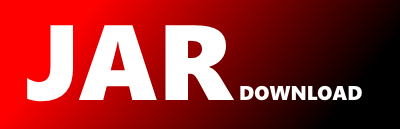
software.amazon.awssdk.services.cloudformation.model.ChangeSetHook Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Specifies the resource, the hook, and the hook version to be invoked.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ChangeSetHook implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField INVOCATION_POINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InvocationPoint").getter(getter(ChangeSetHook::invocationPointAsString))
.setter(setter(Builder::invocationPoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InvocationPoint").build()).build();
private static final SdkField FAILURE_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureMode").getter(getter(ChangeSetHook::failureModeAsString)).setter(setter(Builder::failureMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureMode").build()).build();
private static final SdkField TYPE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TypeName").getter(getter(ChangeSetHook::typeName)).setter(setter(Builder::typeName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TypeName").build()).build();
private static final SdkField TYPE_VERSION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TypeVersionId").getter(getter(ChangeSetHook::typeVersionId)).setter(setter(Builder::typeVersionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TypeVersionId").build()).build();
private static final SdkField TYPE_CONFIGURATION_VERSION_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("TypeConfigurationVersionId")
.getter(getter(ChangeSetHook::typeConfigurationVersionId))
.setter(setter(Builder::typeConfigurationVersionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TypeConfigurationVersionId").build())
.build();
private static final SdkField TARGET_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("TargetDetails")
.getter(getter(ChangeSetHook::targetDetails)).setter(setter(Builder::targetDetails))
.constructor(ChangeSetHookTargetDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetDetails").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays
.asList(INVOCATION_POINT_FIELD, FAILURE_MODE_FIELD, TYPE_NAME_FIELD, TYPE_VERSION_ID_FIELD,
TYPE_CONFIGURATION_VERSION_ID_FIELD, TARGET_DETAILS_FIELD));
private static final long serialVersionUID = 1L;
private final String invocationPoint;
private final String failureMode;
private final String typeName;
private final String typeVersionId;
private final String typeConfigurationVersionId;
private final ChangeSetHookTargetDetails targetDetails;
private ChangeSetHook(BuilderImpl builder) {
this.invocationPoint = builder.invocationPoint;
this.failureMode = builder.failureMode;
this.typeName = builder.typeName;
this.typeVersionId = builder.typeVersionId;
this.typeConfigurationVersionId = builder.typeConfigurationVersionId;
this.targetDetails = builder.targetDetails;
}
/**
*
* Specifies the points in provisioning logic where a hook is invoked.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #invocationPoint}
* will return {@link HookInvocationPoint#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #invocationPointAsString}.
*
*
* @return Specifies the points in provisioning logic where a hook is invoked.
* @see HookInvocationPoint
*/
public final HookInvocationPoint invocationPoint() {
return HookInvocationPoint.fromValue(invocationPoint);
}
/**
*
* Specifies the points in provisioning logic where a hook is invoked.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #invocationPoint}
* will return {@link HookInvocationPoint#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #invocationPointAsString}.
*
*
* @return Specifies the points in provisioning logic where a hook is invoked.
* @see HookInvocationPoint
*/
public final String invocationPointAsString() {
return invocationPoint;
}
/**
*
* Specify the hook failure mode for non-compliant resources in the followings ways.
*
*
* -
*
* FAIL
Stops provisioning resources.
*
*
* -
*
* WARN
Allows provisioning to continue with a warning message.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #failureMode} will
* return {@link HookFailureMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #failureModeAsString}.
*
*
* @return Specify the hook failure mode for non-compliant resources in the followings ways.
*
* -
*
* FAIL
Stops provisioning resources.
*
*
* -
*
* WARN
Allows provisioning to continue with a warning message.
*
*
* @see HookFailureMode
*/
public final HookFailureMode failureMode() {
return HookFailureMode.fromValue(failureMode);
}
/**
*
* Specify the hook failure mode for non-compliant resources in the followings ways.
*
*
* -
*
* FAIL
Stops provisioning resources.
*
*
* -
*
* WARN
Allows provisioning to continue with a warning message.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #failureMode} will
* return {@link HookFailureMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #failureModeAsString}.
*
*
* @return Specify the hook failure mode for non-compliant resources in the followings ways.
*
* -
*
* FAIL
Stops provisioning resources.
*
*
* -
*
* WARN
Allows provisioning to continue with a warning message.
*
*
* @see HookFailureMode
*/
public final String failureModeAsString() {
return failureMode;
}
/**
*
* The unique name for your hook. Specifies a three-part namespace for your hook, with a recommended pattern of
* Organization::Service::Hook
.
*
*
*
* The following organization namespaces are reserved and can't be used in your hook type names:
*
*
* -
*
* Alexa
*
*
* -
*
* AMZN
*
*
* -
*
* Amazon
*
*
* -
*
* ASK
*
*
* -
*
* AWS
*
*
* -
*
* Custom
*
*
* -
*
* Dev
*
*
*
*
*
* @return The unique name for your hook. Specifies a three-part namespace for your hook, with a recommended pattern
* of Organization::Service::Hook
.
*
* The following organization namespaces are reserved and can't be used in your hook type names:
*
*
* -
*
* Alexa
*
*
* -
*
* AMZN
*
*
* -
*
* Amazon
*
*
* -
*
* ASK
*
*
* -
*
* AWS
*
*
* -
*
* Custom
*
*
* -
*
* Dev
*
*
*
*/
public final String typeName() {
return typeName;
}
/**
*
* The version ID of the type specified.
*
*
* @return The version ID of the type specified.
*/
public final String typeVersionId() {
return typeVersionId;
}
/**
*
* The version ID of the type configuration.
*
*
* @return The version ID of the type configuration.
*/
public final String typeConfigurationVersionId() {
return typeConfigurationVersionId;
}
/**
*
* Specifies details about the target that the hook will run against.
*
*
* @return Specifies details about the target that the hook will run against.
*/
public final ChangeSetHookTargetDetails targetDetails() {
return targetDetails;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(invocationPointAsString());
hashCode = 31 * hashCode + Objects.hashCode(failureModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(typeName());
hashCode = 31 * hashCode + Objects.hashCode(typeVersionId());
hashCode = 31 * hashCode + Objects.hashCode(typeConfigurationVersionId());
hashCode = 31 * hashCode + Objects.hashCode(targetDetails());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ChangeSetHook)) {
return false;
}
ChangeSetHook other = (ChangeSetHook) obj;
return Objects.equals(invocationPointAsString(), other.invocationPointAsString())
&& Objects.equals(failureModeAsString(), other.failureModeAsString())
&& Objects.equals(typeName(), other.typeName()) && Objects.equals(typeVersionId(), other.typeVersionId())
&& Objects.equals(typeConfigurationVersionId(), other.typeConfigurationVersionId())
&& Objects.equals(targetDetails(), other.targetDetails());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ChangeSetHook").add("InvocationPoint", invocationPointAsString())
.add("FailureMode", failureModeAsString()).add("TypeName", typeName()).add("TypeVersionId", typeVersionId())
.add("TypeConfigurationVersionId", typeConfigurationVersionId()).add("TargetDetails", targetDetails()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "InvocationPoint":
return Optional.ofNullable(clazz.cast(invocationPointAsString()));
case "FailureMode":
return Optional.ofNullable(clazz.cast(failureModeAsString()));
case "TypeName":
return Optional.ofNullable(clazz.cast(typeName()));
case "TypeVersionId":
return Optional.ofNullable(clazz.cast(typeVersionId()));
case "TypeConfigurationVersionId":
return Optional.ofNullable(clazz.cast(typeConfigurationVersionId()));
case "TargetDetails":
return Optional.ofNullable(clazz.cast(targetDetails()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function