatching the work to the requested Regions, before
* actually creating the first stacks.
*
*
* @return The time at which the operation was initiated. Note that the creation times for the stack set operation
* might differ from the creation time of the individual stacks themselves. This is because CloudFormation
* needs to perform preparatory work for the operation, such as dispatching the work to the requested
* Regions, before actually creating the first stacks.
*/
public final Instant creationTimestamp() {
return creationTimestamp;
}
/**
*
* The time at which the stack set operation ended, across all accounts and Regions specified. Note that this
* doesn't necessarily mean that the stack set operation was successful, or even attempted, in each account or
* Region.
*
*
* @return The time at which the stack set operation ended, across all accounts and Regions specified. Note that
* this doesn't necessarily mean that the stack set operation was successful, or even attempted, in each
* account or Region.
*/
public final Instant endTimestamp() {
return endTimestamp;
}
/**
*
* [Service-managed permissions] The Organizations accounts affected by the stack operation.
*
*
* @return [Service-managed permissions] The Organizations accounts affected by the stack operation.
*/
public final DeploymentTargets deploymentTargets() {
return deploymentTargets;
}
/**
*
* Detailed information about the drift status of the stack set. This includes information about drift operations
* currently being performed on the stack set.
*
*
* This information will only be present for stack set operations whose Action
type is
* DETECT_DRIFT
.
*
*
* For more information, see Detecting Unmanaged
* Changes in Stack Sets in the CloudFormation User Guide.
*
*
* @return Detailed information about the drift status of the stack set. This includes information about drift
* operations currently being performed on the stack set.
*
* This information will only be present for stack set operations whose Action
type is
* DETECT_DRIFT
.
*
*
* For more information, see Detecting
* Unmanaged Changes in Stack Sets in the CloudFormation User Guide.
*/
public final StackSetDriftDetectionDetails stackSetDriftDetectionDetails() {
return stackSetDriftDetectionDetails;
}
/**
*
* The status of the operation in details.
*
*
* @return The status of the operation in details.
*/
public final String statusReason() {
return statusReason;
}
/**
*
* Detailed information about the StackSet operation.
*
*
* @return Detailed information about the StackSet operation.
*/
public final StackSetOperationStatusDetails statusDetails() {
return statusDetails;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(operationId());
hashCode = 31 * hashCode + Objects.hashCode(stackSetId());
hashCode = 31 * hashCode + Objects.hashCode(actionAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(operationPreferences());
hashCode = 31 * hashCode + Objects.hashCode(retainStacks());
hashCode = 31 * hashCode + Objects.hashCode(administrationRoleARN());
hashCode = 31 * hashCode + Objects.hashCode(executionRoleName());
hashCode = 31 * hashCode + Objects.hashCode(creationTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(endTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(deploymentTargets());
hashCode = 31 * hashCode + Objects.hashCode(stackSetDriftDetectionDetails());
hashCode = 31 * hashCode + Objects.hashCode(statusReason());
hashCode = 31 * hashCode + Objects.hashCode(statusDetails());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StackSetOperation)) {
return false;
}
StackSetOperation other = (StackSetOperation) obj;
return Objects.equals(operationId(), other.operationId()) && Objects.equals(stackSetId(), other.stackSetId())
&& Objects.equals(actionAsString(), other.actionAsString())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(operationPreferences(), other.operationPreferences())
&& Objects.equals(retainStacks(), other.retainStacks())
&& Objects.equals(administrationRoleARN(), other.administrationRoleARN())
&& Objects.equals(executionRoleName(), other.executionRoleName())
&& Objects.equals(creationTimestamp(), other.creationTimestamp())
&& Objects.equals(endTimestamp(), other.endTimestamp())
&& Objects.equals(deploymentTargets(), other.deploymentTargets())
&& Objects.equals(stackSetDriftDetectionDetails(), other.stackSetDriftDetectionDetails())
&& Objects.equals(statusReason(), other.statusReason()) && Objects.equals(statusDetails(), other.statusDetails());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StackSetOperation").add("OperationId", operationId()).add("StackSetId", stackSetId())
.add("Action", actionAsString()).add("Status", statusAsString())
.add("OperationPreferences", operationPreferences()).add("RetainStacks", retainStacks())
.add("AdministrationRoleARN", administrationRoleARN()).add("ExecutionRoleName", executionRoleName())
.add("CreationTimestamp", creationTimestamp()).add("EndTimestamp", endTimestamp())
.add("DeploymentTargets", deploymentTargets())
.add("StackSetDriftDetectionDetails", stackSetDriftDetectionDetails()).add("StatusReason", statusReason())
.add("StatusDetails", statusDetails()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "OperationId":
return Optional.ofNullable(clazz.cast(operationId()));
case "StackSetId":
return Optional.ofNullable(clazz.cast(stackSetId()));
case "Action":
return Optional.ofNullable(clazz.cast(actionAsString()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "OperationPreferences":
return Optional.ofNullable(clazz.cast(operationPreferences()));
case "RetainStacks":
return Optional.ofNullable(clazz.cast(retainStacks()));
case "AdministrationRoleARN":
return Optional.ofNullable(clazz.cast(administrationRoleARN()));
case "ExecutionRoleName":
return Optional.ofNullable(clazz.cast(executionRoleName()));
case "CreationTimestamp":
return Optional.ofNullable(clazz.cast(creationTimestamp()));
case "EndTimestamp":
return Optional.ofNullable(clazz.cast(endTimestamp()));
case "DeploymentTargets":
return Optional.ofNullable(clazz.cast(deploymentTargets()));
case "StackSetDriftDetectionDetails":
return Optional.ofNullable(clazz.cast(stackSetDriftDetectionDetails()));
case "StatusReason":
return Optional.ofNullable(clazz.cast(statusReason()));
case "StatusDetails":
return Optional.ofNullable(clazz.cast(statusDetails()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function