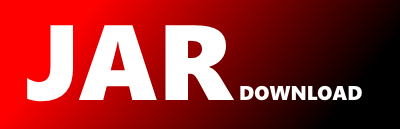
software.amazon.awssdk.services.cloudhsmv2.CloudHsmV2AsyncClient Maven / Gradle / Ivy
Show all versions of cloudhsmv2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudhsmv2;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.cloudhsmv2.model.CopyBackupToRegionRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.CopyBackupToRegionResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.CreateClusterRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.CreateClusterResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.CreateHsmRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.CreateHsmResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.DeleteBackupRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.DeleteBackupResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.DeleteClusterRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.DeleteClusterResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.DeleteHsmRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.DeleteHsmResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.DeleteResourcePolicyRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.DeleteResourcePolicyResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.GetResourcePolicyRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.GetResourcePolicyResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.InitializeClusterRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.InitializeClusterResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.ListTagsRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.ListTagsResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.ModifyBackupAttributesRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.ModifyBackupAttributesResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.ModifyClusterRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.ModifyClusterResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.PutResourcePolicyRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.PutResourcePolicyResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.RestoreBackupRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.RestoreBackupResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.TagResourceRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.TagResourceResponse;
import software.amazon.awssdk.services.cloudhsmv2.model.UntagResourceRequest;
import software.amazon.awssdk.services.cloudhsmv2.model.UntagResourceResponse;
import software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeBackupsPublisher;
import software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeClustersPublisher;
import software.amazon.awssdk.services.cloudhsmv2.paginators.ListTagsPublisher;
/**
* Service client for accessing CloudHSM V2 asynchronously. This can be created using the static {@link #builder()}
* method.The asynchronous client performs non-blocking I/O when configured with any {@code SdkAsyncHttpClient}
* supported in the SDK. However, full non-blocking is not guaranteed as the async client may perform blocking calls in
* some cases such as credentials retrieval and endpoint discovery as part of the async API call.
*
*
* For more information about CloudHSM, see CloudHSM and the CloudHSM User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface CloudHsmV2AsyncClient extends AwsClient {
String SERVICE_NAME = "cloudhsm";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "cloudhsmv2";
/**
*
* Copy an CloudHSM cluster backup to a different region.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM backup in a different Amazon Web
* Services account.
*
*
* @param copyBackupToRegionRequest
* @return A Java Future containing the result of the CopyBackupToRegion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.CopyBackupToRegion
* @see AWS
* API Documentation
*/
default CompletableFuture copyBackupToRegion(CopyBackupToRegionRequest copyBackupToRegionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Copy an CloudHSM cluster backup to a different region.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM backup in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link CopyBackupToRegionRequest.Builder} avoiding the
* need to create one manually via {@link CopyBackupToRegionRequest#builder()}
*
*
* @param copyBackupToRegionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.CopyBackupToRegionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CopyBackupToRegion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.CopyBackupToRegion
* @see AWS
* API Documentation
*/
default CompletableFuture copyBackupToRegion(
Consumer copyBackupToRegionRequest) {
return copyBackupToRegion(CopyBackupToRegionRequest.builder().applyMutation(copyBackupToRegionRequest).build());
}
/**
*
* Creates a new CloudHSM cluster.
*
*
* Cross-account use: Yes. To perform this operation with an CloudHSM backup in a different AWS account,
* specify the full backup ARN in the value of the SourceBackupId parameter.
*
*
* @param createClusterRequest
* @return A Java Future containing the result of the CreateCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.CreateCluster
* @see AWS API
* Documentation
*/
default CompletableFuture createCluster(CreateClusterRequest createClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new CloudHSM cluster.
*
*
* Cross-account use: Yes. To perform this operation with an CloudHSM backup in a different AWS account,
* specify the full backup ARN in the value of the SourceBackupId parameter.
*
*
*
* This is a convenience which creates an instance of the {@link CreateClusterRequest.Builder} avoiding the need to
* create one manually via {@link CreateClusterRequest#builder()}
*
*
* @param createClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.CreateClusterRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.CreateCluster
* @see AWS API
* Documentation
*/
default CompletableFuture createCluster(Consumer createClusterRequest) {
return createCluster(CreateClusterRequest.builder().applyMutation(createClusterRequest).build());
}
/**
*
* Creates a new hardware security module (HSM) in the specified CloudHSM cluster.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM cluster in a different Amazon Web
* Service account.
*
*
* @param createHsmRequest
* @return A Java Future containing the result of the CreateHsm operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.CreateHsm
* @see AWS API
* Documentation
*/
default CompletableFuture createHsm(CreateHsmRequest createHsmRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new hardware security module (HSM) in the specified CloudHSM cluster.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM cluster in a different Amazon Web
* Service account.
*
*
*
* This is a convenience which creates an instance of the {@link CreateHsmRequest.Builder} avoiding the need to
* create one manually via {@link CreateHsmRequest#builder()}
*
*
* @param createHsmRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.CreateHsmRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateHsm operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.CreateHsm
* @see AWS API
* Documentation
*/
default CompletableFuture createHsm(Consumer createHsmRequest) {
return createHsm(CreateHsmRequest.builder().applyMutation(createHsmRequest).build());
}
/**
*
* Deletes a specified CloudHSM backup. A backup can be restored up to 7 days after the DeleteBackup request is
* made. For more information on restoring a backup, see RestoreBackup.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM backup in a different Amazon Web
* Services account.
*
*
* @param deleteBackupRequest
* @return A Java Future containing the result of the DeleteBackup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DeleteBackup
* @see AWS API
* Documentation
*/
default CompletableFuture deleteBackup(DeleteBackupRequest deleteBackupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified CloudHSM backup. A backup can be restored up to 7 days after the DeleteBackup request is
* made. For more information on restoring a backup, see RestoreBackup.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM backup in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteBackupRequest.Builder} avoiding the need to
* create one manually via {@link DeleteBackupRequest#builder()}
*
*
* @param deleteBackupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.DeleteBackupRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteBackup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DeleteBackup
* @see AWS API
* Documentation
*/
default CompletableFuture deleteBackup(Consumer deleteBackupRequest) {
return deleteBackup(DeleteBackupRequest.builder().applyMutation(deleteBackupRequest).build());
}
/**
*
* Deletes the specified CloudHSM cluster. Before you can delete a cluster, you must delete all HSMs in the cluster.
* To see if the cluster contains any HSMs, use DescribeClusters. To delete an HSM, use DeleteHsm.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM cluster in a different Amazon Web
* Services account.
*
*
* @param deleteClusterRequest
* @return A Java Future containing the result of the DeleteCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DeleteCluster
* @see AWS API
* Documentation
*/
default CompletableFuture deleteCluster(DeleteClusterRequest deleteClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified CloudHSM cluster. Before you can delete a cluster, you must delete all HSMs in the cluster.
* To see if the cluster contains any HSMs, use DescribeClusters. To delete an HSM, use DeleteHsm.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM cluster in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteClusterRequest.Builder} avoiding the need to
* create one manually via {@link DeleteClusterRequest#builder()}
*
*
* @param deleteClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.DeleteClusterRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DeleteCluster
* @see AWS API
* Documentation
*/
default CompletableFuture deleteCluster(Consumer deleteClusterRequest) {
return deleteCluster(DeleteClusterRequest.builder().applyMutation(deleteClusterRequest).build());
}
/**
*
* Deletes the specified HSM. To specify an HSM, you can use its identifier (ID), the IP address of the HSM's
* elastic network interface (ENI), or the ID of the HSM's ENI. You need to specify only one of these values. To
* find these values, use DescribeClusters.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM hsm in a different Amazon Web
* Services account.
*
*
* @param deleteHsmRequest
* @return A Java Future containing the result of the DeleteHsm operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DeleteHsm
* @see AWS API
* Documentation
*/
default CompletableFuture deleteHsm(DeleteHsmRequest deleteHsmRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified HSM. To specify an HSM, you can use its identifier (ID), the IP address of the HSM's
* elastic network interface (ENI), or the ID of the HSM's ENI. You need to specify only one of these values. To
* find these values, use DescribeClusters.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM hsm in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteHsmRequest.Builder} avoiding the need to
* create one manually via {@link DeleteHsmRequest#builder()}
*
*
* @param deleteHsmRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.DeleteHsmRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteHsm operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DeleteHsm
* @see AWS API
* Documentation
*/
default CompletableFuture deleteHsm(Consumer deleteHsmRequest) {
return deleteHsm(DeleteHsmRequest.builder().applyMutation(deleteHsmRequest).build());
}
/**
*
* Deletes an CloudHSM resource policy. Deleting a resource policy will result in the resource being unshared and
* removed from any RAM resource shares. Deleting the resource policy attached to a backup will not impact any
* clusters created from that backup.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM resource in a different Amazon Web
* Services account.
*
*
* @param deleteResourcePolicyRequest
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DeleteResourcePolicy
* @see AWS API Documentation
*/
default CompletableFuture deleteResourcePolicy(
DeleteResourcePolicyRequest deleteResourcePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an CloudHSM resource policy. Deleting a resource policy will result in the resource being unshared and
* removed from any RAM resource shares. Deleting the resource policy attached to a backup will not impact any
* clusters created from that backup.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM resource in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteResourcePolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteResourcePolicyRequest#builder()}
*
*
* @param deleteResourcePolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.DeleteResourcePolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DeleteResourcePolicy
* @see AWS API Documentation
*/
default CompletableFuture deleteResourcePolicy(
Consumer deleteResourcePolicyRequest) {
return deleteResourcePolicy(DeleteResourcePolicyRequest.builder().applyMutation(deleteResourcePolicyRequest).build());
}
/**
*
* Gets information about backups of CloudHSM clusters. Lists either the backups you own or the backups shared with
* you when the Shared parameter is true.
*
*
* This is a paginated operation, which means that each response might contain only a subset of all the backups.
* When the response contains only a subset of backups, it includes a NextToken
value. Use this value
* in a subsequent DescribeBackups
request to get more backups. When you receive a response with no
* NextToken
(or an empty or null value), that means there are no more backups to get.
*
*
* Cross-account use: Yes. Customers can describe backups in other Amazon Web Services accounts that are
* shared with them.
*
*
* @param describeBackupsRequest
* @return A Java Future containing the result of the DescribeBackups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DescribeBackups
* @see AWS
* API Documentation
*/
default CompletableFuture describeBackups(DescribeBackupsRequest describeBackupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about backups of CloudHSM clusters. Lists either the backups you own or the backups shared with
* you when the Shared parameter is true.
*
*
* This is a paginated operation, which means that each response might contain only a subset of all the backups.
* When the response contains only a subset of backups, it includes a NextToken
value. Use this value
* in a subsequent DescribeBackups
request to get more backups. When you receive a response with no
* NextToken
(or an empty or null value), that means there are no more backups to get.
*
*
* Cross-account use: Yes. Customers can describe backups in other Amazon Web Services accounts that are
* shared with them.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeBackupsRequest.Builder} avoiding the need
* to create one manually via {@link DescribeBackupsRequest#builder()}
*
*
* @param describeBackupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeBackups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DescribeBackups
* @see AWS
* API Documentation
*/
default CompletableFuture describeBackups(
Consumer describeBackupsRequest) {
return describeBackups(DescribeBackupsRequest.builder().applyMutation(describeBackupsRequest).build());
}
/**
*
* Gets information about backups of CloudHSM clusters. Lists either the backups you own or the backups shared with
* you when the Shared parameter is true.
*
*
* This is a paginated operation, which means that each response might contain only a subset of all the backups.
* When the response contains only a subset of backups, it includes a NextToken
value. Use this value
* in a subsequent DescribeBackups
request to get more backups. When you receive a response with no
* NextToken
(or an empty or null value), that means there are no more backups to get.
*
*
* Cross-account use: Yes. Customers can describe backups in other Amazon Web Services accounts that are
* shared with them.
*
*
* @return A Java Future containing the result of the DescribeBackups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DescribeBackups
* @see AWS
* API Documentation
*/
default CompletableFuture describeBackups() {
return describeBackups(DescribeBackupsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeBackups(software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeBackupsPublisher publisher = client.describeBackupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeBackupsPublisher publisher = client.describeBackupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBackups(software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DescribeBackups
* @see AWS
* API Documentation
*/
default DescribeBackupsPublisher describeBackupsPaginator() {
return describeBackupsPaginator(DescribeBackupsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeBackups(software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeBackupsPublisher publisher = client.describeBackupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeBackupsPublisher publisher = client.describeBackupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBackups(software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsRequest)} operation.
*
*
* @param describeBackupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DescribeBackups
* @see AWS
* API Documentation
*/
default DescribeBackupsPublisher describeBackupsPaginator(DescribeBackupsRequest describeBackupsRequest) {
return new DescribeBackupsPublisher(this, describeBackupsRequest);
}
/**
*
* This is a variant of
* {@link #describeBackups(software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeBackupsPublisher publisher = client.describeBackupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeBackupsPublisher publisher = client.describeBackupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeBackups(software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeBackupsRequest.Builder} avoiding the need
* to create one manually via {@link DescribeBackupsRequest#builder()}
*
*
* @param describeBackupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.DescribeBackupsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DescribeBackups
* @see AWS
* API Documentation
*/
default DescribeBackupsPublisher describeBackupsPaginator(Consumer describeBackupsRequest) {
return describeBackupsPaginator(DescribeBackupsRequest.builder().applyMutation(describeBackupsRequest).build());
}
/**
*
* Gets information about CloudHSM clusters.
*
*
* This is a paginated operation, which means that each response might contain only a subset of all the clusters.
* When the response contains only a subset of clusters, it includes a NextToken
value. Use this value
* in a subsequent DescribeClusters
request to get more clusters. When you receive a response with no
* NextToken
(or an empty or null value), that means there are no more clusters to get.
*
*
* Cross-account use: No. You cannot perform this operation on CloudHSM clusters in a different Amazon Web
* Services account.
*
*
* @param describeClustersRequest
* @return A Java Future containing the result of the DescribeClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DescribeClusters
* @see AWS
* API Documentation
*/
default CompletableFuture describeClusters(DescribeClustersRequest describeClustersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about CloudHSM clusters.
*
*
* This is a paginated operation, which means that each response might contain only a subset of all the clusters.
* When the response contains only a subset of clusters, it includes a NextToken
value. Use this value
* in a subsequent DescribeClusters
request to get more clusters. When you receive a response with no
* NextToken
(or an empty or null value), that means there are no more clusters to get.
*
*
* Cross-account use: No. You cannot perform this operation on CloudHSM clusters in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeClustersRequest.Builder} avoiding the need
* to create one manually via {@link DescribeClustersRequest#builder()}
*
*
* @param describeClustersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DescribeClusters
* @see AWS
* API Documentation
*/
default CompletableFuture describeClusters(
Consumer describeClustersRequest) {
return describeClusters(DescribeClustersRequest.builder().applyMutation(describeClustersRequest).build());
}
/**
*
* Gets information about CloudHSM clusters.
*
*
* This is a paginated operation, which means that each response might contain only a subset of all the clusters.
* When the response contains only a subset of clusters, it includes a NextToken
value. Use this value
* in a subsequent DescribeClusters
request to get more clusters. When you receive a response with no
* NextToken
(or an empty or null value), that means there are no more clusters to get.
*
*
* Cross-account use: No. You cannot perform this operation on CloudHSM clusters in a different Amazon Web
* Services account.
*
*
* @return A Java Future containing the result of the DescribeClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DescribeClusters
* @see AWS
* API Documentation
*/
default CompletableFuture describeClusters() {
return describeClusters(DescribeClustersRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeClusters(software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeClustersPublisher publisher = client.describeClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeClustersPublisher publisher = client.describeClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeClusters(software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DescribeClusters
* @see AWS
* API Documentation
*/
default DescribeClustersPublisher describeClustersPaginator() {
return describeClustersPaginator(DescribeClustersRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #describeClusters(software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeClustersPublisher publisher = client.describeClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeClustersPublisher publisher = client.describeClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeClusters(software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersRequest)}
* operation.
*
*
* @param describeClustersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DescribeClusters
* @see AWS
* API Documentation
*/
default DescribeClustersPublisher describeClustersPaginator(DescribeClustersRequest describeClustersRequest) {
return new DescribeClustersPublisher(this, describeClustersRequest);
}
/**
*
* This is a variant of
* {@link #describeClusters(software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeClustersPublisher publisher = client.describeClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.DescribeClustersPublisher publisher = client.describeClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeClusters(software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeClustersRequest.Builder} avoiding the need
* to create one manually via {@link DescribeClustersRequest#builder()}
*
*
* @param describeClustersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.DescribeClustersRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.DescribeClusters
* @see AWS
* API Documentation
*/
default DescribeClustersPublisher describeClustersPaginator(Consumer describeClustersRequest) {
return describeClustersPaginator(DescribeClustersRequest.builder().applyMutation(describeClustersRequest).build());
}
/**
*
* Retrieves the resource policy document attached to a given resource.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM resource in a different Amazon Web
* Services account.
*
*
* @param getResourcePolicyRequest
* @return A Java Future containing the result of the GetResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.GetResourcePolicy
* @see AWS
* API Documentation
*/
default CompletableFuture getResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the resource policy document attached to a given resource.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM resource in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourcePolicyRequest.Builder} avoiding the need
* to create one manually via {@link GetResourcePolicyRequest#builder()}
*
*
* @param getResourcePolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.GetResourcePolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.GetResourcePolicy
* @see AWS
* API Documentation
*/
default CompletableFuture getResourcePolicy(
Consumer getResourcePolicyRequest) {
return getResourcePolicy(GetResourcePolicyRequest.builder().applyMutation(getResourcePolicyRequest).build());
}
/**
*
* Claims an CloudHSM cluster by submitting the cluster certificate issued by your issuing certificate authority
* (CA) and the CA's root certificate. Before you can claim a cluster, you must sign the cluster's certificate
* signing request (CSR) with your issuing CA. To get the cluster's CSR, use DescribeClusters.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM cluster in a different Amazon Web
* Services account.
*
*
* @param initializeClusterRequest
* @return A Java Future containing the result of the InitializeCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.InitializeCluster
* @see AWS
* API Documentation
*/
default CompletableFuture initializeCluster(InitializeClusterRequest initializeClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Claims an CloudHSM cluster by submitting the cluster certificate issued by your issuing certificate authority
* (CA) and the CA's root certificate. Before you can claim a cluster, you must sign the cluster's certificate
* signing request (CSR) with your issuing CA. To get the cluster's CSR, use DescribeClusters.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM cluster in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link InitializeClusterRequest.Builder} avoiding the need
* to create one manually via {@link InitializeClusterRequest#builder()}
*
*
* @param initializeClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.InitializeClusterRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the InitializeCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.InitializeCluster
* @see AWS
* API Documentation
*/
default CompletableFuture initializeCluster(
Consumer initializeClusterRequest) {
return initializeCluster(InitializeClusterRequest.builder().applyMutation(initializeClusterRequest).build());
}
/**
*
* Gets a list of tags for the specified CloudHSM cluster.
*
*
* This is a paginated operation, which means that each response might contain only a subset of all the tags. When
* the response contains only a subset of tags, it includes a NextToken
value. Use this value in a
* subsequent ListTags
request to get more tags. When you receive a response with no
* NextToken
(or an empty or null value), that means there are no more tags to get.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM resource in a different Amazon Web
* Services account.
*
*
* @param listTagsRequest
* @return A Java Future containing the result of the ListTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.ListTags
* @see AWS API
* Documentation
*/
default CompletableFuture listTags(ListTagsRequest listTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of tags for the specified CloudHSM cluster.
*
*
* This is a paginated operation, which means that each response might contain only a subset of all the tags. When
* the response contains only a subset of tags, it includes a NextToken
value. Use this value in a
* subsequent ListTags
request to get more tags. When you receive a response with no
* NextToken
(or an empty or null value), that means there are no more tags to get.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM resource in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsRequest.Builder} avoiding the need to
* create one manually via {@link ListTagsRequest#builder()}
*
*
* @param listTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.ListTagsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.ListTags
* @see AWS API
* Documentation
*/
default CompletableFuture listTags(Consumer listTagsRequest) {
return listTags(ListTagsRequest.builder().applyMutation(listTagsRequest).build());
}
/**
*
* This is a variant of {@link #listTags(software.amazon.awssdk.services.cloudhsmv2.model.ListTagsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.ListTagsPublisher publisher = client.listTagsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.ListTagsPublisher publisher = client.listTagsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.cloudhsmv2.model.ListTagsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTags(software.amazon.awssdk.services.cloudhsmv2.model.ListTagsRequest)} operation.
*
*
* @param listTagsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.ListTags
* @see AWS API
* Documentation
*/
default ListTagsPublisher listTagsPaginator(ListTagsRequest listTagsRequest) {
return new ListTagsPublisher(this, listTagsRequest);
}
/**
*
* This is a variant of {@link #listTags(software.amazon.awssdk.services.cloudhsmv2.model.ListTagsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.ListTagsPublisher publisher = client.listTagsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.cloudhsmv2.paginators.ListTagsPublisher publisher = client.listTagsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.cloudhsmv2.model.ListTagsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTags(software.amazon.awssdk.services.cloudhsmv2.model.ListTagsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsRequest.Builder} avoiding the need to
* create one manually via {@link ListTagsRequest#builder()}
*
*
* @param listTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.ListTagsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.ListTags
* @see AWS API
* Documentation
*/
default ListTagsPublisher listTagsPaginator(Consumer listTagsRequest) {
return listTagsPaginator(ListTagsRequest.builder().applyMutation(listTagsRequest).build());
}
/**
*
* Modifies attributes for CloudHSM backup.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM backup in a different Amazon Web
* Services account.
*
*
* @param modifyBackupAttributesRequest
* @return A Java Future containing the result of the ModifyBackupAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.ModifyBackupAttributes
* @see AWS API Documentation
*/
default CompletableFuture modifyBackupAttributes(
ModifyBackupAttributesRequest modifyBackupAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies attributes for CloudHSM backup.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM backup in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyBackupAttributesRequest.Builder} avoiding the
* need to create one manually via {@link ModifyBackupAttributesRequest#builder()}
*
*
* @param modifyBackupAttributesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.ModifyBackupAttributesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ModifyBackupAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.ModifyBackupAttributes
* @see AWS API Documentation
*/
default CompletableFuture modifyBackupAttributes(
Consumer modifyBackupAttributesRequest) {
return modifyBackupAttributes(ModifyBackupAttributesRequest.builder().applyMutation(modifyBackupAttributesRequest)
.build());
}
/**
*
* Modifies CloudHSM cluster.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM cluster in a different Amazon Web
* Services account.
*
*
* @param modifyClusterRequest
* @return A Java Future containing the result of the ModifyCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.ModifyCluster
* @see AWS API
* Documentation
*/
default CompletableFuture modifyCluster(ModifyClusterRequest modifyClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies CloudHSM cluster.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM cluster in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link ModifyClusterRequest.Builder} avoiding the need to
* create one manually via {@link ModifyClusterRequest#builder()}
*
*
* @param modifyClusterRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.ModifyClusterRequest.Builder} to create a request.
* @return A Java Future containing the result of the ModifyCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.ModifyCluster
* @see AWS API
* Documentation
*/
default CompletableFuture modifyCluster(Consumer modifyClusterRequest) {
return modifyCluster(ModifyClusterRequest.builder().applyMutation(modifyClusterRequest).build());
}
/**
*
* Creates or updates an CloudHSM resource policy. A resource policy helps you to define the IAM entity (for
* example, an Amazon Web Services account) that can manage your CloudHSM resources. The following resources support
* CloudHSM resource policies:
*
*
* -
*
* Backup - The resource policy allows you to describe the backup and restore a cluster from the backup in another
* Amazon Web Services account.
*
*
*
*
* In order to share a backup, it must be in a 'READY' state and you must own it.
*
*
*
* While you can share a backup using the CloudHSM PutResourcePolicy operation, we recommend using Resource Access
* Manager (RAM) instead. Using RAM provides multiple benefits as it creates the policy for you, allows multiple
* resources to be shared at one time, and increases the discoverability of shared resources. If you use
* PutResourcePolicy and want consumers to be able to describe the backups you share with them, you must promote the
* backup to a standard RAM Resource Share using the RAM PromoteResourceShareCreatedFromPolicy API operation. For
* more information, see Working with
* shared backups in the CloudHSM User Guide
*
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM resource in a different Amazon Web
* Services account.
*
*
* @param putResourcePolicyRequest
* @return A Java Future containing the result of the PutResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.PutResourcePolicy
* @see AWS
* API Documentation
*/
default CompletableFuture putResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates an CloudHSM resource policy. A resource policy helps you to define the IAM entity (for
* example, an Amazon Web Services account) that can manage your CloudHSM resources. The following resources support
* CloudHSM resource policies:
*
*
* -
*
* Backup - The resource policy allows you to describe the backup and restore a cluster from the backup in another
* Amazon Web Services account.
*
*
*
*
* In order to share a backup, it must be in a 'READY' state and you must own it.
*
*
*
* While you can share a backup using the CloudHSM PutResourcePolicy operation, we recommend using Resource Access
* Manager (RAM) instead. Using RAM provides multiple benefits as it creates the policy for you, allows multiple
* resources to be shared at one time, and increases the discoverability of shared resources. If you use
* PutResourcePolicy and want consumers to be able to describe the backups you share with them, you must promote the
* backup to a standard RAM Resource Share using the RAM PromoteResourceShareCreatedFromPolicy API operation. For
* more information, see Working with
* shared backups in the CloudHSM User Guide
*
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM resource in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link PutResourcePolicyRequest.Builder} avoiding the need
* to create one manually via {@link PutResourcePolicyRequest#builder()}
*
*
* @param putResourcePolicyRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.PutResourcePolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the PutResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.PutResourcePolicy
* @see AWS
* API Documentation
*/
default CompletableFuture putResourcePolicy(
Consumer putResourcePolicyRequest) {
return putResourcePolicy(PutResourcePolicyRequest.builder().applyMutation(putResourcePolicyRequest).build());
}
/**
*
* Restores a specified CloudHSM backup that is in the PENDING_DELETION
state. For more information on
* deleting a backup, see DeleteBackup.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM backup in a different Amazon Web
* Services account.
*
*
* @param restoreBackupRequest
* @return A Java Future containing the result of the RestoreBackup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.RestoreBackup
* @see AWS API
* Documentation
*/
default CompletableFuture restoreBackup(RestoreBackupRequest restoreBackupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Restores a specified CloudHSM backup that is in the PENDING_DELETION
state. For more information on
* deleting a backup, see DeleteBackup.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM backup in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link RestoreBackupRequest.Builder} avoiding the need to
* create one manually via {@link RestoreBackupRequest#builder()}
*
*
* @param restoreBackupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.RestoreBackupRequest.Builder} to create a request.
* @return A Java Future containing the result of the RestoreBackup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.RestoreBackup
* @see AWS API
* Documentation
*/
default CompletableFuture restoreBackup(Consumer restoreBackupRequest) {
return restoreBackup(RestoreBackupRequest.builder().applyMutation(restoreBackupRequest).build());
}
/**
*
* Adds or overwrites one or more tags for the specified CloudHSM cluster.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM resource in a different Amazon Web
* Services account.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceLimitExceededException The request was rejected because it exceeds an CloudHSM limit.
*
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds or overwrites one or more tags for the specified CloudHSM cluster.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM resource in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceLimitExceededException The request was rejected because it exceeds an CloudHSM limit.
*
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes the specified tag or tags from the specified CloudHSM cluster.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM resource in a different Amazon Web
* Services account.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tag or tags from the specified CloudHSM cluster.
*
*
* Cross-account use: No. You cannot perform this operation on an CloudHSM resource in a different Amazon Web
* Services account.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.cloudhsmv2.model.UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CloudHsmAccessDeniedException The request was rejected because the requester does not have permission
* to perform the requested operation.
* - CloudHsmInternalFailureException The request was rejected because of an CloudHSM internal failure.
* The request can be retried.
* - CloudHsmInvalidRequestException The request was rejected because it is not a valid request.
* - CloudHsmResourceNotFoundException The request was rejected because it refers to a resource that
* cannot be found.
* - CloudHsmServiceException The request was rejected because an error occurred.
* - CloudHsmTagException The request was rejected because of a tagging failure. Verify the tag conditions
* in all applicable policies, and then retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CloudHsmV2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CloudHsmV2AsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
@Override
default CloudHsmV2ServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link CloudHsmV2AsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static CloudHsmV2AsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link CloudHsmV2AsyncClient}.
*/
static CloudHsmV2AsyncClientBuilder builder() {
return new DefaultCloudHsmV2AsyncClientBuilder();
}
}