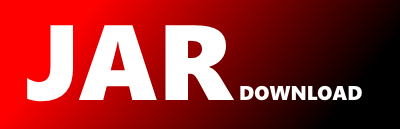
software.amazon.awssdk.services.cloudhsmv2.model.Backup Maven / Gradle / Ivy
Show all versions of cloudhsmv2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudhsmv2.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains information about a backup of an AWS CloudHSM cluster. All backup objects contain the BackupId
,
* BackupState
, ClusterId
, and CreateTimestamp
parameters. Backups that were
* copied into a destination region additionally contain the CopyTimestamp
, SourceBackup
,
* SourceCluster
, and SourceRegion
parameters. A backup that is pending deletion will include
* the DeleteTimestamp
parameter.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Backup implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField BACKUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BackupId").getter(getter(Backup::backupId)).setter(setter(Builder::backupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupId").build()).build();
private static final SdkField BACKUP_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BackupState").getter(getter(Backup::backupStateAsString)).setter(setter(Builder::backupState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupState").build()).build();
private static final SdkField CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterId").getter(getter(Backup::clusterId)).setter(setter(Builder::clusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterId").build()).build();
private static final SdkField CREATE_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreateTimestamp").getter(getter(Backup::createTimestamp)).setter(setter(Builder::createTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreateTimestamp").build()).build();
private static final SdkField COPY_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CopyTimestamp").getter(getter(Backup::copyTimestamp)).setter(setter(Builder::copyTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CopyTimestamp").build()).build();
private static final SdkField NEVER_EXPIRES_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("NeverExpires").getter(getter(Backup::neverExpires)).setter(setter(Builder::neverExpires))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NeverExpires").build()).build();
private static final SdkField SOURCE_REGION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceRegion").getter(getter(Backup::sourceRegion)).setter(setter(Builder::sourceRegion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceRegion").build()).build();
private static final SdkField SOURCE_BACKUP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceBackup").getter(getter(Backup::sourceBackup)).setter(setter(Builder::sourceBackup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceBackup").build()).build();
private static final SdkField SOURCE_CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceCluster").getter(getter(Backup::sourceCluster)).setter(setter(Builder::sourceCluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceCluster").build()).build();
private static final SdkField DELETE_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("DeleteTimestamp").getter(getter(Backup::deleteTimestamp)).setter(setter(Builder::deleteTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeleteTimestamp").build()).build();
private static final SdkField> TAG_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TagList")
.getter(getter(Backup::tagList))
.setter(setter(Builder::tagList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagList").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BACKUP_ID_FIELD,
BACKUP_STATE_FIELD, CLUSTER_ID_FIELD, CREATE_TIMESTAMP_FIELD, COPY_TIMESTAMP_FIELD, NEVER_EXPIRES_FIELD,
SOURCE_REGION_FIELD, SOURCE_BACKUP_FIELD, SOURCE_CLUSTER_FIELD, DELETE_TIMESTAMP_FIELD, TAG_LIST_FIELD));
private static final long serialVersionUID = 1L;
private final String backupId;
private final String backupState;
private final String clusterId;
private final Instant createTimestamp;
private final Instant copyTimestamp;
private final Boolean neverExpires;
private final String sourceRegion;
private final String sourceBackup;
private final String sourceCluster;
private final Instant deleteTimestamp;
private final List tagList;
private Backup(BuilderImpl builder) {
this.backupId = builder.backupId;
this.backupState = builder.backupState;
this.clusterId = builder.clusterId;
this.createTimestamp = builder.createTimestamp;
this.copyTimestamp = builder.copyTimestamp;
this.neverExpires = builder.neverExpires;
this.sourceRegion = builder.sourceRegion;
this.sourceBackup = builder.sourceBackup;
this.sourceCluster = builder.sourceCluster;
this.deleteTimestamp = builder.deleteTimestamp;
this.tagList = builder.tagList;
}
/**
*
* The identifier (ID) of the backup.
*
*
* @return The identifier (ID) of the backup.
*/
public final String backupId() {
return backupId;
}
/**
*
* The state of the backup.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #backupState} will
* return {@link BackupState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #backupStateAsString}.
*
*
* @return The state of the backup.
* @see BackupState
*/
public final BackupState backupState() {
return BackupState.fromValue(backupState);
}
/**
*
* The state of the backup.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #backupState} will
* return {@link BackupState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #backupStateAsString}.
*
*
* @return The state of the backup.
* @see BackupState
*/
public final String backupStateAsString() {
return backupState;
}
/**
*
* The identifier (ID) of the cluster that was backed up.
*
*
* @return The identifier (ID) of the cluster that was backed up.
*/
public final String clusterId() {
return clusterId;
}
/**
*
* The date and time when the backup was created.
*
*
* @return The date and time when the backup was created.
*/
public final Instant createTimestamp() {
return createTimestamp;
}
/**
*
* The date and time when the backup was copied from a source backup.
*
*
* @return The date and time when the backup was copied from a source backup.
*/
public final Instant copyTimestamp() {
return copyTimestamp;
}
/**
*
* Specifies whether the service should exempt a backup from the retention policy for the cluster. True
* exempts a backup from the retention policy. False
means the service applies the backup retention
* policy defined at the cluster.
*
*
* @return Specifies whether the service should exempt a backup from the retention policy for the cluster.
* True
exempts a backup from the retention policy. False
means the service
* applies the backup retention policy defined at the cluster.
*/
public final Boolean neverExpires() {
return neverExpires;
}
/**
*
* The AWS Region that contains the source backup from which the new backup was copied.
*
*
* @return The AWS Region that contains the source backup from which the new backup was copied.
*/
public final String sourceRegion() {
return sourceRegion;
}
/**
*
* The identifier (ID) of the source backup from which the new backup was copied.
*
*
* @return The identifier (ID) of the source backup from which the new backup was copied.
*/
public final String sourceBackup() {
return sourceBackup;
}
/**
*
* The identifier (ID) of the cluster containing the source backup from which the new backup was copied.
*
*
* @return The identifier (ID) of the cluster containing the source backup from which the new backup was copied.
*/
public final String sourceCluster() {
return sourceCluster;
}
/**
*
* The date and time when the backup will be permanently deleted.
*
*
* @return The date and time when the backup will be permanently deleted.
*/
public final Instant deleteTimestamp() {
return deleteTimestamp;
}
/**
* For responses, this returns true if the service returned a value for the TagList property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasTagList() {
return tagList != null && !(tagList instanceof SdkAutoConstructList);
}
/**
*
* The list of tags for the backup.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTagList} method.
*
*
* @return The list of tags for the backup.
*/
public final List tagList() {
return tagList;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(backupId());
hashCode = 31 * hashCode + Objects.hashCode(backupStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(clusterId());
hashCode = 31 * hashCode + Objects.hashCode(createTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(copyTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(neverExpires());
hashCode = 31 * hashCode + Objects.hashCode(sourceRegion());
hashCode = 31 * hashCode + Objects.hashCode(sourceBackup());
hashCode = 31 * hashCode + Objects.hashCode(sourceCluster());
hashCode = 31 * hashCode + Objects.hashCode(deleteTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(hasTagList() ? tagList() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Backup)) {
return false;
}
Backup other = (Backup) obj;
return Objects.equals(backupId(), other.backupId()) && Objects.equals(backupStateAsString(), other.backupStateAsString())
&& Objects.equals(clusterId(), other.clusterId()) && Objects.equals(createTimestamp(), other.createTimestamp())
&& Objects.equals(copyTimestamp(), other.copyTimestamp()) && Objects.equals(neverExpires(), other.neverExpires())
&& Objects.equals(sourceRegion(), other.sourceRegion()) && Objects.equals(sourceBackup(), other.sourceBackup())
&& Objects.equals(sourceCluster(), other.sourceCluster())
&& Objects.equals(deleteTimestamp(), other.deleteTimestamp()) && hasTagList() == other.hasTagList()
&& Objects.equals(tagList(), other.tagList());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Backup").add("BackupId", backupId()).add("BackupState", backupStateAsString())
.add("ClusterId", clusterId()).add("CreateTimestamp", createTimestamp()).add("CopyTimestamp", copyTimestamp())
.add("NeverExpires", neverExpires()).add("SourceRegion", sourceRegion()).add("SourceBackup", sourceBackup())
.add("SourceCluster", sourceCluster()).add("DeleteTimestamp", deleteTimestamp())
.add("TagList", hasTagList() ? tagList() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "BackupId":
return Optional.ofNullable(clazz.cast(backupId()));
case "BackupState":
return Optional.ofNullable(clazz.cast(backupStateAsString()));
case "ClusterId":
return Optional.ofNullable(clazz.cast(clusterId()));
case "CreateTimestamp":
return Optional.ofNullable(clazz.cast(createTimestamp()));
case "CopyTimestamp":
return Optional.ofNullable(clazz.cast(copyTimestamp()));
case "NeverExpires":
return Optional.ofNullable(clazz.cast(neverExpires()));
case "SourceRegion":
return Optional.ofNullable(clazz.cast(sourceRegion()));
case "SourceBackup":
return Optional.ofNullable(clazz.cast(sourceBackup()));
case "SourceCluster":
return Optional.ofNullable(clazz.cast(sourceCluster()));
case "DeleteTimestamp":
return Optional.ofNullable(clazz.cast(deleteTimestamp()));
case "TagList":
return Optional.ofNullable(clazz.cast(tagList()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function