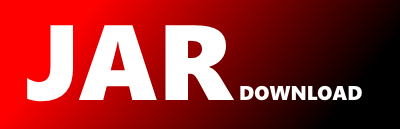
software.amazon.awssdk.services.cloudformation.model.StackInstanceSummary Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The structure that contains summary information about a stack instance.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class StackInstanceSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField STACK_SET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackSetId").getter(getter(StackInstanceSummary::stackSetId)).setter(setter(Builder::stackSetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackSetId").build()).build();
private static final SdkField REGION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Region")
.getter(getter(StackInstanceSummary::region)).setter(setter(Builder::region))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Region").build()).build();
private static final SdkField ACCOUNT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Account")
.getter(getter(StackInstanceSummary::account)).setter(setter(Builder::account))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Account").build()).build();
private static final SdkField STACK_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackId").getter(getter(StackInstanceSummary::stackId)).setter(setter(Builder::stackId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackId").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(StackInstanceSummary::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField STATUS_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StatusReason").getter(getter(StackInstanceSummary::statusReason)).setter(setter(Builder::statusReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusReason").build()).build();
private static final SdkField STACK_INSTANCE_STATUS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StackInstanceStatus")
.getter(getter(StackInstanceSummary::stackInstanceStatus)).setter(setter(Builder::stackInstanceStatus))
.constructor(StackInstanceComprehensiveStatus::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackInstanceStatus").build())
.build();
private static final SdkField ORGANIZATIONAL_UNIT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OrganizationalUnitId").getter(getter(StackInstanceSummary::organizationalUnitId))
.setter(setter(Builder::organizationalUnitId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OrganizationalUnitId").build())
.build();
private static final SdkField DRIFT_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DriftStatus").getter(getter(StackInstanceSummary::driftStatusAsString))
.setter(setter(Builder::driftStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DriftStatus").build()).build();
private static final SdkField LAST_DRIFT_CHECK_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastDriftCheckTimestamp").getter(getter(StackInstanceSummary::lastDriftCheckTimestamp))
.setter(setter(Builder::lastDriftCheckTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastDriftCheckTimestamp").build())
.build();
private static final SdkField LAST_OPERATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LastOperationId").getter(getter(StackInstanceSummary::lastOperationId))
.setter(setter(Builder::lastOperationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastOperationId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STACK_SET_ID_FIELD,
REGION_FIELD, ACCOUNT_FIELD, STACK_ID_FIELD, STATUS_FIELD, STATUS_REASON_FIELD, STACK_INSTANCE_STATUS_FIELD,
ORGANIZATIONAL_UNIT_ID_FIELD, DRIFT_STATUS_FIELD, LAST_DRIFT_CHECK_TIMESTAMP_FIELD, LAST_OPERATION_ID_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String stackSetId;
private final String region;
private final String account;
private final String stackId;
private final String status;
private final String statusReason;
private final StackInstanceComprehensiveStatus stackInstanceStatus;
private final String organizationalUnitId;
private final String driftStatus;
private final Instant lastDriftCheckTimestamp;
private final String lastOperationId;
private StackInstanceSummary(BuilderImpl builder) {
this.stackSetId = builder.stackSetId;
this.region = builder.region;
this.account = builder.account;
this.stackId = builder.stackId;
this.status = builder.status;
this.statusReason = builder.statusReason;
this.stackInstanceStatus = builder.stackInstanceStatus;
this.organizationalUnitId = builder.organizationalUnitId;
this.driftStatus = builder.driftStatus;
this.lastDriftCheckTimestamp = builder.lastDriftCheckTimestamp;
this.lastOperationId = builder.lastOperationId;
}
/**
*
* The name or unique ID of the stack set that the stack instance is associated with.
*
*
* @return The name or unique ID of the stack set that the stack instance is associated with.
*/
public final String stackSetId() {
return stackSetId;
}
/**
*
* The name of the Amazon Web Services Region that the stack instance is associated with.
*
*
* @return The name of the Amazon Web Services Region that the stack instance is associated with.
*/
public final String region() {
return region;
}
/**
*
* [Self-managed permissions] The name of the Amazon Web Services account that the stack instance is associated
* with.
*
*
* @return [Self-managed permissions] The name of the Amazon Web Services account that the stack instance is
* associated with.
*/
public final String account() {
return account;
}
/**
*
* The ID of the stack instance.
*
*
* @return The ID of the stack instance.
*/
public final String stackId() {
return stackId;
}
/**
*
* The status of the stack instance, in terms of its synchronization with its associated stack set.
*
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in an
* unstable state. Stacks in this state are excluded from further UpdateStackSet
operations. You might
* need to perform a DeleteStackInstances
operation, with RetainStacks
set to
* true
, to delete the stack instance, and then delete the stack manually. INOPERABLE
can
* be returned here when the cause is a failed import. If it's due to a failed import, the operation can be retried
* once the failures are fixed. To see if this is due to a failed import, call the DescribeStackInstance API
* operation, look at the DetailedStatus
member returned in the StackInstanceSummary
* member.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed or was
* stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link StackInstanceStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the stack instance, in terms of its synchronization with its associated stack set.
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in
* an unstable state. Stacks in this state are excluded from further UpdateStackSet
operations.
* You might need to perform a DeleteStackInstances
operation, with RetainStacks
* set to true
, to delete the stack instance, and then delete the stack manually.
* INOPERABLE
can be returned here when the cause is a failed import. If it's due to a failed
* import, the operation can be retried once the failures are fixed. To see if this is due to a failed
* import, call the DescribeStackInstance API operation, look at the DetailedStatus
* member returned in the StackInstanceSummary
member.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
* operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed
* or was stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
* @see StackInstanceStatus
*/
public final StackInstanceStatus status() {
return StackInstanceStatus.fromValue(status);
}
/**
*
* The status of the stack instance, in terms of its synchronization with its associated stack set.
*
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in an
* unstable state. Stacks in this state are excluded from further UpdateStackSet
operations. You might
* need to perform a DeleteStackInstances
operation, with RetainStacks
set to
* true
, to delete the stack instance, and then delete the stack manually. INOPERABLE
can
* be returned here when the cause is a failed import. If it's due to a failed import, the operation can be retried
* once the failures are fixed. To see if this is due to a failed import, call the DescribeStackInstance API
* operation, look at the DetailedStatus
member returned in the StackInstanceSummary
* member.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed or was
* stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link StackInstanceStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the stack instance, in terms of its synchronization with its associated stack set.
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in
* an unstable state. Stacks in this state are excluded from further UpdateStackSet
operations.
* You might need to perform a DeleteStackInstances
operation, with RetainStacks
* set to true
, to delete the stack instance, and then delete the stack manually.
* INOPERABLE
can be returned here when the cause is a failed import. If it's due to a failed
* import, the operation can be retried once the failures are fixed. To see if this is due to a failed
* import, call the DescribeStackInstance API operation, look at the DetailedStatus
* member returned in the StackInstanceSummary
member.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
* operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed
* or was stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
* @see StackInstanceStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The explanation for the specific status code assigned to this stack instance.
*
*
* @return The explanation for the specific status code assigned to this stack instance.
*/
public final String statusReason() {
return statusReason;
}
/**
*
* The detailed status of the stack instance.
*
*
* @return The detailed status of the stack instance.
*/
public final StackInstanceComprehensiveStatus stackInstanceStatus() {
return stackInstanceStatus;
}
/**
*
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified for
* DeploymentTargets.
*
*
* @return [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified
* for DeploymentTargets.
*/
public final String organizationalUnitId() {
return organizationalUnitId;
}
/**
*
* Status of the stack instance's actual configuration compared to the expected template and parameter configuration
* of the stack set to which it belongs.
*
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the stack set
* to which it belongs. A stack instance is considered to have drifted if one or more of the resources in the
* associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked if the stack instance differs from its expected stack set
* configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #driftStatus} will
* return {@link StackDriftStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #driftStatusAsString}.
*
*
* @return Status of the stack instance's actual configuration compared to the expected template and parameter
* configuration of the stack set to which it belongs.
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the
* stack set to which it belongs. A stack instance is considered to have drifted if one or more of the
* resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked if the stack instance differs from its expected
* stack set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set
* configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @see StackDriftStatus
*/
public final StackDriftStatus driftStatus() {
return StackDriftStatus.fromValue(driftStatus);
}
/**
*
* Status of the stack instance's actual configuration compared to the expected template and parameter configuration
* of the stack set to which it belongs.
*
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the stack set
* to which it belongs. A stack instance is considered to have drifted if one or more of the resources in the
* associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked if the stack instance differs from its expected stack set
* configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #driftStatus} will
* return {@link StackDriftStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #driftStatusAsString}.
*
*
* @return Status of the stack instance's actual configuration compared to the expected template and parameter
* configuration of the stack set to which it belongs.
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the
* stack set to which it belongs. A stack instance is considered to have drifted if one or more of the
* resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked if the stack instance differs from its expected
* stack set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set
* configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @see StackDriftStatus
*/
public final String driftStatusAsString() {
return driftStatus;
}
/**
*
* Most recent time when CloudFormation performed a drift detection operation on the stack instance. This value will
* be NULL
for any stack instance on which drift detection hasn't yet been performed.
*
*
* @return Most recent time when CloudFormation performed a drift detection operation on the stack instance. This
* value will be NULL
for any stack instance on which drift detection hasn't yet been
* performed.
*/
public final Instant lastDriftCheckTimestamp() {
return lastDriftCheckTimestamp;
}
/**
*
* The last unique ID of a StackSet operation performed on a stack instance.
*
*
* @return The last unique ID of a StackSet operation performed on a stack instance.
*/
public final String lastOperationId() {
return lastOperationId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(stackSetId());
hashCode = 31 * hashCode + Objects.hashCode(region());
hashCode = 31 * hashCode + Objects.hashCode(account());
hashCode = 31 * hashCode + Objects.hashCode(stackId());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusReason());
hashCode = 31 * hashCode + Objects.hashCode(stackInstanceStatus());
hashCode = 31 * hashCode + Objects.hashCode(organizationalUnitId());
hashCode = 31 * hashCode + Objects.hashCode(driftStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(lastDriftCheckTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(lastOperationId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StackInstanceSummary)) {
return false;
}
StackInstanceSummary other = (StackInstanceSummary) obj;
return Objects.equals(stackSetId(), other.stackSetId()) && Objects.equals(region(), other.region())
&& Objects.equals(account(), other.account()) && Objects.equals(stackId(), other.stackId())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(statusReason(), other.statusReason())
&& Objects.equals(stackInstanceStatus(), other.stackInstanceStatus())
&& Objects.equals(organizationalUnitId(), other.organizationalUnitId())
&& Objects.equals(driftStatusAsString(), other.driftStatusAsString())
&& Objects.equals(lastDriftCheckTimestamp(), other.lastDriftCheckTimestamp())
&& Objects.equals(lastOperationId(), other.lastOperationId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StackInstanceSummary").add("StackSetId", stackSetId()).add("Region", region())
.add("Account", account()).add("StackId", stackId()).add("Status", statusAsString())
.add("StatusReason", statusReason()).add("StackInstanceStatus", stackInstanceStatus())
.add("OrganizationalUnitId", organizationalUnitId()).add("DriftStatus", driftStatusAsString())
.add("LastDriftCheckTimestamp", lastDriftCheckTimestamp()).add("LastOperationId", lastOperationId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackSetId":
return Optional.ofNullable(clazz.cast(stackSetId()));
case "Region":
return Optional.ofNullable(clazz.cast(region()));
case "Account":
return Optional.ofNullable(clazz.cast(account()));
case "StackId":
return Optional.ofNullable(clazz.cast(stackId()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "StatusReason":
return Optional.ofNullable(clazz.cast(statusReason()));
case "StackInstanceStatus":
return Optional.ofNullable(clazz.cast(stackInstanceStatus()));
case "OrganizationalUnitId":
return Optional.ofNullable(clazz.cast(organizationalUnitId()));
case "DriftStatus":
return Optional.ofNullable(clazz.cast(driftStatusAsString()));
case "LastDriftCheckTimestamp":
return Optional.ofNullable(clazz.cast(lastDriftCheckTimestamp()));
case "LastOperationId":
return Optional.ofNullable(clazz.cast(lastOperationId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("StackSetId", STACK_SET_ID_FIELD);
map.put("Region", REGION_FIELD);
map.put("Account", ACCOUNT_FIELD);
map.put("StackId", STACK_ID_FIELD);
map.put("Status", STATUS_FIELD);
map.put("StatusReason", STATUS_REASON_FIELD);
map.put("StackInstanceStatus", STACK_INSTANCE_STATUS_FIELD);
map.put("OrganizationalUnitId", ORGANIZATIONAL_UNIT_ID_FIELD);
map.put("DriftStatus", DRIFT_STATUS_FIELD);
map.put("LastDriftCheckTimestamp", LAST_DRIFT_CHECK_TIMESTAMP_FIELD);
map.put("LastOperationId", LAST_OPERATION_ID_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function