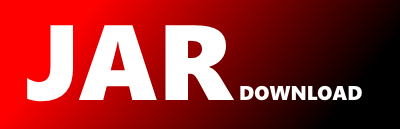
software.amazon.awssdk.services.cloudformation.model.StackEvent Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The StackEvent data type.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class StackEvent implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField STACK_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackId").getter(getter(StackEvent::stackId)).setter(setter(Builder::stackId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackId").build()).build();
private static final SdkField EVENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EventId").getter(getter(StackEvent::eventId)).setter(setter(Builder::eventId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EventId").build()).build();
private static final SdkField STACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackName").getter(getter(StackEvent::stackName)).setter(setter(Builder::stackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackName").build()).build();
private static final SdkField LOGICAL_RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LogicalResourceId").getter(getter(StackEvent::logicalResourceId))
.setter(setter(Builder::logicalResourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogicalResourceId").build()).build();
private static final SdkField PHYSICAL_RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PhysicalResourceId").getter(getter(StackEvent::physicalResourceId))
.setter(setter(Builder::physicalResourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PhysicalResourceId").build())
.build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceType").getter(getter(StackEvent::resourceType)).setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceType").build()).build();
private static final SdkField TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("Timestamp").getter(getter(StackEvent::timestamp)).setter(setter(Builder::timestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Timestamp").build()).build();
private static final SdkField RESOURCE_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceStatus").getter(getter(StackEvent::resourceStatusAsString))
.setter(setter(Builder::resourceStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceStatus").build()).build();
private static final SdkField RESOURCE_STATUS_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceStatusReason").getter(getter(StackEvent::resourceStatusReason))
.setter(setter(Builder::resourceStatusReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceStatusReason").build())
.build();
private static final SdkField RESOURCE_PROPERTIES_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceProperties").getter(getter(StackEvent::resourceProperties))
.setter(setter(Builder::resourceProperties))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceProperties").build())
.build();
private static final SdkField CLIENT_REQUEST_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClientRequestToken").getter(getter(StackEvent::clientRequestToken))
.setter(setter(Builder::clientRequestToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientRequestToken").build())
.build();
private static final SdkField HOOK_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HookType").getter(getter(StackEvent::hookType)).setter(setter(Builder::hookType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HookType").build()).build();
private static final SdkField HOOK_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HookStatus").getter(getter(StackEvent::hookStatusAsString)).setter(setter(Builder::hookStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HookStatus").build()).build();
private static final SdkField HOOK_STATUS_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HookStatusReason").getter(getter(StackEvent::hookStatusReason))
.setter(setter(Builder::hookStatusReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HookStatusReason").build()).build();
private static final SdkField HOOK_INVOCATION_POINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HookInvocationPoint").getter(getter(StackEvent::hookInvocationPointAsString))
.setter(setter(Builder::hookInvocationPoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HookInvocationPoint").build())
.build();
private static final SdkField HOOK_FAILURE_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HookFailureMode").getter(getter(StackEvent::hookFailureModeAsString))
.setter(setter(Builder::hookFailureMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HookFailureMode").build()).build();
private static final SdkField DETAILED_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DetailedStatus").getter(getter(StackEvent::detailedStatusAsString))
.setter(setter(Builder::detailedStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DetailedStatus").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STACK_ID_FIELD,
EVENT_ID_FIELD, STACK_NAME_FIELD, LOGICAL_RESOURCE_ID_FIELD, PHYSICAL_RESOURCE_ID_FIELD, RESOURCE_TYPE_FIELD,
TIMESTAMP_FIELD, RESOURCE_STATUS_FIELD, RESOURCE_STATUS_REASON_FIELD, RESOURCE_PROPERTIES_FIELD,
CLIENT_REQUEST_TOKEN_FIELD, HOOK_TYPE_FIELD, HOOK_STATUS_FIELD, HOOK_STATUS_REASON_FIELD,
HOOK_INVOCATION_POINT_FIELD, HOOK_FAILURE_MODE_FIELD, DETAILED_STATUS_FIELD));
private static final long serialVersionUID = 1L;
private final String stackId;
private final String eventId;
private final String stackName;
private final String logicalResourceId;
private final String physicalResourceId;
private final String resourceType;
private final Instant timestamp;
private final String resourceStatus;
private final String resourceStatusReason;
private final String resourceProperties;
private final String clientRequestToken;
private final String hookType;
private final String hookStatus;
private final String hookStatusReason;
private final String hookInvocationPoint;
private final String hookFailureMode;
private final String detailedStatus;
private StackEvent(BuilderImpl builder) {
this.stackId = builder.stackId;
this.eventId = builder.eventId;
this.stackName = builder.stackName;
this.logicalResourceId = builder.logicalResourceId;
this.physicalResourceId = builder.physicalResourceId;
this.resourceType = builder.resourceType;
this.timestamp = builder.timestamp;
this.resourceStatus = builder.resourceStatus;
this.resourceStatusReason = builder.resourceStatusReason;
this.resourceProperties = builder.resourceProperties;
this.clientRequestToken = builder.clientRequestToken;
this.hookType = builder.hookType;
this.hookStatus = builder.hookStatus;
this.hookStatusReason = builder.hookStatusReason;
this.hookInvocationPoint = builder.hookInvocationPoint;
this.hookFailureMode = builder.hookFailureMode;
this.detailedStatus = builder.detailedStatus;
}
/**
*
* The unique ID name of the instance of the stack.
*
*
* @return The unique ID name of the instance of the stack.
*/
public final String stackId() {
return stackId;
}
/**
*
* The unique ID of this event.
*
*
* @return The unique ID of this event.
*/
public final String eventId() {
return eventId;
}
/**
*
* The name associated with a stack.
*
*
* @return The name associated with a stack.
*/
public final String stackName() {
return stackName;
}
/**
*
* The logical name of the resource specified in the template.
*
*
* @return The logical name of the resource specified in the template.
*/
public final String logicalResourceId() {
return logicalResourceId;
}
/**
*
* The name or unique identifier associated with the physical instance of the resource.
*
*
* @return The name or unique identifier associated with the physical instance of the resource.
*/
public final String physicalResourceId() {
return physicalResourceId;
}
/**
*
* Type of resource. (For more information, go to Amazon
* Web Services Resource Types Reference in the CloudFormation User Guide.)
*
*
* @return Type of resource. (For more information, go to Amazon Web Services Resource Types Reference in the CloudFormation User Guide.)
*/
public final String resourceType() {
return resourceType;
}
/**
*
* Time the status was updated.
*
*
* @return Time the status was updated.
*/
public final Instant timestamp() {
return timestamp;
}
/**
*
* Current status of the resource.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceStatus}
* will return {@link ResourceStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #resourceStatusAsString}.
*
*
* @return Current status of the resource.
* @see ResourceStatus
*/
public final ResourceStatus resourceStatus() {
return ResourceStatus.fromValue(resourceStatus);
}
/**
*
* Current status of the resource.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceStatus}
* will return {@link ResourceStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #resourceStatusAsString}.
*
*
* @return Current status of the resource.
* @see ResourceStatus
*/
public final String resourceStatusAsString() {
return resourceStatus;
}
/**
*
* Success/failure message associated with the resource.
*
*
* @return Success/failure message associated with the resource.
*/
public final String resourceStatusReason() {
return resourceStatusReason;
}
/**
*
* BLOB of the properties used to create the resource.
*
*
* @return BLOB of the properties used to create the resource.
*/
public final String resourceProperties() {
return resourceProperties;
}
/**
*
* The token passed to the operation that generated this event.
*
*
* All events triggered by a given stack operation are assigned the same client request token, which you can use to
* track operations. For example, if you execute a CreateStack
operation with the token
* token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations that are
* initiated from the console use the token format Console-StackOperation-ID, which helps you easily identify
* the stack operation . For example, if you create a stack using the console, each stack event would be assigned
* the same token in the following format: Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
*
*
* @return The token passed to the operation that generated this event.
*
* All events triggered by a given stack operation are assigned the same client request token, which you can
* use to track operations. For example, if you execute a CreateStack
operation with the token
* token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations
* that are initiated from the console use the token format Console-StackOperation-ID, which helps
* you easily identify the stack operation . For example, if you create a stack using the console, each
* stack event would be assigned the same token in the following format:
* Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
*/
public final String clientRequestToken() {
return clientRequestToken;
}
/**
*
* The name of the hook.
*
*
* @return The name of the hook.
*/
public final String hookType() {
return hookType;
}
/**
*
* Provides the status of the change set hook.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #hookStatus} will
* return {@link HookStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #hookStatusAsString}.
*
*
* @return Provides the status of the change set hook.
* @see HookStatus
*/
public final HookStatus hookStatus() {
return HookStatus.fromValue(hookStatus);
}
/**
*
* Provides the status of the change set hook.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #hookStatus} will
* return {@link HookStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #hookStatusAsString}.
*
*
* @return Provides the status of the change set hook.
* @see HookStatus
*/
public final String hookStatusAsString() {
return hookStatus;
}
/**
*
* Provides the reason for the hook status.
*
*
* @return Provides the reason for the hook status.
*/
public final String hookStatusReason() {
return hookStatusReason;
}
/**
*
* Invocation points are points in provisioning logic where hooks are initiated.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #hookInvocationPoint} will return {@link HookInvocationPoint#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #hookInvocationPointAsString}.
*
*
* @return Invocation points are points in provisioning logic where hooks are initiated.
* @see HookInvocationPoint
*/
public final HookInvocationPoint hookInvocationPoint() {
return HookInvocationPoint.fromValue(hookInvocationPoint);
}
/**
*
* Invocation points are points in provisioning logic where hooks are initiated.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #hookInvocationPoint} will return {@link HookInvocationPoint#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #hookInvocationPointAsString}.
*
*
* @return Invocation points are points in provisioning logic where hooks are initiated.
* @see HookInvocationPoint
*/
public final String hookInvocationPointAsString() {
return hookInvocationPoint;
}
/**
*
* Specify the hook failure mode for non-compliant resources in the followings ways.
*
*
* -
*
* FAIL
Stops provisioning resources.
*
*
* -
*
* WARN
Allows provisioning to continue with a warning message.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #hookFailureMode}
* will return {@link HookFailureMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #hookFailureModeAsString}.
*
*
* @return Specify the hook failure mode for non-compliant resources in the followings ways.
*
* -
*
* FAIL
Stops provisioning resources.
*
*
* -
*
* WARN
Allows provisioning to continue with a warning message.
*
*
* @see HookFailureMode
*/
public final HookFailureMode hookFailureMode() {
return HookFailureMode.fromValue(hookFailureMode);
}
/**
*
* Specify the hook failure mode for non-compliant resources in the followings ways.
*
*
* -
*
* FAIL
Stops provisioning resources.
*
*
* -
*
* WARN
Allows provisioning to continue with a warning message.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #hookFailureMode}
* will return {@link HookFailureMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #hookFailureModeAsString}.
*
*
* @return Specify the hook failure mode for non-compliant resources in the followings ways.
*
* -
*
* FAIL
Stops provisioning resources.
*
*
* -
*
* WARN
Allows provisioning to continue with a warning message.
*
*
* @see HookFailureMode
*/
public final String hookFailureModeAsString() {
return hookFailureMode;
}
/**
*
* An optional field containing information about the detailed status of the stack event.
*
*
* -
*
* CONFIGURATION_COMPLETE
- all of the resources in the stack have reached that event. For more
* information, see CloudFormation stack deployment in the CloudFormation User Guide.
*
*
*
*
* -
*
* VALIDATION_FAILED
- template validation failed because of invalid properties in the template. The
* ResourceStatusReason
field shows what properties are defined incorrectly.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #detailedStatus}
* will return {@link DetailedStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #detailedStatusAsString}.
*
*
* @return An optional field containing information about the detailed status of the stack event.
*
* -
*
* CONFIGURATION_COMPLETE
- all of the resources in the stack have reached that event. For more
* information, see CloudFormation stack deployment in the CloudFormation User Guide.
*
*
*
*
* -
*
* VALIDATION_FAILED
- template validation failed because of invalid properties in the
* template. The ResourceStatusReason
field shows what properties are defined incorrectly.
*
*
* @see DetailedStatus
*/
public final DetailedStatus detailedStatus() {
return DetailedStatus.fromValue(detailedStatus);
}
/**
*
* An optional field containing information about the detailed status of the stack event.
*
*
* -
*
* CONFIGURATION_COMPLETE
- all of the resources in the stack have reached that event. For more
* information, see CloudFormation stack deployment in the CloudFormation User Guide.
*
*
*
*
* -
*
* VALIDATION_FAILED
- template validation failed because of invalid properties in the template. The
* ResourceStatusReason
field shows what properties are defined incorrectly.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #detailedStatus}
* will return {@link DetailedStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #detailedStatusAsString}.
*
*
* @return An optional field containing information about the detailed status of the stack event.
*
* -
*
* CONFIGURATION_COMPLETE
- all of the resources in the stack have reached that event. For more
* information, see CloudFormation stack deployment in the CloudFormation User Guide.
*
*
*
*
* -
*
* VALIDATION_FAILED
- template validation failed because of invalid properties in the
* template. The ResourceStatusReason
field shows what properties are defined incorrectly.
*
*
* @see DetailedStatus
*/
public final String detailedStatusAsString() {
return detailedStatus;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(stackId());
hashCode = 31 * hashCode + Objects.hashCode(eventId());
hashCode = 31 * hashCode + Objects.hashCode(stackName());
hashCode = 31 * hashCode + Objects.hashCode(logicalResourceId());
hashCode = 31 * hashCode + Objects.hashCode(physicalResourceId());
hashCode = 31 * hashCode + Objects.hashCode(resourceType());
hashCode = 31 * hashCode + Objects.hashCode(timestamp());
hashCode = 31 * hashCode + Objects.hashCode(resourceStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(resourceStatusReason());
hashCode = 31 * hashCode + Objects.hashCode(resourceProperties());
hashCode = 31 * hashCode + Objects.hashCode(clientRequestToken());
hashCode = 31 * hashCode + Objects.hashCode(hookType());
hashCode = 31 * hashCode + Objects.hashCode(hookStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hookStatusReason());
hashCode = 31 * hashCode + Objects.hashCode(hookInvocationPointAsString());
hashCode = 31 * hashCode + Objects.hashCode(hookFailureModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(detailedStatusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StackEvent)) {
return false;
}
StackEvent other = (StackEvent) obj;
return Objects.equals(stackId(), other.stackId()) && Objects.equals(eventId(), other.eventId())
&& Objects.equals(stackName(), other.stackName())
&& Objects.equals(logicalResourceId(), other.logicalResourceId())
&& Objects.equals(physicalResourceId(), other.physicalResourceId())
&& Objects.equals(resourceType(), other.resourceType()) && Objects.equals(timestamp(), other.timestamp())
&& Objects.equals(resourceStatusAsString(), other.resourceStatusAsString())
&& Objects.equals(resourceStatusReason(), other.resourceStatusReason())
&& Objects.equals(resourceProperties(), other.resourceProperties())
&& Objects.equals(clientRequestToken(), other.clientRequestToken())
&& Objects.equals(hookType(), other.hookType())
&& Objects.equals(hookStatusAsString(), other.hookStatusAsString())
&& Objects.equals(hookStatusReason(), other.hookStatusReason())
&& Objects.equals(hookInvocationPointAsString(), other.hookInvocationPointAsString())
&& Objects.equals(hookFailureModeAsString(), other.hookFailureModeAsString())
&& Objects.equals(detailedStatusAsString(), other.detailedStatusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StackEvent").add("StackId", stackId()).add("EventId", eventId()).add("StackName", stackName())
.add("LogicalResourceId", logicalResourceId()).add("PhysicalResourceId", physicalResourceId())
.add("ResourceType", resourceType()).add("Timestamp", timestamp())
.add("ResourceStatus", resourceStatusAsString()).add("ResourceStatusReason", resourceStatusReason())
.add("ResourceProperties", resourceProperties()).add("ClientRequestToken", clientRequestToken())
.add("HookType", hookType()).add("HookStatus", hookStatusAsString()).add("HookStatusReason", hookStatusReason())
.add("HookInvocationPoint", hookInvocationPointAsString()).add("HookFailureMode", hookFailureModeAsString())
.add("DetailedStatus", detailedStatusAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackId":
return Optional.ofNullable(clazz.cast(stackId()));
case "EventId":
return Optional.ofNullable(clazz.cast(eventId()));
case "StackName":
return Optional.ofNullable(clazz.cast(stackName()));
case "LogicalResourceId":
return Optional.ofNullable(clazz.cast(logicalResourceId()));
case "PhysicalResourceId":
return Optional.ofNullable(clazz.cast(physicalResourceId()));
case "ResourceType":
return Optional.ofNullable(clazz.cast(resourceType()));
case "Timestamp":
return Optional.ofNullable(clazz.cast(timestamp()));
case "ResourceStatus":
return Optional.ofNullable(clazz.cast(resourceStatusAsString()));
case "ResourceStatusReason":
return Optional.ofNullable(clazz.cast(resourceStatusReason()));
case "ResourceProperties":
return Optional.ofNullable(clazz.cast(resourceProperties()));
case "ClientRequestToken":
return Optional.ofNullable(clazz.cast(clientRequestToken()));
case "HookType":
return Optional.ofNullable(clazz.cast(hookType()));
case "HookStatus":
return Optional.ofNullable(clazz.cast(hookStatusAsString()));
case "HookStatusReason":
return Optional.ofNullable(clazz.cast(hookStatusReason()));
case "HookInvocationPoint":
return Optional.ofNullable(clazz.cast(hookInvocationPointAsString()));
case "HookFailureMode":
return Optional.ofNullable(clazz.cast(hookFailureModeAsString()));
case "DetailedStatus":
return Optional.ofNullable(clazz.cast(detailedStatusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function