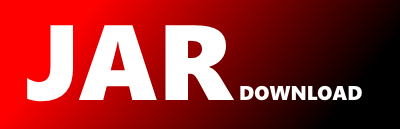
software.amazon.awssdk.services.cloudsearch.model.IndexField Maven / Gradle / Ivy
Show all versions of cloudsearch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudsearch.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Configuration information for a field in the index, including its name, type, and options. The supported options
* depend on the IndexFieldType
.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class IndexField implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField INDEX_FIELD_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IndexFieldName").getter(getter(IndexField::indexFieldName)).setter(setter(Builder::indexFieldName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IndexFieldName").build()).build();
private static final SdkField INDEX_FIELD_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IndexFieldType").getter(getter(IndexField::indexFieldTypeAsString))
.setter(setter(Builder::indexFieldType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IndexFieldType").build()).build();
private static final SdkField INT_OPTIONS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("IntOptions").getter(getter(IndexField::intOptions)).setter(setter(Builder::intOptions))
.constructor(IntOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IntOptions").build()).build();
private static final SdkField DOUBLE_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DoubleOptions")
.getter(getter(IndexField::doubleOptions)).setter(setter(Builder::doubleOptions)).constructor(DoubleOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DoubleOptions").build()).build();
private static final SdkField LITERAL_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("LiteralOptions")
.getter(getter(IndexField::literalOptions)).setter(setter(Builder::literalOptions))
.constructor(LiteralOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LiteralOptions").build()).build();
private static final SdkField TEXT_OPTIONS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("TextOptions").getter(getter(IndexField::textOptions)).setter(setter(Builder::textOptions))
.constructor(TextOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TextOptions").build()).build();
private static final SdkField DATE_OPTIONS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("DateOptions").getter(getter(IndexField::dateOptions)).setter(setter(Builder::dateOptions))
.constructor(DateOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DateOptions").build()).build();
private static final SdkField LAT_LON_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("LatLonOptions")
.getter(getter(IndexField::latLonOptions)).setter(setter(Builder::latLonOptions)).constructor(LatLonOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LatLonOptions").build()).build();
private static final SdkField INT_ARRAY_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("IntArrayOptions")
.getter(getter(IndexField::intArrayOptions)).setter(setter(Builder::intArrayOptions))
.constructor(IntArrayOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IntArrayOptions").build()).build();
private static final SdkField DOUBLE_ARRAY_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DoubleArrayOptions")
.getter(getter(IndexField::doubleArrayOptions)).setter(setter(Builder::doubleArrayOptions))
.constructor(DoubleArrayOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DoubleArrayOptions").build())
.build();
private static final SdkField LITERAL_ARRAY_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("LiteralArrayOptions")
.getter(getter(IndexField::literalArrayOptions)).setter(setter(Builder::literalArrayOptions))
.constructor(LiteralArrayOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LiteralArrayOptions").build())
.build();
private static final SdkField TEXT_ARRAY_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("TextArrayOptions")
.getter(getter(IndexField::textArrayOptions)).setter(setter(Builder::textArrayOptions))
.constructor(TextArrayOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TextArrayOptions").build()).build();
private static final SdkField DATE_ARRAY_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DateArrayOptions")
.getter(getter(IndexField::dateArrayOptions)).setter(setter(Builder::dateArrayOptions))
.constructor(DateArrayOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DateArrayOptions").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(INDEX_FIELD_NAME_FIELD,
INDEX_FIELD_TYPE_FIELD, INT_OPTIONS_FIELD, DOUBLE_OPTIONS_FIELD, LITERAL_OPTIONS_FIELD, TEXT_OPTIONS_FIELD,
DATE_OPTIONS_FIELD, LAT_LON_OPTIONS_FIELD, INT_ARRAY_OPTIONS_FIELD, DOUBLE_ARRAY_OPTIONS_FIELD,
LITERAL_ARRAY_OPTIONS_FIELD, TEXT_ARRAY_OPTIONS_FIELD, DATE_ARRAY_OPTIONS_FIELD));
private static final long serialVersionUID = 1L;
private final String indexFieldName;
private final String indexFieldType;
private final IntOptions intOptions;
private final DoubleOptions doubleOptions;
private final LiteralOptions literalOptions;
private final TextOptions textOptions;
private final DateOptions dateOptions;
private final LatLonOptions latLonOptions;
private final IntArrayOptions intArrayOptions;
private final DoubleArrayOptions doubleArrayOptions;
private final LiteralArrayOptions literalArrayOptions;
private final TextArrayOptions textArrayOptions;
private final DateArrayOptions dateArrayOptions;
private IndexField(BuilderImpl builder) {
this.indexFieldName = builder.indexFieldName;
this.indexFieldType = builder.indexFieldType;
this.intOptions = builder.intOptions;
this.doubleOptions = builder.doubleOptions;
this.literalOptions = builder.literalOptions;
this.textOptions = builder.textOptions;
this.dateOptions = builder.dateOptions;
this.latLonOptions = builder.latLonOptions;
this.intArrayOptions = builder.intArrayOptions;
this.doubleArrayOptions = builder.doubleArrayOptions;
this.literalArrayOptions = builder.literalArrayOptions;
this.textArrayOptions = builder.textArrayOptions;
this.dateArrayOptions = builder.dateArrayOptions;
}
/**
*
* A string that represents the name of an index field. CloudSearch supports regular index fields as well as dynamic
* fields. A dynamic field's name defines a pattern that begins or ends with a wildcard. Any document fields that
* don't map to a regular index field but do match a dynamic field's pattern are configured with the dynamic field's
* indexing options.
*
*
* Regular field names begin with a letter and can contain the following characters: a-z (lowercase), 0-9, and _
* (underscore). Dynamic field names must begin or end with a wildcard (*). The wildcard can also be the only
* character in a dynamic field name. Multiple wildcards, and wildcards embedded within a string are not supported.
*
*
* The name score
is reserved and cannot be used as a field name. To reference a document's ID, you can
* use the name _id
.
*
*
* @return A string that represents the name of an index field. CloudSearch supports regular index fields as well as
* dynamic fields. A dynamic field's name defines a pattern that begins or ends with a wildcard. Any
* document fields that don't map to a regular index field but do match a dynamic field's pattern are
* configured with the dynamic field's indexing options.
*
* Regular field names begin with a letter and can contain the following characters: a-z (lowercase), 0-9,
* and _ (underscore). Dynamic field names must begin or end with a wildcard (*). The wildcard can also be
* the only character in a dynamic field name. Multiple wildcards, and wildcards embedded within a string
* are not supported.
*
*
* The name score
is reserved and cannot be used as a field name. To reference a document's ID,
* you can use the name _id
.
*/
public final String indexFieldName() {
return indexFieldName;
}
/**
* Returns the value of the IndexFieldType property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #indexFieldType}
* will return {@link IndexFieldType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #indexFieldTypeAsString}.
*
*
* @return The value of the IndexFieldType property for this object.
* @see IndexFieldType
*/
public final IndexFieldType indexFieldType() {
return IndexFieldType.fromValue(indexFieldType);
}
/**
* Returns the value of the IndexFieldType property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #indexFieldType}
* will return {@link IndexFieldType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #indexFieldTypeAsString}.
*
*
* @return The value of the IndexFieldType property for this object.
* @see IndexFieldType
*/
public final String indexFieldTypeAsString() {
return indexFieldType;
}
/**
* Returns the value of the IntOptions property for this object.
*
* @return The value of the IntOptions property for this object.
*/
public final IntOptions intOptions() {
return intOptions;
}
/**
* Returns the value of the DoubleOptions property for this object.
*
* @return The value of the DoubleOptions property for this object.
*/
public final DoubleOptions doubleOptions() {
return doubleOptions;
}
/**
* Returns the value of the LiteralOptions property for this object.
*
* @return The value of the LiteralOptions property for this object.
*/
public final LiteralOptions literalOptions() {
return literalOptions;
}
/**
* Returns the value of the TextOptions property for this object.
*
* @return The value of the TextOptions property for this object.
*/
public final TextOptions textOptions() {
return textOptions;
}
/**
* Returns the value of the DateOptions property for this object.
*
* @return The value of the DateOptions property for this object.
*/
public final DateOptions dateOptions() {
return dateOptions;
}
/**
* Returns the value of the LatLonOptions property for this object.
*
* @return The value of the LatLonOptions property for this object.
*/
public final LatLonOptions latLonOptions() {
return latLonOptions;
}
/**
* Returns the value of the IntArrayOptions property for this object.
*
* @return The value of the IntArrayOptions property for this object.
*/
public final IntArrayOptions intArrayOptions() {
return intArrayOptions;
}
/**
* Returns the value of the DoubleArrayOptions property for this object.
*
* @return The value of the DoubleArrayOptions property for this object.
*/
public final DoubleArrayOptions doubleArrayOptions() {
return doubleArrayOptions;
}
/**
* Returns the value of the LiteralArrayOptions property for this object.
*
* @return The value of the LiteralArrayOptions property for this object.
*/
public final LiteralArrayOptions literalArrayOptions() {
return literalArrayOptions;
}
/**
* Returns the value of the TextArrayOptions property for this object.
*
* @return The value of the TextArrayOptions property for this object.
*/
public final TextArrayOptions textArrayOptions() {
return textArrayOptions;
}
/**
* Returns the value of the DateArrayOptions property for this object.
*
* @return The value of the DateArrayOptions property for this object.
*/
public final DateArrayOptions dateArrayOptions() {
return dateArrayOptions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(indexFieldName());
hashCode = 31 * hashCode + Objects.hashCode(indexFieldTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(intOptions());
hashCode = 31 * hashCode + Objects.hashCode(doubleOptions());
hashCode = 31 * hashCode + Objects.hashCode(literalOptions());
hashCode = 31 * hashCode + Objects.hashCode(textOptions());
hashCode = 31 * hashCode + Objects.hashCode(dateOptions());
hashCode = 31 * hashCode + Objects.hashCode(latLonOptions());
hashCode = 31 * hashCode + Objects.hashCode(intArrayOptions());
hashCode = 31 * hashCode + Objects.hashCode(doubleArrayOptions());
hashCode = 31 * hashCode + Objects.hashCode(literalArrayOptions());
hashCode = 31 * hashCode + Objects.hashCode(textArrayOptions());
hashCode = 31 * hashCode + Objects.hashCode(dateArrayOptions());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof IndexField)) {
return false;
}
IndexField other = (IndexField) obj;
return Objects.equals(indexFieldName(), other.indexFieldName())
&& Objects.equals(indexFieldTypeAsString(), other.indexFieldTypeAsString())
&& Objects.equals(intOptions(), other.intOptions()) && Objects.equals(doubleOptions(), other.doubleOptions())
&& Objects.equals(literalOptions(), other.literalOptions()) && Objects.equals(textOptions(), other.textOptions())
&& Objects.equals(dateOptions(), other.dateOptions()) && Objects.equals(latLonOptions(), other.latLonOptions())
&& Objects.equals(intArrayOptions(), other.intArrayOptions())
&& Objects.equals(doubleArrayOptions(), other.doubleArrayOptions())
&& Objects.equals(literalArrayOptions(), other.literalArrayOptions())
&& Objects.equals(textArrayOptions(), other.textArrayOptions())
&& Objects.equals(dateArrayOptions(), other.dateArrayOptions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("IndexField").add("IndexFieldName", indexFieldName())
.add("IndexFieldType", indexFieldTypeAsString()).add("IntOptions", intOptions())
.add("DoubleOptions", doubleOptions()).add("LiteralOptions", literalOptions()).add("TextOptions", textOptions())
.add("DateOptions", dateOptions()).add("LatLonOptions", latLonOptions())
.add("IntArrayOptions", intArrayOptions()).add("DoubleArrayOptions", doubleArrayOptions())
.add("LiteralArrayOptions", literalArrayOptions()).add("TextArrayOptions", textArrayOptions())
.add("DateArrayOptions", dateArrayOptions()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "IndexFieldName":
return Optional.ofNullable(clazz.cast(indexFieldName()));
case "IndexFieldType":
return Optional.ofNullable(clazz.cast(indexFieldTypeAsString()));
case "IntOptions":
return Optional.ofNullable(clazz.cast(intOptions()));
case "DoubleOptions":
return Optional.ofNullable(clazz.cast(doubleOptions()));
case "LiteralOptions":
return Optional.ofNullable(clazz.cast(literalOptions()));
case "TextOptions":
return Optional.ofNullable(clazz.cast(textOptions()));
case "DateOptions":
return Optional.ofNullable(clazz.cast(dateOptions()));
case "LatLonOptions":
return Optional.ofNullable(clazz.cast(latLonOptions()));
case "IntArrayOptions":
return Optional.ofNullable(clazz.cast(intArrayOptions()));
case "DoubleArrayOptions":
return Optional.ofNullable(clazz.cast(doubleArrayOptions()));
case "LiteralArrayOptions":
return Optional.ofNullable(clazz.cast(literalArrayOptions()));
case "TextArrayOptions":
return Optional.ofNullable(clazz.cast(textArrayOptions()));
case "DateArrayOptions":
return Optional.ofNullable(clazz.cast(dateArrayOptions()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function