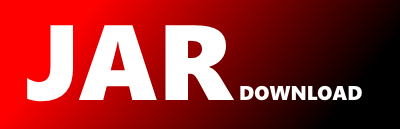
software.amazon.awssdk.services.cloudformation.model.StackResourceDrift Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the drift information for a resource that has been checked for drift. This includes actual and expected
* property values for resources in which AWS CloudFormation has detected drift. Only resource properties explicitly
* defined in the stack template are checked for drift. For more information, see Detecting Unregulated
* Configuration Changes to Stacks and Resources.
*
*
* Resources that do not currently support drift detection cannot be checked. For a list of resources that support drift
* detection, see Resources that Support Drift Detection.
*
*
* Use DetectStackResourceDrift to detect drift on individual resources, or DetectStackDrift to detect
* drift on all resources in a given stack that support drift detection.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class StackResourceDrift implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField STACK_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(StackResourceDrift::stackId)).setter(setter(Builder::stackId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackId").build()).build();
private static final SdkField LOGICAL_RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(StackResourceDrift::logicalResourceId)).setter(setter(Builder::logicalResourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogicalResourceId").build()).build();
private static final SdkField PHYSICAL_RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(StackResourceDrift::physicalResourceId)).setter(setter(Builder::physicalResourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PhysicalResourceId").build())
.build();
private static final SdkField> PHYSICAL_RESOURCE_ID_CONTEXT_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(StackResourceDrift::physicalResourceIdContext))
.setter(setter(Builder::physicalResourceIdContext))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PhysicalResourceIdContext").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PhysicalResourceIdContextKeyValuePair::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(StackResourceDrift::resourceType)).setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceType").build()).build();
private static final SdkField EXPECTED_PROPERTIES_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(StackResourceDrift::expectedProperties)).setter(setter(Builder::expectedProperties))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedProperties").build())
.build();
private static final SdkField ACTUAL_PROPERTIES_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(StackResourceDrift::actualProperties)).setter(setter(Builder::actualProperties))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActualProperties").build()).build();
private static final SdkField> PROPERTY_DIFFERENCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(StackResourceDrift::propertyDifferences))
.setter(setter(Builder::propertyDifferences))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PropertyDifferences").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PropertyDifference::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField STACK_RESOURCE_DRIFT_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(StackResourceDrift::stackResourceDriftStatusAsString))
.setter(setter(Builder::stackResourceDriftStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackResourceDriftStatus").build())
.build();
private static final SdkField TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(StackResourceDrift::timestamp)).setter(setter(Builder::timestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Timestamp").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STACK_ID_FIELD,
LOGICAL_RESOURCE_ID_FIELD, PHYSICAL_RESOURCE_ID_FIELD, PHYSICAL_RESOURCE_ID_CONTEXT_FIELD, RESOURCE_TYPE_FIELD,
EXPECTED_PROPERTIES_FIELD, ACTUAL_PROPERTIES_FIELD, PROPERTY_DIFFERENCES_FIELD, STACK_RESOURCE_DRIFT_STATUS_FIELD,
TIMESTAMP_FIELD));
private static final long serialVersionUID = 1L;
private final String stackId;
private final String logicalResourceId;
private final String physicalResourceId;
private final List physicalResourceIdContext;
private final String resourceType;
private final String expectedProperties;
private final String actualProperties;
private final List propertyDifferences;
private final String stackResourceDriftStatus;
private final Instant timestamp;
private StackResourceDrift(BuilderImpl builder) {
this.stackId = builder.stackId;
this.logicalResourceId = builder.logicalResourceId;
this.physicalResourceId = builder.physicalResourceId;
this.physicalResourceIdContext = builder.physicalResourceIdContext;
this.resourceType = builder.resourceType;
this.expectedProperties = builder.expectedProperties;
this.actualProperties = builder.actualProperties;
this.propertyDifferences = builder.propertyDifferences;
this.stackResourceDriftStatus = builder.stackResourceDriftStatus;
this.timestamp = builder.timestamp;
}
/**
*
* The ID of the stack.
*
*
* @return The ID of the stack.
*/
public String stackId() {
return stackId;
}
/**
*
* The logical name of the resource specified in the template.
*
*
* @return The logical name of the resource specified in the template.
*/
public String logicalResourceId() {
return logicalResourceId;
}
/**
*
* The name or unique identifier that corresponds to a physical instance ID of a resource supported by AWS
* CloudFormation.
*
*
* @return The name or unique identifier that corresponds to a physical instance ID of a resource supported by AWS
* CloudFormation.
*/
public String physicalResourceId() {
return physicalResourceId;
}
/**
*
* Context information that enables AWS CloudFormation to uniquely identify a resource. AWS CloudFormation uses
* context key-value pairs in cases where a resource's logical and physical IDs are not enough to uniquely identify
* that resource. Each context key-value pair specifies a unique resource that contains the targeted resource.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Context information that enables AWS CloudFormation to uniquely identify a resource. AWS CloudFormation
* uses context key-value pairs in cases where a resource's logical and physical IDs are not enough to
* uniquely identify that resource. Each context key-value pair specifies a unique resource that contains
* the targeted resource.
*/
public List physicalResourceIdContext() {
return physicalResourceIdContext;
}
/**
*
* The type of the resource.
*
*
* @return The type of the resource.
*/
public String resourceType() {
return resourceType;
}
/**
*
* A JSON structure containing the expected property values of the stack resource, as defined in the stack template
* and any values specified as template parameters.
*
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not be
* present.
*
*
* @return A JSON structure containing the expected property values of the stack resource, as defined in the stack
* template and any values specified as template parameters.
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will
* not be present.
*/
public String expectedProperties() {
return expectedProperties;
}
/**
*
* A JSON structure containing the actual property values of the stack resource.
*
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not be
* present.
*
*
* @return A JSON structure containing the actual property values of the stack resource.
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will
* not be present.
*/
public String actualProperties() {
return actualProperties;
}
/**
*
* A collection of the resource properties whose actual values differ from their expected values. These will be
* present only for resources whose StackResourceDriftStatus
is MODIFIED
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A collection of the resource properties whose actual values differ from their expected values. These will
* be present only for resources whose StackResourceDriftStatus
is MODIFIED
.
*/
public List propertyDifferences() {
return propertyDifferences;
}
/**
*
* Status of the resource's actual configuration compared to its expected configuration
*
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource has been
* deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in the stack
* template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #stackResourceDriftStatus} will return {@link StackResourceDriftStatus#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #stackResourceDriftStatusAsString}.
*
*
* @return Status of the resource's actual configuration compared to its expected configuration
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource
* has been deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in
* the stack template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
* @see StackResourceDriftStatus
*/
public StackResourceDriftStatus stackResourceDriftStatus() {
return StackResourceDriftStatus.fromValue(stackResourceDriftStatus);
}
/**
*
* Status of the resource's actual configuration compared to its expected configuration
*
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource has been
* deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in the stack
* template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #stackResourceDriftStatus} will return {@link StackResourceDriftStatus#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #stackResourceDriftStatusAsString}.
*
*
* @return Status of the resource's actual configuration compared to its expected configuration
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource
* has been deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in
* the stack template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
* @see StackResourceDriftStatus
*/
public String stackResourceDriftStatusAsString() {
return stackResourceDriftStatus;
}
/**
*
* Time at which AWS CloudFormation performed drift detection on the stack resource.
*
*
* @return Time at which AWS CloudFormation performed drift detection on the stack resource.
*/
public Instant timestamp() {
return timestamp;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(stackId());
hashCode = 31 * hashCode + Objects.hashCode(logicalResourceId());
hashCode = 31 * hashCode + Objects.hashCode(physicalResourceId());
hashCode = 31 * hashCode + Objects.hashCode(physicalResourceIdContext());
hashCode = 31 * hashCode + Objects.hashCode(resourceType());
hashCode = 31 * hashCode + Objects.hashCode(expectedProperties());
hashCode = 31 * hashCode + Objects.hashCode(actualProperties());
hashCode = 31 * hashCode + Objects.hashCode(propertyDifferences());
hashCode = 31 * hashCode + Objects.hashCode(stackResourceDriftStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(timestamp());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StackResourceDrift)) {
return false;
}
StackResourceDrift other = (StackResourceDrift) obj;
return Objects.equals(stackId(), other.stackId()) && Objects.equals(logicalResourceId(), other.logicalResourceId())
&& Objects.equals(physicalResourceId(), other.physicalResourceId())
&& Objects.equals(physicalResourceIdContext(), other.physicalResourceIdContext())
&& Objects.equals(resourceType(), other.resourceType())
&& Objects.equals(expectedProperties(), other.expectedProperties())
&& Objects.equals(actualProperties(), other.actualProperties())
&& Objects.equals(propertyDifferences(), other.propertyDifferences())
&& Objects.equals(stackResourceDriftStatusAsString(), other.stackResourceDriftStatusAsString())
&& Objects.equals(timestamp(), other.timestamp());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("StackResourceDrift").add("StackId", stackId()).add("LogicalResourceId", logicalResourceId())
.add("PhysicalResourceId", physicalResourceId()).add("PhysicalResourceIdContext", physicalResourceIdContext())
.add("ResourceType", resourceType()).add("ExpectedProperties", expectedProperties())
.add("ActualProperties", actualProperties()).add("PropertyDifferences", propertyDifferences())
.add("StackResourceDriftStatus", stackResourceDriftStatusAsString()).add("Timestamp", timestamp()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StackId":
return Optional.ofNullable(clazz.cast(stackId()));
case "LogicalResourceId":
return Optional.ofNullable(clazz.cast(logicalResourceId()));
case "PhysicalResourceId":
return Optional.ofNullable(clazz.cast(physicalResourceId()));
case "PhysicalResourceIdContext":
return Optional.ofNullable(clazz.cast(physicalResourceIdContext()));
case "ResourceType":
return Optional.ofNullable(clazz.cast(resourceType()));
case "ExpectedProperties":
return Optional.ofNullable(clazz.cast(expectedProperties()));
case "ActualProperties":
return Optional.ofNullable(clazz.cast(actualProperties()));
case "PropertyDifferences":
return Optional.ofNullable(clazz.cast(propertyDifferences()));
case "StackResourceDriftStatus":
return Optional.ofNullable(clazz.cast(stackResourceDriftStatusAsString()));
case "Timestamp":
return Optional.ofNullable(clazz.cast(timestamp()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function