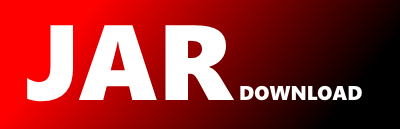
software.amazon.awssdk.services.cloudformation.model.StackSummary Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The StackSummary Data Type
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class StackSummary implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField STACK_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackId").getter(getter(StackSummary::stackId)).setter(setter(Builder::stackId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackId").build()).build();
private static final SdkField STACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackName").getter(getter(StackSummary::stackName)).setter(setter(Builder::stackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackName").build()).build();
private static final SdkField TEMPLATE_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateDescription").getter(getter(StackSummary::templateDescription))
.setter(setter(Builder::templateDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateDescription").build())
.build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTime").getter(getter(StackSummary::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField LAST_UPDATED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastUpdatedTime").getter(getter(StackSummary::lastUpdatedTime)).setter(setter(Builder::lastUpdatedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastUpdatedTime").build()).build();
private static final SdkField DELETION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("DeletionTime").getter(getter(StackSummary::deletionTime)).setter(setter(Builder::deletionTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeletionTime").build()).build();
private static final SdkField STACK_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackStatus").getter(getter(StackSummary::stackStatusAsString)).setter(setter(Builder::stackStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackStatus").build()).build();
private static final SdkField STACK_STATUS_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackStatusReason").getter(getter(StackSummary::stackStatusReason))
.setter(setter(Builder::stackStatusReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackStatusReason").build()).build();
private static final SdkField PARENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ParentId").getter(getter(StackSummary::parentId)).setter(setter(Builder::parentId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ParentId").build()).build();
private static final SdkField ROOT_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("RootId")
.getter(getter(StackSummary::rootId)).setter(setter(Builder::rootId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RootId").build()).build();
private static final SdkField DRIFT_INFORMATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DriftInformation")
.getter(getter(StackSummary::driftInformation)).setter(setter(Builder::driftInformation))
.constructor(StackDriftInformationSummary::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DriftInformation").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STACK_ID_FIELD,
STACK_NAME_FIELD, TEMPLATE_DESCRIPTION_FIELD, CREATION_TIME_FIELD, LAST_UPDATED_TIME_FIELD, DELETION_TIME_FIELD,
STACK_STATUS_FIELD, STACK_STATUS_REASON_FIELD, PARENT_ID_FIELD, ROOT_ID_FIELD, DRIFT_INFORMATION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("StackId", STACK_ID_FIELD);
put("StackName", STACK_NAME_FIELD);
put("TemplateDescription", TEMPLATE_DESCRIPTION_FIELD);
put("CreationTime", CREATION_TIME_FIELD);
put("LastUpdatedTime", LAST_UPDATED_TIME_FIELD);
put("DeletionTime", DELETION_TIME_FIELD);
put("StackStatus", STACK_STATUS_FIELD);
put("StackStatusReason", STACK_STATUS_REASON_FIELD);
put("ParentId", PARENT_ID_FIELD);
put("RootId", ROOT_ID_FIELD);
put("DriftInformation", DRIFT_INFORMATION_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String stackId;
private final String stackName;
private final String templateDescription;
private final Instant creationTime;
private final Instant lastUpdatedTime;
private final Instant deletionTime;
private final String stackStatus;
private final String stackStatusReason;
private final String parentId;
private final String rootId;
private final StackDriftInformationSummary driftInformation;
private StackSummary(BuilderImpl builder) {
this.stackId = builder.stackId;
this.stackName = builder.stackName;
this.templateDescription = builder.templateDescription;
this.creationTime = builder.creationTime;
this.lastUpdatedTime = builder.lastUpdatedTime;
this.deletionTime = builder.deletionTime;
this.stackStatus = builder.stackStatus;
this.stackStatusReason = builder.stackStatusReason;
this.parentId = builder.parentId;
this.rootId = builder.rootId;
this.driftInformation = builder.driftInformation;
}
/**
*
* Unique stack identifier.
*
*
* @return Unique stack identifier.
*/
public final String stackId() {
return stackId;
}
/**
*
* The name associated with the stack.
*
*
* @return The name associated with the stack.
*/
public final String stackName() {
return stackName;
}
/**
*
* The template description of the template used to create the stack.
*
*
* @return The template description of the template used to create the stack.
*/
public final String templateDescription() {
return templateDescription;
}
/**
*
* The time the stack was created.
*
*
* @return The time the stack was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* The time the stack was last updated. This field will only be returned if the stack has been updated at least
* once.
*
*
* @return The time the stack was last updated. This field will only be returned if the stack has been updated at
* least once.
*/
public final Instant lastUpdatedTime() {
return lastUpdatedTime;
}
/**
*
* The time the stack was deleted.
*
*
* @return The time the stack was deleted.
*/
public final Instant deletionTime() {
return deletionTime;
}
/**
*
* The current status of the stack.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #stackStatus} will
* return {@link StackStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stackStatusAsString}.
*
*
* @return The current status of the stack.
* @see StackStatus
*/
public final StackStatus stackStatus() {
return StackStatus.fromValue(stackStatus);
}
/**
*
* The current status of the stack.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #stackStatus} will
* return {@link StackStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stackStatusAsString}.
*
*
* @return The current status of the stack.
* @see StackStatus
*/
public final String stackStatusAsString() {
return stackStatus;
}
/**
*
* Success/Failure message associated with the stack status.
*
*
* @return Success/Failure message associated with the stack status.
*/
public final String stackStatusReason() {
return stackStatusReason;
}
/**
*
* For nested stacks--stacks created as resources for another stack--the stack ID of the direct parent of this
* stack. For the first level of nested stacks, the root stack is also the parent stack.
*
*
* For more information, see Embed stacks
* within other stacks using nested stacks in the CloudFormation User Guide.
*
*
* @return For nested stacks--stacks created as resources for another stack--the stack ID of the direct parent of
* this stack. For the first level of nested stacks, the root stack is also the parent stack.
*
* For more information, see Embed
* stacks within other stacks using nested stacks in the CloudFormation User Guide.
*/
public final String parentId() {
return parentId;
}
/**
*
* For nested stacks--stacks created as resources for another stack--the stack ID of the top-level stack to which
* the nested stack ultimately belongs.
*
*
* For more information, see Embed stacks
* within other stacks using nested stacks in the CloudFormation User Guide.
*
*
* @return For nested stacks--stacks created as resources for another stack--the stack ID of the top-level stack to
* which the nested stack ultimately belongs.
*
* For more information, see Embed
* stacks within other stacks using nested stacks in the CloudFormation User Guide.
*/
public final String rootId() {
return rootId;
}
/**
*
* Summarizes information about whether a stack's actual configuration differs, or has drifted, from its
* expected configuration, as defined in the stack template and any values specified as template parameters. For
* more information, see Detect unmanaged
* configuration changes to stacks and resources with drift detection.
*
*
* @return Summarizes information about whether a stack's actual configuration differs, or has drifted, from
* its expected configuration, as defined in the stack template and any values specified as template
* parameters. For more information, see Detect
* unmanaged configuration changes to stacks and resources with drift detection.
*/
public final StackDriftInformationSummary driftInformation() {
return driftInformation;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(stackId());
hashCode = 31 * hashCode + Objects.hashCode(stackName());
hashCode = 31 * hashCode + Objects.hashCode(templateDescription());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdatedTime());
hashCode = 31 * hashCode + Objects.hashCode(deletionTime());
hashCode = 31 * hashCode + Objects.hashCode(stackStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(stackStatusReason());
hashCode = 31 * hashCode + Objects.hashCode(parentId());
hashCode = 31 * hashCode + Objects.hashCode(rootId());
hashCode = 31 * hashCode + Objects.hashCode(driftInformation());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StackSummary)) {
return false;
}
StackSummary other = (StackSummary) obj;
return Objects.equals(stackId(), other.stackId()) && Objects.equals(stackName(), other.stackName())
&& Objects.equals(templateDescription(), other.templateDescription())
&& Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(lastUpdatedTime(), other.lastUpdatedTime())
&& Objects.equals(deletionTime(), other.deletionTime())
&& Objects.equals(stackStatusAsString(), other.stackStatusAsString())
&& Objects.equals(stackStatusReason(), other.stackStatusReason()) && Objects.equals(parentId(), other.parentId())
&& Objects.equals(rootId(), other.rootId()) && Objects.equals(driftInformation(), other.driftInformation());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StackSummary").add("StackId", stackId()).add("StackName", stackName())
.add("TemplateDescription", templateDescription()).add("CreationTime", creationTime())
.add("LastUpdatedTime", lastUpdatedTime()).add("DeletionTime", deletionTime())
.add("StackStatus", stackStatusAsString()).add("StackStatusReason", stackStatusReason())
.add("ParentId", parentId()).add("RootId", rootId()).add("DriftInformation", driftInformation()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackId":
return Optional.ofNullable(clazz.cast(stackId()));
case "StackName":
return Optional.ofNullable(clazz.cast(stackName()));
case "TemplateDescription":
return Optional.ofNullable(clazz.cast(templateDescription()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "LastUpdatedTime":
return Optional.ofNullable(clazz.cast(lastUpdatedTime()));
case "DeletionTime":
return Optional.ofNullable(clazz.cast(deletionTime()));
case "StackStatus":
return Optional.ofNullable(clazz.cast(stackStatusAsString()));
case "StackStatusReason":
return Optional.ofNullable(clazz.cast(stackStatusReason()));
case "ParentId":
return Optional.ofNullable(clazz.cast(parentId()));
case "RootId":
return Optional.ofNullable(clazz.cast(rootId()));
case "DriftInformation":
return Optional.ofNullable(clazz.cast(driftInformation()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function