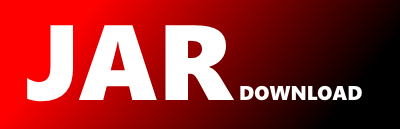
software.amazon.awssdk.services.cloudformation.model.CreateStackSetRequest Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateStackSetRequest extends CloudFormationRequest implements
ToCopyableBuilder {
private static final SdkField STACK_SET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackSetName").getter(getter(CreateStackSetRequest::stackSetName)).setter(setter(Builder::stackSetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackSetName").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CreateStackSetRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField TEMPLATE_BODY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateBody").getter(getter(CreateStackSetRequest::templateBody)).setter(setter(Builder::templateBody))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateBody").build()).build();
private static final SdkField TEMPLATE_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateURL").getter(getter(CreateStackSetRequest::templateURL)).setter(setter(Builder::templateURL))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateURL").build()).build();
private static final SdkField STACK_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackId").getter(getter(CreateStackSetRequest::stackId)).setter(setter(Builder::stackId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackId").build()).build();
private static final SdkField> PARAMETERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Parameters")
.getter(getter(CreateStackSetRequest::parameters))
.setter(setter(Builder::parameters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Parameters").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Parameter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CAPABILITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Capabilities")
.getter(getter(CreateStackSetRequest::capabilitiesAsStrings))
.setter(setter(Builder::capabilitiesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Capabilities").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateStackSetRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ADMINISTRATION_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AdministrationRoleARN").getter(getter(CreateStackSetRequest::administrationRoleARN))
.setter(setter(Builder::administrationRoleARN))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdministrationRoleARN").build())
.build();
private static final SdkField EXECUTION_ROLE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExecutionRoleName").getter(getter(CreateStackSetRequest::executionRoleName))
.setter(setter(Builder::executionRoleName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExecutionRoleName").build()).build();
private static final SdkField PERMISSION_MODEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PermissionModel").getter(getter(CreateStackSetRequest::permissionModelAsString))
.setter(setter(Builder::permissionModel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PermissionModel").build()).build();
private static final SdkField AUTO_DEPLOYMENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AutoDeployment")
.getter(getter(CreateStackSetRequest::autoDeployment)).setter(setter(Builder::autoDeployment))
.constructor(AutoDeployment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoDeployment").build()).build();
private static final SdkField CALL_AS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("CallAs")
.getter(getter(CreateStackSetRequest::callAsAsString)).setter(setter(Builder::callAs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CallAs").build()).build();
private static final SdkField CLIENT_REQUEST_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ClientRequestToken")
.getter(getter(CreateStackSetRequest::clientRequestToken))
.setter(setter(Builder::clientRequestToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientRequestToken").build(),
DefaultValueTrait.idempotencyToken()).build();
private static final SdkField MANAGED_EXECUTION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ManagedExecution")
.getter(getter(CreateStackSetRequest::managedExecution)).setter(setter(Builder::managedExecution))
.constructor(ManagedExecution::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ManagedExecution").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STACK_SET_NAME_FIELD,
DESCRIPTION_FIELD, TEMPLATE_BODY_FIELD, TEMPLATE_URL_FIELD, STACK_ID_FIELD, PARAMETERS_FIELD, CAPABILITIES_FIELD,
TAGS_FIELD, ADMINISTRATION_ROLE_ARN_FIELD, EXECUTION_ROLE_NAME_FIELD, PERMISSION_MODEL_FIELD, AUTO_DEPLOYMENT_FIELD,
CALL_AS_FIELD, CLIENT_REQUEST_TOKEN_FIELD, MANAGED_EXECUTION_FIELD));
private final String stackSetName;
private final String description;
private final String templateBody;
private final String templateURL;
private final String stackId;
private final List parameters;
private final List capabilities;
private final List tags;
private final String administrationRoleARN;
private final String executionRoleName;
private final String permissionModel;
private final AutoDeployment autoDeployment;
private final String callAs;
private final String clientRequestToken;
private final ManagedExecution managedExecution;
private CreateStackSetRequest(BuilderImpl builder) {
super(builder);
this.stackSetName = builder.stackSetName;
this.description = builder.description;
this.templateBody = builder.templateBody;
this.templateURL = builder.templateURL;
this.stackId = builder.stackId;
this.parameters = builder.parameters;
this.capabilities = builder.capabilities;
this.tags = builder.tags;
this.administrationRoleARN = builder.administrationRoleARN;
this.executionRoleName = builder.executionRoleName;
this.permissionModel = builder.permissionModel;
this.autoDeployment = builder.autoDeployment;
this.callAs = builder.callAs;
this.clientRequestToken = builder.clientRequestToken;
this.managedExecution = builder.managedExecution;
}
/**
*
* The name to associate with the stack set. The name must be unique in the Region where you create your stack set.
*
*
*
* A stack name can contain only alphanumeric characters (case-sensitive) and hyphens. It must start with an
* alphabetic character and can't be longer than 128 characters.
*
*
*
* @return The name to associate with the stack set. The name must be unique in the Region where you create your
* stack set.
*
* A stack name can contain only alphanumeric characters (case-sensitive) and hyphens. It must start with an
* alphabetic character and can't be longer than 128 characters.
*
*/
public final String stackSetName() {
return stackSetName;
}
/**
*
* A description of the stack set. You can use the description to identify the stack set's purpose or other
* important information.
*
*
* @return A description of the stack set. You can use the description to identify the stack set's purpose or other
* important information.
*/
public final String description() {
return description;
}
/**
*
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of 51,200
* bytes. For more information, see Template Anatomy
* in the CloudFormation User Guide.
*
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*
*
* @return The structure that contains the template body, with a minimum length of 1 byte and a maximum length of
* 51,200 bytes. For more information, see Template
* Anatomy in the CloudFormation User Guide.
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*/
public final String templateBody() {
return templateBody;
}
/**
*
* The location of the file that contains the template body. The URL must point to a template (maximum size: 460,800
* bytes) that's located in an Amazon S3 bucket or a Systems Manager document. For more information, see Template Anatomy
* in the CloudFormation User Guide.
*
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*
*
* @return The location of the file that contains the template body. The URL must point to a template (maximum size:
* 460,800 bytes) that's located in an Amazon S3 bucket or a Systems Manager document. For more information,
* see Template
* Anatomy in the CloudFormation User Guide.
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*/
public final String templateURL() {
return templateURL;
}
/**
*
* The stack ID you are importing into a new stack set. Specify the Amazon Resource Name (ARN) of the stack.
*
*
* @return The stack ID you are importing into a new stack set. Specify the Amazon Resource Name (ARN) of the stack.
*/
public final String stackId() {
return stackId;
}
/**
* For responses, this returns true if the service returned a value for the Parameters property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasParameters() {
return parameters != null && !(parameters instanceof SdkAutoConstructList);
}
/**
*
* The input parameters for the stack set template.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasParameters} method.
*
*
* @return The input parameters for the stack set template.
*/
public final List parameters() {
return parameters;
}
/**
*
* In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities in
* order for CloudFormation to create the stack set and related stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account; for
* example, by creating new Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
* AWS::IAM::Role
*
*
*
* -
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates reference macros. If your stack set template references one or more macros, you must create the
* stack set directly from the processed template, without first reviewing the resulting changes in a change set. To
* create the stack set directly, you must acknowledge this capability. For more information, see Using CloudFormation
* Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets with service-managed permissions don't currently support the use of macros in templates. (This
* includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.) Even if you specify this capability
* for a stack set with service-managed permissions, if you reference a macro in your template the stack set
* operation will fail.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapabilities} method.
*
*
* @return In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities
* in order for CloudFormation to create the stack set and related stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stack sets,
* you must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates reference macros. If your stack set template references one or more macros, you must
* create the stack set directly from the processed template, without first reviewing the resulting changes
* in a change set. To create the stack set directly, you must acknowledge this capability. For more
* information, see Using
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets with service-managed permissions don't currently support the use of macros in templates. (This
* includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.) Even if you specify this
* capability for a stack set with service-managed permissions, if you reference a macro in your template
* the stack set operation will fail.
*
*
*/
public final List capabilities() {
return CapabilitiesCopier.copyStringToEnum(capabilities);
}
/**
* For responses, this returns true if the service returned a value for the Capabilities property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCapabilities() {
return capabilities != null && !(capabilities instanceof SdkAutoConstructList);
}
/**
*
* In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities in
* order for CloudFormation to create the stack set and related stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services account; for
* example, by creating new Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
* AWS::IAM::Role
*
*
*
* -
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates reference macros. If your stack set template references one or more macros, you must create the
* stack set directly from the processed template, without first reviewing the resulting changes in a change set. To
* create the stack set directly, you must acknowledge this capability. For more information, see Using CloudFormation
* Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets with service-managed permissions don't currently support the use of macros in templates. (This
* includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.) Even if you specify this capability
* for a stack set with service-managed permissions, if you reference a macro in your template the stack set
* operation will fail.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapabilities} method.
*
*
* @return In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities
* in order for CloudFormation to create the stack set and related stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your Amazon Web Services
* account; for example, by creating new Identity and Access Management (IAM) users. For those stack sets,
* you must explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates reference macros. If your stack set template references one or more macros, you must
* create the stack set directly from the processed template, without first reviewing the resulting changes
* in a change set. To create the stack set directly, you must acknowledge this capability. For more
* information, see Using
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets with service-managed permissions don't currently support the use of macros in templates. (This
* includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by CloudFormation.) Even if you specify this
* capability for a stack set with service-managed permissions, if you reference a macro in your template
* the stack set operation will fail.
*
*
*/
public final List capabilitiesAsStrings() {
return capabilities;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. CloudFormation also
* propagates these tags to supported resources that are created in the stacks. A maximum number of 50 tags can be
* specified.
*
*
* If you specify tags as part of a CreateStackSet
action, CloudFormation checks to see if you have the
* required IAM permission to tag resources. If you don't, the entire CreateStackSet
action fails with
* an access denied
error, and the stack set is not created.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The key-value pairs to associate with this stack set and the stacks created from it. CloudFormation also
* propagates these tags to supported resources that are created in the stacks. A maximum number of 50 tags
* can be specified.
*
* If you specify tags as part of a CreateStackSet
action, CloudFormation checks to see if you
* have the required IAM permission to tag resources. If you don't, the entire CreateStackSet
* action fails with an access denied
error, and the stack set is not created.
*/
public final List tags() {
return tags;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role to use to create this stack set.
*
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups can
* manage specific stack sets within the same administrator account. For more information, see Prerequisites:
* Granting Permissions for Stack Set Operations in the CloudFormation User Guide.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role to use to create this stack set.
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups
* can manage specific stack sets within the same administrator account. For more information, see Prerequisites: Granting Permissions for Stack Set Operations in the CloudFormation User
* Guide.
*/
public final String administrationRoleARN() {
return administrationRoleARN;
}
/**
*
* The name of the IAM execution role to use to create the stack set. If you do not specify an execution role,
* CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack set operation.
*
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources users and
* groups can include in their stack sets.
*
*
* @return The name of the IAM execution role to use to create the stack set. If you do not specify an execution
* role, CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack set
* operation.
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources
* users and groups can include in their stack sets.
*/
public final String executionRoleName() {
return executionRoleName;
}
/**
*
* Describes how the IAM roles required for stack set operations are created. By default, SELF-MANAGED
* is specified.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #permissionModel}
* will return {@link PermissionModels#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #permissionModelAsString}.
*
*
* @return Describes how the IAM roles required for stack set operations are created. By default,
* SELF-MANAGED
is specified.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles
* required to deploy to target accounts. For more information, see Grant Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
*/
public final PermissionModels permissionModel() {
return PermissionModels.fromValue(permissionModel);
}
/**
*
* Describes how the IAM roles required for stack set operations are created. By default, SELF-MANAGED
* is specified.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #permissionModel}
* will return {@link PermissionModels#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #permissionModelAsString}.
*
*
* @return Describes how the IAM roles required for stack set operations are created. By default,
* SELF-MANAGED
is specified.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles
* required to deploy to target accounts. For more information, see Grant Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
*/
public final String permissionModelAsString() {
return permissionModel;
}
/**
*
* Describes whether StackSets automatically deploys to Organizations accounts that are added to the target
* organization or organizational unit (OU). Specify only if PermissionModel
is
* SERVICE_MANAGED
.
*
*
* @return Describes whether StackSets automatically deploys to Organizations accounts that are added to the target
* organization or organizational unit (OU). Specify only if PermissionModel
is
* SERVICE_MANAGED
.
*/
public final AutoDeployment autoDeployment() {
return autoDeployment;
}
/**
*
* [Service-managed permissions] Specifies whether you are acting as an account administrator in the organization's
* management account or as a delegated administrator in a member account.
*
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed permissions.
*
*
* -
*
* To create a stack set with service-managed permissions while signed in to the management account, specify
* SELF
.
*
*
* -
*
* To create a stack set with service-managed permissions while signed in to a delegated administrator account,
* specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated admin in the management account. For more
* information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
*
*
* Stack sets with service-managed permissions are created in the management account, including stack sets that are
* created by delegated administrators.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #callAs} will
* return {@link CallAs#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #callAsAsString}.
*
*
* @return [Service-managed permissions] Specifies whether you are acting as an account administrator in the
* organization's management account or as a delegated administrator in a member account.
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed
* permissions.
*
*
* -
*
* To create a stack set with service-managed permissions while signed in to the management account, specify
* SELF
.
*
*
* -
*
* To create a stack set with service-managed permissions while signed in to a delegated administrator
* account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated admin in the management account. For
* more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
*
*
* Stack sets with service-managed permissions are created in the management account, including stack sets
* that are created by delegated administrators.
* @see CallAs
*/
public final CallAs callAs() {
return CallAs.fromValue(callAs);
}
/**
*
* [Service-managed permissions] Specifies whether you are acting as an account administrator in the organization's
* management account or as a delegated administrator in a member account.
*
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed permissions.
*
*
* -
*
* To create a stack set with service-managed permissions while signed in to the management account, specify
* SELF
.
*
*
* -
*
* To create a stack set with service-managed permissions while signed in to a delegated administrator account,
* specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated admin in the management account. For more
* information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
*
*
* Stack sets with service-managed permissions are created in the management account, including stack sets that are
* created by delegated administrators.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #callAs} will
* return {@link CallAs#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #callAsAsString}.
*
*
* @return [Service-managed permissions] Specifies whether you are acting as an account administrator in the
* organization's management account or as a delegated administrator in a member account.
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed
* permissions.
*
*
* -
*
* To create a stack set with service-managed permissions while signed in to the management account, specify
* SELF
.
*
*
* -
*
* To create a stack set with service-managed permissions while signed in to a delegated administrator
* account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated admin in the management account. For
* more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
*
*
* Stack sets with service-managed permissions are created in the management account, including stack sets
* that are created by delegated administrators.
* @see CallAs
*/
public final String callAsAsString() {
return callAs;
}
/**
*
* A unique identifier for this CreateStackSet
request. Specify this token if you plan to retry
* requests so that CloudFormation knows that you're not attempting to create another stack set with the same name.
* You might retry CreateStackSet
requests to ensure that CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* @return A unique identifier for this CreateStackSet
request. Specify this token if you plan to retry
* requests so that CloudFormation knows that you're not attempting to create another stack set with the
* same name. You might retry CreateStackSet
requests to ensure that CloudFormation
* successfully received them.
*
* If you don't specify an operation ID, the SDK generates one automatically.
*/
public final String clientRequestToken() {
return clientRequestToken;
}
/**
*
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting operations.
*
*
* @return Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting
* operations.
*/
public final ManagedExecution managedExecution() {
return managedExecution;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(stackSetName());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(templateBody());
hashCode = 31 * hashCode + Objects.hashCode(templateURL());
hashCode = 31 * hashCode + Objects.hashCode(stackId());
hashCode = 31 * hashCode + Objects.hashCode(hasParameters() ? parameters() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCapabilities() ? capabilitiesAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(administrationRoleARN());
hashCode = 31 * hashCode + Objects.hashCode(executionRoleName());
hashCode = 31 * hashCode + Objects.hashCode(permissionModelAsString());
hashCode = 31 * hashCode + Objects.hashCode(autoDeployment());
hashCode = 31 * hashCode + Objects.hashCode(callAsAsString());
hashCode = 31 * hashCode + Objects.hashCode(clientRequestToken());
hashCode = 31 * hashCode + Objects.hashCode(managedExecution());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateStackSetRequest)) {
return false;
}
CreateStackSetRequest other = (CreateStackSetRequest) obj;
return Objects.equals(stackSetName(), other.stackSetName()) && Objects.equals(description(), other.description())
&& Objects.equals(templateBody(), other.templateBody()) && Objects.equals(templateURL(), other.templateURL())
&& Objects.equals(stackId(), other.stackId()) && hasParameters() == other.hasParameters()
&& Objects.equals(parameters(), other.parameters()) && hasCapabilities() == other.hasCapabilities()
&& Objects.equals(capabilitiesAsStrings(), other.capabilitiesAsStrings()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(administrationRoleARN(), other.administrationRoleARN())
&& Objects.equals(executionRoleName(), other.executionRoleName())
&& Objects.equals(permissionModelAsString(), other.permissionModelAsString())
&& Objects.equals(autoDeployment(), other.autoDeployment())
&& Objects.equals(callAsAsString(), other.callAsAsString())
&& Objects.equals(clientRequestToken(), other.clientRequestToken())
&& Objects.equals(managedExecution(), other.managedExecution());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateStackSetRequest").add("StackSetName", stackSetName()).add("Description", description())
.add("TemplateBody", templateBody()).add("TemplateURL", templateURL()).add("StackId", stackId())
.add("Parameters", hasParameters() ? parameters() : null)
.add("Capabilities", hasCapabilities() ? capabilitiesAsStrings() : null).add("Tags", hasTags() ? tags() : null)
.add("AdministrationRoleARN", administrationRoleARN()).add("ExecutionRoleName", executionRoleName())
.add("PermissionModel", permissionModelAsString()).add("AutoDeployment", autoDeployment())
.add("CallAs", callAsAsString()).add("ClientRequestToken", clientRequestToken())
.add("ManagedExecution", managedExecution()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackSetName":
return Optional.ofNullable(clazz.cast(stackSetName()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "TemplateBody":
return Optional.ofNullable(clazz.cast(templateBody()));
case "TemplateURL":
return Optional.ofNullable(clazz.cast(templateURL()));
case "StackId":
return Optional.ofNullable(clazz.cast(stackId()));
case "Parameters":
return Optional.ofNullable(clazz.cast(parameters()));
case "Capabilities":
return Optional.ofNullable(clazz.cast(capabilitiesAsStrings()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "AdministrationRoleARN":
return Optional.ofNullable(clazz.cast(administrationRoleARN()));
case "ExecutionRoleName":
return Optional.ofNullable(clazz.cast(executionRoleName()));
case "PermissionModel":
return Optional.ofNullable(clazz.cast(permissionModelAsString()));
case "AutoDeployment":
return Optional.ofNullable(clazz.cast(autoDeployment()));
case "CallAs":
return Optional.ofNullable(clazz.cast(callAsAsString()));
case "ClientRequestToken":
return Optional.ofNullable(clazz.cast(clientRequestToken()));
case "ManagedExecution":
return Optional.ofNullable(clazz.cast(managedExecution()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function