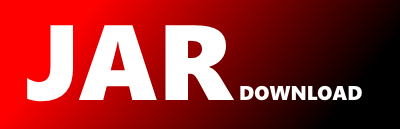
software.amazon.awssdk.services.cloudsearch.model.DomainStatus Maven / Gradle / Ivy
Show all versions of cloudsearch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudsearch.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The current status of the search domain.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DomainStatus implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField DOMAIN_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DomainId").getter(getter(DomainStatus::domainId)).setter(setter(Builder::domainId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DomainId").build()).build();
private static final SdkField DOMAIN_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DomainName").getter(getter(DomainStatus::domainName)).setter(setter(Builder::domainName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DomainName").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("ARN")
.getter(getter(DomainStatus::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ARN").build()).build();
private static final SdkField CREATED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Created").getter(getter(DomainStatus::created)).setter(setter(Builder::created))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Created").build()).build();
private static final SdkField DELETED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Deleted").getter(getter(DomainStatus::deleted)).setter(setter(Builder::deleted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Deleted").build()).build();
private static final SdkField DOC_SERVICE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DocService")
.getter(getter(DomainStatus::docService)).setter(setter(Builder::docService)).constructor(ServiceEndpoint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DocService").build()).build();
private static final SdkField SEARCH_SERVICE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("SearchService")
.getter(getter(DomainStatus::searchService)).setter(setter(Builder::searchService))
.constructor(ServiceEndpoint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SearchService").build()).build();
private static final SdkField REQUIRES_INDEX_DOCUMENTS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("RequiresIndexDocuments").getter(getter(DomainStatus::requiresIndexDocuments))
.setter(setter(Builder::requiresIndexDocuments))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequiresIndexDocuments").build())
.build();
private static final SdkField PROCESSING_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Processing").getter(getter(DomainStatus::processing)).setter(setter(Builder::processing))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Processing").build()).build();
private static final SdkField SEARCH_INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SearchInstanceType").getter(getter(DomainStatus::searchInstanceType))
.setter(setter(Builder::searchInstanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SearchInstanceType").build())
.build();
private static final SdkField SEARCH_PARTITION_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SearchPartitionCount").getter(getter(DomainStatus::searchPartitionCount))
.setter(setter(Builder::searchPartitionCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SearchPartitionCount").build())
.build();
private static final SdkField SEARCH_INSTANCE_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SearchInstanceCount").getter(getter(DomainStatus::searchInstanceCount))
.setter(setter(Builder::searchInstanceCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SearchInstanceCount").build())
.build();
private static final SdkField LIMITS_FIELD = SdkField. builder(MarshallingType.SDK_POJO).memberName("Limits")
.getter(getter(DomainStatus::limits)).setter(setter(Builder::limits)).constructor(Limits::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Limits").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DOMAIN_ID_FIELD,
DOMAIN_NAME_FIELD, ARN_FIELD, CREATED_FIELD, DELETED_FIELD, DOC_SERVICE_FIELD, SEARCH_SERVICE_FIELD,
REQUIRES_INDEX_DOCUMENTS_FIELD, PROCESSING_FIELD, SEARCH_INSTANCE_TYPE_FIELD, SEARCH_PARTITION_COUNT_FIELD,
SEARCH_INSTANCE_COUNT_FIELD, LIMITS_FIELD));
private static final long serialVersionUID = 1L;
private final String domainId;
private final String domainName;
private final String arn;
private final Boolean created;
private final Boolean deleted;
private final ServiceEndpoint docService;
private final ServiceEndpoint searchService;
private final Boolean requiresIndexDocuments;
private final Boolean processing;
private final String searchInstanceType;
private final Integer searchPartitionCount;
private final Integer searchInstanceCount;
private final Limits limits;
private DomainStatus(BuilderImpl builder) {
this.domainId = builder.domainId;
this.domainName = builder.domainName;
this.arn = builder.arn;
this.created = builder.created;
this.deleted = builder.deleted;
this.docService = builder.docService;
this.searchService = builder.searchService;
this.requiresIndexDocuments = builder.requiresIndexDocuments;
this.processing = builder.processing;
this.searchInstanceType = builder.searchInstanceType;
this.searchPartitionCount = builder.searchPartitionCount;
this.searchInstanceCount = builder.searchInstanceCount;
this.limits = builder.limits;
}
/**
* Returns the value of the DomainId property for this object.
*
* @return The value of the DomainId property for this object.
*/
public final String domainId() {
return domainId;
}
/**
* Returns the value of the DomainName property for this object.
*
* @return The value of the DomainName property for this object.
*/
public final String domainName() {
return domainName;
}
/**
* Returns the value of the ARN property for this object.
*
* @return The value of the ARN property for this object.
*/
public final String arn() {
return arn;
}
/**
*
* True if the search domain is created. It can take several minutes to initialize a domain when CreateDomain
* is called. Newly created search domains are returned from DescribeDomains with a false value for Created
* until domain creation is complete.
*
*
* @return True if the search domain is created. It can take several minutes to initialize a domain when
* CreateDomain is called. Newly created search domains are returned from DescribeDomains with
* a false value for Created until domain creation is complete.
*/
public final Boolean created() {
return created;
}
/**
*
* True if the search domain has been deleted. The system must clean up resources dedicated to the search domain
* when DeleteDomain is called. Newly deleted search domains are returned from DescribeDomains with a
* true value for IsDeleted for several minutes until resource cleanup is complete.
*
*
* @return True if the search domain has been deleted. The system must clean up resources dedicated to the search
* domain when DeleteDomain is called. Newly deleted search domains are returned from
* DescribeDomains with a true value for IsDeleted for several minutes until resource cleanup is
* complete.
*/
public final Boolean deleted() {
return deleted;
}
/**
*
* The service endpoint for updating documents in a search domain.
*
*
* @return The service endpoint for updating documents in a search domain.
*/
public final ServiceEndpoint docService() {
return docService;
}
/**
*
* The service endpoint for requesting search results from a search domain.
*
*
* @return The service endpoint for requesting search results from a search domain.
*/
public final ServiceEndpoint searchService() {
return searchService;
}
/**
*
* True if IndexDocuments needs to be called to activate the current domain configuration.
*
*
* @return True if IndexDocuments needs to be called to activate the current domain configuration.
*/
public final Boolean requiresIndexDocuments() {
return requiresIndexDocuments;
}
/**
*
* True if processing is being done to activate the current domain configuration.
*
*
* @return True if processing is being done to activate the current domain configuration.
*/
public final Boolean processing() {
return processing;
}
/**
*
* The instance type that is being used to process search requests.
*
*
* @return The instance type that is being used to process search requests.
*/
public final String searchInstanceType() {
return searchInstanceType;
}
/**
*
* The number of partitions across which the search index is spread.
*
*
* @return The number of partitions across which the search index is spread.
*/
public final Integer searchPartitionCount() {
return searchPartitionCount;
}
/**
*
* The number of search instances that are available to process search requests.
*
*
* @return The number of search instances that are available to process search requests.
*/
public final Integer searchInstanceCount() {
return searchInstanceCount;
}
/**
* Returns the value of the Limits property for this object.
*
* @return The value of the Limits property for this object.
*/
public final Limits limits() {
return limits;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(domainId());
hashCode = 31 * hashCode + Objects.hashCode(domainName());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(created());
hashCode = 31 * hashCode + Objects.hashCode(deleted());
hashCode = 31 * hashCode + Objects.hashCode(docService());
hashCode = 31 * hashCode + Objects.hashCode(searchService());
hashCode = 31 * hashCode + Objects.hashCode(requiresIndexDocuments());
hashCode = 31 * hashCode + Objects.hashCode(processing());
hashCode = 31 * hashCode + Objects.hashCode(searchInstanceType());
hashCode = 31 * hashCode + Objects.hashCode(searchPartitionCount());
hashCode = 31 * hashCode + Objects.hashCode(searchInstanceCount());
hashCode = 31 * hashCode + Objects.hashCode(limits());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DomainStatus)) {
return false;
}
DomainStatus other = (DomainStatus) obj;
return Objects.equals(domainId(), other.domainId()) && Objects.equals(domainName(), other.domainName())
&& Objects.equals(arn(), other.arn()) && Objects.equals(created(), other.created())
&& Objects.equals(deleted(), other.deleted()) && Objects.equals(docService(), other.docService())
&& Objects.equals(searchService(), other.searchService())
&& Objects.equals(requiresIndexDocuments(), other.requiresIndexDocuments())
&& Objects.equals(processing(), other.processing())
&& Objects.equals(searchInstanceType(), other.searchInstanceType())
&& Objects.equals(searchPartitionCount(), other.searchPartitionCount())
&& Objects.equals(searchInstanceCount(), other.searchInstanceCount()) && Objects.equals(limits(), other.limits());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DomainStatus").add("DomainId", domainId()).add("DomainName", domainName()).add("ARN", arn())
.add("Created", created()).add("Deleted", deleted()).add("DocService", docService())
.add("SearchService", searchService()).add("RequiresIndexDocuments", requiresIndexDocuments())
.add("Processing", processing()).add("SearchInstanceType", searchInstanceType())
.add("SearchPartitionCount", searchPartitionCount()).add("SearchInstanceCount", searchInstanceCount())
.add("Limits", limits()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DomainId":
return Optional.ofNullable(clazz.cast(domainId()));
case "DomainName":
return Optional.ofNullable(clazz.cast(domainName()));
case "ARN":
return Optional.ofNullable(clazz.cast(arn()));
case "Created":
return Optional.ofNullable(clazz.cast(created()));
case "Deleted":
return Optional.ofNullable(clazz.cast(deleted()));
case "DocService":
return Optional.ofNullable(clazz.cast(docService()));
case "SearchService":
return Optional.ofNullable(clazz.cast(searchService()));
case "RequiresIndexDocuments":
return Optional.ofNullable(clazz.cast(requiresIndexDocuments()));
case "Processing":
return Optional.ofNullable(clazz.cast(processing()));
case "SearchInstanceType":
return Optional.ofNullable(clazz.cast(searchInstanceType()));
case "SearchPartitionCount":
return Optional.ofNullable(clazz.cast(searchPartitionCount()));
case "SearchInstanceCount":
return Optional.ofNullable(clazz.cast(searchInstanceCount()));
case "Limits":
return Optional.ofNullable(clazz.cast(limits()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function