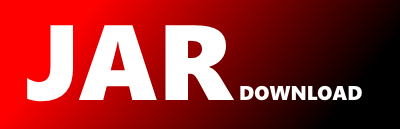
software.amazon.awssdk.services.cloudformation.model.CreateStackRequest Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The input for CreateStack action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateStackRequest extends CloudFormationRequest implements
ToCopyableBuilder {
private static final SdkField STACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackName").getter(getter(CreateStackRequest::stackName)).setter(setter(Builder::stackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackName").build()).build();
private static final SdkField TEMPLATE_BODY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateBody").getter(getter(CreateStackRequest::templateBody)).setter(setter(Builder::templateBody))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateBody").build()).build();
private static final SdkField TEMPLATE_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateURL").getter(getter(CreateStackRequest::templateURL)).setter(setter(Builder::templateURL))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateURL").build()).build();
private static final SdkField> PARAMETERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Parameters")
.getter(getter(CreateStackRequest::parameters))
.setter(setter(Builder::parameters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Parameters").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Parameter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DISABLE_ROLLBACK_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("DisableRollback").getter(getter(CreateStackRequest::disableRollback))
.setter(setter(Builder::disableRollback))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DisableRollback").build()).build();
private static final SdkField ROLLBACK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("RollbackConfiguration")
.getter(getter(CreateStackRequest::rollbackConfiguration)).setter(setter(Builder::rollbackConfiguration))
.constructor(RollbackConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RollbackConfiguration").build())
.build();
private static final SdkField TIMEOUT_IN_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("TimeoutInMinutes").getter(getter(CreateStackRequest::timeoutInMinutes))
.setter(setter(Builder::timeoutInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeoutInMinutes").build()).build();
private static final SdkField> NOTIFICATION_AR_NS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NotificationARNs")
.getter(getter(CreateStackRequest::notificationARNs))
.setter(setter(Builder::notificationARNs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationARNs").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CAPABILITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Capabilities")
.getter(getter(CreateStackRequest::capabilitiesAsStrings))
.setter(setter(Builder::capabilitiesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Capabilities").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> RESOURCE_TYPES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ResourceTypes")
.getter(getter(CreateStackRequest::resourceTypes))
.setter(setter(Builder::resourceTypes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceTypes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleARN").getter(getter(CreateStackRequest::roleARN)).setter(setter(Builder::roleARN))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleARN").build()).build();
private static final SdkField ON_FAILURE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OnFailure").getter(getter(CreateStackRequest::onFailureAsString)).setter(setter(Builder::onFailure))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OnFailure").build()).build();
private static final SdkField STACK_POLICY_BODY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackPolicyBody").getter(getter(CreateStackRequest::stackPolicyBody))
.setter(setter(Builder::stackPolicyBody))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackPolicyBody").build()).build();
private static final SdkField STACK_POLICY_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackPolicyURL").getter(getter(CreateStackRequest::stackPolicyURL))
.setter(setter(Builder::stackPolicyURL))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackPolicyURL").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateStackRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CLIENT_REQUEST_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClientRequestToken").getter(getter(CreateStackRequest::clientRequestToken))
.setter(setter(Builder::clientRequestToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientRequestToken").build())
.build();
private static final SdkField ENABLE_TERMINATION_PROTECTION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EnableTerminationProtection")
.getter(getter(CreateStackRequest::enableTerminationProtection))
.setter(setter(Builder::enableTerminationProtection))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnableTerminationProtection")
.build()).build();
private static final SdkField RETAIN_EXCEPT_ON_CREATE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("RetainExceptOnCreate").getter(getter(CreateStackRequest::retainExceptOnCreate))
.setter(setter(Builder::retainExceptOnCreate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RetainExceptOnCreate").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STACK_NAME_FIELD,
TEMPLATE_BODY_FIELD, TEMPLATE_URL_FIELD, PARAMETERS_FIELD, DISABLE_ROLLBACK_FIELD, ROLLBACK_CONFIGURATION_FIELD,
TIMEOUT_IN_MINUTES_FIELD, NOTIFICATION_AR_NS_FIELD, CAPABILITIES_FIELD, RESOURCE_TYPES_FIELD, ROLE_ARN_FIELD,
ON_FAILURE_FIELD, STACK_POLICY_BODY_FIELD, STACK_POLICY_URL_FIELD, TAGS_FIELD, CLIENT_REQUEST_TOKEN_FIELD,
ENABLE_TERMINATION_PROTECTION_FIELD, RETAIN_EXCEPT_ON_CREATE_FIELD));
private final String stackName;
private final String templateBody;
private final String templateURL;
private final List parameters;
private final Boolean disableRollback;
private final RollbackConfiguration rollbackConfiguration;
private final Integer timeoutInMinutes;
private final List notificationARNs;
private final List capabilities;
private final List resourceTypes;
private final String roleARN;
private final String onFailure;
private final String stackPolicyBody;
private final String stackPolicyURL;
private final List tags;
private final String clientRequestToken;
private final Boolean enableTerminationProtection;
private final Boolean retainExceptOnCreate;
private CreateStackRequest(BuilderImpl builder) {
super(builder);
this.stackName = builder.stackName;
this.templateBody = builder.templateBody;
this.templateURL = builder.templateURL;
this.parameters = builder.parameters;
this.disableRollback = builder.disableRollback;
this.rollbackConfiguration = builder.rollbackConfiguration;
this.timeoutInMinutes = builder.timeoutInMinutes;
this.notificationARNs = builder.notificationARNs;
this.capabilities = builder.capabilities;
this.resourceTypes = builder.resourceTypes;
this.roleARN = builder.roleARN;
this.onFailure = builder.onFailure;
this.stackPolicyBody = builder.stackPolicyBody;
this.stackPolicyURL = builder.stackPolicyURL;
this.tags = builder.tags;
this.clientRequestToken = builder.clientRequestToken;
this.enableTerminationProtection = builder.enableTerminationProtection;
this.retainExceptOnCreate = builder.retainExceptOnCreate;
}
/**
*
* The name that's associated with the stack. The name must be unique in the Region in which you are creating the
* stack.
*
*
*
* A stack name can contain only alphanumeric characters (case sensitive) and hyphens. It must start with an
* alphabetical character and can't be longer than 128 characters.
*
*
*
* @return The name that's associated with the stack. The name must be unique in the Region in which you are
* creating the stack.
*
* A stack name can contain only alphanumeric characters (case sensitive) and hyphens. It must start with an
* alphabetical character and can't be longer than 128 characters.
*
*/
public final String stackName() {
return stackName;
}
/**
*
* Structure containing the template body with a minimum length of 1 byte and a maximum length of 51,200 bytes. For
* more information, go to Template anatomy
* in the CloudFormation User Guide.
*
*
* Conditional: You must specify either the TemplateBody
or the TemplateURL
parameter, but
* not both.
*
*
* @return Structure containing the template body with a minimum length of 1 byte and a maximum length of 51,200
* bytes. For more information, go to Template
* anatomy in the CloudFormation User Guide.
*
* Conditional: You must specify either the TemplateBody
or the TemplateURL
* parameter, but not both.
*/
public final String templateBody() {
return templateBody;
}
/**
*
* Location of file containing the template body. The URL must point to a template (max size: 460,800 bytes) that's
* located in an Amazon S3 bucket or a Systems Manager document. For more information, go to the Template anatomy
* in the CloudFormation User Guide. The location for an Amazon S3 bucket must start with
* https://
.
*
*
* Conditional: You must specify either the TemplateBody
or the TemplateURL
parameter, but
* not both.
*
*
* @return Location of file containing the template body. The URL must point to a template (max size: 460,800 bytes)
* that's located in an Amazon S3 bucket or a Systems Manager document. For more information, go to the Template
* anatomy in the CloudFormation User Guide. The location for an Amazon S3 bucket must start with
* https://
.
*
* Conditional: You must specify either the TemplateBody
or the TemplateURL
* parameter, but not both.
*/
public final String templateURL() {
return templateURL;
}
/**
* For responses, this returns true if the service returned a value for the Parameters property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasParameters() {
return parameters != null && !(parameters instanceof SdkAutoConstructList);
}
/**
*
* A list of Parameter
structures that specify input parameters for the stack. For more information,
* see the
* Parameter
data type.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasParameters} method.
*
*
* @return A list of Parameter
structures that specify input parameters for the stack. For more
* information, see the
* Parameter
data type.
*/
public final List parameters() {
return parameters;
}
/**
*
* Set to true
to disable rollback of the stack if stack creation failed. You can specify either
* DisableRollback
or OnFailure
, but not both.
*
*
* Default: false
*
*
* @return Set to true
to disable rollback of the stack if stack creation failed. You can specify
* either DisableRollback
or OnFailure
, but not both.
*
* Default: false
*/
public final Boolean disableRollback() {
return disableRollback;
}
/**
*
* The rollback triggers for CloudFormation to monitor during stack creation and updating operations, and for the
* specified monitoring period afterwards.
*
*
* @return The rollback triggers for CloudFormation to monitor during stack creation and updating operations, and
* for the specified monitoring period afterwards.
*/
public final RollbackConfiguration rollbackConfiguration() {
return rollbackConfiguration;
}
/**
*
* The amount of time that can pass before the stack status becomes CREATE_FAILED
; if
* DisableRollback
is not set or is set to false
, the stack will be rolled back.
*
*
* @return The amount of time that can pass before the stack status becomes CREATE_FAILED
; if
* DisableRollback
is not set or is set to false
, the stack will be rolled back.
*/
public final Integer timeoutInMinutes() {
return timeoutInMinutes;
}
/**
* For responses, this returns true if the service returned a value for the NotificationARNs property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNotificationARNs() {
return notificationARNs != null && !(notificationARNs instanceof SdkAutoConstructList);
}
/**
*
* The Amazon Simple Notification Service (Amazon SNS) topic ARNs to publish stack related events. You can find your
* Amazon SNS topic ARNs using the Amazon SNS console or your Command Line Interface (CLI).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNotificationARNs} method.
*
*
* @return The Amazon Simple Notification Service (Amazon SNS) topic ARNs to publish stack related events. You can
* find your Amazon SNS topic ARNs using the Amazon SNS console or your Command Line Interface (CLI).
*/
public final List notificationARNs() {
return notificationARNs;
}
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in order
* for CloudFormation to create the stack.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account; for
* example, by creating new Identity and Access Management (IAM) users. For those stacks, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple actions like
* find-and-replace operations, all the way to extensive transformations of entire templates. Because of this, users
* typically create a change set from the processed template, so that they can review the changes resulting from the
* macros before actually creating the stack. If your stack template contains one or more macros, and you choose to
* create a stack directly from the processed template, without first reviewing the resulting changes in a change
* set, you must acknowledge this capability. This includes the AWS::Include
and
* AWS::Serverless
transforms, which are macros hosted by CloudFormation.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you must
* create the stack directly from the template using this capability.
*
*
*
* You should only create stacks directly from a stack template that contains macros if you know what processing the
* macro performs.
*
*
* Each macro relies on an underlying Lambda service function for processing stack templates. Be aware that the
* Lambda function owner can update the function operation without CloudFormation being notified.
*
*
*
* For more information, see Using CloudFormation
* macros to perform custom processing on templates.
*
*
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapabilities} method.
*
*
* @return In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for CloudFormation to create the stack.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stacks, you
* must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple
* actions like find-and-replace operations, all the way to extensive transformations of entire templates.
* Because of this, users typically create a change set from the processed template, so that they can review
* the changes resulting from the macros before actually creating the stack. If your stack template contains
* one or more macros, and you choose to create a stack directly from the processed template, without first
* reviewing the resulting changes in a change set, you must acknowledge this capability. This includes the
* AWS::Include
and
* AWS::Serverless
transforms, which are macros hosted by CloudFormation.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you
* must create the stack directly from the template using this capability.
*
*
*
* You should only create stacks directly from a stack template that contains macros if you know what
* processing the macro performs.
*
*
* Each macro relies on an underlying Lambda service function for processing stack templates. Be aware that
* the Lambda function owner can update the function operation without CloudFormation being notified.
*
*
*
* For more information, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
*/
public final List capabilities() {
return CapabilitiesCopier.copyStringToEnum(capabilities);
}
/**
* For responses, this returns true if the service returned a value for the Capabilities property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCapabilities() {
return capabilities != null && !(capabilities instanceof SdkAutoConstructList);
}
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in order
* for CloudFormation to create the stack.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account; for
* example, by creating new Identity and Access Management (IAM) users. For those stacks, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple actions like
* find-and-replace operations, all the way to extensive transformations of entire templates. Because of this, users
* typically create a change set from the processed template, so that they can review the changes resulting from the
* macros before actually creating the stack. If your stack template contains one or more macros, and you choose to
* create a stack directly from the processed template, without first reviewing the resulting changes in a change
* set, you must acknowledge this capability. This includes the AWS::Include
and
* AWS::Serverless
transforms, which are macros hosted by CloudFormation.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you must
* create the stack directly from the template using this capability.
*
*
*
* You should only create stacks directly from a stack template that contains macros if you know what processing the
* macro performs.
*
*
* Each macro relies on an underlying Lambda service function for processing stack templates. Be aware that the
* Lambda function owner can update the function operation without CloudFormation being notified.
*
*
*
* For more information, see Using CloudFormation
* macros to perform custom processing on templates.
*
*
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapabilities} method.
*
*
* @return In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for CloudFormation to create the stack.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stacks, you
* must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple
* actions like find-and-replace operations, all the way to extensive transformations of entire templates.
* Because of this, users typically create a change set from the processed template, so that they can review
* the changes resulting from the macros before actually creating the stack. If your stack template contains
* one or more macros, and you choose to create a stack directly from the processed template, without first
* reviewing the resulting changes in a change set, you must acknowledge this capability. This includes the
* AWS::Include
and
* AWS::Serverless
transforms, which are macros hosted by CloudFormation.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you
* must create the stack directly from the template using this capability.
*
*
*
* You should only create stacks directly from a stack template that contains macros if you know what
* processing the macro performs.
*
*
* Each macro relies on an underlying Lambda service function for processing stack templates. Be aware that
* the Lambda function owner can update the function operation without CloudFormation being notified.
*
*
*
* For more information, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
*/
public final List capabilitiesAsStrings() {
return capabilities;
}
/**
* For responses, this returns true if the service returned a value for the ResourceTypes property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasResourceTypes() {
return resourceTypes != null && !(resourceTypes instanceof SdkAutoConstructList);
}
/**
*
* The template resource types that you have permissions to work with for this create stack action, such as
* AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
. Use the
* following syntax to describe template resource types: AWS::*
(for all Amazon Web Services
* resources), Custom::*
(for all custom resources), Custom::logical_ID
(for a
* specific custom resource), AWS::service_name::*
(for all resources of a particular Amazon Web
* Services service), and AWS::service_name::resource_logical_ID
(for a specific Amazon
* Web Services resource).
*
*
* If the list of resource types doesn't include a resource that you're creating, the stack creation fails. By
* default, CloudFormation grants permissions to all resource types. Identity and Access Management (IAM) uses this
* parameter for CloudFormation-specific condition keys in IAM policies. For more information, see Controlling Access
* with Identity and Access Management.
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasResourceTypes} method.
*
*
* @return The template resource types that you have permissions to work with for this create stack action, such as
* AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
. Use
* the following syntax to describe template resource types: AWS::*
(for all Amazon Web
* Services resources), Custom::*
(for all custom resources),
* Custom::logical_ID
(for a specific custom resource),
* AWS::service_name::*
(for all resources of a particular Amazon Web Services service),
* and AWS::service_name::resource_logical_ID
(for a specific Amazon Web
* Services resource).
*
* If the list of resource types doesn't include a resource that you're creating, the stack creation fails.
* By default, CloudFormation grants permissions to all resource types. Identity and Access Management (IAM)
* uses this parameter for CloudFormation-specific condition keys in IAM policies. For more information, see
*
* Controlling Access with Identity and Access Management.
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
*/
public final List resourceTypes() {
return resourceTypes;
}
/**
*
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation assumes to
* create the stack. CloudFormation uses the role's credentials to make calls on your behalf. CloudFormation always
* uses this role for all future operations on the stack. Provided that users have permission to operate on the
* stack, CloudFormation uses this role even if the users don't have permission to pass it. Ensure that the role
* grants least privilege.
*
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack. If no
* role is available, CloudFormation uses a temporary session that's generated from your user credentials.
*
*
* @return The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation
* assumes to create the stack. CloudFormation uses the role's credentials to make calls on your behalf.
* CloudFormation always uses this role for all future operations on the stack. Provided that users have
* permission to operate on the stack, CloudFormation uses this role even if the users don't have permission
* to pass it. Ensure that the role grants least privilege.
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack.
* If no role is available, CloudFormation uses a temporary session that's generated from your user
* credentials.
*/
public final String roleARN() {
return roleARN;
}
/**
*
* Determines what action will be taken if stack creation fails. This must be one of: DO_NOTHING
,
* ROLLBACK
, or DELETE
. You can specify either OnFailure
or
* DisableRollback
, but not both.
*
*
* Default: ROLLBACK
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #onFailure} will
* return {@link OnFailure#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #onFailureAsString}.
*
*
* @return Determines what action will be taken if stack creation fails. This must be one of:
* DO_NOTHING
, ROLLBACK
, or DELETE
. You can specify either
* OnFailure
or DisableRollback
, but not both.
*
* Default: ROLLBACK
* @see OnFailure
*/
public final OnFailure onFailure() {
return OnFailure.fromValue(onFailure);
}
/**
*
* Determines what action will be taken if stack creation fails. This must be one of: DO_NOTHING
,
* ROLLBACK
, or DELETE
. You can specify either OnFailure
or
* DisableRollback
, but not both.
*
*
* Default: ROLLBACK
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #onFailure} will
* return {@link OnFailure#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #onFailureAsString}.
*
*
* @return Determines what action will be taken if stack creation fails. This must be one of:
* DO_NOTHING
, ROLLBACK
, or DELETE
. You can specify either
* OnFailure
or DisableRollback
, but not both.
*
* Default: ROLLBACK
* @see OnFailure
*/
public final String onFailureAsString() {
return onFailure;
}
/**
*
* Structure containing the stack policy body. For more information, go to Prevent
* Updates to Stack Resources in the CloudFormation User Guide. You can specify either the
* StackPolicyBody
or the StackPolicyURL
parameter, but not both.
*
*
* @return Structure containing the stack policy body. For more information, go to
* Prevent Updates to Stack Resources in the CloudFormation User Guide. You can specify either
* the StackPolicyBody
or the StackPolicyURL
parameter, but not both.
*/
public final String stackPolicyBody() {
return stackPolicyBody;
}
/**
*
* Location of a file containing the stack policy. The URL must point to a policy (maximum size: 16 KB) located in
* an S3 bucket in the same Region as the stack. The location for an Amazon S3 bucket must start with
* https://
. You can specify either the StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
*
*
* @return Location of a file containing the stack policy. The URL must point to a policy (maximum size: 16 KB)
* located in an S3 bucket in the same Region as the stack. The location for an Amazon S3 bucket must start
* with https://
. You can specify either the StackPolicyBody
or the
* StackPolicyURL
parameter, but not both.
*/
public final String stackPolicyURL() {
return stackPolicyURL;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* Key-value pairs to associate with this stack. CloudFormation also propagates these tags to the resources created
* in the stack. A maximum number of 50 tags can be specified.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return Key-value pairs to associate with this stack. CloudFormation also propagates these tags to the resources
* created in the stack. A maximum number of 50 tags can be specified.
*/
public final List tags() {
return tags;
}
/**
*
* A unique identifier for this CreateStack
request. Specify this token if you plan to retry requests
* so that CloudFormation knows that you're not attempting to create a stack with the same name. You might retry
* CreateStack
requests to ensure that CloudFormation successfully received them.
*
*
* All events initiated by a given stack operation are assigned the same client request token, which you can use to
* track operations. For example, if you execute a CreateStack
operation with the token
* token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations that are
* initiated from the console use the token format Console-StackOperation-ID, which helps you easily identify
* the stack operation . For example, if you create a stack using the console, each stack event would be assigned
* the same token in the following format: Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
*
*
* @return A unique identifier for this CreateStack
request. Specify this token if you plan to retry
* requests so that CloudFormation knows that you're not attempting to create a stack with the same name.
* You might retry CreateStack
requests to ensure that CloudFormation successfully received
* them.
*
* All events initiated by a given stack operation are assigned the same client request token, which you can
* use to track operations. For example, if you execute a CreateStack
operation with the token
* token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations
* that are initiated from the console use the token format Console-StackOperation-ID, which helps
* you easily identify the stack operation . For example, if you create a stack using the console, each
* stack event would be assigned the same token in the following format:
* Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
*/
public final String clientRequestToken() {
return clientRequestToken;
}
/**
*
* Whether to enable termination protection on the specified stack. If a user attempts to delete a stack with
* termination protection enabled, the operation fails and the stack remains unchanged. For more information, see Protecting a
* Stack From Being Deleted in the CloudFormation User Guide. Termination protection is deactivated on
* stacks by default.
*
*
* For nested
* stacks, termination protection is set on the root stack and can't be changed directly on the nested stack.
*
*
* @return Whether to enable termination protection on the specified stack. If a user attempts to delete a stack
* with termination protection enabled, the operation fails and the stack remains unchanged. For more
* information, see Protecting a Stack From Being Deleted in the CloudFormation User Guide. Termination
* protection is deactivated on stacks by default.
*
* For nested
* stacks, termination protection is set on the root stack and can't be changed directly on the nested
* stack.
*/
public final Boolean enableTerminationProtection() {
return enableTerminationProtection;
}
/**
*
* When set to true
, newly created resources are deleted when the operation rolls back. This includes
* newly created resources marked with a deletion policy of Retain
.
*
*
* Default: false
*
*
* @return When set to true
, newly created resources are deleted when the operation rolls back. This
* includes newly created resources marked with a deletion policy of Retain
.
*
* Default: false
*/
public final Boolean retainExceptOnCreate() {
return retainExceptOnCreate;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(stackName());
hashCode = 31 * hashCode + Objects.hashCode(templateBody());
hashCode = 31 * hashCode + Objects.hashCode(templateURL());
hashCode = 31 * hashCode + Objects.hashCode(hasParameters() ? parameters() : null);
hashCode = 31 * hashCode + Objects.hashCode(disableRollback());
hashCode = 31 * hashCode + Objects.hashCode(rollbackConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(timeoutInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(hasNotificationARNs() ? notificationARNs() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCapabilities() ? capabilitiesAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasResourceTypes() ? resourceTypes() : null);
hashCode = 31 * hashCode + Objects.hashCode(roleARN());
hashCode = 31 * hashCode + Objects.hashCode(onFailureAsString());
hashCode = 31 * hashCode + Objects.hashCode(stackPolicyBody());
hashCode = 31 * hashCode + Objects.hashCode(stackPolicyURL());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(clientRequestToken());
hashCode = 31 * hashCode + Objects.hashCode(enableTerminationProtection());
hashCode = 31 * hashCode + Objects.hashCode(retainExceptOnCreate());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateStackRequest)) {
return false;
}
CreateStackRequest other = (CreateStackRequest) obj;
return Objects.equals(stackName(), other.stackName()) && Objects.equals(templateBody(), other.templateBody())
&& Objects.equals(templateURL(), other.templateURL()) && hasParameters() == other.hasParameters()
&& Objects.equals(parameters(), other.parameters()) && Objects.equals(disableRollback(), other.disableRollback())
&& Objects.equals(rollbackConfiguration(), other.rollbackConfiguration())
&& Objects.equals(timeoutInMinutes(), other.timeoutInMinutes())
&& hasNotificationARNs() == other.hasNotificationARNs()
&& Objects.equals(notificationARNs(), other.notificationARNs()) && hasCapabilities() == other.hasCapabilities()
&& Objects.equals(capabilitiesAsStrings(), other.capabilitiesAsStrings())
&& hasResourceTypes() == other.hasResourceTypes() && Objects.equals(resourceTypes(), other.resourceTypes())
&& Objects.equals(roleARN(), other.roleARN()) && Objects.equals(onFailureAsString(), other.onFailureAsString())
&& Objects.equals(stackPolicyBody(), other.stackPolicyBody())
&& Objects.equals(stackPolicyURL(), other.stackPolicyURL()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(clientRequestToken(), other.clientRequestToken())
&& Objects.equals(enableTerminationProtection(), other.enableTerminationProtection())
&& Objects.equals(retainExceptOnCreate(), other.retainExceptOnCreate());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateStackRequest").add("StackName", stackName()).add("TemplateBody", templateBody())
.add("TemplateURL", templateURL()).add("Parameters", hasParameters() ? parameters() : null)
.add("DisableRollback", disableRollback()).add("RollbackConfiguration", rollbackConfiguration())
.add("TimeoutInMinutes", timeoutInMinutes())
.add("NotificationARNs", hasNotificationARNs() ? notificationARNs() : null)
.add("Capabilities", hasCapabilities() ? capabilitiesAsStrings() : null)
.add("ResourceTypes", hasResourceTypes() ? resourceTypes() : null).add("RoleARN", roleARN())
.add("OnFailure", onFailureAsString()).add("StackPolicyBody", stackPolicyBody())
.add("StackPolicyURL", stackPolicyURL()).add("Tags", hasTags() ? tags() : null)
.add("ClientRequestToken", clientRequestToken())
.add("EnableTerminationProtection", enableTerminationProtection())
.add("RetainExceptOnCreate", retainExceptOnCreate()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackName":
return Optional.ofNullable(clazz.cast(stackName()));
case "TemplateBody":
return Optional.ofNullable(clazz.cast(templateBody()));
case "TemplateURL":
return Optional.ofNullable(clazz.cast(templateURL()));
case "Parameters":
return Optional.ofNullable(clazz.cast(parameters()));
case "DisableRollback":
return Optional.ofNullable(clazz.cast(disableRollback()));
case "RollbackConfiguration":
return Optional.ofNullable(clazz.cast(rollbackConfiguration()));
case "TimeoutInMinutes":
return Optional.ofNullable(clazz.cast(timeoutInMinutes()));
case "NotificationARNs":
return Optional.ofNullable(clazz.cast(notificationARNs()));
case "Capabilities":
return Optional.ofNullable(clazz.cast(capabilitiesAsStrings()));
case "ResourceTypes":
return Optional.ofNullable(clazz.cast(resourceTypes()));
case "RoleARN":
return Optional.ofNullable(clazz.cast(roleARN()));
case "OnFailure":
return Optional.ofNullable(clazz.cast(onFailureAsString()));
case "StackPolicyBody":
return Optional.ofNullable(clazz.cast(stackPolicyBody()));
case "StackPolicyURL":
return Optional.ofNullable(clazz.cast(stackPolicyURL()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "ClientRequestToken":
return Optional.ofNullable(clazz.cast(clientRequestToken()));
case "EnableTerminationProtection":
return Optional.ofNullable(clazz.cast(enableTerminationProtection()));
case "RetainExceptOnCreate":
return Optional.ofNullable(clazz.cast(retainExceptOnCreate()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* A stack name can contain only alphanumeric characters (case sensitive) and hyphens. It must start with
* an alphabetical character and can't be longer than 128 characters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stackName(String stackName);
/**
*
* Structure containing the template body with a minimum length of 1 byte and a maximum length of 51,200 bytes.
* For more information, go to Template
* anatomy in the CloudFormation User Guide.
*
*
* Conditional: You must specify either the TemplateBody
or the TemplateURL
parameter,
* but not both.
*
*
* @param templateBody
* Structure containing the template body with a minimum length of 1 byte and a maximum length of 51,200
* bytes. For more information, go to Template
* anatomy in the CloudFormation User Guide.
*
* Conditional: You must specify either the TemplateBody
or the TemplateURL
* parameter, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder templateBody(String templateBody);
/**
*
* Location of file containing the template body. The URL must point to a template (max size: 460,800 bytes)
* that's located in an Amazon S3 bucket or a Systems Manager document. For more information, go to the Template
* anatomy in the CloudFormation User Guide. The location for an Amazon S3 bucket must start with
* https://
.
*
*
* Conditional: You must specify either the TemplateBody
or the TemplateURL
parameter,
* but not both.
*
*
* @param templateURL
* Location of file containing the template body. The URL must point to a template (max size: 460,800
* bytes) that's located in an Amazon S3 bucket or a Systems Manager document. For more information, go
* to the Template
* anatomy in the CloudFormation User Guide. The location for an Amazon S3 bucket must start
* with https://
.
*
* Conditional: You must specify either the TemplateBody
or the TemplateURL
* parameter, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder templateURL(String templateURL);
/**
*
* A list of Parameter
structures that specify input parameters for the stack. For more
* information, see the
* Parameter
data type.
*
*
* @param parameters
* A list of Parameter
structures that specify input parameters for the stack. For more
* information, see the
* Parameter
data type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder parameters(Collection parameters);
/**
*
* A list of Parameter
structures that specify input parameters for the stack. For more
* information, see the
* Parameter
data type.
*
*
* @param parameters
* A list of Parameter
structures that specify input parameters for the stack. For more
* information, see the
* Parameter
data type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder parameters(Parameter... parameters);
/**
*
* A list of Parameter
structures that specify input parameters for the stack. For more
* information, see the
* Parameter
data type.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudformation.model.Parameter.Builder} avoiding the need to create
* one manually via {@link software.amazon.awssdk.services.cloudformation.model.Parameter#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudformation.model.Parameter.Builder#build()} is called immediately
* and its result is passed to {@link #parameters(List)}.
*
* @param parameters
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudformation.model.Parameter.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #parameters(java.util.Collection)
*/
Builder parameters(Consumer... parameters);
/**
*
* Set to true
to disable rollback of the stack if stack creation failed. You can specify either
* DisableRollback
or OnFailure
, but not both.
*
*
* Default: false
*
*
* @param disableRollback
* Set to true
to disable rollback of the stack if stack creation failed. You can specify
* either DisableRollback
or OnFailure
, but not both.
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder disableRollback(Boolean disableRollback);
/**
*
* The rollback triggers for CloudFormation to monitor during stack creation and updating operations, and for
* the specified monitoring period afterwards.
*
*
* @param rollbackConfiguration
* The rollback triggers for CloudFormation to monitor during stack creation and updating operations, and
* for the specified monitoring period afterwards.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder rollbackConfiguration(RollbackConfiguration rollbackConfiguration);
/**
*
* The rollback triggers for CloudFormation to monitor during stack creation and updating operations, and for
* the specified monitoring period afterwards.
*
* This is a convenience method that creates an instance of the {@link RollbackConfiguration.Builder} avoiding
* the need to create one manually via {@link RollbackConfiguration#builder()}.
*
*
* When the {@link Consumer} completes, {@link RollbackConfiguration.Builder#build()} is called immediately and
* its result is passed to {@link #rollbackConfiguration(RollbackConfiguration)}.
*
* @param rollbackConfiguration
* a consumer that will call methods on {@link RollbackConfiguration.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #rollbackConfiguration(RollbackConfiguration)
*/
default Builder rollbackConfiguration(Consumer rollbackConfiguration) {
return rollbackConfiguration(RollbackConfiguration.builder().applyMutation(rollbackConfiguration).build());
}
/**
*
* The amount of time that can pass before the stack status becomes CREATE_FAILED
; if
* DisableRollback
is not set or is set to false
, the stack will be rolled back.
*
*
* @param timeoutInMinutes
* The amount of time that can pass before the stack status becomes CREATE_FAILED
; if
* DisableRollback
is not set or is set to false
, the stack will be rolled
* back.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder timeoutInMinutes(Integer timeoutInMinutes);
/**
*
* The Amazon Simple Notification Service (Amazon SNS) topic ARNs to publish stack related events. You can find
* your Amazon SNS topic ARNs using the Amazon SNS console or your Command Line Interface (CLI).
*
*
* @param notificationARNs
* The Amazon Simple Notification Service (Amazon SNS) topic ARNs to publish stack related events. You
* can find your Amazon SNS topic ARNs using the Amazon SNS console or your Command Line Interface (CLI).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder notificationARNs(Collection notificationARNs);
/**
*
* The Amazon Simple Notification Service (Amazon SNS) topic ARNs to publish stack related events. You can find
* your Amazon SNS topic ARNs using the Amazon SNS console or your Command Line Interface (CLI).
*
*
* @param notificationARNs
* The Amazon Simple Notification Service (Amazon SNS) topic ARNs to publish stack related events. You
* can find your Amazon SNS topic ARNs using the Amazon SNS console or your Command Line Interface (CLI).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder notificationARNs(String... notificationARNs);
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for CloudFormation to create the stack.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account;
* for example, by creating new Identity and Access Management (IAM) users. For those stacks, you must
* explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple actions
* like find-and-replace operations, all the way to extensive transformations of entire templates. Because of
* this, users typically create a change set from the processed template, so that they can review the changes
* resulting from the macros before actually creating the stack. If your stack template contains one or more
* macros, and you choose to create a stack directly from the processed template, without first reviewing the
* resulting changes in a change set, you must acknowledge this capability. This includes the AWS::Include
and
* AWS::Serverless
transforms, which are macros hosted by CloudFormation.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you must
* create the stack directly from the template using this capability.
*
*
*
* You should only create stacks directly from a stack template that contains macros if you know what processing
* the macro performs.
*
*
* Each macro relies on an underlying Lambda service function for processing stack templates. Be aware that the
* Lambda function owner can update the function operation without CloudFormation being notified.
*
*
*
* For more information, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities
* in order for CloudFormation to create the stack.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stacks,
* you must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
* .
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions
* associated with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple
* actions like find-and-replace operations, all the way to extensive transformations of entire
* templates. Because of this, users typically create a change set from the processed template, so that
* they can review the changes resulting from the macros before actually creating the stack. If your
* stack template contains one or more macros, and you choose to create a stack directly from the
* processed template, without first reviewing the resulting changes in a change set, you must
* acknowledge this capability. This includes the AWS::Include
and
* AWS::Serverless
transforms, which are macros hosted by CloudFormation.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you
* must create the stack directly from the template using this capability.
*
*
*
* You should only create stacks directly from a stack template that contains macros if you know what
* processing the macro performs.
*
*
* Each macro relies on an underlying Lambda service function for processing stack templates. Be aware
* that the Lambda function owner can update the function operation without CloudFormation being
* notified.
*
*
*
* For more information, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilitiesWithStrings(Collection capabilities);
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for CloudFormation to create the stack.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account;
* for example, by creating new Identity and Access Management (IAM) users. For those stacks, you must
* explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple actions
* like find-and-replace operations, all the way to extensive transformations of entire templates. Because of
* this, users typically create a change set from the processed template, so that they can review the changes
* resulting from the macros before actually creating the stack. If your stack template contains one or more
* macros, and you choose to create a stack directly from the processed template, without first reviewing the
* resulting changes in a change set, you must acknowledge this capability. This includes the AWS::Include
and
* AWS::Serverless
transforms, which are macros hosted by CloudFormation.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you must
* create the stack directly from the template using this capability.
*
*
*
* You should only create stacks directly from a stack template that contains macros if you know what processing
* the macro performs.
*
*
* Each macro relies on an underlying Lambda service function for processing stack templates. Be aware that the
* Lambda function owner can update the function operation without CloudFormation being notified.
*
*
*
* For more information, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities
* in order for CloudFormation to create the stack.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stacks,
* you must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
* .
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions
* associated with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple
* actions like find-and-replace operations, all the way to extensive transformations of entire
* templates. Because of this, users typically create a change set from the processed template, so that
* they can review the changes resulting from the macros before actually creating the stack. If your
* stack template contains one or more macros, and you choose to create a stack directly from the
* processed template, without first reviewing the resulting changes in a change set, you must
* acknowledge this capability. This includes the AWS::Include
and
* AWS::Serverless
transforms, which are macros hosted by CloudFormation.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you
* must create the stack directly from the template using this capability.
*
*
*
* You should only create stacks directly from a stack template that contains macros if you know what
* processing the macro performs.
*
*
* Each macro relies on an underlying Lambda service function for processing stack templates. Be aware
* that the Lambda function owner can update the function operation without CloudFormation being
* notified.
*
*
*
* For more information, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilitiesWithStrings(String... capabilities);
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for CloudFormation to create the stack.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account;
* for example, by creating new Identity and Access Management (IAM) users. For those stacks, you must
* explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple actions
* like find-and-replace operations, all the way to extensive transformations of entire templates. Because of
* this, users typically create a change set from the processed template, so that they can review the changes
* resulting from the macros before actually creating the stack. If your stack template contains one or more
* macros, and you choose to create a stack directly from the processed template, without first reviewing the
* resulting changes in a change set, you must acknowledge this capability. This includes the AWS::Include
and
* AWS::Serverless
transforms, which are macros hosted by CloudFormation.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you must
* create the stack directly from the template using this capability.
*
*
*
* You should only create stacks directly from a stack template that contains macros if you know what processing
* the macro performs.
*
*
* Each macro relies on an underlying Lambda service function for processing stack templates. Be aware that the
* Lambda function owner can update the function operation without CloudFormation being notified.
*
*
*
* For more information, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities
* in order for CloudFormation to create the stack.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stacks,
* you must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
* .
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions
* associated with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple
* actions like find-and-replace operations, all the way to extensive transformations of entire
* templates. Because of this, users typically create a change set from the processed template, so that
* they can review the changes resulting from the macros before actually creating the stack. If your
* stack template contains one or more macros, and you choose to create a stack directly from the
* processed template, without first reviewing the resulting changes in a change set, you must
* acknowledge this capability. This includes the AWS::Include
and
* AWS::Serverless
transforms, which are macros hosted by CloudFormation.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you
* must create the stack directly from the template using this capability.
*
*
*
* You should only create stacks directly from a stack template that contains macros if you know what
* processing the macro performs.
*
*
* Each macro relies on an underlying Lambda service function for processing stack templates. Be aware
* that the Lambda function owner can update the function operation without CloudFormation being
* notified.
*
*
*
* For more information, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilities(Collection capabilities);
/**
*
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities in
* order for CloudFormation to create the stack.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account;
* for example, by creating new Identity and Access Management (IAM) users. For those stacks, you must
* explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple actions
* like find-and-replace operations, all the way to extensive transformations of entire templates. Because of
* this, users typically create a change set from the processed template, so that they can review the changes
* resulting from the macros before actually creating the stack. If your stack template contains one or more
* macros, and you choose to create a stack directly from the processed template, without first reviewing the
* resulting changes in a change set, you must acknowledge this capability. This includes the AWS::Include
and
* AWS::Serverless
transforms, which are macros hosted by CloudFormation.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you must
* create the stack directly from the template using this capability.
*
*
*
* You should only create stacks directly from a stack template that contains macros if you know what processing
* the macro performs.
*
*
* Each macro relies on an underlying Lambda service function for processing stack templates. Be aware that the
* Lambda function owner can update the function operation without CloudFormation being notified.
*
*
*
* For more information, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack template contains certain capabilities
* in order for CloudFormation to create the stack.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stacks,
* you must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
* .
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions
* associated with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some template contain macros. Macros perform custom processing on templates; this can include simple
* actions like find-and-replace operations, all the way to extensive transformations of entire
* templates. Because of this, users typically create a change set from the processed template, so that
* they can review the changes resulting from the macros before actually creating the stack. If your
* stack template contains one or more macros, and you choose to create a stack directly from the
* processed template, without first reviewing the resulting changes in a change set, you must
* acknowledge this capability. This includes the AWS::Include
and
* AWS::Serverless
transforms, which are macros hosted by CloudFormation.
*
*
* If you want to create a stack from a stack template that contains macros and nested stacks, you
* must create the stack directly from the template using this capability.
*
*
*
* You should only create stacks directly from a stack template that contains macros if you know what
* processing the macro performs.
*
*
* Each macro relies on an underlying Lambda service function for processing stack templates. Be aware
* that the Lambda function owner can update the function operation without CloudFormation being
* notified.
*
*
*
* For more information, see Using
* CloudFormation macros to perform custom processing on templates.
*
*
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capabilities(Capability... capabilities);
/**
*
* The template resource types that you have permissions to work with for this create stack action, such as
* AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
. Use the
* following syntax to describe template resource types: AWS::*
(for all Amazon Web Services
* resources), Custom::*
(for all custom resources), Custom::logical_ID
(for a
* specific custom resource), AWS::service_name::*
(for all resources of a particular Amazon
* Web Services service), and AWS::service_name::resource_logical_ID
(for a specific
* Amazon Web Services resource).
*
*
* If the list of resource types doesn't include a resource that you're creating, the stack creation fails. By
* default, CloudFormation grants permissions to all resource types. Identity and Access Management (IAM) uses
* this parameter for CloudFormation-specific condition keys in IAM policies. For more information, see Controlling
* Access with Identity and Access Management.
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
*
*
* @param resourceTypes
* The template resource types that you have permissions to work with for this create stack action, such
* as AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
* . Use the following syntax to describe template resource types: AWS::*
(for all Amazon
* Web Services resources), Custom::*
(for all custom resources),
* Custom::logical_ID
(for a specific custom resource),
* AWS::service_name::*
(for all resources of a particular Amazon Web Services
* service), and AWS::service_name::resource_logical_ID
(for a specific
* Amazon Web Services resource).
*
* If the list of resource types doesn't include a resource that you're creating, the stack creation
* fails. By default, CloudFormation grants permissions to all resource types. Identity and Access
* Management (IAM) uses this parameter for CloudFormation-specific condition keys in IAM policies. For
* more information, see Controlling Access with Identity and Access Management.
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder resourceTypes(Collection resourceTypes);
/**
*
* The template resource types that you have permissions to work with for this create stack action, such as
* AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
. Use the
* following syntax to describe template resource types: AWS::*
(for all Amazon Web Services
* resources), Custom::*
(for all custom resources), Custom::logical_ID
(for a
* specific custom resource), AWS::service_name::*
(for all resources of a particular Amazon
* Web Services service), and AWS::service_name::resource_logical_ID
(for a specific
* Amazon Web Services resource).
*
*
* If the list of resource types doesn't include a resource that you're creating, the stack creation fails. By
* default, CloudFormation grants permissions to all resource types. Identity and Access Management (IAM) uses
* this parameter for CloudFormation-specific condition keys in IAM policies. For more information, see Controlling
* Access with Identity and Access Management.
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
*
*
* @param resourceTypes
* The template resource types that you have permissions to work with for this create stack action, such
* as AWS::EC2::Instance
, AWS::EC2::*
, or Custom::MyCustomInstance
* . Use the following syntax to describe template resource types: AWS::*
(for all Amazon
* Web Services resources), Custom::*
(for all custom resources),
* Custom::logical_ID
(for a specific custom resource),
* AWS::service_name::*
(for all resources of a particular Amazon Web Services
* service), and AWS::service_name::resource_logical_ID
(for a specific
* Amazon Web Services resource).
*
* If the list of resource types doesn't include a resource that you're creating, the stack creation
* fails. By default, CloudFormation grants permissions to all resource types. Identity and Access
* Management (IAM) uses this parameter for CloudFormation-specific condition keys in IAM policies. For
* more information, see Controlling Access with Identity and Access Management.
*
*
*
* Only one of the Capabilities
and ResourceType
parameters can be specified.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder resourceTypes(String... resourceTypes);
/**
*
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation assumes to
* create the stack. CloudFormation uses the role's credentials to make calls on your behalf. CloudFormation
* always uses this role for all future operations on the stack. Provided that users have permission to operate
* on the stack, CloudFormation uses this role even if the users don't have permission to pass it. Ensure that
* the role grants least privilege.
*
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack. If
* no role is available, CloudFormation uses a temporary session that's generated from your user credentials.
*
*
* @param roleARN
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation
* assumes to create the stack. CloudFormation uses the role's credentials to make calls on your behalf.
* CloudFormation always uses this role for all future operations on the stack. Provided that users have
* permission to operate on the stack, CloudFormation uses this role even if the users don't have
* permission to pass it. Ensure that the role grants least privilege.
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the
* stack. If no role is available, CloudFormation uses a temporary session that's generated from your
* user credentials.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder roleARN(String roleARN);
/**
*
* Determines what action will be taken if stack creation fails. This must be one of: DO_NOTHING
,
* ROLLBACK
, or DELETE
. You can specify either OnFailure
or
* DisableRollback
, but not both.
*
*
* Default: ROLLBACK
*
*
* @param onFailure
* Determines what action will be taken if stack creation fails. This must be one of:
* DO_NOTHING
, ROLLBACK
, or DELETE
. You can specify either
* OnFailure
or DisableRollback
, but not both.
*
* Default: ROLLBACK
* @see OnFailure
* @return Returns a reference to this object so that method calls can be chained together.
* @see OnFailure
*/
Builder onFailure(String onFailure);
/**
*
* Determines what action will be taken if stack creation fails. This must be one of: DO_NOTHING
,
* ROLLBACK
, or DELETE
. You can specify either OnFailure
or
* DisableRollback
, but not both.
*
*
* Default: ROLLBACK
*
*
* @param onFailure
* Determines what action will be taken if stack creation fails. This must be one of:
* DO_NOTHING
, ROLLBACK
, or DELETE
. You can specify either
* OnFailure
or DisableRollback
, but not both.
*
* Default: ROLLBACK
* @see OnFailure
* @return Returns a reference to this object so that method calls can be chained together.
* @see OnFailure
*/
Builder onFailure(OnFailure onFailure);
/**
*
* Structure containing the stack policy body. For more information, go to Prevent
* Updates to Stack Resources in the CloudFormation User Guide. You can specify either the
* StackPolicyBody
or the StackPolicyURL
parameter, but not both.
*
*
* @param stackPolicyBody
* Structure containing the stack policy body. For more information, go to
* Prevent Updates to Stack Resources in the CloudFormation User Guide. You can specify either
* the StackPolicyBody
or the StackPolicyURL
parameter, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stackPolicyBody(String stackPolicyBody);
/**
*
* Location of a file containing the stack policy. The URL must point to a policy (maximum size: 16 KB) located
* in an S3 bucket in the same Region as the stack. The location for an Amazon S3 bucket must start with
* https://
. You can specify either the StackPolicyBody
or the
* StackPolicyURL
parameter, but not both.
*
*
* @param stackPolicyURL
* Location of a file containing the stack policy. The URL must point to a policy (maximum size: 16 KB)
* located in an S3 bucket in the same Region as the stack. The location for an Amazon S3 bucket must
* start with https://
. You can specify either the StackPolicyBody
or the
* StackPolicyURL
parameter, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stackPolicyURL(String stackPolicyURL);
/**
*
* Key-value pairs to associate with this stack. CloudFormation also propagates these tags to the resources
* created in the stack. A maximum number of 50 tags can be specified.
*
*
* @param tags
* Key-value pairs to associate with this stack. CloudFormation also propagates these tags to the
* resources created in the stack. A maximum number of 50 tags can be specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* Key-value pairs to associate with this stack. CloudFormation also propagates these tags to the resources
* created in the stack. A maximum number of 50 tags can be specified.
*
*
* @param tags
* Key-value pairs to associate with this stack. CloudFormation also propagates these tags to the
* resources created in the stack. A maximum number of 50 tags can be specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* Key-value pairs to associate with this stack. CloudFormation also propagates these tags to the resources
* created in the stack. A maximum number of 50 tags can be specified.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.cloudformation.model.Tag.Builder} avoiding the need to create one
* manually via {@link software.amazon.awssdk.services.cloudformation.model.Tag#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.cloudformation.model.Tag.Builder#build()} is called immediately and
* its result is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.cloudformation.model.Tag.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(java.util.Collection)
*/
Builder tags(Consumer... tags);
/**
*
* A unique identifier for this CreateStack
request. Specify this token if you plan to retry
* requests so that CloudFormation knows that you're not attempting to create a stack with the same name. You
* might retry CreateStack
requests to ensure that CloudFormation successfully received them.
*
*
* All events initiated by a given stack operation are assigned the same client request token, which you can use
* to track operations. For example, if you execute a CreateStack
operation with the token
* token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations that
* are initiated from the console use the token format Console-StackOperation-ID, which helps you easily
* identify the stack operation . For example, if you create a stack using the console, each stack event would
* be assigned the same token in the following format:
* Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
*
*
* @param clientRequestToken
* A unique identifier for this CreateStack
request. Specify this token if you plan to retry
* requests so that CloudFormation knows that you're not attempting to create a stack with the same name.
* You might retry CreateStack
requests to ensure that CloudFormation successfully received
* them.
*
* All events initiated by a given stack operation are assigned the same client request token, which you
* can use to track operations. For example, if you execute a CreateStack
operation with the
* token token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations
* that are initiated from the console use the token format Console-StackOperation-ID, which helps
* you easily identify the stack operation . For example, if you create a stack using the console, each
* stack event would be assigned the same token in the following format:
* Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clientRequestToken(String clientRequestToken);
/**
*
* Whether to enable termination protection on the specified stack. If a user attempts to delete a stack with
* termination protection enabled, the operation fails and the stack remains unchanged. For more information,
* see
* Protecting a Stack From Being Deleted in the CloudFormation User Guide. Termination protection is
* deactivated on stacks by default.
*
*
* For nested
* stacks, termination protection is set on the root stack and can't be changed directly on the nested
* stack.
*
*
* @param enableTerminationProtection
* Whether to enable termination protection on the specified stack. If a user attempts to delete a stack
* with termination protection enabled, the operation fails and the stack remains unchanged. For more
* information, see Protecting a Stack From Being Deleted in the CloudFormation User Guide. Termination
* protection is deactivated on stacks by default.
*
* For nested stacks, termination protection is set on the root stack and can't be changed directly on
* the nested stack.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder enableTerminationProtection(Boolean enableTerminationProtection);
/**
*
* When set to true
, newly created resources are deleted when the operation rolls back. This
* includes newly created resources marked with a deletion policy of Retain
.
*
*
* Default: false
*
*
* @param retainExceptOnCreate
* When set to true
, newly created resources are deleted when the operation rolls back. This
* includes newly created resources marked with a deletion policy of Retain
.
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder retainExceptOnCreate(Boolean retainExceptOnCreate);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends CloudFormationRequest.BuilderImpl implements Builder {
private String stackName;
private String templateBody;
private String templateURL;
private List parameters = DefaultSdkAutoConstructList.getInstance();
private Boolean disableRollback;
private RollbackConfiguration rollbackConfiguration;
private Integer timeoutInMinutes;
private List notificationARNs = DefaultSdkAutoConstructList.getInstance();
private List capabilities = DefaultSdkAutoConstructList.getInstance();
private List resourceTypes = DefaultSdkAutoConstructList.getInstance();
private String roleARN;
private String onFailure;
private String stackPolicyBody;
private String stackPolicyURL;
private List tags = DefaultSdkAutoConstructList.getInstance();
private String clientRequestToken;
private Boolean enableTerminationProtection;
private Boolean retainExceptOnCreate;
private BuilderImpl() {
}
private BuilderImpl(CreateStackRequest model) {
super(model);
stackName(model.stackName);
templateBody(model.templateBody);
templateURL(model.templateURL);
parameters(model.parameters);
disableRollback(model.disableRollback);
rollbackConfiguration(model.rollbackConfiguration);
timeoutInMinutes(model.timeoutInMinutes);
notificationARNs(model.notificationARNs);
capabilitiesWithStrings(model.capabilities);
resourceTypes(model.resourceTypes);
roleARN(model.roleARN);
onFailure(model.onFailure);
stackPolicyBody(model.stackPolicyBody);
stackPolicyURL(model.stackPolicyURL);
tags(model.tags);
clientRequestToken(model.clientRequestToken);
enableTerminationProtection(model.enableTerminationProtection);
retainExceptOnCreate(model.retainExceptOnCreate);
}
public final String getStackName() {
return stackName;
}
public final void setStackName(String stackName) {
this.stackName = stackName;
}
@Override
public final Builder stackName(String stackName) {
this.stackName = stackName;
return this;
}
public final String getTemplateBody() {
return templateBody;
}
public final void setTemplateBody(String templateBody) {
this.templateBody = templateBody;
}
@Override
public final Builder templateBody(String templateBody) {
this.templateBody = templateBody;
return this;
}
public final String getTemplateURL() {
return templateURL;
}
public final void setTemplateURL(String templateURL) {
this.templateURL = templateURL;
}
@Override
public final Builder templateURL(String templateURL) {
this.templateURL = templateURL;
return this;
}
public final List getParameters() {
List result = ParametersCopier.copyToBuilder(this.parameters);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setParameters(Collection parameters) {
this.parameters = ParametersCopier.copyFromBuilder(parameters);
}
@Override
public final Builder parameters(Collection parameters) {
this.parameters = ParametersCopier.copy(parameters);
return this;
}
@Override
@SafeVarargs
public final Builder parameters(Parameter... parameters) {
parameters(Arrays.asList(parameters));
return this;
}
@Override
@SafeVarargs
public final Builder parameters(Consumer... parameters) {
parameters(Stream.of(parameters).map(c -> Parameter.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final Boolean getDisableRollback() {
return disableRollback;
}
public final void setDisableRollback(Boolean disableRollback) {
this.disableRollback = disableRollback;
}
@Override
public final Builder disableRollback(Boolean disableRollback) {
this.disableRollback = disableRollback;
return this;
}
public final RollbackConfiguration.Builder getRollbackConfiguration() {
return rollbackConfiguration != null ? rollbackConfiguration.toBuilder() : null;
}
public final void setRollbackConfiguration(RollbackConfiguration.BuilderImpl rollbackConfiguration) {
this.rollbackConfiguration = rollbackConfiguration != null ? rollbackConfiguration.build() : null;
}
@Override
public final Builder rollbackConfiguration(RollbackConfiguration rollbackConfiguration) {
this.rollbackConfiguration = rollbackConfiguration;
return this;
}
public final Integer getTimeoutInMinutes() {
return timeoutInMinutes;
}
public final void setTimeoutInMinutes(Integer timeoutInMinutes) {
this.timeoutInMinutes = timeoutInMinutes;
}
@Override
public final Builder timeoutInMinutes(Integer timeoutInMinutes) {
this.timeoutInMinutes = timeoutInMinutes;
return this;
}
public final Collection getNotificationARNs() {
if (notificationARNs instanceof SdkAutoConstructList) {
return null;
}
return notificationARNs;
}
public final void setNotificationARNs(Collection notificationARNs) {
this.notificationARNs = NotificationARNsCopier.copy(notificationARNs);
}
@Override
public final Builder notificationARNs(Collection notificationARNs) {
this.notificationARNs = NotificationARNsCopier.copy(notificationARNs);
return this;
}
@Override
@SafeVarargs
public final Builder notificationARNs(String... notificationARNs) {
notificationARNs(Arrays.asList(notificationARNs));
return this;
}
public final Collection getCapabilities() {
if (capabilities instanceof SdkAutoConstructList) {
return null;
}
return capabilities;
}
public final void setCapabilities(Collection capabilities) {
this.capabilities = CapabilitiesCopier.copy(capabilities);
}
@Override
public final Builder capabilitiesWithStrings(Collection capabilities) {
this.capabilities = CapabilitiesCopier.copy(capabilities);
return this;
}
@Override
@SafeVarargs
public final Builder capabilitiesWithStrings(String... capabilities) {
capabilitiesWithStrings(Arrays.asList(capabilities));
return this;
}
@Override
public final Builder capabilities(Collection capabilities) {
this.capabilities = CapabilitiesCopier.copyEnumToString(capabilities);
return this;
}
@Override
@SafeVarargs
public final Builder capabilities(Capability... capabilities) {
capabilities(Arrays.asList(capabilities));
return this;
}
public final Collection getResourceTypes() {
if (resourceTypes instanceof SdkAutoConstructList) {
return null;
}
return resourceTypes;
}
public final void setResourceTypes(Collection resourceTypes) {
this.resourceTypes = ResourceTypesCopier.copy(resourceTypes);
}
@Override
public final Builder resourceTypes(Collection resourceTypes) {
this.resourceTypes = ResourceTypesCopier.copy(resourceTypes);
return this;
}
@Override
@SafeVarargs
public final Builder resourceTypes(String... resourceTypes) {
resourceTypes(Arrays.asList(resourceTypes));
return this;
}
public final String getRoleARN() {
return roleARN;
}
public final void setRoleARN(String roleARN) {
this.roleARN = roleARN;
}
@Override
public final Builder roleARN(String roleARN) {
this.roleARN = roleARN;
return this;
}
public final String getOnFailure() {
return onFailure;
}
public final void setOnFailure(String onFailure) {
this.onFailure = onFailure;
}
@Override
public final Builder onFailure(String onFailure) {
this.onFailure = onFailure;
return this;
}
@Override
public final Builder onFailure(OnFailure onFailure) {
this.onFailure(onFailure == null ? null : onFailure.toString());
return this;
}
public final String getStackPolicyBody() {
return stackPolicyBody;
}
public final void setStackPolicyBody(String stackPolicyBody) {
this.stackPolicyBody = stackPolicyBody;
}
@Override
public final Builder stackPolicyBody(String stackPolicyBody) {
this.stackPolicyBody = stackPolicyBody;
return this;
}
public final String getStackPolicyURL() {
return stackPolicyURL;
}
public final void setStackPolicyURL(String stackPolicyURL) {
this.stackPolicyURL = stackPolicyURL;
}
@Override
public final Builder stackPolicyURL(String stackPolicyURL) {
this.stackPolicyURL = stackPolicyURL;
return this;
}
public final List getTags() {
List result = TagsCopier.copyToBuilder(this.tags);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTags(Collection tags) {
this.tags = TagsCopier.copyFromBuilder(tags);
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagsCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final String getClientRequestToken() {
return clientRequestToken;
}
public final void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
@Override
public final Builder clientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
return this;
}
public final Boolean getEnableTerminationProtection() {
return enableTerminationProtection;
}
public final void setEnableTerminationProtection(Boolean enableTerminationProtection) {
this.enableTerminationProtection = enableTerminationProtection;
}
@Override
public final Builder enableTerminationProtection(Boolean enableTerminationProtection) {
this.enableTerminationProtection = enableTerminationProtection;
return this;
}
public final Boolean getRetainExceptOnCreate() {
return retainExceptOnCreate;
}
public final void setRetainExceptOnCreate(Boolean retainExceptOnCreate) {
this.retainExceptOnCreate = retainExceptOnCreate;
}
@Override
public final Builder retainExceptOnCreate(Boolean retainExceptOnCreate) {
this.retainExceptOnCreate = retainExceptOnCreate;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateStackRequest build() {
return new CreateStackRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}