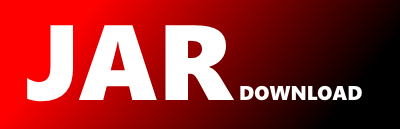
software.amazon.awssdk.services.cloudformation.model.UpdateStackInstancesRequest Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateStackInstancesRequest extends CloudFormationRequest implements
ToCopyableBuilder {
private static final SdkField STACK_SET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackSetName").getter(getter(UpdateStackInstancesRequest::stackSetName))
.setter(setter(Builder::stackSetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackSetName").build()).build();
private static final SdkField> ACCOUNTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Accounts")
.getter(getter(UpdateStackInstancesRequest::accounts))
.setter(setter(Builder::accounts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Accounts").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DEPLOYMENT_TARGETS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DeploymentTargets")
.getter(getter(UpdateStackInstancesRequest::deploymentTargets)).setter(setter(Builder::deploymentTargets))
.constructor(DeploymentTargets::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeploymentTargets").build()).build();
private static final SdkField> REGIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Regions")
.getter(getter(UpdateStackInstancesRequest::regions))
.setter(setter(Builder::regions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Regions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> PARAMETER_OVERRIDES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ParameterOverrides")
.getter(getter(UpdateStackInstancesRequest::parameterOverrides))
.setter(setter(Builder::parameterOverrides))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ParameterOverrides").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Parameter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField OPERATION_PREFERENCES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OperationPreferences")
.getter(getter(UpdateStackInstancesRequest::operationPreferences)).setter(setter(Builder::operationPreferences))
.constructor(StackSetOperationPreferences::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OperationPreferences").build())
.build();
private static final SdkField OPERATION_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OperationId")
.getter(getter(UpdateStackInstancesRequest::operationId))
.setter(setter(Builder::operationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OperationId").build(),
DefaultValueTrait.idempotencyToken()).build();
private static final SdkField CALL_AS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("CallAs")
.getter(getter(UpdateStackInstancesRequest::callAsAsString)).setter(setter(Builder::callAs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CallAs").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STACK_SET_NAME_FIELD,
ACCOUNTS_FIELD, DEPLOYMENT_TARGETS_FIELD, REGIONS_FIELD, PARAMETER_OVERRIDES_FIELD, OPERATION_PREFERENCES_FIELD,
OPERATION_ID_FIELD, CALL_AS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("StackSetName", STACK_SET_NAME_FIELD);
put("Accounts", ACCOUNTS_FIELD);
put("DeploymentTargets", DEPLOYMENT_TARGETS_FIELD);
put("Regions", REGIONS_FIELD);
put("ParameterOverrides", PARAMETER_OVERRIDES_FIELD);
put("OperationPreferences", OPERATION_PREFERENCES_FIELD);
put("OperationId", OPERATION_ID_FIELD);
put("CallAs", CALL_AS_FIELD);
}
});
private final String stackSetName;
private final List accounts;
private final DeploymentTargets deploymentTargets;
private final List regions;
private final List parameterOverrides;
private final StackSetOperationPreferences operationPreferences;
private final String operationId;
private final String callAs;
private UpdateStackInstancesRequest(BuilderImpl builder) {
super(builder);
this.stackSetName = builder.stackSetName;
this.accounts = builder.accounts;
this.deploymentTargets = builder.deploymentTargets;
this.regions = builder.regions;
this.parameterOverrides = builder.parameterOverrides;
this.operationPreferences = builder.operationPreferences;
this.operationId = builder.operationId;
this.callAs = builder.callAs;
}
/**
*
* The name or unique ID of the stack set associated with the stack instances.
*
*
* @return The name or unique ID of the stack set associated with the stack instances.
*/
public final String stackSetName() {
return stackSetName;
}
/**
* For responses, this returns true if the service returned a value for the Accounts property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasAccounts() {
return accounts != null && !(accounts instanceof SdkAutoConstructList);
}
/**
*
* [Self-managed permissions] The names of one or more Amazon Web Services accounts for which you want to update
* parameter values for stack instances. The overridden parameter values will be applied to all stack instances in
* the specified accounts and Amazon Web Services Regions.
*
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAccounts} method.
*
*
* @return [Self-managed permissions] The names of one or more Amazon Web Services accounts for which you want to
* update parameter values for stack instances. The overridden parameter values will be applied to all stack
* instances in the specified accounts and Amazon Web Services Regions.
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*/
public final List accounts() {
return accounts;
}
/**
*
* [Service-managed permissions] The Organizations accounts for which you want to update parameter values for stack
* instances. If your update targets OUs, the overridden parameter values only apply to the accounts that are
* currently in the target OUs and their child OUs. Accounts added to the target OUs and their child OUs in the
* future won't use the overridden values.
*
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*
*
* @return [Service-managed permissions] The Organizations accounts for which you want to update parameter values
* for stack instances. If your update targets OUs, the overridden parameter values only apply to the
* accounts that are currently in the target OUs and their child OUs. Accounts added to the target OUs and
* their child OUs in the future won't use the overridden values.
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*/
public final DeploymentTargets deploymentTargets() {
return deploymentTargets;
}
/**
* For responses, this returns true if the service returned a value for the Regions property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasRegions() {
return regions != null && !(regions instanceof SdkAutoConstructList);
}
/**
*
* The names of one or more Amazon Web Services Regions in which you want to update parameter values for stack
* instances. The overridden parameter values will be applied to all stack instances in the specified accounts and
* Amazon Web Services Regions.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRegions} method.
*
*
* @return The names of one or more Amazon Web Services Regions in which you want to update parameter values for
* stack instances. The overridden parameter values will be applied to all stack instances in the specified
* accounts and Amazon Web Services Regions.
*/
public final List regions() {
return regions;
}
/**
* For responses, this returns true if the service returned a value for the ParameterOverrides property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasParameterOverrides() {
return parameterOverrides != null && !(parameterOverrides instanceof SdkAutoConstructList);
}
/**
*
* A list of input parameters whose values you want to update for the specified stack instances.
*
*
* Any overridden parameter values will be applied to all stack instances in the specified accounts and Amazon Web
* Services Regions. When specifying parameters and their values, be aware of how CloudFormation sets parameter
* values during stack instance update operations:
*
*
* -
*
* To override the current value for a parameter, include the parameter and specify its value.
*
*
* -
*
* To leave an overridden parameter set to its present value, include the parameter and specify
* UsePreviousValue
as true
. (You can't specify both a value and set
* UsePreviousValue
to true
.)
*
*
* -
*
* To set an overridden parameter back to the value specified in the stack set, specify a parameter list but don't
* include the parameter in the list.
*
*
* -
*
* To leave all parameters set to their present values, don't specify this property at all.
*
*
*
*
* During stack set updates, any parameter values overridden for a stack instance aren't updated, but retain their
* overridden value.
*
*
* You can only override the parameter values that are specified in the stack set; to add or delete a
* parameter itself, use UpdateStackSet
to update the stack set template. If you add a parameter to a
* template, before you can override the parameter value specified in the stack set you must first use UpdateStackSet to update all stack instances with the updated template and parameter value specified in the
* stack set. Once a stack instance has been updated with the new parameter, you can then override the parameter
* value using UpdateStackInstances
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasParameterOverrides} method.
*
*
* @return A list of input parameters whose values you want to update for the specified stack instances.
*
* Any overridden parameter values will be applied to all stack instances in the specified accounts and
* Amazon Web Services Regions. When specifying parameters and their values, be aware of how CloudFormation
* sets parameter values during stack instance update operations:
*
*
* -
*
* To override the current value for a parameter, include the parameter and specify its value.
*
*
* -
*
* To leave an overridden parameter set to its present value, include the parameter and specify
* UsePreviousValue
as true
. (You can't specify both a value and set
* UsePreviousValue
to true
.)
*
*
* -
*
* To set an overridden parameter back to the value specified in the stack set, specify a parameter list but
* don't include the parameter in the list.
*
*
* -
*
* To leave all parameters set to their present values, don't specify this property at all.
*
*
*
*
* During stack set updates, any parameter values overridden for a stack instance aren't updated, but retain
* their overridden value.
*
*
* You can only override the parameter values that are specified in the stack set; to add or delete a
* parameter itself, use UpdateStackSet
to update the stack set template. If you add a
* parameter to a template, before you can override the parameter value specified in the stack set you must
* first use UpdateStackSet to update all stack instances with the updated template and parameter value specified
* in the stack set. Once a stack instance has been updated with the new parameter, you can then override
* the parameter value using UpdateStackInstances
.
*/
public final List parameterOverrides() {
return parameterOverrides;
}
/**
*
* Preferences for how CloudFormation performs this stack set operation.
*
*
* @return Preferences for how CloudFormation performs this stack set operation.
*/
public final StackSetOperationPreferences operationPreferences() {
return operationPreferences;
}
/**
*
* The unique identifier for this stack set operation.
*
*
* The operation ID also functions as an idempotency token, to ensure that CloudFormation performs the stack set
* operation only once, even if you retry the request multiple times. You might retry stack set operation requests
* to ensure that CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* @return The unique identifier for this stack set operation.
*
* The operation ID also functions as an idempotency token, to ensure that CloudFormation performs the stack
* set operation only once, even if you retry the request multiple times. You might retry stack set
* operation requests to ensure that CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*/
public final String operationId() {
return operationId;
}
/**
*
* [Service-managed permissions] Specifies whether you are acting as an account administrator in the organization's
* management account or as a delegated administrator in a member account.
*
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management account. For
* more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #callAs} will
* return {@link CallAs#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #callAsAsString}.
*
*
* @return [Service-managed permissions] Specifies whether you are acting as an account administrator in the
* organization's management account or as a delegated administrator in a member account.
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed
* permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management
* account. For more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
* @see CallAs
*/
public final CallAs callAs() {
return CallAs.fromValue(callAs);
}
/**
*
* [Service-managed permissions] Specifies whether you are acting as an account administrator in the organization's
* management account or as a delegated administrator in a member account.
*
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management account. For
* more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #callAs} will
* return {@link CallAs#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #callAsAsString}.
*
*
* @return [Service-managed permissions] Specifies whether you are acting as an account administrator in the
* organization's management account or as a delegated administrator in a member account.
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed
* permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management
* account. For more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
* @see CallAs
*/
public final String callAsAsString() {
return callAs;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(stackSetName());
hashCode = 31 * hashCode + Objects.hashCode(hasAccounts() ? accounts() : null);
hashCode = 31 * hashCode + Objects.hashCode(deploymentTargets());
hashCode = 31 * hashCode + Objects.hashCode(hasRegions() ? regions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasParameterOverrides() ? parameterOverrides() : null);
hashCode = 31 * hashCode + Objects.hashCode(operationPreferences());
hashCode = 31 * hashCode + Objects.hashCode(operationId());
hashCode = 31 * hashCode + Objects.hashCode(callAsAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateStackInstancesRequest)) {
return false;
}
UpdateStackInstancesRequest other = (UpdateStackInstancesRequest) obj;
return Objects.equals(stackSetName(), other.stackSetName()) && hasAccounts() == other.hasAccounts()
&& Objects.equals(accounts(), other.accounts()) && Objects.equals(deploymentTargets(), other.deploymentTargets())
&& hasRegions() == other.hasRegions() && Objects.equals(regions(), other.regions())
&& hasParameterOverrides() == other.hasParameterOverrides()
&& Objects.equals(parameterOverrides(), other.parameterOverrides())
&& Objects.equals(operationPreferences(), other.operationPreferences())
&& Objects.equals(operationId(), other.operationId()) && Objects.equals(callAsAsString(), other.callAsAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateStackInstancesRequest").add("StackSetName", stackSetName())
.add("Accounts", hasAccounts() ? accounts() : null).add("DeploymentTargets", deploymentTargets())
.add("Regions", hasRegions() ? regions() : null)
.add("ParameterOverrides", hasParameterOverrides() ? parameterOverrides() : null)
.add("OperationPreferences", operationPreferences()).add("OperationId", operationId())
.add("CallAs", callAsAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackSetName":
return Optional.ofNullable(clazz.cast(stackSetName()));
case "Accounts":
return Optional.ofNullable(clazz.cast(accounts()));
case "DeploymentTargets":
return Optional.ofNullable(clazz.cast(deploymentTargets()));
case "Regions":
return Optional.ofNullable(clazz.cast(regions()));
case "ParameterOverrides":
return Optional.ofNullable(clazz.cast(parameterOverrides()));
case "OperationPreferences":
return Optional.ofNullable(clazz.cast(operationPreferences()));
case "OperationId":
return Optional.ofNullable(clazz.cast(operationId()));
case "CallAs":
return Optional.ofNullable(clazz.cast(callAsAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function