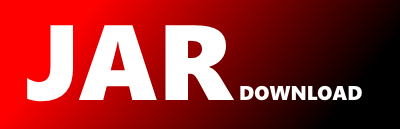
software.amazon.awssdk.services.cloudfrontkeyvaluestore.DefaultCloudFrontKeyValueStoreClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudfrontkeyvaluestore Show documentation
Show all versions of cloudfrontkeyvaluestore Show documentation
The AWS Java SDK for Cloud Front Key Value Store module holds the client classes that are used for
communicating with Cloud Front Key Value Store.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudfrontkeyvaluestore;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.internal.CloudFrontKeyValueStoreServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.AccessDeniedException;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.CloudFrontKeyValueStoreException;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.ConflictException;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.DeleteKeyRequest;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.DeleteKeyResponse;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.DescribeKeyValueStoreRequest;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.DescribeKeyValueStoreResponse;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.GetKeyRequest;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.GetKeyResponse;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.InternalServerException;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.ListKeysRequest;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.ListKeysResponse;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.PutKeyRequest;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.PutKeyResponse;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.ResourceNotFoundException;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.UpdateKeysRequest;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.UpdateKeysResponse;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.model.ValidationException;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.transform.DeleteKeyRequestMarshaller;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.transform.DescribeKeyValueStoreRequestMarshaller;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.transform.GetKeyRequestMarshaller;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.transform.ListKeysRequestMarshaller;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.transform.PutKeyRequestMarshaller;
import software.amazon.awssdk.services.cloudfrontkeyvaluestore.transform.UpdateKeysRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link CloudFrontKeyValueStoreClient}.
*
* @see CloudFrontKeyValueStoreClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultCloudFrontKeyValueStoreClient implements CloudFrontKeyValueStoreClient {
private static final Logger log = Logger.loggerFor(DefaultCloudFrontKeyValueStoreClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.REST_JSON).build();
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultCloudFrontKeyValueStoreClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Deletes the key value pair specified by the key.
*
*
* @param deleteKeyRequest
* @return Result of the DeleteKey operation returned by the service.
* @throws ConflictException
* Resource is not in expected state.
* @throws ValidationException
* Validation failed.
* @throws InternalServerException
* Internal server error.
* @throws ServiceQuotaExceededException
* Limit exceeded.
* @throws ResourceNotFoundException
* Resource was not found.
* @throws AccessDeniedException
* Access denied.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFrontKeyValueStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFrontKeyValueStoreClient.DeleteKey
* @see AWS API Documentation
*/
@Override
public DeleteKeyResponse deleteKey(DeleteKeyRequest deleteKeyRequest) throws ConflictException, ValidationException,
InternalServerException, ServiceQuotaExceededException, ResourceNotFoundException, AccessDeniedException,
AwsServiceException, SdkClientException, CloudFrontKeyValueStoreException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteKeyRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFront KeyValueStore");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteKey");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteKey").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(deleteKeyRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteKeyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns metadata information about Key Value Store.
*
*
* @param describeKeyValueStoreRequest
* @return Result of the DescribeKeyValueStore operation returned by the service.
* @throws ConflictException
* Resource is not in expected state.
* @throws InternalServerException
* Internal server error.
* @throws ResourceNotFoundException
* Resource was not found.
* @throws AccessDeniedException
* Access denied.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFrontKeyValueStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFrontKeyValueStoreClient.DescribeKeyValueStore
* @see AWS API Documentation
*/
@Override
public DescribeKeyValueStoreResponse describeKeyValueStore(DescribeKeyValueStoreRequest describeKeyValueStoreRequest)
throws ConflictException, InternalServerException, ResourceNotFoundException, AccessDeniedException,
AwsServiceException, SdkClientException, CloudFrontKeyValueStoreException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeKeyValueStoreResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeKeyValueStoreRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeKeyValueStoreRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFront KeyValueStore");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeKeyValueStore");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeKeyValueStore").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeKeyValueStoreRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeKeyValueStoreRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a key value pair.
*
*
* @param getKeyRequest
* @return Result of the GetKey operation returned by the service.
* @throws ConflictException
* Resource is not in expected state.
* @throws InternalServerException
* Internal server error.
* @throws ResourceNotFoundException
* Resource was not found.
* @throws AccessDeniedException
* Access denied.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFrontKeyValueStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFrontKeyValueStoreClient.GetKey
* @see AWS API Documentation
*/
@Override
public GetKeyResponse getKey(GetKeyRequest getKeyRequest) throws ConflictException, InternalServerException,
ResourceNotFoundException, AccessDeniedException, AwsServiceException, SdkClientException,
CloudFrontKeyValueStoreException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getKeyRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFront KeyValueStore");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetKey");
return clientHandler.execute(new ClientExecutionParams().withOperationName("GetKey")
.withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(getKeyRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetKeyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of key value pairs.
*
*
* @param listKeysRequest
* @return Result of the ListKeys operation returned by the service.
* @throws ConflictException
* Resource is not in expected state.
* @throws ValidationException
* Validation failed.
* @throws InternalServerException
* Internal server error.
* @throws ResourceNotFoundException
* Resource was not found.
* @throws AccessDeniedException
* Access denied.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFrontKeyValueStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFrontKeyValueStoreClient.ListKeys
* @see AWS API Documentation
*/
@Override
public ListKeysResponse listKeys(ListKeysRequest listKeysRequest) throws ConflictException, ValidationException,
InternalServerException, ResourceNotFoundException, AccessDeniedException, AwsServiceException, SdkClientException,
CloudFrontKeyValueStoreException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListKeysResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listKeysRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listKeysRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFront KeyValueStore");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListKeys");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListKeys").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(listKeysRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListKeysRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new key value pair or replaces the value of an existing key.
*
*
* @param putKeyRequest
* A key value pair.
* @return Result of the PutKey operation returned by the service.
* @throws ConflictException
* Resource is not in expected state.
* @throws ValidationException
* Validation failed.
* @throws InternalServerException
* Internal server error.
* @throws ServiceQuotaExceededException
* Limit exceeded.
* @throws ResourceNotFoundException
* Resource was not found.
* @throws AccessDeniedException
* Access denied.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFrontKeyValueStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFrontKeyValueStoreClient.PutKey
* @see AWS API Documentation
*/
@Override
public PutKeyResponse putKey(PutKeyRequest putKeyRequest) throws ConflictException, ValidationException,
InternalServerException, ServiceQuotaExceededException, ResourceNotFoundException, AccessDeniedException,
AwsServiceException, SdkClientException, CloudFrontKeyValueStoreException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putKeyRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFront KeyValueStore");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutKey");
return clientHandler.execute(new ClientExecutionParams().withOperationName("PutKey")
.withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(putKeyRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutKeyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Puts or Deletes multiple key value pairs in a single, all-or-nothing operation.
*
*
* @param updateKeysRequest
* @return Result of the UpdateKeys operation returned by the service.
* @throws ConflictException
* Resource is not in expected state.
* @throws ValidationException
* Validation failed.
* @throws InternalServerException
* Internal server error.
* @throws ServiceQuotaExceededException
* Limit exceeded.
* @throws ResourceNotFoundException
* Resource was not found.
* @throws AccessDeniedException
* Access denied.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CloudFrontKeyValueStoreException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CloudFrontKeyValueStoreClient.UpdateKeys
* @see AWS API Documentation
*/
@Override
public UpdateKeysResponse updateKeys(UpdateKeysRequest updateKeysRequest) throws ConflictException, ValidationException,
InternalServerException, ServiceQuotaExceededException, ResourceNotFoundException, AccessDeniedException,
AwsServiceException, SdkClientException, CloudFrontKeyValueStoreException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateKeysResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateKeysRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateKeysRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "CloudFront KeyValueStore");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateKeys");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateKeys").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(updateKeysRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateKeysRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
CloudFrontKeyValueStoreServiceClientConfigurationBuilder serviceConfigBuilder = new CloudFrontKeyValueStoreServiceClientConfigurationBuilder(
configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(CloudFrontKeyValueStoreException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(409).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceQuotaExceededException")
.exceptionBuilderSupplier(ServiceQuotaExceededException::builder).httpStatusCode(402).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(404).build());
}
@Override
public final CloudFrontKeyValueStoreServiceClientConfiguration serviceClientConfiguration() {
return new CloudFrontKeyValueStoreServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public void close() {
clientHandler.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy