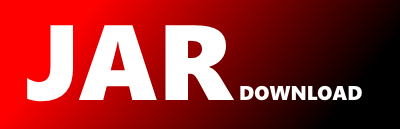
software.amazon.awssdk.services.cloudformation.model.ResourceChange Maven / Gradle / Ivy
Show all versions of cloudformation Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudformation.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The ResourceChange
structure describes the resource and the action that AWS CloudFormation will perform
* on it if you execute this change set.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ResourceChange implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ACTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ResourceChange::actionAsString)).setter(setter(Builder::action))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Action").build()).build();
private static final SdkField LOGICAL_RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ResourceChange::logicalResourceId)).setter(setter(Builder::logicalResourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogicalResourceId").build()).build();
private static final SdkField PHYSICAL_RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ResourceChange::physicalResourceId)).setter(setter(Builder::physicalResourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PhysicalResourceId").build())
.build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ResourceChange::resourceType)).setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceType").build()).build();
private static final SdkField REPLACEMENT_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ResourceChange::replacementAsString)).setter(setter(Builder::replacement))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Replacement").build()).build();
private static final SdkField> SCOPE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(ResourceChange::scopeAsStrings))
.setter(setter(Builder::scopeWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Scope").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> DETAILS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(ResourceChange::details))
.setter(setter(Builder::details))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Details").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ResourceChangeDetail::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACTION_FIELD,
LOGICAL_RESOURCE_ID_FIELD, PHYSICAL_RESOURCE_ID_FIELD, RESOURCE_TYPE_FIELD, REPLACEMENT_FIELD, SCOPE_FIELD,
DETAILS_FIELD));
private static final long serialVersionUID = 1L;
private final String action;
private final String logicalResourceId;
private final String physicalResourceId;
private final String resourceType;
private final String replacement;
private final List scope;
private final List details;
private ResourceChange(BuilderImpl builder) {
this.action = builder.action;
this.logicalResourceId = builder.logicalResourceId;
this.physicalResourceId = builder.physicalResourceId;
this.resourceType = builder.resourceType;
this.replacement = builder.replacement;
this.scope = builder.scope;
this.details = builder.details;
}
/**
*
* The action that AWS CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), or Remove
(deletes a resource).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #action} will
* return {@link ChangeAction#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #actionAsString}.
*
*
* @return The action that AWS CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), or Remove
(deletes a resource).
* @see ChangeAction
*/
public ChangeAction action() {
return ChangeAction.fromValue(action);
}
/**
*
* The action that AWS CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), or Remove
(deletes a resource).
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #action} will
* return {@link ChangeAction#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #actionAsString}.
*
*
* @return The action that AWS CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), or Remove
(deletes a resource).
* @see ChangeAction
*/
public String actionAsString() {
return action;
}
/**
*
* The resource's logical ID, which is defined in the stack's template.
*
*
* @return The resource's logical ID, which is defined in the stack's template.
*/
public String logicalResourceId() {
return logicalResourceId;
}
/**
*
* The resource's physical ID (resource name). Resources that you are adding don't have physical IDs because they
* haven't been created.
*
*
* @return The resource's physical ID (resource name). Resources that you are adding don't have physical IDs because
* they haven't been created.
*/
public String physicalResourceId() {
return physicalResourceId;
}
/**
*
* The type of AWS CloudFormation resource, such as AWS::S3::Bucket
.
*
*
* @return The type of AWS CloudFormation resource, such as AWS::S3::Bucket
.
*/
public String resourceType() {
return resourceType;
}
/**
*
* For the Modify
action, indicates whether AWS CloudFormation will replace the resource by creating a
* new one and deleting the old one. This value depends on the value of the RequiresRecreation
property
* in the ResourceTargetDefinition
structure. For example, if the RequiresRecreation
field
* is Always
and the Evaluation
field is Static
, Replacement
is
* True
. If the RequiresRecreation
field is Always
and the
* Evaluation
field is Dynamic
, Replacement
is Conditionally
.
*
*
* If you have multiple changes with different RequiresRecreation
values, the Replacement
* value depends on the change with the most impact. A RequiresRecreation
value of Always
* has the most impact, followed by Conditionally
, and then Never
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #replacement} will
* return {@link Replacement#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #replacementAsString}.
*
*
* @return For the Modify
action, indicates whether AWS CloudFormation will replace the resource by
* creating a new one and deleting the old one. This value depends on the value of the
* RequiresRecreation
property in the ResourceTargetDefinition
structure. For
* example, if the RequiresRecreation
field is Always
and the
* Evaluation
field is Static
, Replacement
is True
. If
* the RequiresRecreation
field is Always
and the Evaluation
field is
* Dynamic
, Replacement
is Conditionally
.
*
* If you have multiple changes with different RequiresRecreation
values, the
* Replacement
value depends on the change with the most impact. A
* RequiresRecreation
value of Always
has the most impact, followed by
* Conditionally
, and then Never
.
* @see Replacement
*/
public Replacement replacement() {
return Replacement.fromValue(replacement);
}
/**
*
* For the Modify
action, indicates whether AWS CloudFormation will replace the resource by creating a
* new one and deleting the old one. This value depends on the value of the RequiresRecreation
property
* in the ResourceTargetDefinition
structure. For example, if the RequiresRecreation
field
* is Always
and the Evaluation
field is Static
, Replacement
is
* True
. If the RequiresRecreation
field is Always
and the
* Evaluation
field is Dynamic
, Replacement
is Conditionally
.
*
*
* If you have multiple changes with different RequiresRecreation
values, the Replacement
* value depends on the change with the most impact. A RequiresRecreation
value of Always
* has the most impact, followed by Conditionally
, and then Never
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #replacement} will
* return {@link Replacement#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #replacementAsString}.
*
*
* @return For the Modify
action, indicates whether AWS CloudFormation will replace the resource by
* creating a new one and deleting the old one. This value depends on the value of the
* RequiresRecreation
property in the ResourceTargetDefinition
structure. For
* example, if the RequiresRecreation
field is Always
and the
* Evaluation
field is Static
, Replacement
is True
. If
* the RequiresRecreation
field is Always
and the Evaluation
field is
* Dynamic
, Replacement
is Conditionally
.
*
* If you have multiple changes with different RequiresRecreation
values, the
* Replacement
value depends on the change with the most impact. A
* RequiresRecreation
value of Always
has the most impact, followed by
* Conditionally
, and then Never
.
* @see Replacement
*/
public String replacementAsString() {
return replacement;
}
/**
*
* For the Modify
action, indicates which resource attribute is triggering this update, such as a
* change in the resource attribute's Metadata
, Properties
, or Tags
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return For the Modify
action, indicates which resource attribute is triggering this update, such as
* a change in the resource attribute's Metadata
, Properties
, or Tags
* .
*/
public List scope() {
return ScopeCopier.copyStringToEnum(scope);
}
/**
*
* For the Modify
action, indicates which resource attribute is triggering this update, such as a
* change in the resource attribute's Metadata
, Properties
, or Tags
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return For the Modify
action, indicates which resource attribute is triggering this update, such as
* a change in the resource attribute's Metadata
, Properties
, or Tags
* .
*/
public List scopeAsStrings() {
return scope;
}
/**
*
* For the Modify
action, a list of ResourceChangeDetail
structures that describes the
* changes that AWS CloudFormation will make to the resource.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return For the Modify
action, a list of ResourceChangeDetail
structures that describes
* the changes that AWS CloudFormation will make to the resource.
*/
public List details() {
return details;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(actionAsString());
hashCode = 31 * hashCode + Objects.hashCode(logicalResourceId());
hashCode = 31 * hashCode + Objects.hashCode(physicalResourceId());
hashCode = 31 * hashCode + Objects.hashCode(resourceType());
hashCode = 31 * hashCode + Objects.hashCode(replacementAsString());
hashCode = 31 * hashCode + Objects.hashCode(scopeAsStrings());
hashCode = 31 * hashCode + Objects.hashCode(details());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ResourceChange)) {
return false;
}
ResourceChange other = (ResourceChange) obj;
return Objects.equals(actionAsString(), other.actionAsString())
&& Objects.equals(logicalResourceId(), other.logicalResourceId())
&& Objects.equals(physicalResourceId(), other.physicalResourceId())
&& Objects.equals(resourceType(), other.resourceType())
&& Objects.equals(replacementAsString(), other.replacementAsString())
&& Objects.equals(scopeAsStrings(), other.scopeAsStrings()) && Objects.equals(details(), other.details());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ResourceChange").add("Action", actionAsString()).add("LogicalResourceId", logicalResourceId())
.add("PhysicalResourceId", physicalResourceId()).add("ResourceType", resourceType())
.add("Replacement", replacementAsString()).add("Scope", scopeAsStrings()).add("Details", details()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Action":
return Optional.ofNullable(clazz.cast(actionAsString()));
case "LogicalResourceId":
return Optional.ofNullable(clazz.cast(logicalResourceId()));
case "PhysicalResourceId":
return Optional.ofNullable(clazz.cast(physicalResourceId()));
case "ResourceType":
return Optional.ofNullable(clazz.cast(resourceType()));
case "Replacement":
return Optional.ofNullable(clazz.cast(replacementAsString()));
case "Scope":
return Optional.ofNullable(clazz.cast(scopeAsStrings()));
case "Details":
return Optional.ofNullable(clazz.cast(details()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function