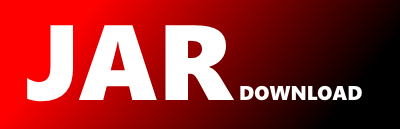
software.amazon.awssdk.services.cloudformation.model.StackSetOperationPreferences Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The user-specified preferences for how CloudFormation performs a stack set operation.
*
*
* For more information about maximum concurrent accounts and failure tolerance, see Stack
* set operation options.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class StackSetOperationPreferences implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField REGION_CONCURRENCY_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RegionConcurrencyType").getter(getter(StackSetOperationPreferences::regionConcurrencyTypeAsString))
.setter(setter(Builder::regionConcurrencyType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RegionConcurrencyType").build())
.build();
private static final SdkField> REGION_ORDER_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("RegionOrder")
.getter(getter(StackSetOperationPreferences::regionOrder))
.setter(setter(Builder::regionOrder))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RegionOrder").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField FAILURE_TOLERANCE_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("FailureToleranceCount").getter(getter(StackSetOperationPreferences::failureToleranceCount))
.setter(setter(Builder::failureToleranceCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureToleranceCount").build())
.build();
private static final SdkField FAILURE_TOLERANCE_PERCENTAGE_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("FailureTolerancePercentage")
.getter(getter(StackSetOperationPreferences::failureTolerancePercentage))
.setter(setter(Builder::failureTolerancePercentage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureTolerancePercentage").build())
.build();
private static final SdkField MAX_CONCURRENT_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxConcurrentCount").getter(getter(StackSetOperationPreferences::maxConcurrentCount))
.setter(setter(Builder::maxConcurrentCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxConcurrentCount").build())
.build();
private static final SdkField MAX_CONCURRENT_PERCENTAGE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxConcurrentPercentage").getter(getter(StackSetOperationPreferences::maxConcurrentPercentage))
.setter(setter(Builder::maxConcurrentPercentage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxConcurrentPercentage").build())
.build();
private static final SdkField CONCURRENCY_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ConcurrencyMode").getter(getter(StackSetOperationPreferences::concurrencyModeAsString))
.setter(setter(Builder::concurrencyMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConcurrencyMode").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REGION_CONCURRENCY_TYPE_FIELD,
REGION_ORDER_FIELD, FAILURE_TOLERANCE_COUNT_FIELD, FAILURE_TOLERANCE_PERCENTAGE_FIELD, MAX_CONCURRENT_COUNT_FIELD,
MAX_CONCURRENT_PERCENTAGE_FIELD, CONCURRENCY_MODE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("RegionConcurrencyType", REGION_CONCURRENCY_TYPE_FIELD);
put("RegionOrder", REGION_ORDER_FIELD);
put("FailureToleranceCount", FAILURE_TOLERANCE_COUNT_FIELD);
put("FailureTolerancePercentage", FAILURE_TOLERANCE_PERCENTAGE_FIELD);
put("MaxConcurrentCount", MAX_CONCURRENT_COUNT_FIELD);
put("MaxConcurrentPercentage", MAX_CONCURRENT_PERCENTAGE_FIELD);
put("ConcurrencyMode", CONCURRENCY_MODE_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String regionConcurrencyType;
private final List regionOrder;
private final Integer failureToleranceCount;
private final Integer failureTolerancePercentage;
private final Integer maxConcurrentCount;
private final Integer maxConcurrentPercentage;
private final String concurrencyMode;
private StackSetOperationPreferences(BuilderImpl builder) {
this.regionConcurrencyType = builder.regionConcurrencyType;
this.regionOrder = builder.regionOrder;
this.failureToleranceCount = builder.failureToleranceCount;
this.failureTolerancePercentage = builder.failureTolerancePercentage;
this.maxConcurrentCount = builder.maxConcurrentCount;
this.maxConcurrentPercentage = builder.maxConcurrentPercentage;
this.concurrencyMode = builder.concurrencyMode;
}
/**
*
* The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at a time.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #regionConcurrencyType} will return {@link RegionConcurrencyType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #regionConcurrencyTypeAsString}.
*
*
* @return The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at
* a time.
* @see RegionConcurrencyType
*/
public final RegionConcurrencyType regionConcurrencyType() {
return RegionConcurrencyType.fromValue(regionConcurrencyType);
}
/**
*
* The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at a time.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #regionConcurrencyType} will return {@link RegionConcurrencyType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #regionConcurrencyTypeAsString}.
*
*
* @return The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at
* a time.
* @see RegionConcurrencyType
*/
public final String regionConcurrencyTypeAsString() {
return regionConcurrencyType;
}
/**
* For responses, this returns true if the service returned a value for the RegionOrder property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasRegionOrder() {
return regionOrder != null && !(regionOrder instanceof SdkAutoConstructList);
}
/**
*
* The order of the Regions where you want to perform the stack operation.
*
*
*
* RegionOrder
isn't followed if AutoDeployment
is enabled.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRegionOrder} method.
*
*
* @return The order of the Regions where you want to perform the stack operation.
*
* RegionOrder
isn't followed if AutoDeployment
is enabled.
*
*/
public final List regionOrder() {
return regionOrder;
}
/**
*
* The number of accounts, per Region, for which this operation can fail before CloudFormation stops the operation
* in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the operation in any
* subsequent Regions.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
(but not both).
*
*
* By default, 0
is specified.
*
*
* @return The number of accounts, per Region, for which this operation can fail before CloudFormation stops the
* operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the
* operation in any subsequent Regions.
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
(but not both).
*
*
* By default, 0
is specified.
*/
public final Integer failureToleranceCount() {
return failureToleranceCount;
}
/**
*
* The percentage of accounts, per Region, for which this stack operation can fail before CloudFormation stops the
* operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the operation
* in any subsequent Regions.
*
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to
* the next whole number.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
, but not both.
*
*
* By default, 0
is specified.
*
*
* @return The percentage of accounts, per Region, for which this stack operation can fail before CloudFormation
* stops the operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't
* attempt the operation in any subsequent Regions.
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds
* down to the next whole number.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
, but not both.
*
*
* By default, 0
is specified.
*/
public final Integer failureTolerancePercentage() {
return failureTolerancePercentage;
}
/**
*
* The maximum number of accounts in which to perform this operation at one time. This can depend on the value of
* FailureToleranceCount
depending on your ConcurrencyMode
.
* MaxConcurrentCount
is at most one more than the FailureToleranceCount
if you're using
* STRICT_FAILURE_TOLERANCE
.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under certain
* circumstances the actual number of accounts acted upon concurrently may be lower due to service throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or MaxConcurrentPercentage
, but
* not both.
*
*
* By default, 1
is specified.
*
*
* @return The maximum number of accounts in which to perform this operation at one time. This can depend on the
* value of FailureToleranceCount
depending on your ConcurrencyMode
.
* MaxConcurrentCount
is at most one more than the FailureToleranceCount
if you're
* using STRICT_FAILURE_TOLERANCE
.
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under
* certain circumstances the actual number of accounts acted upon concurrently may be lower due to service
* throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or
* MaxConcurrentPercentage
, but not both.
*
*
* By default, 1
is specified.
*/
public final Integer maxConcurrentCount() {
return maxConcurrentCount;
}
/**
*
* The maximum percentage of accounts in which to perform this operation at one time.
*
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to the next
* whole number. This is true except in cases where rounding down would result is zero. In this case, CloudFormation
* sets the number as one instead.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under certain
* circumstances the actual number of accounts acted upon concurrently may be lower due to service throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or MaxConcurrentPercentage
, but
* not both.
*
*
* By default, 1
is specified.
*
*
* @return The maximum percentage of accounts in which to perform this operation at one time.
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to
* the next whole number. This is true except in cases where rounding down would result is zero. In this
* case, CloudFormation sets the number as one instead.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under
* certain circumstances the actual number of accounts acted upon concurrently may be lower due to service
* throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or
* MaxConcurrentPercentage
, but not both.
*
*
* By default, 1
is specified.
*/
public final Integer maxConcurrentPercentage() {
return maxConcurrentPercentage;
}
/**
*
* Specifies how the concurrency level behaves during the operation execution.
*
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the number
* of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial actual
* concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the value of
* FailureToleranceCount
+1. The actual concurrency is then reduced proportionally by the number of
* failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the actual
* concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number of
* failures.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #concurrencyMode}
* will return {@link ConcurrencyMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #concurrencyModeAsString}.
*
*
* @return Specifies how the concurrency level behaves during the operation execution.
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the
* number of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial
* actual concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the
* value of FailureToleranceCount
+1. The actual concurrency is then reduced proportionally by
* the number of failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the
* actual concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number
* of failures.
*
*
* @see ConcurrencyMode
*/
public final ConcurrencyMode concurrencyMode() {
return ConcurrencyMode.fromValue(concurrencyMode);
}
/**
*
* Specifies how the concurrency level behaves during the operation execution.
*
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the number
* of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial actual
* concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the value of
* FailureToleranceCount
+1. The actual concurrency is then reduced proportionally by the number of
* failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the actual
* concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number of
* failures.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #concurrencyMode}
* will return {@link ConcurrencyMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #concurrencyModeAsString}.
*
*
* @return Specifies how the concurrency level behaves during the operation execution.
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the
* number of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial
* actual concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the
* value of FailureToleranceCount
+1. The actual concurrency is then reduced proportionally by
* the number of failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the
* actual concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number
* of failures.
*
*
* @see ConcurrencyMode
*/
public final String concurrencyModeAsString() {
return concurrencyMode;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(regionConcurrencyTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasRegionOrder() ? regionOrder() : null);
hashCode = 31 * hashCode + Objects.hashCode(failureToleranceCount());
hashCode = 31 * hashCode + Objects.hashCode(failureTolerancePercentage());
hashCode = 31 * hashCode + Objects.hashCode(maxConcurrentCount());
hashCode = 31 * hashCode + Objects.hashCode(maxConcurrentPercentage());
hashCode = 31 * hashCode + Objects.hashCode(concurrencyModeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StackSetOperationPreferences)) {
return false;
}
StackSetOperationPreferences other = (StackSetOperationPreferences) obj;
return Objects.equals(regionConcurrencyTypeAsString(), other.regionConcurrencyTypeAsString())
&& hasRegionOrder() == other.hasRegionOrder() && Objects.equals(regionOrder(), other.regionOrder())
&& Objects.equals(failureToleranceCount(), other.failureToleranceCount())
&& Objects.equals(failureTolerancePercentage(), other.failureTolerancePercentage())
&& Objects.equals(maxConcurrentCount(), other.maxConcurrentCount())
&& Objects.equals(maxConcurrentPercentage(), other.maxConcurrentPercentage())
&& Objects.equals(concurrencyModeAsString(), other.concurrencyModeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StackSetOperationPreferences").add("RegionConcurrencyType", regionConcurrencyTypeAsString())
.add("RegionOrder", hasRegionOrder() ? regionOrder() : null)
.add("FailureToleranceCount", failureToleranceCount())
.add("FailureTolerancePercentage", failureTolerancePercentage()).add("MaxConcurrentCount", maxConcurrentCount())
.add("MaxConcurrentPercentage", maxConcurrentPercentage()).add("ConcurrencyMode", concurrencyModeAsString())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RegionConcurrencyType":
return Optional.ofNullable(clazz.cast(regionConcurrencyTypeAsString()));
case "RegionOrder":
return Optional.ofNullable(clazz.cast(regionOrder()));
case "FailureToleranceCount":
return Optional.ofNullable(clazz.cast(failureToleranceCount()));
case "FailureTolerancePercentage":
return Optional.ofNullable(clazz.cast(failureTolerancePercentage()));
case "MaxConcurrentCount":
return Optional.ofNullable(clazz.cast(maxConcurrentCount()));
case "MaxConcurrentPercentage":
return Optional.ofNullable(clazz.cast(maxConcurrentPercentage()));
case "ConcurrencyMode":
return Optional.ofNullable(clazz.cast(concurrencyModeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function