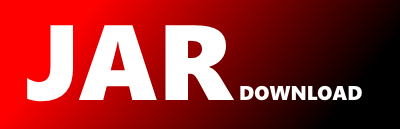
software.amazon.awssdk.services.cloudformation.DefaultCloudFormationAsyncClient Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.query.AwsQueryProtocolFactory;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.cloudformation.internal.CloudFormationServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.cloudformation.model.ActivateOrganizationsAccessRequest;
import software.amazon.awssdk.services.cloudformation.model.ActivateOrganizationsAccessResponse;
import software.amazon.awssdk.services.cloudformation.model.ActivateTypeRequest;
import software.amazon.awssdk.services.cloudformation.model.ActivateTypeResponse;
import software.amazon.awssdk.services.cloudformation.model.AlreadyExistsException;
import software.amazon.awssdk.services.cloudformation.model.BatchDescribeTypeConfigurationsRequest;
import software.amazon.awssdk.services.cloudformation.model.BatchDescribeTypeConfigurationsResponse;
import software.amazon.awssdk.services.cloudformation.model.CancelUpdateStackRequest;
import software.amazon.awssdk.services.cloudformation.model.CancelUpdateStackResponse;
import software.amazon.awssdk.services.cloudformation.model.CfnRegistryException;
import software.amazon.awssdk.services.cloudformation.model.ChangeSetNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.CloudFormationException;
import software.amazon.awssdk.services.cloudformation.model.ConcurrentResourcesLimitExceededException;
import software.amazon.awssdk.services.cloudformation.model.ContinueUpdateRollbackRequest;
import software.amazon.awssdk.services.cloudformation.model.ContinueUpdateRollbackResponse;
import software.amazon.awssdk.services.cloudformation.model.CreateChangeSetRequest;
import software.amazon.awssdk.services.cloudformation.model.CreateChangeSetResponse;
import software.amazon.awssdk.services.cloudformation.model.CreateGeneratedTemplateRequest;
import software.amazon.awssdk.services.cloudformation.model.CreateGeneratedTemplateResponse;
import software.amazon.awssdk.services.cloudformation.model.CreateStackInstancesRequest;
import software.amazon.awssdk.services.cloudformation.model.CreateStackInstancesResponse;
import software.amazon.awssdk.services.cloudformation.model.CreateStackRequest;
import software.amazon.awssdk.services.cloudformation.model.CreateStackResponse;
import software.amazon.awssdk.services.cloudformation.model.CreateStackSetRequest;
import software.amazon.awssdk.services.cloudformation.model.CreateStackSetResponse;
import software.amazon.awssdk.services.cloudformation.model.CreatedButModifiedException;
import software.amazon.awssdk.services.cloudformation.model.DeactivateOrganizationsAccessRequest;
import software.amazon.awssdk.services.cloudformation.model.DeactivateOrganizationsAccessResponse;
import software.amazon.awssdk.services.cloudformation.model.DeactivateTypeRequest;
import software.amazon.awssdk.services.cloudformation.model.DeactivateTypeResponse;
import software.amazon.awssdk.services.cloudformation.model.DeleteChangeSetRequest;
import software.amazon.awssdk.services.cloudformation.model.DeleteChangeSetResponse;
import software.amazon.awssdk.services.cloudformation.model.DeleteGeneratedTemplateRequest;
import software.amazon.awssdk.services.cloudformation.model.DeleteGeneratedTemplateResponse;
import software.amazon.awssdk.services.cloudformation.model.DeleteStackInstancesRequest;
import software.amazon.awssdk.services.cloudformation.model.DeleteStackInstancesResponse;
import software.amazon.awssdk.services.cloudformation.model.DeleteStackRequest;
import software.amazon.awssdk.services.cloudformation.model.DeleteStackResponse;
import software.amazon.awssdk.services.cloudformation.model.DeleteStackSetRequest;
import software.amazon.awssdk.services.cloudformation.model.DeleteStackSetResponse;
import software.amazon.awssdk.services.cloudformation.model.DeregisterTypeRequest;
import software.amazon.awssdk.services.cloudformation.model.DeregisterTypeResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeAccountLimitsResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeChangeSetHooksRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeChangeSetHooksResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeChangeSetRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeChangeSetResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeGeneratedTemplateRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeGeneratedTemplateResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeOrganizationsAccessRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeOrganizationsAccessResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribePublisherRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribePublisherResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeResourceScanRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeResourceScanResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackDriftDetectionStatusRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackDriftDetectionStatusResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackEventsRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackEventsResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackInstanceRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackInstanceResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceDriftsRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceDriftsResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackResourceResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackResourcesRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackResourcesResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackSetOperationRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackSetOperationResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackSetRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStackSetResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeStacksRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeStacksResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeTypeRegistrationRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeTypeRegistrationResponse;
import software.amazon.awssdk.services.cloudformation.model.DescribeTypeRequest;
import software.amazon.awssdk.services.cloudformation.model.DescribeTypeResponse;
import software.amazon.awssdk.services.cloudformation.model.DetectStackDriftRequest;
import software.amazon.awssdk.services.cloudformation.model.DetectStackDriftResponse;
import software.amazon.awssdk.services.cloudformation.model.DetectStackResourceDriftRequest;
import software.amazon.awssdk.services.cloudformation.model.DetectStackResourceDriftResponse;
import software.amazon.awssdk.services.cloudformation.model.DetectStackSetDriftRequest;
import software.amazon.awssdk.services.cloudformation.model.DetectStackSetDriftResponse;
import software.amazon.awssdk.services.cloudformation.model.EstimateTemplateCostRequest;
import software.amazon.awssdk.services.cloudformation.model.EstimateTemplateCostResponse;
import software.amazon.awssdk.services.cloudformation.model.ExecuteChangeSetRequest;
import software.amazon.awssdk.services.cloudformation.model.ExecuteChangeSetResponse;
import software.amazon.awssdk.services.cloudformation.model.GeneratedTemplateNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.GetGeneratedTemplateRequest;
import software.amazon.awssdk.services.cloudformation.model.GetGeneratedTemplateResponse;
import software.amazon.awssdk.services.cloudformation.model.GetStackPolicyRequest;
import software.amazon.awssdk.services.cloudformation.model.GetStackPolicyResponse;
import software.amazon.awssdk.services.cloudformation.model.GetTemplateRequest;
import software.amazon.awssdk.services.cloudformation.model.GetTemplateResponse;
import software.amazon.awssdk.services.cloudformation.model.GetTemplateSummaryRequest;
import software.amazon.awssdk.services.cloudformation.model.GetTemplateSummaryResponse;
import software.amazon.awssdk.services.cloudformation.model.HookResultNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.ImportStacksToStackSetRequest;
import software.amazon.awssdk.services.cloudformation.model.ImportStacksToStackSetResponse;
import software.amazon.awssdk.services.cloudformation.model.InsufficientCapabilitiesException;
import software.amazon.awssdk.services.cloudformation.model.InvalidChangeSetStatusException;
import software.amazon.awssdk.services.cloudformation.model.InvalidOperationException;
import software.amazon.awssdk.services.cloudformation.model.InvalidStateTransitionException;
import software.amazon.awssdk.services.cloudformation.model.LimitExceededException;
import software.amazon.awssdk.services.cloudformation.model.ListChangeSetsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListChangeSetsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListExportsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListExportsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListGeneratedTemplatesRequest;
import software.amazon.awssdk.services.cloudformation.model.ListGeneratedTemplatesResponse;
import software.amazon.awssdk.services.cloudformation.model.ListHookResultsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListHookResultsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListImportsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListImportsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListResourceScanRelatedResourcesRequest;
import software.amazon.awssdk.services.cloudformation.model.ListResourceScanRelatedResourcesResponse;
import software.amazon.awssdk.services.cloudformation.model.ListResourceScanResourcesRequest;
import software.amazon.awssdk.services.cloudformation.model.ListResourceScanResourcesResponse;
import software.amazon.awssdk.services.cloudformation.model.ListResourceScansRequest;
import software.amazon.awssdk.services.cloudformation.model.ListResourceScansResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStackInstanceResourceDriftsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStackInstanceResourceDriftsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStackInstancesRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStackInstancesResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStackResourcesRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStackResourcesResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetAutoDeploymentTargetsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetAutoDeploymentTargetsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationResultsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationResultsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetOperationsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStackSetsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListStacksRequest;
import software.amazon.awssdk.services.cloudformation.model.ListStacksResponse;
import software.amazon.awssdk.services.cloudformation.model.ListTypeRegistrationsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListTypeRegistrationsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListTypeVersionsRequest;
import software.amazon.awssdk.services.cloudformation.model.ListTypeVersionsResponse;
import software.amazon.awssdk.services.cloudformation.model.ListTypesRequest;
import software.amazon.awssdk.services.cloudformation.model.ListTypesResponse;
import software.amazon.awssdk.services.cloudformation.model.NameAlreadyExistsException;
import software.amazon.awssdk.services.cloudformation.model.OperationIdAlreadyExistsException;
import software.amazon.awssdk.services.cloudformation.model.OperationInProgressException;
import software.amazon.awssdk.services.cloudformation.model.OperationNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.OperationStatusCheckFailedException;
import software.amazon.awssdk.services.cloudformation.model.PublishTypeRequest;
import software.amazon.awssdk.services.cloudformation.model.PublishTypeResponse;
import software.amazon.awssdk.services.cloudformation.model.RecordHandlerProgressRequest;
import software.amazon.awssdk.services.cloudformation.model.RecordHandlerProgressResponse;
import software.amazon.awssdk.services.cloudformation.model.RegisterPublisherRequest;
import software.amazon.awssdk.services.cloudformation.model.RegisterPublisherResponse;
import software.amazon.awssdk.services.cloudformation.model.RegisterTypeRequest;
import software.amazon.awssdk.services.cloudformation.model.RegisterTypeResponse;
import software.amazon.awssdk.services.cloudformation.model.ResourceScanInProgressException;
import software.amazon.awssdk.services.cloudformation.model.ResourceScanLimitExceededException;
import software.amazon.awssdk.services.cloudformation.model.ResourceScanNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.RollbackStackRequest;
import software.amazon.awssdk.services.cloudformation.model.RollbackStackResponse;
import software.amazon.awssdk.services.cloudformation.model.SetStackPolicyRequest;
import software.amazon.awssdk.services.cloudformation.model.SetStackPolicyResponse;
import software.amazon.awssdk.services.cloudformation.model.SetTypeConfigurationRequest;
import software.amazon.awssdk.services.cloudformation.model.SetTypeConfigurationResponse;
import software.amazon.awssdk.services.cloudformation.model.SetTypeDefaultVersionRequest;
import software.amazon.awssdk.services.cloudformation.model.SetTypeDefaultVersionResponse;
import software.amazon.awssdk.services.cloudformation.model.SignalResourceRequest;
import software.amazon.awssdk.services.cloudformation.model.SignalResourceResponse;
import software.amazon.awssdk.services.cloudformation.model.StackInstanceNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.StackNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.StackSetNotEmptyException;
import software.amazon.awssdk.services.cloudformation.model.StackSetNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.StaleRequestException;
import software.amazon.awssdk.services.cloudformation.model.StartResourceScanRequest;
import software.amazon.awssdk.services.cloudformation.model.StartResourceScanResponse;
import software.amazon.awssdk.services.cloudformation.model.StopStackSetOperationRequest;
import software.amazon.awssdk.services.cloudformation.model.StopStackSetOperationResponse;
import software.amazon.awssdk.services.cloudformation.model.TestTypeRequest;
import software.amazon.awssdk.services.cloudformation.model.TestTypeResponse;
import software.amazon.awssdk.services.cloudformation.model.TokenAlreadyExistsException;
import software.amazon.awssdk.services.cloudformation.model.TypeConfigurationNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.TypeNotFoundException;
import software.amazon.awssdk.services.cloudformation.model.UpdateGeneratedTemplateRequest;
import software.amazon.awssdk.services.cloudformation.model.UpdateGeneratedTemplateResponse;
import software.amazon.awssdk.services.cloudformation.model.UpdateStackInstancesRequest;
import software.amazon.awssdk.services.cloudformation.model.UpdateStackInstancesResponse;
import software.amazon.awssdk.services.cloudformation.model.UpdateStackRequest;
import software.amazon.awssdk.services.cloudformation.model.UpdateStackResponse;
import software.amazon.awssdk.services.cloudformation.model.UpdateStackSetRequest;
import software.amazon.awssdk.services.cloudformation.model.UpdateStackSetResponse;
import software.amazon.awssdk.services.cloudformation.model.UpdateTerminationProtectionRequest;
import software.amazon.awssdk.services.cloudformation.model.UpdateTerminationProtectionResponse;
import software.amazon.awssdk.services.cloudformation.model.ValidateTemplateRequest;
import software.amazon.awssdk.services.cloudformation.model.ValidateTemplateResponse;
import software.amazon.awssdk.services.cloudformation.transform.ActivateOrganizationsAccessRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ActivateTypeRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.BatchDescribeTypeConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.CancelUpdateStackRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ContinueUpdateRollbackRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.CreateChangeSetRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.CreateGeneratedTemplateRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.CreateStackInstancesRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.CreateStackRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.CreateStackSetRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DeactivateOrganizationsAccessRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DeactivateTypeRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DeleteChangeSetRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DeleteGeneratedTemplateRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DeleteStackInstancesRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DeleteStackRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DeleteStackSetRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DeregisterTypeRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeAccountLimitsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeChangeSetHooksRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeChangeSetRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeGeneratedTemplateRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeOrganizationsAccessRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribePublisherRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeResourceScanRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeStackDriftDetectionStatusRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeStackEventsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeStackInstanceRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeStackResourceDriftsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeStackResourceRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeStackResourcesRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeStackSetOperationRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeStackSetRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeStacksRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeTypeRegistrationRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DescribeTypeRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DetectStackDriftRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DetectStackResourceDriftRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.DetectStackSetDriftRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.EstimateTemplateCostRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ExecuteChangeSetRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.GetGeneratedTemplateRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.GetStackPolicyRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.GetTemplateRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.GetTemplateSummaryRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ImportStacksToStackSetRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListChangeSetsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListExportsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListGeneratedTemplatesRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListHookResultsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListImportsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListResourceScanRelatedResourcesRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListResourceScanResourcesRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListResourceScansRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListStackInstanceResourceDriftsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListStackInstancesRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListStackResourcesRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListStackSetAutoDeploymentTargetsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListStackSetOperationResultsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListStackSetOperationsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListStackSetsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListStacksRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListTypeRegistrationsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListTypeVersionsRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ListTypesRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.PublishTypeRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.RecordHandlerProgressRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.RegisterPublisherRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.RegisterTypeRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.RollbackStackRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.SetStackPolicyRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.SetTypeConfigurationRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.SetTypeDefaultVersionRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.SignalResourceRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.StartResourceScanRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.StopStackSetOperationRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.TestTypeRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.UpdateGeneratedTemplateRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.UpdateStackInstancesRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.UpdateStackRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.UpdateStackSetRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.UpdateTerminationProtectionRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.transform.ValidateTemplateRequestMarshaller;
import software.amazon.awssdk.services.cloudformation.waiters.CloudFormationAsyncWaiter;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link CloudFormationAsyncClient}.
*
* @see CloudFormationAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultCloudFormationAsyncClient implements CloudFormationAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultCloudFormationAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.QUERY).build();
private final AsyncClientHandler clientHandler;
private final AwsQueryProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final ScheduledExecutorService executorService;
protected DefaultCloudFormationAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init();
this.executorService = clientConfiguration.option(SdkClientOption.SCHEDULED_EXECUTOR_SERVICE);
}
/**
*
* Activate trusted access with Organizations. With trusted access between StackSets and Organizations activated,
* the management account has permissions to create and manage StackSets for your organization.
*
*
* @param activateOrganizationsAccessRequest
* @return A Java Future containing the result of the ActivateOrganizationsAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The specified operation isn't valid.
* - OperationNotFoundException The specified ID refers to an operation that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.ActivateOrganizationsAccess
* @see AWS API Documentation
*/
@Override
public CompletableFuture activateOrganizationsAccess(
ActivateOrganizationsAccessRequest activateOrganizationsAccessRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(activateOrganizationsAccessRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, activateOrganizationsAccessRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ActivateOrganizationsAccess");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ActivateOrganizationsAccessResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ActivateOrganizationsAccess").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ActivateOrganizationsAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(activateOrganizationsAccessRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Activates a public third-party extension, making it available for use in stack templates. Once you have activated
* a public third-party extension in your account and Region, use SetTypeConfiguration to specify configuration properties for the extension. For more information, see Using public
* extensions in the CloudFormation User Guide.
*
*
* @param activateTypeRequest
* @return A Java Future containing the result of the ActivateType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CfnRegistryException An error occurred during a CloudFormation registry operation.
* - TypeNotFoundException The specified extension doesn't exist in the CloudFormation registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.ActivateType
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture activateType(ActivateTypeRequest activateTypeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(activateTypeRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, activateTypeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ActivateType");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ActivateTypeResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ActivateType").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ActivateTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(activateTypeRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns configuration data for the specified CloudFormation extensions, from the CloudFormation registry for the
* account and Region.
*
*
* For more information, see Edit
* configuration data for extensions in your account in the CloudFormation User Guide.
*
*
* @param batchDescribeTypeConfigurationsRequest
* @return A Java Future containing the result of the BatchDescribeTypeConfigurations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TypeConfigurationNotFoundException The specified extension configuration can't be found.
* - CfnRegistryException An error occurred during a CloudFormation registry operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.BatchDescribeTypeConfigurations
* @see AWS API Documentation
*/
@Override
public CompletableFuture batchDescribeTypeConfigurations(
BatchDescribeTypeConfigurationsRequest batchDescribeTypeConfigurationsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchDescribeTypeConfigurationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
batchDescribeTypeConfigurationsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDescribeTypeConfigurations");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(BatchDescribeTypeConfigurationsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchDescribeTypeConfigurations").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchDescribeTypeConfigurationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchDescribeTypeConfigurationsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels an update on the specified stack. If the call completes successfully, the stack rolls back the update and
* reverts to the previous stack configuration.
*
*
*
* You can cancel only stacks that are in the UPDATE_IN_PROGRESS
state.
*
*
*
* @param cancelUpdateStackRequest
* The input for the CancelUpdateStack action.
* @return A Java Future containing the result of the CancelUpdateStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TokenAlreadyExistsException A client request token already exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.CancelUpdateStack
* @see AWS API Documentation
*/
@Override
public CompletableFuture cancelUpdateStack(CancelUpdateStackRequest cancelUpdateStackRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelUpdateStackRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelUpdateStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelUpdateStack");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CancelUpdateStackResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelUpdateStack").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelUpdateStackRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelUpdateStackRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* For a specified stack that's in the UPDATE_ROLLBACK_FAILED
state, continues rolling it back to the
* UPDATE_ROLLBACK_COMPLETE
state. Depending on the cause of the failure, you can manually fix the error and continue the rollback. By continuing the rollback, you can return your stack to a working
* state (the UPDATE_ROLLBACK_COMPLETE
state), and then try to update the stack again.
*
*
* A stack goes into the UPDATE_ROLLBACK_FAILED
state when CloudFormation can't roll back all changes
* after a failed stack update. For example, you might have a stack that's rolling back to an old database instance
* that was deleted outside of CloudFormation. Because CloudFormation doesn't know the database was deleted, it
* assumes that the database instance still exists and attempts to roll back to it, causing the update rollback to
* fail.
*
*
* @param continueUpdateRollbackRequest
* The input for the ContinueUpdateRollback action.
* @return A Java Future containing the result of the ContinueUpdateRollback operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TokenAlreadyExistsException A client request token already exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.ContinueUpdateRollback
* @see AWS API Documentation
*/
@Override
public CompletableFuture continueUpdateRollback(
ContinueUpdateRollbackRequest continueUpdateRollbackRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(continueUpdateRollbackRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, continueUpdateRollbackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ContinueUpdateRollback");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ContinueUpdateRollbackResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ContinueUpdateRollback").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ContinueUpdateRollbackRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(continueUpdateRollbackRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a list of changes that will be applied to a stack so that you can review the changes before executing
* them. You can create a change set for a stack that doesn't exist or an existing stack. If you create a change set
* for a stack that doesn't exist, the change set shows all of the resources that CloudFormation will create. If you
* create a change set for an existing stack, CloudFormation compares the stack's information with the information
* that you submit in the change set and lists the differences. Use change sets to understand which resources
* CloudFormation will create or change, and how it will change resources in an existing stack, before you create or
* update a stack.
*
*
* To create a change set for a stack that doesn't exist, for the ChangeSetType
parameter, specify
* CREATE
. To create a change set for an existing stack, specify UPDATE
for the
* ChangeSetType
parameter. To create a change set for an import operation, specify IMPORT
* for the ChangeSetType
parameter. After the CreateChangeSet
call successfully completes,
* CloudFormation starts creating the change set. To check the status of the change set or to review it, use the
* DescribeChangeSet action.
*
*
* When you are satisfied with the changes the change set will make, execute the change set by using the
* ExecuteChangeSet action. CloudFormation doesn't make changes until you execute the change set.
*
*
* To create a change set for the entire stack hierarchy, set IncludeNestedStacks
to True
.
*
*
* @param createChangeSetRequest
* The input for the CreateChangeSet action.
* @return A Java Future containing the result of the CreateChangeSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AlreadyExistsException The resource with the name requested already exists.
* - InsufficientCapabilitiesException The template contains resources with capabilities that weren't
* specified in the Capabilities parameter.
* - LimitExceededException The quota for the resource has already been reached.
*
* For information about resource and stack limitations, see CloudFormation quotas in the CloudFormation User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.CreateChangeSet
* @see AWS API Documentation
*/
@Override
public CompletableFuture createChangeSet(CreateChangeSetRequest createChangeSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createChangeSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createChangeSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateChangeSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateChangeSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateChangeSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateChangeSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createChangeSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a template from existing resources that are not already managed with CloudFormation. You can check the
* status of the template generation using the DescribeGeneratedTemplate
API action.
*
*
* @param createGeneratedTemplateRequest
* @return A Java Future containing the result of the CreateGeneratedTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AlreadyExistsException The resource with the name requested already exists.
* - LimitExceededException The quota for the resource has already been reached.
*
* For information about resource and stack limitations, see CloudFormation quotas in the CloudFormation User Guide.
* - ConcurrentResourcesLimitExceededException No more than 5 generated templates can be in an
*
InProgress
or Pending
status at one time. This error is also returned if a
* generated template that is in an InProgress
or Pending
status is attempted to
* be updated or deleted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.CreateGeneratedTemplate
* @see AWS API Documentation
*/
@Override
public CompletableFuture createGeneratedTemplate(
CreateGeneratedTemplateRequest createGeneratedTemplateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createGeneratedTemplateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createGeneratedTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateGeneratedTemplate");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateGeneratedTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateGeneratedTemplate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateGeneratedTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createGeneratedTemplateRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a stack as specified in the template. After the call completes successfully, the stack creation starts.
* You can check the status of the stack through the DescribeStacks operation.
*
*
* For more information about creating a stack and monitoring stack progress, see Managing Amazon Web Services
* resources as a single unit with CloudFormation stacks in the CloudFormation User Guide.
*
*
* @param createStackRequest
* The input for CreateStack action.
* @return A Java Future containing the result of the CreateStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException The quota for the resource has already been reached.
*
* For information about resource and stack limitations, see CloudFormation quotas in the CloudFormation User Guide.
* - AlreadyExistsException The resource with the name requested already exists.
* - TokenAlreadyExistsException A client request token already exists.
* - InsufficientCapabilitiesException The template contains resources with capabilities that weren't
* specified in the Capabilities parameter.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.CreateStack
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createStack(CreateStackRequest createStackRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createStackRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateStack");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateStackResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateStack").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateStackRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createStackRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates stack instances for the specified accounts, within the specified Amazon Web Services Regions. A stack
* instance refers to a stack in a specific account and Region. You must specify at least one value for either
* Accounts
or DeploymentTargets
, and you must specify at least one value for
* Regions
.
*
*
* @param createStackInstancesRequest
* @return A Java Future containing the result of the CreateStackInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - StackSetNotFoundException The specified stack set doesn't exist.
* - OperationInProgressException Another operation is currently in progress for this stack set. Only one
* operation can be performed for a stack set at a given time.
* - OperationIdAlreadyExistsException The specified operation ID already exists.
* - StaleRequestException Another operation has been performed on this stack set since the specified
* operation was performed.
* - InvalidOperationException The specified operation isn't valid.
* - LimitExceededException The quota for the resource has already been reached.
*
* For information about resource and stack limitations, see CloudFormation quotas in the CloudFormation User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.CreateStackInstances
* @see AWS API Documentation
*/
@Override
public CompletableFuture createStackInstances(
CreateStackInstancesRequest createStackInstancesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createStackInstancesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createStackInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateStackInstances");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateStackInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateStackInstances").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateStackInstancesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createStackInstancesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a stack set.
*
*
* @param createStackSetRequest
* @return A Java Future containing the result of the CreateStackSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NameAlreadyExistsException The specified name is already in use.
* - CreatedButModifiedException The specified resource exists, but has been changed.
* - LimitExceededException The quota for the resource has already been reached.
*
* For information about resource and stack limitations, see CloudFormation quotas in the CloudFormation User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.CreateStackSet
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createStackSet(CreateStackSetRequest createStackSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createStackSetRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createStackSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateStackSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateStackSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateStackSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateStackSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createStackSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deactivates trusted access with Organizations. If trusted access is deactivated, the management account does not
* have permissions to create and manage service-managed StackSets for your organization.
*
*
* @param deactivateOrganizationsAccessRequest
* @return A Java Future containing the result of the DeactivateOrganizationsAccess operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The specified operation isn't valid.
* - OperationNotFoundException The specified ID refers to an operation that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DeactivateOrganizationsAccess
* @see AWS API Documentation
*/
@Override
public CompletableFuture deactivateOrganizationsAccess(
DeactivateOrganizationsAccessRequest deactivateOrganizationsAccessRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deactivateOrganizationsAccessRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deactivateOrganizationsAccessRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeactivateOrganizationsAccess");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeactivateOrganizationsAccessResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeactivateOrganizationsAccess").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeactivateOrganizationsAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deactivateOrganizationsAccessRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deactivates a public extension that was previously activated in this account and Region.
*
*
* Once deactivated, an extension can't be used in any CloudFormation operation. This includes stack update
* operations where the stack template includes the extension, even if no updates are being made to the extension.
* In addition, deactivated extensions aren't automatically updated if a new version of the extension is released.
*
*
* @param deactivateTypeRequest
* @return A Java Future containing the result of the DeactivateType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CfnRegistryException An error occurred during a CloudFormation registry operation.
* - TypeNotFoundException The specified extension doesn't exist in the CloudFormation registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DeactivateType
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deactivateType(DeactivateTypeRequest deactivateTypeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deactivateTypeRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deactivateTypeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeactivateType");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeactivateTypeResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeactivateType").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeactivateTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deactivateTypeRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified change set. Deleting change sets ensures that no one executes the wrong change set.
*
*
* If the call successfully completes, CloudFormation successfully deleted the change set.
*
*
* If IncludeNestedStacks
specifies True
during the creation of the nested change set,
* then DeleteChangeSet
will delete all change sets that belong to the stacks hierarchy and will also
* delete all change sets for nested stacks with the status of REVIEW_IN_PROGRESS
.
*
*
* @param deleteChangeSetRequest
* The input for the DeleteChangeSet action.
* @return A Java Future containing the result of the DeleteChangeSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidChangeSetStatusException The specified change set can't be used to update the stack. For
* example, the change set status might be
CREATE_IN_PROGRESS
, or the stack status might be
* UPDATE_IN_PROGRESS
.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DeleteChangeSet
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteChangeSet(DeleteChangeSetRequest deleteChangeSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteChangeSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteChangeSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteChangeSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteChangeSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteChangeSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteChangeSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteChangeSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deleted a generated template.
*
*
* @param deleteGeneratedTemplateRequest
* @return A Java Future containing the result of the DeleteGeneratedTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GeneratedTemplateNotFoundException The generated template was not found.
* - ConcurrentResourcesLimitExceededException No more than 5 generated templates can be in an
*
InProgress
or Pending
status at one time. This error is also returned if a
* generated template that is in an InProgress
or Pending
status is attempted to
* be updated or deleted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DeleteGeneratedTemplate
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteGeneratedTemplate(
DeleteGeneratedTemplateRequest deleteGeneratedTemplateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteGeneratedTemplateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteGeneratedTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteGeneratedTemplate");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteGeneratedTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteGeneratedTemplate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteGeneratedTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteGeneratedTemplateRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specified stack. Once the call completes successfully, stack deletion starts. Deleted stacks don't show
* up in the DescribeStacks operation if the deletion has been completed successfully.
*
*
* For more information about deleting a stack, see Delete a
* stack from the CloudFormation console in the CloudFormation User Guide.
*
*
* @param deleteStackRequest
* The input for DeleteStack action.
* @return A Java Future containing the result of the DeleteStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TokenAlreadyExistsException A client request token already exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DeleteStack
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteStack(DeleteStackRequest deleteStackRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteStackRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteStack");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteStackResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteStack").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteStackRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteStackRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes stack instances for the specified accounts, in the specified Amazon Web Services Regions.
*
*
* @param deleteStackInstancesRequest
* @return A Java Future containing the result of the DeleteStackInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - StackSetNotFoundException The specified stack set doesn't exist.
* - OperationInProgressException Another operation is currently in progress for this stack set. Only one
* operation can be performed for a stack set at a given time.
* - OperationIdAlreadyExistsException The specified operation ID already exists.
* - StaleRequestException Another operation has been performed on this stack set since the specified
* operation was performed.
* - InvalidOperationException The specified operation isn't valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DeleteStackInstances
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteStackInstances(
DeleteStackInstancesRequest deleteStackInstancesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteStackInstancesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteStackInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteStackInstances");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteStackInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteStackInstances").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteStackInstancesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteStackInstancesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a stack set. Before you can delete a stack set, all its member stack instances must be deleted. For more
* information about how to complete this, see DeleteStackInstances.
*
*
* @param deleteStackSetRequest
* @return A Java Future containing the result of the DeleteStackSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - StackSetNotEmptyException You can't yet delete this stack set, because it still contains one or more
* stack instances. Delete all stack instances from the stack set before deleting the stack set.
* - OperationInProgressException Another operation is currently in progress for this stack set. Only one
* operation can be performed for a stack set at a given time.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DeleteStackSet
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteStackSet(DeleteStackSetRequest deleteStackSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteStackSetRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteStackSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteStackSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteStackSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteStackSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteStackSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteStackSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Marks an extension or extension version as DEPRECATED
in the CloudFormation registry, removing it
* from active use. Deprecated extensions or extension versions cannot be used in CloudFormation operations.
*
*
* To deregister an entire extension, you must individually deregister all active versions of that extension. If an
* extension has only a single active version, deregistering that version results in the extension itself being
* deregistered and marked as deprecated in the registry.
*
*
* You can't deregister the default version of an extension if there are other active version of that extension. If
* you do deregister the default version of an extension, the extension type itself is deregistered as well and
* marked as deprecated.
*
*
* To view the deprecation status of an extension or extension version, use DescribeType.
*
*
* @param deregisterTypeRequest
* @return A Java Future containing the result of the DeregisterType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CfnRegistryException An error occurred during a CloudFormation registry operation.
* - TypeNotFoundException The specified extension doesn't exist in the CloudFormation registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DeregisterType
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deregisterType(DeregisterTypeRequest deregisterTypeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deregisterTypeRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterTypeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterType");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeregisterTypeResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterType").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeregisterTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deregisterTypeRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves your account's CloudFormation limits, such as the maximum number of stacks that you can create in your
* account. For more information about account limits, see Understand
* CloudFormation quotas in the CloudFormation User Guide.
*
*
* @param describeAccountLimitsRequest
* The input for the DescribeAccountLimits action.
* @return A Java Future containing the result of the DescribeAccountLimits operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeAccountLimits
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeAccountLimits(
DescribeAccountLimitsRequest describeAccountLimitsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeAccountLimitsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAccountLimitsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAccountLimits");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeAccountLimitsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeAccountLimits").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeAccountLimitsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeAccountLimitsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the inputs for the change set and a list of changes that CloudFormation will make if you execute the
* change set. For more information, see Update CloudFormation stacks using change sets in the CloudFormation User Guide.
*
*
* @param describeChangeSetRequest
* The input for the DescribeChangeSet action.
* @return A Java Future containing the result of the DescribeChangeSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ChangeSetNotFoundException The specified change set name or ID doesn't exit. To view valid change
* sets for a stack, use the
ListChangeSets
operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeChangeSet
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeChangeSet(DescribeChangeSetRequest describeChangeSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeChangeSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeChangeSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeChangeSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeChangeSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeChangeSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeChangeSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeChangeSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns hook-related information for the change set and a list of changes that CloudFormation makes when you run
* the change set.
*
*
* @param describeChangeSetHooksRequest
* @return A Java Future containing the result of the DescribeChangeSetHooks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ChangeSetNotFoundException The specified change set name or ID doesn't exit. To view valid change
* sets for a stack, use the
ListChangeSets
operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeChangeSetHooks
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeChangeSetHooks(
DescribeChangeSetHooksRequest describeChangeSetHooksRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeChangeSetHooksRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeChangeSetHooksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeChangeSetHooks");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeChangeSetHooksResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeChangeSetHooks").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeChangeSetHooksRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeChangeSetHooksRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes a generated template. The output includes details about the progress of the creation of a generated
* template started by a CreateGeneratedTemplate
API action or the update of a generated template
* started with an UpdateGeneratedTemplate
API action.
*
*
* @param describeGeneratedTemplateRequest
* @return A Java Future containing the result of the DescribeGeneratedTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - GeneratedTemplateNotFoundException The generated template was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeGeneratedTemplate
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeGeneratedTemplate(
DescribeGeneratedTemplateRequest describeGeneratedTemplateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeGeneratedTemplateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeGeneratedTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeGeneratedTemplate");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeGeneratedTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeGeneratedTemplate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeGeneratedTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeGeneratedTemplateRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about the account's OrganizationAccess
status. This API can be called either
* by the management account or the delegated administrator by using the CallAs
parameter. This API can
* also be called without the CallAs
parameter by the management account.
*
*
* @param describeOrganizationsAccessRequest
* @return A Java Future containing the result of the DescribeOrganizationsAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidOperationException The specified operation isn't valid.
* - OperationNotFoundException The specified ID refers to an operation that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeOrganizationsAccess
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeOrganizationsAccess(
DescribeOrganizationsAccessRequest describeOrganizationsAccessRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeOrganizationsAccessRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeOrganizationsAccessRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeOrganizationsAccess");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeOrganizationsAccessResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeOrganizationsAccess").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeOrganizationsAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeOrganizationsAccessRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about a CloudFormation extension publisher.
*
*
* If you don't supply a PublisherId
, and you have registered as an extension publisher,
* DescribePublisher
returns information about your own publisher account.
*
*
* For more information about registering as a publisher, see:
*
*
* -
*
*
* -
*
* Publishing
* extensions to make them available for public use in the CloudFormation Command Line Interface (CLI) User
* Guide
*
*
*
*
* @param describePublisherRequest
* @return A Java Future containing the result of the DescribePublisher operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CfnRegistryException An error occurred during a CloudFormation registry operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribePublisher
* @see AWS API Documentation
*/
@Override
public CompletableFuture describePublisher(DescribePublisherRequest describePublisherRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describePublisherRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describePublisherRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribePublisher");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribePublisherResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribePublisher").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribePublisherRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describePublisherRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes details of a resource scan.
*
*
* @param describeResourceScanRequest
* @return A Java Future containing the result of the DescribeResourceScan operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceScanNotFoundException The resource scan was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeResourceScan
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeResourceScan(
DescribeResourceScanRequest describeResourceScanRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeResourceScanRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeResourceScanRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeResourceScan");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeResourceScanResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeResourceScan").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeResourceScanRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeResourceScanRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about a stack drift detection operation. A stack drift detection operation detects whether a
* stack's actual configuration differs, or has drifted, from its expected configuration, as defined in the
* stack template and any values specified as template parameters. A stack is considered to have drifted if one or
* more of its resources have drifted. For more information about stack and resource drift, see Detect unmanaged
* configuration changes to stacks and resources with drift detection.
*
*
* Use DetectStackDrift to initiate a stack drift detection operation. DetectStackDrift
returns
* a StackDriftDetectionId
you can use to monitor the progress of the operation using
* DescribeStackDriftDetectionStatus
. Once the drift detection operation has completed, use
* DescribeStackResourceDrifts to return drift information about the stack and its resources.
*
*
* @param describeStackDriftDetectionStatusRequest
* @return A Java Future containing the result of the DescribeStackDriftDetectionStatus operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeStackDriftDetectionStatus
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeStackDriftDetectionStatus(
DescribeStackDriftDetectionStatusRequest describeStackDriftDetectionStatusRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStackDriftDetectionStatusRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeStackDriftDetectionStatusRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStackDriftDetectionStatus");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeStackDriftDetectionStatusResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStackDriftDetectionStatus").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeStackDriftDetectionStatusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeStackDriftDetectionStatusRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns all stack related events for a specified stack in reverse chronological order. For more information about
* a stack's event history, see Understand CloudFormation stack creation events in the CloudFormation User Guide.
*
*
*
* You can list events for stacks that have failed to create or have been deleted by specifying the unique stack
* identifier (stack ID).
*
*
*
* @param describeStackEventsRequest
* The input for DescribeStackEvents action.
* @return A Java Future containing the result of the DescribeStackEvents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeStackEvents
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeStackEvents(
DescribeStackEventsRequest describeStackEventsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStackEventsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStackEventsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStackEvents");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeStackEventsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStackEvents").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeStackEventsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeStackEventsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the stack instance that's associated with the specified StackSet, Amazon Web Services account, and Amazon
* Web Services Region.
*
*
* For a list of stack instances that are associated with a specific StackSet, use ListStackInstances.
*
*
* @param describeStackInstanceRequest
* @return A Java Future containing the result of the DescribeStackInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - StackSetNotFoundException The specified stack set doesn't exist.
* - StackInstanceNotFoundException The specified stack instance doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeStackInstance
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeStackInstance(
DescribeStackInstanceRequest describeStackInstanceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStackInstanceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStackInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStackInstance");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeStackInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStackInstance").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeStackInstanceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeStackInstanceRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a description of the specified resource in the specified stack.
*
*
* For deleted stacks, DescribeStackResource returns resource information for up to 90 days after the stack has been
* deleted.
*
*
* @param describeStackResourceRequest
* The input for DescribeStackResource action.
* @return A Java Future containing the result of the DescribeStackResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeStackResource
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeStackResource(
DescribeStackResourceRequest describeStackResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStackResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStackResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStackResource");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeStackResourceResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStackResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeStackResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeStackResourceRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns drift information for the resources that have been checked for drift in the specified stack. This
* includes actual and expected configuration values for resources where CloudFormation detects configuration drift.
*
*
* For a given stack, there will be one StackResourceDrift
for each stack resource that has been
* checked for drift. Resources that haven't yet been checked for drift aren't included. Resources that don't
* currently support drift detection aren't checked, and so not included. For a list of resources that support drift
* detection, see Resource type support for imports and drift detection.
*
*
* Use DetectStackResourceDrift to detect drift on individual resources, or DetectStackDrift to detect
* drift on all supported resources for a given stack.
*
*
* @param describeStackResourceDriftsRequest
* @return A Java Future containing the result of the DescribeStackResourceDrifts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeStackResourceDrifts
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeStackResourceDrifts(
DescribeStackResourceDriftsRequest describeStackResourceDriftsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStackResourceDriftsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStackResourceDriftsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStackResourceDrifts");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeStackResourceDriftsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStackResourceDrifts").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeStackResourceDriftsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeStackResourceDriftsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns Amazon Web Services resource descriptions for running and deleted stacks. If StackName
is
* specified, all the associated resources that are part of the stack are returned. If
* PhysicalResourceId
is specified, the associated resources of the stack that the resource belongs to
* are returned.
*
*
*
* Only the first 100 resources will be returned. If your stack has more resources than this, you should use
* ListStackResources
instead.
*
*
*
* For deleted stacks, DescribeStackResources
returns resource information for up to 90 days after the
* stack has been deleted.
*
*
* You must specify either StackName
or PhysicalResourceId
, but not both. In addition, you
* can specify LogicalResourceId
to filter the returned result. For more information about resources,
* the LogicalResourceId
and PhysicalResourceId
, see the CloudFormation User Guide.
*
*
*
* A ValidationError
is returned if you specify both StackName
and
* PhysicalResourceId
in the same request.
*
*
*
* @param describeStackResourcesRequest
* The input for DescribeStackResources action.
* @return A Java Future containing the result of the DescribeStackResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeStackResources
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeStackResources(
DescribeStackResourcesRequest describeStackResourcesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStackResourcesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStackResourcesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStackResources");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeStackResourcesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStackResources").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeStackResourcesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeStackResourcesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the description of the specified StackSet.
*
*
* @param describeStackSetRequest
* @return A Java Future containing the result of the DescribeStackSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - StackSetNotFoundException The specified stack set doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeStackSet
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeStackSet(DescribeStackSetRequest describeStackSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStackSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStackSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStackSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeStackSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStackSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeStackSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeStackSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the description of the specified StackSet operation.
*
*
* @param describeStackSetOperationRequest
* @return A Java Future containing the result of the DescribeStackSetOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - StackSetNotFoundException The specified stack set doesn't exist.
* - OperationNotFoundException The specified ID refers to an operation that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeStackSetOperation
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeStackSetOperation(
DescribeStackSetOperationRequest describeStackSetOperationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStackSetOperationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStackSetOperationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStackSetOperation");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeStackSetOperationResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStackSetOperation").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeStackSetOperationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeStackSetOperationRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the description for the specified stack; if no stack name was specified, then it returns the description
* for all the stacks created. For more information about a stack's event history, see Understand CloudFormation stack creation events in the CloudFormation User Guide.
*
*
*
* If the stack doesn't exist, a ValidationError
is returned.
*
*
*
* @param describeStacksRequest
* The input for DescribeStacks action.
* @return A Java Future containing the result of the DescribeStacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeStacks
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeStacks(DescribeStacksRequest describeStacksRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStacksRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStacksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStacks");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeStacksResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStacks").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeStacksRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeStacksRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns detailed information about an extension that has been registered.
*
*
* If you specify a VersionId
, DescribeType
returns information about that specific
* extension version. Otherwise, it returns information about the default extension version.
*
*
* @param describeTypeRequest
* @return A Java Future containing the result of the DescribeType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CfnRegistryException An error occurred during a CloudFormation registry operation.
* - TypeNotFoundException The specified extension doesn't exist in the CloudFormation registry.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeType
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeType(DescribeTypeRequest describeTypeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeTypeRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTypeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeType");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeTypeResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeType").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeTypeRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about an extension's registration, including its current status and type and version
* identifiers.
*
*
* When you initiate a registration request using RegisterType, you can then use
* DescribeTypeRegistration to monitor the progress of that registration request.
*
*
* Once the registration request has completed, use DescribeType to return detailed information about an
* extension.
*
*
* @param describeTypeRegistrationRequest
* @return A Java Future containing the result of the DescribeTypeRegistration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CfnRegistryException An error occurred during a CloudFormation registry operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudFormationException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample CloudFormationAsyncClient.DescribeTypeRegistration
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeTypeRegistration(
DescribeTypeRegistrationRequest describeTypeRegistrationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeTypeRegistrationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTypeRegistrationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFormation");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTypeRegistration");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeTypeRegistrationResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams