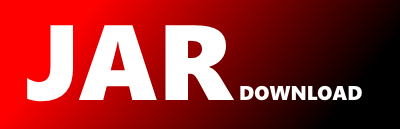
software.amazon.awssdk.services.cloudsearch.model.LiteralOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudsearch Show documentation
Show all versions of cloudsearch Show documentation
The AWS Java SDK for Amazon CloudSearch module holds the client classes that are used for communicating
with Amazon CloudSearch Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cloudsearch.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Options for literal field. Present if IndexFieldType
specifies the field is of type literal
* . All options are enabled by default.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LiteralOptions implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField DEFAULT_VALUE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DefaultValue").getter(getter(LiteralOptions::defaultValue)).setter(setter(Builder::defaultValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DefaultValue").build()).build();
private static final SdkField SOURCE_FIELD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceField").getter(getter(LiteralOptions::sourceField)).setter(setter(Builder::sourceField))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceField").build()).build();
private static final SdkField FACET_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("FacetEnabled").getter(getter(LiteralOptions::facetEnabled)).setter(setter(Builder::facetEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FacetEnabled").build()).build();
private static final SdkField SEARCH_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("SearchEnabled").getter(getter(LiteralOptions::searchEnabled)).setter(setter(Builder::searchEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SearchEnabled").build()).build();
private static final SdkField RETURN_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ReturnEnabled").getter(getter(LiteralOptions::returnEnabled)).setter(setter(Builder::returnEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReturnEnabled").build()).build();
private static final SdkField SORT_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("SortEnabled").getter(getter(LiteralOptions::sortEnabled)).setter(setter(Builder::sortEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SortEnabled").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DEFAULT_VALUE_FIELD,
SOURCE_FIELD_FIELD, FACET_ENABLED_FIELD, SEARCH_ENABLED_FIELD, RETURN_ENABLED_FIELD, SORT_ENABLED_FIELD));
private static final long serialVersionUID = 1L;
private final String defaultValue;
private final String sourceField;
private final Boolean facetEnabled;
private final Boolean searchEnabled;
private final Boolean returnEnabled;
private final Boolean sortEnabled;
private LiteralOptions(BuilderImpl builder) {
this.defaultValue = builder.defaultValue;
this.sourceField = builder.sourceField;
this.facetEnabled = builder.facetEnabled;
this.searchEnabled = builder.searchEnabled;
this.returnEnabled = builder.returnEnabled;
this.sortEnabled = builder.sortEnabled;
}
/**
* A value to use for the field if the field isn't specified for a document.
*
* @return A value to use for the field if the field isn't specified for a document.
*/
public final String defaultValue() {
return defaultValue;
}
/**
* Returns the value of the SourceField property for this object.
*
* @return The value of the SourceField property for this object.
*/
public final String sourceField() {
return sourceField;
}
/**
*
* Whether facet information can be returned for the field.
*
*
* @return Whether facet information can be returned for the field.
*/
public final Boolean facetEnabled() {
return facetEnabled;
}
/**
*
* Whether the contents of the field are searchable.
*
*
* @return Whether the contents of the field are searchable.
*/
public final Boolean searchEnabled() {
return searchEnabled;
}
/**
*
* Whether the contents of the field can be returned in the search results.
*
*
* @return Whether the contents of the field can be returned in the search results.
*/
public final Boolean returnEnabled() {
return returnEnabled;
}
/**
*
* Whether the field can be used to sort the search results.
*
*
* @return Whether the field can be used to sort the search results.
*/
public final Boolean sortEnabled() {
return sortEnabled;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(defaultValue());
hashCode = 31 * hashCode + Objects.hashCode(sourceField());
hashCode = 31 * hashCode + Objects.hashCode(facetEnabled());
hashCode = 31 * hashCode + Objects.hashCode(searchEnabled());
hashCode = 31 * hashCode + Objects.hashCode(returnEnabled());
hashCode = 31 * hashCode + Objects.hashCode(sortEnabled());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LiteralOptions)) {
return false;
}
LiteralOptions other = (LiteralOptions) obj;
return Objects.equals(defaultValue(), other.defaultValue()) && Objects.equals(sourceField(), other.sourceField())
&& Objects.equals(facetEnabled(), other.facetEnabled()) && Objects.equals(searchEnabled(), other.searchEnabled())
&& Objects.equals(returnEnabled(), other.returnEnabled()) && Objects.equals(sortEnabled(), other.sortEnabled());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("LiteralOptions").add("DefaultValue", defaultValue()).add("SourceField", sourceField())
.add("FacetEnabled", facetEnabled()).add("SearchEnabled", searchEnabled()).add("ReturnEnabled", returnEnabled())
.add("SortEnabled", sortEnabled()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DefaultValue":
return Optional.ofNullable(clazz.cast(defaultValue()));
case "SourceField":
return Optional.ofNullable(clazz.cast(sourceField()));
case "FacetEnabled":
return Optional.ofNullable(clazz.cast(facetEnabled()));
case "SearchEnabled":
return Optional.ofNullable(clazz.cast(searchEnabled()));
case "ReturnEnabled":
return Optional.ofNullable(clazz.cast(returnEnabled()));
case "SortEnabled":
return Optional.ofNullable(clazz.cast(sortEnabled()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy